Apple Interview Questions: 30+ Important Coding, Technical & HR Questions Answered
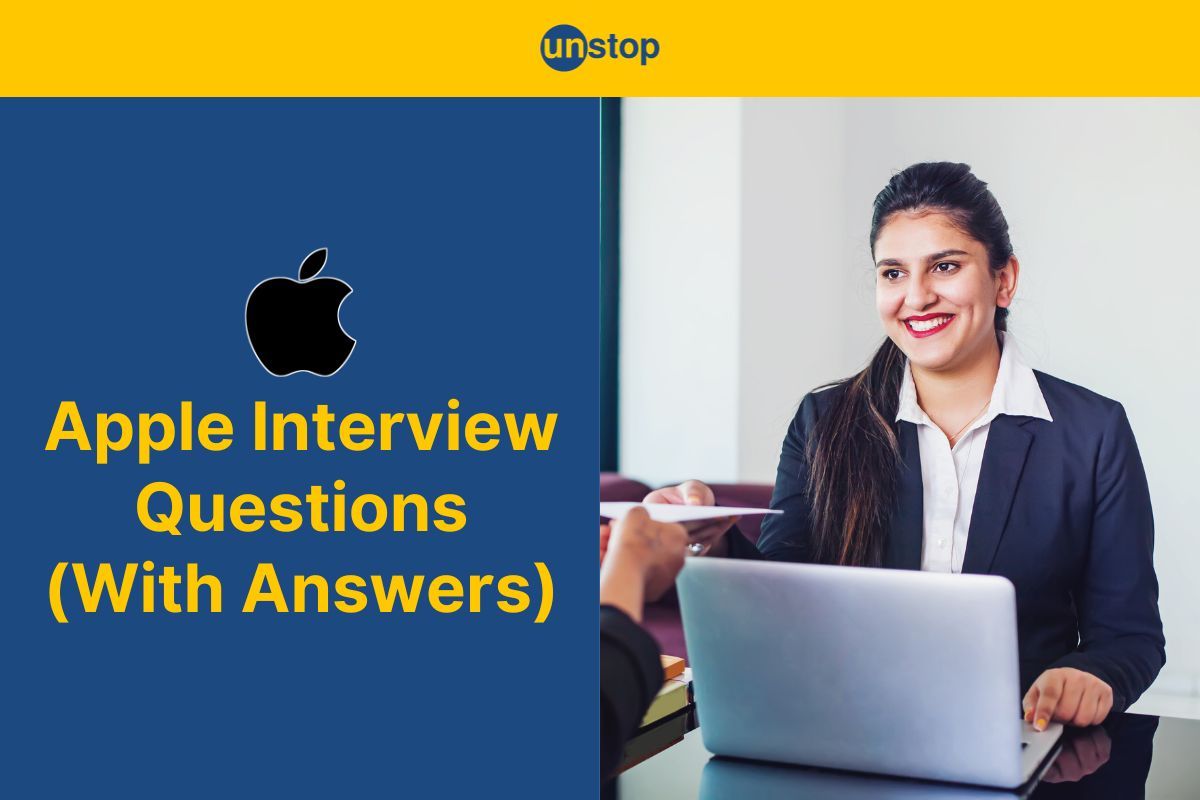
Table of content:
- The Apple Interview Process
- Apple Interview Questions: Technical & Coding Knowledge
- Apple Interview Questions: Quality Assurance
- Apple Interview Questions: Program Management
- Top Apple Interview Questions: Behavioral Skills
- Apple Interview Questions: Popular Topics
- Tips For Answering Apple Interview Questions
- Frequently Asked Questions (FAQs)
Apple is a leading tech company renowned for its innovation and global influence. It has earned its reputation as one of the top tech companies due to its groundbreaking products, such as the iPhone and Mac, and its commitment to user experience. Apple's cutting-edge technology, strong brand image, and vast resources make it an attractive choice for tech talent seeking to work in a dynamic and forward-thinking environment.
Image: Apple
With a rigorous hiring process and a history of attracting top talent, understanding the ins and outs of Apple's interview questions is essential. Apple interviews are known for their competitive nature, where candidates are tested on their technical knowledge, problem-solving abilities, and cultural fit. By familiarizing yourself with the interview process and knowing what to expect from Apple interview questions, you can increase your chances of success.
The Apple Interview Process
Stages of the Apple interview process:
The Apple interview process consists of several stages that candidates must navigate. These stages typically include a phone screening, technical interviews, and an onsite interview. Each stage serves a specific purpose in evaluating the candidate's skills and fit for the role.
Here’s a quick overview of the Apple Interview Stages (these are subject to change, depending on the role and candidates’ experience):
Stage | Rounds | Time | Purpose | Type of Questions/ Topics Covered |
Phone Screening | 2 | 30 min | Informal conversation with a recruiter and team lead to assess your interest in the company and role and possible company/culture fit |
|
Technical Interview | 1 | 45-60 min (30 min to code) | The hiring manager or team lead assesses technical skills through specific questions, coding challenges, etc. | Depends on the role. For example, for frontend engineers, Apple focuses on proficiency in HTML, CSS, JavaScript, and frameworks like React or Angular. Software engineers are asked about their experience with data structures and algorithms. |
On-Site Interview | Multiple rounds | An entire day | HR and team lead/ department heads will assess your soft skills and technical knowledge. You may face both, solo interviewers and panels. | Combination of questions on behavioral skills, domain knowledge, and coding. |
Take a look at the detailed Apple Recruitment Process here.
What are recruiters looking for in candidate answers?
When answering interview questions for Apple, recruiters are looking for candidates who demonstrate alignment with the company's values and possess the necessary skills to excel in their roles. They seek individuals who can think critically, adapt quickly to change, collaborate effectively with others, and have a passion for innovation.
Managing expectations during the lengthy process
The Apple interview process can be lengthy, often lasting several weeks or even months. Candidates need to manage their expectations throughout this period. They should stay proactive by following up with recruiters, maintaining open communication, and continuing their job search in case other opportunities arise.
Remember that patience is key when going through such a rigorous hiring process like Apple's. Stay focused on your goals, keep preparing diligently, and maintain a positive mindset throughout.
Read ahead to find common Apple interview questions that you may be asked, along with questions specific to roles.
Apple Interview Questions: Technical & Coding Knowledge
Apple interview questions are tailored to each role. Here’s a look at some of the most common questions that engineers (frontend and/or software, etc.) may face:
Q. How do you find the shortest path between two nodes in a graph?
Finding the shortest path between two nodes in a graph is a common problem in computer science and can be solved using various algorithms. The choice of algorithm depends on the type of graph (e.g., directed or undirected) and the specific requirements of the problem. Here is the most commonly used algorithm for finding the shortest path in a graph:
Dijkstra's Algorithm:
-
Dijkstra's algorithm is used to find the shortest path in a weighted graph (both directed and undirected graphs) with non-negative edge weights.
-
It maintains a set of visited nodes and a set of tentative distances from the source node to all other nodes. Initially, all distances are set to infinity except the source node (which is set to 0).
-
The algorithm iteratively selects the unvisited node with the smallest tentative distance, adds it to the set of visited nodes, and updates the distances to its neighbors if a shorter path is found.
-
The process continues until the destination node is reached or all nodes have been visited.
Pseudocode for Dijkstra's Algorithm:
function Dijkstra(graph, source, destination):
create a set of unvisited nodes
set the distance to source to 0, and all other distances to infinity
while the set of unvisited nodes is not empty:
select the unvisited node with the smallest distance
mark it as visited
for each neighbor of the current node:
calculate the tentative distance to the neighbor
if it's shorter than the current assigned distance, update it
return the shortest path from source to destination
Q. How can we design an algorithm for finding the maximum sum subarray?
The problem of finding the maximum sum subarray in an array can be efficiently solved using a well-known algorithm called Kadane's Algorithm. This algorithm has a time complexity of O(n), where n is the number of elements in the array. Here's how you can design an algorithm to find the maximum sum subarray:
Kadane's Algorithm:
-
Initialize two variables, max_so_far and max_ending_here, to negative infinity or a very small number, and a variable start to 0. These variables will keep track of the maximum subarray sum found so far, the maximum subarray sum ending at the current position, and the starting index of the maximum subarray.
-
Iterate through the array from left to right, and for each element arr[i]:
a. Update max_ending_here by taking the maximum of the current element or the sum of the current element and max_ending_here.
b. If max_ending_here becomes greater than max_so_far, update max_so_far to max_ending_here.
c. If max_ending_here becomes negative, reset max_ending_here to 0 and update the start variable to i + 1.
-
At the end of the loop, max_so_far will hold the maximum sum of the subarray, and you can find the starting and ending indices of the subarray by tracing back from the start variable and identifying where max_ending_here became 0.
HTML SNIPPET IS HERE
Q. What’s the process of checking whether a string is a palindrome?
To check whether a string is a palindrome, you need to determine if it reads the same forwards and backward, ignoring spaces, punctuation, and letter casing. Here's a common process to check for palindromes in a string:
Preprocess the String:
-
Remove any non-alphanumeric characters (such as spaces, punctuation, and special symbols) from the string.
-
Convert all characters to the same letter casing (usually lowercase) to ensure case-insensitive comparison.
Check for Palindrome:
Compare the modified string with its reverse. If they are the same, the original string is a palindrome.
Code:
def is_palindrome(s):
Preprocess the string: remove non-alphanumeric characters and convert to lowercase
s = ''.join(e for e in s if e.isalnum()).lower()
# Compare the string with its reverse
return s == s[::-1]
Example usage:
In this example, the function is_palindrome takes a string as input, preprocesses it by removing non-alphanumeric characters and converting it to lowercase, and then checks if the resulting string is equal to its reverse. If they are equal, the input string is a palindrome.
Q. What’s the process for implementing a function to find the longest common subsequence between two strings?
A subsequence is a sequence of characters that appears in the same order in both strings, but not necessarily consecutively. Here's a common process for implementing a function to find the LCS between two strings:
Dynamic Programming Approach:
-
Use a dynamic programming table to store the length of the LCS at each pair of positions in the two strings.
-
The dimensions of the table will be len(s1) + 1 by len(s2) + 1, where s1 and s2 are the input strings.
Fill in the Dynamic Programming Table:
Iterate through both strings character by character, comparing them:
-
If the characters match, increase the LCS length by 1 compared to the LCS length at the previous positions: dp[i][j] = dp[i-1][j-1] + 1.
-
If the characters don't match, take the maximum of the LCS lengths from the positions above and to the left: dp[i][j] = max(dp[i-1][j], dp[i][j-1]).
Retrieve the Longest Common Subsequence:
Starting from the bottom-right cell of the dynamic programming table (dp[len(s1)][len(s2)]), backtrack to find the characters that make up the LCS. Follow these rules:
-
If the characters match, move diagonally to the top-left cell and append the character to the LCS.
-
If the characters don't match, move to the cell with the greater value (i.e., either up or left).
Return the Longest Common Subsequence:
The LCS is stored in reverse order during the backtracking process, so you may need to reverse it before returning it as the final result.
Code:
Backtrack to find the LCS
Example usage:
Q. Designing an algorithm to efficiently find the top K elements from a large dataset.
To efficiently find the top K elements from a large dataset, you can use a data structure called a min-heap (or min-priority queue). The idea is to maintain a min-heap with a size of K, where the top of the heap contains the K smallest elements seen so far. As you iterate through the dataset, you compare each element with the smallest element in the heap. If the current element is larger, you replace the smallest element in the heap with the current element. This way, the heap always contains the top K elements. Here's an algorithm to achieve this:
-
Initialize an empty min-heap with a maximum size of K.
-
Iterate through the dataset, and for each element: a. If the size of the heap is less than K, add the element to the heap. b. If the size of the heap is already K, compare the element with the smallest element (the root) of the heap.
- If the current element is larger, remove the smallest element from the heap and add the current element.
- If the current element is smaller or equal, move to the next element in the dataset.
-
After processing all elements in the dataset, the min-heap will contain the top K elements.
-
To retrieve the top K elements in descending order (from largest to smallest), you can pop elements from the min-heap and store them in a list.
Q. Differentiate between swap and compare algorithms in Java.
Operation | Purpose | Example Usage |
---|---|---|
Swap | Swaps two elements in an array or collection. It is used to rearrange elements to bring the smaller or larger element to the correct position in sorting algorithms. | int temp = array[i]; array[i] = array[j]; array[j] = temp; |
Compare | Compares two elements to determine their order (e.g., less than, equal, or greater than). This comparison is used to establish the correct order of elements in sorting algorithms. | int result = element1.compareTo(element2); |
Here's a simple example in Java that demonstrates the use of both "swap" and "compare" operations in a sorting algorithm:
Swap two elements
Compare two elements
In this example, we first swap two elements in the array, and then we compare two elements to determine their order. Both operations are vital in sorting algorithms like bubble sort, quicksort, and merge sort, among others.
Q. Is it possible to have an empty catch block while programming in Java?
Yes, it is possible to have an empty catch block in Java. An empty catch block is a catch block that doesn't contain any code to handle exceptions. However, using empty catch blocks is generally considered bad practice and is discouraged.
Empty catch blocks effectively "swallow" exceptions, making it difficult to diagnose and debug issues in your code. It's important to handle exceptions appropriately by either logging the error, taking corrective action, or at the very least, providing some indication that an exception occurred.
For example, instead of an empty catch block:
try {
// Code that may throw an exception
} catch (Exception e) {
// Empty catch block
}
It is recommended to log the exception or take some action, like this:
try {
// Code that may throw an exception
} catch (Exception e) {
// Log the exception or handle it appropriately
e.printStackTrace(); // Logging the exception for debugging
}
Q. What is the purpose of a composting layer in CSS3?
In CSS3, a "compositing layer" refers to a graphical layer created by the browser's rendering engine to optimize the rendering of web pages. These layers are used to accelerate and improve the performance of web page rendering, animations, and transitions.
Compositing layers are created for specific parts of the web page, often triggered by certain CSS properties or HTML elements, such as elements with transform, opacity, or will-change properties. By isolating and rendering these layers separately, the browser can apply hardware acceleration, which results in smoother animations and more efficient rendering.
Some key benefits of compositing layers in CSS3 include reduced repaints and reflows, better utilization of the GPU (Graphics Processing Unit), and improved performance. Compositing layers can help minimize flickering and jank in animations, creating a more visually pleasing and responsive web experience.
Q. What do you know about Cocoa and Cocoa Touch?
Cocoa and Cocoa Touch are frameworks developed by Apple for building applications on macOS and iOS, respectively. Here's a comparison of these two frameworks in a table:
Aspect | Cocoa (macOS) | Cocoa Touch (iOS) |
---|---|---|
Platform | macOS (formerly known as OS X) | iOS (and other Apple mobile platforms) |
Primary Language | Objective-C and Swift | Objective-C and Swift |
User Interface | AppKit framework for GUI (NSView, NSWindow, etc.) | UIKit framework for GUI (UIView, UIViewController, etc.) |
Development Tools | Xcode, Interface Builder | Xcode, Interface Builder |
App Types | Desktop applications (macOS) | Mobile applications (iOS) |
App Distribution | Mac App Store, direct downloads, third-party distribution | App Store, ad-hoc distribution, Enterprise deployment |
Key Features | Extensive support for desktop app features such as windows, menus, and file handling | Optimized for touch-based interaction, with UI elements like gestures, navigation controllers, and view controllers |
Frameworks and APIs | Includes AppKit for desktop app development, Core Data, Core Animation, etc. | Includes UIKit for mobile app development, Core Data, Core Animation, etc. |
Design Paradigm | macOS apps follow a more traditional desktop application design paradigm | iOS apps follow a mobile-first design paradigm, optimized for small touch screens |
Screen Sizes | Supports various screen sizes and resolutions on Mac computers | Primarily designed for mobile devices with varying screen sizes |
User Interaction | Typically relies on mouse and keyboard input | Designed for touch input, including gestures and multi-touch |
File System Access | Full access to the macOS file system | Limited access to sandboxed file storage and iCloud |
Device Capabilities | macOS supports more diverse hardware peripherals | iOS is designed for mobile devices with constrained hardware |
Development Challenges | Adapting to various screen sizes and resolutions | Ensuring a consistent and responsive user experience on different iOS devices |
Mac Catalyst | Mac Catalyst allows porting iPad apps to macOS | N/A |
Both Cocoa and Cocoa Touch provide comprehensive frameworks for developing applications on Apple platforms, with their respective libraries, design paradigms, and tools. Developers can choose the framework that aligns with their target platform and device to create desktop or mobile applications for macOS and iOS.
Q. List the advantages of the JSON framework supported by iOS.
iOS (and macOS) provides native support for handling JSON data through the Foundation framework, which includes the JSONSerialization class. JSON (JavaScript Object Notation) is a widely used data interchange format, and the iOS platform offers several advantages for working with JSON data:
-
Native Support: iOS includes built-in support for JSON data, making it easy to parse and generate JSON without the need for third-party libraries.
-
Efficiency: The JSON parsing and serialization in iOS are highly efficient, which is important for mobile devices with limited resources.
-
Interoperability: JSON data can be easily converted to native Swift or Objective-C data structures, such as dictionaries and arrays, and vice versa, allowing seamless integration with your app's data model.
-
Standardized Format: JSON is a widely adopted and standardized data format, making it easy to exchange data between different platforms and services.
-
Readable and Lightweight: JSON is a human-readable format, which makes it easy to work with and debug. It's also a lightweight format, reducing the size of data payloads in network requests.
-
Support for Complex Structures: JSON supports nested structures, allowing you to represent complex data relationships and hierarchies straightforwardly.
-
Security: JSON is considered safer than other data formats like XML because it doesn't support executable code.
-
Easy Integration with Web Services: Many web services and APIs provide data in JSON format, making it a natural choice for iOS apps that communicate with external services.
-
Robust Validation: JSON data can be validated to ensure that it adheres to a specific schema, helping to catch errors in data formats early.
-
Compatibility: JSON is compatible with a wide range of programming languages and platforms, so you can easily exchange data between your iOS app and other systems.
-
Support for Codable: In Swift, you can leverage the Codable protocol to easily serialize and deserialize custom data types to and from JSON with minimal code.
-
Flexibility: JSON can represent a wide range of data types, including strings, numbers, arrays, and objects, making it suitable for various types of data.
Q. How to achieve concurrency in iOS?
Achieving concurrency in iOS allows you to perform multiple tasks simultaneously, improving your app's performance and responsiveness. iOS provides several mechanisms for concurrency. Here are some common ways to achieve concurrency in iOS:
1. Grand Central Dispatch (GCD):
- GCD is a high-level framework for concurrent code execution. It abstracts many low-level details and provides a simple and efficient way to perform tasks concurrently.
- You can use GCD to create and manage dispatch queues, which are used to schedule tasks for concurrent execution. GCD offers global queues and custom queues.
2. Operation Queue (NSOperationQueue):
Operation Queue is an Objective-C framework built on top of GCD. It provides additional control and features for managing concurrent tasks using NSOperation objects. You can create custom operations by subclassing NSOperation and adding them to operation queues.
3. Asynchronous Programming with Callbacks and Closures:
You can use asynchronous APIs, such as network requests or database queries, with callbacks and closures to perform tasks concurrently. Many iOS APIs, like URLSession for network requests, provide asynchronous interfaces that execute code on completion.
4. Concurrency with Threads:
You can use threads and the Thread class for concurrency, but this approach is less common in modern iOS development due to the complexity of managing threads.
Be cautious when working with threads to avoid issues like data races or deadlocks.
5. Combine and Async/Await (iOS 15 and later):
Apple introduced Combine for reactive programming and Async/Await for asynchronous programming in iOS 15. These new technologies simplify asynchronous and concurrent code. Combine enables you to work with asynchronous data streams, while Async/Await simplifies asynchronous tasks with a more synchronous-looking code style.
Q. Explain what a binary search tree (BST) is and how it differs from a binary tree. Can you describe the key properties of a BST?
A binary search tree (BST) is a type of binary tree with specific properties that distinguish it from a general binary tree. The key characteristics of a BST are:
-
Binary Tree Structure:
- Like a binary tree, a BST is a hierarchical data structure where each node has, at most, two child nodes: a left child and a right child. Nodes may contain data values, and they are connected in a parent-child relationship.
-
Ordering Property:
- The most significant property that distinguishes a BST from a regular binary tree is its ordering property. In a BST, for any given node:
- All nodes in the left subtree have values less than the node's value.
- All nodes in the right subtree have values greater than the node's value.
- This ordering principle allows for efficient searching, insertion, and deletion operations.
- The most significant property that distinguishes a BST from a regular binary tree is its ordering property. In a BST, for any given node:
-
Search Efficiency:
- Due to the ordering property, searching for a specific value in a BST is very efficient. You can traverse the tree by comparing the target value with the current node's value, which leads to a significant reduction in the number of comparisons compared to a regular binary tree.
-
Insertion and Deletion:
- Inserting a new node and deleting a node while maintaining the BST properties are straightforward operations. They typically involve finding the correct location in the tree and then adjusting the tree structure accordingly.
-
In-Order Traversal:
- An in-order traversal of a BST visits nodes in ascending order. This property is particularly useful for tasks like printing the elements in sorted order.
-
Balanced vs. Unbalanced BSTs:
- The balance of a BST can significantly impact its performance. A well-balanced BST has roughly equal numbers of nodes in the left and right subtrees, resulting in efficient operations. An unbalanced BST, on the other hand, can degrade to a linear data structure, causing worst-case performance.
Q. Could you please explain the concepts of INNER JOIN, LEFT JOIN, FULL JOIN, and FULL OUTER JOIN in SQL and their use in database queries?
In SQL, these are different types of JOIN operations used to combine data from two or more database tables. Here's an explanation of each:
-
INNER JOIN:
- An INNER JOIN returns only the rows that have matching values in both tables.
- It filters out rows where there is no match between the specified columns in the tables being joined.
- Example: SELECT employees.name, departments.department_name
FROM employees
INNER JOIN departments
ON employees.department_id = departments.department_id;
-
LEFT JOIN (or LEFT OUTER JOIN):
- A LEFT JOIN returns all the rows from the left table and the matched rows from the right table. If there are no matches in the right table, NULL values are returned for right table columns.
- It is useful when you want to retrieve all records from the left table and the matching records from the right table.
- Example: SELECT customers.customer_name, orders.order_date
FROM customers
LEFT JOIN orders
ON customers.customer_id = orders.customer_id;
-
FULL JOIN (or FULL OUTER JOIN):
- A FULL JOIN returns all rows from both the left and right tables. If there are no matches for a row in one of the tables, NULL values are used for the columns of the missing table.
- It is used to retrieve all records from both tables, regardless of whether they have matching values.
- Example: SELECT students.student_name, courses.course_name
FROM students
FULL JOIN courses
ON students.student_id = courses.student_id;
-
FULL OUTER JOIN (or FULL JOIN, which is often referred to as such):
- A FULL OUTER JOIN is similar to a FULL JOIN, but it ensures that there are no missing values in either table.
- It combines the functionality of both LEFT JOIN and RIGHT JOIN, returning all rows from both tables and filling in NULLs as needed.
- Example: SELECT employees.employee_name, departments.department_name
FROM employees
FULL OUTER JOIN departments
ON employees.department_id = departments.department_id;
Q. Is it possible to roll back changes after executing the ALTER command in a relational database?
In general, the ability to roll back changes after using the ALTER command in a relational database depends on several factors, including the specific database management system (DBMS) being used and the type of changes made. Here are some key points to consider:
-
Transaction Support: Most modern relational databases, such as PostgreSQL, MySQL, Oracle, and SQL Server, support transactions. Transactions provide the ability to group one or more database operations (including ALTER statements) into a single logical unit. If a transaction fails or is rolled back, any changes made, including ALTER operations, can be undone.
-
ALTER Types: The impact of an ALTER operation on rollback depends on the type of ALTER statement used. For example, some ALTER operations may involve non-reversible changes, like dropping a table or deleting data, which cannot be undone.
-
Autocommit Mode: In some DBMSs, certain types of ALTER statements may be executed immediately if the database is in the autocommit mode, meaning each statement is treated as a separate transaction. In such cases, rolling back may not be possible.
-
Data Backup: To mitigate the risk of data loss during an ALTER operation, it's a best practice to create a backup of the database before making significant changes. In the event of an irreparable mistake, you can restore the database from the backup.
-
DBMS-Specific Behavior: The behavior of ALTER statements and rollback capabilities can vary from one DBMS to another. Always refer to the documentation of your specific DBMS to understand how it handles ALTER operations and transactions.
Q. How can we differentiate between the Truncate and Drop commands?
Aspect | TRUNCATE Command | DROP Command |
---|---|---|
Purpose | Removes all rows from a table, leaving the table structure intact. | Deletes the entire table, including its structure. |
Effect on Table | Leaves the table structure (columns, constraints, indexes) intact. | Removes the table structure along with the data. |
Data Integrity | Can maintain foreign key constraints and triggers for data integrity. | Doesn't maintain foreign key constraints or triggers. |
Performance Impact | Generally faster for removing all rows in the table. | Can be slower, especially for large tables, due to removing the entire table structure. |
Transaction Behavior | Typically allows the operation to be rolled back within a transaction. | Irreversible within the same transaction; the table is gone. |
Usage Restrictions | Can be used on tables and partitioned tables. | Can be used on tables and other database objects like views, indexes, etc. |
Permissions | Requires DELETE privilege on the table. | Requires DROP privilege on the table. |
In summary, TRUNCATE removes all rows from a table while keeping the table structure intact and is often faster than DROP for large data removal. DROP, on the other hand, deletes the entire table, including its structure, and is irreversible within the same transaction.
Explore the differences between TRUNCATE, DROP and DELETE commands in detail.
Apple Interview Questions: Quality Assurance
Q. How do you prioritize and categorize bugs based on severity and impact?
Prioritizing and categorizing bugs based on severity and impact is a crucial part of the software development process. It helps ensure that the most critical issues are addressed promptly, providing a better user experience and a more stable product. Here's how I prioritize and categorize bugs based on severity and impact:
-
Assess the Severity: I begin by assessing the severity of each reported bug. Severity typically falls into categories such as Critical, Major, Moderate, Minor, and Trivial. Each category represents the impact of the bug on the application's functionality and usability.
-
Critical Bugs: Critical bugs are those that render the software unusable, potentially leading to data loss or posing a security risk. These are showstoppers and require immediate attention. They can include crashes, security vulnerabilities, and major data corruption issues.
-
Major Bugs: Major bugs are serious issues that significantly impede the application's functionality or usability. While they don't completely break the application, they have a substantial negative impact. These issues are also high-priority and need to be addressed urgently.
-
Moderate Bugs: Moderate bugs are less severe than major ones but still impact the user experience or core functionality. They may include issues like incorrect calculations, UI glitches, or performance bottlenecks. These are typically addressed in the next development cycle or release.
-
Minor Bugs: Minor bugs are nuisances but have minimal impact on the overall functionality or user experience. They might include cosmetic issues, typos, or minor usability annoyances. These are usually addressed in future releases or when higher-priority work allows.
-
Trivial Bugs: Trivial bugs are very low-priority issues that have minimal impact and can be easily ignored for a long time. They might include minor formatting issues or cosmetic problems that are unlikely to be fixed unless there is spare development capacity.
-
-
Consider User Feedback: User feedback is valuable for understanding the real-world impact of bugs. If a bug is reported frequently or has a significant negative impact on users, it may be escalated to priority.
-
Document and Communicate: I maintain clear documentation of each bug's severity, impact, and status. Effective communication within the development team is essential to ensure everyone is aligned on the prioritization.
-
Regularly Reevaluate: Prioritization is not static; it evolves as new bugs are reported and existing ones are fixed. Regularly reevaluating and reprioritizing the backlog is essential to ensure that the most critical issues are always addressed first.
-
Consider Project Goals and Deadlines: Project goals, milestones, and deadlines also influence bug prioritization. Sometimes, a critical bug may need to wait if it doesn't align with the current project phase.
Q. How would you test a Vending Machine?
Testing a vending machine involves a combination of functional and usability checks to ensure its proper functionality and user-friendliness. Functionality tests cover core aspects such as power management, accurate product dispensing, payment processing, and inventory control. Usability tests focus on the user experience, evaluating the clarity of the interface, the convenience of payment processes, error handling, accessibility, and more. Additionally, load testing is performed to assess its ability to handle high-traffic scenarios without issues. It's vital to consider environmental conditions, regulatory compliance, and the ease of maintenance and cleaning. Collecting user feedback and conducting regular regression tests for updates or changes are also essential steps in ensuring a reliable and efficient vending machine experience for both customers and operators.
Q. How do you deal with scalability issues?
Dealing with scalability issues can be challenging but is essential for the growth and success of any system or project. Here's how I approach scalability issues:
-
Understand the Problem: The first step is to thoroughly understand the scalability problem. Identify which aspects of the system are causing performance bottlenecks. Is it the database, the web servers, the network, or a combination of factors?
-
Plan for Growth: Anticipate scalability needs from the start. Scalability should be a fundamental consideration in the design and architecture of a system. It's easier to plan for growth upfront than to retrofit scalability later.
-
Optimize Code: Review and optimize the codebase. Look for areas where performance can be improved, such as inefficient algorithms, resource leaks, or unnecessary bottlenecks.
-
Leverage Caching: Implement caching mechanisms to reduce the load on the system. Caching frequently accessed data can significantly improve response times.
-
Database Scaling: If the database is a bottleneck, consider options like database sharding, replication, or using NoSQL databases, depending on your data access patterns and needs.
Q. In what scenarios or applications would you employ CRUD testing?
CRUD testing, which stands for Create, Read, Update, and Delete testing, is a set of functional tests performed on software applications, particularly database-driven systems. It's used to validate that the system can correctly perform these four fundamental operations on data. Here are some scenarios and applications where CRUD testing is essential:
-
Database-Driven Applications: CRUD testing is commonly used in applications that rely on databases to store, retrieve, update, and delete data. This includes web applications, content management systems (CMS), e-commerce platforms, and more.
-
Content Management Systems (CMS): CMS platforms, used for creating, managing, and delivering digital content, heavily rely on CRUD operations. Testing ensures that users can create, edit, publish, and delete content.
-
E-commerce Websites: In online shopping platforms, CRUD testing verifies that products can be added to the catalog, edited with new information, removed from the inventory, and purchased by customers.
-
Inventory Management Systems: Systems that handle inventory for retail, manufacturing, or warehousing depend on CRUD operations to add, update, and remove products or items.
-
Customer Relationship Management (CRM) Software: CRM software requires CRUD testing for managing customer records, interactions, and sales leads. It ensures that customer data is accurately stored and accessible.
-
User Account Management: In applications with user registration and authentication, CRUD testing confirms that user accounts can be created, updated, deactivated, or deleted.
Q. Can you explain what a traceability matrix is and its significance in the context of project management and software development?
A traceability matrix is a documentation tool used in project management, particularly in software development, to establish and track the relationships between different project elements. It provides a clear and structured way to link requirements, design components, test cases, and other artifacts, helping ensure that project objectives are met and that there is consistency throughout the project's lifecycle.
Key aspects of a traceability matrix:
-
Requirements Mapping: It allows you to link project requirements (functional, non-functional, and business requirements) to design specifications, test cases, and other work items. This helps ensure that all requirements are addressed in the project's various phases.
-
Bi-Directional Tracing: A traceability matrix offers bi-directional tracing, meaning that it not only tracks the relationship between requirements and downstream artifacts but also provides a way to trace back from the downstream artifacts to their originating requirements. This ensures that every project element has a clear source and purpose.
-
Change Impact Analysis: When requirements or design components change, the traceability matrix helps identify the potential impact on other project elements. It assists in managing change requests and assessing the consequences of modifications throughout the project.
-
Test Coverage: In software development, it is often used to link test cases to requirements. This ensures that test cases cover all specified requirements, helping to validate that the software functions as intended.
-
Quality Assurance: It enhances quality assurance by serving as a tool to verify that project elements are correctly implemented and tested. This, in turn, reduces the risk of defects and project failures.
-
Audit and Compliance: In regulated industries, such as healthcare and finance, traceability matrices are vital for audits and compliance. They provide a clear record of how requirements are met and validated.
Q. How do you ensure thoroughness when testing software applications?
When testing software applications, I ensure thoroughness by following a systematic approach. First, I carefully review the requirements and specifications to gain a clear understanding of the expected functionality. Then, I create detailed test cases that cover all possible scenarios and edge cases. During the testing process, I meticulously execute these test cases, paying close attention to the application's behavior and any unexpected outcomes. I also leverage automation tools to perform repetitive tests and ensure consistent results. Additionally, I document any issues or inconsistencies I encounter, providing clear steps to reproduce them. By being thorough in my testing approach, I can identify and address potential bugs or issues before the software is released.
Q. Can you provide an example of a time when your keen eye for detail helped identify a critical bug?
When I was testing a financial software application, I was verifying the accuracy of calculations for complex financial transactions. While reviewing the results, I noticed a discrepancy in the final amounts. Upon further investigation, I discovered that the application was not considering certain tax deductions correctly, leading to incorrect calculations. This bug had the potential to cause significant financial losses for users if left undetected. By meticulously examining the calculations and comparing them to the expected results, I was able to identify this critical bug and work with the development team to fix it before the software was released.
Some other QA questions that you can prepare for:
-
What testing techniques do you find most effective in identifying software defects?
-
How would you approach testing a complex feature or functionality within a tight deadline?
-
Can you describe an instance where your knowledge of different testing methodologies improved the overall quality of a product?
You may also face multiple questions on the bug life cycle in software testing. Study the topic in detail here.
Apple Interview Questions: Program Management
Program managers play a crucial role in product development at Apple. They collaborate with cross-functional teams, ensuring smooth coordination between engineering, design, marketing, and other departments. Recruiters look for candidates who can effectively communicate with various stakeholders and drive projects to successful completion.
Program management roles at Apple require a specific set of skills and qualities. Here are some Apple interview questions for a program management role:
Questions on Leadership Skills
1. How do you motivate your team to achieve their goals?
To inspire my team to reach their objectives, I believe in establishing clear and attainable goals. I make sure that each team member understands the significance of their role in accomplishing these goals and offer regular feedback and acknowledgment for their hard work. I also promote open communication and collaboration, allowing team members to share their ideas and contribute to the decision-making process.
2. Can you provide an example of a time when you had to resolve conflicts within your team?
In a previous role, I had to resolve conflicts within my team when there was a disagreement over the allocation of resources. I facilitated a meeting where each team member had the opportunity to express their concerns and perspectives. I actively listened to each person and encouraged them to find common ground. Through effective communication and compromise, we were able to reach a resolution that satisfied everyone involved.
3. Imagine you have a difficult team member or stakeholder. What’s your approach to dealing with them?
I believe in first understanding their perspectives and motivations. I try to have open and honest conversations to address any concerns or issues they may have. If necessary, I involve a neutral third party to mediate the situation. I also focus on finding common ground and working towards a mutually beneficial solution. Ultimately, I aim to create a positive and productive working relationship while ensuring that the team's objectives are met.
Questions on Project Management Expertise
1. Describe your approach to managing complex projects with tight deadlines.
When managing complex projects with tight deadlines, my approach is to first break down the project into smaller tasks that are more manageable. Next, I prioritize the tasks based on their relative importance before creating a detailed project plan and timeline. Additionally, I regularly communicate with stakeholders to provide updates and address any potential issues that may arise.
2. Can you share an example of how you managed a project from start to finish?
In my previous role, I successfully led a team in developing a new software application. I began by conducting a thorough analysis of the project requirements and creating a detailed project plan. I assigned tasks to team members based on their expertise and availability. Throughout the project, I closely monitored progress, provided guidance and support when needed, and ensured that the project stayed on track. By effectively managing resources, communicating with stakeholders, and addressing any challenges that arose, we were able to deliver the software application on time and within budget.
Questions on Problem-Solving Abilities
1. Tell us about a time when you encountered a major obstacle during a project and how you overcame it.
During a project, I encountered a major obstacle when our team faced a shortage of resources. To overcome this, we reassessed our priorities and reallocated available resources to focus on critical tasks. We also reached out to other teams for support and collaborated with them to find creative solutions. By being adaptable and resourceful, we completed the project on time.
2. How do you approach identifying and mitigating risks in a project?
I first conduct a thorough risk assessment to identify the impact of potential risks. Then, I prioritize the risks based on their severity and likelihood. To mitigate these risks, I develop contingency plans and establish preventive measures. Regular monitoring and communication with team members help in identifying any new risks that may arise during the project.
3. Give us an example of how you used data analysis to make informed decisions.
In a previous project, we were tasked with improving customer satisfaction. I analyzed customer feedback data to identify the key pain points. By examining trends and patterns in the data, I was able to pinpoint areas of improvement. Based on these insights, we implemented targeted changes in our processes and customer service, resulting in a significant increase in customer satisfaction ratings.
Top Apple Interview Questions: Behavioral Skills
During an interview with Apple, you can expect questions that assess your skills, experience, and fit within the company culture. Here are some common interview questions asked by Apple and detailed responses that can help you prepare:
1. Tell me about yourself.
When answering this question, focus on highlighting those experiences and skills that align with the role.
Sample Answer:
"I have a strong background in project management, having successfully led cross-functional teams in my previous role at XYZ Company. I am highly organized, detail-oriented, and thrive in fast-paced environments."
Find out how to best answer this interview question in this detailed guide.
2. Why do you want to work for Apple?
Show your enthusiasm for the company's products, values, and mission. Mention specific reasons why you admire Apple's innovation and how it aligns with your own career goals.
Sample Answer:
I have always been fascinated by Apple's innovative products. As an avid user of Apple products myself, I have experienced firsthand the seamless integration, user-friendly interface, and exceptional quality that Apple is known for. Working for Apple would allow me to contribute my skills and knowledge to a company I admire, while also providing me with the opportunity to grow in a dynamic environment. I believe that Apple's values align with my own, and I am confident that I would thrive in a culture that encourages creativity, collaboration, and excellence
3. Do you have a favourite Apple device? What is it, and why is it your favourite?
Through this question, interviewers are evaluating multiple things, from your interest in the company and role to your knowledge of Apple products, and even your ability to align your interest with your qualifications.
Sample Answer:
As an engineer, it's difficult for me to pick just one favorite Apple device because I appreciate the innovation and engineering that goes into all of their products. However, if I had to choose, I would say that my favorite Apple device is the iPhone. The iPhone has revolutionized the way we communicate and access information, and I am constantly impressed by its sleek design and powerful capabilities. From a technical standpoint, I admire the seamless integration of hardware and software in the iPhone, which allows for a smooth and intuitive user experience. Additionally, Apple's commitment to privacy and security is evident in the iPhone's robust encryption and privacy features, which is something I greatly value. Overall, the iPhone represents the epitome of Apple's dedication to excellence and innovation, and I would be thrilled to contribute to the development of such groundbreaking devices at Apple.
Important: This is one of the most common interview questions at Apple. Be sure to have an answer in mind for this interview question!
4. Describe a time when you faced a challenge at work.
Share a specific example of a professional challenge you encountered and explain how you overcame it using problem-solving skills or teamwork.
Sample Answer:
When I was leading a team on a tight deadline to develop a new software feature, we encountered a critical bug that was causing the feature to malfunction. It seemed like we would not be able to meet the deadline. However, instead of panicking, I gathered the team together to brainstorm possible solutions. We conducted thorough testing and analysis to identify the root cause of the bug. Through collaboration and open communication, we were able to come up with a workaround that fixed the issue and allowed us to deliver the feature on time. This experience taught me the importance of teamwork and problem-solving skills in overcoming challenges at work.
Apple Interview Questions: Popular Topics
During technical interviews at Apple, candidates can expect to face a range of coding questions that test their algorithmic knowledge, problem-solving abilities, and familiarity with data structures. Here are some common topics that you can prepare for:
-
Apple often poses questions related to algorithms, such as sorting and searching algorithms, dynamic programming problems, and graph traversal.
-
Candidates may also encounter questions involving data structures like arrays, linked lists, stacks, queues, trees, and hash tables.
-
Problem-solving skills are put to the test through logical puzzles or challenges that require a creative bend of mind.
-
Candidates should be well-prepared in fundamental computer science concepts such as time complexity analysis and space complexity analysis.
Cocoa and Cocoa Touch in iOS
Cocoa is a framework that primarily focuses on developing applications for macOS, while Cocoa Touch is specifically designed for iOS app development. Both frameworks provide a set of pre-built components, libraries, and tools that simplify the process of creating iOS applications. These frameworks provide a foundation for building applications on Apple devices like iPhones and iPads.
Having a solid understanding of Cocoa and Cocoa Touch is essential for candidates interviewing at Apple for iOS developer roles. It demonstrates their familiarity with the foundational frameworks used in Apple's ecosystem. Interviewers may ask questions about specific APIs or coding practices related to these frameworks to assess a candidate's proficiency level.
Key concepts and features within Cocoa and Cocoa Touch
Within the Cocoa framework, developers work with Objective-C or Swift programming languages to build macOS applications. On the other hand, within the Cocoa Touch framework, they use Swift to develop apps specifically for iOS devices.
Some key concepts within these frameworks include view controllers (managing user interface), model-view-controller architecture (separating application logic), delegates (handling events), gesture recognizers (user interactions), and many others.
Interested in working for tech giants like Apple, Google and Meta? Here are the programming languages you should know.
Candidates who can showcase their expertise in using these frameworks will have an advantage over others as it showcases their ability to create efficient, well-structured iOS applications.
Tips For Answering Apple Interview Questions
To effectively demonstrate your expertise during an interview with Apple, preparation is key. Candidates should research the company, familiarize themselves with common interview questions, and practice their answers. It's also essential to showcase relevant skills and experiences that align with Apple's values.
Here are some tips to keep in mind when preparing for Apple interview questions:
-
Highlight relevant experience: Share examples of projects or work experiences that showcase your technical skills.
-
Demonstrate teamwork: Discuss instances where you successfully collaborated with team members or managed cross-functional teams.
-
Showcase problem-solving abilities: Walk through how you approached a challenging problem or implemented an innovative solution.
-
Communicate effectively: Clearly articulate your thoughts and ideas during the interview process. Be concise and structure your responses using the STAR (Situation, Task, Action, and Result) framework to provide a clear and organized answer.
-
Research Apple products and Apple’s values: Familiarize yourself with Apple's products and services to show your enthusiasm and understanding of their ecosystem. Also, gain an understanding of Apple's core values and incorporate them into your answers, wherever possible.
-
Preparation tips to excel in technical coding interviews with Apple
By honing your algorithmic knowledge, and problem-solving abilities, practicing coding challenges, and seeking feedback from experienced developers or mentors you can increase your chances of excelling in interviews with Apple.
Note: Remember that the interview is also an opportunity for you to learn more about the company culture at Apple. Prepare thoughtful questions about the role's responsibilities, career growth opportunities, and the impact your role has on Apple’s product development.
Frequently Asked Questions (FAQs)
1. What is the best way to prepare for an interview at Apple?
To prepare for an interview at Apple, it is crucial to research the company thoroughly. Develop a thorough understanding of their products, services, culture, and recent developments. Practice answering common technical coding or QA questions that are often asked during interviews.
2. How can I demonstrate my passion for technology during an Apple interview?
One way to showcase your passion for technology during an Apple interview is by discussing personal projects or side endeavors related to technology. Highlight any relevant experience or achievements that demonstrate your enthusiasm and dedication in the field.
3. Do Apple interview questions include topics on behavioral skills?
Yes, behavioral questions are significant during an Apple interview as they allow recruiters to assess your soft skills. Simultaneously, recruiters use these questions to assess your cultural fit within the company. Be prepared to provide examples of how you have handled challenging situations or worked collaboratively in a team environment.
4. Is it necessary to have prior experience with macOS/iOS development for a software engineering role at Apple?
While prior experience with macOS/iOS development is advantageous, it may not be an absolute requirement for all software engineering roles at Apple. However, a strong foundation in programming languages and the ability to quickly learn and adapt are essential qualities that Apple looks for in candidates.
By preparing for these Apple interview questions, you can be one step closer to landing your dream job.
You might also be interested in the following:
- Best MySQL Interview Questions With Answers For Revision
- Top 35 Interview Questions For Freshers With Answers
- Top 101 Java Interview Questions And Answers That IT Companies Ask!
- 50+ MongoDB Interview Questions That Can Up Your Game
- Top 50 data structure interview questions and answers that tech giants ask!
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Blogs you need to hog!
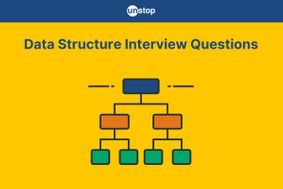
55+ Data Structure Interview Questions For 2024 (Detailed Answers)
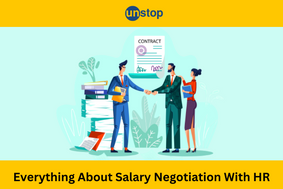
How To Negotiate Salary With HR: Tips And Insider Advice
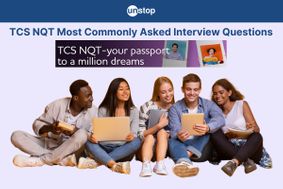
Best 80+ TCS NQT Most Commonly Asked Interview Questions for 2025
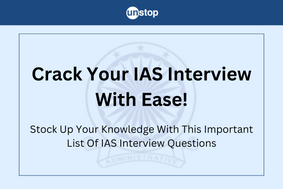
Comments
Add comment