- What Is f-string In Python?
- Basic Usage Of f-string In Python Programming
- Advanced Operations With f-string In Python
- Format Specifiers & Escape Sequences With f-string In Python
- Calling Functions Inside f-string In Python
- Padding & Alignment With f-string In Python
- Common Mistakes When Using f-string In Python
- Best Practices For Using f-string In Python
- Comparing f-strings With Other String Formatting Methods In Python
- Advantages Of f-strings Over Other Formatting Methods
- Conclusion
- Frequently Asked Questions
f-string In Python | Syntax & Usage Explained (+Code Examples)
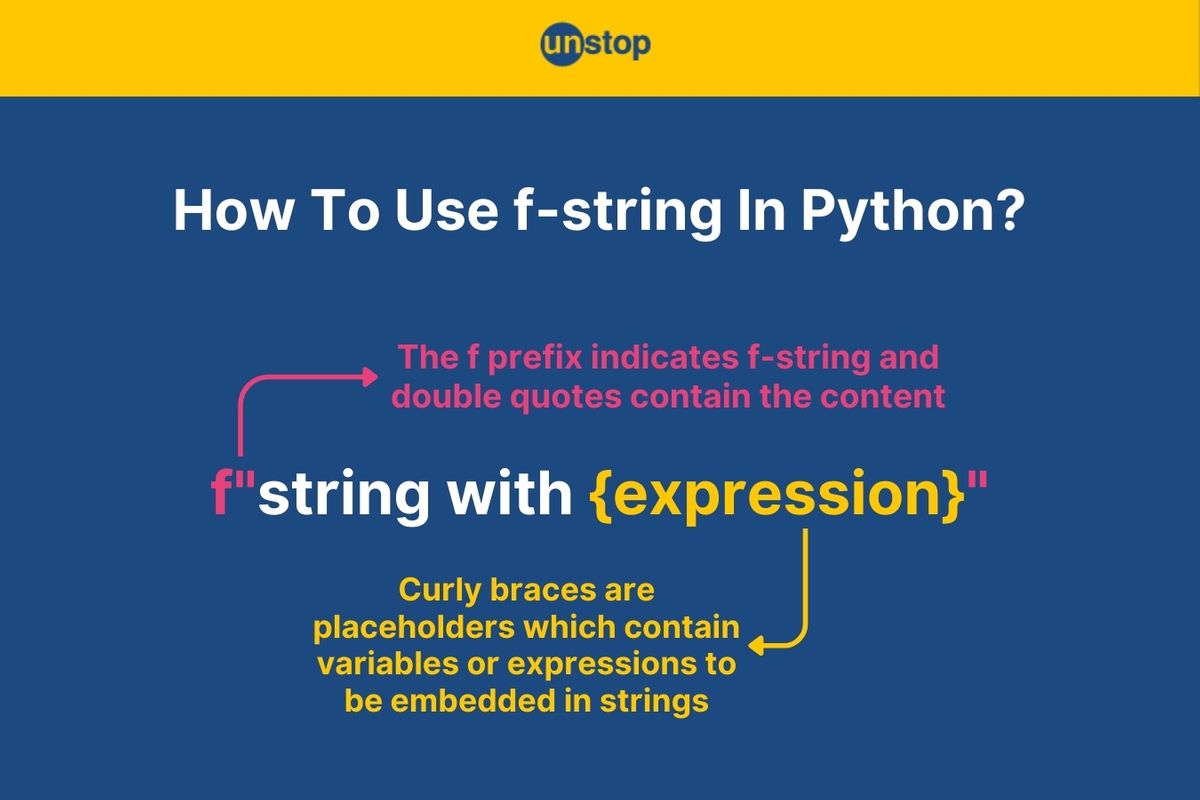
Imagine trying to assemble furniture with instructions written in riddles—confusing and inefficient, right? That’s how string formatting in Python used to feel before f-strings arrived. Introduced in Python 3.6, f-strings revolutionized the way developers handle string interpolation, making it both simpler and more intuitive.
In this article, we will discuss everything you need to know about f-strings in Python, from the basics to advanced applications. Whether you’re formatting text, embedding expressions, or simplifying your code, f-strings are about to become your new favorite Python feature.
What Is f-string In Python?
An f-string, short for formatted string literals, is one of Python’s most powerful and readable features for embedding expressions directly within string literals. Introduced in Python 3.6, it simplifies string formatting by eliminating the need for concatenation or methods like str.format() or percentage formatting. With f-strings, you can easily include variables or evaluate expressions in strings by prefixing them with f or F.
Syntax Of f-string In Python
f"string with {expression}"
Components Of f-string In Python
- Prefix: An f or F at the beginning of the string literal signifies that we are using the f-string technique to format the text information/ string.
- Single/ Double Quotes: These contain the actual content, wherein we can use other components to format what is printed to the console.
- Placeholders or Curly Braces {}: Variables or expressions that need to be embedded are enclosed in curly braces. They are hence referred to as placeholders and are placed wherever needed in between the text contained in quotes.
- Expressions: Any valid Python expression, such as variables, function calls, or mathematical operations, can be placed inside the braces.
Key Features Of f-string In Python
- Simplifies string interpolation: Directly embeds variables or expressions without additional syntax overhead.
- Supports inline evaluation: You can execute simple expressions like arithmetic operations directly within the string.
- Boosts readability and performance: f-strings are concise and faster than older methods like str.format() or string concatenation in Python.
Let’s look at a simple Python program example that illustrates the basic use of the f-string and its components.
Code Example:
#Creating variables
name = "Shivani"
age = 35
#Using f-string inside print() to format the output
print(f"My name is {name}, and I am {age} years old.")
Output:
My name is Shivani, and I am 35 years old.
Code Explanation:
In the simple Python code example:
- We begin by defining two variables, name and age, with the values Shivani and 35, respectively.
- Then, we use the f-string to display the value of the variables formatted with textual information.
- Inside, the f prefix marks the string as an f-string, and the placeholders contain the names of the variables whose values we want to insert into the text.
- Python evaluates the variables and replaces the placeholders with their respective values.
- Note that we use the f-string inside the print() function, which displays the info to the console.
Also read: Python Strings | Create, Format, Reassign & More (+Examples)
Basic Usage Of f-string In Python Programming
The f-strings offer a clean and intuitive way to format strings, making them ideal for embedding variables, formatting numbers, working with dates, and more. In this section, we will look at the most common use cases, showing how f-strings simplify text handling in Python programming.
Printing Variables With f-string In Python
You can use f-string in Python language to directly embed variables into a string, eliminating the need for concatenation or additional formatting methods.
Code Example:
#Creating variables with string values
city = "Delhi"
country = "India"
#Using f-string to present the information
print(f"I live in {city}, which is in {country}.")
Output:
I live in Delhi, which is in India.
Code Explanation:
In the basic Python program example:
- We create two string variables, city and country, with the values “Delhi” and “India,” respectively.
- Then, we embed these variables inside the f-string and use print to display the information directly.
- Alternatively, we would have to use string concatenation to embed the values. But the use of f-string simplifies the process and helps combine the text and the variable values seamlessly.
Formatting Numbers & Currencies Using f-string In Python
The f-strings support formatting numbers, allowing you to control precision, display percentages, or include currency symbols effortlessly.
Code Example:
#Creating variables with numerical values
price = 1299.99
discount = 15.5
#Using f-string with format specifiers to embed the values
print(f"The product costs â¹{price:.2f} after a discount of {discount:.1f}%.")
Output:
The product costs ₹1299.99 after a discount of 15.5%.
Code Explanation:
In the basic Python code example:
- Precision Control: The format specifier (:.2f) ensures the price is displayed with two decimal places.
- Percentage Display: {discount:.1f} formats the discount value with one decimal place.
- Currency Symbol: ₹ is added directly into the string for context.
Formatting Dates & Times Using f-string In Python
The f-strings work well with Python’s datetime module, allowing you to format dates and times for better readability.
Code Example:
#Importing the datetime module
from datetime import datetime
#Callind the now() method from datetime
now = datetime.now()
print(f"Today's date is {now:%d-%m-%Y}, and the time is {now:%H:%M:%S}.")
Output:
Today's date is 10-01-2025, and the time is 14:30:45.
Code Explanation:
In the Python program example:
- We begin by importing the datetime module from the Python libraries.
- Then, we call the now() method from the module to get the current date and time and store it in the now variable.
- Next, we use f-string inside the built-in Python function print() to display this information in proper format.
- Date Formatting: The format specifiers %d, %m, and %Y extract the day, month, and year, respectively.
- Time Formatting: %H, %M, and %S display the hour, minute, and second in 24-hour format.
Quotation Marks & f-strings In Python
The f-strings allow flexibility with single and double quotes, enabling seamless integration of quotes within the string.
Code Example:
#Creating two string variables
quote = "Life is what happens when you're busy making other plans."
author = "John Lennon"
#Using single quotes for f-string and double for a portion of text
print(f'"{quote}" - {author}')
Output:
"Life is what happens when you're busy making other plans." - John Lennon
Code Explanation:
In the example Python program:
- We create two string variables: quote and author.
- Then, we use f-string to print the text, where we format the first variable value with quotations.
- Here, we wrap the entire string in single quotes to accommodate double quotes inside.
- We embed the quote, and author variables are embedded without any additional concatenation.
Check out this amazing course to become the best version of the Python programmer you can be.
Advanced Operations With f-string In Python
The f-strings aren't just limited to embedding variables; they also support more advanced operations like evaluating expressions, nesting f-strings, and using format specifiers. In this section, we’ll explore these advanced features to show how powerful and flexible f-strings can be.
Interpolating/ Evaluating Expressions Within f-string In Python
The f-strings allow you to evaluate expressions, including mathematical operations or function calls, directly inside the curly braces. This makes it convenient to perform calculations or dynamically generate values in strings. With f-strings, you can embed simple expressions, perform arithmetic, or even call functions directly inside curly braces.
Code Example:
#Creating variables to store the dimensions of a rectangle
length = 15
breadth = 10
#Embedding the expression to calculate the area inside the f-string
print(f"The area of the rectangle is {length * breadth} square units.")
Output:
The area of the rectangle is 150 square units.
Code Explanation:
In the Python code example:
- We create two variables: length and breadth.
- Then, we use an expression inside the f-string placeholder {length * breadth} to calculate the area.
- It evaluates the product of the variables and dynamically inserts them into the string that we print to the console.
- This dynamic evaluation eliminates the need for intermediate steps or additional concatenation.
Nesting f-strings In Python
You can also nest f-strings inside other f-strings. This allows you to include evaluated expressions or formatted strings inside a larger f-string, providing an even more flexible way to manage string formatting.
Code Example:
name = "Shivani"
age = 35
#Nested f-strings
formatted_string = f"Hello, {f'Your name is {name} and age is {age}'}!"
print(formatted_string)
Output:
Hello, Your name is Shivani and age is 35!
Code Explanation:
In the example Python code:
- We begin by creating two variables, name and age, with the values “Shivani” and 35, respectively.
- Then, we use nested f-strings, where the f-string inside the main f-string is evaluated first, and the result is inserted into the outer f-string.
- This shows how you can evaluate smaller expressions within a larger string, adding layers of formatting or computation.
- We store the outcome of the nested string in formatted_string and then print it to the console.
Conditional Statements With f-string In Python
The f-strings in Python support conditional expressions directly within the placeholders, allowing dynamic string construction based on logic. This capability makes f-strings highly versatile when generating context-dependent outputs, such as personalized messages or dynamic responses.
Code Example:
#Creating a numerical value variable
score = 85
#Using if-else statement inside f-string
print(f"You {'passed' if score >= 50 else 'failed'} the test with a score of {score}.")
Output:
You passed the test with a score of 85.
Code Explanation:
In the Python program sample:
- We create a variable score and assign the value 85 to it.
- Then, we use the f-string and print() to display it with additional information.
- In that, we use the if-else conditional statement to embed the terms ‘failed’ or ‘passed’ depending on the individual's score.
- Inside the curly braces {}, the expression 'passed' if score >= 50. Else, 'failed' evaluates whether the score is greater than or equal to 50.
- If the condition is True, it inserts 'passed'; otherwise, it inserts 'failed'.
- The f-string combines the result of the conditional expression with the variable score to create a meaningful and dynamic string output.
Format Specifiers & Escape Sequences With f-string In Python
The f-strings support format specifiers to control how values are displayed, such as rounding numbers or adding commas for better readability. Escape sequences, on the other hand, allow you to include special characters like newlines or quotes within the string. Together, they make f-strings a versatile tool for generating clean and formatted outputs.
Using Format Specifiers With f-strings
Format specifiers define how a value should be displayed. They are added after a colon (:) inside the curly braces {}. Common use cases include:
- Decimal Precision: Control the number of digits after the decimal point.
- Thousand Separators: Add commas to separate large numbers.
- Scientific Notation: Represent numbers in exponential format.
Code Example:
#Printing floating-point value with f-string and format specifier
pi = 3.14159265359
print(f"Value of Ï: {pi:.3f}")
#Printing a large value with f-string and format specifier
population = 123456789
print(f"Population: {population:,}")
#Printing scientific notation with f-string and format specifier
distance = 1.496e11
print(f"Distance to the Sun: {distance:.2e} meters")
Output:
Value of π: 3.142
Population: 123,456,789
Distance to the Sun: 1.50e+11 meters
Code Explanation:
In the sample Python program:
- We begin by creating a floating point variable and then use f-string with print() to format and display it to the console.
- Here, we use the (:.3f) format specifier for decimal precision. It rounds the value of pi to 3 decimal places.
- Then, we create a variable to a large numerical value and then use the comma (:,) as a format specifier in f-string. This acts as a thousand separator, which formats the number with commas to separate thousands for better readability.
- Finally, we format a distance value using the (:.2e) specifier, which represents the distance value in scientific notation with 2 decimal places.
Using Escape Sequences With f-string In Python
Escape sequences are special characters prefixed by a backslash (\) that allow you to include formatting or special symbols in strings. Common escape sequences include:
- \n: Inserts a newline.
- \t: Inserts a tab space.
- \\: Prints a single backslash.
- \": Includes a double quote within a string.
Code Example:
#String variable
name = "Shivani"
#Escape sequences inside f-stirng to format text
print(f"Hello, \"{name}\"!\nWelcome to Python programming.")
Output:
Hello, "Shivani"!
Welcome to Python programming.
Code Explanation:
In the sample Python code:
- We create a string variable and insert it in an f-string to format along with other text.
- Here, we use the backslash with quotes (\"), which allows the double quotes to be displayed in the output.
- We also use the newline escape sequence (\n), which inserts a newline after the greeting.
Calling Functions Inside f-string In Python
The f-strings allow you to call functions directly within placeholders, making them a powerful tool for creating dynamic and efficient strings. This feature enables seamless integration of function outputs into your formatted strings, eliminating the need for intermediate variables or additional lines of code.
Code Example:
#Defining a function
def greet(name):
return f"Hello, {name}!"
#Creating a variable
name = "Shivani"
#Calling function inside the f-string, passing variable as argument
print(f"{greet(name)} Welcome to Python programming.")
Output:
Hello, Shivani! Welcome to Python programming.
Code Explanation:
In the Python code sample:
- We begin by defining a function named greet, which takes the variable name as an argument and returns a greeting string formatted using an f-string.
- Then, we create the variable name and assign the value “Shivani” to it.
- Next, we call the greet() function inside the f-string to embed the function’s return value.
- Inside the f-string in the print statement, the placeholder {greet(name)} calls the greet function with the argument name.
- The result of the function call ("Hello, Shivani!") is directly embedded into the string, along with additional text.
Looking for guidance? Find the perfect mentor from select experienced coding & software development experts here.
Padding & Alignment With f-string In Python
One of the standout features of f-strings is their ability to provide fine-grained control over the alignment and padding of strings and numbers.
- When dealing with formatted output, especially in scenarios like generating reports, creating tables, or ensuring consistent output for user-facing data, alignment and padding are essential.
- The f-strings allow you to specify exactly how the content should be positioned within a given width, and you can also pad with spaces or other characters for a cleaner, more organized appearance.
With Python’s f-strings, you can easily define the following:
- Alignment: Whether the content should be aligned to the left, right, or center.
- Padding: How much space should be added to the output, and which characters to use for padding.
- Width: The total number of characters (including padding) that should be allocated to the field.
This level of control makes f-strings particularly useful when working with structured data that needs to be displayed neatly.
Code Example:
#Creating two list
items = ["Apples", "Bananas", "Cherries"]
prices = [1.2, 0.5, 2.75]
#Using for loop with f-string to format the information in output
for item, price in zip(items, prices):
print(f"{item:<10} | {price:>6.2f}")
Output:
Apples | 1.20
Bananas | 0.50
Cherries | 2.75
Code Explanation:
- We begin by creating two lists, one containing 3 string values and another containing 3 numerical values.
- Then, we use a for loop to traverse the lists and zip() function to pair each item with its corresponding price.
- The loop then prints the pairs to the console, where we use f-string to format the output.
- Here, we ensure that each item name is left-aligned within the width of 10 characters, using the (:<10) notation. If the item name is shorter than 10 characters, spaces are added to the right.
- We also ensure that the prices are displayed as floating-point numbers with 2 decimal places using the .2f specifier.
- And they are right-aligned within 6 characters, padding with spaces on the left if necessary using the (:>6) notation.
Common Mistakes When Using f-string In Python
While f-strings provide an elegant way to format strings, they come with their own set of challenges. Common mistakes often stem from misunderstandings of syntax, improper handling of special characters, or misuse of expressions inside the placeholders. Being aware of these pitfalls can help you write cleaner and more reliable code.
Issues With Backslashes In Python’s f-strings
When using backslashes in f-strings, Python may interpret them as escape characters, which could lead to unexpected results, especially when trying to print a literal backslash or escape certain characters.
Code Example:
#Creating a string variable to contain a path to a folder
path = "C:\\Users\\Shivani\\Documents"
#Printing the path using f-string
print(f"The file is located at {path}")
Output:
The file is located at C:\Users\Shivani\Documents
Code Explanation:
- In f-strings, the backslash \ is interpreted as an escape character, which means it could be used to escape quotes or other special characters.
- If you need to include a literal backslash in the string, you should either escape the backslash itself (as \\) or use raw strings (r"string").
Issues With Brace Printing In f-strings
In f-strings, curly braces {} are used to enclose expressions. If you need to print literal curly braces, you must escape them by using double braces {{}}. Failing to do so will lead to syntax errors or unexpected behavior. For example:
print(f"This is a brace: {{}}")
#Output: This is a brace: {}
Here, we put curly braces inside placeholders (which are also curly braces) to output literal curly braces.
Precision Formatting Challenges With f-strings In Python
The f-strings allow specification of the precision for floating-point numbers, but requesting excessive precision can lead to unexpected or excessively long outputs. It’s essential to use precision that makes sense for the specific context.
Code Example:
#Creating a floating-point variable
value = 3.14159
#Printing the value using f-string for precision
print(f"Value: {value:.100f}")
Output:
Value: 3.1415900000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000
Code Explanation:
Here, although f-strings let you specify the number of decimal places (e.g., .100f), such large precision values can result in very long numbers with extra zeros, which may not be needed and could clutter the output. It's important to consider the meaningful precision of the data being displayed.
Inline Comments & Their Limitations With f-string In Python
In Python, inline comments are preceded by the hashtag symbol (#). However, when used inside f-strings, anything after # is treated as part of the string and not a comment. This can result in the unintended inclusion of comment text in the final output.
Code Example:
#Creating a variable
x = 10
#Printing using f-string for formatting
print(f"x is {x} # This is a comment")
Output:
x is 10 # This is a comment
Code Explanation:
Here, the comment symbol # in the f-string doesn't signify the start of a comment inside the string. Instead, it becomes part of the printed output. To prevent this, comments should be placed outside the f-string to avoid including them in the string's value.
Best Practices For Using f-string In Python
The f-strings provide a more readable and efficient way to format strings in Python. Following these best practices ensures clean, maintainable, and Pythonic code.
- Keep Expressions Simple Inside f-strings: Avoid embedding complex expressions within f-strings. This can make the code harder to read and maintain. Instead, evaluate expressions outside the f-string and insert the results into the string. Example:
result = (x + y) * 2
print(f"Result: {result}")
- Use f-strings for Readability, Not Performance: While f-strings are faster than other formatting methods, prioritize readability and clarity over performance gains, especially in cases where the performance difference is negligible. Good practice:
name = "Shivani"
print(f"My name is {name}")
- Avoid Mixing f-strings with Other Formatting Methods: To maintain consistency and readability, avoid mixing f-strings with other string formatting methods like str.format() or the %-formatting style within the same block of code.
- Handle Special Characters Properly: Ensure that special characters (e.g., backslashes or quotes) are properly escaped inside f-strings to avoid syntax errors or unexpected outputs. Example:
path = "C:\\Users\\Shivani"
print(f"File path: {path}")
- Prefer Using f-strings in Places Where Readability Matters: Use f-strings in places where string formatting enhances readability, such as in logging or string outputs that display variable values. They provide a cleaner, more intuitive syntax than older methods.
Comparing f-strings With Other String Formatting Methods In Python
Python offers several ways to format strings, and while f-strings are the most recent and efficient, understanding how they compare to older methods can help you make informed decisions on when to use each.
The f-strings vs. str.format() Method
The str.format() function in Python is an older, flexible way to format strings. Although it supports more advanced features like keyword arguments and reordering, f-strings are often preferred due to their simpler syntax and better performance.
Key Differences:
- Syntax: f-strings are more concise, while str.format() requires placeholders and additional syntax.
- Performance: f-strings are faster because they are evaluated at runtime, whereas str.format() involves method calls.
- Readability: f-strings improve readability by embedding expressions directly within strings.
Code Example:
name = "Shivani"
age = 30
# Using f-string
print(f"My name is {name} and I am {age} years old.")
# Using str.format()
print("My name is {} and I am {} years old.".format(name, age))
Here, while the output remains the same, the process of inserting values is much more seamless in f-strings. Use str.format() when you need more complex formatting, like reordering arguments or passing keyword arguments.
The f-strings vs. Percent Formatting (%)
Percent formatting, the oldest string formatting method, uses the % operator to format strings. It is still used in some codebases but is considered less readable and more error-prone compared to f-strings.
Key Differences:
- Syntax: Percent formatting uses placeholders like %s or %d, whereas f-strings are more intuitive with direct expressions inside curly braces.
- Performance: f-strings are generally faster, with less overhead than percent formatting.
- Readability: f-strings are clearer, especially when dealing with multiple variables.
Code Example:
name = "Shivani"
age = 30
# Using f-string
print(f"My name is {name} and I am {age} years old.")
# Using % formatting
print("My name is %s and I am %d years old." % (name, age))
Percent formatting can be useful for older code or when working with legacy systems, but it’s generally not recommended for new projects.
Advantages Of f-strings Over Other Formatting Methods
the f-strings offer a range of advantages that make them the preferred choice for string formatting in modern Python code. Here's a quick overview of their benefits compared to traditional string formatting methods.
- Simpler Syntax: f-strings are more concise and easier to read. Unlike str.format() or percent formatting, f-strings allow direct insertion of expressions inside curly braces, making the code cleaner and more intuitive.
- Better Performance: f-strings are evaluated at runtime and are typically faster than the older str.format() method and percent formatting. This performance boost comes from f-strings being parsed directly during execution rather than needing extra method calls or operations.
- Easier to Debug: Because f-strings allow you to embed expressions directly inside strings, debugging becomes more straightforward. You can quickly see the variable’s value in the output without needing to track down where the format method is applied.
- Supports Inline Expression Evaluation: With f-strings, you can include any valid Python expression (e.g., calculations, function calls) inside the string without needing extra logic or concatenation. This flexibility makes them a powerful tool for dynamic content generation. Example:
x = 5
y = 10
print(f"Sum of x and y is {x + y}.")
- No Need for Type Specifiers: Unlike percent formatting or str.format(), f-strings automatically determine the type of data to format, making them more user-friendly. This eliminates the need for explicit type specifiers like %d or {:.2f} in many cases.
- More Readable and Pythonic: f-strings enhance readability, especially when formatting multiple variables or complex expressions. Their clear syntax makes Python code more Pythonic and easier for developers to understand at a glance.
Ready to upskill your Python game? Dive deeper into actual problem statements and elevate your coding game with hands-on practice!
Conclusion
The f-string in Python is a versatile way of formatting textual information. It is useful for various scenarios ranging from basic usage to advanced operations and formatting features.
- Efficiency and Simplicity: f-strings are easier to read, write, and understand compared to older methods like str.format() or percent formatting.
- Improved Performance: Their runtime evaluation makes f-strings faster, leading to better overall performance in your code.
- Flexibility: With support for inline expressions, type specifiers, and dynamic content generation, f-strings allow you to create more flexible and concise string outputs.
- Best Practices: By following simple best practices, like keeping expressions simple and avoiding mixing formatting methods, you can use f-strings effectively in any Python project.
As Python continues to evolve, f-strings will remain a powerful tool for any developer looking to write clean, efficient, and readable code.
Frequently Asked Questions
Q1. What versions of Python support f-strings?
The f-strings were introduced in Python 3.6, so they are available in all versions from Python 3.6 onwards. If you’re using an older version of Python, you’ll need to use other string formatting methods like str.format() or percent formatting.
Q2. Can I use f-strings with complex expressions?
Yes, f-strings support any valid Python expression inside the curly braces. This means you can include mathematical operations, function calls, or even nested expressions directly in the string. Example:
x = 5
y = 10
print(f"The result is {x * y + 3}.")
Q3. Are f-strings more efficient than other string formatting methods?
Yes, f-strings are generally more efficient than str.format() and percent formatting because they are evaluated at runtime and avoid the overhead of method calls. This makes them a preferred choice when performance is a concern.
Q4. Can I format dates using f-strings?
Yes, you can format dates using f-strings. By calling the strftime() method on a datetime object, you can include the formatted date in your string. Example:
from datetime import datetime
today = datetime.today()
print(f"Today's date is {today:%B %d, %Y}.")
Q5. Is it possible to use f-strings with dictionaries and lists?
Absolutely! You can access dictionary values and list elements directly within f-strings. This allows you to format data from complex structures in a simple and readable way. Example:
data = {"name": "Shivani", "age": 30}
print(f"Name: {data['name']}, Age: {data['age']}")
Q6. Can I escape curly braces in f-strings?
Yes, you can escape curly braces by doubling them. This is useful when you need to display the literal curly braces in the string, rather than using them for expressions. Example:
print(f"{{This is inside curly braces}}") #Output: {This is inside curly braces}
Q7. Can I use f-strings in older Python versions (3.5 and below)?
No, f-strings are not supported in Python versions before 3.6. If you're using an older version, you'll need to rely on alternatives like str.format() or percent formatting.
This compiles our discussion on the f-string in Python. Here are a few more topics you must know:
- Python String.Replace() And 8 Other Ways Explained (+Examples)
- String Slicing In Python | Syntax, Usage & More (+Code Examples)
- 12 Ways To Compare Strings In Python Explained (With Examples)
- How To Reverse A String In Python? 10 Easy Ways With Examples
- Python input() Function (+Input Casting & Handling With Examples)
An economics graduate with a passion for storytelling, I thrive on crafting content that blends creativity with technical insight. At Unstop, I create in-depth, SEO-driven content that simplifies complex tech topics and covers a wide array of subjects, all designed to inform, engage, and inspire our readers. My goal is to empower others to truly #BeUnstoppable through content that resonates. When I’m not writing, you’ll find me immersed in art, food, or lost in a good book—constantly drawing inspiration from the world around me.
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
Comments
Add comment