Table of content:
- What Is zip() Function In Python?
- How Does Python zip() Function Work?
- Using Python zip() With Two Iterables
- Using Python zip() With Multiple Iterables
- Using Python zip() To Handle Unequal Lengths
- Using Python zip() To Handle No Or Single Argument
- Using Python zip() For Unzipping Data
- Practical Applications Of Python zip() Function
- Comparing zip() Function In Different Python Versions
- Conclusion
- Frequently Asked Questions
Python zip() Function | Syntax, Working, Uses & More (+Examples)
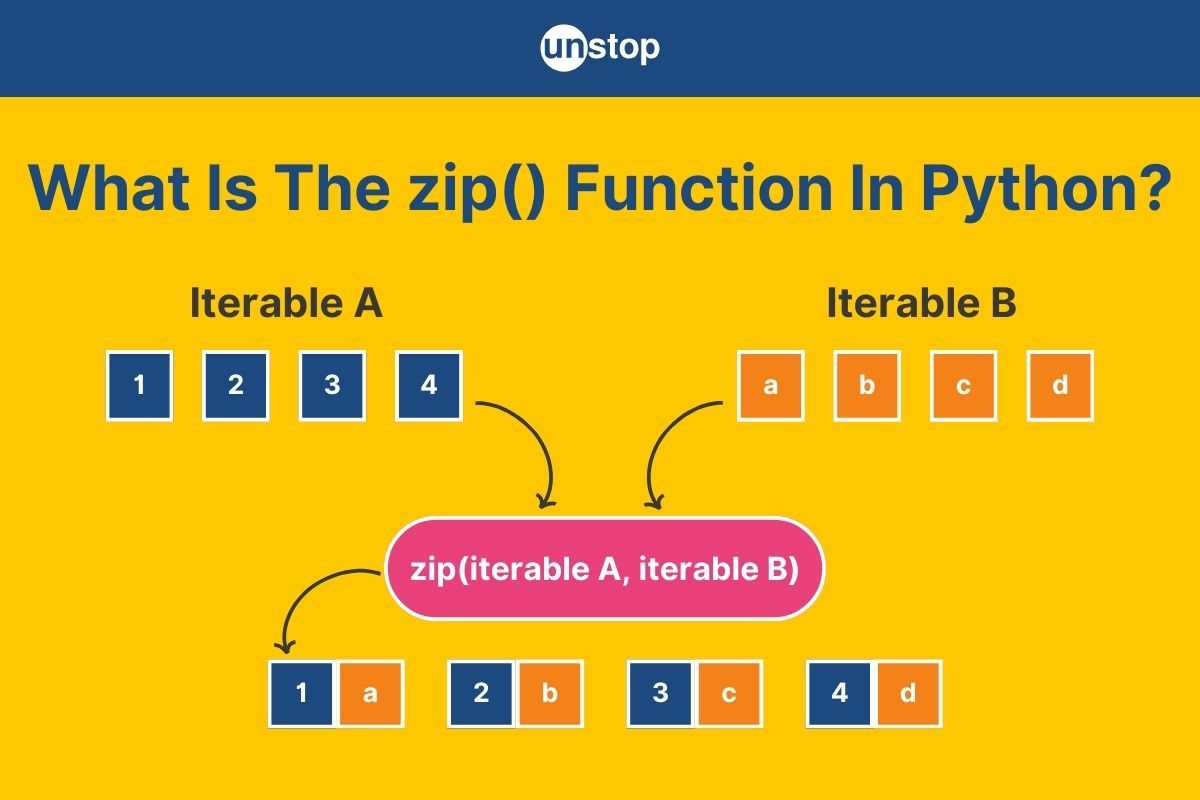
The zip() function in Python is a built-in tool that allows you to combine multiple iterables (like lists, tuples, or strings) into a single iterator of tuples. Each tuple contains elements from the provided iterables, paired by their corresponding positions. This function is particularly useful when you need to work with data from different sources or perform operations that involve parallel iteration over multiple collections.
In this article, we will explore the syntax and functionality of the zip() function, demonstrate how it can be applied in different scenarios, and show how to work with the results efficiently in Python programming.
What Is zip() Function In Python?
The zip() function in Python is a built-in function that allows you to pair elements from multiple iterables (such as lists, tuples, or strings) together into tuples, creating a new iterable of tuples. It is especially useful when you need to combine corresponding elements from different data structures or iterate over multiple sequences simultaneously.
Essentially, zip() takes two or more iterables as input and creates an iterator that aggregates elements based on their position in each iterable. The resulting iterator produces tuples, where the first element of each passed iterable is paired together, the second element with the second, and so on.
Syntax Of Python zip() Function
zip(*iterables)
Here:
-
*iterables: The zip() function accepts one or more iterable objects (like lists, tuples, etc.). The * operator allows passing multiple iterables as arguments.
Return Value Of Python zip() Function
The function returns an iterator of tuples, where each tuple contains elements from the input iterables at the same index. If the input iterables have different lengths, zip() stops creating tuples when the shortest iterable is exhausted.
Explore this amazing course and master all the key concepts of Python programming effortlessly!
How Does Python zip() Function Work?
Here's how the zip() function works in Python:
- Takes Multiple Iterables as Input: The zip() function can accept two or more iterables (e.g., lists, tuples, or strings). You can pass any iterable, and zip() will aggregate the corresponding elements together.
- Pairs Elements Based on Position: It combines the elements from the input iterables into tuples, pairing elements that are at the same index in each iterable. The first element of each iterable forms the first tuple, the second element forms the second tuple, and so on.
- Stops at the Shortest Iterable: If the input iterables have unequal lengths, zip() stops creating tuples when the shortest iterable is exhausted. This prevents creating incomplete tuples from the longer iterables.
- Returns an Iterator of Tuples: Python zip() returns an iterator, not a list. This iterator yields tuples that contain elements from the input iterables. You can convert this iterator into a list or other collection type to view the result.
- Can Handle Any Iterable: Python zip() works with any iterable, whether it’s a list, tuple, string, or other iterable objects. This makes it versatile for a wide range of use cases.
- Can Be Used with Unpacking: You can use the * operator to unpack iterables or elements from a zipped result, which allows for easy extraction of data.
Using Python zip() With Two Iterables
The zip() function in Python allows you to combine multiple iterables (such as lists, tuples, or strings) into a single iterator of tuples. Each tuple contains the corresponding elements from the input iterables, paired together by their position. Given below is an example of using zip() with two iterables: list1 and list2.
Code Example:
list1 = [1, 2, 3]
list2 = ['a', 'b', 'c']
result = zip(list1, list2)
print(list(result))
Output:
[(1, 'a'), (2, 'b'), (3, 'c')]
Explanation:
In the above code example-
- We start by defining two lists: list1 with integers [1, 2, 3] and list2 with characters ['a', 'b', 'c'].
- We then use the zip() function, which combines elements from both lists into pairs. The function creates an iterator where each item is a tuple containing one element from each list at the corresponding position.
- The result of zip(list1, list2) is an iterator, which we convert into a list using the list() function.
- Finally, we print the result, which outputs [(1, 'a'), (2, 'b'), (3, 'c')], showing the paired elements from both lists.
Using Python zip() With Multiple Iterables
We can also combine more than two iterables with zip() to create tuples containing elements from each iterable.
Code Example:
# Example with three lists
list1 = [1, 2, 3]
list2 = ['a', 'b', 'c']
list3 = ['x', 'y', 'z']
# Using zip() to pair elements from all three lists
zipped = zip(list1, list2, list3)
# Converting the zip object to a list
zipped_list = list(zipped)
print("Multiple iterables result:", zipped_list)
Output:
Multiple iterables result: [(1, 'a', 'x'), (2, 'b', 'y'), (3, 'c', 'z')]
Explanation:
In the above code exmaple-
- We first define three lists: list1 with integers [1, 2, 3], list2 with characters ['a', 'b', 'c'], and list3 with characters ['x', 'y', 'z'].
- We then use the zip() function to combine elements from all three lists. This function creates an iterator where each item is a tuple containing one element from each list at the same position.
- The result of zip(list1, list2, list3) is an iterator, so we convert it into a list using list() function.
- We then print the result to the console, showing the paired elements from all three lists.
Using Python zip() To Handle Unequal Lengths
When we use Python zip() with iterables of different lengths, it creates tuples only up to the length of the shortest iterable. Once the shortest iterable has no remaining elements, zip() stops pairing, leaving any extra elements in the longer iterable unused.
Code Example:
# Example with lists of unequal lengths
list1 = [1, 2, 3, 4]
list2 = ['a', 'b', 'c']
# Using zip() with unequal lengths
zipped = zip(list1, list2)
# Converting the zip object to a list
zipped_list = list(zipped)
print("Unequal lengths result:", zipped_list)
Output:
Unequal lengths result: [(1, 'a'), (2, 'b'), (3, 'c')]
Explanation:
In the above code example-
- We define two lists: list1 with integers [1, 2, 3, 4] and list2 with characters ['a', 'b', 'c'].
- Next, we use the zip() function to pair elements from both lists, even though they are of unequal lengths.
- The zip() function pairs elements only up to the length of the shorter list, so the extra element in list1 is ignored.
- We convert the zip object into a list with list() function.
- Finally, we print the result, to the console, showing the paired elements up to the third position.
Sharpen your coding skills with Unstop's 100-Day Coding Sprint and compete now for a top spot on the leaderboard!
Using Python zip() To Handle No Or Single Argument
The zip() function in Python can also handle cases where no arguments or only a single iterable is passed.
- No Arguments: If zip() is called with no arguments, it returns an empty iterator. Since there are no iterables to combine, the function doesn't create any tuples.
- Single Iterable: If zip() is called with a single iterable, it creates an iterator of single-element tuples.
Code Example:
# No Arguments
zipped_no_args = zip()
zipped_no_args_list = list(zipped_no_args)
print("No Arguments:", zipped_no_args_list)
# Single Iterable
zipped_single = zip([1, 2, 3])
zipped_single_list = list(zipped_single)
print("Single Iterable:", zipped_single_list)
Output:
No Arguments: []
Single Iterable: [(1,), (2,), (3,)]
Explanation:
In the above code example-
- In the first part, we call zip() function with no arguments, which results in an empty iterator.
- We convert this empty iterator into a list using list(), resulting in an empty list.
- We print the result, to the console, showing that no elements are paired because no iterables were provided.
- In the second part, we pass a single iterable, [1, 2, 3], to the zip() function.
- Since only one iterable is provided, zip() pairs each element with an implicit None value (the default for single iterable zipping).
- We convert the zip object into a list, which results in [(1,), (2,), (3,)], where each element of the original iterable is now a tuple with one element.
- Finally, we print the result, to the console, showing the individual elements from the list wrapped in tuples.
Using Python zip() For Unzipping Data
Unzipping data in Python with the zip() function involves separating a list of tuples or paired elements back into individual lists. By combining zip() with the unpacking operator *, you can easily "unzip" the original grouped data. This is particularly useful for splitting up paired data into separate lists to perform individual operations on each list independently.
Code Example:
# Original zipped data
zipped_data = [(1, 'a'), (2, 'b'), (3, 'c')]
# Unzipping into individual lists
numbers, letters = zip(*zipped_data)
print("Numbers:", numbers)
print("Letters:", letters)
Output:
Numbers: (1, 2, 3)
Letters: ('a', 'b', 'c')
Explanation:
In the above code example-
- We start with zipped_data, a list of tuples [(1, 'a'), (2, 'b'), (3, 'c')], which contains paired data.
- Next, we use the zip(*zipped_data) to "unzip" the data. The asterisk * is used to unpack the list of tuples, effectively separating the first elements from the second elements.
- The zip() function then returns two separate tuples: one for all the first elements (numbers) and another for all the second elements (letters).
- We assign these tuples to the variables numbers and letters, respectively.
- Finally, we print the results to the console, showing the unzipped data as two separate tuples.
Practical Applications Of Python zip() Function
Here are some practical applications of the zip() function in Python, demonstrating how it can be used in various scenarios:
-
Creating Dictionaries from Two Lists: Python zip() allows you to pair two lists — one as keys and the other as values — into a single dictionary, which is especially useful for organizing paired data. For Example-
keys = ['name', 'age', 'city']
values = ['Alice', 25, 'New York']
result = dict(zip(keys, values))
print(result)
-
Transposing a Matrix: You can use zip() to transpose a matrix, converting rows into columns and vice versa. This is helpful for matrix manipulation in data processing and mathematical operations. For Example-
matrix = [
[1, 2, 3],
[4, 5, 6],
[7, 8, 9]
]
transposed = list(zip(*matrix))
print(transposed)
-
Parallel Iteration Over Multiple Lists: Python zip() enables parallel iteration, allowing you to loop through two or more lists simultaneously by pairing their elements based on position, which is useful for aligned data processing. For Example-
names = ['Alia', 'Bhaskar', 'Charan']
scores = [85, 92, 78]
for name, score in zip(names, scores):
print(f"{name} scored {score}")
-
Element-wise Addition or Processing of Lists: Python zip() helps in performing element-wise operations, like addition or multiplication, across multiple lists, allowing you to combine or manipulate data directly. For Example-
list1 = [1, 2, 3]
list2 = [4, 5, 6]
sums = [a + b for a, b in zip(list1, list2)]
print(sums)
-
Aligning Different Length Lists with itertools.zip_longest(): With zip_longest(), you can handle lists of unequal lengths by filling missing elements, ensuring all data aligns even when lists don’t match in size. For Example-
from itertools import zip_longest
list1 = [1, 2, 3]
list2 = ['a', 'b']
result = list(zip_longest(list1, list2, fillvalue='N/A'))
print(result)
-
Creating Pairwise Combinations for Lists: Python zip() enables you to create paired combinations from two lists, often used in data analysis for matching features with values in a simple, readable way. For Example-
features = ['height', 'weight']
values = [170, 65]
pairs = zip(features, values)
for feature, value in pairs:
print(f"{feature}: {value}")
-
Zipping and Iterating with Indexes: Combine Python zip() with enumerate() to get both the index and the item from multiple iterables during iteration. This is useful for iterating over lists and needing the index as well. For Example-
items = ['apple', 'banana', 'cherry']
quantities = [10, 15, 7]
for index, (item, quantity) in enumerate(zip(items, quantities)):
print(f"Index {index}: {item} - {quantity}")
Comparing zip() Function In Different Python Versions
Given below is a table that compares the differences in how the zip() function works in Python 2 and Python 3:
Feature |
Python 2 |
Python 3 |
---|---|---|
Return Type |
Returns a list of tuples. |
Returns an iterator (must be converted to a list if needed). |
Handling Unequal Lengths |
Stops at the shortest iterable and discards excess elements. |
Same behavior, but itertools.zip_longest() can be used for padding. |
Memory Efficiency |
Less memory-efficient (stores all results in memory). |
More memory-efficient (uses iterators to generate values on-the-fly). |
Unpacking |
Can unpack results like a list. |
Can unpack results, but must convert to a list or iterate directly. |
itertools.zip_longest() |
No equivalent function. |
Introduced in Python 3, allows handling unequal lengths with a fill value. |
Searching for someone to answer your programming-related queries? Find the perfect mentor here.
Conclusion
The zip() function in Python is an incredibly useful tool for combining multiple iterables like lists, tuples, or strings into one, pairing elements from each iterable by their position. It simplifies tasks like aggregating data, iterating over multiple sequences simultaneously, and even handling unequal lengths of iterables gracefully.
With its ability to work efficiently, especially with large data sets, zip() not only makes your code more concise but also enhances readability and performance. It's widely used in real-world applications, such as data manipulation, merging lists, and even unzipping data. Understanding how to use zip() effectively can help you write cleaner, more efficient Python code in a variety of scenarios.
Frequently Asked Questions
Q. What is the purpose of the zip() function in Python?
The zip() function in Python is used to combine multiple iterables (such as lists, tuples, or strings) element-wise into a single iterable of tuples. Each tuple contains one element from each of the iterables, positioned at the same index. This is particularly useful for pairing data together, making it easier to iterate over multiple sequences simultaneously.
The zip() function stops creating tuples when the shortest iterable is exhausted, ensuring that the resulting output aligns with the length of the shortest iterable. It's commonly used for tasks like data alignment, aggregation, and comparison.
Q. Can I use zip() with lists and tuples?
Yes, you can use the zip() function with both lists and tuples in Python. Since zip() works with any iterable, it can combine elements from a list and a tuple together, creating pairs (or tuples) with corresponding elements from each. For example, if you have a list and a tuple of the same length, zip() will pair each element from the list with the corresponding element from the tuple, resulting in a new iterable of tuples. The zip() function is flexible and can be used with a combination of lists, tuples, or even other types of iterables.
Q. How does zip() handle different lengths of iterables?
When the iterables passed to the zip() function have different lengths, it stops creating tuples when the shortest iterable is exhausted. This means that any extra elements in the longer iterables are ignored.
As a result, the output consists of tuples with the same number of elements as the shortest iterable, ensuring that the function does not attempt to access elements that don't exist in the shorter iterables. If you want to handle unequal lengths more gracefully, you can use itertools.zip_longest(), which fills missing values from the shorter iterables with a specified value.
Q. Can I convert the result of zip() back to a list?
Yes, you can convert the result of the zip() function back to a list. Since zip() returns an iterator, you can use the list() function to convert it into a list of tuples. Each tuple will contain elements from the corresponding position of the input iterables. Here's an example:
list1 = [1, 2, 3]
list2 = ['a', 'b', 'c']
# Zipping the two lists
zipped_result = zip(list1, list2)
# Converting the result to a list
result_list = list(zipped_result)
print(result_list)
Output:
[(1, 'a'), (2, 'b'), (3, 'c')]
This allows you to work with the zipped data as a list, which can be helpful for further processing or manipulation.
Q. Is zip() available in all Python versions?
Yes, the zip() function is available in all Python versions starting from Python 2.x. However, there are some differences in behavior between Python 2 and Python 3:
- In Python 2: The zip() function returns a list of tuples, which contains all the pairs from the iterables.
- In Python 3: The zip() function returns an iterator that yields tuples one at a time, which is more memory efficient, especially when dealing with large datasets.
In both versions, zip() serves the same basic purpose—combining multiple iterables element-wise—but the returned data type differs, with Python 3 promoting an iterator-based approach to reduce memory usage. To convert the result of zip() into a list in Python 3, you can explicitly call list() on the result, just like in the examples shown earlier.
With this we conclude our discussion on the zip() function in Python. Here are a few other Python topics that you might be interested in reading:
- Fibonacci Series In Python & Nth Term | Generate & Print (+Codes)
- Python Program To Find LCM Of Two Numbers | 5 Methods With Examples
- Convert Int To String In Python | Learn 6 Methods With Examples
- 12 Ways To Compare Strings In Python Explained (With Examples)
- Flask vs Django: Understand Which Python Framework You Should Choose
I’m a Computer Science graduate with a knack for creative ventures. Through content at Unstop, I am trying to simplify complex tech concepts and make them fun. When I’m not decoding tech jargon, you’ll find me indulging in great food and then burning it out at the gym.
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
Comments
Add comment