Table of content:
- What Is List Comprehension In Python?
- Incorporating Conditional Statements With List Comprehension In Python
- List Comprehension In Python With range()
- Filtering Lists Effectively With List Comprehension In Python
- Nested Loops With List Comprehension In Python
- Flattening Nested Lists With List Comprehension In Python
- Handling Exceptions In List Comprehension In Python
- Common Use Cases For List Comprehensions
- Advantages & Disadvantages Of List Comprehension In Python
- Best Practices For Using List Comprehension In Python
- Performance Considerations For List Comprehension In Python
- For Loops & List Comprehension In Python: A Comparison
- Difference Between Generator Expression & List Comprehension In Python
- Conclusion
- Frequently Asked Questions
List Comprehension In Python | A Complete Guide With Example Codes
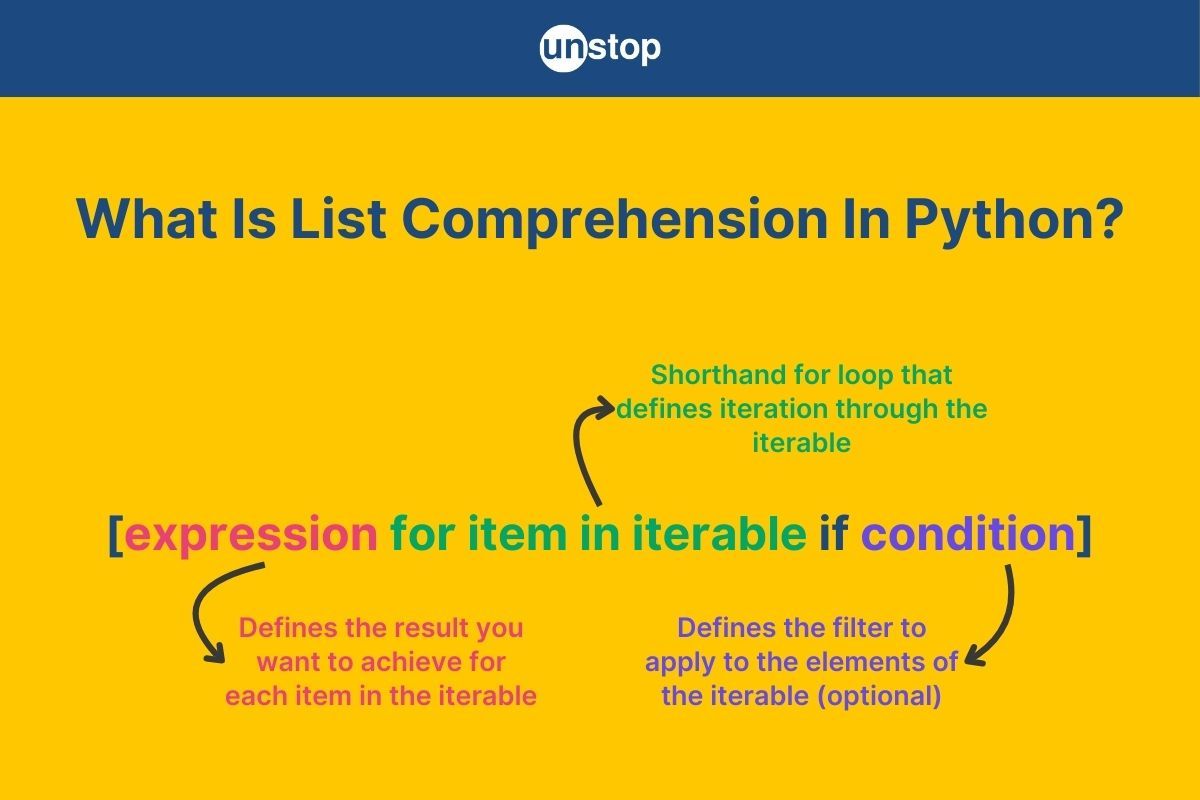
List comprehensions in Python are like shortcuts that allow you to create and manipulate lists in a more concise, readable, and elegant way. Instead of using cumbersome loops to generate or transform lists, you can do it in one clean, powerful line of code. This article will explore everything you need to know about list comprehensions—from their basic syntax to practical use cases and advanced techniques. Whether you're a beginner or a seasoned Pythonista, understanding list comprehensions will level up your coding game!
What Is List Comprehension In Python?
List comprehension is a compact way to create lists in Python and manipulate/modify them. It's a single line of code that replaces the need for a traditional loop when generating or transforming lists.
- It combines the logic of loops with the elegance of Python’s functional programming features, making it a go-to for efficient list creation and transformation.
- In simpler terms, list comprehension allows you to write a loop and an expression in one line, making your code more compact and often easier to read.
Syntax Of List Comprehension In Python
The syntax of list comprehension might look compact, but it's easy to understand once broken down into its key components. The basic syntax follows this structure:
[expression for item in iterable if condition]
Components Of List Comprehension In Python
Understanding the components of list comprehension in Python programming is essential to mastering its syntax and using it effectively.
- Expression: This is the result you want to achieve for each item in the iterable. It can be anything from a simple value, a mathematical operation, or even a function call.
Example: x**2 where x is the element from the iterable. - For Item in Iterable: This part iterates through the iterable (like a list, range, or string) and can be considered a shorthand form of the for loop. For each iteration, the item takes the value of the current element from the iterable.
Example: for x in range(5) iterates through the numbers 0 to 4. - Condition (optional): This is a filter to apply to the elements of the iterable (a shorthand form of if-else/conditional statements]. If the condition evaluates to True, the expression is included in the resulting list. If the condition is omitted, all elements from the iterable are included.
Example: if x % 2 == 0 includes only even numbers.
The basic Python program example below illustrates how we can use list comprehension to modify a list of string values, given a certain condition is fulfilled.
Code Example:
I09yaWdpbmFsIGxpc3Qgb2Ygc3RyaW5ncwpVbnN0b3AgPSBbInVwc2tpbGwiLCAibGVhcm4iLCAibWVudG9ycyIsICJwcmFjdGljZSJdCgojIEdldCB0aGUgdXBwZXJjYXNlIHZlcnNpb24gb2Ygc3RyaW5ncyB0aGF0IGNvbnRhaW4gdGhlIGxldHRlciAnZScKcmVzdWx0ID0gW3dvcmQudXBwZXIoKSBmb3Igd29yZCBpbiBVbnN0b3AgaWYgJ2UnIGluIHdvcmRdCgpwcmludChyZXN1bHQp
Output:
['LEARN', 'MENTORS', 'PRACTICE']
Code Explanation:
In the basic Python code example:
- We begin by creating a list called Unstop, containing four string values, of which three contain the letter ‘e’.
- Then, we use list comprehension to modify the list. Inside, we have the expression where we use upper() on the list to convert the string values from lower to uppercase.
- Following this, we have the for item part which stipulates that the function be applied to a word inside the Unstop list.
- Further, we add a condition that stipulates that the expression be applied only for words that have the letter ‘e’ in them.
- As shown in the output, the single line list comprehension carries out the modification seamlessly.
Note: We have used the Python built-in function upper() which is commonly used for string manipulation/ modification.
Incorporating Conditional Statements With List Comprehension In Python
One of the most powerful features of list comprehension is the ability to incorporate conditional statements. This allows you to filter elements or apply different transformations based on specific conditions, all within a single line of code.
How It Works: Conditional statements in list comprehensions can be:
- Filters: Used to include elements that meet certain criteria.
- Conditional Expressions: Used to apply different transformations based on conditions.
Using Filters In List Comprehension
Filters allow you to include only the elements that satisfy a condition. For example, you want to extract only even numbers from a list of numbers. You can use the modulus operator to check if the remainder of the division by 2 is zero and use this condition to filter even numbers.
Code Example:
I09yaWdpbmFsIGxpc3Qgb2YgbnVtYmVycwpudW1iZXJzID0gWzEsIDIsIDMsIDQsIDUsIDZdCgojVXNpbmcgbGlzdCBjb21wcmVoZW5zaW9uIHRvIGZpbHRlciBldmVuIG51bWJlcnMKZXZlbl9udW1iZXJzID0gW251bSBmb3IgbnVtIGluIG51bWJlcnMgaWYgbnVtICUgMiA9PSAwXQoKcHJpbnQoZXZlbl9udW1iZXJzKQ==
Output:
[2, 4, 6]
Code Explanation:
In the simple Python program example:
- We have an original list called numbers containing 6 values.
- Then, we use list comprehension with the expression num, iterable the numbers list and the condition if num % 2 == 0, to filter out odd numbers.
- This filters out the odd numbers and creates a new list containing only even numbers.
- We use the print() function to display the new list to the console.
Using Conditional Expressions In List Comprehension
Conditional expressions allow you to transform elements differently based on conditions. For example, say you want to replace the even numbers in a list of numbers with the word "Even" and odd numbers with "Odd". The example below illustrates how you can do this using list comprehension in Python programs.
Code Example:
I0xpc3Qgb2YgbnVtYmVycwpudW1iZXJzID0gWzEsIDIsIDMsIDQsIDUsIDZdCgojQ3JlYXRpbmcgYSBuZXcgbGlzdCB1c2luZyBsaXN0IGNvbXByZWhlbnNpb24KbGFiZWxzID0gWyJFdmVuIiBpZiBudW0gJSAyID09IDAgZWxzZSAiT2RkIiBmb3IgbnVtIGluIG51bWJlcnNdCgpwcmludChsYWJlbHMp
Output:
['Odd', 'Even', 'Odd', 'Even', 'Odd', 'Even']
Code Explanation:
In the simple Python code example:
- We begin with the same list as before and use list comprehension to modify it.
- Here, we use the expression "Even" if num % 2 == 0 else, "Odd".
- The iterable is the numbers list and each item/ number is checked against the condition, and the corresponding label is applied based on the result.
- The new list contains labels instead of numbers and we print it to the console.
Combining Multiple Conditions In List Comprehension In Python
You can also combine multiple conditions in a list comprehension for more complex filtering. For example, say you want to extract numbers divisible by both 2 and 3. The Python program example below illustrates how to use list comprehension and conditional conditions to get this done.
Code Example:
I09yaWdpbmFsIGxpc3QKbnVtYmVycyA9IGxpc3QocmFuZ2UoMSwgMjEpKQoKI1VzaW5nIGxpc3QgY29tcHJlaGVuc2lvbiB0byBmaWx0ZXIgaXRlbXMgd2l0aCB0d28gY29uZGl0aW9ucwpkaXZpc2libGVfYnlfMl9hbmRfMyA9IFtudW0gZm9yIG51bSBpbiBudW1iZXJzIGlmIG51bSAlIDIgPT0gMCBhbmQgbnVtICUgMyA9PSAwXQoKcHJpbnQoZGl2aXNpYmxlX2J5XzJfYW5kXzMp
Output:
[6, 12, 18]
Code Explanation:
In the Python code example:
- We have the original numbers list and use list comprehension with two conditions.
- Here, we have the expression num, the iterable is the list range(1, 21).
- We have two conditions connected with the logical and operator, i.e., if num % 2 == 0 and num % 3 == 0, ensuring only numbers divisible by both 2 and 3 are included.
Incorporating conditional statements makes list comprehensions incredibly versatile. They allow you to write cleaner and more efficient code for filtering, transforming, or processing lists.
List Comprehension In Python With range()
The range() function in Python is one of the most commonly used iterables with list comprehensions. It allows you to generate sequences of numbers efficiently, which can then be transformed or filtered within the comprehension.
How It Works: Using range() in a list comprehension is straightforward. You specify the start, stop, and step values for the sequence, and the comprehension processes each number in the range. Let’s look at a few examples illustrating how we can use the range() function with list comprehension.
Example 1: Creating List Of Squares
You can use list comprehension to generate a list of squares for numbers from 0 to 9.
Code Example:
I1VzaW5nIGxpc3QgY29tcHJlaGVuc2lvbiB0byBjcmVhdGUgYSBsaXN0CnNxdWFyZXMgPSBbeCoqMiBmb3IgeCBpbiByYW5nZSgxMCldCgpwcmludChzcXVhcmVzKQ==
Output:
[0, 1, 4, 9, 16, 25, 36, 49, 64, 81]
Code Explanation:
In the example Python program:
- We use list comprehension with the expression x**2, which calculates the square of each number.
- The iterable range(10) generates numbers from 0 to 9.
- The list created contains the squares of numbers between 0 and 9, which we print to the console.
Example 2: Skipping Numbers With A Step Value
You can use the range() function with more parameters to not just generate a list in a certain range but also add another condition with the set parameter. The example below illustrates how to generate a list of numbers from 0 to 20, skipping every second number.
Code Example:
I1VzaW5nIHJhbmdlKCkgaW4gbGlzdCBjb21wcmVoZW5zaW9uIHdpdGggc3RlcCBvZiAyCm51bWJlcnMgPSBbeCBmb3IgeCBpbiByYW5nZSgwLCAyMSwgMildCgpwcmludChudW1iZXJzKQ==
Output:
[0, 2, 4, 6, 8, 10, 12, 14, 16, 18, 20]
Code Explanation:
In the example Python code:
- The iterable range(0, 21, 2) generates numbers from 0 to 20 with a step value of 2.
- This step argument means we skip one number and move to the 2nd one, beginning from zero and stopping at 21.
- The expression is simply x, as no transformation is applied.
We can use the range() function to filter out numbers based on a variety of conditions. List comprehension with range() is an efficient way to generate and manipulate numerical lists. It’s especially handy for tasks like creating sequences, applying transformations, and filtering numbers.
Filtering Lists Effectively With List Comprehension In Python
Filtering lists is one of the most popular use cases for list comprehensions. We have already discussed how to filter odd numbers and use range() with comprehension to filter lists. There are more ways we can filter a list as well as nested lists in Python, using list comprehension.
For example, we can add varied conditions to extract only the elements that meet specific criteria, making data processing more efficient and concise. Below are some examples for the same.
Example 1: Filtering Positive Numbers
In the example below, we have illustrated how to use list comprehension to filter out positive numbers from a pre-existing list of numbers.
Code Example:
I0xpc3Qgd2l0aCBwb3NpdGl2ZSBhbmQgbmVnYXRpdmUgbnVtYmVycwpudW1iZXJzID0gWy0xMCwgLTUsIDAsIDUsIDEwXQoKI0xpc3QgY29tcHJlaGVuc2lvbiB0byBmaWx0ZXIgcG9zaXRpdmVzCnBvc2l0aXZlX251bWJlcnMgPSBbbnVtIGZvciBudW0gaW4gbnVtYmVycyBpZiBudW0gPiAwXQoKcHJpbnQocG9zaXRpdmVfbnVtYmVycyk=
Output:
[5, 10]
Code Explanation:
In the sample Python code:
- We begin with a list containing a mix of negative and positive numbers.
- Then, we build list comprehension with the expression num, which represents the elements to keep, and the iterable is the numbers list.
- In the condition, we use a relational operator, i.e., if num > 0, to filter out non-positive numbers.
Example 2: Filtering Strings Based On Length
We can also filter out a list of string values where we exclude elements that exceed a certain length. The example below illustrates the same.
Code Example:
I0xpc3Qgb2Ygc3RyaW5ncwp3b3JkcyA9IFsibGVhcm4iLCAicHJhY3RpY2UiLCAibWVudG9ycyIsICJqb2JzIl0KCiNVc2luZyBsaXN0IGNvbXByZWhlbnNpb24gd2l0aCBsZW4oKSBmdW5jdGlvbgpsb25nX3dvcmRzID0gW3dvcmQgZm9yIHdvcmQgaW4gd29yZHMgaWYgbGVuKHdvcmQpID4gNV0KCnByaW50KGxvbmdfd29yZHMp
Output:
['practice', 'mentors']
Code Explanation:
In the sample Python program:
- We begin with a list of strings called words, with four elements.
- Then, we use comprehension with the expression as word representing the strings to keep and the iterable is the list words.
- In the condition, we use the len() function to calculate the length of a string and then filter out words that are shorter than 5, i.e., len(word) > 5.
Example 3: Filtering Nested Lists
List comprehension works just as well with nested lists, as it does normal lists in Python. In the example below, we have illustrated how to use it to filter sublists that contain more than two elements.
Code Example:
I09yaWdpbmFsIG5lc3RlZCBsaXN0Cm5lc3RlZF9saXN0cyA9IFtbMV0sIFsxLCAyXSwgWzEsIDIsIDNdLCBbMSwgMiwgMywgNF1dCgojRXhjbHVkaW5nIHN1Ymxpc3RzIHdpdGggbGVzcyB0aGFuIDIgZWxlbWVudHMKbGFyZ2Vfc3VibGlzdHMgPSBbc3VibGlzdCBmb3Igc3VibGlzdCBpbiBuZXN0ZWRfbGlzdHMgaWYgbGVuKHN1Ymxpc3QpID4gMl0KCnByaW50KGxhcmdlX3N1Ymxpc3RzKQ==
Output:
[[1, 2, 3], [1, 2, 3, 4]]
Code Explanation:
In the Python code sample:
- We have a nested list containing four sublists of numbers.
- We use list comprehension on the nested list, which is the iterable, and the expression sublist, representing the lists to keep.
- With the condition if len(sublist) > 2, we filter out sublists that contain less than two elements.
Filtering lists with list comprehensions reduces the need for multiple lines of code, making the logic easy to understand and implement. We can also combine more than one condition to filter out elements from lists.
Check out this amazing course to become the best version of the Python programmer you can be.
Nested Loops With List Comprehension In Python
List comprehensions support nested loops, making it possible to iterate over multiple sequences in a single line of code. This feature is particularly useful for generating combinations, creating grids, or processing nested data structures.
Example 1: Generating Pair Combinations
In the example below we have illustrated how we can use nested for loops inside list comprehension to create all possible pairs of elements from two lists.
Code Example:
I09yaWdpbmFsIGxpc3RzCmxpc3QxID0gWyJBIiwgIkIiLCAiQyJdCmxpc3QyID0gWzEsIDIsIDNdCgojVXNpbmcgbGlzdCBjb21wcmVoZW5zaW9uIHRvIGNyZWF0ZSBsaXN0IHdpdGggcGFpcnMKcGFpcnMgPSBbKHgsIHkpIGZvciB4IGluIGxpc3QxIGZvciB5IGluIGxpc3QyXQoKcHJpbnQocGFpcnMp
Output:
[('A', 1), ('A', 2), ('A', 3), ('B', 1), ('B', 2), ('B', 3), ('C', 1), ('C', 2), ('C', 3)]
Code Explanation:
In the Python program sample:
- We begin with two lists, one containing three characters and one containing three numbers.
- Then, we use nested loops in list comprehension where the outer loop for x in list1 iterates over list1.
- The inner loop for y in list2 iterates over list2 for each element of list1.
- The result is a list of all possible pairs as suggested by the expression (x,y).
Similarly, we can use nested loops inside list comprehension to perform other manipulations. Like, you can create a list of products from two separate lists of numbers:
products = [x * y for x in list1 for y in list2]
You can also create a list of pairs of numbers (x, y) where both x and y are odd.
pairs = [(x, y) for x in range(1, 6) for y in range(1, 6) if x % 2 != 0 and y % 2 != 0]
Example 2: Nested List Comprehensions
In addition to using nested loops with list comprehension, we can also nest one list comprehension inside another (nested list comprehensions). The example below illustrates how to use nested list comprehension to generate a multiplication table from 1 to 3.
Code Example:
I1VzaW5nIG5lc3RlZCBsaXN0IGNvbXByZWhlbnNpb24KbXVsdGlwbGljYXRpb25fdGFibGUgPSBbW3ggKiB5IGZvciB5IGluIHJhbmdlKDEsIDQpXSBmb3IgeCBpbiByYW5nZSgxLCA0KV0KCiNQcmludGluZyB0aGUgbGlzdCBvZiB0YWJsZQpwcmludChtdWx0aXBsaWNhdGlvbl90YWJsZSk=
Output:
[[1, 2, 3], [2, 4, 6], [3, 6, 9]]
Code Explanation:
In the Python example:
- The inner comprehension [x * y for y in range(1, 4)] generates a row of the multiplication table for each x.
- The outer comprehension [... for x in range(1, 4)] repeats the process for numbers 1 to 3.
Flattening Nested Lists With List Comprehension In Python
Nested lists, also known as lists of lists, are common when working with multidimensional data. List comprehension provides an elegant way to flatten such structures into a single-level list, making the data easier to process.
Example 1: Flattening A Simple Nested List
In the Python program below, we have illustrated how to use list comprehension to flatten a nested list that forms a 2D table /grid.
Code Example:
I05lc3RlZCBsaXN0IHdpdGggdGhyZWUgc3VibGlzdHMKbmVzdGVkX2xpc3QgPSBbWzEsIDJdLCBbMywgNF0sIFs1LCA2XV0KCiNGbGF0dGVuaW5nIG5lc3RlZCBpbnRvIGEgc2luZ2xlIGxpbmVhciBsaXN0CmZsYXR0ZW5lZCA9IFtpdGVtIGZvciBzdWJsaXN0IGluIG5lc3RlZF9saXN0IGZvciBpdGVtIGluIHN1Ymxpc3RdCgpwcmludChmbGF0dGVuZWQp
Output:
[1, 2, 3, 4, 5, 6]
Code Explanation:
- We begin with the nested list containing three sublists of two numbers each.
- Then, we use nested loops in list comprehension to flatten them.
- Here, the outer loop for sublist in nested_list iterates over each sublist.
- The inner loop for item in sublist iterates over each element in the sublist.
- The result is a flat list of all elements in nested_list.
Example 2: Flattening A Deeply Nested List
In the example below, we illustrate how to flatten deeply nested lists, i.e., a list containing nested lists using nested loops and list comprehension.
Code Example:
I09yaWdpbmFsIGxpc3QKZGVlcF9uZXN0ZWRfbGlzdCA9IFtbWzEsIDJdLCBbMywgNF1dLCBbWzUsIDZdLCBbNywgOF1dXQoKI0xpc3QgY29tcHJlaGVuc2lvbiB3aXRoIHRocmVlIG5lc3RlZCBmb3IgbG9vcHMgdG8gZmxhdHRlbiBvcmlnaW5hbCBsaXN0CmZsYXR0ZW5lZCA9IFtudW0gZm9yIHN1Ymxpc3QxIGluIGRlZXBfbmVzdGVkX2xpc3QgZm9yIHN1Ymxpc3QyIGluIHN1Ymxpc3QxIGZvciBudW0gaW4gc3VibGlzdDJdCgpwcmludChmbGF0dGVuZWQp
Output:
[1, 2, 3, 4, 5, 6, 7, 8]
Code Explanation:
- We begin with a nested list containing more nested lists, named deep_nested_list.
- Then, we use list comprehension with three nested loops to flatten the list.
- Here, the outer loop for sublist1 in deep_nested_list iterates over the first level of lists.
- The middle loop for sublist2 in sublist1 iterates over the second level.
- The inner loop for num in sublist2 extracts the individual numbers.
In addition to flattening lists, you can also use specific conditions to filter or transform the lists while flattening. For example, say you want to flatten a nested list and filter even numbers. Here is what the list comprehension would look like:
flattened_evens = [num for sublist in nested_list for num in sublist if num % 2 == 0]
Similarly, you can perform other operations on list elements while flattening. For example, square each element while converting a nested list into a flat list:
squared_flattened = [num**2 for sublist in nested_list for num in sublist]
All in all, flattening nested lists with list comprehensions simplifies complex operations and allows for filtering or transforming elements in a single step.
Handling Exceptions In List Comprehension In Python
List comprehensions are typically used for creating lists, but what happens when an exception is encountered during the iteration? Python doesn’t allow for direct exception handling inside list comprehensions, but there are ways to handle errors gracefully.
1. Using Try-Except Inside List Comprehension
You can't directly use a try-except block inside the list comprehension syntax, but you can handle exceptions by using a function. Look at the example below to know how.
Code Example:
I0RlZmluaW5nIGEgZnVuY3Rpb24gdG8gaGFuZGxlIGV4Y2VwdGlvbnMKZGVmIHNhZmVfc3F1YXJlKHgpOgogICAgdHJ5OgogICAgICAgIHJldHVybiB4ICoqIDIKICAgIGV4Y2VwdCBUeXBlRXJyb3I6CiAgICAgIHJldHVybiBOb25lCgojTGlzdCBjb250YWluaW5nIG51bWJlcnMgYW5kIGNoYXJhY3RlcgpudW1iZXJzID0gWzEsICdhJywgM10KCiNTcXVhcmluZyBldmVyeSBlbGVtZW50IHVzaW5nIGxpc3QgY29tcHJlaGVuc2lvbgpzcXVhcmVkX251bWJlcnMgPSBbc2FmZV9zcXVhcmUobnVtKSBmb3IgbnVtIGluIG51bWJlcnNdCgpwcmludChzcXVhcmVkX251bWJlcnMp
Output:
[1, None, 9]
Here, safe_square() handles the exception if a non-numeric value is encountered.
2. Filtering Out Errors
We can also make use of the conditions inside the list comprehension to filter out problematic values. This helps avoid raising exceptions.
Code Example:
I0xpc3QgY29udGFpbmluZyBudW1iZXJzIGFuZCBjaGFyYWN0ZXJzCm51bWJlcnMgPSBbMSwgJ2EnLCAzXQoKI1NxdWFyaW5nIGVsZW1lbnRzIHVzaW5nIGxpc3QgY29tcHJlaGVuc2lvbgpzcXVhcmVkX251bWJlcnMgPSBbbnVtKioyIGZvciBudW0gaW4gbnVtYmVycyBpZiBpc2luc3RhbmNlKG51bSwgaW50KV0KCnByaW50KHNxdWFyZWRfbnVtYmVycyk=
Output:
[1, 9]
Here, we add a condition inside the list comprehension, which checks the type of the element of the original list. By adding a condition to check the type using the instanceof() function, you can avoid exceptions while processing.
3. Handling Specific Errors
When you expect specific errors, handle them selectively to avoid the program crashing. For example, you can put a condition in place so that the program behaves a certain way when it encounters problematic values/ elements.
Code Example:
bnVtYmVycyA9IFsxLCAyLCAzLCAneCddCgpyZXN1bHQgPSBbbnVtICogMiBpZiBpc2luc3RhbmNlKG51bSwgaW50KSBlbHNlICdFcnJvcicgZm9yIG51bSBpbiBudW1iZXJzXQoKcHJpbnQocmVzdWx0KQ==
Output:
[2, 4, 6, 'Error']
Here, 'x' is replaced with 'Error' instead of causing a crash.
Common Use Cases For List Comprehensions
List comprehensions shine in various scenarios where concise, efficient list creation and manipulation is required. To better understand the power of list comprehensions, let’s explore how they can be applied in real-world scenarios across different industries:
- Data Processing in Finance
In the finance industry, data is often processed in bulk. List comprehensions can be used to efficiently filter out transactions based on certain criteria, such as identifying all transactions above a certain amount or filtering by date.
Example: You could use list comprehension to extract transactions over a threshold amount from a large dataset, saving both memory and time in real-time systems. - Web Scraping for E-commerce
E-commerce websites often require scraping product details from multiple pages. List comprehensions can be used to pull specific data, like product prices, names, and availability, into structured lists.
Example: A scraper could use list comprehension to filter and collect products that meet certain criteria (e.g., within a price range or specific category). - Natural Language Processing (NLP)
In NLP, you may need to process and clean large sets of textual data. List comprehensions can quickly filter out unnecessary words, transform text into lowercase, or extract specific terms such as keywords or entities.
Example: You can use list comprehensions to clean text data by filtering out stop words or transforming text into a usable format for analysis or machine learning models. - Inventory Management in Logistics
In logistics, list comprehensions can help manage inventory by filtering items based on stock levels, shipment status, or product categories.
Example: For instance, list comprehensions can quickly create a list of items that need to be reordered or have reached a low stock level, which can then trigger an automatic restocking process. - Image Processing in Healthcare
In healthcare, especially in medical imaging, list comprehensions can be used to process large numbers of image files to identify patterns, filter images based on quality, or extract metadata from them for further analysis.
Example: For instance, list comprehensions could be used to process medical images by filtering out low-quality scans or extracting specific image features needed for diagnostic models. - Data Transformation Across Industries
List comprehensions are essential for reshaping or converting data into desired formats, making them highly versatile across domains like education, weather analysis, and business intelligence.
Example: To convert temperatures from Celsius to Fahrenheit for a weather report, transform student grades into pass/fail results in an education dataset, and normalize numerical data by scaling values for machine learning models. This flexibility makes list comprehensions a go-to tool for efficient data handling.
Advantages & Disadvantages Of List Comprehension In Python
List comprehensions are a powerful feature of Python, but they come with both benefits and limitations. Here’s a quick comparison to help you weigh their utility:
Advantages |
Disadvantages |
Concise and Readable Syntax: Compact, expressive code. |
Reduced Readability for Complex Logic: Difficult to debug. |
Improved Performance: Faster than traditional loops. |
Memory Consumption: Creates the entire list in memory. |
Combines Filtering and Transformation: One-liner tasks. |
Not Intuitive for Beginners: Syntax may overwhelm new learners. |
Eliminates Boilerplate Code: No initialization or appends. |
Lack of Flexibility: Not suited for multi-step operations. |
When To Use (Avoid) List Comprehension In Python Programs
List comprehensions are a great choice for tasks where:
- You need to transform or filter data in a single step.
- The operation is simple and doesn’t involve complex logic or debugging.
- The dataset size is manageable within available memory.
Avoid using them for:
- Large datasets, where memory efficiency is critical.
- Tasks with complex logic, where traditional loops or functions provide clarity.
Using list comprehension in Python is all about balance—leveraging their strengths while being mindful of their limitations ensures optimal code.
Looking for guidance? Find the perfect mentor from select experienced coding & software development experts here.
Best Practices For Using List Comprehension In Python
List comprehensions are powerful, but maintaining readability and clarity is key. Here are some tips to write effective list comprehension in Python programs:
- Keep It Simple: Avoid overloading a single comprehension with too much logic. If it’s hard to understand, switch to a loop or use helper functions.
- Use Descriptive Names: Use clear variable names to convey the purpose of the comprehension instead of relying on single-letter variables.
- Limit Nesting: Minimize nested comprehensions. Stick to one level for better readability.
- Avoid Side Effects: List comprehensions should be used for creating lists, not for side-effect operations (e.g., printing). Keep them focused.
- Optimize for Readability: If the comprehension is complex, split it into multiple lines or a traditional loop for clarity.
By following these practices, you can make sure your list comprehensions are both efficient and easy to understand.
Performance Considerations For List Comprehension In Python
List comprehensions can be faster than traditional loops, but their performance depends on the task at hand. Let’s consider key points for optimal use of list comprehension in Python:
- Speed: List comprehensions are often more efficient than regular for-loops due to their internal optimizations in Python. They work well for tasks like filtering or transforming data where the operation is straightforward.
- Memory Usage: List comprehensions create the entire list in memory, which can be inefficient for large datasets. For larger data, consider generator expressions, which generate items on-the-fly, saving memory.
- Avoid Unnecessary Computations: When filtering, ensure the condition is efficient. Avoid performing expensive operations in the comprehension.
- Use Cases for Performance: List comprehensions provide both speed and clarity for small to medium-sized datasets. However, when it comes to larger data sets, consider using generators or other methods that optimize memory usage.
In short, while list comprehensions offer speed, one must consider their memory impact and dataset size before using them for larger operations.
For Loops & List Comprehension In Python: A Comparison
While both for loops and list comprehensions allow iteration over data, they offer distinct advantages based on the context. Let’s break down the differences:
Aspect |
For Loops |
List Comprehensions |
Readability |
More readable for complex operations involving multiple steps. They are also easier to debug and understand, especially for beginners. |
Concise and compact, ideal for simple transformations. However, it can become hard to read for complex operations or nested loops. |
Performance |
Slower than list comprehensions due to the extra overhead of initialization, iteration, and appending. |
Faster for simple tasks since it executes in a single step and is optimized internally by Python. |
Flexibility |
More flexible and can handle multiple operations, conditions, and transformations. |
Less flexible; ideal for simple tasks, but doesn’t work well for complex logic with multiple steps. |
Use Case |
Suitable for multi-step operations, tasks with complex conditions, or when side effects (e.g., printing) are involved. |
Best for simple filtering, transformation, or generating new lists with a single expression. |
In short, for loops are best for flexibility, complex logic, and handling multiple conditions or side effects. List comprehensions are perfect for simple, efficient operations like transformations or filtering, but readability decreases with complexity.
Difference Between Generator Expression & List Comprehension In Python
While list comprehensions and generator expressions share a similar syntax, they differ in how they handle memory and execution. Let’s break down the key differences:
Aspect |
List Comprehensions |
Generator Expressions |
Memory Usage |
List comprehensions generate the entire list in memory. |
Generator expressions generate items on-the-fly, making them more memory-efficient. |
Performance |
Faster for smaller datasets as they return the entire list at once. |
Slower for accessing items one by one, but better for large datasets where memory is a concern. |
Return Type |
Returns a complete list. |
Returns a generator object, which can be iterated over but doesn’t store the data in memory. |
Use Case |
Ideal for tasks where the entire list is needed at once. |
Ideal for large datasets where you need to iterate without holding everything in memory. |
Syntax |
Written in square brackets [ ]. |
Written in parentheses ( ). |
Use list comprehensions when you need the entire list available immediately and memory isn’t a concern. Use generator expressions when dealing with large datasets or when memory efficiency is a priority.
Conclusion
List comprehensions in Python are a versatile and elegant tool for creating, transforming, and filtering lists. By combining power and simplicity, they allow developers to write concise and efficient code, making them a must-know feature for Python enthusiasts. Whether you're working with conditional statements, nested loops, or handling complex data structures, list comprehensions can simplify your workflow and improve code readability.
However, as with any tool, moderation and thoughtfulness are key. By adhering to best practices and understanding when to prioritize readability or performance, you can harness their full potential without compromising maintainability. From data processing to web scraping and beyond, list comprehensions are more than a syntax shortcut—they’re a productivity booster for developers across industries.
Ready to upskill your Python knowledge? Dive deeper into list comprehensions and elevate your coding game with hands-on practice!
Frequently Asked Questions
Q1. What is the main purpose of list comprehensions in Python?
List comprehensions provide a concise way to create, transform, and filter lists in Python, allowing for more readable and efficient code compared to traditional loops.
Q2. Can list comprehensions be used with conditional statements?
Yes, list comprehensions support conditional statements. You can use if conditions to filter items and if-else for conditional transformations within the comprehension.
Q3. How do list comprehensions differ from generator expressions?
The main difference is that list comprehensions generate the entire list in memory, while generator expressions yield items one at a time, making them more memory-efficient for large datasets.
Q4. Are list comprehensions always faster than for loops?
Not always. While list comprehensions are generally faster due to their optimized implementation, their speed advantage diminishes for very complex logic or nested operations.
Q5. When should I avoid using list comprehensions?
Avoid using list comprehensions if:
- The logic is too complex, making the code harder to read.
- The dataset is extremely large and memory usage is a concern (use generator expressions instead).
Q6. Can list comprehensions work with multiple input lists?
Yes, you can use nested loops in list comprehensions to process multiple input lists, making them a great tool for combining or transforming data from multiple sources.
Q7. Are list comprehensions supported in all versions of Python?
List comprehensions have been a part of Python since version 2.0. However, some advanced features, such as conditional expressions, are available only in Python 2.7 and later versions.
This compiles our discussion on list comprehension in Python. Here are some more interesting topics you must explore:
- Python List Slice Technique | Syntax & Use Cases (+Code Examples)
- Python List index() Method | Use Cases Explained (+Code Examples)
- Python List insert() Method Explained With Detailed Code Examples
- Python List sort() | All Use Cases Explained (+Code Examples)
- Python Linked Lists | A Comprehensive Guide (With Code Examples)
- Python List append() | Syntax & Working Explained (+Code Examples)
An economics graduate with a passion for storytelling, I thrive on crafting content that blends creativity with technical insight. At Unstop, I create in-depth, SEO-driven content that simplifies complex tech topics and covers a wide array of subjects, all designed to inform, engage, and inspire our readers. My goal is to empower others to truly #BeUnstoppable through content that resonates. When I’m not writing, you’ll find me immersed in art, food, or lost in a good book—constantly drawing inspiration from the world around me.
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
Comments
Add comment