Table of content:
- What Is A List In Python?
- How To Find Length Of List In Python?
- For Loop To Get Python List Length (Naive Approach)
- The len() Function To Get Length Of List In Python
- The length_hint() Function To Find Length Of List In Python
- The sum() Function To Find The Length Of List In Python
- The enumerate() Function To Find Python List Length
- The Counter Class From collections To Find Python List Length
- The List Comprehension To Find Python List Length
- Find The Length Of List In Python Using Recursion
- Comparison Between Ways To Find Python List Length
- Conclusion
- Frequently Asked Questions
Find Length Of List In Python | 8 Ways (+Examples) & Analysis
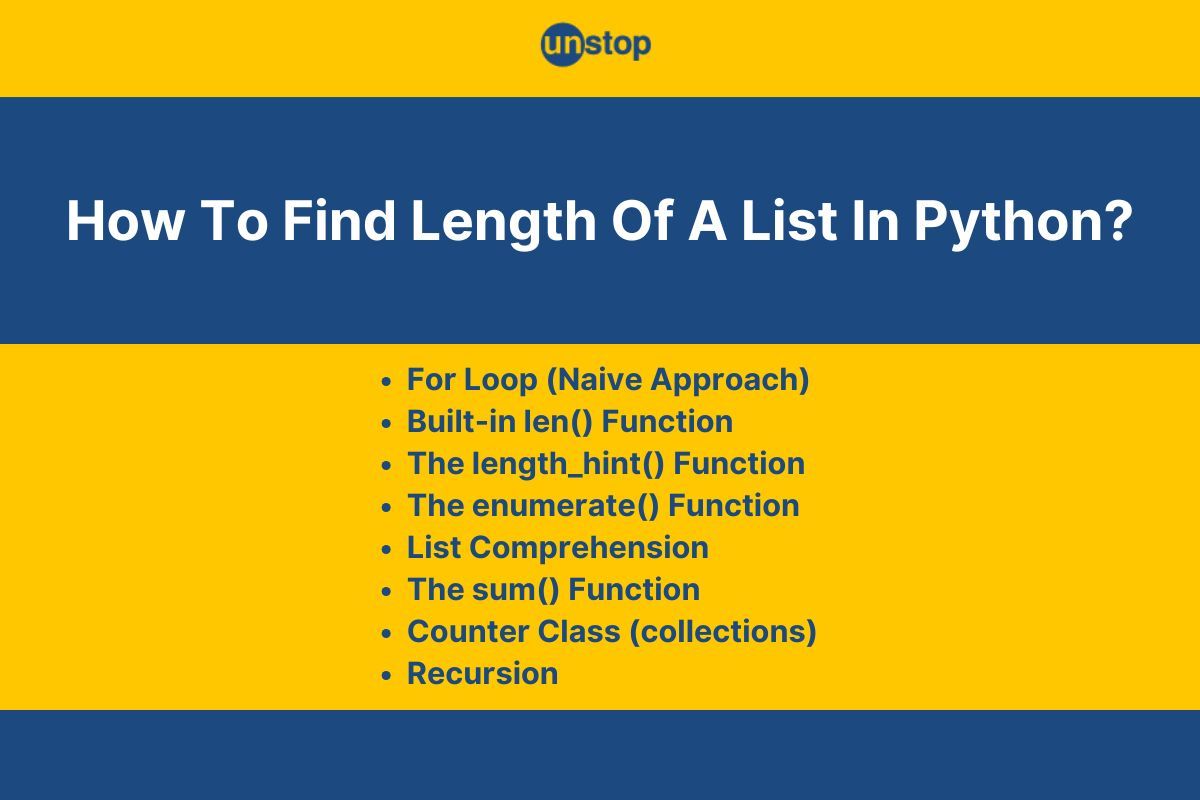
Lists are one of Python’s most versatile data structures, allowing you to store and manage collections of elements with ease. And knowing the Python list length is crucial to working with them effectively. Imagine trying to bake without knowing how many ingredients to use—chaos! Similarly, knowing the Python list length is important, whether you are iterating through, validating or optimizing data.
In this article, we’ll explore all the ways to find the length of a Python list, from the straightforward len() function to alternative approaches with code examples. We will also compare their efficiency to help you choose the best method for your needs.
What Is A List In Python?
A Python list is a versatile data structure used for storing multiple items in a single variable. Think of it as a container that holds anything from numbers to strings and even other lists. The simple Python program example below illustrates how to create a list.
Code Example:
IyBBIHNpbXBsZSBsaXN0IG9mIGludGVnZXJzCm15X2xpc3QgPSBbMSwgMiwgMywgNCwgNV3CoAoKIyBBIG1peGVkIGxpc3QKbWl4ZWRfbGlzdCA9IFsxLCAiSGVsbG8iLCAzLjE0LCBbNywgOF1d
Lists are dynamic, meaning you can add, remove, or modify elements as needed. For an in-depth guide, check out– Python List | Everything You Need To Know (With Detailed Examples)
Why Find Python List Length?
Here are a few scenarios where determining the length of a list in Python programming comes in handy:
- Iteration Control: When looping through a list, knowing its length ensures you can set proper bounds for your loops.
- Input Validation: For user inputs or data validation, checking the length of a list helps verify if the correct number of elements is present.
- Performance Optimization: In some cases, knowing the list size can help you optimize algorithms, especially when working with large datasets.
- Dynamic Operations: Lists are dynamic, and operations like adding, removing, or slicing elements often require knowing the current list size to avoid errors or inefficiencies.
Now that we understand why list length matters let’s explore the different methods you can use to find the Python list length.
How To Find Length Of List In Python?
There are several ways to determine the length of a Python list, each with its advantages depending on the context. These methods include:
- For Loop: A manual approach that counts elements one by one.
- len() Function: A built-in function in Python to get the length.
- length_hint() Function: A lesser-known function primarily used for iterators.
- sum() Function: Using the sum() function in Python to count elements of an iterable.
- enumerate() Function: A unique approach using the iterator protocol.
- Counter from collections: A method from the collections module that can count elements in a list.
- List Comprehension: A Pythonic one-liner using list comprehension.
- Recursion: A more advanced, functional programming approach.
In the following sections, we’ll discuss these methods to find Python list length in depth with code examples and explanations.
For Loop To Get Python List Length (Naive Approach)
A for loop iterates through a range and performs a task iteratively. We can use this loop to count elements in a Python list and find its length. For this, you must manually iterate over each element in the list and increment a counter to keep track of the number of items. The simple Python code example below illustrates this approach to finding the Python list length.
Code Example:
IyBVc2luZyBhIGZvciBsb29wIHRvIGNvdW50IGVsZW1lbnRzIGluIGEgbGlzdApteV9saXN0ID0gWzEwLCAyMCwgMzAsIDQwLCA1MF0KY291bnQgPSAwICNWYXJpYWJsZSB0byBzdG9yZSB0aGUgbGVuZ3RoCgojVXNpbmcgZm9yIGxvb3AgdG8gZmluZCBQeXRob24gbGlzdCBsZW5ndGgKZm9yIGl0ZW0gaW4gbXlfbGlzdDoKICBjb3VudCArPSAxCnByaW50KCJMZW5ndGggb2YgdGhlIGxpc3Q6IiwgY291bnQp
Output:
Length of the list: 5
Explanation:
In this Python code example,
- We first create a list my_list containing integer values. Then, we initialize a variable called count with the value 0 to store the count of elements in the list.
- Next, we use a for loop to iterate through the list, and each time we encounter an element, we increment count by 1.
- After the loop finishes, the value of the count will be the total number of elements in the list.
While this method is clear and works for small lists, it is inefficient for large datasets due to its O(n) time complexity. For large lists, you may want to use more optimized methods like len(), which we will discuss in the next section.
The len() Function To Get Length Of List In Python
The len() function is the go-to method for finding the length of a list in Python. It is fast, efficient, and easy to use, making it the most common approach in Python programming. The reason it’s so effective is that Python internally keeps track of the number of elements in the list, so when you call len(), it can return the length instantly without needing to iterate over the list.
Code Example:
IyBVc2luZyBsZW4oKSB0byBnZXQgdGhlIGxlbmd0aCBvZiBhIGxpc3QKbXlfbGlzdCA9IFsxMCwgMjAsIDMwLCA0MCwgNTBdCgojIENhbGxpbmcgbGVuKCkgdG8gZ2V0IHRoZSBsZW5ndGgKbGVuZ3RoID0gbGVuKG15X2xpc3QpCgojIERpc3BsYXlpbmcgdGhlIHJlc3VsdApwcmludCgiTGVuZ3RoIG9mIHRoZSBsaXN0OiIsIGxlbmd0aCk=
Output:
Length of the list: 5
Code Explanation:
In the basic Python program example,
- We first create a list my_list containing 5 integers: [10, 20, 30, 40, 50].
- Next, we call the len() function, passing my_list as an argument. The function returns the length that we store in the variable length. Python does this without needing to loop through the elements because it tracks the list’s size automatically.
- After that, we use the print() function to display the length to the console.
The len() function is preferred for its simplicity and efficiency. Unlike using a manual for loop, where you count elements one by one, len() is optimized and runs in constant time—O(1). This means that no matter how large the list is, len() will return the length in the same amount of time.
Check out this amazing course to become the best version of the Python programmer you can be.
The length_hint() Function To Find Length Of List In Python
The length_hint() function is part of Python’s operator module and is typically used with iterators to estimate the length of an iterable.
- The function returns a hint of how many elements are remaining in the iterable, which can be helpful in certain scenarios, such as optimizing the performance of algorithms that process large data sets.
- While not specifically designed for lists, it can be used to find the length of lists, especially when working with iterators or other iterable types.
Note that the length_hint() doesn’t actually iterate over the list like a loop does. Instead, it provides an estimate, which means it may not always return the exact length if the list is dynamically changing.
Code Example:
IyBVc2luZyBsZW5ndGhfaGludCgpIHRvIGdldCB0aGUgbGVuZ3RoIG9mIGEgbGlzdApmcm9tIG9wZXJhdG9yIGltcG9ydCBsZW5ndGhfaGludCAjaW1wb3J0aW5nIHRoZSBmdW5jdGlvbgoKVW5zdG9wID0gWyJsZWFybiIsICJ1cHNraWxsIiwgImNvbXBldGUiLCAiZ2V0IGhpcmVkIiwgInByYWN0aWNlIl0gCgojIENhbGxpbmcgbGVuZ3RoX2hpbnQoKSB0byBnZXQgdGhlIGxlbmd0aCBlc3RpbWF0ZQpsZW5ndGggPSBsZW5ndGhfaGludChpdGVyKFVuc3RvcCkpCgojIERpc3BsYXlpbmcgdGhlIHJlc3VsdApwcmludCgiRXN0aW1hdGVkIGxlbmd0aCBvZiB0aGUgbGlzdDoiLCBsZW5ndGgp
Output:
Estimated length of the list: 5
Explanation:
In the example Python program,
- We start by importing length_hint() function from the operator module.
- Then, we create a list Unstop containing five elements of string type.
- Next, we convert the list Unstop into an iterator using the iter() function, as length_hint() works with iterators, not lists directly.
- We then call the length_hint() function on the iterator, i.e., length_hint(iter(Unstop)).
- The function returns the estimated length of the iterator, which is 5.
- Finally, we print the estimated length to the console.
Although length_hint() can provide a quick estimate of the length, its time complexity is O(1) for lists, meaning it doesn’t need to traverse the elements. However, this method is not as widely used or preferred to find the length of a list in Python compared to len().
The sum() Function To Find The Length Of List In Python
The sum() function is generally used to add numbers in an iterable, but it can also be used to get the length of a list in Python.
- In this approach, you must first generate a list of 1s (one for each element in the original list) and use sum() to add the 1s and effectively return the total count of elements in the list.
- This method provides an interesting way to use Python's built-in functions in combination for tasks beyond their traditional use.
Code Example:
IyBVc2luZyBzdW0oKSB0byBnZXQgdGhlIGxlbmd0aCBvZiBhIGxpc3QKVW5zdG9wID0gWyJsZWFybiIsICJ1cHNraWxsIiwgImNvbXBldGUiLCAiZ2V0IGhpcmVkIiwgInByYWN0aWNlIl0KCiMgVXNpbmcgc3VtKCkgdG8gY291bnQgZWxlbWVudHMgYnkgYWRkaW5nIDEgZm9yIGVhY2gKbGVuZ3RoID0gc3VtKDEgZm9yIF8gaW4gVW5zdG9wKQoKIyBEaXNwbGF5aW5nIHRoZSByZXN1bHQKcHJpbnQoIkxlbmd0aCBvZiB0aGUgbGlzdDoiLCBsZW5ndGgp
Output:
Length of the list: 5
Explanation:
In the example Python code,
- First, we create the Unstop list containing 5 string elements.
- Next, we use a generator expression inside the sum() function to count the number of elements and get the length of the list.
- This works by iterating through each element in Unstop and returning 1 for each iteration.
- The sum() function then adds these 1s together to calculate the total length of the list.
- Finally, we print the calculated length to the console.
The use of the sum() function to find the Python list length has an O(n) time complexity due to the iteration through all the elements and the additional operation for each element. In comparison, the len() function remains a faster and more reliable choice for finding Python list length.
The enumerate() Function To Find Python List Length
The enumerate() function in Python is typically used to iterate over a list while keeping track of the index of each element.
- In other words, it adds a counter to an iterable, returning both the index and the value of each element.
- While it is most commonly used for retrieving index-value pairs, it can also be adapted to find the length of a list in Python by iterating over the elements and counting them.
This method demonstrates the flexibility of enumerate(), allowing you to leverage it for more than just indexing.
Code Example:
IyBVc2luZyBlbnVtZXJhdGUoKSB0byBnZXQgdGhlIGxlbmd0aCBvZiBhIGxpc3QKVW5zdG9wID0gWyJsZWFybiIsICJ1cHNraWxsIiwgImNvbXBldGUiLCAiZ2V0IGhpcmVkIiwgInByYWN0aWNlIl0KCiMgVXNpbmcgZW51bWVyYXRlKCkgdG8gY291bnQgZWxlbWVudHMKbGVuZ3RoID0gc3VtKDEgZm9yIF8sIF8gaW4gZW51bWVyYXRlKFVuc3RvcCkpCgojIERpc3BsYXlpbmcgdGhlIHJlc3VsdApwcmludCgiTGVuZ3RoIG9mIHRoZSBsaXN0OiIsIGxlbmd0aCk=
Output:
Length of the list: 5
Explanation:
In the Python program example,
- We start by creating a list Unstop containing the strings "learn", "upskill", "compete", "get hired", and "practice".
- Next, we call the enumerate() function on the list Unstop, to iterate over it.
- The enumerate() function returns each element's index and value, but in this case, we’re only interested in the values, so we discard the index by using _.
- The generator expression sum(1 for _, _ in enumerate(Unstop)) iterates over all elements in the list and sums 1 for each element, effectively counting the number of items.
- We store the outcome in the variable length and then print it to the console.
While this method works, it’s not the most efficient. It still requires iteration through the list, resulting in an O(n) time complexity.
The Counter Class From collections To Find Python List Length
The Counter class from Python’s collections module is a powerful tool for data analysis and is typically used to count the frequency of elements in an iterable. Even though the function is designed to count the occurrences of items, you can use it to determine the length of a list in Python programs. For this, you must create a Counter object from the list, and then derive the length by simply looking at the number of unique elements.
While not the most efficient and commonly used method for finding Python list length, it showcases the versatility of the Counter class.
Code Example:
IyBVc2luZyBDb3VudGVyIHRvIGdldCB0aGUgbGVuZ3RoIG9mIGEgbGlzdApmcm9tIGNvbGxlY3Rpb25zIGltcG9ydCBDb3VudGVyCgpVbnN0b3AgPSBbImxlYXJuIiwgInVwc2tpbGwiLCAiY29tcGV0ZSIsICJnZXQgaGlyZWQiLCAicHJhY3RpY2UiXQoKIyBVc2luZyBDb3VudGVyIHRvIGNvdW50IGVsZW1lbnRzCmNvdW50ZXIgPSBDb3VudGVyKFVuc3RvcCkKbGVuZ3RoID0gc3VtKGNvdW50ZXIudmFsdWVzKCkpCgojIERpc3BsYXlpbmcgdGhlIHJlc3VsdApwcmludCgiTGVuZ3RoIG9mIHRoZSBsaXN0OiIsIGxlbmd0aCk=
Output:
Length of the list: 5
Explanation:
In the sample Python program,
- We begin by importing the Counter class from the collections module.
- Then, we create a list Unstop containing the strings "learn", "upskill", "compete", "get hired", and "practice".
- Next, we pass this list to the Counter() constructor, which creates a dictionary-like object where the keys are the elements of the list and the values are their frequencies.
- We then use sum(counter.values()) to sum up all the values in the Counter object, which essentially counts the total number of elements in the list.
- Finally, we print the length to the console.
The use of Counter class to find Python list length has a time complexity of O(n) due to the iteration through the list to build the counter. This shows that while the Counter class is quite powerful for counting element frequencies, using it just to find the length of a list can be overkill and inefficient.
Level up your coding skills with the 100-Day Coding Sprint at Unstop and get the bragging rights, now!
The List Comprehension To Find Python List Length
List comprehension is a concise and efficient way to generate lists in Python, and it can also be leveraged to find the length of a list.
- The idea is to create a temporary list based on the given list (typically by iterating over it) and then use sum() to count the number of elements.
- This approach combines the readability and expressiveness of list comprehension with the power of built-in functions, though it’s not the most optimized in terms of performance.
It is an interesting alternative method that showcases how list comprehension can be creatively applied beyond its typical use case, offering a more flexible approach to the task.
Code Example:
IyBVc2luZyBsaXN0IGNvbXByZWhlbnNpb24gdG8gZ2V0IHRoZSBsZW5ndGggb2YgYSBsaXN0ClVuc3RvcCA9IFsibGVhcm4iLCAidXBza2lsbCIsICJjb21wZXRlIiwgImdldCBoaXJlZCIsICJwcmFjdGljZSJdCgojIFVzaW5nIGxpc3QgY29tcHJlaGVuc2lvbiB0byBjb3VudCBlbGVtZW50cwpsZW5ndGggPSBzdW0oWzEgZm9yIF8gaW4gVW5zdG9wXSkKCiMgRGlzcGxheWluZyB0aGUgcmVzdWx0CnByaW50KCJMZW5ndGggb2YgdGhlIGxpc3Q6IiwgbGVuZ3RoKQ==
Output:
Length of the list: 5
Explanation:
In the sample Python code,
- We begin by creating the Unstop list, which contains the strings "learn", "upskill", "compete", "get hired", and "practice".
- Next, we use list comprehension to iterate over each element in the list.
- The list comprehension generates a 1 for every element, effectively creating a new list where each element is 1.
- We then pass this list to the sum() function, which adds up all the 1s, giving us the length of the original list.
- Finally, we print the result.
This method is an elegant and concise way of finding the length of a list in Python. However, it is not the most efficient way because it requires creating a new list and performing an additional summation operation. Both these contribute to a time complexity of O(n).
Find The Length Of List In Python Using Recursion
Recursion is a programming technique where a problem is broken down into smaller, similar sub-problems that can be solved iteratively. In this case, we define a function that calls itself, reducing the problem size each time until we reach the base case. Similarly, you can use recursion to find the length of a list in Python by iterating through each element and reducing the list size with each call.
Although this method serves more as a programming exercise than a practical solution for large lists, it effectively demonstrates the concept of recursion and can still be used to determine the list length.
Code Example:
IyBVc2luZyByZWN1cnNpb24gdG8gZ2V0IHRoZSBsZW5ndGggb2YgYSBsaXN0CmRlZiBnZXRfbGVuZ3RoKGxzdCk6CiAgIyBCYXNlIGNhc2U6IGlmIHRoZSBsaXN0IGlzIGVtcHR5LCByZXR1cm4gMAogIGlmIG5vdCBsc3Q6CiAgICByZXR1cm4gMAogICMgUmVjdXJzaXZlIGNhc2U6IHJlbW92ZSB0aGUgZmlyc3QgZWxlbWVudCBhbmQgY291bnQgdGhlIHJlc3QKICByZXR1cm4gMSArIGdldF9sZW5ndGgobHN0WzE6XSkKCiMgVGVzdGluZyB0aGUgZnVuY3Rpb24KVW5zdG9wID0gWyJsZWFybiIsICJ1cHNraWxsIiwgImNvbXBldGUiLCAiZ2V0IGhpcmVkIiwgInByYWN0aWNlIl0KbGVuZ3RoID0gZ2V0X2xlbmd0aChVbnN0b3ApCgojIERpc3BsYXlpbmcgdGhlIHJlc3VsdApwcmludCgiTGVuZ3RoIG9mIHRoZSBsaXN0OiIsIGxlbmd0aCk=
Output:
Length of the list: 5
Explanation:
In the Python code sample,
- We use the recursive approach by defining a function get_length(), which accepts a list as a parameter.
- The function first checks if the list is empty, which serves as the base case. If the list is empty, the function returns 0, indicating no elements left to count.
- If the list is not empty, the function calls itself with the rest of the list (lst[1:]), effectively reducing the size of the problem by removing the first element.
- Each recursive call adds 1 to the count, and when the base case is reached, it returns the total length.
This method is an interesting demonstration of recursion, but it’s not the most efficient method to find the length of a list in Python. This is because it has a time complexity of O(n), and the overhead of function calls and slicing the list makes it less optimal than iterative methods.
Comparison Between Ways To Find Python List Length
Now that we’ve explored various methods for finding the length of a Python list let's compare their performance and efficiency by looking at their time and space complexities. In this section, we’ll break down the time complexities of each method and provide a practical example using the timeit module to measure execution time.
The table below lists the time and space complexities of various ways to find the length of a list in Python, along with remarks.
Method |
Time Complexity |
Space Complexity |
Remarks |
len() |
O(1) |
O(1) |
It is the fastest and most efficient method as it directly retrieves the size and is optimized internally by the Python language. |
For Loop |
O(n) |
O(1) |
It iterates through each element, making it slower for larger lists. |
length_hint() |
O(1) |
O(1) |
This function is optimized for iterators and works in constant time for lists; it is efficient but limited to iterators. |
List Comprehension + sum() |
O(n) |
O(n) |
Involves creating an additional list, which increases memory usage and decreases efficiency compared to len(). |
enumerate() |
O(n) |
O(1) |
It is useful for generating index-value pairs but slower in comparison to len() to find the length of the list in Python. |
Counter from collections |
O(n) |
O(n) |
Using counter just to find the length of list in Python might be inefficient (and overkill) as it involves extra overhead. Also, it requires extra memory for dictionary storage. |
Recursion |
O(n) |
O(n) |
The numerous function calls and list slicing lead to significant memory overhead and make this approach inefficient. |
As is evident, while some methods are more intuitive or creative, others are optimized for speed. Next, we’ll use the timeit module to measure the execution time of each method for a list of varying sizes. This will provide us with a better understanding of how each approach performs in a real-world scenario.
Code Example:
aW1wb3J0IHRpbWVpdApmcm9tIGNvbGxlY3Rpb25zIGltcG9ydCBDb3VudGVyCmZyb20gb3BlcmF0b3IgaW1wb3J0IGxlbmd0aF9oaW50CgojIERlZmluZSB0aGUgbWV0aG9kcyBmb3IgZmluZGluZyB0aGUgbGVuZ3RoIG9mIGEgbGlzdAoKIyBNZXRob2QgMTogbGVuKCkKZGVmIGxlbl9tZXRob2QobXlfbGlzdCk6CiAgICByZXR1cm4gbGVuKG15X2xpc3QpCgojIE1ldGhvZCAyOiBGb3IgTG9vcApkZWYgZm9yX2xvb3BfbWV0aG9kKG15X2xpc3QpOgogICAgY291bnQgPSAwCiAgICBmb3IgaXRlbSBpbiBteV9saXN0OgogICAgICAgIGNvdW50ICs9IDEKICAgIHJldHVybiBjb3VudAoKIyBNZXRob2QgMzogbGVuZ3RoX2hpbnQoKQpkZWYgbGVuZ3RoX2hpbnRfbWV0aG9kKG15X2xpc3QpOgogICAgaXRlcl9saXN0ID0gaXRlcihteV9saXN0KQogICAgcmV0dXJuIGxlbmd0aF9oaW50KGl0ZXJfbGlzdCkKCiMgTWV0aG9kIDQ6IExpc3QgY29tcHJlaGVuc2lvbiArIHN1bSgpCmRlZiBzdW1fbWV0aG9kKG15X2xpc3QpOgogICAgcmV0dXJuIHN1bSgxIGZvciBfIGluIG15X2xpc3QpCgojIE1ldGhvZCA1OiBlbnVtZXJhdGUoKQpkZWYgZW51bWVyYXRlX21ldGhvZChteV9saXN0KToKICAgIGNvdW50ID0gMAogICAgZm9yIF8sIF8gaW4gZW51bWVyYXRlKG15X2xpc3QpOgogICAgICAgIGNvdW50ICs9IDEKICAgIHJldHVybiBjb3VudAoKIyBNZXRob2QgNjogQ291bnRlciBmcm9tIGNvbGxlY3Rpb25zCmRlZiBjb3VudGVyX21ldGhvZChteV9saXN0KToKICAgIHJldHVybiBsZW4oQ291bnRlcihteV9saXN0KSkKCiMgTWV0aG9kIDc6IFJlY3Vyc2lvbgpkZWYgcmVjdXJzaW9uX21ldGhvZChteV9saXN0KToKICAgIGlmIG5vdCBteV9saXN0OgogICAgICAgIHJldHVybiAwCiAgICBlbHNlOgogICAgICAgIHJldHVybiAxICsgcmVjdXJzaW9uX21ldGhvZChteV9saXN0WzE6XSkKCiMgQ3JlYXRlIGEgc2FtcGxlIGxpc3QKbXlfbGlzdCA9IFsibGVhcm4iLCAidXBza2lsbCIsICJjb21wZXRlIiwgImdldCBoaXJlZCIsICJwcmFjdGljZSJdCgojIFRpbWluZyBlYWNoIG1ldGhvZCB3aXRoIHRpbWVpdAptZXRob2RzID0gewogICAgImxlbigpIjogbGVuX21ldGhvZCwKICAgICJGb3IgTG9vcCI6IGZvcl9sb29wX21ldGhvZCwKICAgICJsZW5ndGhfaGludCgpIjogbGVuZ3RoX2hpbnRfbWV0aG9kLAogICAgIkxpc3QgY29tcHJlaGVuc2lvbiArIHN1bSgpIjogc3VtX21ldGhvZCwKICAgICJlbnVtZXJhdGUoKSI6IGVudW1lcmF0ZV9tZXRob2QsCiAgICAiQ291bnRlciBmcm9tIGNvbGxlY3Rpb25zIjogY291bnRlcl9tZXRob2QsCiAgICAiUmVjdXJzaW9uIjogcmVjdXJzaW9uX21ldGhvZCwKfQoKIyBUaW1pbmcgZXhlY3V0aW9uIGZvciBlYWNoIG1ldGhvZAp0aW1pbmdfcmVzdWx0cyA9IHt9CmZvciBtZXRob2RfbmFtZSwgbWV0aG9kIGluIG1ldGhvZHMuaXRlbXMoKToKICAgIHRpbWVfdGFrZW4gPSB0aW1laXQudGltZWl0KGxhbWJkYTogbWV0aG9kKG15X2xpc3QpLCBudW1iZXI9MTAwMDAwKQogICAgdGltaW5nX3Jlc3VsdHNbbWV0aG9kX25hbWVdID0gdGltZV90YWtlbgoKIyBEaXNwbGF5IHRoZSByZXN1bHRzCnByaW50KGYiVGltaW5nIFJlc3VsdHMgZm9yIEVhY2ggTWV0aG9kIChleGVjdXRlZCAxMDAsMDAwIHRpbWVzKToiKQpmb3IgbWV0aG9kX25hbWUsIHRpbWVfdGFrZW4gaW4gdGltaW5nX3Jlc3VsdHMuaXRlbXMoKToKICAgIHByaW50KGYie21ldGhvZF9uYW1lfToge3RpbWVfdGFrZW46LjEwZn0gc2Vjb25kcyIp
Output:
Timing Results for Each Method (executed 100,000 times):
len() : 0.003985 seconds
For Loop : 0.018656 seconds
length_hint() : 0.006000 seconds
List Comprehension + sum() : 0.033507 seconds
enumerate() : 0.048228 seconds
Counter : 0.133247 seconds
Recursion : 0.062270 seconds
Explanation:
In the Python example code,
- We start by importing the timeit module to measure the execution time of each method, Counter from collections for counting elements in a list, and length_hint from operator for estimating the length of iterators.
- Next, we define seven different methods for calculating the length of a list. Each method is implemented as a function:
- len_method(): The function uses Python's built-in len() function to return the length.
- for_loop_method(): Loops through each item in the list, incrementing a counter.
- length_hint_method(): Uses length_hint() on an iterator created from the list.
- sum_method(): Uses a generator expression within sum() to count the elements.
- enumerate_method(): Uses enumerate() to iterate through the list and count elements.
- counter_method(): Uses Counter to count occurrences of elements in the list and returns its length.
- recursion_method(): Using an if-else statement, we define a base case where function returns zero if list is empty, else it calculates the length by reducing the list size one at a time.
- Then, we create a list my_list containing five string elements: "learn", "upskill", "compete", "get hired", "practice".
- We then use a dictionary called methods to map each method name to its corresponding function.
- After that, we use the timeit.timeit() function to measure the execution time of each method 100,000 times. For each method, we run timeit() 100,000 times to collect accurate data on how long each method takes to execute.
- The results of each method are stored in the timing_results dictionary.
- After executing all the methods, we print the execution time for each method.
The output provides a clear comparison of how long each approach takes when executed 100,000 times, offering insight into the efficiency of the methods.
Key Takeaways:
- Performance: As demonstrated, len() is by far the fastest and most efficient method, operating in constant time, O(1), due to Python’s internal tracking of list size.
- For Loop: While more intuitive, it has a linear time complexity O(n), which makes it slower than len() for larger lists.
- length_hint(): This method works similarly to len() in terms of time complexity, O(1), but is limited to iterators and not general lists.
- List Comprehension + sum(): Although functional and elegant, it creates an extra generator object and is less memory-efficient than len(), making it slower.
- Enumerate(): Iterating over the list using enumerate() is useful when you need both the index and value, but it introduces additional overhead and is slower than len() to get just the length of a list in Python.
- Counter from collections: Using Counter to find the length is an overkill for this specific task, as it creates a dictionary of element counts, adding unnecessary memory overhead.
- Recursion: This method is primarily useful as a programming exercise to understand recursion. It has both time and space complexity of O(n), making it less efficient for large lists.
Looking for guidance? Find the perfect mentor from select experienced coding & software development experts here.
Conclusion
Knowing the length of a Python list is fundamental for many tasks, from iterating through data to optimizing performance. In this article, we’ve explored various methods to determine the Python list length, ranging from the standard and efficient len() function to more unconventional approaches like recursion and Counter().
- The len() function stands out as the fastest and most efficient, offering constant time complexity (O(1)), making it ideal for most scenarios.
- While other methods like for loops, sum(), and enumerate() are functional, they tend to be less efficient and involve additional processing steps that increase execution time.
- Advanced methods like length_hint() and recursion, though interesting, are not typically used for this task due to their performance drawbacks. Their performance can vary depending on the situation, but they generally don’t outperform len() for standard list operations.
A comparison of the time and space complexities of each method helps highlight the importance of choosing the right approach based on your use case. In most cases, the len() function will be the best bet for finding the length of a list in Python programs.
Frequently Asked Questions
Q1. Is the len() function specific to lists?
No, the len() function is not specific to Python lists. It can be used to find the length of other iterable objects, such as tuples, strings, dictionaries, and sets. It is important to note that Python internally tracks the size of these data structures so that len() can quickly return their size.
Q2. Why is len() preferred over other methods to find Python list length?
The len() function is the most efficient method for finding the length of a list in Python because it operates in constant time, O(1). Python tracks the list size internally, so calling len() does not require iterating through the elements, making it faster than other methods like using a for loop or recursion.
Q3. What is the time complexity of the methods to find Python list length?
The time complexity of the various ways to find the length of a list in Python language are as follows:
- len(): O(1) — Constant time.
- for loop: O(n) — Linear time, as it iterates over each element.
- sum() with list comprehension: O(n) — Requires iterating through all elements.
- enumerate(): O(n) — Iterates through the list, but adds the overhead of generating index-value pairs.
- Counter(): O(n) — Counts occurrences of elements, iterating through the list.
- Recursion: O(n) — Each recursive call processes one element, leading to a linear time complexity.
Q4. How can I improve the performance of my program when calculating the length of a list in Python?
To optimize performance, always use the len() function, as it is the fastest and most efficient way to get the length of a Python list. Avoid manual counting with loops or recursion unless necessary for a specific task.
Q5. When should I use alternative methods to find the length of a Python list?
Alternative methods like recursion, Counter(), or sum() are generally not as efficient as len(). However, they may be useful in specialized scenarios, such as when working with iterators, processing data while counting, or incorporating these methods into a larger algorithm that involves additional logic beyond just finding the length of a list in Python.
You might also be interested in reading the following:
- Python Program To Convert Kilometers To Miles (+Detailed Examples)
- Python While Loop | Types With Control Statements (Code Examples)
- Python Break Statement Explained (With All Loops + Code Examples)
- Python String.Replace() And 8 Other Ways Explained (+Examples)
- Python Assert Keyword | Types, Uses, Best Practices (+Code Examples)
- Fibonacci Series In Python & Nth Term | Generate & Print (+Codes)
An economics graduate with a passion for storytelling, I thrive on crafting content that blends creativity with technical insight. At Unstop, I create in-depth, SEO-driven content that simplifies complex tech topics and covers a wide array of subjects, all designed to inform, engage, and inspire our readers. My goal is to empower others to truly #BeUnstoppable through content that resonates. When I’m not writing, you’ll find me immersed in art, food, or lost in a good book—constantly drawing inspiration from the world around me.
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
Comments
Add comment