Table of content:
- List of Hibernate Interview Questions (2023)
60+ Important Hibernate Interview Questions & Answers (2024)
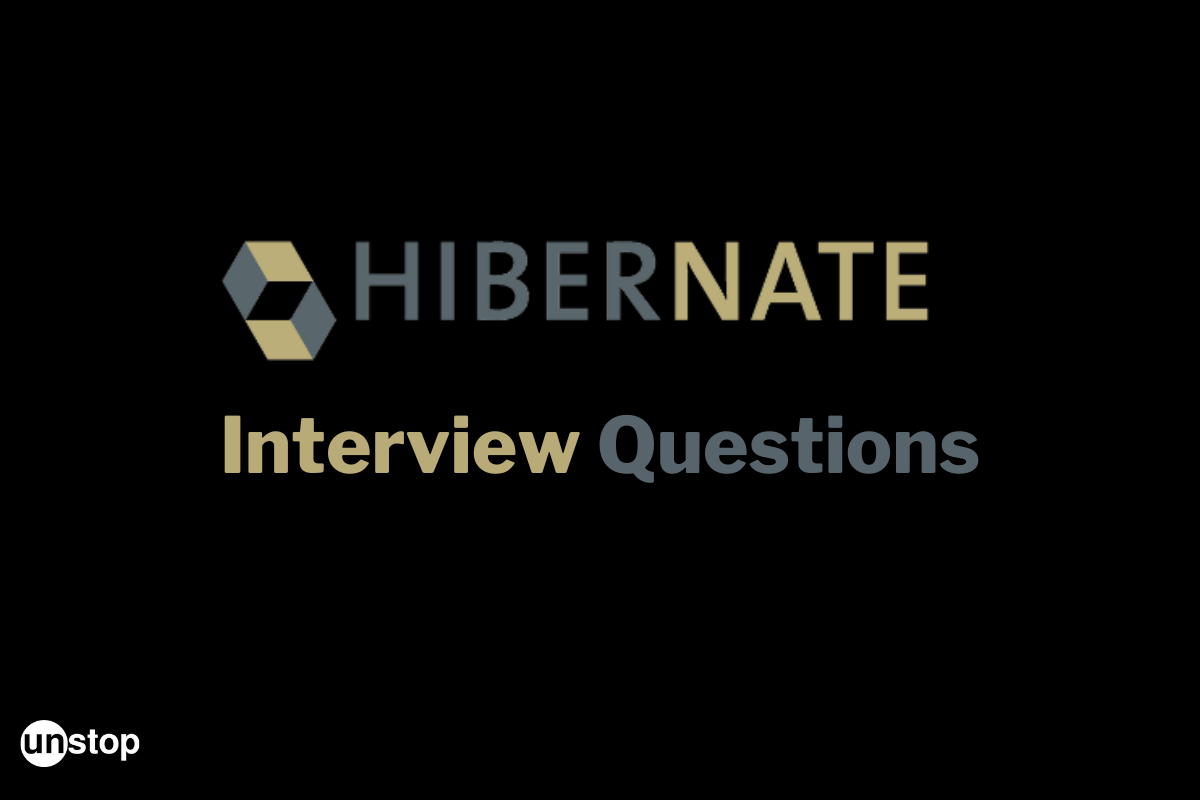
Hibernate is a Java-based ORM (Object Relational Mapping) framework that provides object-oriented data mapping from relational databases to Java objects. It allows developers to store data and access it in a database without needing to write SQL queries directly, thus making development quicker and easier.
Hibernate simplifies complex joins between tables by managing them internally, allowing developers more time for developing business logic instead of writing tedious join statements over multiple tables. With features like connection pooling, caching, and query optimization built into it, Hibernate makes accessing information faster than traditional JDBC methods used for querying databases.
Hibernate offers automatic object/relational mapping and provides more accessible and faster transaction management that is transparent to the developer. It manages transactions internally so developers can spend more time writing code for their applications instead of worrying about managing connections with the database. Hibernate supports Java Persistence API (JPA) annotations, making it easy to work with any legacy or new databases without having to write custom SQL queries.
Upskill yourself! Explore exciting courses
List of Hibernate Interview Questions (2024)
Q1. Explain ORM in Hibernate.
Object Relational Mapping (ORM) in Hibernate is a technique to map an application's object model with its relational database. The relational databases tables and columns are translated to the data objects in your application code. This makes it easier for developers, as they don't have to write extra code or complex SQL queries while dealing with different types of databases such as Oracle, MySQL or Postgres, etc.
Q2. What are the features of Hibernate?
1. Automatic Key Generation: Hibernate supports automatic key generation in tables with primary keys. It simplifies the code and eliminates the need to explicitly create fundamental values when inserting new records into a table.
2. Query Language (HQL): Hibernate implements its query language called HQL(Hibernate Query Language), which allows developers to write queries based on object-oriented concepts rather than relational database tables and columns. It thus makes it easier to maintain SQL statements as they are not tied directly with any specific database type or version of SQL being used by an application's backend system.
3. Lazy Loading: The lazy loading feature of Hibernate helps you load data from connected objects only when needed. This helps to improve overall performance significantly while reducing memory consumption at runtime in larger applications where there can be hundreds, if not thousands, of connections between different entities/objects within an application's data model structure.
4. Transaction Isolation Levels Support: It supports multiple transaction isolation levels such as read committed, repeatable read, and serializable through various connection settings, thus allowing developers greater control over how their transactions interact during concurrent accesses across database systems.
5. Caching System: Hibernate offers a caching system that can store data retrieved from the database to reduce subsequent trips for accessing duplicate records and thus improve overall application performance.
Q3. What are the advantages of Hibernate?
1. Improved Productivity: Hibernate reduces development time, increases productivity, eliminates the need to write complex SQL queries, and allows developers to focus more on business logic than data-related operations within their applications.
2. Database Independent Query: It supports independent database query, and you do not have to rewrite your query for different types of databases like Oracle, MySQL or Postgres, etc., which makes coding much simpler compared with traditional JDBC calls.
3. Transaction Management: It provides a transaction management system called JTA (Java Transaction API) that enables developers to create code with robust transactions across multiple resources in an application environment without worrying about maintaining the integrity of the individual resource processes themselves.
4. Automatic Table Creation: Hibernate can generate tables automatically when needed based on certain mapping information given by developer(s).
5. Caching System: Last but certainly not least, Hibernate offers a two-level caching system that helps reduce overhead in accessing duplicate records repeatedly and thus improving the overall performance of applications significantly.
Q4. Explain the architecture of Hibernate.
Hibernate architecture comprises three parts:
1. Java Application/Java code: This application layer interacts with the user interface, defines business logic, and uses Hibernate API to store and retrieve data from a database.
2. Database Layer: It stores and retrieves persistent objects in the database using SQL queries generated by Hibernate engine automatically based on mapping information given by developers.
3. Connection Provider: This part lies in between the above layers. Its job is to effectively manage connections between either layer, like connection pools, data sources, etc. This allows quick access for each without having to worry about creating them manually all the time while running various tasks against databases, such as retrieving records & writing entities back into storage systems.
Q5. Explain the framework of Hibernate.
The Hibernate framework consists of several components and layers which work in tandem to provide an efficient way for developers to create, maintain, and access databases.
1. Session Factory: It is a factory class used by application layer code to generate 'Session' objects that provide API methods for executing all the necessary database operations like inserting/updating records or querying them from tables etc.
2. Configuration: This component helps initialize Sessions Factory classes based on configuration information provided via text files (commonly known as cfg xmls) or using annotations present within POJO classes used by applications.
3. Transaction Manager: Its job is to handle transactions across different resources without compromising atomicity & performance while maintaining the overall integrity of their underlying processes.
4. Database Connectivity Layer: A JDBC Connection pool helps establish connections with DB servers quickly & effectively when needed while reducing overhead caused by performing those tasks manually each time such operation needs to be done while running your web/desktop applications.
5. ORM Mapping Layer: It is responsible for mapping the object-oriented concepts of an application codebase, such as classes, fields & methods with relational database tables & columns defined by said data sources like Oracle or MySQL. This helps reduce the time spent on writing native SQL queries during the development process.
Q6. What is the purpose of Hibernate Session Objects?
Hibernate Session Objects are used to manage the persistent objects within an application and allow for transactions to be executed both from inside the session and outside of it.
Q7. How can you make persistent objects using Hibernate?
Persistent objects can be created using Hibernate by mapping their fields to database table columns with annotations or XML files to store them on disk or in memory (depending on configuration).
Q8. What advantages come with leveraging a proxy object in Hibernate?
By leveraging proxy objects within Hibernate, one performs better due to its ability to initiate domain objects only when needed instead of initializing all domain classes at once. This will cause higher overhead in loading times and heavy use of resources like CPU cycles. Other features include shallow fetches, deep fetches-related entities, etc.
Q9. What role does the configuration file play in setting up and configuring for using Hibernate framework?
The configuration file plays a major role while setting up & configuring applications for usage through Hibernate framework since this defines properties such as setting connection URL/driver class name and dialects. Hence, the ORM layer is aware of the type of DB vendor should access during runtime time operations -- thus, allowing developers to define custom configurations easily without having to re-write huge amounts of codes each time.
Q10. Explain what Second-Level Cache means within the context of Hibernate.
The second-level cache is an ORM feature that provides a caching mechanism on top of the first-level cache. This allows users to store result sets or objects in RAM instead of querying every time from DB ( when using the same set of parameters), thus significantly enhancing the query performance and response times.
Q11. How does the query cache work with the first-level cache to make queries efficient through stored resultsets and objects in the second-level cache?
When it comes to making queries efficient, query cache makes use along with first level caching facility as it stores resultsets and objects that can be reused again for further requests while maintaining data consistency.
Q12. Can detached objects be used from outside session boundaries? If so, how?
Detached object instances can be used outside session boundaries provided this object has been serialized manually or through Hibernate facilities like the saveOrUpdate method.
Q13. What benefits do we obtain by enabling the first-level cache feature while developing applications with the Hibernate ORM layer enabled?
First-level caching helps improves the application's scalability since this cache commonly uses database queries & their associated result set/objects inside memory, thereby improving performance much faster than if these were loaded up from disk/network, consequently improving overall running speed. Also, leading to fewer sessions needed where possible, i.e., parallel operations, etc.
Q14. How are mapping files defined for defining relationships between tables/classes as well as column mappings to fields when dealing with databases via the Hibernate persistence facility?
Mapping files are XML-based documents defined within Hibernate framework, which developers use to define relationships between tables/classes and column mappings to fields when dealing with relational databases. Using this, developers can construct a bridge between entities and their properties in persistent storage.
Q15. What should users consider while creating/managing each mapping file, which includes describing entities & their associations ( one-to-one, many-to-many) inside a Hibernate Mapping File?
When creating/managing individual mapping files, i.e., Hibernate Mapping File, users must consider aspects like describing entities & their associations (one-to-one or many-to-many) as well as column mappings inside the document for ease of access per entity when building applications on top of them subsequently.
Q16. What is a concrete class in Hibernate?
A concrete class refers to an entity object that can be mapped with the associated table in the database. It contains all necessary setter/getter methods for its properties and any other business logic required by an application, such as validation.
Q17. How does the dirty checking feature work in Hibernate?
Dirty checking is a mechanism used in object-relational mapping (ORM) frameworks to track changes made to objects in memory and determine which changes need to be persisted back to the database.
When an object is initially loaded from the database, its state is recorded as the "first state." Any modifications made to the object are tracked, and the new state is compared to the first state, which is the "second state." This comparison helps identify which fields have changed.
If there are differences between the first and second states, it indicates that some fields have been modified. These changes need to be saved back to the database to keep the persistent representation of the object up to date.
By using dirty checking, ORM frameworks can optimize database interactions by only persisting the modified fields rather than persisting the entire object. This approach helps reduce the number of database queries and improves performance.
Q18. What are the uses of Hibernate configuration file?
The Hibernate configuration file, commonly referred to as "hibernate.cfg.xml" contains all the necessary settings and properties which are used for making a successful connection with the database and helps in defining how session objects will interact with the application or framework (e.g., fetch size, cache sizes, etc.).
It can also contain information such as the dialect used by your particular database engine and other custom parameters explicitly defined for your web application's needs.
Q19. What is a Factory Method in Hibernate?
Factory Method in Hibernate is a design pattern used to create objects without exposing the instantiation logic and increasing flexibility when programming for specific data types or classes.
Q20. Explain Core Interfaces.
The Core Interfaces of Hibernate are Session, Transaction, Query, Criteria Query, and Automapping.
Q21. How does an Atomic Unit work within Hibernate?
An atomic unit in Hibernation works by making sure that each operation within an atomic unit either succeeds ultimately or fails if it cannot be executed as a whole due to any system exception or other issue, such as deadlock conditions, etc., which could throw off database operations and cause damage to the transaction log file.
Q22. How do you implement Session Cache with Hibernate?
Implementing Session Cache with Hibernate can be done using two approaches:
- First, use second-level cache providers like Ehcache.
- Secondly, enable query caches through configuration settings in your ORM provider's properties file(s).
Both these techniques will help increase overall application performance by caching common query resultsets, thus reducing the redundant load on RDBMS systems.
Q23. What is a Persistent Entity in relation to hibernation tasks?
Persistent Entities refer to entities managed by a persistence mechanism like JPA/Hibernate, which have their state persisted into one or more tables within the database schema associated with the underlying ORM provider.
Q24. Describe the one-to-one association function within the context of hibernation applications.
One-to-One association links two entities in a relational model, where each entity is associated with the other but not necessarily dependent upon it for its existence. In Hibernation applications, this type of association can be set up using annotations like @ManyToOne and @JoinColumns or through XML-based mappings configured within the ORM provider's configuration files.
Q25. Can you explain the general architecture of a typical Hibernate application?
The general architecture of a typical Hibernate application consists of four main layers:
- Presentation layer (servlet/JSP)
- Business logic layer (POJO etc.)
- Persistence layer (ORM)
- Database access objects that interact directly with data sources like JDBC etc.,
All these components communicate via APIs exposed by ORM providers, thus creating an integrated environment required to perform CRUD operations against databases.
Q26. How will database transactions be managed using Hibernate tools and components?
Hibernate enables developers to manage database transactions programmatically and declaratively, allowing them complete control over when transactions begin and commit or rollback according to their needs without forcing any specific implementation constraints on assuring transaction isolation levels across distributed networks.
Q27. Explain one-to-many relationships in Hibernate.
An object of one class may have numerous related objects of another class, which is known as a one-to-many relationship in Hibernate. Because the linkages between various database table items are already defined, this form of interaction enables effective data storage and speedy retrieval.
Q28. How do you create setter methods in Hibernate?
Hibernate's setter methods are used to set values into specific fields within entity mappings before or after persisting them as part of a broader transaction context into a database table record.
These setter methods usually accept parameters for each field defined by the annotation mapping definitions, which specify how domain objects should be mapped into the tables' columns of SQL statements and databases, using syntax like @Column(name=").
Q29. What is an example of the transaction interface provided by Hibernate?
An example transaction interface provided by Hibernate would allow developers to control individual operations within atomic transactions, allowing them to accept or reject changes depending on whether specific criteria were met upon their execution, such as verification check constraints like existing unique keys.
Furthermore, this interface also allows for transactional isolation properties across multiple threads accessing the same data concurrently, ensuring safety and not corrupting the said records due to thread interference, amongst other design best practices intended for maintaining Entity integrity over time.
Q30. Can you describe what a session instance means in relation to using Hibernate?
A session instance represents a single Unit Of Work (now) and holds a state for the entity, being kept alive until it is explicitly closed or garbage collected.
It also serves as a gateway to persist entities into the database table utilizing session instance methods such as save(), delete(), etc., for object execution of DB operations against records in the mentioned context.
Q31. When to use child objects with objects handled by the ORM framework, such as hibernation, over native query statements?
Child objects should be used when constructing parent-child relationships between two distinct domain objects, which map directly to different tables with primitive references stored on either side, like Foreign Keys.
This way, data integrity can be ensured across multiple transactions where child row lifecycles are tied back to their originating record parent rows throughout application layer calls over native query statements that generally lack transactional safety properties enforced by an ORM framework/layer.
Q32. How does object mapping work when using Hibernation for relational databases?
Object mapping allows developers to define how Java classes map onto related SQL statement types based on annotated structure within declared Entity class definitions containing necessary field attributes required for operation such as @Column(name=" field_Name").
All defined entities will then reference each other accordingly when any CRUD actions are committed via Hibernation Session mapper instances, establishing a compact fluent interconnectivity scheme at runtime without relying on complex joins & nested conditions manually written by hand!
Q33. What is an entity class in Hibernate?
An entity class is a Java class that defines the mapping of data to objects, and these are used for accessing, persisting, and managing data between an object-oriented application and a relational database.
34. What is the role of the session interface in Hibernate?
The session interface provides methods for managing operations such as creating new instances, loading existing ones from databases, or updating them into persistent storage.
It also references second-level caches like query cache, etc., allowing access through one central point within applications that interact with multiple databases simultaneously.
Q35. What is a lightweight object in Hibernate?
Lightweight objects, also known as transient objects, represent a representation of the data that is not yet persistent or what some call non-persistent. When these lightweight objects are saved into the database, they turn from normal Java classes to fully managed entities by hibernating persistence context.
This conversion ensures that entity state transitions work in an expected way, ensuring newly created instances become 'persisted' once written out without further intervention required. In contrast, other existing ones continue living their life cycles according to new modifications applied to them.
Q36. How do you collect mapping in Hibernate?
Collection mappings within Hibernate involve either one-to-many associations or many-to-many associations, depending on the relationship between different entities involved with each other.
For example, when mapping two different tables where there's a need for collection configuring, this would occur between Person (entity class) and Account (another entity class). One Person can have multiple accounts, which requires configuration through @OneToMany annotation. In contrast, if both sides could own multiple entries, it should be marked with @ManyToMany annotation instead, indicating the association type accordingly.
Q37. What is a session factory in Hibernate?
A session factory is an object usually created while performing configuration of Hibernate, which provides the means to interact with persistent stores when it comes to handling data using plain Java objects.
These factories are able to provide sessions (connections) either from obtained database connections or by creating them on-demand, for instance, when the first access time takes place, requiring immediate connection establishment.
Q38. Explain the difference between many-to-many association and one-to-many association.
Many–to–many associations describe a relationship between two entities where each entity can be related to any number of entries present within the opposite side, thus allowing both sides ownership of certain records.
Still, at the same time, its contents cannot directly be linkable to each other unless given a foreign key column, linking part plays an essential role throughout the whole process execution stages, ultimately becoming what we call a 'joint table,' also referred to as 'bridge.'
Q39. What is a key column in Hibernate?
A key column refers to an attribute (column) placed within data model mapping against fields that store primary keys for given records.
These columns act as indicators letting database servers know whether specific values should become part of composite primary key definition when it comes to identifying distinct records from the group having the same name but different content variations.
Its primary role consists of bringing uniqueness whenever many-to-many mappings take place, requiring unique identifiers across all sides being affected throughout operation stages accordingly.
Q40. Can you explain how Hibernate proxy works?
Hibernate Proxy enables a lazy loading feature by wrapping objects while working through various communications layers representing persistent storage layer, meaning no information will get loaded before accessing specific attributes defined through the interface structure.
Once the proxy grabs hold of the target object, it loads all its managed properties. This kind of feature makes code more concise while reducing memory outages due to a large number of records that can be retrieved without blocking main thread execution stages after one time initialization phase.
Q41. How do you map objects to database tables in Hibernate?
Hibernate maps objects to database tables via object-relational mapping (ORM). Java classes that represent database entities are created and then annotated with ORM annotations corresponding to the table names, columns, constraints, or other pertinent elements. The SQL instructions generated by these classes are then used as templates by Hibernate's query language (HQL) for saving or retrieving data from a database. Additionally, Hibernate offers tools for automatically creating mappings from pre-existing database schemas.
Q42. What is the purpose of Java Database Connectivity (JDBC)?
The purpose of Java Database Connectivity (JDBC) is to provide a standard interface that enables the development of database-independent applications in Java. It provides standardized APIs for interacting with databases, creating and executing SQL statements, retrieving query results, and handling errors and transactions. With JDBC, developers can create portable code which runs on any platform supporting the JDBC specification. This enables developers to write a single application deployed on multiple database platforms.
JDBC provides the following core features:
- Connectivity: Enables applications and applets written in Java to connect with databases such as Oracle, MS Access etc.
- Query execution: Provides ability for querying data from tables stored in relational databases using standard SQL statements.
- SQL syntax support: Supports different types of SQL Syntax (structured query language). The JDBC driver translates these commands into specific database calls allowing your program portability across many DBMSs, so you don’t have to worry about writing platform-specific queries when coding your Java programs.
- Transaction Management: Enables control over transactions through commit(), rollback(), or setAutoCommit(boolean) methods available within its API library, ensuring ACID properties of RDBMS system are maintained along with supporting distributed transaction management capabilities too.
Q43. How do you get class details in Hibernate?
The process of getting class details in Hibernate involves using the SessionFactory object. The SessionFactory contains metadata about all classes mapped to a database table, including each property/field's name and type. It is used to obtain information on every field for any given persistent entity class.
To get this data, you’ll need to use either Configuration or Metadata Sources methods:
- Configuration - Using Configuration#getClassMapping(String) method passes in a fully qualified classname string (e.g., MyEntityName). It will return an org.hibernate.mapping Class object with accessors retrieve various bits of meta-data associated with your mappings like column names and types etc.
- MetadataSources - Alternatively, you may want or need more control over how identity objects are queried. Instead, you could opt for Metadatasources, where one needs full programmatic control even at initial setup time – if their application requires it.
Here’s an example configuration :
StandardServiceRegistry standard registry = new StandardServiceRegistryBuilder() .configure("hibernate.cfg.xml")
.build();
MetadataSources sources = new MetadataSources(standard registry);
EntityType entity = sources.getEntityBinding(MyEntityName).getEntityPersister().getMappedClass();
MyEntityName Iterator props = entity.getPropertyIterator();
while (props.hasNext()) {
Property prop=(Property)props )next();
String name=prop..name; Type type=prop..type;
System out println("property:"+name+" with type:" +type );
}
Output:
property: id with type: org.hibernate.type.IntegerType
property: name with type: org.hibernate.type.StringType
Q44. What are model classes in Hibernate?
A model class represents the structure (schema) that its associated relational table should have accordingly. This means each annotation reference configured within the class definition will result in a particular column being created during runtime stages while new properties added cause existing columns to become modified unless stated otherwise regarding SQL dialects running beneath those configurations.
Model classes should be well designed before continuing with persistence-related tasks since altering their structure afterward can cause problems due to already created tables having different properties, which will no longer work like expected after changes occur.
Q45. What is a persistent class in Hibernate?
A persistent class in Hibernate is a Java Class with an associated mapping file containing the metadata for the objects of this particular type. The object properties are then mapped to columns in designated tables or tables.
When using Hibernate, a 'persistent instance' can be asked to save and retrieve values from its application database by executing appropriate SQL statements. These actions happen transparently without manual interaction with the underlying SQL commands being executed against the database schema and/or data dictionary.
Q46. What are the benefits of second-level caching?
The benefits of second-level caching are:
1. Improved performance: Caching of frequently used data reduces the load on the database server and increases the application’s responsiveness.
2. Reduced memory footprint: Second-level cache stores the most-used data in a serialized format, minimizing the amount of memory needed to store it.
3. Scalability: Since cached objects can be shared between multiple instances of an application (e.g., web applications deployed onto servers), this helps reduce the number of requests made against the underlying databases resulting in increased scalability for highly concurrent systems.
4. Flexibility: Caches are typically pluggable, allowing developers to choose different strategies that best fit their particular needs.
5. Increase in availability: Caching increases the available time for an application, reducing downtime due to database outages or maintenance.
Q47. How do you implement caching strategies in Hibernate?
Caching strategies in Hibernate can be implemented using an external caching provider, such as Ehcache or Infinispan. These providers allow for configuring various cache settings, such as expiration time and memory size. Caching strategies are then defined through either annotations or XML files, which specify the type (such as READ_ONLY) and parameters related to how a given entity should be cached.
Once configured, these entities will automatically take advantage of efficient uses of second-level caches managed by their respective caching solutions behind the scenes, allowing applications to benefit from improved performance with minimal additional development effort on the part of developers tasked with building out these systems.
Q48. What makes the methods GetCurrentSession() and OpenSession() distinct from one another?
GetCurrentSession() is a method used in the Hibernate framework to obtain the current Session object associated with an application's persistence context. This Session will be bound to a thread and can be reused until it is closed or another session replaces it.
The GetCurrentSession() method returns either an existing open session or, if one exists; otherwise, it creates and opens up a new session by calling OpenSession().
OpenSession(), on the other hand, creates and opens up new sessions each time this method is called without binding any underlying threads. It brings about more flexibility as multiple isolated persistent contexts within one runtime environment can now exist that all work independently of each other yet share some common properties like configured data source settings etc., making them immune from being affected by changes made to any single instance.
Q49. What function does the criteria API serve?
The criteria API defines query parameters for creating typed and dynamic queries in Hibernate, allowing developers to easily create and execute database queries without having to write SQL code. It also facilitates easier manipulation of objects returned from the query results while supporting pagination.
Q50. How does a single-threaded, short-lived object differ from a persistent object?
Single-threaded short-lived objects live only until their work is done or the particular task needs them. In contrast, persistence objects are those which can be stored outside of the scope they were created in, as long as the need may arise such that they can be reused when needed.
The caching mechanism helps improve speed by storing data across multiple requests such that each time a request comes, the same data set isn't evaluated again, leading us to save resources like CPU cycles & memory consumption. Heap Memory, on the contrary, works the opposite.
Q51. What are some key differences between Hibernate APIs and Oracle Database configurations?
Fundamental differences exist with regard to syntax, configuration options, security controls, type safety, etc., between Hibernate APIs and Oracle Database configurations.
Hibernate offers a Relational Object Mapping(ORM) framework usually written using Java language compared to Oracle databases written in SQL, PL/SQL, offering a comprehensive set of tools and features.
Q52. Can you explain what role helper classes play when using Hibernate Sessions factory?
Helper classes are used to offer support services when using the Hibernate SessionFactory.
They offer abstraction for developers by streamlining the codebase and making it simpler to use various components, such as JDBC drivers or ORM frameworks so that we can focus on solving business problems than worrying about complex coding patterns, which would otherwise require more effort.
Q53. What caching mechanisms are available when working with Hibernate applications?
Different types of Caching strategies exist, and each has pros & cons depending upon application usage, such as 1st Level Caching (Session Cache), 2nd level Caching(Query cache), Time To Live Policy wherein data gets removed from memory after a certain period, etc.
Q54. How does heap memory work compared to stack memory within enterprise applications built using Java?
Heap memory is an area of memory used for dynamic allocation. It stores objects and other data structures at runtime in a variable-size pool managed by the Java virtual machine (JVM).
Heap is divided into two generations: young and old, subdivided further to provide more precise control over how much time garbage collection should take. Unlike stack memory, a heap can increase or decrease its size according to the program's needs during runtime.
Stack Memory, on the other hand, is reserved for static variables, method invocations, etc., thus making it faster than Heap, where there might be lots of object movement due to the Garbage Collection process periodically occurring inside JVM while executing programs. Stack has fixed sizes and grows very slowly due to pre-allocated blocks, making them ideal when speed remains constant throughout enterprise applications built using Java.
Q55. How can we create an instance of a class without a default constructor or -args constructor in Java?
We can create an instance without a default constructor or -args constructor by creating overloaded constructors within the class definition, followed by factory pattern creation sent out as a public method to generate an instance of the desired class.
The default constructor of an entity class is mandatory in Hibernate because Hibernate internally uses the reflection API to create objects and invoke methods. The default constructor must be public or protected and should not accept any arguments.
Q56. What is eager loading, and why it's important for query execution & speed optimization with Hibernate?
Eager loading is a technique used in Hibernate applications where related information/data can be fetched from the database by firing separate or a few optimized queries based on defined rules. This approach allows developers to take control of the optimization process & increase performance while retrieving data.
import org.hibernate.Session;
import org.hibernate.SessionFactory;
import org.hibernate.cfg.Configuration;
import java.util.List;
public class EagerLoadingExample {
public static void main(String[] args) {
// Create the Hibernate configuration and session factory
Configuration configuration = new Configuration().configure();
SessionFactory sessionFactory = configuration.buildSessionFactory();
// Open a session
Session session = sessionFactory.openSession();
try {
// Perform the eager loading query
List<Author> authorList = session.createQuery("from Author a join fetch a.books", Author.class).list();
// Print the authors and their associated books
for (Author author : authorList) {
System.out.println("Author: " + author.getName());
System.out.println("Books:");
for (Book book : author.getBooks()) {
System.out.println("- " + book.getTitle());
}
System.out.println();
}
} finally {
// Close the session and session factory
session.close();
sessionFactory.close();
}
}
}
Q57. What parameters should be specified while creating queries through hibernates Query interface?
When creating a Query interface, at least two parameters need to be passed, namely, 1st parameter being 'Query String' and 2nd one being 'a List of positional Parameters', which would get converted into actual arguments listed in the first argument
Example:
// Get the session from SessionFactory instance 'sessionFactory'
Session currentSession = sessionFactory.openSession();
// Create an instance of Query interface / NamedQuery, etc.
String hqlQuery = "from Employee where userName = ?";
Query<Employee> employeeQuery = currentSession.createQuery(hqlQuery);
// Set the parameters to our query
employeeQuery.setParameter(0, "john"); // Where 0 is the index of the parameter and "john" is its value
List<Employee> employeeList = employeeQuery.getResultList();
// Get the result list of our query
Q58. Can you provide a code snippet demonstrating the usage of heap memory within the enterprise application development process by utilizing Java technology stacks?
The code snippet demonstrates the usage of Heap Memory within the Enterprise application development process utilizing Java technology stacks:
//Create object array with references pointing to heap memory
Object[] objects= new Object[scope]; for(int i = 0 ; i<objects.length;i++){
Objects[i] =new MyObject();
}
Q59. What types of caches does Hibernate use, and why?
First-level cache and second-level cache are the two types employed by Hibernate. The default level for caching objects in a session is the first-level cache. The second-level cache is an optional feature that is used to enhance performance by simultaneously caching data across numerous sessions.
Q60. How can a one-to-many mapping relationship be implemented using Hibernate?
A one-to-many mapping relationship between entities can be implemented using Hibernate with annotations on both sides of the mapping. On the many sides, you would use @OneToMany, specifying which entity object should act as a foreign key reference within your model class code
For example: @OneToMany(mappedBy = "exampleEntity")
Q61. How can a one-to-one mapping relationship be implemented using Hibernate?
One-to-one mapping relationships between entities can also be implemented using Hibernate with similar types of annotations. However, this time, it gets more specific because there will always be only one record associated with each other in the table.
Example: If you have an entity called Person and it has a one-to-one relationship with another entity called Address, then on the Person side of the mapping, we would use annotations such as @PrimaryKeyJoinColumn or @OneToOne to specify which field should act as a foreign key reference from address table (for example@PrimaryKeyJoinColumn(name="address_id")).
Q62. What are the different types of interfaces available in Hibernate?
The different types of interfaces available in Hibernate are:
- Session Factory interface: manages all interactions within the database.
Configuration Interface: allows setting up configuration options when creating an instance of the session factory. - Transaction interface: manages transactions that span across multiple query objects
- Query interface: helps us build dynamic queries using HQL dialects offered by Hibernate framework.
Q63. Explain the configuration object in Hibernate.
Configuration object sets up all the needed environments and properties to create a SessionFactory. It contains database-specific properties such as dialect, driver_class, URL, etc., which the Database requires for creating connections. We can also configure JNDI-related information via this file. Apart from these settings, it can be used for configuring Hibernate level settings such as showSql, formatSql & some other advanced Hibernate features like batch size or how to handle multiple application sessions.
Hibernate automatically connects to the database, as opposed to JDBC, which requires the user to make this connection. It is the language that Hibernate employs to execute the queries. In Hibernate, a session does much more than just assist an application in establishing a connection only with the database; it also contributes to the process of storing and retrieving the persistent object. And this makes it an important tool for software developers.
We hope the above Hibernate interview questions will be helpful in your preparation for your dream job. For more such content, stay tuned to Unstop.
You may also like to read:
I am a biotechnologist-turned-content writer and try to add an element of science in my writings wherever possible. Apart from writing, I like to cook, read and travel.
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
Blogs you need to hog!
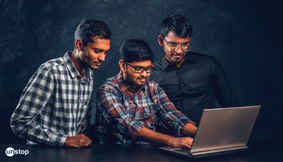
This Is My First Hackathon, How Should I Prepare? (Tips & Hackathon Questions Inside)
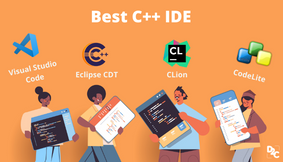
10 Best C++ IDEs That Developers Mention The Most!
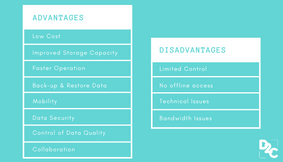
Advantages and Disadvantages of Cloud Computing that you should know!
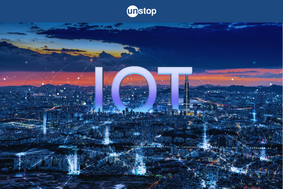
Comments
Add comment