Table of content:
- Digital Coding Questions: Topics Covered
- TCS Digital Previous Year Coding Questions
- Top 5 Digital Coding Questions with Solution
- Digital Advanced Coding Questions
- TCS Digital Coding Round Questions
- Digital Coding Questions for Freshers
- How to Prepare for TCS Digital Coding Exam?
- Conclusion
- Frequently Asked Questions (FAQs)
TCS Digital Coding Questions: Top 5 Coding Questions & Solutions
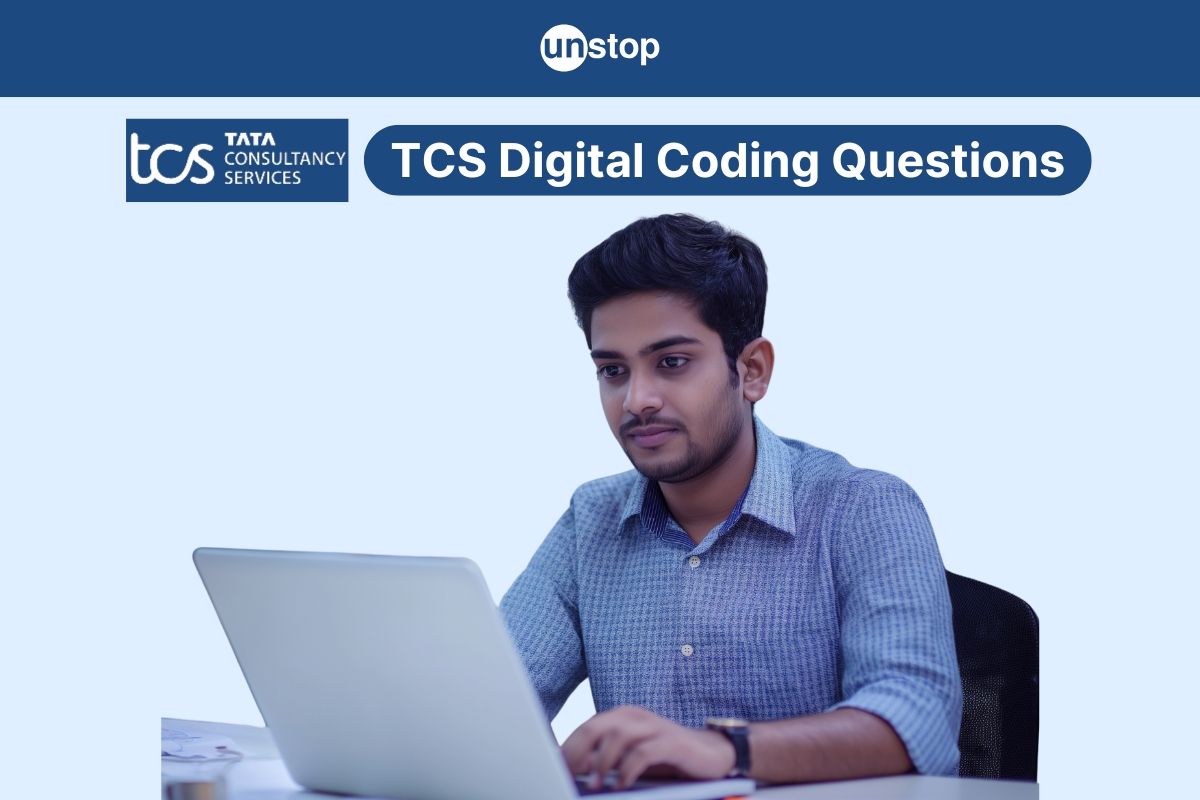
TCS Digital is a premium role offered by Tata Consultancy Services (TCS) that focuses on digital technologies. To secure this role, candidates must go through a rigorous selection process, including a challenging coding round. This guide provides a comprehensive overview of TCS Digital coding questions, covering various aspects such as previous year questions, advanced coding problems, and the types of questions to expect.
TCS Digital Coding Round Overview
The coding round is a crucial stage in the TCS Digital hiring process. It typically consists of 2-3 questions that test the candidate's problem-solving abilities, coding skills, and algorithmic knowledge. The time duration for this round is usually between 60 to 90 minutes.
Category | Details |
---|---|
Number of Questions | 2-3 coding problems |
Duration | 60 to 90 minutes |
Difficulty Level | Moderate to Advanced |
Languages Allowed | C, C++, Java, Python, etc. |
Focus Areas | Data Structures, Algorithms, Dynamic Programming |
Digital Coding Questions: Topics Covered
The questions in TCS Digital coding assessments cover a wide range of programming topics. Here's a breakdown of the topics and the types of questions you may encounter:
Topic | Description | Example Questions |
---|---|---|
Data Structures | Implementing and manipulating data structures such as arrays, linked lists, stacks, and trees. | Reverse a linked list, Find a cycle in a graph |
Algorithms | Questions involving searching, sorting, and optimization techniques. | Quick Sort, Binary Search, Dijkstra's Algorithm |
Dynamic Programming (DP) | Problems requiring memoization or tabulation to optimize solutions. | Knapsack problem, Longest Common Subsequence |
Greedy Algorithms | Optimization problems are solved through the greedy approach. | Activity selection, Fractional Knapsack |
String Manipulation | String-related problems such as pattern matching and transformations. | Palindrome check, Anagram detection |
Recursion & Backtracking | Recursive problems that involve decision trees and combinatorial searches. | N-Queens, Subset Sum problem |
TCS Digital Previous Year Coding Questions
TCS Digital often repeats coding patterns from previous years with slight modifications. Here are some examples of the previous year's coding questions:
Question | Type | Topic | Difficulty |
---|---|---|---|
Write a program to find the shortest path in a weighted graph using Dijkstra's algorithm. | Algorithm | Graph Theory | Advanced |
Given a matrix, rotate it 90 degrees clockwise. | Data Structures | Matrix Manipulation | Moderate |
Implement a function to check if two strings are anagrams of each other. | String Manipulation | Sorting, String | Easy |
Solve the 0/1 Knapsack problem using dynamic programming. | Dynamic Programming | DP, Recursion | Advanced |
Find all permutations of a given string using recursion. | Backtracking | String, Recursion | Moderate |
Enhance your coding skills by practicing coding questions across various levels. Click here to start your coding journey and master programming!
Top 5 Digital Coding Questions with Solution
Problem Statement 1
One day, Jack finds a string of characters. He is very keen to arrange them in reverse order, i.e., the first characters become the last characters, the second characters become the second-last characters, and so on.
Now he wants your help to find the kth character from the new string formed after reverse the original string.
Note: String contains only lowercase Latin letters.
Input Format
The first line contains two integers n, k — the length of array and the value of k respectively.
The second line contains a string containing n characters.
Output Format
Print a single line containing the kth character of the string.
Constraints
1 ≤ k ≤ n≤ 10^6
Testcase Input
5 2
abdfa
Testcase Output
f
Solution 1: C++
YiNpbmNsdWRlIDxiaXRzL3N0ZGMrKy5oPgojaW5jbHVkZSA8ZXh0L3BiX2RzL2Fzc29jX2NvbnRhaW5lci5ocHA+CiNpbmNsdWRlIDxleHQvcGJfZHMvdHJlZV9wb2xpY3kuaHBwPgoKdXNpbmcgbmFtZXNwYWNlIF9fZ251X3BiZHM7CnVzaW5nIG5hbWVzcGFjZSBzdGQ7CnVzaW5nIGxsID0gbG9uZyBsb25nOwoKI2RlZmluZSBhbGwoYSkgICAgICAgICAgICAgKGEpLmJlZ2luKCksIChhKS5lbmQoKQojZGVmaW5lIHJhbGwoYSkgICAgICAgICAgICAoYSkucmJlZ2luKCksIChhKS5yZW5kKCkKI2RlZmluZSBzeihhKSAgICAgICAgICAgICAgKGludCkgKChhKS5zaXplKCkpCiNkZWZpbmUgcGIgICAgICAgICAgICAgICAgIHB1c2hfYmFjawojZGVmaW5lIGZpICAgICAgICAgICAgICAgICBmaXJzdAojZGVmaW5lIHNlICAgICAgICAgICAgICAgICBzZWNvbmQKI2RlZmluZSBmMHIoaSwgYSwgYikgICAgICAgZm9yKGludCBpID0gKGEpOyBpIDwgKGIpOyArK2kpCiNkZWZpbmUgZjBycihpLCBhLCBiKSAgICAgIGZvcihpbnQgaSA9IChhIC0gMSk7IGkgPj0gKGIpOyAtLWkpCiNkZWZpbmUgdHJhdihpLCB2KSAgICAgICAgIGZvcihhdXRvICZpIDogKHYpKQoKdGVtcGxhdGU8Y2xhc3MgVD4gdXNpbmcgVHJlZSA9IHRyZWU8VCwgbnVsbF90eXBlLCBsZXNzPFQ+LCByYl90cmVlX3RhZyx0cmVlX29yZGVyX3N0YXRpc3RpY3Nfbm9kZV91cGRhdGU+Owp0ZW1wbGF0ZTx0eXBlbmFtZSBUPiBUIHBvdyhUIGEsIFQgYikgeyBUIHJlcyA9IDE7IGYwcihpLCAwLCBiKSByZXMgPSByZXMgKiBhOyByZXR1cm4gcmVzOyB9CnRlbXBsYXRlPHR5cGVuYW1lIFQ+IHZvaWQgY2ttYXgoVCAmYSwgVCBiKSB7IGEgPSBtYXgoYSwgYik7ICB9CnRlbXBsYXRlPHR5cGVuYW1lIFQ+IHZvaWQgY2ttaW4oVCAmYSwgVCBiKSB7IGEgPSBtaW4oYSwgYik7ICB9CgppbnQgZHg0W10gPSB7MCwgMSwgMCwgLTF9OwppbnQgZHk0W10gPSB7MSwgMCwgLTEsIDB9OwppbnQgZHg4W10gPSB7LTEsIC0xLCAtMSwgMCwgMSwgMSwgMSwgMH07CmludCBkeThbXSA9IHstMSwgMCwgMSwgMSwgMSwgMCwgLTEsIC0xfTsKCmNvbnN0IGludCBOMiA9IDEwMDQsIE4gPSAyMDAwMDQ7CmNvbnN0IGxsIGxpbmYgPSAxMDAwMDAwMDAwMDAwMDAwMDAwOwpjb25zdCBpbnQgaW5mID0gMTAwMDAxMDAwMDsKCmludCBuLCBtLCB4LCB5Owp2ZWN0b3I8aW50PiB2KE4pLCBkcChOKTsKdmVjdG9yPHZlY3RvcjxpbnQ+PiBtYXQoTjIsIHZlY3RvcjxpbnQ+IChOMikpOwovLyA8PT09PT09PT09PT09PT09PT09PT09PT09PT09PT09PT09PT09PT09PT09PT09PT09PT09PT09PT09PT09PT09PT09PT09PT09PT09PT09PT09PT09PT09PT09PT09PT09PT09PgoKaW50IG1haW4oKXsKICAgY2luLnRpZShudWxscHRyKS0+c3luY193aXRoX3N0ZGlvKGZhbHNlKTsKCiAgIGNsb2NrX3QgdGltZV9yZXE7ICAKICAgdGltZV9yZXEgPSBjbG9jaygpOwogICAKICAgaW50IHR0ID0gMTsKICAgLy8gY2luID4+IHR0OwogICBmMHIoVCwgMSwgdHQgKyAxKSB7CiAgICAgIGNpbiA+PiBuID4+IG07CiAgICAgIHN0cmluZyBzOwogICAgICBjaW4gPj4gczsKICAgICAgLS1tOwogICAgICB3aGlsZShzeihzKSAmJiBtKSB7CiAgICAgICAgIHMucG9wX2JhY2soKTsKICAgICAgICAgLS1tOwogICAgICB9CiAgICAgIGNvdXQgPDwgcy5iYWNrKCkgOwogICB9CiAgIAogICB0aW1lX3JlcSA9IGNsb2NrKCkgLSB0aW1lX3JlcTsKLy8gICAgY2VyciA8PCAiVGltZSBUYWtlbjogIjw8IChmbG9hdCl0aW1lX3JlcS9DTE9DS1NfUEVSX1NFQyA8PCAiIHNlY29uZHMiIDw8ICJcbiI7CiAgIAogICByZXR1cm4gMDsKfQoK
Solution 1: Java
aW1wb3J0IGphdmEuaW8uKjsKaW1wb3J0IGphdmEudXRpbC4qOwppbXBvcnQgamF2YS50ZXh0Lio7CmltcG9ydCBqYXZhLm1hdGguKjsKaW1wb3J0IGphdmEudXRpbC5yZWdleC4qOwoKY2xhc3MgTWFpbiB7CgogICAgcHVibGljIHN0YXRpYyB2b2lkIG1haW4oU3RyaW5nW10gYXJncykgewogICAgICAgIC8qIEVudGVyIHlvdXIgY29kZSBoZXJlLiBSZWFkIGlucHV0IGZyb20gU1RESU4uIFByaW50IG91dHB1dCB0byBTVERPVVQuIFlvdXIgY2xhc3Mgc2hvdWxkIGJlIG5hbWVkIFNvbHV0aW9uLiAqLwogICAgICAgIFNjYW5uZXIgc2MgPSBuZXcgU2Nhbm5lcihTeXN0ZW0uaW4pOwogICAgICAgIGludCBuID0gc2MubmV4dEludCgpOwogICAgICAgIGludCBrID0gc2MubmV4dEludCgpOwogICAgICAgIFN0cmluZyBuYW1lcyA9IHNjLm5leHQoKTsKICAgICAgICBTeXN0ZW0ub3V0LnByaW50bG4obmFtZXMuY2hhckF0KG4taykpOyAgIAogICAgfQp9Cg==
Solution 3: Python
IyBFbnRlciB5b3VyIGNvZGUgaGVyZS4gUmVhZCBpbnB1dCBmcm9tIFNURElOLiBQcmludCBvdXRwdXQgdG8gU1RET1VUDQpuLCBrID0gbWFwKGludCwgaW5wdXQoKS5zcGxpdCgpKQ0KcyA9IGlucHV0KCkNCnByaW50KHNbOjotMV1bay0xXSkNCg==
Problem Statement 2
Alice challenged Bob to write the same word as his on a typewriter. Both are kids and are making some mistakes in typing and are making use of the ‘#’ key on a typewriter to delete the last character printed on it.
An empty text remains empty even after backspaces.
Input Format
The first line contains a string typed by Bob.
The second line contains a string typed by Alice.
Output Format
The first line contains ‘YES’ if Alice is able to print the exact words as Bob , otherwise ‘NO’.
Constraints
1 <= Bob.length
Alice.length <= 100000
Bob and Alice only contain lowercase letters and '#' characters.
Testcase Input
ab#c
ad#c
Testcase Output
YES
Solution 1: C++
I2luY2x1ZGUgPGJpdHMvc3RkYysrLmg+CnVzaW5nIG5hbWVzcGFjZSBzdGQ7CgovLyBGdW5jdGlvbiB0byBwcm9jZXNzIHRoZSBzdHJpbmcgYWNjb3JkaW5nIHRvIHRoZSB0eXBld3JpdGVyIHJ1bGVzCnN0cmluZyBwcm9jZXNzU3RyaW5nKGNvbnN0IHN0ZDo6c3RyaW5nICZzKSB7CiAgICBzdGFjazxjaGFyPiBzdDsKICAgIGZvciAoY2hhciBjIDogcykgewogICAgICAgIGlmIChjID09ICcjJykgewogICAgICAgICAgICBpZiAoIXN0LmVtcHR5KCkpIHsKICAgICAgICAgICAgICAgIHN0LnBvcCgpOwogICAgICAgICAgICB9CiAgICAgICAgfSBlbHNlIHsKICAgICAgICAgICAgc3QucHVzaChjKTsKICAgICAgICB9CiAgICB9CiAgICAKICAgIC8vIEJ1aWxkIHRoZSByZXN1bHRpbmcgc3RyaW5nIGZyb20gdGhlIHN0YWNrCiAgICBzdHJpbmcgcmVzdWx0OwogICAgd2hpbGUgKCFzdC5lbXB0eSgpKSB7CiAgICAgICAgcmVzdWx0ICs9IHN0LnRvcCgpOwogICAgICAgIHN0LnBvcCgpOwogICAgfQogICAgCiAgICAvLyBSZXZlcnNlIHRoZSByZXN1bHQgYmVjYXVzZSBzdGFjayBnaXZlcyB1cyBjaGFyYWN0ZXJzIGluIHJldmVyc2Ugb3JkZXIKICAgIHJldmVyc2UocmVzdWx0LmJlZ2luKCksIHJlc3VsdC5lbmQoKSk7CiAgICByZXR1cm4gcmVzdWx0Owp9CgppbnQgbWFpbigpIHsKICAgIHN0cmluZyBib2IsIGFsaWNlOwogICAgY2luID4+IGJvYjsKICAgIGNpbiA+PiBhbGljZTsKICAgIAogICAgc3RyaW5nIHByb2Nlc3NlZEJvYiA9IHByb2Nlc3NTdHJpbmcoYm9iKTsKICAgIHN0cmluZyBwcm9jZXNzZWRBbGljZSA9IHByb2Nlc3NTdHJpbmcoYWxpY2UpOwogICAgCiAgICBpZiAocHJvY2Vzc2VkQm9iID09IHByb2Nlc3NlZEFsaWNlKSB7CiAgICAgICAgY291dCA8PCAiWUVTIiA8PCBlbmRsOwogICAgfSBlbHNlIHsKICAgICAgICBjb3V0IDw8ICJOTyIgPDwgZW5kbDsKICAgIH0KICAgIAogICAgcmV0dXJuIDA7Cn0K
Solution 2: Python
IyBFbnRlciB5b3VyIGNvZGUgaGVyZS4gUmVhZCBpbnB1dCBmcm9tIFNURElOLiBQcmludCBvdXRwdXQgdG8gU1RET1VUDQpkZWYgcHJvY2Vzc19zdHJpbmcocyk6DQogICAgcmVzdWx0ID0gW10NCiAgICBmb3IgY2hhciBpbiBzOg0KICAgICAgICBpZiBjaGFyID09ICcjJzoNCiAgICAgICAgICAgIGlmIHJlc3VsdDoNCiAgICAgICAgICAgICAgICByZXN1bHQucG9wKCkNCiAgICAgICAgZWxzZToNCiAgICAgICAgICAgIHJlc3VsdC5hcHBlbmQoY2hhcikNCiAgICByZXR1cm4gJycuam9pbihyZXN1bHQpDQoNCmRlZiBjb21wYXJlX3N0cmluZ3MoYm9iLCBhbGljZSk6DQogICAgcmV0dXJuIHByb2Nlc3Nfc3RyaW5nKGJvYikgPT0gcHJvY2Vzc19zdHJpbmcoYWxpY2UpDQoNCiMgSW5wdXQNCmJvYiA9IGlucHV0KCkuc3RyaXAoKQ0KYWxpY2UgPSBpbnB1dCgpLnN0cmlwKCkNCg0KIyBPdXRwdXQNCmlmIGNvbXBhcmVfc3RyaW5ncyhib2IsIGFsaWNlKToNCiAgICBwcmludCgiWUVTIikNCmVsc2U6DQogICAgcHJpbnQoIk5PIikNCg==
Solution 3: Java
ICBpbXBvcnQgamF2YS51dGlsLlN0YWNrOwppbXBvcnQgamF2YS51dGlsLio7CgpwdWJsaWMgY2xhc3MgTWFpbiB7CgogICAgcHVibGljIHN0YXRpYyBib29sZWFuIGJhY2tzcGFjZUNvbXBhcmUoU3RyaW5nIGJvYiwgU3RyaW5nIGFsaWNlKSB7CiAgICAgICAgU3RyaW5nIGJvYlByb2Nlc3NlZCA9IHByb2Nlc3NTdHJpbmcoYm9iKTsKICAgICAgICBTdHJpbmcgYWxpY2VQcm9jZXNzZWQgPSBwcm9jZXNzU3RyaW5nKGFsaWNlKTsKICAgICAgICByZXR1cm4gYm9iUHJvY2Vzc2VkLmVxdWFscyhhbGljZVByb2Nlc3NlZCk7CiAgICB9CgogICAgcHJpdmF0ZSBzdGF0aWMgU3RyaW5nIHByb2Nlc3NTdHJpbmcoU3RyaW5nIHMpIHsKICAgICAgICBTdGFjazxDaGFyYWN0ZXI+IHN0YWNrID0gbmV3IFN0YWNrPD4oKTsKICAgICAgICBmb3IgKGNoYXIgYyA6IHMudG9DaGFyQXJyYXkoKSkgewogICAgICAgICAgICBpZiAoYyA9PSAnIycpIHsKICAgICAgICAgICAgICAgIGlmICghc3RhY2suaXNFbXB0eSgpKSB7CiAgICAgICAgICAgICAgICAgICAgc3RhY2sucG9wKCk7CiAgICAgICAgICAgICAgICB9CiAgICAgICAgICAgIH0gZWxzZSB7CiAgICAgICAgICAgICAgICBzdGFjay5wdXNoKGMpOwogICAgICAgICAgICB9CiAgICAgICAgfQogICAgICAgIFN0cmluZ0J1aWxkZXIgc2IgPSBuZXcgU3RyaW5nQnVpbGRlcigpOwogICAgICAgIGZvciAoY2hhciBjIDogc3RhY2spIHsKICAgICAgICAgICAgc2IuYXBwZW5kKGMpOwogICAgICAgIH0KICAgICAgICByZXR1cm4gc2IudG9TdHJpbmcoKTsKICAgIH0KCiAgICBwdWJsaWMgc3RhdGljIHZvaWQgbWFpbihTdHJpbmdbXSBhcmdzKSB7CiAgICAgICAgLy8gVGVzdCBjYXNlIDAKICAgICAgICBTY2FubmVyIHNjID0gbmV3IFNjYW5uZXIoU3lzdGVtLmluKTsKICAgICAgICBTdHJpbmcgc3RyMSA9IHNjLm5leHRMaW5lKCk7IAogICAgICAgIFN0cmluZyBzdHIyID0gc2MubmV4dExpbmUoKTsKICAgICAgICBTeXN0ZW0ub3V0LnByaW50bG4oKGJhY2tzcGFjZUNvbXBhcmUoc3RyMSxzdHIyKSA/ICJZRVMiIDogIk5PIikpOwoKICAgICAgCiAgICB9Cn0K
Problem Statement 3
Ram gave Shyaam a challenge, he gave shyaam the head of a linked list, and an integer k. He asked Shyaam to swap the values of the Kth node from the beginning and the Kth node from the end (the list is 1-indexed).
Note: The number of nodes in the list is N.
Input Format
The first line contains an integer N, representing the number of nodes in the linked list.
The second line contains N space-separated integers, each representing the value of a node in the linked list.
The third line contains an integer K, indicating the positions of the nodes to be swapped.
Output Format
Output the linked list after swapping the values of the two specified nodes.
Constraints
1 <= K <= N <= 10^5
0 <= Node.val <= 10^2
Testcase Input
5
1 2 3 4 5
2
Testcase Output
1 4 3 2 5
Solution 1: Java
aW1wb3J0IGphdmEuaW8uKjsKaW1wb3J0IGphdmEudXRpbC4qOwppbXBvcnQgamF2YS50ZXh0Lio7CmltcG9ydCBqYXZhLm1hdGguKjsKaW1wb3J0IGphdmEudXRpbC5yZWdleC4qOwoKcHVibGljIGNsYXNzICBNYWluIHsKCiAgICBwdWJsaWMgc3RhdGljIHZvaWQgbWFpbihTdHJpbmdbXSBhcmdzKSB7CiAgICAgICAgLyogRW50ZXIgeW91ciBjb2RlIGhlcmUuIFJlYWQgaW5wdXQgZnJvbSBTVERJTi4gUHJpbnQgb3V0cHV0IHRvIFNURE9VVC4gWW91ciBjbGFzcyBzaG91bGQgYmUgbmFtZWQgU29sdXRpb24uICovCiAgICBMaW5rZWRMaXN0PEludGVnZXI+IGwgPSBuZXcgTGlua2VkTGlzdDw+KCk7CiAgICBTY2FubmVyIGluID0gbmV3IFNjYW5uZXIoU3lzdGVtLmluKTsKICAgIGludCBuPSBpbi5uZXh0SW50KCk7CiAgICBmb3IoaW50IGk9MDtpPG47aSsrKXsKICAgICAgICBsLmFkZChpbi5uZXh0SW50KCkpOwogICAgfQogICAgaW50IGs9aW4ubmV4dEludCgpOwogICAgaW50IHRlbXA9bC5nZXQoay0xKTsKICAgIGwuc2V0KGstMSxsLmdldChuLWspKTsKICAgIGwuc2V0KG4tayx0ZW1wKTsKICAgIGZvcihpbnQgZTogbCl7CiAgICAgICAgU3lzdGVtLm91dC5wcmludChlKyIgIik7CiAgICB9CiAgICB9Cn0K
Solution 2: C++
I2luY2x1ZGUgPGxpc3Q+CiNpbmNsdWRlIDxpb3N0cmVhbT4KI2luY2x1ZGUgPGl0ZXJhdG9yPgp1c2luZyBuYW1lc3BhY2Ugc3RkOwppbnQgbWFpbigpIHsKICAgIC8qIEVudGVyIHlvdXIgY29kZSBoZXJlLiBSZWFkIGlucHV0IGZyb20gU1RESU4uIFByaW50IG91dHB1dCB0byBTVERPVVQgKi8gCiAgICBpbnQgbjsKICAgIGNpbj4+bjsKICAgIGxpc3Q8aW50PiBMMTsKICAgIGZvcihpbnQgaT0wO2k8bjtpKyspewogICAgICAgIGludCBkdW1teTsKICAgICAgICBjaW4+PmR1bW15OwogICAgICAgIEwxLnB1c2hfYmFjayhkdW1teSk7CiAgICB9IAogICAgaW50IGluZGV4OwogICAgY2luPj5pbmRleDsKICAgIGxpc3Q8aW50Pjo6aXRlcmF0b3IgaXRyOwogICAgbGlzdDxpbnQ+OjpyZXZlcnNlX2l0ZXJhdG9yIHB0cjsKICAgIGludCBjb3VudGVyPTA7CiAgICBmb3IoaXRyPUwxLmJlZ2luKCkscHRyPUwxLnJiZWdpbigpO2l0ciE9TDEuZW5kKCkgJiYgcHRyIT1MMS5yZW5kKCk7aXRyKysscHRyKyspewogICAgICAgIGlmKGNvdW50ZXI9PWluZGV4LTEpewogICAgICAgICAgICBpbnQgdGVtcD0qaXRyOwogICAgICAgICAgICAqaXRyPSpwdHI7CiAgICAgICAgICAgICpwdHI9dGVtcDsKICAgICAgICB9CiAgICAgICAgY291bnRlcisrOwogICAgfQogICAgZm9yKGF1dG8gaXQ9TDEuYmVnaW4oKTtpdCE9TDEuZW5kKCk7aXQrKyl7CiAgICAgICAgY291dDw8Kml0PDwiICI7CiAgICB9CiAgICByZXR1cm4gMDsKfQo=
Solution 3: Python
IyBFbnRlciB5b3VyIGNvZGUgaGVyZS4gUmVhZCBpbnB1dCBmcm9tIFNURElOLiBQcmludCBvdXRwdXQgdG8gU1RET1VUDQpjbGFzcyBMaXN0Tm9kZToNCiAgICBkZWYgX19pbml0X18oc2VsZiwgdmFsPTAsIG5leHQ9Tm9uZSk6DQogICAgICAgIHNlbGYudmFsID0gdmFsDQogICAgICAgIHNlbGYubmV4dCA9IG5leHQNCg0KIyBIZWxwZXIgZnVuY3Rpb24gdG8gY3JlYXRlIGEgbGlua2VkIGxpc3QgZnJvbSBhIGxpc3Qgb2YgdmFsdWVzDQpkZWYgY3JlYXRlX2xpbmtlZF9saXN0KHZhbHVlcyk6DQogICAgaWYgbm90IHZhbHVlczoNCiAgICAgICAgcmV0dXJuIE5vbmUNCiAgICBoZWFkID0gTGlzdE5vZGUodmFsdWVzWzBdKQ0KICAgIGN1cnJlbnQgPSBoZWFkDQogICAgZm9yIHZhbHVlIGluIHZhbHVlc1sxOl06DQogICAgICAgIGN1cnJlbnQubmV4dCA9IExpc3ROb2RlKHZhbHVlKQ0KICAgICAgICBjdXJyZW50ID0gY3VycmVudC5uZXh0DQogICAgcmV0dXJuIGhlYWQNCg0KIyBIZWxwZXIgZnVuY3Rpb24gdG8gY29udmVydCBhIGxpbmtlZCBsaXN0IGJhY2sgdG8gYSBQeXRob24gbGlzdA0KZGVmIGxpbmtlZF9saXN0X3RvX2xpc3QoaGVhZCk6DQogICAgdmFsdWVzID0gW10NCiAgICBjdXJyZW50ID0gaGVhZA0KICAgIHdoaWxlIGN1cnJlbnQ6DQogICAgICAgIHZhbHVlcy5hcHBlbmQoY3VycmVudC52YWwpDQogICAgICAgIGN1cnJlbnQgPSBjdXJyZW50Lm5leHQNCiAgICByZXR1cm4gdmFsdWVzDQoNCiMgRnVuY3Rpb24gdG8gc3dhcCB0aGUgS3RoIG5vZGUgZnJvbSB0aGUgc3RhcnQgYW5kIHRoZSBLdGggbm9kZSBmcm9tIHRoZSBlbmQNCmRlZiBzd2FwX25vZGVzKGhlYWQsIE4sIEspOg0KICAgIGlmIG5vdCBoZWFkIG9yIEsgPiBOOg0KICAgICAgICByZXR1cm4gaGVhZA0KICAgIA0KICAgICMgRmluZCBLdGggbm9kZSBmcm9tIHRoZSBzdGFydA0KICAgIGZpcnN0X25vZGUgPSBoZWFkDQogICAgZm9yIF8gaW4gcmFuZ2UoSyAtIDEpOg0KICAgICAgICBmaXJzdF9ub2RlID0gZmlyc3Rfbm9kZS5uZXh0DQogICAgDQogICAgIyBGaW5kIEt0aCBub2RlIGZyb20gdGhlIGVuZCAoTi1LKzEgdGggbm9kZSBmcm9tIHRoZSBzdGFydCkNCiAgICBzZWNvbmRfbm9kZSA9IGhlYWQNCiAgICBmb3IgXyBpbiByYW5nZShOIC0gSyk6DQogICAgICAgIHNlY29uZF9ub2RlID0gc2Vjb25kX25vZGUubmV4dA0KICAgIA0KICAgICMgU3dhcCB0aGUgdmFsdWVzIG9mIHRoZSB0d28gbm9kZXMNCiAgICBmaXJzdF9ub2RlLnZhbCwgc2Vjb25kX25vZGUudmFsID0gc2Vjb25kX25vZGUudmFsLCBmaXJzdF9ub2RlLnZhbA0KICAgIA0KICAgIHJldHVybiBoZWFkDQoNCiMgTWFpbiBmdW5jdGlvbiB0byBoYW5kbGUgaW5wdXQvb3V0cHV0DQppZiBfX25hbWVfXyA9PSAiX19tYWluX18iOg0KICAgICMgUmVhZCBpbnB1dHMNCiAgICBOID0gaW50KGlucHV0KCkpICAjIE51bWJlciBvZiBub2RlcyBpbiB0aGUgbGlua2VkIGxpc3QNCiAgICB2YWx1ZXMgPSBsaXN0KG1hcChpbnQsIGlucHV0KCkuc3BsaXQoKSkpICAjIFRoZSBsaXN0IG9mIG5vZGUgdmFsdWVzDQogICAgSyA9IGludChpbnB1dCgpKSAgIyBQb3NpdGlvbiBLDQogICAgDQogICAgIyBDcmVhdGUgbGlua2VkIGxpc3QgZnJvbSB0aGUgaW5wdXQgdmFsdWVzDQogICAgaGVhZCA9IGNyZWF0ZV9saW5rZWRfbGlzdCh2YWx1ZXMpDQogICAgDQogICAgIyBTd2FwIHRoZSBLdGggbm9kZSBmcm9tIHRoZSBzdGFydCB3aXRoIHRoZSBLdGggbm9kZSBmcm9tIHRoZSBlbmQNCiAgICBoZWFkID0gc3dhcF9ub2RlcyhoZWFkLCBOLCBLKQ0KICAgIA0KICAgICMgQ29udmVydCB0aGUgbGlua2VkIGxpc3QgYmFjayB0byBhIGxpc3QgYW5kIHByaW50IHRoZSByZXN1bHQNCiAgICByZXN1bHQgPSBsaW5rZWRfbGlzdF90b19saXN0KGhlYWQpDQogICAgcHJpbnQoIiAiLmpvaW4obWFwKHN0ciwgcmVzdWx0KSkpDQo=
Problem Statement 4
There are n spaceship at given lightyears away from the earth and traveling to reach a distant star system at k lightyear away from earth. You are given two integer arrays, position and speed, both of length n, where
P[i] is the current distance of the ith spaceship
S[i] is the speed of the ith spaceship in lightyears per year.
As the spaceships travel toward the star system, an interesting phenomenon occurs: when a faster spaceship catches up to a slower one, it reduces its speed to match the slower spaceship's speed, forming a fleet. A fleet is a group of one or more spaceships that travel together at the same speed.
Given this information, determine the number of distinct spacecraft fleets that will arrive at the destination star system. A fleet is considered distinct if no other fleet arrives at the destination at the same time while traveling together.
Input Format
The first line contains an integer 'n', representing the total number of spaceships.
The second line contains an integer 'k', representing the distance of the star system from Earth.
The third line contains 'n' space-separated integers denoting the current distance of the i-th spaceship from Earth.
The fourth line contains 'n' space-separated integers denoting the speed of the i-th spaceship.
Output Format
Return the number of spacecraft fleets that will arrive at the destination.
Constraints
1 <= n <= 10^5
0 < k <= 10^6
0 <= P[i] < target
0 < S[i] <= 10
Testcase Input
4
14
10 8 5 3
2 4 1 3
Testcase Output
2
Solution 1: Python
IyBFbnRlciB5b3VyIGNvZGUgaGVyZS4gUmVhZCBpbnB1dCBmcm9tIFNURElOLiBQcmludCBvdXRwdXQgdG8gU1RET1VUDQpkZWYgY291bnRfZmxlZXRzKG4sIGssIHBvc2l0aW9uLCBzcGVlZCk6DQogICAgIyBTdGVwIDE6IENhbGN1bGF0ZSB0aGUgdGltZSBmb3IgZWFjaCBzcGFjZXNoaXAgdG8gcmVhY2ggdGhlIHRhcmdldA0KICAgIHRpbWUgPSBbKGsgLSBwb3NpdGlvbltpXSkgLyBzcGVlZFtpXSBmb3IgaSBpbiByYW5nZShuKV0NCiAgICANCiAgICAjIFN0ZXAgMjogU29ydCBzcGFjZXNoaXBzIGJ5IHRoZWlyIHBvc2l0aW9uIGZyb20gZmFydGhlc3QgdG8gY2xvc2VzdA0KICAgIHNoaXBzID0gc29ydGVkKHppcChwb3NpdGlvbiwgdGltZSksIHJldmVyc2U9VHJ1ZSkNCiAgICANCiAgICAjIFN0ZXAgMzogQ291bnQgdGhlIG51bWJlciBvZiBkaXN0aW5jdCBmbGVldHMNCiAgICBmbGVldHMgPSAwDQogICAgY3VycmVudF90aW1lID0gMA0KICAgIA0KICAgIGZvciBfLCB0IGluIHNoaXBzOg0KICAgICAgICBpZiB0ID4gY3VycmVudF90aW1lOg0KICAgICAgICAgICAgZmxlZXRzICs9IDENCiAgICAgICAgICAgIGN1cnJlbnRfdGltZSA9IHQNCiAgICANCiAgICByZXR1cm4gZmxlZXRzDQoNCiMgSW5wdXQNCm4gPSBpbnQoaW5wdXQoKSkgICMgTnVtYmVyIG9mIHNwYWNlc2hpcHMNCmsgPSBpbnQoaW5wdXQoKSkgICMgVGFyZ2V0IGRpc3RhbmNlDQpwb3NpdGlvbiA9IGxpc3QobWFwKGludCwgaW5wdXQoKS5zcGxpdCgpKSkgICMgQ3VycmVudCBwb3NpdGlvbnMgb2YgdGhlIHNwYWNlc2hpcHMNCnNwZWVkID0gbGlzdChtYXAoaW50LCBpbnB1dCgpLnNwbGl0KCkpKSAgIyBTcGVlZHMgb2YgdGhlIHNwYWNlc2hpcHMNCg0KIyBPdXRwdXQgdGhlIG51bWJlciBvZiBmbGVldHMNCnByaW50KGNvdW50X2ZsZWV0cyhuLCBrLCBwb3NpdGlvbiwgc3BlZWQpKQ0K
Solution 2: C++
I2luY2x1ZGUgPGlvc3RyZWFtPgojaW5jbHVkZSA8dmVjdG9yPgojaW5jbHVkZSA8YWxnb3JpdGhtPgoKdXNpbmcgbmFtZXNwYWNlIHN0ZDsKCmludCBjb3VudEZsZWV0cyhpbnQgbiwgaW50IGssIHZlY3RvcjxpbnQ+JiBwb3NpdGlvbiwgdmVjdG9yPGludD4mIHNwZWVkKSB7CiAgICB2ZWN0b3I8cGFpcjxpbnQsIGRvdWJsZT4+IHNoaXBzKG4pOyAvLyBUbyBzdG9yZSBwb3NpdGlvbnMgYW5kIGNvcnJlc3BvbmRpbmcgdGltZXMgdG8gcmVhY2ggdGhlIHRhcmdldAogICAgCiAgICAvLyBDYWxjdWxhdGUgdGhlIHRpbWUgZm9yIGVhY2ggc3BhY2VzaGlwIHRvIHJlYWNoIHRoZSB0YXJnZXQKICAgIGZvciAoaW50IGkgPSAwOyBpIDwgbjsgaSsrKSB7CiAgICAgICAgZG91YmxlIHRpbWUgPSAoZG91YmxlKShrIC0gcG9zaXRpb25baV0pIC8gc3BlZWRbaV07CiAgICAgICAgc2hpcHNbaV0gPSB7cG9zaXRpb25baV0sIHRpbWV9OwogICAgfQogICAgCiAgICAvLyBTb3J0IHNoaXBzIGJhc2VkIG9uIHRoZWlyIHN0YXJ0aW5nIHBvc2l0aW9ucyAoZmFydGhlc3QgZmlyc3QpCiAgICBzb3J0KHNoaXBzLmJlZ2luKCksIHNoaXBzLmVuZCgpLCBncmVhdGVyPHBhaXI8aW50LCBkb3VibGU+PigpKTsKICAgIAogICAgaW50IGZsZWV0cyA9IDA7CiAgICBkb3VibGUgbGFzdEZsZWV0VGltZSA9IDAuMDsKICAgIAogICAgLy8gSXRlcmF0ZSBvdmVyIHNvcnRlZCBzaGlwcyB0byBjb3VudCBmbGVldHMKICAgIGZvciAoaW50IGkgPSAwOyBpIDwgbjsgaSsrKSB7CiAgICAgICAgaWYgKHNoaXBzW2ldLnNlY29uZCA+IGxhc3RGbGVldFRpbWUpIHsKICAgICAgICAgICAgZmxlZXRzKys7CiAgICAgICAgICAgIGxhc3RGbGVldFRpbWUgPSBzaGlwc1tpXS5zZWNvbmQ7IC8vIFVwZGF0ZSB0aGUgbGF0ZXN0IGZsZWV0J3MgdGltZQogICAgICAgIH0KICAgIH0KICAgIAogICAgcmV0dXJuIGZsZWV0czsKfQoKaW50IG1haW4oKSB7CiAgICBpbnQgbiwgazsKICAgIGNpbiA+PiBuID4+IGs7CiAgICAKICAgIHZlY3RvcjxpbnQ+IHBvc2l0aW9uKG4pLCBzcGVlZChuKTsKICAgIGZvciAoaW50IGkgPSAwOyBpIDwgbjsgaSsrKSB7CiAgICAgICAgY2luID4+IHBvc2l0aW9uW2ldOwogICAgfQogICAgCiAgICBmb3IgKGludCBpID0gMDsgaSA8IG47IGkrKykgewogICAgICAgIGNpbiA+PiBzcGVlZFtpXTsKICAgIH0KICAgIAogICAgLy8gT3V0cHV0IHRoZSBudW1iZXIgb2YgZGlzdGluY3QgZmxlZXRzCiAgICBjb3V0IDw8IGNvdW50RmxlZXRzKG4sIGssIHBvc2l0aW9uLCBzcGVlZCkgPDwgZW5kbDsKICAgIAogICAgcmV0dXJuIDA7Cn0K
Solution 3: Java
aW1wb3J0IGphdmEudXRpbC4qOwoKcHVibGljIGNsYXNzIE1haW4gewogICAgcHVibGljIHN0YXRpYyBpbnQgY291bnRGbGVldHMoaW50IG4sIGludCB0YXJnZXQsIGludFtdIHBvc2l0aW9uLCBpbnRbXSBzcGVlZCkgewogICAgICAgIExpc3Q8UGFpcj4gc29ydGVkID0gbmV3IEFycmF5TGlzdDw+KCk7IC8vIFN0b3JlIHBvc2l0aW9uIGFuZCB0aW1lIHRvIHJlYWNoIHRhcmdldAoKICAgICAgICAvLyBDYWxjdWxhdGUgdGltZSB0YWtlbiBieSBlYWNoIHNwYWNlY3JhZnQgdG8gcmVhY2ggdGFyZ2V0CiAgICAgICAgZm9yIChpbnQgaSA9IDA7IGkgPCBuOyArK2kpIHsKICAgICAgICAgICAgc29ydGVkLmFkZChuZXcgUGFpcihwb3NpdGlvbltpXSwgKGRvdWJsZSkodGFyZ2V0IC0gcG9zaXRpb25baV0pIC8gc3BlZWRbaV0pKTsKICAgICAgICB9CgogICAgICAgIC8vIFNvcnQgc3BhY2VjcmFmdCBieSBwb3NpdGlvbiBpbiBkZXNjZW5kaW5nIG9yZGVyCiAgICAgICAgQ29sbGVjdGlvbnMuc29ydChzb3J0ZWQsIChhLCBiKSAtPiBiLnBvc2l0aW9uIC0gYS5wb3NpdGlvbik7CgogICAgICAgIGludCBmbGVldHMgPSAwOwogICAgICAgIGRvdWJsZSBtYXhUaW1lID0gMDsKCiAgICAgICAgLy8gSXRlcmF0ZSBvdmVyIHNvcnRlZCBzcGFjZWNyYWZ0CiAgICAgICAgZm9yIChQYWlyIHAgOiBzb3J0ZWQpIHsKICAgICAgICAgICAgaWYgKHAudGltZSA+IG1heFRpbWUpIHsKICAgICAgICAgICAgICAgIG1heFRpbWUgPSBwLnRpbWU7CiAgICAgICAgICAgICAgICBmbGVldHMrKzsgLy8gSW5jcmVtZW50IGZsZWV0IGNvdW50IGlmIHNwYWNlY3JhZnQgdGFrZXMgbW9yZSB0aW1lIHRvIHJlYWNoIHRhcmdldAogICAgICAgICAgICB9CiAgICAgICAgfQoKICAgICAgICByZXR1cm4gZmxlZXRzOwogICAgfQoKICAgIHB1YmxpYyBzdGF0aWMgdm9pZCBtYWluKFN0cmluZ1tdIGFyZ3MpIHsKICAgICAgICBTY2FubmVyIHNjID0gbmV3IFNjYW5uZXIoU3lzdGVtLmluKTsKICAgICAgICAKICAgICAgICBpbnQgbiA9IHNjLm5leHRJbnQoKTsKICAgICAgICBpbnQgdGFyZ2V0ID0gc2MubmV4dEludCgpOwogICAgICAgIAogICAgICAgIGludFtdIHBvc2l0aW9uID0gbmV3IGludFtuXTsKICAgICAgICBpbnRbXSBzcGVlZCA9IG5ldyBpbnRbbl07CgogICAgICAgIGZvciAoaW50IGkgPSAwOyBpIDwgbjsgKytpKSB7CiAgICAgICAgICAgIHBvc2l0aW9uW2ldID0gc2MubmV4dEludCgpOwogICAgICAgIH0KCiAgICAgICAgZm9yIChpbnQgaSA9IDA7IGkgPCBuOyArK2kpIHsKICAgICAgICAgICAgc3BlZWRbaV0gPSBzYy5uZXh0SW50KCk7CiAgICAgICAgfQoKICAgICAgICBpbnQgcmVzdWx0ID0gY291bnRGbGVldHMobiwgdGFyZ2V0LCBwb3NpdGlvbiwgc3BlZWQpOwogICAgICAgIFN5c3RlbS5vdXQucHJpbnRsbihyZXN1bHQpOwogICAgfQoKICAgIHN0YXRpYyBjbGFzcyBQYWlyIHsKICAgICAgICBpbnQgcG9zaXRpb247CiAgICAgICAgZG91YmxlIHRpbWU7CgogICAgICAgIFBhaXIoaW50IHBvc2l0aW9uLCBkb3VibGUgdGltZSkgewogICAgICAgICAgICB0aGlzLnBvc2l0aW9uID0gcG9zaXRpb247CiAgICAgICAgICAgIHRoaXMudGltZSA9IHRpbWU7CiAgICAgICAgfQogICAgfQp9Cg==
Problem Statement 5
You are provided with a 2D array(N*M). Your task is to create an ArrayList of Node objects, where each row of the 2D array corresponds to one entry in the ArrayList. After that, a doubly-linked list is constructed, arranging nodes first by even indices from the ArrayList, followed by the odd indices.
Input Format
The first line contains an integer N, representing the size of the array row.
The second line contains an integer M, representing the size of array col.
The third line contains an array.
Output Format
return the linked list
Constraints
1 <= N < 10^2
1 <= M < 10^2
Testcase Input
4
6
2 4 5 3 6 7
5 3 6 3 6 7
3 6 7 2 6 8
4 2 1 6 8 9
Testcase Output
2 <---> 4 <---> 5 <---> 3 <---> 6 <---> 7 <---> 3 <---> 6 <---> 7 <---> 2 <---> 6 <---> 8 <---> 5 <---> 3 <---> 6 <---> 3 <---> 6 <---> 7 <---> 4 <---> 2 <---> 1 <---> 6 <---> 8 <---> 9 <---> null
Solution 1: Java
aW1wb3J0IGphdmEudXRpbC4qOwoKcHVibGljIGNsYXNzIE1haW4gewogIAogICAgc3RhdGljIGNsYXNzIGRvdWJsZU5vZGUgewogICAgICAgIGludCBkb3VibGVkYXRhOwogICAgICAgIGRvdWJsZU5vZGUgZG91YmxlcHJldjsKICAgICAgICBkb3VibGVOb2RlIGRvdWJsZW5leHQ7CgogICAgICAgZG91YmxlTm9kZShpbnQgZG91YmxlZGF0YSkgewogICAgICAgICAgICB0aGlzLmRvdWJsZWRhdGEgPSBkb3VibGVkYXRhOwogICAgICAgIH0KICAgIH0gIAogIAogICAgc3RhdGljIGNsYXNzIE5vZGUgewogICAgICAgIGludCBkYXRhOwogICAgICAgIE5vZGUgbmV4dDsKCiAgICAgICAgTm9kZShpbnQgZGF0YSkgewogICAgICAgICAgICB0aGlzLmRhdGEgPSBkYXRhOwogICAgICAgICAgICBuZXh0ID0gbnVsbDsKICAgICAgICB9CiAgICB9CiAgICBzdGF0aWMgdm9pZCBDcmVhdGVOb2RlTGlzdChpbnQgW11bXSBhcnIgLCAgTGlzdDxOb2RlPiBsaXN0ICl7CiAgICAgIAogICAgIGZvcihpbnQgaSA9IDAgOyBpPCBhcnIubGVuZ3RoOyBpKyspewogICAgICAgICBOb2RlIGhlYWQgPSBudWxsLCBwcmV2ID0gbnVsbDsKICAgICAgIGZvcihpbnQgaiA9IDA7IGogPCBhcnJbMF0ubGVuZ3RoOyBqKyspewogICAgICAgICBpZiAoaGVhZCA9PSBudWxsKSB7CiAgICAgICAgICAgICAgICAgICAgICAgIGhlYWQgPSBuZXcgTm9kZShhcnJbaV1bal0pOwogICAgICAgICAgICAgICAgICAgICAgICBwcmV2ID0gaGVhZDsKICAgICAgICAgICAgICAgICAgICB9IGVsc2UgewogICAgICAgICAgICAgICAgICAgICAgICBwcmV2Lm5leHQgPSBuZXcgTm9kZShhcnJbaV1bal0pOwogICAgICAgICAgICAgICAgICAgICAgICBwcmV2ID0gcHJldi5uZXh0OwogICAgICAgICAgICAgICAgICAgIH0KICAgICAgIH0KICAgICAgIGxpc3QuYWRkKGhlYWQpOwogICAgIH0KICAgIH0KICAgIAogICAgc3RhdGljIGRvdWJsZU5vZGUgQ3JlYXRlTGlua2VkTGlzdChMaXN0PE5vZGU+IGxpc3QgKXsKICAgICAgZG91YmxlTm9kZSBoZWFkID0gbnVsbCA7CiAgICAgIGRvdWJsZU5vZGUgdGFpbCA9IG51bGw7CiAgICAKICAgIGZvcihpbnQgaSA9MCA7IGkgPCBsaXN0LnNpemUoKTsgaSsrKXsKICAgICAgTm9kZSBubiA9IGxpc3QuZ2V0KGkpOwogICAgICB3aGlsZSggbm4gIT0gbnVsbCl7CiAgICAgICAgZG91YmxlTm9kZSBuID0gbmV3IGRvdWJsZU5vZGUobm4uZGF0YSk7CiAgICAgICAgICBpZihoZWFkID09IG51bGwpewogICAgICAgICAgICBoZWFkID0gbjsKICAgICAgICAgICAgdGFpbCA9IGhlYWQ7CiAgICAgICAgICAgIGhlYWQuZG91YmxlcHJldiA9IG51bGw7CiAgICAgICAgICAgIHRhaWwuZG91YmxlbmV4dCA9IG51bGw7CiAgICAgICAgICAgIAogICAgICAgICAgfWVsc2V7CiAgICAgICAgICAgIHRhaWwuZG91YmxlbmV4dCA9IG47CiAgICAgICAgICAgIG4uZG91YmxlcHJldiA9IHRhaWw7CiAgICAgICAgICAgIHRhaWwgPSBuOwogICAgICAgICAgICB0YWlsLmRvdWJsZW5leHQgPSBudWxsOwogICAgICAgICAgCiAgICAgICAgICB9CiAgICAgICAgICBubiA9IG5uLm5leHQ7CiAgICAgIH0KICAgICAgaSsrOwogICAgfQogICAgCiAgICAgZm9yKGludCBpID0xIDsgaSA8IGxpc3Quc2l6ZSgpOyBpKyspewogICAgICBOb2RlIG5uID0gbGlzdC5nZXQoaSk7CiAgICAgIAogICAgICAgd2hpbGUoIG5uICE9IG51bGwpewogICAgICAgICBkb3VibGVOb2RlIG4gPSBuZXcgZG91YmxlTm9kZShubi5kYXRhKTsKICAgICAgICAgIGlmKGhlYWQgPT0gbnVsbCl7CiAgICAgICAgICAgIGhlYWQgPSBuOwogICAgICAgICAgICB0YWlsID0gaGVhZDsKICAgICAgICAgICAgaGVhZC5kb3VibGVwcmV2ID0gbnVsbDsKICAgICAgICAgICAgdGFpbC5kb3VibGVuZXh0ID0gbnVsbDsKICAgICAgICAgICAgCiAgICAgICAgICB9ZWxzZXsKICAgICAgICAgICAgdGFpbC5kb3VibGVuZXh0ID0gbjsKICAgICAgICAgICAgbi5kb3VibGVwcmV2ID0gdGFpbDsKICAgICAgICAgICAgdGFpbCA9IG47CiAgICAgICAgICAgIHRhaWwuZG91YmxlbmV4dCA9IG51bGw7CiAgICAgICAgICAKICAgICAgICAgIH0KICAgICAgICAgICBubiA9IG5uLm5leHQ7CiAgICAgIH0KICAgICAgaSsrOwogICAgfQogICAgICAgIAogICAgICAgIAogICAgICByZXR1cm4gaGVhZDsKICAgIH0KCiAgICAgcHVibGljIHN0YXRpYyB2b2lkIG1haW4oU3RyaW5nW10gYXJncykgewogICAgICAgIFNjYW5uZXIgc2Nhbm5lciA9IG5ldyBTY2FubmVyKFN5c3RlbS5pbik7CiAgICAgICAgaW50IG4gPSBzY2FubmVyLm5leHRJbnQoKTsKICAgICAgICBpbnQgbSA9IHNjYW5uZXIubmV4dEludCgpOwogICAgICAgIGludFtdW10gYXJyID0gbmV3IGludFtuXVttXTsKCiAgICAgICAgLy8gSW5wdXQgdGhlIDJEIGFycmF5CiAgICAgICAgZm9yIChpbnQgaSA9IDA7IGkgPCBuOyBpKyspIHsKICAgICAgICAgICAgZm9yIChpbnQgaiA9IDA7IGogPCBtOyBqKyspIHsKICAgICAgICAgICAgICAgIGFycltpXVtqXSA9IHNjYW5uZXIubmV4dEludCgpOwogICAgICAgICAgICB9CiAgICAgICAgfQoKICAgICAgICAvLyBDcmVhdGUgbm9kZSBBcnJheUxpc3QKICAgICAgICBMaXN0PE5vZGU+IGxpc3QgPSBuZXcgQXJyYXlMaXN0PD4oKTsKICAgICAgICBDcmVhdGVOb2RlTGlzdChhcnIsIGxpc3QpOwoKICAgICAgICAvLyBDcmVhdGUgbGlua2VkIGxpc3QKICAgICAgICBkb3VibGVOb2RlIGN1cnJlbnQgPSBDcmVhdGVMaW5rZWRMaXN0KGxpc3QpOwoKICAgICAgICAvLyBQcmludCBsaW5rZWQgbGlzdAogICAgICAgIHdoaWxlIChjdXJyZW50ICE9IG51bGwpIHsKICAgICAgICAgICAgU3lzdGVtLm91dC5wcmludChjdXJyZW50LmRvdWJsZWRhdGEgKyAiIDwtLS0+ICIpOwogICAgICAgICAgICBjdXJyZW50ID0gY3VycmVudC5kb3VibGVuZXh0OwogICAgICAgIH0KICAgICAgICBTeXN0ZW0ub3V0LnByaW50KCJudWxsIik7CiAgICB9Cn0K
Solution 2: C++
I2luY2x1ZGUgPGlvc3RyZWFtPgojaW5jbHVkZSA8dmVjdG9yPgoKdXNpbmcgbmFtZXNwYWNlIHN0ZDsKCmNsYXNzIERvdWJsZU5vZGUgewpwdWJsaWM6CiAgICBpbnQgZG91YmxlZGF0YTsKICAgIERvdWJsZU5vZGUqIGRvdWJsZXByZXY7CiAgICBEb3VibGVOb2RlKiBkb3VibGVuZXh0OwoKICAgIERvdWJsZU5vZGUoaW50IGRhdGEpIHsKICAgICAgICB0aGlzLT5kb3VibGVkYXRhID0gZGF0YTsKICAgICAgICB0aGlzLT5kb3VibGVwcmV2ID0gbnVsbHB0cjsKICAgICAgICB0aGlzLT5kb3VibGVuZXh0ID0gbnVsbHB0cjsKICAgIH0KfTsKCmNsYXNzIE5vZGUgewpwdWJsaWM6CiAgICBpbnQgZGF0YTsKICAgIE5vZGUqIG5leHQ7CgogICAgTm9kZShpbnQgZGF0YSkgewogICAgICAgIHRoaXMtPmRhdGEgPSBkYXRhOwogICAgICAgIHRoaXMtPm5leHQgPSBudWxscHRyOwogICAgfQp9OwoKdm9pZCBjcmVhdGVOb2RlTGlzdCh2ZWN0b3I8dmVjdG9yPGludD4+JiBhcnIsIHZlY3RvcjxOb2RlKj4mIG5vZGVMaXN0KSB7CiAgICBmb3IgKGludCBpID0gMDsgaSA8IGFyci5zaXplKCk7IGkrKykgewogICAgICAgIE5vZGUqIGhlYWQgPSBudWxscHRyOwogICAgICAgIE5vZGUqIHByZXYgPSBudWxscHRyOwogICAgICAgIGZvciAoaW50IGogPSAwOyBqIDwgYXJyWzBdLnNpemUoKTsgaisrKSB7CiAgICAgICAgICAgIE5vZGUqIG5ld05vZGUgPSBuZXcgTm9kZShhcnJbaV1bal0pOwogICAgICAgICAgICBpZiAoIWhlYWQpIHsKICAgICAgICAgICAgICAgIGhlYWQgPSBuZXdOb2RlOwogICAgICAgICAgICB9IGVsc2UgewogICAgICAgICAgICAgICAgcHJldi0+bmV4dCA9IG5ld05vZGU7CiAgICAgICAgICAgIH0KICAgICAgICAgICAgcHJldiA9IG5ld05vZGU7CiAgICAgICAgfQogICAgICAgIG5vZGVMaXN0LnB1c2hfYmFjayhoZWFkKTsKICAgIH0KfQoKRG91YmxlTm9kZSogY3JlYXRlRG91YmxlTGlua2VkTGlzdCh2ZWN0b3I8Tm9kZSo+JiBub2RlTGlzdCkgewogICAgRG91YmxlTm9kZSogaGVhZCA9IG51bGxwdHI7CiAgICBEb3VibGVOb2RlKiB0YWlsID0gbnVsbHB0cjsKICAgIHZlY3RvcjxpbnQ+IHRlbXBMaXN0OwoKICAgIC8vIEFkZCBldmVuLWluZGV4IG5vZGVzIGZpcnN0CiAgICBmb3IgKGludCBpID0gMDsgaSA8IG5vZGVMaXN0LnNpemUoKTsgaSArPSAyKSB7CiAgICAgICAgTm9kZSogY3VycmVudCA9IG5vZGVMaXN0W2ldOwogICAgICAgIHdoaWxlIChjdXJyZW50KSB7CiAgICAgICAgICAgIHRlbXBMaXN0LnB1c2hfYmFjayhjdXJyZW50LT5kYXRhKTsKICAgICAgICAgICAgY3VycmVudCA9IGN1cnJlbnQtPm5leHQ7CiAgICAgICAgfQogICAgfQoKICAgIC8vIEFkZCBvZGQtaW5kZXggbm9kZXMgbmV4dAogICAgZm9yIChpbnQgaSA9IDE7IGkgPCBub2RlTGlzdC5zaXplKCk7IGkgKz0gMikgewogICAgICAgIE5vZGUqIGN1cnJlbnQgPSBub2RlTGlzdFtpXTsKICAgICAgICB3aGlsZSAoY3VycmVudCkgewogICAgICAgICAgICB0ZW1wTGlzdC5wdXNoX2JhY2soY3VycmVudC0+ZGF0YSk7CiAgICAgICAgICAgIGN1cnJlbnQgPSBjdXJyZW50LT5uZXh0OwogICAgICAgIH0KICAgIH0KCiAgICBmb3IgKGludCBpID0gMDsgaSA8IHRlbXBMaXN0LnNpemUoKTsgaSsrKSB7CiAgICAgICAgRG91YmxlTm9kZSogbmV3Tm9kZSA9IG5ldyBEb3VibGVOb2RlKHRlbXBMaXN0W2ldKTsKICAgICAgICBpZiAoIWhlYWQpIHsKICAgICAgICAgICAgaGVhZCA9IG5ld05vZGU7CiAgICAgICAgICAgIHRhaWwgPSBuZXdOb2RlOwogICAgICAgIH0gZWxzZSB7CiAgICAgICAgICAgIHRhaWwtPmRvdWJsZW5leHQgPSBuZXdOb2RlOwogICAgICAgICAgICBuZXdOb2RlLT5kb3VibGVwcmV2ID0gdGFpbDsKICAgICAgICAgICAgdGFpbCA9IG5ld05vZGU7CiAgICAgICAgfQogICAgfQogICAgcmV0dXJuIGhlYWQ7Cn0KCnZvaWQgcHJpbnREb3VibGVMaW5rZWRMaXN0KERvdWJsZU5vZGUqIGhlYWQpIHsKICAgIERvdWJsZU5vZGUqIGN1cnJlbnQgPSBoZWFkOwogICAgd2hpbGUgKGN1cnJlbnQpIHsKICAgICAgICBjb3V0IDw8IGN1cnJlbnQtPmRvdWJsZWRhdGEgPDwgIiA8LS0tPiAiOwogICAgICAgIGN1cnJlbnQgPSBjdXJyZW50LT5kb3VibGVuZXh0OwogICAgfQogICAgY291dCA8PCAibnVsbCIgPDwgZW5kbDsKfQoKaW50IG1haW4oKSB7CiAgICBpbnQgbiwgbTsKICAgIGNpbiA+PiBuID4+IG07CgogICAgdmVjdG9yPHZlY3RvcjxpbnQ+PiBhcnIobiwgdmVjdG9yPGludD4obSkpOwogICAgZm9yIChpbnQgaSA9IDA7IGkgPCBuOyBpKyspIHsKICAgICAgICBmb3IgKGludCBqID0gMDsgaiA8IG07IGorKykgewogICAgICAgICAgICBjaW4gPj4gYXJyW2ldW2pdOwogICAgICAgIH0KICAgIH0KCiAgICB2ZWN0b3I8Tm9kZSo+IG5vZGVMaXN0OwogICAgY3JlYXRlTm9kZUxpc3QoYXJyLCBub2RlTGlzdCk7CgogICAgRG91YmxlTm9kZSogZG91YmxlTGlua2VkTGlzdCA9IGNyZWF0ZURvdWJsZUxpbmtlZExpc3Qobm9kZUxpc3QpOwogICAgcHJpbnREb3VibGVMaW5rZWRMaXN0KGRvdWJsZUxpbmtlZExpc3QpOwoKICAgIHJldHVybiAwOwp9Cg==
Solution 3: Python
Y2xhc3MgRG91YmxlTm9kZToNCiAgICBkZWYgX19pbml0X18oc2VsZiwgZG91YmxlZGF0YSk6DQogICAgICAgIHNlbGYuZG91YmxlZGF0YSA9IGRvdWJsZWRhdGENCiAgICAgICAgc2VsZi5kb3VibGVwcmV2ID0gTm9uZQ0KICAgICAgICBzZWxmLmRvdWJsZW5leHQgPSBOb25lDQoNCmNsYXNzIE5vZGU6DQogICAgZGVmIF9faW5pdF9fKHNlbGYsIGRhdGEpOg0KICAgICAgICBzZWxmLmRhdGEgPSBkYXRhDQogICAgICAgIHNlbGYubmV4dCA9IE5vbmUNCg0KZGVmIGNyZWF0ZV9ub2RlX2xpc3QoYXJyKToNCiAgICBsaXN0X29mX25vZGVzID0gW10NCiAgICBmb3Igcm93IGluIGFycjoNCiAgICAgICAgaGVhZCA9IE5vbmUNCiAgICAgICAgcHJldiA9IE5vbmUNCiAgICAgICAgZm9yIHZhbHVlIGluIHJvdzoNCiAgICAgICAgICAgIG5ld19ub2RlID0gTm9kZSh2YWx1ZSkNCiAgICAgICAgICAgIGlmIGhlYWQgaXMgTm9uZToNCiAgICAgICAgICAgICAgICBoZWFkID0gbmV3X25vZGUNCiAgICAgICAgICAgIGVsc2U6DQogICAgICAgICAgICAgICAgcHJldi5uZXh0ID0gbmV3X25vZGUNCiAgICAgICAgICAgIHByZXYgPSBuZXdfbm9kZQ0KICAgICAgICBsaXN0X29mX25vZGVzLmFwcGVuZChoZWFkKQ0KICAgIHJldHVybiBsaXN0X29mX25vZGVzDQoNCmRlZiBjcmVhdGVfZG91YmxlX2xpbmtlZF9saXN0KGxpc3Rfb2Zfbm9kZXMpOg0KICAgIGhlYWQgPSBOb25lDQogICAgdGFpbCA9IE5vbmUNCiAgICANCiAgICAjIEZpcnN0IGFkZCBub2RlcyBmcm9tIGV2ZW4gaW5kZXggcm93cw0KICAgIGZvciBpIGluIHJhbmdlKDAsIGxlbihsaXN0X29mX25vZGVzKSwgMik6DQogICAgICAgIGN1cnJlbnQgPSBsaXN0X29mX25vZGVzW2ldDQogICAgICAgIHdoaWxlIGN1cnJlbnQ6DQogICAgICAgICAgICBuZXdfZG91YmxlX25vZGUgPSBEb3VibGVOb2RlKGN1cnJlbnQuZGF0YSkNCiAgICAgICAgICAgIGlmIGhlYWQgaXMgTm9uZToNCiAgICAgICAgICAgICAgICBoZWFkID0gbmV3X2RvdWJsZV9ub2RlDQogICAgICAgICAgICAgICAgdGFpbCA9IGhlYWQNCiAgICAgICAgICAgIGVsc2U6DQogICAgICAgICAgICAgICAgdGFpbC5kb3VibGVuZXh0ID0gbmV3X2RvdWJsZV9ub2RlDQogICAgICAgICAgICAgICAgbmV3X2RvdWJsZV9ub2RlLmRvdWJsZXByZXYgPSB0YWlsDQogICAgICAgICAgICAgICAgdGFpbCA9IG5ld19kb3VibGVfbm9kZQ0KICAgICAgICAgICAgY3VycmVudCA9IGN1cnJlbnQubmV4dA0KDQogICAgIyBUaGVuIGFkZCBub2RlcyBmcm9tIG9kZCBpbmRleCByb3dzDQogICAgZm9yIGkgaW4gcmFuZ2UoMSwgbGVuKGxpc3Rfb2Zfbm9kZXMpLCAyKToNCiAgICAgICAgY3VycmVudCA9IGxpc3Rfb2Zfbm9kZXNbaV0NCiAgICAgICAgd2hpbGUgY3VycmVudDoNCiAgICAgICAgICAgIG5ld19kb3VibGVfbm9kZSA9IERvdWJsZU5vZGUoY3VycmVudC5kYXRhKQ0KICAgICAgICAgICAgaWYgaGVhZCBpcyBOb25lOg0KICAgICAgICAgICAgICAgIGhlYWQgPSBuZXdfZG91YmxlX25vZGUNCiAgICAgICAgICAgICAgICB0YWlsID0gaGVhZA0KICAgICAgICAgICAgZWxzZToNCiAgICAgICAgICAgICAgICB0YWlsLmRvdWJsZW5leHQgPSBuZXdfZG91YmxlX25vZGUNCiAgICAgICAgICAgICAgICBuZXdfZG91YmxlX25vZGUuZG91YmxlcHJldiA9IHRhaWwNCiAgICAgICAgICAgICAgICB0YWlsID0gbmV3X2RvdWJsZV9ub2RlDQogICAgICAgICAgICBjdXJyZW50ID0gY3VycmVudC5uZXh0DQoNCiAgICByZXR1cm4gaGVhZA0KDQpkZWYgcHJpbnRfZG91YmxlX2xpbmtlZF9saXN0KGhlYWQpOg0KICAgIGN1cnJlbnQgPSBoZWFkDQogICAgd2hpbGUgY3VycmVudDoNCiAgICAgICAgcHJpbnQoZiJ7Y3VycmVudC5kb3VibGVkYXRhfSA8LS0tPiAiLCBlbmQ9IiIpDQogICAgICAgIGN1cnJlbnQgPSBjdXJyZW50LmRvdWJsZW5leHQNCiAgICBwcmludCgibnVsbCIpDQoNCmlmIF9fbmFtZV9fID09ICJfX21haW5fXyI6DQogICAgbiA9IGludChpbnB1dCgpKQ0KICAgIG0gPSBpbnQoaW5wdXQoKSkNCiAgICBhcnIgPSBbbGlzdChtYXAoaW50LCBpbnB1dCgpLnNwbGl0KCkpKSBmb3IgXyBpbiByYW5nZShuKV0NCg0KICAgICMgQ3JlYXRlIG5vZGUgbGlua2VkIGxpc3RzIGZyb20gMkQgYXJyYXkNCiAgICBsaXN0X29mX25vZGVzID0gY3JlYXRlX25vZGVfbGlzdChhcnIpDQoNCiAgICAjIENyZWF0ZSBkb3VibGUgbGlua2VkIGxpc3QNCiAgICBkb3VibGVfbGlua2VkX2xpc3QgPSBjcmVhdGVfZG91YmxlX2xpbmtlZF9saXN0KGxpc3Rfb2Zfbm9kZXMpDQoNCiAgICAjIFByaW50IHRoZSBkb3VibGUgbGlua2VkIGxpc3QNCiAgICBwcmludF9kb3VibGVfbGlua2VkX2xpc3QoZG91YmxlX2xpbmtlZF9saXN0KQ0K
Digital Advanced Coding Questions
TCS Digital coding questions for advanced levels test not only your knowledge but also your efficiency in solving complex problems within tight time constraints. These questions often involve multiple layers of logic and optimization techniques. Here are a few sample advanced coding problems:
Problem | Topic | Approach |
---|---|---|
Implement a Least Recently Used (LRU) Cache using linked lists and hashmaps. | Data Structures (Linked List, HashMap) | Efficient use of space and time |
Find the longest increasing subsequence in a given array. | Dynamic Programming | Memoization, Recursion |
Given a binary tree, convert it into its mirror image. | Trees | Recursive Tree Manipulation |
Implement a system to schedule jobs on multiple processors using priority queues. | Greedy Algorithms | Queue Manipulation, Optimization |
Design an algorithm to find the maximum profit that can be made by buying and selling stocks. | Dynamic Programming, Greedy | Max Profit, Min Cost Calculation |
TCS Digital Coding Round Questions
The coding round for TCS Digital typically involves a mix of medium to high difficulty problems. Here is an example format of a typical coding round:
Question Type | Sample Question | Solution Approach |
---|---|---|
Medium-Level Algorithm | Find the second largest element in an unsorted array. | Use sorting or linear scan |
Advanced-Data Structure | Implement a priority queue using a min-heap. | Heap-based operations |
Dynamic Programming | Find the longest palindromic subsequence in a string. | DP table with memoization |
Greedy Algorithm | Schedule tasks with given deadlines and maximize profit. | Greedy method with sorting |
String Manipulation | Find the smallest window in a string containing all characters of another string. | Sliding window technique |
Digital Coding Questions for Freshers
While the coding questions for TCS Digital are challenging, they are also solvable with proper preparation. Freshers are typically expected to demonstrate a strong grasp of fundamental algorithms and data structures. Commonly asked coding questions for freshers include:
Question | Topic | Difficulty |
---|---|---|
Implement binary search on a sorted array. | Searching Algorithm | Easy |
Write a function to check if a string is a palindrome. | String Manipulation | Easy |
Reverse the elements of a linked list. | Linked List | Moderate |
Sort an array using merge sort. | Sorting Algorithms | Moderate |
Find the number of ways to reach the nth stair if you can climb either 1 or 2 steps at a time. | Dynamic Programming | Moderate |
How to Prepare for TCS Digital Coding Exam?
To succeed in TCS Digital coding rounds, you need to focus on understanding and practising core topics:
Preparation Strategy | Description |
---|---|
Master Data Structures & Algorithms | Study data structures like arrays, linked lists, trees, and algorithms such as sorting, searching, and graph traversal. |
Practice Dynamic Programming | Solve DP-based problems to build optimization skills. |
Time Management | Practice solving problems under timed conditions. |
Focus on Optimization | Practice reducing time and space complexity for efficient solutions. |
Disclaimer: While we have gathered as much information from TCS’s official website as possible, we have also included sources gathered from available online sources. Therefore, readers are advised to check and stay updated with the official website.
Conclusion
TCS Digital coding questions challenge candidates on multiple levels, requiring proficiency in algorithms, data structures, dynamic programming, and more. Whether you're a fresher or an experienced professional, thorough preparation and continuous practice of advanced coding problems are essential for cracking the TCS Digital coding round.
Use the past questions as practice and focus on time-bound solving to improve speed and accuracy. By mastering the essential topics and practising a variety of coding problems, you can significantly increase your chances of securing a role in TCS Digital.
Frequently Asked Questions (FAQs)
1. What is the difficulty level of coding questions in the TCS Digital exam?
The coding questions in TCS Digital range from moderate to advanced. They often test knowledge of algorithms, data structures, and problem-solving with an emphasis on optimization.
2. Which programming languages can I use in the TCS Digital coding round?
You can choose from popular languages such as C, C++, Java, Python, and sometimes JavaScript, depending on the platform used for the coding assessment.
3. How many coding questions are asked in the TCS Digital exam?
Typically, the TCS Digital exam includes 2-3 coding questions to be solved within 60 to 90 minutes.
4. What are the most common topics for TCS Digital coding questions?
The most common topics include dynamic programming, string manipulation, sorting and searching algorithms, graph theory, and data structures like arrays, trees, and linked lists.
5. How can I prepare for the TCS Digital advanced coding questions?
To prepare for advanced coding questions, focus on solving complex problems related to dynamic programming, graph algorithms, and system design. Practising on coding platforms like LeetCode and Codeforces can also be helpful.
Suggested reads:
-
Deloitte Coding Questions: Top 5 Coding Questions for Freshers
-
TCS Digital Recruitment Process: Latest Updates & Tips for Freshers
-
HCL Logical Reasoning Questions and Answers: Top 5 Sample MCQs
-
HCL Aptitude Test: Top 5 Questions and Answers for Freshers (MCQs)
-
TCS Digital Aptitude Questions and Answers: Top 5 Sample MCQs
Instinctively, I fall for nature, music, humour, reading, writing, listening, travelling, observing, learning, unlearning, friendship, exercise, etc., all these from the cradle to the grave- that's ME! It's my irrefutable belief in the uniqueness of all. I'll vehemently defend your right to be your best while I expect the same from you!
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
Blogs you need to hog!
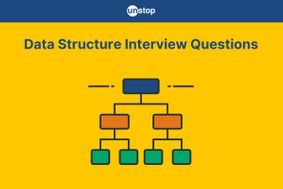
55+ Data Structure Interview Questions For 2025 (Detailed Answers)
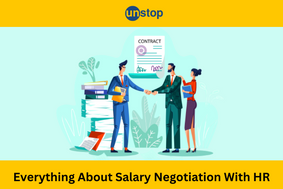
How To Negotiate Salary With HR: Tips And Insider Advice
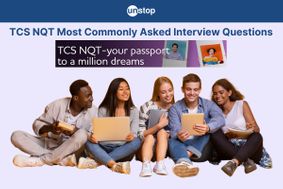
Best 80+ TCS NQT Most Commonly Asked Interview Questions for 2025
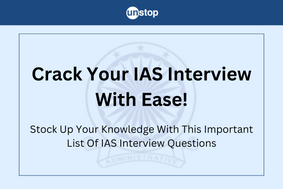
Comments
Add comment