Python Infinite Loop | Types, Applications & More (+Code Examples)
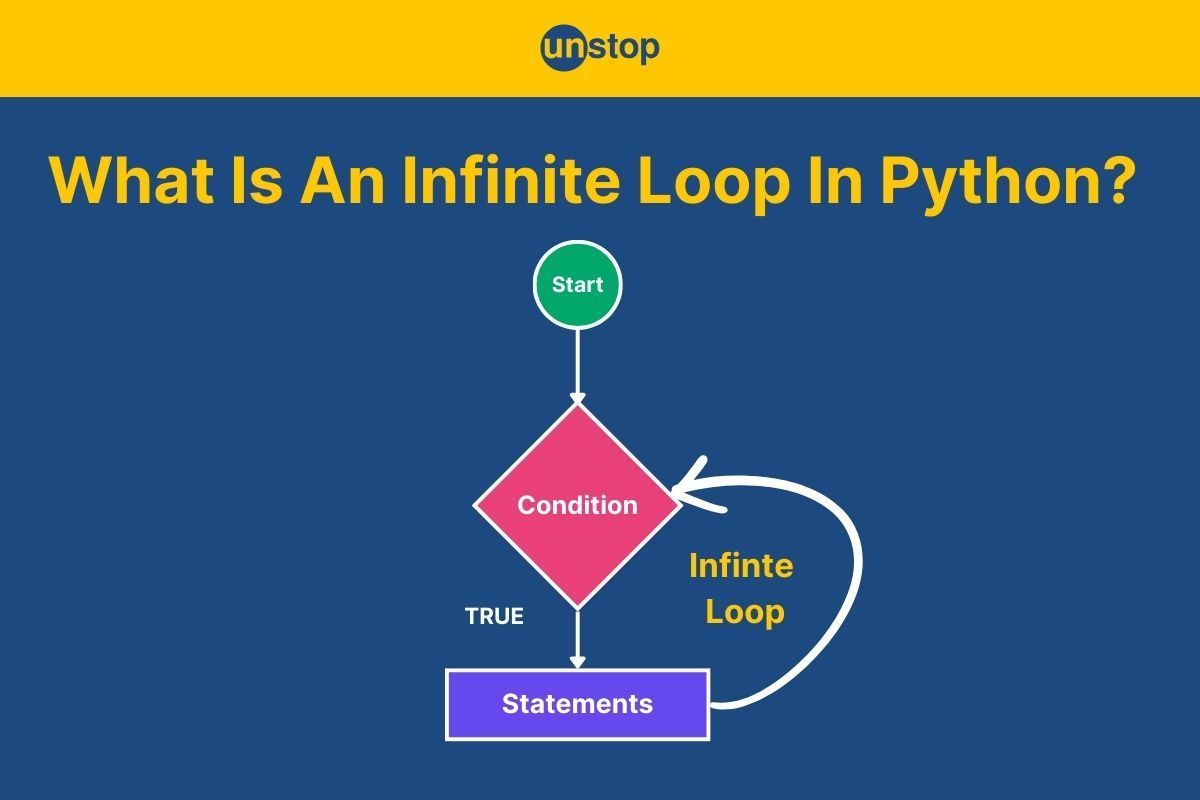
Infinite loops are a fundamental concept in programming, and Python is no exception. An infinite loop occurs when a sequence of instructions continues to execute endlessly without a defined endpoint, often due to the loop's condition being perpetually met. While this might sound problematic, infinite loops can be incredibly useful in various scenarios, such as handling real-time data, creating interactive applications, and maintaining ongoing processes.
Understanding how to effectively create, manage, and exit infinite loops is crucial for any Python developer. In this article, we will explore the mechanics of infinite loops, their practical applications and different types, and the best practices for avoiding common pitfalls associated with them.
Understanding Python Infinite Loops
An infinite loop in Python programming language is a sequence that continues indefinitely until it is externally stopped. This type of loop does not have a terminating condition. It often uses conditions that are always true, which causes the loop to never end. In Python, infinite loops can be intentional or unintentional. Developers may create them for specific tasks. However, they can also occur by mistake when a condition is incorrectly set.
Real-World Example:
A real-world example of an infinite loop can be found in the context of a simple command-line-based chatbot. In this scenario, the chatbot continuously interacts with users, responding to their inputs until the user decides to end the conversation.
How To Write An Infinite Loop In Python
In Python, you can create an infinite loop using a while loop with the condition set to True. Here's the process:
- Define the Loop Structure: An infinite while loop is a common way to create an endless loop in Python.
- Initiate the Loop: Use the while True: statement to start it. This means the loop will run indefinitely until a break condition is met.
while True: # Code to execute repeatedly
print("This loop will run forever")
-
Set Up Loop Conditions: You can include control statements like break or continue. For example, if a user input matches a specific value, you can use break to exit the loop.
while True:
user_input = input("Type 'exit' to stop the loop: ")
if user_input == "exit":
break # Exits the loop if user types 'exit'
-
Testing and Debugging: Testing and debugging are crucial. Use print statements to monitor variables inside the loop. This helps ensure that the infinite iteration behaves as expected.
counter = 0
while True:
counter += 1
print(f"Counter: {counter}") # Monitor counter value
if counter >= 5:
break # Exit the loop after 5 iterations
By following these steps, you can effectively create and manage infinite loops in Python.
When Are Infinite Loops In Python Necessary?
Python infinite loops are necessary or useful in several scenarios, particularly when you need to continuously perform an action without knowing ahead of time when the loop should stop. Here are some common situations:
-
Event-Driven Programs: Servers and GUI programs often rely on infinite loops to keep the system responsive, continuously waiting for events (e.g., a button click, incoming data, etc.). The loop runs until an external condition causes it to stop. For Example: A web server continuously listens for incoming requests.
while True:
client_socket, addr = server_socket.accept()
# Handle request
-
Monitoring and Polling: Infinite loops can be used to monitor a condition or poll a resource at regular intervals. For instance, checking the status of a system or service. For Example: Monitoring a file or process.
while True:
check_system_status()
time.sleep(5) # Pause for 5 seconds before checking again
-
Game Loops: Many games have a main loop that keeps running until the game ends. This loop continuously updates the game state, processes input, and renders graphics. For Example: A basic game loop.
while True:
process_player_input()
update_game_state()
render_graphics()
if game_over():
break
- Real-Time Systems: Systems that require real-time processing (e.g., embedded systems or IoT devices) often use infinite loops to handle continuous input or perform actions in real time.
- Daemon/Background Services: Many background processes or services run indefinitely until explicitly stopped, such as a logging service that collects logs from various sources.
- User Input until Exit Condition: If you're waiting for user input indefinitely (e.g., a command-line tool that keeps accepting commands until the user types "exit"), an infinite loop is often employed.
while True:
command = input("Enter a command: ")
if command == "exit":
break
Types Of Infinite Loops In Python
The infinite loops in Python can be categorized into different types based on the intent behind their design: fake infinite loops, intended infinite loops, and unintended infinite loops. Each type serves a unique purpose or may result from logical errors in the code.
Fake Infinite Loops In Python
Fake infinite loops appear to run forever. However, they contain a hidden termination condition. These loops are often used in testing environments. They simulate long-running processes without actual risks.
- For example, a developer can create a fake loop to mimic a server running indefinitely. This helps test how other parts of the system react under load.
- Fake infinite loops allow developers to observe behavior without crashing the program. They provide a safe space for experimentation.
- By using them, developers can ensure their code handles unexpected situations well. This method of testing is crucial for building reliable software.
Code Example:
Output:
Counter: 0
Counter: 1
Counter: 2
Counter: 3
Counter: 4
Exiting loop
Explanation:
In the above code example-
- We start by initializing a variable counter to 0.
- Next, we enter an infinite while loop using while True, which keeps running until we explicitly stop it.
- Inside the loop, we print the current value of counter using an f-string to format the output.
- After printing, we increment the counter by 1 with counter += 1.
- We then check if counter has reached 5. If it has, we print "Exiting loop" to signal that we're about to stop the loop.
- Finally, the break statement ends the loop, stopping further execution.
Intended Infinite Loops In Python
Intended infinite loops serve specific purposes. They are designed to run continuously until manually stopped. Many systems require this type of loop for constant operation.
- For instance, IoT devices often use intended infinite loops to stay ready for user input or data collection.
- These loops must have a clear exit strategy. Without one, they could lead to resource exhaustion or system crashes.
- A common practice is to monitor conditions within the loop. If certain criteria are met, the loop can safely terminate. This approach ensures that the system remains responsive and efficient.
Code Example:
Output:
Type 'exit' to stop the loop: hello
Type 'exit' to stop the loop: world
Type 'exit' to stop the loop: exit
Loop stopped.
Explanation:
In the above code example-
- We start an infinite loop using while True, which will continue running until we manually stop it.
- Inside the loop, we prompt the user for input by defining a function input() and asking them to type "exit" to stop the loop.
- The user's input is stored in the variable user_input.
- We then check if user_input is equal to "exit".
- If it is, we print "Loop stopped." to inform the user that the loop is ending.
- Finally, the break statement is used to exit the loop, stopping its execution.
Unintended Infinite Loops In Python
Unintended infinite loops occur due to logical errors in the code. These mistakes can stem from missing or incorrect loop control statements.
- For example, if a loop condition never becomes false, it will run forever.
- The impact of unintended infinite loops can be severe. They may cause programs to freeze or crash. This situation leads to frustrating experiences for users and developers alike.
- To prevent these issues, thorough code reviews and testing are essential. Developers should carefully examine their logic before finalizing their code.
Code Example:
Output:
Counter: 0
Counter: 0
Counter: 0
Counter: 0
...
Explanation:
In the above code example-
- First, we initialize the variable counter to 0.
- We enter a while loop with the condition counter < 5, meaning the loop will run as long as counter is less than 5.
- Inside the loop, we print the current value of counter using an f-string.
- However, we forgot to increment the counter, so it stays at 0, causing the condition counter < 5 to always be true.
- As a result, the loop continues indefinitely because the counter never changes.
Explore this amazing course and master all the key concepts of Python programming effortlessly!
Python Infinite Loop With Control Statements
Here's how different control statements like for, while, if, break, and continue interact with Python infinite loops:
Python Infinite Loop With While Statement
A while loop is commonly used to create an infinite loop by setting its condition to True. The loop will continue running until it encounters a break statement or an external stop.
Code Example:
Output:
This loop will run forever!
Explanation:
In the above code example-
- We start an infinite loop using while True, which is designed to run continuously.
- Inside the while loop, we print the message "This loop will run forever!" to indicate that the loop is active.
- Immediately after printing, we use the break statement to exit the loop.
- This means the loop will only run for one iteration before stopping, so even though it was set to run forever, it effectively ends after displaying the message once.
Python Infinite Loop With For Statement
The for loops aren't typically used for infinite loops, but they can be made infinite by looping over an endlessly repeating generator or sequence, like itertools.cycle.
Code Example:
Output:
Infinite loop with for!
Explanation:
In the above code example-
- We begin by importing the itertools module, which provides various functions that work on iterators.
- We use itertools.cycle([1]), which creates an iterator that will cycle through the list [1] infinitely.
- We then set up a for loop that iterates over this infinite iterator, but we use _ as the loop variable since we don't need to use its value.
- Inside the loop, we print the message "Infinite loop with for!" to indicate that the loop is executing.
- Immediately after printing, we include a break statement to exit the loop.
- This results in the loop running only once, even though it was set up to cycle infinitely, as it stops right after displaying the message.
Python Infinite Loop With If Statement
Integrating if statements within a while loop provides more control over execution. The if conditions inside infinite loops are useful for checking conditions dynamically and deciding whether to break or continue the loop.
Code Example:
Output:
Counter: 1
Counter: 2
Counter: 3
Breaking the loop
Explanation:
In the above code example-
- We start by initializing a variable counter to 0.
- We then enter an infinite loop using while True, which will run until we explicitly stop it.
- Inside the loop, we increment the counter by 1 with counter += 1.
- We then print the current value of counter using an f-string.
- Next, we check if counter has reached 3 using an if-statement. If it has, we print the message "Breaking the loop" to indicate that we're about to stop the loop.
- Finally, we use the break statement to exit the loop when counter equals 3, ending the loop's execution.
Python Infinite Loop With Break Statement
The break statement serves a crucial role in managing loops. It allows for premature termination of a loop when certain conditions are met. In infinite loops, break is essential to stopping the loop when a certain condition is met.
Code Example:
Output:
Type 'stop' to break the loop: hello
Type 'stop' to break the loop: test
Type 'stop' to break the loop: stop
Loop stopped.
Explanation:
In the above code example-
- We start an infinite loop using while True, which will continue running until we manually stop it.
- Inside the loop, we prompt the user for input with input() function, asking them to type "stop" to break the loop.
- We store the user's input in the variable user_input.
- We then check if user_input is equal to "stop".
- If it is, we print the message "Loop stopped." to inform the user that the loop is ending.
- Finally, we use the break statement to exit the loop, stopping its execution when the user types "stop".
Python Infinite Loop With Continue Statement
The continue statement helps refine loop logic by skipping the current iteration without stopping the entire loop. When executed, it jumps back to the beginning of the loop for the next cycle. In an infinite loop, it can be used to bypass certain code when specific conditions are met.
Code Example:
Output:
Odd Counter: 1
Odd Counter: 3
Odd Counter: 5
Explanation:
In the above code example-
- We start by initializing a variable counter to 0.
- Next, we enter an infinite loop using while True, which will run continuously until we stop it explicitly.
- Inside the loop, we increment the counter by 1 with counter += 1.
- We then check if counter is even by using the condition counter % 2 == 0. If this condition is true, we use the continue statement to skip the rest of the loop for that iteration.
- If counter is odd, we print the current value as "Odd Counter: {counter}".
- We then check if counter has reached 5. If it has, we use the break statement to exit the loop.
- As a result, the loop will only print odd values of counter (1, 3, 5) and will stop when counter equals 5.
Sharpen your coding skills with Unstop's 100-Day Coding Sprint and compete now for a top spot on the leaderboard!
Finite Vs Infinite Loops In Python
Python infinite loops and finite loops differ in structure and application. A finite loop runs a specific number of times. An infinite loop, on the other hand, continues until an external factor stops it. Here's a table highlighting the key differences between finite loops and infinite loops in Python:
Aspect | Finite Loop | Infinite Loop |
---|---|---|
Definition | A loop that runs a predefined number of times or until a specific condition is met. | A loop that runs indefinitely without a predefined endpoint unless externally interrupted. |
Exit Condition | Contains a clear exit condition that is eventually met. | May have no exit condition, or the condition is never met, causing continuous execution. |
Typical Use Cases | Iterating over a range, list, or collection; performing tasks that have a clear completion point. | Event-driven programs (servers, daemons), monitoring tasks, user-driven loops, games. |
Termination | Stops execution when the exit condition is fulfilled (e.g., reaching the end of a list or satisfying a counter condition). | Runs forever unless explicitly broken (e.g., with break) or interrupted externally (e.g., user input or system signal). |
Risk of Program Hang | Low, since the loop eventually terminates. | High if the loop is unintended or lacks proper handling; can lead to unresponsiveness or excessive resource use. |
Code Example | python for i in range(5): print(i) | python while True: print("Running...") |
Common Pitfalls | Logical errors in conditions may lead to early termination or failure to iterate correctly. | Logical errors may lead to unintended infinite loops, causing resource exhaustion or crashes. |
Performance Impact | Limited and predictable, based on the number of iterations. | May consume CPU, memory, or other resources continuously, impacting system performance. |
How To Avoid Python Infinite Loops?
Avoiding infinite loops in Python is crucial for ensuring your programs run efficiently and do not become unresponsive. Here are several strategies to prevent infinite loops:
- Set Clear Exit Conditions: Always ensure that your loop has a clear and achievable exit condition. For example, when using a while loop, make sure the condition will eventually evaluate to False.
- Use Loop Counters: If your loop is meant to run a specific number of times, use a counter to track iterations and break the loop when the counter reaches a defined limit.
- Implement break Statements: Use break statements to exit loops based on certain conditions being met. This is especially useful in while loops.
- Validate User Input: When using loops that depend on user input, validate the input to ensure it can lead to an exit condition. Avoid relying solely on user input that might not be controlled.
- Set a Maximum Iteration Limit: You can implement a maximum iteration limit in your loops, especially in cases where conditions might not be guaranteed to change.
- Use Debugging Techniques: Utilize debugging tools or print statements to monitor variable states within your loops. This can help identify if your loop is behaving as expected.
- Employ Unit Testing: Write unit tests for your functions that involve loops to ensure that they behave correctly under various conditions, preventing infinite loops from sneaking into your code.
- Review and Refactor Code: Regularly review and refactor your code to identify potential infinite loops, especially after making changes. Peer reviews can also be beneficial for catching logical errors.
By applying these strategies, you can significantly reduce the chances of encountering infinite loops in your Python programs, leading to more robust and reliable code.
Searching for someone to answer your programming-related queries? Find the perfect mentor here.
Conclusion
Python infinite loops are powerful constructs that can serve various purposes, from creating responsive applications to managing continuous processes. While they offer flexibility and control, it’s essential to use them with caution to avoid unintended consequences such as unresponsive programs and excessive resource consumption. By understanding how to create, manage, and exit infinite loops effectively, developers can leverage their capabilities in scenarios like event-driven programming and real-time systems.
Additionally, implementing strategies to prevent infinite loops—such as setting clear exit conditions and validating user input—can lead to more robust and efficient code. As with any programming concept, the key to harnessing the power of infinite loops lies in careful planning, testing, and debugging.
Frequently Asked Questions
Q. What is an infinite loop in Python?
An infinite loop in Python is a loop that continues to execute indefinitely without terminating. It occurs when the loop's exit condition is never met or when the loop is explicitly designed to run forever (e.g., using while True:). These loops can be intentional, such as for server processes, or unintentional due to logic errors.
Q. How can I create an infinite loop in Python?
You can create an infinite loop in Python using a while loop with the condition set to True. For example:
while True:
print("This loop will run forever!")
Alternatively, you can use a for loop with an infinitely repeating generator, such as itertools.cycle, although it's less common.
Q. When are infinite loops useful?
Infinite loops are useful in several scenarios, including:
- Event-driven programming: Waiting for user actions, such as in graphical user interfaces (GUIs) or servers that handle incoming requests.
- Monitoring systems: Continuously checking the status of a service or resource until a certain condition is met.
- Game loops: Running the main logic of a game, updating the state, and rendering graphics.
- Real-time systems: Embedded systems that require constant monitoring and control.
Q. How do I exit an infinite loop?
You can exit an infinite loop using the break statement. This allows you to terminate the loop based on a specific condition. For example:
while True:
user_input = input("Type 'exit' to stop the loop: ")
if user_input == "exit":
break # Exit the loop
Additionally, you can interrupt the program externally (e.g., by pressing Ctrl + C in the terminal) to stop an infinite loop.
Q. What are the risks of using infinite loops?
The risks of using infinite loops include:
- Resource exhaustion: They can consume CPU cycles and memory, leading to unresponsive applications or system crashes.
- Unintended behavior: Logic errors can result in loops that run longer than expected or indefinitely without a way to exit.
- Difficult debugging: Finding the source of an unintended infinite loop can be challenging, especially in complex codebases.
Q. Can you provide a practical example of an infinite loop?
Given below is a basic infinite loop examples that asks the user for input until they choose to exit:
In this example, the loop continues to prompt the user for input until they type 'quit', at which point the break statement is triggered, exiting the loop. This kind of infinite loop is commonly used in command-line applications and interactive scripts.
With this, we conclude our discussion on Python infinite loops. Here are a few other Python topics that you might be interested in reading:
- 12 Ways To Compare Strings In Python Explained (With Examples)
- Python Logical Operators, Short-Circuiting & More (With Examples)
- Python Bitwise Operators | Positive & Negative Numbers (+Examples)
- Python String.Replace() And 8 Other Ways Explained (+Examples)
- How To Reverse A String In Python? 10 Easy Ways With Examples!
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment