HCL Coding Questions: Coding Pattern with Questions & Solutions
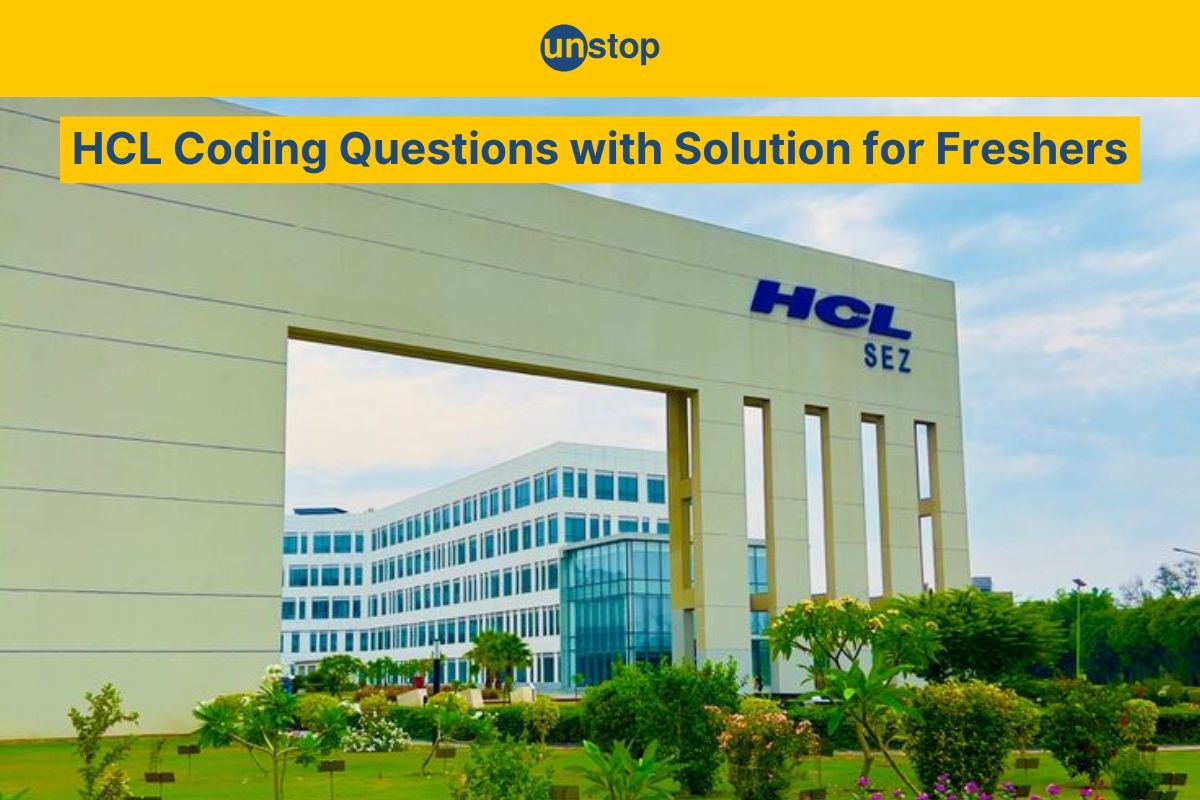
HCL coding questions in placement exams typically test fundamental programming knowledge, problem-solving skills, and the candidate's ability to write efficient and logical code. These questions are commonly part of the technical assessment phase and may be asked in an online test format.
Overview of HCL Coding Questions
Question Type | Description | Sample Question | Skills Assessed |
---|---|---|---|
Basic Programming | Tests knowledge of programming fundamentals such as variables, loops, and conditions. | Write a program to find the factorial of a number. | Control structures, loops, syntax |
Data Structures | Requires the use of data structures like arrays, lists, stacks, queues, and trees to solve problems. | Given an array, find the maximum difference between two elements. | Array manipulation, indexing |
String Manipulation | Involves working with strings, including operations like reversing, substring finding, and pattern matching. | Write a function to check if a string is a palindrome. | String handling, logic |
Sorting and Searching | Tests the ability to implement or apply sorting and searching algorithms for given datasets. | Implement a function to sort an array using the bubble sort algorithm. | Algorithm implementation |
Mathematical Problems | Tests the application of mathematical formulas, number theory, and logical math operations. | Find the GCD of two numbers. | Math operations, efficiency |
Dynamic Programming | Advanced questions involving optimization problems are commonly used in more challenging coding assessments. | Write a program to find the nth Fibonacci number using dynamic programming. | Recursion, efficiency, optimization |
Object-Oriented Concepts | Often includes questions about classes, inheritance, polymorphism, and encapsulation in OOP languages. | Create a class in Python that models a bank account with deposit and withdrawal methods. | Object-oriented design, encapsulation |
Common Languages for Coding Questions
-
Java and Python are often preferred for coding questions due to their simplicity in syntax and versatility.
-
C++ is also common for data structure-related questions, especially when dealing with memory management or efficiency.
Enhance your coding skills by practising coding questions across various levels. Click here to start your coding journey and master programming!
HCL Coding Interview Questions
HCL coding interviews typically focus on assessing the candidate's problem-solving approach, programming knowledge, and ability to optimize solutions. The interview questions often involve practical coding challenges along with discussions on algorithms and data structures. Here’s a breakdown of common question types and expectations:
Question Category | Description | Sample Question | Key Points in Answering |
---|---|---|---|
Algorithm Design | Design algorithms to solve specific problems efficiently. | Design an algorithm to merge two sorted arrays. | Focus on time complexity and optimization. |
Array and String Problems | Frequently used for assessing understanding of data indexing, manipulation, and storage. | Reverse an array in place without using extra space. | Provide clear steps and consider edge cases. |
Linked Lists | Tests knowledge of pointer-based data structures and traversal techniques. | Detect if a linked list has a cycle and remove it if found. | Explain concepts of pointers and traversal. |
Recursion and Backtracking | Used for solving complex problems that require trial and error, often in combinatorial contexts. | Write a recursive function to solve the N-Queens problem. | Explain base cases and recursive calls. |
Database-Related Coding | Tests SQL knowledge, especially for software development roles that involve database management. | Write an SQL query to retrieve the top 3 highest-paid employees. | Focus on efficient SQL joins and functions. |
Binary Trees and Graphs | Common for technical roles requiring an understanding of hierarchical or networked data structures. | Write a function to perform a breadth-first traversal on a binary tree. | Explain tree traversal techniques. |
Dynamic Programming | Tests optimization techniques using memoization or bottom-up approaches. | Solve the knapsack problem using dynamic programming. | Emphasize time complexity improvements. |
Detailed Breakdown of HCL Coding Interview Phases
Interview Phase | Description | Tips for Success |
---|---|---|
Online Coding Round | An online test where candidates solve programming questions in a limited time, typically via a coding platform. | Practice coding on platforms like HackerRank or LeetCode; focus on completing problems accurately within time. |
Technical Interview | In-person or virtual interviews where candidates are asked to solve coding problems on a whiteboard or shared screen. | Explain thought processes clearly, use efficient algorithms and write clean, readable code. |
Coding Challenge | Some roles involve live coding with a team or interviewer, emphasizing real-time problem-solving skills. | Stay calm, communicate clearly, and break down problems into smaller tasks for clarity. |
Examples of HCL Coding Interview Questions with Explanation
-
Problem: Write a program to find the second largest element in an array.
-
Explanation: Candidates should sort the array or maintain two variables to track the largest and second-largest elements, ensuring that the solution is efficient.
-
-
Problem: Implement a function to check if two strings are anagrams of each other.
-
Explanation: A good solution would involve sorting both strings and comparing them or using hash maps to count character frequencies.
-
-
Problem: Given a linked list, write a function to reverse it.
-
Explanation: Candidates should be familiar with pointer manipulation and understand iterative or recursive solutions for reversing linked lists.
-
-
Problem: Write a recursive function to calculate the factorial of a number.
-
Explanation: Demonstrates understanding of recursion with a base case and recursive call.
-
-
Problem: Create a SQL query to find employees earning more than the average salary in each department.
-
Explanation: Tests SQL skills, especially with aggregate functions and GROUP BY.
-
Coding Problem Statements with Solutions
Problem Statement 1
Rahul has an integer array called 'arr' of length N containing unique values. He wants to create a balanced tree where each parent node has smaller valued nodes on its left and larger valued nodes on its right. This balanced tree should ensure that the depth of the two subtrees for every node doesn't differ by more than one.
Your task is to assist him in creating this type of tree.
The output contains N lines denoting the pre-order traversal of nodes. If the left child of the node contains not null value then print the value else print a dot(.) , a similar process for the right child also. Each right child value is separated from the node by “->” sign and each left child by a left arrow sign
Input Format
First line contains an integer N representing the size of the array arr
The second line contains N unique space-separated integers representing the elements of the array arr
Output Format
The output contains N lines denoting the pre-order traversal of nodes. If the left child of the node contains not null value then print the value else print a dot(.) , a similar process for the right child also. Each right child value is separated from the node by “->” sign and each left child by a left arrow sign
Constraints
1<= N <= 10^5
1 <= arr[i] <= 10^9
Solution 1: Python
Solution 2: Java
Problem Statement 2
John works in a coin factory where each coin has an ID. He has an array 'nums' of size N. In the factory, coins are assigned to boxes based on the sum of the digits of their IDs. For example, if the ID of a coin is 321, the sum of its digits is 6 (since 3 + 2 + 1 = 6), so the coin will be placed in box 6.
John has been given a task to work with coins that have IDs in the range from the smallest number in 'nums', called the low-limit, to the largest number in 'nums', called the high-limit. For each number in this range (from low-limit to high-limit, inclusive), John needs to calculate the sum of the digits and place the coin in the corresponding box.
Help John determine which box contains the most coins and output the number of coins in that box.
Note: The factory has coins with IDs ranging from 0 to infinity, but John only needs to work with coins whose IDs lie between the low-limit and high-limit (inclusive).
Input Format
The first line contains an integer N, representing the number of elements in nums.
The second line contains n space-separated integers representing the elements of the array nums
Output Format
Print a single integer representing the maximum number of coins placed in any one box.
Constraints
1 <=N <=10^5
0 <= nums[i] <= 10^4
Solution 1: Python
Solution 2: Java
Problem Statement 3
Tom and Jerry are very naughty they always fight each other even for little things. This time they started fighting over a small cookie. The situation is such that there is a stream of numbers on a number line where there is a cookie present at the kth largest element in the array. Since Tom always gets the cookie from the master. Can you help jerry in getting the cookie as fast as possible?
Note: The kth largest element should be considered with repetition.
Input Format
The first line contains an integer that denotes the number of elements in the stream.
The second line contains K whose large value has the cookie.
The third line contains the stream of numbers
Output Format
The output contains a single integer which denotes the number at which cookie is there
Constraints
1 <= n <= 10^5
.-10^4 <= k, arr[i] <= 10^4
Testcase Input
30
5
10 10 1 0 1 1 1 9 10 7 2 6 8 7 7 1 5 1 4 5 6 4 6 5 7 8 2 7 9 2
Testcase Output
9
Solution 1: Java
Solution 2: Python
Solution 3: C++
Problem Statement 4
You are given two sorted arrays, a1 and a2, of size m and n, respectively. Now we combine the values of these two arrays to make a mega array. The resulting mega array is also sorted in nature.
Your task is to find the median of this mega array.
Input Format
Each Input has three lines
The first line contains 2 values m and n respectively.
The second line contains m numbers that signify nums1.
The third line contains n numbers that signify nums2.
Output Format
Return a Double data type number, which is the median of two arrays.
Constraints
nums1.length == m
nums2.length == n
0 <= m <= 10^6
0 <= n <= 10^6
Solution 1: Python
Solution 2: Java
Problem Statement 5
From your balcony, you can see N trees standing in a line numbered from 1 to N. The ith tree has height hi. Using your binoculars, you can only look at K trees at once in a single frame. A frame is defined by the index of the leftmost tree in it and contains exactly K trees. Starting from frame 1 (the leftmost frame), you move your binoculars towards the right until you reach frame N-K+1 (the rightmost possible frame). You want to find the height of the highest tree in each frame that is captured by your binoculars
Input Format
The first line of the input contains two integers N and K denoting the total number of trees and number of trees in a frame.
The second line contains N integers a1, a2, .., aN where ai represents the height of each tree.
Output Format
Print N-K+1 space-separated integers the height of the highest tree in each frame.
Constraints
1 <= N <= 10^9
1 <= K <= N
1 <= ai <= 10^9
Testcase Input
8 3
1 3 1 2 5 3 6 7
Testcase Output
3 3 5 5 6 7
Solution 1: C++
Solution 2: Python
Conclusion
HCL coding questions and interview questions are structured to assess technical expertise, problem-solving efficiency, and understanding of data structures and algorithms. Candidates can benefit from a structured preparation approach that focuses on common coding challenges, algorithm design, and optimization techniques.
Disclaimer: While we have gathered as much information from HCL's official website as possible, we have also included sources gathered from available online sources. Therefore, readers are advised to check and stay updated with the official website.
Frequently Asked Questions (FAQs)
1. What programming languages should I focus on for HCL coding questions?
HCL generally allows candidates to code in languages like Java, Python, and C++, as these are versatile and widely used in their technical assessments. It’s beneficial to be proficient in at least one of these, with a strong knowledge of data structures and algorithms.
2. What type of coding questions are typically asked in HCL’s online test?
The online coding test usually includes questions on data structures (like arrays, strings, and linked lists), algorithms (sorting, searching), and sometimes dynamic programming. Additionally, there may be questions on recursion, string manipulation, and basic database queries.
3. Are HCL coding questions timed, and how should I manage my time?
Yes, HCL coding assessments are timed, typically allowing candidates around 45-60 minutes to solve 2-3 coding problems. Time management is crucial, so it’s best to start with the easier problem, ensure accuracy, and allocate more time to complex questions while focusing on writing optimized code.
4. How can I prepare effectively for HCL coding questions?
Practice and focus on data structures, problem-solving, and algorithm-based questions. Reviewing HCL’s previous year coding questions and improving efficiency in coding will help in preparation.
5. What is the difficulty level of HCL coding questions, and is optimization important?
The difficulty level ranges from moderate to high, with some questions specifically testing algorithm optimization skills. Optimization is key, as it demonstrates the ability to solve problems within time and space constraints—especially for roles requiring high technical proficiency.
Suggested reads:
-
Deloitte Coding Questions: Top 5 Coding Questions for Freshers
-
TCS Digital Recruitment Process: Latest Updates & Tips for Freshers
-
HCL Logical Reasoning Questions and Answers: Top 5 Sample MCQs
-
HCL Aptitude Test: Top 5 Questions and Answers for Freshers (MCQs)
-
TCS Digital Aptitude Questions and Answers: Top 5 Sample MCQs
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Blogs you need to hog!
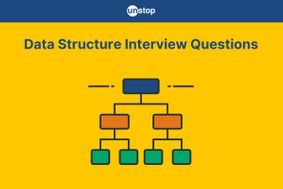
55+ Data Structure Interview Questions For 2024 (Detailed Answers)
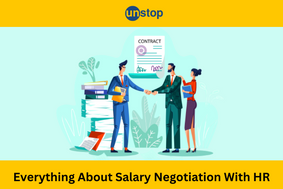
How To Negotiate Salary With HR: Tips And Insider Advice
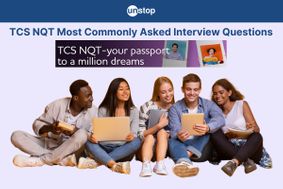
Best 80+ TCS NQT Most Commonly Asked Interview Questions for 2025
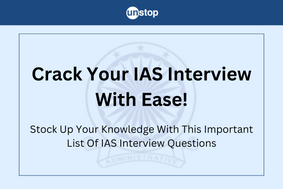
Comments
Add comment