Table of content:
- Understanding Low-Level Languages
- Importance Of Low-Level Languages In System Programming & Hardware Interaction
- Types of Low-Level Languages
- Machine Language (First-Generation Language - 1GL)
- Assembly Language (Second-Generation Language - 2GL)
- Advantages Of Low-Level Languages
- Disadvantages Of Low-Level Languages
- Applications Of Low-Level Languages
- Conclusion
- Frequently Asked Questions
What Is Low-Level Language? All Types Explained (+Examples)
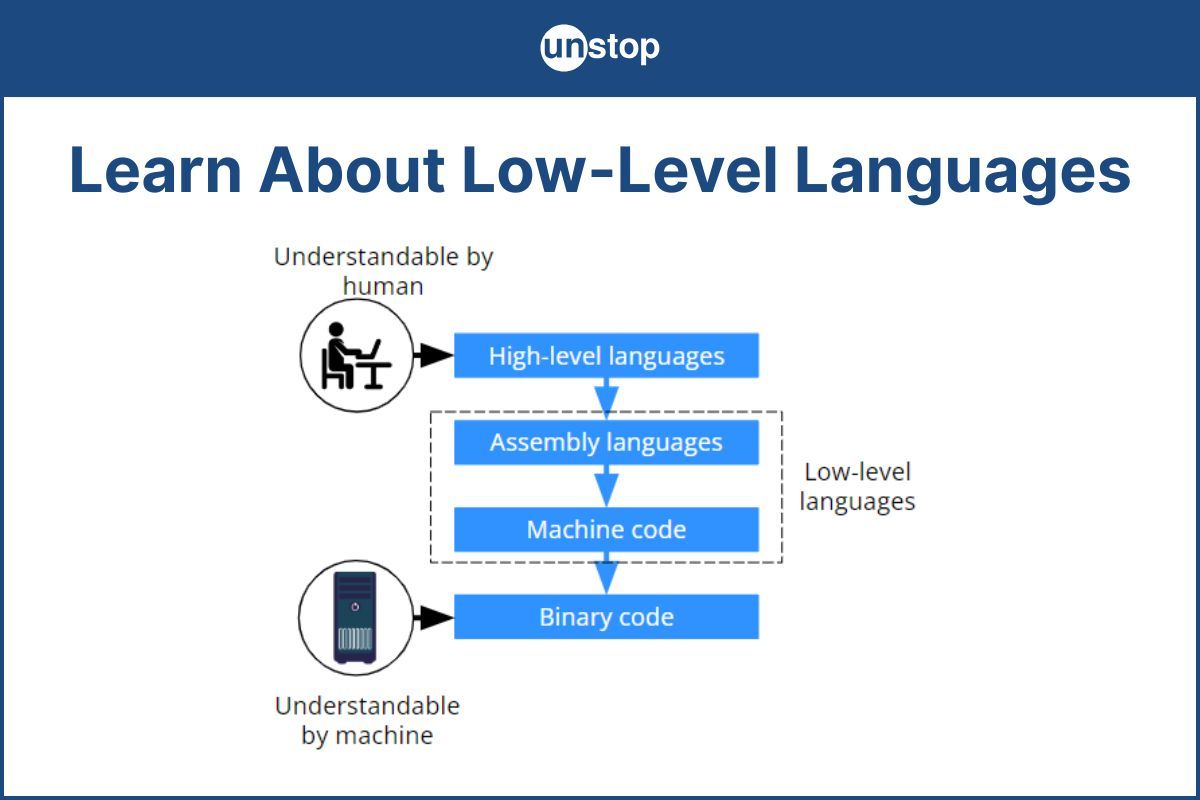
A low-level language is a type of programming language that is closer to machine code and hardware instructions, making it more efficient but less human-readable. These languages provide minimal abstraction from the computer's architecture, allowing programmers to directly manage memory, registers, and CPU operations.
In this article, we will explore the characteristics of low-level languages, their types, advantages, and drawbacks, along with real-world applications where they are still widely used.
Understanding Low-Level Languages
Programming languages can be broadly categorized based on their level of abstraction—how closely they interact with the hardware. Low-level languages are those that operate close to the hardware, offering fine-grained control over system resources like memory, CPU registers, and input/output operations.
What Makes a Language "Low-Level"?
A low-level language is characterized by:
- Minimal abstraction: It provides little to no simplification of hardware functions.
- Direct hardware interaction: The code is closely tied to the computer’s architecture.
- Manual memory management: Programmers have to allocate and manage memory manually.
- Machine dependency: Code written in a low-level language usually works on a specific processor or system.
Think of it like driving a manual transmission car—you have full control over gear shifts, acceleration, and engine performance, but you also need to know the mechanics to operate it effectively.
Comparison with High-Level Languages
Here are the key differences between low-level and high-level languages:
Aspect |
Low-Level Language |
High-Level Language |
Abstraction |
Close to hardware |
Abstracted from hardware |
Ease of Use |
Difficult, complex syntax |
User-friendly, readable syntax |
Performance |
Very fast, optimized |
Slightly slower due to abstraction |
Memory Control |
Full control |
Automatic memory management |
Portability |
Low, hardware-specific |
High, runs on multiple platforms |
A high-level language is like driving an automatic car—you don’t need to worry about gear shifts; the system handles it for you, making it easier to drive. However, you lose some level of control over how the car operates internally.
Importance Of Low-Level Languages In System Programming & Hardware Interaction
Low-level languages are crucial for tasks where performance, precision, and direct hardware access are required, such as:
- Operating system development (e.g., Linux kernel, Windows)
- Embedded systems (e.g., microcontrollers in washing machines, medical devices)
- Device drivers (software that enables OS-hardware communication)
- Cybersecurity & reverse engineering (understanding malware, finding vulnerabilities)
- Game engines & high-performance computing (optimizing performance in graphics-heavy applications)
Analogy:
Imagine a chef working directly with raw ingredients and a farmer managing the soil—this represents low-level programming, where everything is controlled manually. In contrast, a high-level language is like using a pre-packaged meal kit where the complexity is handled for you.
Low-level languages demand skill and effort, but they offer unmatched efficiency and control over how a system operates.
Types of Low-Level Languages
Low-level languages are broadly classified into two types:
- Machine Language (First-Generation Language - 1GL)
- Assembly Language (Second-Generation Language - 2GL)
These languages provide direct interaction with the computer’s hardware, offering precise control over system operations. Let’s explore them in detail.
Machine Language (First-Generation Language - 1GL)
Machine language is the most fundamental form of programming language. It consists purely of binary digits (0s and 1s), which the computer’s processor directly understands and executes.
Characteristics
- No abstraction – Directly communicates with the hardware.
- Fastest execution speed – No need for translation, as the CPU processes it directly.
- Difficult to read and write – Binary code is extremely complex and unintuitive.
- Machine-dependent – A program written in machine code for one processor will not work on another.
Example
A machine language instruction for adding two numbers might look like this:
10110000 01100001
Here, the first part (10110000) is the operation (e.g., move data), and the second part (01100001) is the operand (data or memory location).
Real-Life Analogy
Imagine communicating using Morse code—each dot and dash represents a letter, just like 0s and 1s in machine language. It’s precise but difficult to understand without deep knowledge.
Use Cases
- Embedded systems (e.g., microcontrollers, robotics).
- Firmware programming (e.g., BIOS, low-level hardware control).
Assembly Language (Second-Generation Language - 2GL)
Assembly language is a human-readable representation of machine code. Instead of binary digits, it uses mnemonics (short, readable codes) to represent operations, making programming slightly easier.
Characteristics
- More readable than machine language – Uses mnemonics like MOV, ADD, SUB, JMP, etc.
- Requires an assembler – Converts assembly code into machine code for execution.
- Machine-dependent – Still tied to a specific CPU architecture.
- Faster than high-level languages – Less abstraction means greater efficiency.
Example
A simple Assembly program to add two numbers:
MOV AL, 5 ; Move value 5 into register AL
ADD AL, 3 ; Add 3 to AL
Here, MOV and ADD are mnemonics that replace complex binary instructions.
Real-Life Analogy
Think of assembly language as shorthand notes—it’s still detailed but easier to understand than full binary data, just like how doctors use abbreviations when writing prescriptions.
Use Cases
- Operating System Kernels – Some parts of Linux, Windows, and MacOS are written in assembly for performance.
- Device Drivers – Software that interacts with hardware (e.g., printers, graphics cards).
- Game Development – Optimizing game engines for high performance.
- Cybersecurity – Used in reverse engineering, hacking, and malware analysis.
Comparison of Machine Language vs. Assembly Language
Feature |
Machine Language (1GL) |
Assembly Language (2GL) |
Readability |
Hard to read (binary) |
Easier with mnemonics |
Execution Speed |
Fastest (directly executed by CPU) |
Slightly slower (needs translation) |
Ease of Programming |
Very difficult |
Easier than machine language |
Portability |
Machine-dependent |
Machine-dependent |
Usage |
Direct hardware control |
System programming, drivers, OS development |
Advantages Of Low-Level Languages
Some common advantages of low-level languages are:
- High Performance & Speed
- Direct interaction with hardware ensures faster execution without interpretation overhead.
- Ideal for real-time systems, game engines, and operating system kernels.
- Efficient Memory Management
- Provides complete control over memory allocation and deallocation.
- Avoids unnecessary memory overhead, making it suitable for embedded systems.
- Direct Hardware Interaction
- Can access CPU registers, memory addresses, and I/O devices directly.
- Essential for device drivers, firmware, and hardware programming.
- Minimal System Overhead
- No dependency on heavy runtime environments or interpreters.
- Used in low-resource environments like microcontrollers and IoT devices.
- Greater Precision & Optimization
- Allows fine-tuning of code for performance optimization.
- Used in high-performance computing, cybersecurity, and AI models.
- Reliability & Stability
- Less prone to unexpected behavior due to direct control over execution flow.
- Used in mission-critical systems like medical devices and aerospace applications.
- Better Understanding of Computer Architecture
- Helps programmers learn how processors, memory, and instructions work.
- Useful for security experts, system programmers, and reverse engineers.
Disadvantages Of Low-Level Languages
Some common disadvantages of low-level languages are:
- Difficult to Learn & Code
- Requires a deep understanding of computer architecture and instruction sets.
- Code is complex and less readable compared to high-level languages.
- Machine Dependent
- Programs written for one CPU architecture (e.g., x86, ARM) may not work on another without modification.
- Lacks portability, unlike high-level languages.
- Time-Consuming Development
- Requires manual handling of memory, CPU registers, and low-level operations.
- Even simple tasks require multiple instructions, increasing development time.
- Error-Prone & Difficult Debugging
- No built-in debugging tools or high-level error handling mechanisms.
- Small mistakes can cause system crashes or critical failures.
- Limited Abstraction
- Does not support modern programming concepts like object-oriented programming (OOP) or built-in libraries.
- Requires writing extensive code for tasks that are simpler in high-level languages.
- Higher Maintenance Effort
- Code modifications and updates require significant effort due to low readability.
- Debugging and troubleshooting are complex, making maintenance challenging.
Applications Of Low-Level Languages
Low-level languages play a crucial role in system programming, hardware interaction, and performance-critical applications. Since they provide direct access to the hardware and allow fine-tuned control over system resources, they are widely used in various domains. Below are some key applications:
1. Operating Systems Development
- Operating systems like Windows, Linux, and macOS are built using low-level languages such as C and Assembly.
- They require direct interaction with CPU, memory management, and hardware control, which low-level languages efficiently handle.
Example: The Linux kernel is primarily written in C with some Assembly code for architecture-specific optimizations.
2. Embedded Systems & IoT Devices
- Embedded systems include microcontrollers, medical devices, automotive control units, and smart appliances.
- These systems require minimal memory usage and high efficiency, which low-level languages provide.
Example: The firmware in an airbag control system in cars is written in Assembly or C to ensure fast response times.
3. Device Drivers & Firmware Development
- Low-level languages are used to develop device drivers that enable communication between hardware components and the OS.
- Firmware, the permanent software embedded in hardware devices, is often written in Assembly or C.
Example: Graphics card drivers from NVIDIA and AMD are written in low-level languages for optimal performance.
4. Game Development & Graphics Rendering
- Game engines and rendering software require real-time processing with minimal delay.
- Low-level languages help in optimizing frame rates, memory usage, and CPU/GPU performance.
Example: Game engines like Unreal Engine use C++ with low-level Assembly optimizations for smooth performance.
5. Cybersecurity & Reverse Engineering
- Security professionals use low-level languages to analyze malware, identify vulnerabilities, and develop security patches.
- Reverse engineers study compiled binaries to understand or modify software behavior.
Example: Ethical hackers analyze Assembly code to detect security flaws in operating systems.
6. High-Performance Computing (HPC)
- Supercomputers used for weather forecasting, scientific simulations, and AI training require extreme processing power.
- Low-level languages allow precise optimization for parallel computing and resource utilization.
Example: NASA uses low-level programming for high-speed simulations in spacecraft design.
7. Aerospace & Defense Systems
- Systems in aerospace and defense must be highly reliable and fail-safe.
- Low-level languages ensure precision and predictable behavior in critical operations.
Example: The software in autopilot systems in aircraft is written in low-level languages for real-time responsiveness.
8. Database & Storage Systems
- Databases need efficient file management and memory handling for quick data retrieval and storage.
- Low-level programming helps optimize disk operations and reduce latency.
Example: The MySQL database engine is written in C for performance and efficiency.
Conclusion
Low-level languages serve as the backbone of system programming, providing direct hardware control, high efficiency, and real-time execution. While they are more complex and require in-depth knowledge of computer architecture, their advantages make them indispensable in areas like operating system development, embedded systems, cybersecurity, and high-performance computing.
Despite the rise of high-level languages that simplify coding and improve productivity, low-level languages remain crucial for performance-critical applications where speed, precision, and resource management are top priorities. Understanding these languages not only helps developers optimize software but also deepens their knowledge of how computers operate at a fundamental level.
Ultimately, choosing between low-level and high-level languages depends on the specific requirements of a project. When efficiency and hardware interaction are essential, low-level languages are the ideal choice.
Frequently Asked Questions
Q. Why are low-level languages considered difficult to learn and use?
Low-level languages, such as Assembly and machine language, require a deep understanding of computer architecture, including memory management, CPU registers, and instruction sets. Unlike high-level languages that provide built-in functions and abstractions, low-level languages demand manual handling of operations like data movement, memory allocation, and hardware interactions. Additionally, their syntax is less readable and more complex, making debugging and maintenance challenging.
Q. How do low-level languages differ from high-level languages in terms of abstraction?
The primary difference lies in the level of abstraction:
- Low-level languages provide minimal abstraction from the hardware, meaning programmers must directly manage system resources, making them faster but harder to code.
- High-level languages (e.g., Python, Java) offer significant abstraction, using human-readable syntax and automated memory management, which simplifies development but adds processing overhead.
For example, writing a simple loop in Assembly requires multiple instructions to manage registers and memory, whereas in Python, a single for loop handles the iteration effortlessly.
Q. In which scenarios should low-level languages be preferred over high-level languages?
Low-level languages should be used when performance, efficiency, and direct hardware interaction are the top priorities. Common scenarios include:
- Operating System Development – OS kernels need direct access to CPU and memory.
- Embedded Systems – Microcontrollers in medical devices, IoT devices, and automotive systems require minimal memory usage.
- Game Development – Graphics rendering and game engines rely on low-level optimization for smooth performance.
- Cybersecurity & Reverse Engineering – Security professionals analyze low-level code to detect vulnerabilities.
- Aerospace & Defense – Mission-critical applications require high reliability and real-time execution.
In contrast, high-level languages are preferred for general-purpose applications, web development, and data processing due to their ease of use and cross-platform compatibility.
Q. How do low-level languages impact system performance compared to high-level languages?
Low-level languages provide better performance because they:
- Execute instructions directly on the CPU without requiring interpretation or compilation overhead.
- Allow fine-tuned control over memory allocation and processor registers, reducing unnecessary system overhead.
- Enable programmers to write optimized code tailored for specific hardware, leading to faster execution.
In contrast, high-level languages introduce additional processing layers, such as garbage collection, dynamic typing, and runtime interpretation, which can slow down execution. This is why performance-critical applications, such as gaming engines, real-time systems, and high-performance computing, rely on low-level programming.
Q. What challenges do developers face when working with low-level languages?
Some key challenges include:
- Complexity & Readability – Code is harder to read and understand compared to high-level languages.
- Time-Consuming Development – Writing efficient code takes longer due to the lack of built-in functions and abstractions.
- Debugging Difficulties – Errors can be harder to detect, and debugging tools are less advanced than those available for high-level languages.
- Platform Dependency – Low-level programs often need to be rewritten for different hardware architectures.
Despite these challenges, learning low-level programming is valuable for gaining deeper insights into system behavior, performance optimization, and hardware interactions.
Suggested Reads:
- Difference Between Encoder And Decoder Explained!
- Difference Between Static And Dynamic Memory Allocation (With Comparison Chart)
- Functional Units of Computer: Decoded and Explained
- What Is The Difference Between Procedural And Object-Oriented Programming?
- What Is The Difference Between HTML and CSS?
I’m a Computer Science graduate with a knack for creative ventures. Through content at Unstop, I am trying to simplify complex tech concepts and make them fun. When I’m not decoding tech jargon, you’ll find me indulging in great food and then burning it out at the gym.
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
Comments
Add comment