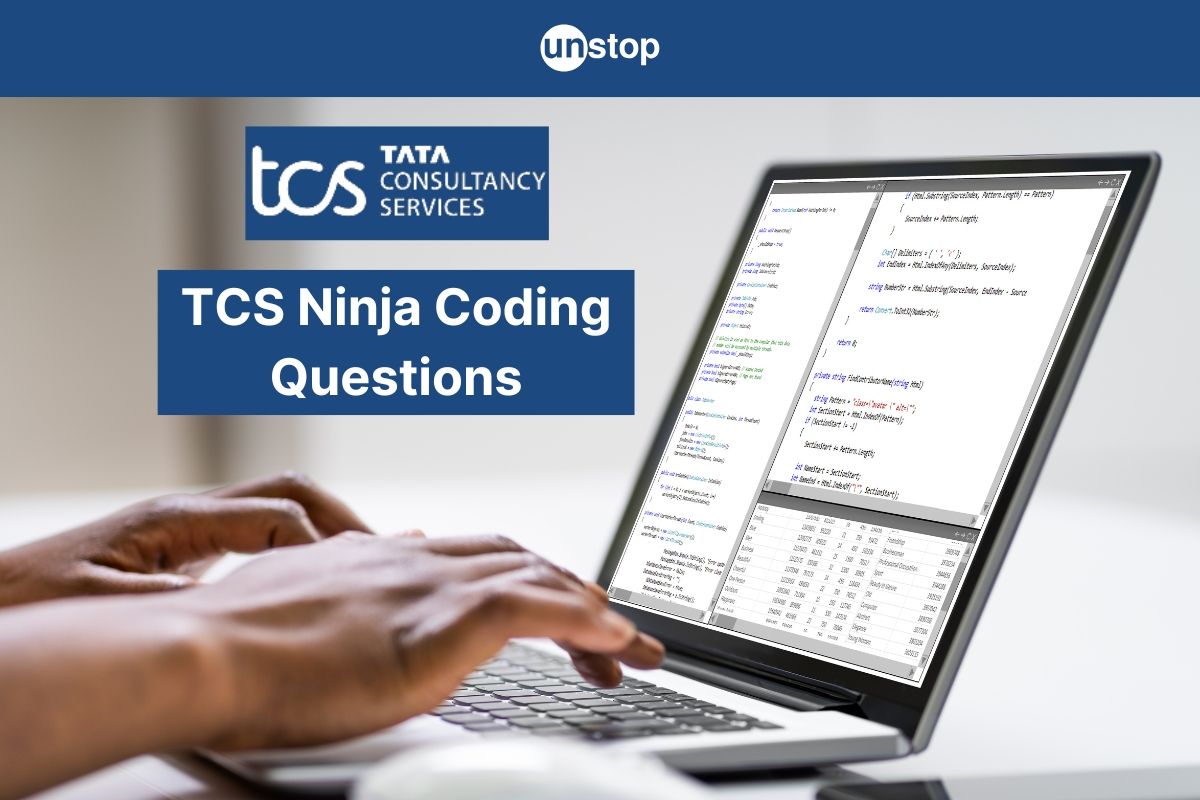
TCS Ninja is a highly sought-after entry-level role at Tata Consultancy Services (TCS). As part of the selection process, candidates go through a series of assessments, including a coding round that tests their programming skills. This detailed guide covers the TCS Ninja coding questions, previous year patterns, and tips for preparation.
TCS Ninja Coding Round Overview
The TCS Ninja coding round is designed to assess a candidate’s ability to solve algorithmic and data structure-related problems. The difficulty level ranges from easy to moderate, targeting fundamental concepts that every programmer should know.
Here's an overview of what the coding section typically looks like:
Category | Details |
---|---|
Number of Questions | 1-2 coding problems |
Duration | 30 to 45 minutes |
Difficulty Level | Easy to Moderate |
Programming Languages | C, C++, Java, Python |
Key Topics Covered | Data Structures, Algorithms, String Manipulation |
Platform Used | TCS CodeVita or iON platform |
Topics for TCS Ninja Coding Questions
The questions in the TCS Ninja coding section focus on core computer science topics. Below is a breakdown of the most common topics and typical questions asked:
Topic | Description | Example Questions |
---|---|---|
Arrays | Problems involving array manipulations and operations. | Find the maximum sum of a subarray, Rotate an array. |
Strings | String manipulation, pattern matching, and transformations. | Check if a string is a palindrome, Find anagram pairs. |
Sorting & Searching | Implementing basic sorting and searching algorithms. | Binary search, Merge sort, Bubble sort. |
Recursion | Problems that require recursive solutions. | Factorial of a number, Fibonacci sequence using recursion. |
Dynamic Programming | Optimization problems using dynamic programming. | 0/1 Knapsack, Longest common subsequence. |
Greedy Algorithms | Problems solved using the greedy method. | Activity selection, Minimum coins for change. |
Questions Format for Ninja Coding
TCS Ninja coding questions typically come in different formats. Here's what you can expect:
Question Type | Description | Example |
---|---|---|
Simple Algorithmic Problem | Basic algorithmic problems that test your knowledge of loops, conditionals, and basic logic. | Write a program to find the greatest of three numbers. |
Data Structure Manipulation | Problems related to arrays, linked lists, or stacks. | Reverse a linked list, Sort an array using merge sort. |
Pattern-based Questions | String or array pattern problems. | Check for an anagram, Find all unique substrings of a string. |
Dynamic Programming Problems | Optimization problems requiring memoization or tabulation. | Solve the 0/1 knapsack problem using dynamic programming. |
Top 5 Sample Questions with Solution
Problem Statement 1
Ram and Shyam are two friends belonging to two different cities. They exchange messages weekly. The messages are very crucial so that no third person can see the message. But they sent a message via Jadav, a clever person. He usually reads the given messages. So, Ram and Shyam started talking in codewords. They set a convention for every letter in the English Alphabet.
Like ‘a’ to ‘i’ code is 1 to 9 and ‘j’ to ‘z’ code is 10# to 26#.
Now suppose, Ram is sending a message to Shyam denoted by String S.
Can you tell me the decoded message that Shyam receives?
Input Format
The first line of input contains the string S.
Output Format
Return the string which denotes the decoded message that Shyam receives
Constraints
1<=s.length<=10^9
Testcase Input
26#21#13#13#114#97820#
Testcase Output
zummanight
Solution 1: Java
Solution 2: C++
Solution 3: Python
Problem Statement 2
You're in a world where magical creatures engage in a peculiar contest, and you've been entrusted with keeping track of the matches. The rules are as follows:
If there is an even number of creatures, they form pairs, and a total of n/2 matches are played. The victors of these matches advance to the next round.
When there's an odd number of creatures, an intriguing twist unfolds. One of them is granted a special power and advances directly to the next stage, while the remaining creatures pair off for matches. The number of matches in this scenario is (n - 1)/2. The creature with the special power joins the winners of these matches in the next round.
Your mission is to determine the overall number of matches that take place in this magical contest until a single champion emerges. How many matches will be required to decide the ultimate winner?
Input Format
An integer n represents the initial number of magical creatures in the contest.
Output Format
An integer represents the total number of matches played in the magical contest until a single champion is determined.
Constraints
1 <= n <= 200
Testcase Input
5
Testcase Output
4
Solution 1: C++
Solution 2: Java
Solution 3: Python
Problem Statement 3
You are a shopkeeper and bought N pairs of socks of several colours in bulk. The colour of each pair of socks is represented as a non-negative integer. The socks are sold as sets of 3 each. A set of socks consists of 3 socks of the same colour.
You want to find the number of different sets that can be made from the N pairs of socks you bought today.
Note: The order of indices of sock pairs in the set does not matter.
Input Format
The first line of the input contains a single integer N which denotes the number of pairs of socks that you have.
The second line of the input contains n space-separated integers a1, a2, …, an, where ai represents the colour of the ith pair.
Output Format
Print a single integer representing the total number of different sets of 3 socks that can be formed from the N pairs of socks.
Constraints
1 <= N <= 105
0 <= ai <= 10^3
Testcase Input
7
1 3 3 1 1 3 3
Testcase Output
5
Solution 1: Python
Solution 2: C++
Solution 3: Java
Problem Statement 4
Ram gave Shyaam a challenge, he gave shyaam the head of a linked list, and an integer k.
He asked Shyaam to swap the values of the Kth node from the beginning and the Kth node from the end (the list is 1-indexed).
Note: The number of nodes in the list is N.
Input Format
The first line contains an integer N, representing the number of nodes in the linked list.
The second line contains N space-separated integers, each representing the value of a node in the linked list.
The third line contains an integer K, indicating the positions of the nodes to be swapped.
Output Format
Output the linked list after swapping the values of the two specified nodes.
Constraints
1 <= K <= N <= 10^5
0 <= Node.val <= 10^2
Testcase Input
5
1 2 3 4 5
2
Testcase Output
1 4 3 2 5
Solution 1: Java
Solution 2: C++
Solution 3: Python
Problem Statement 5
There are n spaceships at given lightyears away from the Earth and travelling to reach a distant star system at k lightyears away from Earth. You are given two integer arrays, position and speed, both of length n, where
P[i] is the current distance of the ith spaceship
S[i] is the speed of the ith spaceship in lightyears per year.
As the spaceships travel toward the star system, an interesting phenomenon occurs: when a faster spaceship catches up to a slower one, it reduces its speed to match the slower spaceship's speed, forming a fleet. A fleet is a group of one or more spaceships that travel together at the same speed.
Given this information, determine the number of distinct spacecraft fleets that will arrive at the destination star system. A fleet is considered distinct if no other fleet arrives at the destination at the same time while travelling together.
Input Format
The first line contains an integer 'n', representing the total number of spaceships.
The second line contains an integer 'k', representing the distance of the star system from Earth.
The third line contains 'n' space-separated integers denoting the current distance of the i-th spaceship from Earth.
The fourth line contains 'n' space-separated integers denoting the speed of the i-th spaceship.
Output Format
Return the number of spacecraft fleets that will arrive at the destination.
Constraints
1 <= n <= 10^5
0 < k <= 10^6
0 <= P[i] < target
0 < S[i] <= 10
Testcase Input
4
14
10 8 5 3
2 4 1 3
Testcase Output
2
Solution 1: Python
Solution 2: C++
Enhance your coding skills by practicing coding questions across various levels. Click here to start your coding journey and master programming!
Preparation Tips
Here are some tips to ace the TCS Ninja coding round:
Strategy | Description |
---|---|
Practice Core Concepts | Focus on mastering basic data structures and algorithms. Ensure you understand how to implement sorting algorithms, recursion, and string manipulations. |
Time Management | The coding round is timed, so practice solving problems quickly and accurately. Split time effectively between easy and moderate-level questions. |
Optimize Code | Practice writing code that is not only correct but also efficient. Focus on reducing time complexity wherever possible. |
Conclusion
The TCS Ninja coding round is a critical part of the recruitment process, testing your problem-solving ability and programming knowledge. Whether you’re a fresher or have some experience, consistent practice in algorithms, data structures, and coding problems will help you excel. Focus on solving previous year's questions, improve your speed, and keep practising to enhance your coding skills.
Disclaimer: While we have gathered as much information from Deloitte's official website as possible, we have also included sources gathered from available online sources. Therefore, readers are advised to check and stay updated with the official website.
Frequently Asked Questions (FAQs)
1. What is the difficulty level of the coding questions in TCS Ninja?
The coding questions in the TCS Ninja exam are generally of easy to moderate difficulty, focusing on fundamental concepts like arrays, strings, and basic algorithms.
2. How many coding questions are asked in the TCS Ninja exam?
Typically, the exam includes 1-2 coding questions that need to be solved within 30 to 45 minutes.
3. Which programming languages can be used in the TCS Ninja coding round?
You can use programming languages such as C, C++, Java, Python, or Perl, depending on the platform.
4. What topics should I focus on for TCS Ninja coding questions?
Key topics include arrays, strings, recursion, sorting and searching algorithms, and basic dynamic programming problems.
Suggested reads:
-
Deloitte Coding Questions: Top 5 Coding Questions for Freshers
-
TCS Digital Recruitment Process: Latest Updates & Tips for Freshers
-
HCL Logical Reasoning Questions and Answers: Top 5 Sample MCQs
-
HCL Aptitude Test: Top 5 Questions and Answers for Freshers (MCQs)
-
TCS Digital Aptitude Questions and Answers: Top 5 Sample MCQs
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Blogs you need to hog!
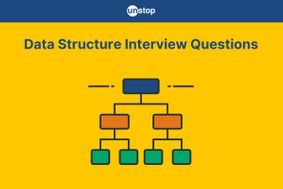
55+ Data Structure Interview Questions For 2024 (Detailed Answers)
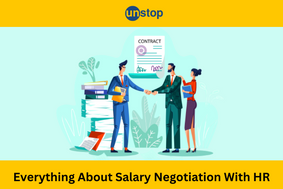
How To Negotiate Salary With HR: Tips And Insider Advice
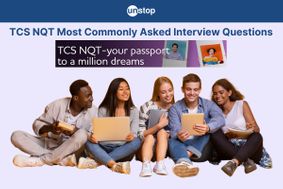
Best 80+ TCS NQT Most Commonly Asked Interview Questions for 2025
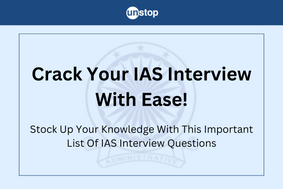
Comments
Add comment