Identifiers In Java | Types, Conventions, Errors & More (+Examples)
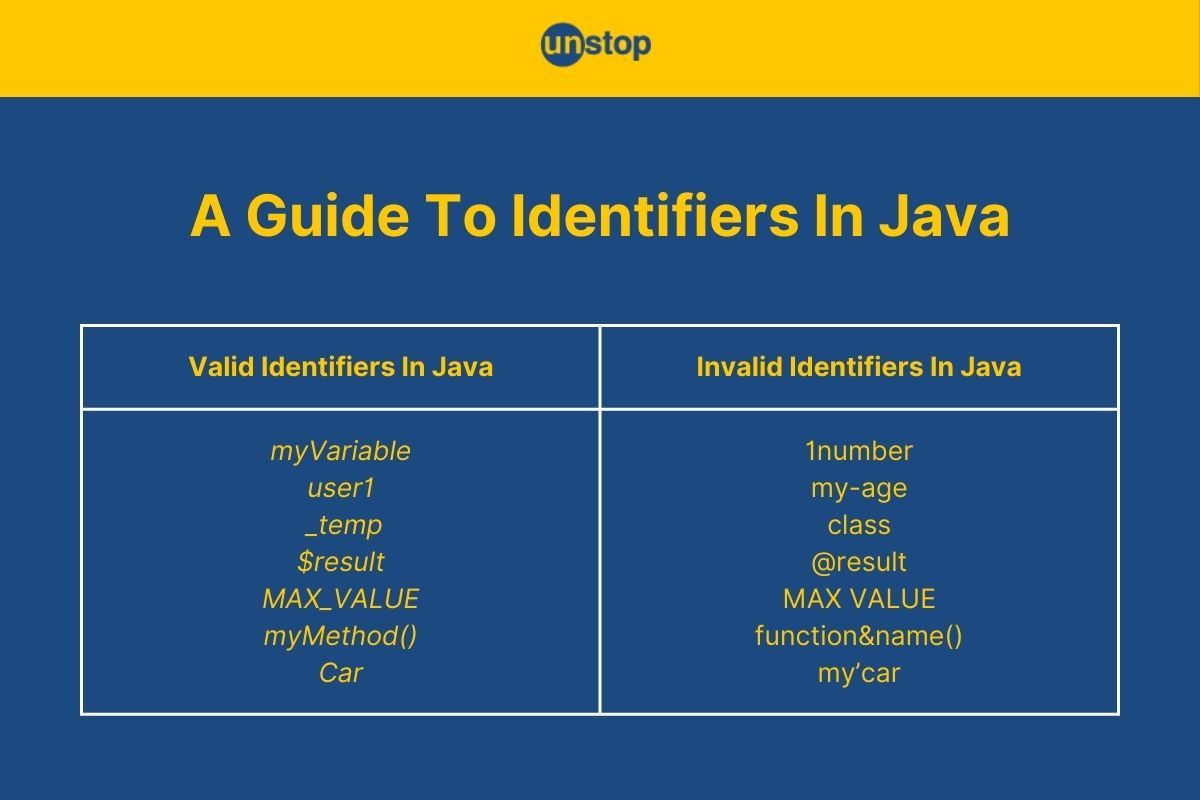
Identifiers in Java programming (and even other languages) are essential elements serving as names for various components of code. These components include variables, functions, classes, objects, etc., to distinguish them within the code. The identifiers are more than just labels; they interact deeply with Java's syntax and semantics, playing a critical role in making code efficient, readable, and maintainable.
In this article, we will explore the rules and standards for Java identifiers, including reserved keywords (their effect on identifier naming conventions), syntactic guidelines, naming standards, and best practices for clear and maintainable code. We will also discuss typical identifier usage errors and hazards, focusing on the "identifier expected" error in your code, its causes, and easy ways to prevent it.
What Are Identifiers In Java?
Identifiers are the names given to various elements in Java programming, such as variables, methods, classes, interfaces, packages, and objects.
- These names are essential as they allow us to identify and differentiate between different elements within our code.
- Software developers refer to these code parts by these names. Programmers may make code easier to comprehend and maintain by assigning a human-readable name to data structures using identifiers.
- Note that a variable cannot have the same name as another variable inside the same procedure, even though they may be used in different ways.
- This means that each identifier must be unique within its scope.
Let’s look at a simple Java program example to better understand the concept of identifiers.
Code Example:
Explanation:
- In the example, we first defined a class named Car (identifier) using the public access modifier and class keyword for declaration.
- Inside the class, we declare two private instance variables, speed and color (identifiers), of types int and String, respectively.
- Then, we have a class constructor (of the same name/ identifier as the class), which initializes the color instance variable with the provided argument and sets the speed instance variable to 0.
- Next, we define a method with name/ identifier accelerate(), which increases the speed instance variable by the value provided by the variable increment (identifier).
- We then define another method identified as displayInfo(), which returns a string that includes the car's color and current speed in a formatted string using concatenation.
- In the main method, we create an instance of the Car class named myCar (identifier) with the color field initialized with Red, using the new keyword. This calls the constructor and initializes the color variable to "Red" and speed to 0.
- Next, we call the accelerate() method on the myCar object with an argument of 50, which increases the speed variable by 50. Note that here, we use the method identifier/name to call it.
- Finally, we print the car's information to the console by calling the displayInfo() method on the myCar object and passing its return value to System.out.println. This outputs the car's color and current speed in a formatted string.
Here is a list of the identifiers in Java program above:
- Car: This is the name of the class.
- speed: This is a variable that stores the speed of the car. Note how it is a meaningful identifier, as it depicts the purpose of the variable.
- color: This is a variable that stores the color of the car.
- Car (constructor): This is a constructor method for the Car class, which initializes new objects.
- accelerate: A method that increases the car's speed by a specified increment
- increment: A parameter in the accelerate method that represents the amount by which to increase the speed.
- displayInfo: This is a method that returns information about the car.
- myCar: The instance of the Car class.
Each of these identifiers is chosen to be descriptive and clear (symbolic names), indicating what the variable or method is likely used for or what kind of data it handles.
Syntax Rules For Identifiers In Java
Identifiers in Java follow specific syntax rules that dictate how they can be named. Adhering to these rules is essential to ensure that your code is syntactically correct and avoids any compile-time errors. Here are some of the key syntax rules for identifiers in Java, along with examples of identifiers, both correct and incorrect usage:
-
Identifiers must start with a letter, underscore (_), or dollar sign ($):
- Correct Example: myVariable, _variable, $variable
- Incorrect Example: 9pins (starts with a digit)
- After the first character, identifiers can contain letters, digits, underscores, or dollar signs:
- Correct Example: var123, var_123, var$123
- Incorrect Example: var-123 (hyphen is not allowed)
-
Identifiers are case-sensitive:
- Correct Example: myvariable and myVariable are different identifiers.
- Incorrect Example: Treating myVariable and MyVariable as the same identifier.
-
Identifiers cannot be a Java-reserved word:
- Correct Example: myClass, userInput
- Incorrect Example: class, int (these are reserved keywords)
-
Identifiers should not contain white space between characters/ words:
- Correct Example: userName
- Incorrect Example: user name (white space between words)
-
Java identifiers can be of any length:
- Correct Example: x, score, numberOfUsers
- Incorrect Example: While there's no length limit, excessively long identifiers can make code hard to read, though technically they are not incorrect.
-
Avoid using special symbols/ characters other than the underscore and the dollar symbol:
- Correct Example: _name, $value
- Incorrect Example: name# (hash symbol is not allowed)
By adhering to these rules, you can ensure that your identifiers are valid in the Java programming language. Following these guidelines helps avoid syntax errors, maintain consistency, and make your code easier to understand.
Now that we know the basics of identifiers in Java and the syntax rules/ standard conventions let's explore the two primary types of identifiers in detail.
Valid Identifiers in Java
An identifier that follows the specific syntax rules dictating its structure and composition is referred to as a valid identifier in Java.
- In other words, a valid identifier must start with a letter, an underscore (_), or a dollar sign ($) and can include letters, digits, underscores, and dollar signs.
- These identifiers are used to name variables, methods, classes, and other elements in a Java program.
- It is important to note that identifiers are case-sensitive, meaning that the Java compiler recognizes variable, Variable, and VARIABLE as distinct names.
Here's a table that illustrates various examples of valid identifiers in Java:
Valid Identifier |
Explanation |
age |
Begins with a letter |
_age |
Begins with an underscore |
$age |
Begins with a dollar sign |
age3 |
Combines letters and digits |
a3g_e |
Includes an underscore within the name |
AGE |
Completely uppercase |
aGe1 |
Mixed case with digits |
Each of these identifiers adheres to the rules set forth for naming, making them valid for use in Java programs. Let's look at a sample Java program illustrating these identifiers.
Code Example:
Output:
Name: Aditi, Age: 30
Explanation:
In this Java example-
- First, we define a public class named Person (identifier) using the class keyword (not identifier).
- The class has two private instance variables/ fields: name (string variable) and age (integer variable).
- Then, we have a class constructor, Person, which initializes the fields (name and age) of the instance object/ variable with the values passed as arguments.
- Next, we define a method displayInfo() that prints the fields of the instance variable to the console using System.out.println() and string concatenation.
- In the main method, we instantiate an object person (of class person) with the name Aditi and age 30 using the new keyword.
- Lastly, we call the displayInfo() method is called on the person object printing the fields to the console.
The Java identifiers used in this code are:
- Person: This is the class name. It starts with a capital letter, which is a common convention for class names in Java.
- name and age: These are variable identifiers that are a part of the class. They use simple, lowercase identifiers, following the convention, for instance, variables.
- Person (constructor): This special method uses the same name as the class to initialize new objects of class Person.
- displayInfo: This method name is in camelCase, starting with a lowercase letter and capitalizing the first letter of each subsequent concatenated word, which is standard for method names.
- main: The main method is the entry point for any standalone Java application. The identifier main is conventional and reserved for this specific purpose.
This example demonstrates how different types of valid identifiers can be used effectively in a Java program to enhance readability and maintainability.
Invalid Identifiers in Java
As discussed in the previous section, an identifier must follow specific guidelines to be deemed valid for identification purposes in Java. Identifiers that do not comply with these requirements will result in compilation problems. They are hence referred to as invalid identifiers in the Java language.
Below is a table providing examples of incorrect identifiers in Java and the explanations for each one:
Invalid Identifier |
Reason For Invalidity |
3age |
Begins with a digit |
my-age |
Contains a hyphen, which is not allowed |
class |
Reserved/ predefined class declaration keyword |
first name |
Contains a space, which is not allowed |
@username |
Contains special character '@', which is not allowed |
These identifiers do not conform to the syntax rules for naming identifiers in Java, hence they are considered invalid. Below is a Java code snippet that contains an example of an invalid identifier:
Code Example:
Explanation:
- 3age: This identifier is invalid because it begins with a digit.
- my-age: This identifier is invalid because it contains a hyphen.
- class: This identifier is invalid because it is a reserved keyword in Java.
- first name: This identifier is invalid because it contains a space.
- @username: This identifier is invalid because it contains a special character '@'.
These names violate the rules for valid identifiers in Java. When you try to compile this code, you will encounter compilation errors indicating the invalid identifiers. These errors must be corrected by adhering to the syntax rules for identifiers in Java.
By understanding these common mistakes, you can avoid them in your own code, ensuring that your identifiers are always valid and your Java programs compile without errors.
Java Reserved Keywords
In Java, keywords are predefined, reserved words that have specific meanings and roles within the language. These keywords cannot be used as identifiers (e.g., variable names, method names, or class names) because they are integral to Java’s syntax and semantics. Attempting to use a reserved keyword as an identifier will result in a compilation error.
Here's a table listing the reserved keywords in Java:
Keyword |
Description |
abstract |
Used to declare abstract classes and methods |
assert |
Used for debugging purposes |
boolean |
Used to declare boolean variables |
break |
Used to terminate a loop or switch statement |
byte |
Used to declare byte variables |
case |
Used in switch statements to define different cases |
catch |
Used to catch exceptions in exception handling |
char |
Used to declare character variables |
class |
Used to declare a class |
const |
Reserved for future use, not currently used |
continue |
Used to skip the current iteration of a loop |
default |
Used in switch statements as a default case |
do |
Used to start a do-while loop |
double |
Used to declare double data type variables, i.e., double-precision floating-point values |
else |
Used in conditional statements as an alternative |
enum |
Used to declare enumerated types |
extends |
Used to extend a class or interface |
final |
Used to declare constants or to prevent inheritance or method overriding |
finally |
Used in exception handling to execute code regardless of an exception |
float |
Used to declare float data type variables, i.e., single-precision floating-point values |
for |
Used to start a for loop |
if |
Used in conditional statements to perform actions based on a condition |
goto |
Reserved for future use, not currently used |
implements |
Used to implement an interface |
import |
Used to import classes, interfaces, or packages |
instanceof |
Used to test if an object is an instance of a particular class |
int |
Used to declare integer variables |
interface |
Used to declare interfaces |
long |
Used to declare long integer variables |
native |
Used to declare native methods |
new |
Used to create new objects |
null |
Used to represent a null reference |
package |
Used to declare a package |
private |
Used to declare private access to classes, variables, and methods |
protected |
Used to declare protected access to classes, variables, and methods |
public |
Used to declare public access to classes, variables, and methods |
return |
Used to return a value from a method |
short |
Used to declare short integer variables |
static |
Used to declare static variables and methods |
strictfp |
Used to restrict floating-point calculations to ensure portability |
super |
Used to refer to the superclass in a subclass |
switch |
Used to switch between multiple cases |
synchronized |
Used to specify synchronized access to methods or blocks |
this |
Used to refer to the current instance of the class |
throw |
Used to throw an exception |
throws |
Used to declare exceptions that a method may throw |
transient |
Used to specify that a variable is not part of the serialized state of an object |
try |
Used to start a block of code to be tested for exceptions |
void |
Used to declare methods that do not return a value |
volatile |
Used to indicate that a variable may change asynchronously |
while |
Used to start a while loop |
By understanding and adhering to Java's reserved keywords, you can avoid syntax errors and ensure that your code adheres to the language's standards.
Example of Reserved Keyword Error
Let’s try to understand it better with the help of an example. Consider the following Java code snippet:
class Main {
public static void main(String[] args) {
int switch = 10; // Using switch keyword as an identifier
System.out.println(switch);
}
}
Explanation:
In this example, the identifier switch is a reserved keyword. Attempting to use it as a variable name will result in compilation errors. The Java compiler will indicate that switch cannot be used as an identifier due to its role as a reserved keyword. The errors might look like this:
Main.java:3: error: <identifier> expected
int switch = 10; // Using "switch" as an identifier
^
Main.java:3: error: ';' expected
int switch = 10; // Using "switch" as an identifier
^
Main.java:4: error: reached end of file while parsing
}
^
3 errors
These errors occur because the compiler expects a valid identifier but encounters a reserved keyword instead. To fix this erroneous code, choose an alternative name that does not conflict with Java's reserved keywords and does not lead to a syntax error.
Naming Conventions & Best Practices For Identifiers In Java
In Java programming language, naming conventions and best practices help ensure that code is readable, maintainable, and consistent. While syntax rules dictate the fundamental structure of identifiers, naming conventions focus on making identifiers descriptive and meaningful. Adhering to both improves code quality and collaboration within development teams.
Common Naming Conventions and Best Practices in Java:
-
CamelCase: You must use camelCase to name variables, methods, and packages.
- Format: Start with a lowercase letter and capitalize the first letter of each subsequent word.
- Example: myVariable, calculateTotalAmount(), com.example.package
-
PascalCase: We often use PascalCase to name classes and interfaces.
- Format: Start with an uppercase letter and capitalize the first letter of each subsequent word.
- Example: MyClass, MyInterface
-
ALL_CAPS: Use all capital letters to name constants.
- Format: Use all capital letters and separate words with underscores.
- Example: MAX_VALUE, PI
-
Meaningful Names: It is best practice to choose descriptive names for variables, methods, and classes to enhance code readability and maintainability. For example, instead of temp, use temperatureInCelsius.
-
Avoid Single-letter Names: Avoid using single-letter names for variables (except loop variables in short loops), as they often lack clarity. Instead, use descriptive. meaningful names that convey the purpose of the variable. For example, use index instead of just i for non-loop-related variables.
-
Package Naming: Use a reverse domain name format for package naming to avoid naming conflicts. For example: com.example.package.
-
Class and Interface Naming: Use nouns or noun phrases for classes and interfaces, as they represent objects or types. For example: UserProfile, DataProcessor, etc.
-
Method Naming: Use verbs or verb phrases for methods, as they represent actions or behaviors. For example: calculateTotal(), fetchData()
-
Abbreviations: Avoid excessive or unclear abbreviations. Use commonly understood abbreviations or spell out words fully for clarity. For example, use authentication instead of auth.
-
Consistency: Maintain consistency in naming conventions throughout your codebase to improve readability and maintainability.
By adhering to these naming conventions and best practices, you can ensure that your Java code is both easy to understand and consistent with industry standards. This not only makes your code more readable but also improves collaboration and maintenance.
What Is An Identifier Expected Error In Java?
When a compiler encounters a situation where it expects an identifier (such as a variable name, method name, or class name) but something else is provided, the compiler will encounter an "Identifier Expected" error in Java.
In other words, the "Identifier Expected" error occurs when the compiler anticipates an identifier but something else is provided. This error typically arises from syntax issues or incorrect naming conventions. The code snippet below demonstrates the "Identifier Expected" error.
public class Main {
public static void main(String[] args) {
int 1stNumber = 10; // Attempting to use a number as the variable name
System.out.println(1stNumber);
}
}
Compilation Errors- When you try to compile this code, the compiler will generate the following errors:
Main.java:3: error: <identifier> expected
int 1stNumber = 10; // Attempting to use a number as the variable name
^
Main.java:3: error: ';' expected
int 1stNumber = 10; // Attempting to use a number as the variable name
^
2 errors
Explanation: The error message indicates that the compiler expected a valid identifier but found a number (1stNumber) instead. In Java, identifiers must start with a letter, underscore (_), or dollar sign ($), and cannot start with a digit. This is why 1stNumber is invalid.
Corrected Code Example:
public class Main {
public static void main(String[] args) {
int firstNumber = 10; // Changed variable name to start with a letter
System.out.println(firstNumber);
}
}
In this corrected version, the variable name firstNumber starts with a letter, which is a valid identifier according to Java syntax rules.
So, the "Identifier Expected" error is caused by using invalid syntax for identifiers. To avoid this error, ensure that all identifiers in your code start with a letter, underscore, or dollar sign, and avoid starting identifiers with digits or using invalid characters. Following these guidelines will help maintain correct syntax and avoid compilation errors.
Reasons The Identifier Expected Error Occurs
As mentioned above, the "Identifier Expected" error in Java often results from issues related to incorrect syntax or invalid use of identifiers. Below are some common scenarios where this error might occur:
1. Invalid Identifier Naming
Identifiers in Java must begin with a letter, an underscore (_), or a dollar sign ($). Starting an identifier with a digit or using invalid characters leads to an error.
Code Example:
public class Example {
public static void main(String[] args) {
int 9lives = 7; // Incorrect: starts with a digit
}
}
Error Message:
error: <identifier> expected
int 9lives = 7;
^
Explanation: The identifier 9lives is invalid because it starts with a digit, which is not allowed.
2. Missing Identifier in Declarations
Forgetting to provide an identifier name during variable or method declarations will cause this error.
Code Example:
public class Example {
public static void main(String[] args) {
int = 10; // Incorrect or missing identifier
}
}
Error Message:
error: <identifier> expected
int = 10;
^
Explanation: The declaration int = 10 lacks an identifier for the variable, causing a syntax error.
3. Incorrect Syntax in Class or Method Declarations
Using reserved keywords or incorrect syntax for class or method declarations results in this error.
Code Example:
public class public { // Incorrect: "public" cannot be used as a class name
// body of class
}
Error Message:
error: <identifier> expected
public class public {
^
Explanation: The class name public is a reserved keyword (for access specifiers) and cannot be used as an identifier for a class.
4. Missing Method Name
Omitting the method name while declaring a method but providing parameters and a body leads to this error.
Example:
public class Example {
public void (int value) { // Incorrect: missing method name
System.out.println(value);
}
}
Error Message:
error: <identifier> expected
public void (int value) {
^
Explanation: The method declaration public void (int value) is missing a method name, which causes a syntax error.
How To Fix/ Resolve Identifier Expected Errors In Java?
To fix "Identifier Expected" errors, ensure that the syntax adheres strictly to Java’s rules for identifiers and declarations. Here are solutions for the issues listed above:
1. Correct Naming Conventions
Ensure that all variable names start with a valid character, such as a letter, underscore, or dollar sign. Avoid using reserved keywords as identifiers.
Corrected Example:
public class Example {
public static void main(String[] args) {
int nineLives = 7; // Corrected
}
}
2. Complete Missing Identifiers
Always include an identifier name when declaring a variable or method to avoid syntax errors.
Corrected Example:
public class Example {
public static void main(String[] args) {
int number = 10; // Added identifier name
}
}
The name number included in the updated example completes the variable declaration, making the syntax valid.
3. Use Valid Identifiers for Classes, Methods, and Interfaces
Avoid using reserved keywords or invalid syntax for naming classes, methods, or interfaces.
Corrected Example:
public class MyClass { // Changed from public to MyClass
// body of class
}
The class name has been changed from public to MyClass, which is a valid identifier in Java written in PascalCase.
4. Ensure Correct Method Declarations
Include the method name when declaring a method to avoid syntax errors.
Corrected Example:
public class Example {
public void printValue(int value) { // Added method name
System.out.println(value);
}
}
By following these guidelines and correcting syntax errors, you can resolve "Identifier Expected" issues effectively and enhance the clarity and correctness of your Java code.
Conclusion
Identifiers in Java- such as variable names, method names, class names, and interface names-are fundamental to writing clear and maintainable code. Proper usage of identifiers ensures that code is not only syntactically correct but also readable and functional.
- Identifiers must adhere to Java's rules, i.e., they should start with a letter, underscore (_), or dollar sign ($), and must not begin with a digit or use reserved keywords.
- Those identifiers that follow syntax rules and naming conventions are valid identifiers, and if not, they are referred to as invalid identifiers in Java.
- A situation where the compiler encounters an invalid identifier due to naming or syntactical issues results in an Identifier Expected error.
- Common causes include starting identifiers with numbers, using reserved keywords, or omitting identifier names in declarations.
To ensure correct use of identifiers in Java, you must follow naming conventions—such as using camelCase for variables and methods, PascalCase for classes and interfaces, and ALL_CAPS for constants. This way, developers can avoid these errors and enhance their code's readability and maintainability.
Also read: Top 100+ Java Interview Questions And Answers (2024)
Frequently Asked Questions
Q. How many types of identifiers are there in Java?
The names assigned to various components, like variables, methods, classes, packages, and interfaces, are known as identifiers in Java. These identifiers can be broadly categorized based on their usage and conventions:
- Class Names: Identifiers used to define class names should typically start with uppercase letters and follow the PascalCase convention, for example, String, ArrayList, etc. This helps in distinguishing class names from other types of identifiers.
- Interface Names: Similar to class names, interface names also start with uppercase letters and use the PascalCase contention, for example, Runnable and Serializable.
- Method Names: These should typically start with a lowercase letter and follow the camelCase convention. For example, toString() and addActionListener().
- Variable Names: These also begin with lowercase letters and follow the camelCase convention. For instance, firstName and orderNumber.
- Constants: They are usually defined using only uppercase letters with underscores to separate words (e.g., MAX_PRIORITY, MIN_VALUE). These are typically declared as static final.
- Package Names: Package names are typically all lowercase, to avoid conflicts with class names. For example, java.util, org.springframework.
- Generic Type Names: In generics, type parameter names are typically single uppercase letters. Common conventions include T for a generic data type, E for element type, K for key, and V for value.
- Enums: Enum names generally follow the same conventions as constants because they represent fixed sets of constants (e.g., ENUM_CONSTANT).
Q. Is 10 a valid identifier in Java?
No, 10 is not a valid identifier in Java because identifiers cannot begin with a digit. They must start with a letter (A-Z or a-z), an underscore (_), or a dollar sign ($). While digits can be included in an identifier, they cannot appear at the beginning. For example, number10 is a valid identifier, but 10number is not.
Q. What are the five rules of identifiers in Java language?
In Java, identifiers are the names given to variables, methods, classes, packages, and interfaces. They must follow certain rules to be valid. Here are the five primary rules for identifiers in Java:
- Alphanumeric Characters Only: Identifiers can only contain letters (A-Z, a-z), digits (0-9), underscore (_), and the dollar sign ($). No other characters, including spaces, are allowed.
- Cannot Start with a Digit: An identifier must begin with a letter (A-Z or a-z), an underscore (_), or a dollar sign ($). It cannot start with a digit.
- No Reserved Keywords: Identifiers in Java cannot be the same as the reserved keywords, like class, void, and static. This is because they have predefined meanings in Java's programming syntax.
- Case Sensitivity: Java identifiers are case-sensitive. This means that variable, Variable, and VARIABLE are considered different identifiers.
- No Length Limitation: While identifiers in Java can be of any length, shorter and meaningful names are generally preferred for readability.
Following these rules ensures that Java code is syntactically correct and avoids compilation errors related to invalid identifiers.
Q. Which identifier can be used as a class identifier in Java?
Class identifiers in Java must adhere to the same basic rules as other identifiers but follow additional conventions:
- Alphanumeric Characters and Symbols: Class names can include letters, digits, underscores (_), and dollar signs ($), just like any other identifiers in Java. However, using underscores and dollar signs in class names is uncommon.
- No Reserved Keywords: As per syntax rules, reserved keywords cannot be used as class identifiers in Java programs.
- Not Allowed to Begin with a Digit: Class names must start with a letter or an underscore (though a letter is preferred).
- Case Sensitivity: Java is case-sensitive, meaning MyClass and myclass are considered completely different classes.
- Naming Convention (PascalCase): By convention, class names start with an uppercase letter and follow PascalCase notation. For example, MyClass, AudioSystem, and Character.
Q. How do you identify Java identifiers?
Identifiers in Javam follow specific rules to write clear and error-free code:
- Composition: Can include letters (A-Z, a-z), digits (0-9), underscores (_), and dollar signs ($). They cannot start with a digit.
- No Reserved Keywords: Identifiers cannot be Java reserved words like class or int.
- Case Sensitivity: Identifiers are case-sensitive (variable is different from Variable).
- Unlimited Length: No length limit, but shorter, meaningful names are recommended.
- Conventions: Class names start with uppercase (PascalCase), methods and variables start with lowercase (camelCase), and constants are all uppercase with underscores (e.g., MAX_HEIGHT).
Q. What is an example of an invalid identifier in Java?
An example of an invalid identifier in Java would be a variable name starting with a digit, such as 123abc, violating the rule that identifiers cannot begin with a number.
Q. What is an example of a valid identifier in Java?
Some examples of valid identifiers in Java are myVariable, user1, _temp, $result, MAX_VALUE, myMethod(), Car, etc. These examples adhere to the syntax rules for definition identifiers in Java.
Here are a few more articles you must explore:
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment