Table of content:
- What is the IBM Coding Assessment?
- Exam Pattern and Key Topics
- Sample Question Types
- Top 5 Problem Statements for IBM Coding Assessment
- Preparation Tips for IBM Coding
- Conclusion
- Frequently Asked Questions (FAQs)
IBM Coding Assessment Questions and Solutions for Freshers 2025
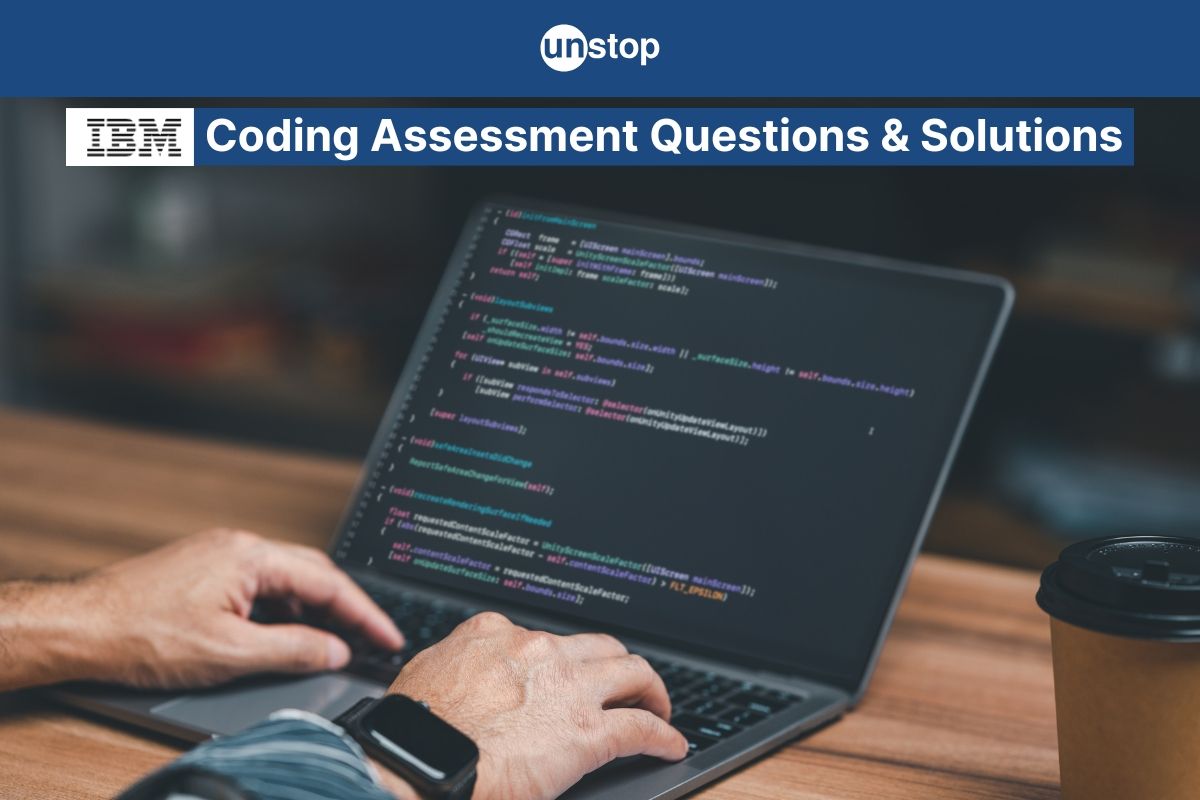
IBM's hiring process is structured to assess technical proficiency, problem-solving capabilities, and adaptability, with the IBM Coding Assessment being a critical step. For freshers aspiring to work at IBM, acing this assessment requires a combination of technical knowledge and strategic preparation.
What is the IBM Coding Assessment?
The IBM Coding Assessment evaluates candidates on their ability to solve real-world problems using programming skills. It typically features:
- Coding Challenges: Focus on algorithms, data structures, and logical reasoning.
- Programming Languages: You can choose from popular options like Python, Java, and C++.
Exam Pattern and Key Topics
Exam Structure
Section |
Number of Questions |
Duration |
Key Focus Areas |
Problem Solving |
2-3 |
60-90 mins |
Algorithms, logic, and recursion |
Debugging |
1-2 |
15-20 mins |
Code corrections and optimisation |
Applied Problems |
1 |
30-45 mins |
Real-world programming scenarios |
Core Topics to Cover
- Data Structures: Arrays, strings, stacks, and queues.
- Algorithms: Sorting, searching, recursion, and dynamic programming.
- Real-world scenarios: Data analysis, system design, and error handling.
- Code Debugging: Identifying and fixing syntax and logic errors in the provided code.
Sample Question Types
Question Type |
Example |
Problem-Solving |
Write a program to sort a list of names alphabetically. |
Debugging |
Identify the bug in a provided loop and correct it. |
Real-World Scenario |
Write a program to calculate monthly electricity usage. |
Are you looking for IBM coding assessment questions? Click here to access coding practice sessions from moderate to challenging levels.
Top 5 Problem Statements for IBM Coding Assessment
Problem Statement 1
John works in a coin factory where each coin has an ID. He has an array 'nums' of size N. In the factory, coins are assigned to boxes based on the sum of the digits of their IDs. For example, if the ID of a coin is 321, the sum of its digits is 6 (since 3 + 2 + 1 = 6), so the coin will be placed in box 6.
John has been given a task to work with coins that have IDs in the range from the smallest number in 'nums', called the low limit, to the largest number in 'nums', called the high limit. For each number in this range (from low-limit to high-limit, inclusive), John needs to calculate the sum of the digits and place the coin in the corresponding box.
Help John determine which box contains the most coins and output the number of coins in that box.
Note: The factory has coins with IDs ranging from 0 to infinity, but John only needs to work with coins whose IDs lie between the low limit and high limit (inclusive).
Input Format
The first line contains an integer N, representing the number of elements in nums.
The second line contains n space-separated integers representing the elements of the array nums.
Output Format
Print a single integer representing the maximum number of coins placed in any one box.
Constraints
1 <=N <=10^5
0 <= nums[i] <= 10^4
Solution C++
#include<bits/stdc++.h>
using namespace std;
#define mod 1e9 + 7
typedef long long ll;
int countBalls1(int lowLimit, int highLimit) {
// creating 46 sized count array
int cnt[46] = {};
// looping from lowLimit to highLimit
for (auto i = lowLimit; i <= highLimit; ++i) {
// sum variable for calculating the sum
int sum = 0, n = i;
// summing up the digits
while(n) {
sum += n % 10;
n /= 10;
}
// incrementing the cnt[sum]
++cnt[sum];
}
// returning the max element from cnt vector
return *max_element(begin(cnt), end(cnt));
}
int countBalls(vector<int>& arr){
// sorting the arr vector
sort(arr.begin(),arr.end());
// finding low and high
int low=arr[0],high=arr[arr.size()-1];
// returning answer
return countBalls1(low,high);
}
int main(){
int n;
cin>>n;
vector<int>arr(n);
for(int i=0;i<arr.size();i++){
cin>>arr[i];
}
cout<<countBalls(arr)<<endl;
return 0;
}
Solution Java
import java.util.*;
public class Main {
static int countBalls1(int lowLimit, int highLimit) {
int[] cnt = new int[46];
for (int i = lowLimit; i <= highLimit; ++i) {
int sum = 0, n = i;
while (n > 0) {
sum += n % 10;
n /= 10;
}
++cnt[sum];
}
return Arrays.stream(cnt).max().getAsInt();
}
static int countBalls(List<Integer> arr) {
Collections.sort(arr);
int low = arr.get(0);
int high = arr.get(arr.size() - 1);
return countBalls1(low, high);
}
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int n = sc.nextInt();
List<Integer> arr = new ArrayList<>();
for (int i = 0; i < n; i++) {
arr.add(sc.nextInt());
}
System.out.println(countBalls(arr));
}
}
Solution Python
def countBalls1(lowLimit, highLimit):
cnt = [0] * 46
for i in range(lowLimit, highLimit + 1):
sum_digits = 0
n = i
while n:
sum_digits += n % 10
n //= 10
cnt[sum_digits] += 1
return max(cnt)
def countBalls(arr):
arr.sort()
low = arr[0]
high = arr[-1]
return countBalls1(low, high)
n = int(input())
arr = list(map(int, input().split()))
print(countBalls(arr))
Problem Statement 2
You were learning the array data structure till your friend challenged you with a problem. Given an array of queries of positive integers between 1 and k, you have to process all queries[i] according to the following rules:
In the beginning, you have the permutation P=[1,2,3,...,m].
For the current i, find the position of queries[i] in the permutation P (indexing from 0) and then move this at the beginning of the permutation P.
Notice that the position of queries[i] in the permutation P is the result for queries[i]. Print an array containing the result for the given queries.
Input Format
The first line contains m, the initial permutation P = [1,2,3,….,m]
The following line contains q, the number of queries
The following line contains q numbers denoting the ith query, starting from 0 to q-1.
Output Format
Print the result of all queries in a space-separated single-line fashion.
Constraints
1<=m<=5*10^4
1<=q<=m
1<=queries[i]<=m
Solution C++
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
int main() {
int m, q;
cin >> m;
vector<int> permutation(m);
for (int i = 0; i < m; i++) {
permutation[i] = i + 1;
}
cin >> q;
vector<int> queries(q);
for (int i = 0; i < q; i++) {
cin >> queries[i];
}
vector<int> result(q);
for (int i = 0; i < q; i++) {
int query = queries[i];
int index = find(permutation.begin(), permutation.end(), query) - permutation.begin();
result[i] = index;
// Move the query element to the beginning of the permutation
rotate(permutation.begin(), permutation.begin() + index, permutation.begin() + index + 1);
}
// Print the result
for (int i = 0; i < q; i++) {
cout << result[i] << " ";
}
cout << endl;
return 0;
}
Solution Java
import java.util.*;
public class Main {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
// Read the initial size of the permutation array
int m = scanner.nextInt();
// Initialize the permutation array P = [1, 2, 3, ..., m]
List<Integer> permutation = new ArrayList<>();
for (int i = 1; i <= m; i++) {
permutation.add(i);
}
// Read the number of queries
int q = scanner.nextInt();
// Read the queries
List<Integer> queries = new ArrayList<>();
for (int i = 0; i < q; i++) {
queries.add(scanner.nextInt());
}
// Result array to store the results of each query
List<Integer> result = new ArrayList<>();
// Process each query
for (int i = 0; i < q; i++) {
int query = queries.get(i);
// Find the index of the query in the permutation list
int index = permutation.indexOf(query);
// Store the result (the position of the query)
result.add(index);
// Move the query element to the beginning of the permutation
// Remove the element from its current position and add it to the front
permutation.remove(index);
permutation.add(0, query);
}
// Print the result array
for (int res : result) {
System.out.print(res + " ");
}
scanner.close();
}
}
Solution Python
def process_queries(m, queries):
# Initialize the permutation P
P = list(range(1, m + 1))
results = []
# Process each query
for query in queries:
# Find the position of the current query element
pos = P.index(query)
# Append the result (position) to the results list
results.append(pos)
# Move the queried element to the beginning of the permutation
P.pop(pos)
P.insert(0, query)
return results
# Example usage:
if __name__ == "__main__":
import sys
input = sys.stdin.read
data = input().split()
m = int(data[0])
q = int(data[1])
queries = list(map(int, data[2:2+q]))
result = process_queries(m, queries)
print(' '.join(map(str, result)))
Problem Statement 3
Ram spots a line of N cars on the busy street, each with a unique feature. You're provided with an array called "features," where features[i] represent a distinctive feature of the ith car. Ram's quest is to discover the widest separation between two cars with different features. Can you help Ram with his quest?
Remember, this distance is determined by the absolute difference in their positions (abs(i - j)).
Input Format
The first line contains an integer N, the number of cars.
The second line contains N space-separated integers, where each integer denotes the feature of the i-th car.
Output Format
Print a single integer, which is the widest separation between any two cars that have different features.
Constraints
2 <= N <= 10^2
0 <= features[i] <= 10^3
Solution C++
#include <bits/stdc++.h>
using namespace std;
int maxDistance(vector<int> cs) {
int n = cs.size(), i = 0, j = n - 1;
while (cs[0] == cs[j])
--j;
while (cs[n - 1] == cs[i])
++i;
return max(j, n - i - 1);
}
int main() {
int n;
cin>>n;
vector<int> a(n);
for(auto &x : a) cin>>x;
int ans=maxDistance(a);
cout<<ans<<endl;
}
Solution Java
import java.util.*;
public class CarFeatureSeparation {
public static int maxDistance(List<Integer> cs) {
int n = cs.size();
int i = 0, j = n - 1;
// Find the first car with a feature different from the last car's feature
while (cs.get(0).equals(cs.get(j))) {
--j;
}
// Find the last car with a feature different from the first car's feature
while (cs.get(n - 1).equals(cs.get(i))) {
++i;
}
// Return the maximum distance
return Math.max(j, n - i - 1);
}
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
// Read the number of cars
int n = scanner.nextInt();
// Read the features of the cars
List<Integer> a = new ArrayList<>();
for (int i = 0; i < n; i++) {
a.add(scanner.nextInt());
}
// Find the maximum separation
int ans = maxDistance(a);
// Print the result
System.out.println(ans);
scanner.close();
}
}
Solution Python
def max_distance(cs):
n = len(cs)
i = 0
j = n - 1
# Find the first car with a feature different from the last car's feature
while cs[0] == cs[j]:
j -= 1
# Find the last car with a feature different from the first car's feature
while cs[n - 1] == cs[i]:
i += 1
# Return the maximum distance
return max(j, n - i - 1)
def main():
# Read the number of cars
n = int(input())
# Read the features of the cars
a = list(map(int, input().split()))
# Find the maximum separation
ans = max_distance(a)
# Print the result
print(ans)
if __name__ == "__main__":
main()
Problem Statement 4
Given a n * m matrix of ones and zeros, where 0 denotes water and 1 denotes island now you have to count the number of square sub-island.
Input Format
First line contains n and m, where n is the number of rows and m is the column.
Second line contains the element of the arr matrix.
Output Format
Print the count of the number of square sub-island.
Constraints
1 <= arr.length <= 300
1 <= arr[0].length <= 300
0 <= arr[i][j] <= 1
Solution C++
#include <bits/stdc++.h>
using namespace std;
#define int int64_t
int solve(vector<vector<int>>& arr) {
int n = arr.size();
int m = arr[0].size();
vector<vector<int>> dp(n, vector<int>(m, 0));
for(int i=0; i<n; i++) {
for(int j=0; j<m; j++) {
if(i == 0 || j == 0) dp[i][j] = arr[i][j];
else {
dp[i][j] = (arr[i][j] == 1) ? 1 + min({dp[i-1][j], dp[i-1][j-1], dp[i][j-1]}) : 0;
}
}
}
int sum = 0;
for(int i=0; i<n; i++) {
for(int j=0; j<m; j++) {
sum += dp[i][j];
}
}
return sum;
}
signed main() {
int n, m;
cin>>n>>m;
vector<vector<int>> vec(n, vector<int>(m));
for(int i=0; i<n; i++) {
for(int j=0; j<m; j++) {
cin>>vec[i][j];
}
}
cout<<solve(vec)<<endl;
return 0;
}
Solution Java
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
int row = scanner.nextInt();
int col = scanner.nextInt();
int[][] grid = new int[row][col];
for (int j = 0; j < row; j++) {
for (int k = 0; k < col; k++) {
grid[j][k] = scanner.nextInt();
}
}
int ans = 0;
for (int j = 1; j < row; j++) {
for (int k = 1; k < col; k++) {
if (grid[j][k] == 1) {
grid[j][k] = 1 + Math.min(grid[j - 1][k], Math.min(grid[j][k - 1], grid[j - 1][k - 1]));
}
}
}
for (int j = 0; j < row; j++) {
for (int k = 0; k < col; k++) {
ans += grid[j][k];
}
}
System.out.println(ans);
}
}
Solution Python
def countSquares(matrix):
# Get the dimensions of the matrix
n = len(matrix)
m = len(matrix[0])
# DP table to store the size of the largest square sub-island ending at (i, j)
dp = [[0] * m for _ in range(n)]
# Variable to store the total count of square sub-islands
total_count = 0
# Iterate through each cell in the matrix
for i in range(n):
for j in range(m):
if matrix[i][j] == 1:
# If it's the first row or first column, the largest square is just the current cell
if i == 0 or j == 0:
dp[i][j] = 1
else:
# DP relation: take the minimum of the three neighboring squares and add 1
dp[i][j] = min(dp[i-1][j], dp[i][j-1], dp[i-1][j-1]) + 1
# Add the size of the square ending at (i, j) to the total count
total_count += dp[i][j]
return total_count
# Input reading
n, m = map(int, input().split()) # n rows and m columns
matrix = [list(map(int, input().split())) for _ in range(n)] # Input the matrix
# Get the result
result = countSquares(matrix)
# Output the result
print(result)
Problem Statement 5
Aryan and Ankit play a game with N boxes. There are an even number of boxes arranged in a row, and each box has a positive integer number of stone balls, denoted by balls(i). The objective of the game is to end with the most balls. The total number of balls across all the boxes is odd, so there are no ties.
Rules:
Aryan and Ankit take turns picking all the balls from either the beginning or the end of the row of boxes. Aryan always plays first. Both players play optimally, meaning they make decisions to maximize their number of balls.
The game ends when there are no more boxes left. Assume Aryan and Ankit play optimally. Print Aryan if Aryan wins, and print Ankit if Ankit wins.
Input Format
The first line of input contains an integer N, representing the size of the array.
The second line of input contains N space-separated integers representing the number of balls in the boxes.
Output Format
Display "Aryan" if Aryan wins or Display"Ankit" if Ankit wins.
Constraints
1<=N<=10^2
Assuming Arr denotes the array and Arr(i) denotes array element at ith index of the array.
1<=Arr(i)<=10^4
Solution C++
#include <bits/stdc++.h>
#include <ext/pb_ds/assoc_container.hpp>
#include <ext/pb_ds/tree_policy.hpp>
using namespace __gnu_pbds;
using namespace std;
using ll = long long;
#define all(a) (a).begin(), (a).end()
#define rall(a) (a).rbegin(), (a).rend()
#define sz(a) (int) ((a).size())
#define pb push_back
#define fi first
#define se second
#define f0r(i, a, b) for(int i = (a); i < (b); ++i)
#define f0rr(i, a, b) for(int i = (a - 1); i >= (b); --i)
#define trav(i, v) for(auto &i : (v))
template<class T> using Tree = tree<T, null_type, less<T>, rb_tree_tag,tree_order_statistics_node_update>;
template<typename T> T pow(T a, T b) { T res = 1; f0r(i, 0, b) res = res * a; return res; }
template<typename T> void ckmax(T &a, T b) { a = max(a, b); }
template<typename T> void ckmin(T &a, T b) { a = min(a, b); }
int dx4[] = {0, 1, 0, -1};
int dy4[] = {1, 0, -1, 0};
int dx8[] = {-1, -1, -1, 0, 1, 1, 1, 0};
int dy8[] = {-1, 0, 1, 1, 1, 0, -1, -1};
const int N2 = 1004, N = 200004;
const ll linf = 1000000000000000000;
const int inf = 1000010000;
int n, m, x, y;
ll v[N], a[N], b[N];
ll dp[N2][N2];
// <===================================================================================================>
ll f(int i, int j) {
if(i > j) return 0LL;
if(dp[i][j] != -1) return dp[i][j];
ll ans = 0;
ans = v[i] + f(i + 1, j);
ckmax(ans, v[j] + f(i, j - 1));
return dp[i][j] = ans;
}
int main(){
cin.tie(nullptr)->sync_with_stdio(false);
int tt = 1;
// cin >> tt;
f0r(T, 1, tt + 1) {
cin >> n;
ll tot = 0;
f0r(i, 1, n + 1) {
cin >> v[i];
tot += v[i];
}
memset(dp, -1, sizeof(dp));
f0r(i, 1, n + 1) {
dp[i][i] = v[i];
}
ll alice = f(1, n);
ll bob = tot - alice;
if(alice > bob) cout << "Aryan";
else cout << "Ankit";
}
return 0;
}
Solution Java
import java.io.*;
import java.util.*;
import java.text.*;
import java.math.*;
import java.util.regex.*;
public class Solution {
public static void main(String[] args) {
/* Enter your code here. Read input from STDIN. Print output to STDOUT. Your class should be named Solution. */
System.out.println("Aryan");
}
}
Solution Python
# Enter your code here. Read input from STDIN. Print output to STDOUT
n=int(input())
l=list(map(int, input().split()))
p=len(l)
s=0
k=0
for i in range(p//2):
s+=l[i]
for i in range((p//2),p):
k+=l[i]
if(l[0]>l[-1]):
if(s>k):
print("Aryan")
else:
print("Ankit")
else:
if(k>s):
print("Aryan")
else:
print("Ankit")
Preparation Tips for IBM Coding
- Practice Coding Daily: Search for available online platforms to practice IBM-style problems.
- Master Time Management: Simulate test conditions to improve speed and accuracy.
- Review Past Questions: Many coding patterns repeat, so reviewing previous IBM questions is invaluable.
- Learn Debugging: Develop the ability to quickly identify and resolve issues in code.
Conclusion
The IBM Coding Assessment is your gateway to joining one of the most innovative tech companies. With structured preparation and regular practice, freshers can enhance their chances of success. Focus on understanding core concepts, practising coding daily, and learning from past papers to approach the test confidently.
Frequently Asked Questions (FAQs)
1. What programming languages are supported in IBM’s coding assessment?
Most common programming languages like Python, Java, and C++ are allowed.
2. What is the level of difficulty for IBM coding questions?
Questions range from beginner to intermediate levels, with occasional advanced challenges.
3. Can I revisit questions during the assessment?
The ability to navigate between questions depends on the test platform used.
4. What resources can help with preparation?
Search for available online platforms to practice coding prep resources, which are highly recommended.
5. Does IBM evaluate only coding skills?
Along with coding, IBM assesses problem-solving, logical reasoning, and adaptability.
Disclaimer: While we strive for accuracy, we do not guarantee its completeness or reliability. Readers are encouraged to verify all facts and statistics from the official company website or check independently before making decisions.
Suggested reads:
- Accenture Coding Questions: 5 Coding Solutions for Freshers 2025
- HCL Coding Questions: Coding Pattern with Questions & Solutions
- TCS Ninja Coding Questions: Top 5 Coding Questions with Solution
- Deloitte Coding Questions: Top 5 Coding Questions for Freshers
- TCS Digital Coding Questions: Top 5 Coding Questions & Solutions
Instinctively, I fall for nature, music, humour, reading, writing, listening, travelling, observing, learning, unlearning, friendship, exercise, etc., all these from the cradle to the grave- that's ME! It's my irrefutable belief in the uniqueness of all. I'll vehemently defend your right to be your best while I expect the same from you!
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
Blogs you need to hog!
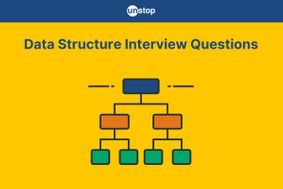
55+ Data Structure Interview Questions For 2025 (Detailed Answers)
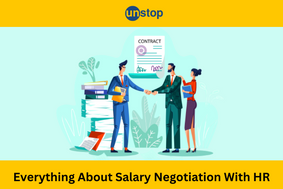
How To Negotiate Salary With HR: Tips And Insider Advice
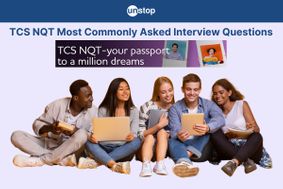
Best 80+ TCS NQT Most Commonly Asked Interview Questions for 2025
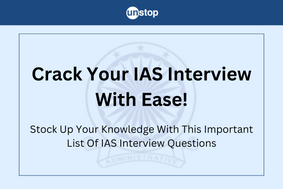
Comments
Add comment