Table of content:
- What Is Python List Slicing?
- What Is Indexing In Python Lists?
- Python List Slice Method To Retrieve All List Elements
- Getting Elements Before A Specific Index/Position Using Python List Slice Method (First N Elements)
- Selecting Elements After Specific Index/Position Using Python List Slice Method
- Extract Slices Between Two Indices (From One Position To Another) Using Python List Slice Method
- Adjust Step Size In Python List Slice Method To Get Element At Specified Interval (Every Nth List Element)
- Python List Slice To Reverse A List
- Modify A List Using Python List Slice Method
- Resize List/Remove Elements Using Python List Slice Method
- Using Python List Slice To Extract Elements Of A Nested List
- When Does Python Slice Method Return Empty Slices?
- Common Mistakes To Avoid With Python List Slice
- Conclusion
- Frequently Asked Questions
Python List Slice Technique | Syntax & Use Cases (+Code Examples)
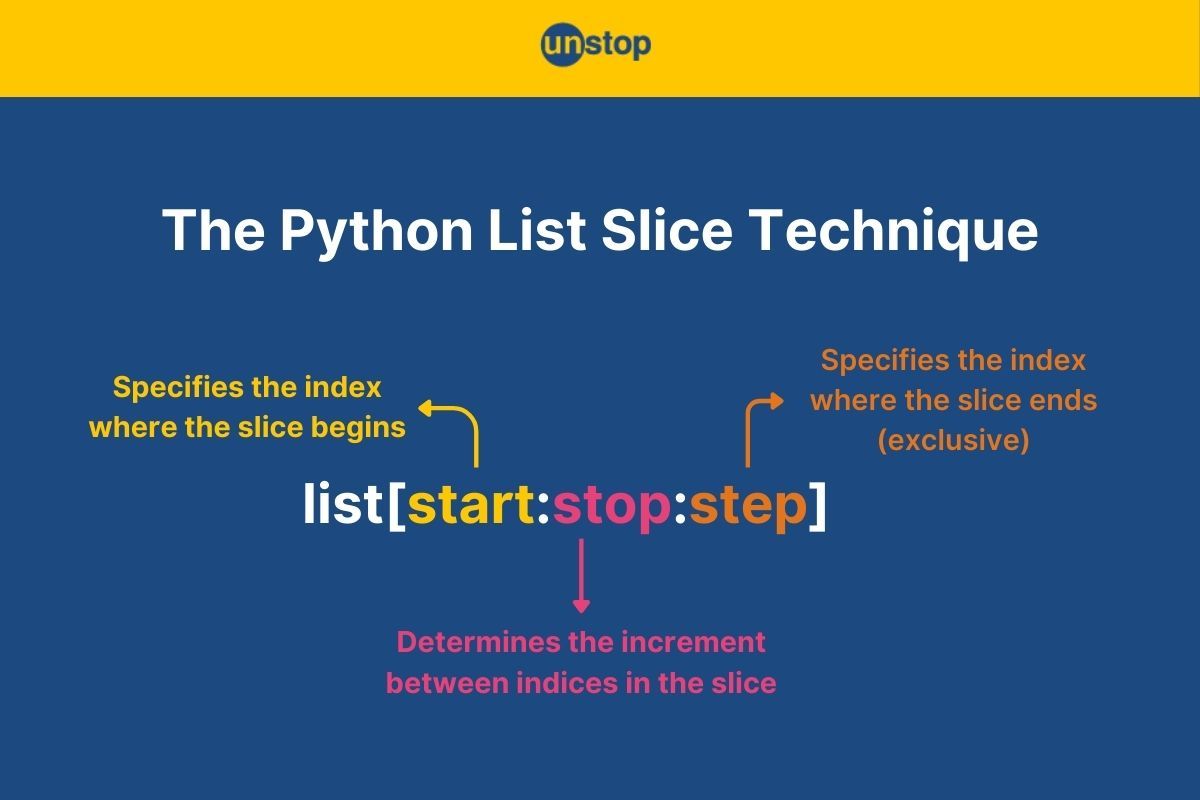
Python lists are data structures that can hold a mix of values, from numbers to strings, in a single collection. But what if you want to access just a portion of this list? Enter the Python list slice method—a technique that allows you to extract, modify, or analyze specific parts of a list effortlessly.
In this article, we’ll explore the ins and outs of Python list slicing, covering its syntax, parameters, and practical applications.
What Is Python List Slicing?
List slicing in Python is a way to extract a part of a list by specifying a range of indices. Think of it as cutting a piece from a loaf of bread—you take only what you need while leaving the rest intact. For example, if you have a list of city names, slicing lets you pick only the first three cities or skip every alternate city in the list.
Imagine you’re sorting through a digital photo album. You don’t flip through every photo; instead, you might pick photos from a specific vacation or skip every few pages to look at highlights. Python list slicing works similarly—it lets you pick the sections you’re interested in without altering the original list.
With list slicing, you can:
- Retrieve specific parts of a list.
- Modify portions of the list directly.
- Handle subsets of nested lists.
This makes the Python list slice technique a powerful tool for data manipulation, whether you’re working with simple lists or complex data structures.
Python List Slicing Syntax
The syntax for the Python list slice method is simple and intuitive:
list[start:stop:step]
Parameters Of Python List Slice Method
The Python list slicing syntax has three key parameters: start, stop, and step. Each parameter offers flexibility in determining which part of the list to extract. Let’s explore these in detail:
- start: The start parameter specifies the index where the slice begins.
Default Value: If the start is not specified, it defaults to the beginning of the list (0).
Usage:
my_list = [10, 20, 30, 40, 50]
print(my_list[1:]) # [20, 30, 40, 50]
In this example, slicing starts at index 1 (second element).
- stop: The stop parameter specifies the index where the slice ends (exclusive).
Default Value: If stop is not specified, slicing goes up to the end of the list.
Usage:
my_list = [10, 20, 30, 40, 50]
print(my_list[:4]) # [10, 20, 30, 40]
Here, slicing stops at index 4, excluding the fifth element (50).
- step: The step parameter determines the increment between indices in the slice.
Default Value: If step is not specified, it defaults to 1.
Negative Step: A negative step value slices the list in reverse order.
Usage:
my_list = [10, 20, 30, 40, 50]
print(my_list[::2]) # [10, 30, 50]
print(my_list[::-1]) # [50, 40, 30, 20, 10]
In the first example, my_list[::2], the step of 2 skips every second element, selecting [10, 30, 50]. In the second example, my_list[::-1], the negative step of -1 reverses the list, producing [50, 40, 30, 20, 10].
Return Value Of Python List Slice
The Python list slice method always returns a new list containing the extracted elements based on the specified start, stop, and step parameters. The original list remains unchanged, as slicing creates a shallow copy of the selected portion. If no elements match the slicing criteria, an empty list ([]) is returned instead of raising an error, ensuring safe and predictable behavior.
What Is Indexing In Python Lists?
Indexing is a method to access individual elements in a Python list by referring to their position. In Python programming, lists support two types of indexing: positive and negative, both of which are essential for slicing.
Positive Indexing
Positive indexing starts from 0 and moves forward, with each index representing the position of an element in the list.
Example:
my_list = ['a', 'b', 'c', 'd', 'e']
print(my_list[0]) # 'a'
print(my_list[3]) # 'd'
Here, 0 refers to the first element, and 3 refers to the fourth element.
Negative Indexing
Negative indexing starts from -1 and moves backward, making it convenient to access elements relative to the end of the list.
Example:
my_list = ['a', 'b', 'c', 'd', 'e']
print(my_list[-1]) # 'e'
print(my_list[-3]) # 'c'
In this case, -1 refers to the last element, and -3 refers to the third element from the end.
Indexing is fundamental to list slicing as it defines the positions of elements to include in the slice. It allows precise extraction of subsets by specifying start, stop, and step values. For example, you can use positive indexing to get [1:4] for specific elements or negative indexing to reverse a list with [::-1].
Python List Slice Method To Retrieve All List Elements
The Python list slicing method allows you to extract all elements from a list effortlessly. You can do this by omitting the start, stop, and step parameters in the slicing syntax. This can be useful when you want a shallow copy of the list or when manipulating slices without altering the original. The basic Python program example below illustrates this method.
Code Example:
IyBPcmlnaW5hbCBsaXN0Cm15X2xpc3QgPSBbJ3Vwc2tpbGwnLCAnbGVhcm4nLCAncHJhY3RpY2UnLCAnZ2V0IGhpcmVkJ10KCiMgVXNpbmcgc2xpY2luZyB0byByZXRyaWV2ZSBhbGwgZWxlbWVudHMKYWxsX2VsZW1lbnRzID0gbXlfbGlzdFs6XQoKcHJpbnQoYWxsX2VsZW1lbnRzKQ==
Output:
['upskill', 'learn', 'practice', 'get hired']
Code Explanation:
In the basic Python code example,
- We begin by creating a list called my_list containing four string elements.
- Then, we use the slicing syntax [:] while omitting start and stop parameters.
- As a result, slicing begins from the first element (index 0) and goes up to the end of the list. We also omit the step so that default 1 ensures that every element is consecutive.
- We store the outcome in the variable all_elements, a new list containing all elements of my_list. This ensures the original list remains unchanged while you work with its copy.
This approach is especially useful when you need to operate on a copy of the list without modifying the original data structure.
Check out this amazing course to become the best version of the Python programmer you can be.
Getting Elements Before A Specific Index/Position Using Python List Slice Method (First N Elements)
In Python, you can easily retrieve all elements before a specific index or position by specifying a stop value and omitting the start and step. This is like retrieving the first n elements from the beginning of the list up to, but not including, the given index.
This can be helpful when you need a subset of the list that doesn't include certain elements at the end. The simple Python program example below illustrates this scenario.
Code Example:
IyBPcmlnaW5hbCBsaXN0Cm15X2xpc3QgPSBbJ3Vwc2tpbGwnLCAnbGVhcm4nLCAncHJhY3RpY2UnLCAnZ2V0IGhpcmVkJ10KCiMgVXNpbmcgc2xpY2luZyB0byBnZXQgZWxlbWVudHMgYmVmb3JlIGluZGV4IDMKYmVmb3JlX2luZGV4ID0gbXlfbGlzdFs6M10KCnByaW50KGJlZm9yZV9pbmRleCk=
Output:
['upskill', 'learn', 'practice']
Code Explanation:
In the simple Python code example,
- We begin with a list my_list contains four string elements: ['upskill', 'learn', 'practice', 'get hired'].
- Then, we use slicing syntax with only the stop position specified, i.e., [:3].
- We omit start and step, so the slice starts from the beginning of the list (index 0) and default step of 1 selects consecutive elements.
- The stop is specified as 3, meaning the slice will include elements from index 0 up to, but not including, index 3.
- We create a new list before_index to store the outcome and display the same using the print() function.
This technique is useful when you need to work with the initial part of a list, such as getting a subset of items before a specific position without affecting the rest of the list.
Selecting Elements After Specific Index/Position Using Python List Slice Method
Python’s list slicing method also allows you to retrieve elements after a certain index or position, by specifying the start index and omitting the stop and step. This is particularly useful when you want to process elements starting from a certain point in the list, ignoring everything before it.
Code Example:
IyBPcmlnaW5hbCBsaXN0Cm15X2xpc3QgPSBbJ3Vwc2tpbGwnLCAnbGVhcm4nLCAncHJhY3RpY2UnLCAnZ2V0IGhpcmVkJ10KCiMgVXNpbmcgc2xpY2luZyB0byBnZXQgZWxlbWVudHMgYWZ0ZXIgaW5kZXggMgphZnRlcl9pbmRleCA9IG15X2xpc3RbMzpdCgpwcmludChhZnRlcl9pbmRleCk=
Output:
['get hired']
Code Explanation:
In the Python program example,
- We begin with the same list my_list and then use the slicing index with only start parameter set to 3, i.e., [3:].
- This means the slice will begin at the fourth element (index 3), which is 'get hired'.
- The stop and step are omitted, so the slice continues to the end of the list and selects every element from index 3 onward.
- We store the outcome in the new list after_index, which we then print to the console. Elements before index 3 (i.e., 'upskill', 'learn', and 'practice') are excluded.
This approach is ideal for working with the tail end of a list or when you want to focus on elements that appear after a specific position.
Extract Slices Between Two Indices (From One Position To Another) Using Python List Slice Method
List slicing in Python allows you to extract a range of elements between two indices by specifying both a start and a stop value. This is useful when you need a specific subset of the list, excluding everything before the starting index and after the stopping index.
Code Example:
IyBPcmlnaW5hbCBsaXN0Cm15X2xpc3QgPSBbJ3Vwc2tpbGwnLCAnbGVhcm4nLCAncHJhY3RpY2UnLCAnZ2V0IGhpcmVkJ10KCiMgVXNpbmcgc2xpY2luZyB0byBnZXQgZWxlbWVudHMgYmV0d2VlbiBpbmRleCAxIGFuZCAzCnJhbmdlX3NsaWNlID0gbXlfbGlzdFsxOjNdCgpwcmludChyYW5nZV9zbGljZSk=
Output:
['learn', 'practice']
Code Explanation:
In the Python code example,
- We create a list named my_list with four string elements and then use the slice syntax with step and stop arguments set at 1 and 3, respectively (i.e., [1:3]).
- With the start index at 1, the slice begins at the second element, 'learn', and stops at index 3, i.e., before the fourth element, 'get hired'.
- Since we omitted the step, every element in the specified range is selected consecutively.
- We store the outcome in range_slice and print it. The elements before index 1 and after index 2 are excluded from the new list.
This slicing method is particularly useful when you need to extract a subset of elements from the middle of a list, defined by specific starting and stopping points.
Adjust Step Size In Python List Slice Method To Get Element At Specified Interval (Every Nth List Element)
Using the step parameter in Python list slicing, you can select elements at specified intervals. This method helps when you want to pick every nth element from the list, whether it’s every second, third, or another interval. The step size provides flexibility in skipping over elements in a sequence.
Code Example:
IyBPcmlnaW5hbCBsaXN0Cm15X2xpc3QgPSBbJ3Vwc2tpbGwnLCAnbGVhcm4nLCAncHJhY3RpY2UnLCAnZ2V0IGhpcmVkJywgJ25ldHdvcmsnLCAnZ3JvdyddCgojIFVzaW5nIHNsaWNpbmcgdG8gZ2V0IGV2ZXJ5IHNlY29uZCBlbGVtZW50CmludGVydmFsX3NsaWNlID0gbXlfbGlzdFs6OjJdCgpwcmludChpbnRlcnZhbF9zbGljZSk=
Output:
['upskill', 'practice', 'network']
Code Explanation:
In the example Python program,
- We create a list my_list containing six string elements and use the slice syntax with step argument set to 2, i.e., [::2].
- Since we omit start and stop, the slice starts from the first element and continues to the end of the list.
- The step of 2 means every second element will be selected. The resulting list includes elements at indices 0, 2, and 4.
- We store the outcome in the variable interval_slice that holds the list ['upskill', 'practice', 'network'], containing every second element from the original list.
Customizing the step size allows you to retrieve elements at regular intervals, such as every nth element, making it a powerful tool for creating patterns or skipping elements in a sequence.
Level up your coding skills with the 100-Day Coding Sprint at Unstop and get the bragging rights now!
Python List Slice To Reverse A List
Python list slicing provides an elegant way to reverse a list without needing to use loops or additional methods. This can be done by setting the step parameter to -1, which will reverse the order of elements in the list, starting from the last element and moving towards the first.
Code Example:
IyBPcmlnaW5hbCBsaXN0Cm15X2xpc3QgPSBbJ3Vwc2tpbGwnLCAnbGVhcm4nLCAncHJhY3RpY2UnLCAnZ2V0IGhpcmVkJ10KCiMgUmV2ZXJzaW5nIHRoZSBsaXN0IHVzaW5nIHNsaWNpbmcKcmV2ZXJzZWRfbGlzdCA9IG15X2xpc3RbOjotMV0KCnByaW50KHJldmVyc2VkX2xpc3Qp
Output:
['get hired', 'practice', 'learn', 'upskill']
Code Explanation:
In the example Python code,
- We begin with the list my_list containing four elements and use the slicing method with negative indexing, i.e., [::-1].
- Here, we set the step argument -1, which indicates the direction of slicing (from right to left), effectively reversing the order of elements in the list.
- Since we omit start and stop, the slice starts from the end of the list and proceeds towards the beginning.
- We store the outcome in reversed_list that contains ['get hired', 'practice', 'learn', 'upskill'], which is the original list but in reverse order.
Reversing a list using slicing is a concise and Pythonic way to achieve this, making it more readable and efficient compared to traditional methods.
Modify A List Using Python List Slice Method
List slicing in Python isn’t just for retrieving elements; it can also be used to modify lists. By assigning a new value to a slice of a list, you can replace elements in a specified range or even insert new elements. This makes list slicing a powerful tool for in-place modification of lists.
Code Example:
IyBPcmlnaW5hbCBsaXN0Cm15X2xpc3QgPSBbJ3Vwc2tpbGwnLCAnbGVhcm4nLCAncHJhY3RpY2UnLCAnZ2V0IGhpcmVkJ10KCiMgVXNpbmcgc2xpY2luZyB0byBtb2RpZnkgdGhlIGxpc3QKbXlfbGlzdFsxOjNdID0gWydhZHZhbmNlJywgJ21hc3RlciddCgpwcmludChteV9saXN0KQ==
Output:
['upskill', 'advance', 'master', 'get hired']
Code Explanation:
In the sample Python program,
- We create a list my_list with four elements and use the slice method to modify its elements.
- Here, we use the slice notation with start set to 1 and stop set to 3, i.e., [1:3]. This means that slicing will begin at index 1 (the element 'learn') and end just before index 3 (the element 'practice').
- We use the direct assignment operator to assign new values ['advance', 'master'] to this slice, which replaces the original elements at indices 1 and 2.
- After the modification, my_list becomes ['upskill', 'advance', 'master', 'get hired'], with the elements 'learn' and 'practice' replaced by 'advance' and 'master'.
- We print the modified list using the print() function in Python.
Using list slicing to modify a list allows you to replace a range of elements in a single operation, making it a flexible way to update or rearrange list contents without having to loop through the list.
Resize List/Remove Elements Using Python List Slice Method
Python list slicing provides an efficient way to both resize and remove elements from a list. By specifying a slice, you can either select a subset of elements to retain or remove unwanted elements by replacing them with an empty list. This flexibility allows for quick and intuitive list modification.
Code Example:
IyBPcmlnaW5hbCBsaXN0Cm15X2xpc3QgPSBbJ3Vwc2tpbGwnLCAnbGVhcm4nLCAncHJhY3RpY2UnLCAnZ2V0IGhpcmVkJ10KCiMgUmVzaXppbmcgdGhlIGxpc3QgdG8gaW5jbHVkZSBvbmx5IHRoZSBmaXJzdCB0d28gZWxlbWVudHMKbXlfbGlzdCA9IG15X2xpc3RbOjJdCnByaW50KG15X2xpc3QpCgojIFJlbW92aW5nIGVsZW1lbnRzIGZyb20gaW5kZXggMSB0byAyIHVzaW5nIHNsaWNpbmcKbXlfbGlzdFsxOjJdID0gW10KcHJpbnQobXlfbGlzdCk=
Output:
['upskill', 'learn']
['upskill']
Code Explanation:
In the sample Python code,
- We begin with the my_list containing four string elements.
- Resizing the List: The first slice my_list[:2] selects the first two elements of the list. The list is then resized to ['upskill', 'learn'].
- Removing Elements: In the second operation, the slice [1:2] selects the element at index 1 (i.e., 'learn') and replaces it with an empty list [], effectively removing it from the list.
- Resulting List: After the resizing and removal operations, the final list is ['upskill'].
By combining these techniques, you can easily resize or remove elements from a list in a single operation, offering a concise and efficient approach for list modification.
Looking for guidance? Find the perfect mentor from select experienced coding & software development experts here.
Using Python List Slice To Extract Elements Of A Nested List
When working with nested lists (lists containing other lists), you can use list slicing not only to extract individual elements but also to access sublists within those nested structures. By applying slicing to a nested list, you can extract specific elements or sublists at various levels of nesting.
Code Example:
IyBPcmlnaW5hbCBuZXN0ZWQgbGlzdApuZXN0ZWRfbGlzdCA9IFtbJ3Vwc2tpbGwnLCAnbGVhcm4nXSwgW2NvbXBldGUnLCAnZ2V0IGhpcmVkJ10sIFsnY29kZScsICdwcmFjdGljZScsICdncm93J11dCgojIEV4dHJhY3RpbmcgdGhlIGZpcnN0IHN1Ymxpc3QKZmlyc3Rfc3VibGlzdCA9IG5lc3RlZF9saXN0WzBdCnByaW50KGZpcnN0X3N1Ymxpc3QpCgojIEV4dHJhY3RpbmcgdGhlIGZpcnN0IHR3byBlbGVtZW50cyBmcm9tIHRoZSBzZWNvbmQgc3VibGlzdApzZWNvbmRfc3VibGlzdCA9IG5lc3RlZF9saXN0WzFdWzoyXQpwcmludChzZWNvbmRfc3VibGlzdCk=
Output:
['upskill', 'learn']
['practice', 'get hired']
Code Explanation:
In the Python program sample,
- We create a nested list named nested_list, which contains three sublists: [['upskill', 'learn'], ['compete', 'get hired'], ['code', 'practice', 'grow']].
- Extracting the First Sublist: The slice nested_list[0] accesses the first sublist ['upskill', 'learn'] by using the index 0 on the outer list.
- Extracting Elements from a Sublist: The slice nested_list[1][:2] accesses the second sublist ['compete', 'get hired'], and the inner slice [:2] extracts the first two elements of this sublist.
- We print the respective sublists to the console using the print() built-in function.
This technique allows for precise manipulation of nested lists, whether you want to extract a full sublist or specific elements from within those sublists. Python’s slicing capability makes it easy to access deeply nested data structures with minimal effort.
When Does Python Slice Method Return Empty Slices?
Empty slices in Python can occur for several reasons, and it's important to understand the scenarios in which they arise. Below, we’ll cover two key cases that typically result in empty slices: when indices are out of bounds and when the step exceeds the length of the list.
Handling Out-of-Bounds Indices
When working with Python list slicing, it's important to handle cases where indices are out of bounds. Fortunately, Python handles out-of-bounds indices gracefully, so you don’t need to worry about errors like the IndexError (Index out of range). Python simply returns an empty list or the available elements within the valid range, depending on the situation.
Code Example:
IyBPcmlnaW5hbCBsaXN0Cm15X2xpc3QgPSBbJ3Vwc2tpbGwnLCAnbGVhcm4nLCAncHJhY3RpY2UnLCAnZ2V0IGhpcmVkJ10KCiMgQXR0ZW1wdGluZyB0byBzbGljZSBiZXlvbmQgdGhlIGxpc3Qgc2l6ZQpvdXRfb2ZfYm91bmRzID0gbXlfbGlzdFsxMDoxNV0KCnByaW50KG91dF9vZl9ib3VuZHMp
Output:
[]
Code Explanation:
In the Python code sample,
- We create a list my_list containing four elements: ['upskill', 'learn', 'practice', 'get hired'].
- Then, we use the slice method to extract elements from the index position 10 to 15, i.e., [10:15].
- But these indexes are beyond the length of the list.
- Instead of throwing an error, Python returns an empty list [] (as shown in the output), since there are no elements in the specified range.
2. When Step Exceeds List Length
In Python, an empty slice occurs when the slice range does not correspond to any elements in the list. This might happen if the start and stop indices do not match any elements, or if the step size is such that no elements are selected. Python returns an empty list in such cases, which is useful in various situations like conditionally slicing or avoiding errors when indices are out of range.
Code Example:
IyBPcmlnaW5hbCBsaXN0Cm15X2xpc3QgPSBbJ3Vwc2tpbGwnLCAnbGVhcm4nLCAncHJhY3RpY2UnLCAnZ2V0IGhpcmVkJ10KCiMgRW1wdHkgc2xpY2Ugd2hlbiB0aGUgc3RhcnQgaW5kZXggaXMgZ3JlYXRlciB0aGFuIHRoZSBzdG9wIGluZGV4CmVtcHR5X3NsaWNlID0gbXlfbGlzdFszOjJdCnByaW50KGVtcHR5X3NsaWNlKQoKIyBFbXB0eSBzbGljZSB3aGVuIHVzaW5nIGEgc3RlcCBzaXplIGxhcmdlciB0aGFuIHRoZSBsZW5ndGggb2YgdGhlIGxpc3QKc3RlcF9zbGljZSA9IG15X2xpc3RbOjo1XQpwcmludChzdGVwX3NsaWNlKQ==
Output:
[]
[]
Code Explanation:
In the example, we begin with the same list as above.
- First Empty Slice [3:2]: The slice [3:2] specifies a start index of 3 and a stop index of 2, which does not make sense because the start index is greater than the stop index. As a result, Python returns an empty list [].
- Second Empty Slice [::5]: Here, the slice has no start or stop specified, but the step is 5, which is greater than the length of the list. This results in an empty list because no element is spaced 5 indices apart in the list.
- In both cases, the output is an empty list [].
Empty slices are a normal part of Python list slicing and occur when the slicing criteria cannot be met. They can be useful for safely handling situations where the data may or may not meet certain conditions, without raising errors.
Common Mistakes To Avoid With Python List Slice
While Python list slicing is powerful and flexible, there are a few common mistakes that you must note. Understanding these errors can help avoid confusion and make your slicing experience more efficient.
Mistake 1: Not Understanding the Stop Parameter Behavior
One common mistake is misunderstanding how the stop parameter works in slicing. The stop index is exclusive, meaning that the element at the stop index is not included in the slice, which can lead to off-by-one errors. Example:
my_list = [10, 20, 30, 40, 50]
print(my_list[:3]) # [10, 20, 30] (not [10, 20, 30, 40])
Mistake 2: Confusing Positive and Negative Indexing
Another mistake is not fully understanding the difference between positive and negative indexing. Negative indices count from the end of the list, but some may get confused about how they map to list positions. Example:
my_list = [10, 20, 30, 40, 50]
print(my_list[-1]) # 50 (last element)
print(my_list[:-1]) # [10, 20, 30, 40] (all but the last element)
Mistake 3: Slicing with an Incorrect Step Value
Using an incorrect or inappropriate step value can lead to unexpected results, such as skipping elements unintentionally or getting an empty list when the step is too large. Example:
my_list = [10, 20, 30, 40, 50]
print(my_list[::10]) # [10] (step is too large, only the first element is included)
Mistake 4: Modifying the List While Slicing
While it’s tempting to modify the list within the slice itself, such as replacing elements, it's important to ensure you're not accidentally affecting the list you're iterating over in a way that leads to logical errors. Example:
my_list = [10, 20, 30, 40, 50]
my_list[1:3] = [100, 200] # Replaces the second and third elements with new values
print(my_list) # [10, 100, 200, 40, 50]
By being aware of these common mistakes and knowing how to avoid them, you can use Python list slice technique more effectively and avoid some of the pitfalls that might cause confusion or unexpected behavior.
Conclusion
The Python slice method allows us to extract, modify, and manipulate portions of lists efficiently with just a few lines of code. The slicing index allows for three optional parameters start (default 0), stop (default end), and step (default 1). Using these parameters efficiently as well as handling out-of-bounds indices and nested lists, you can enhance your ability to work with lists in Python.
List slicing is not only concise but also powerful, offering flexibility in many scenarios. Whether you’re extracting elements, modifying parts of a list, or working with intervals, Python’s slicing capabilities provide a versatile way to handle lists.
Frequently Asked Questions
Q1. What is Python list slicing?
Python list slicing allows you to extract a portion of a list by specifying a start index, a stop index, and an optional step size. It provides a concise way to manipulate and access parts of a list.
Q2. How do I slice a list in Python?
You can slice a list using the syntax: my_list[start:stop:step]. The start index is where the slice begins, stop is where it ends (exclusive), and step determines the interval between elements.
Q3. What happens if I use a stop index greater than the list length?
If the stop index exceeds the length of the list, Python will simply return elements up to the end of the list without raising an error.
Q4. Can I slice a list in reverse order?
Yes, you can reverse a list in Python by using the slice method with a negative step value. For example, my_list[::-1] will reverse the list.
Q5. What is the difference between list slicing and list indexing?
Indexing retrieves a single element from the list, while slicing extracts a sublist. Slicing allows you to work with a range of elements, whereas indexing gives you access to one specific element.
Q6. Can I modify a list using slicing?
Yes, you can modify elements of a list using slicing. For instance, you can replace a part of the list or even delete elements using direct slice assignment.
Q7. What happens if the slice range is invalid, like my_list[5:2]?
When the slice range is invalid (start index is greater than stop), Python returns an empty list. It doesn’t throw an error but simply gives you an empty list.
Q8. How do I slice a nested list?
You can slice nested lists in the same way as regular lists. For example, nested_list[1][:2] retrieves the first two elements of the second sublist in a nested list.
By now, you must know all about the uses and versatility of the Python list slice technique. Do check out the following for more interesting reads:
- Python List sort() | All Use Cases Explained (+Code Examples)
- Remove Duplicates From Python List | 12 Ways With Code Examples
- Python Libraries | Standard, Third-Party & More (Lists + Examples)
- Python String Concatenation In 10 Easy Ways Explained (+Examples)
- How To Reverse A String In Python? 10 Easy Ways With Examples
- If-Else Statement In Python | All Conditional Statements + Examples
An economics graduate with a passion for storytelling, I thrive on crafting content that blends creativity with technical insight. At Unstop, I create in-depth, SEO-driven content that simplifies complex tech topics and covers a wide array of subjects, all designed to inform, engage, and inspire our readers. My goal is to empower others to truly #BeUnstoppable through content that resonates. When I’m not writing, you’ll find me immersed in art, food, or lost in a good book—constantly drawing inspiration from the world around me.
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
Comments
Add comment