Key Features Of Java, Java 8 & Java 11 Explained (+Code Examples)
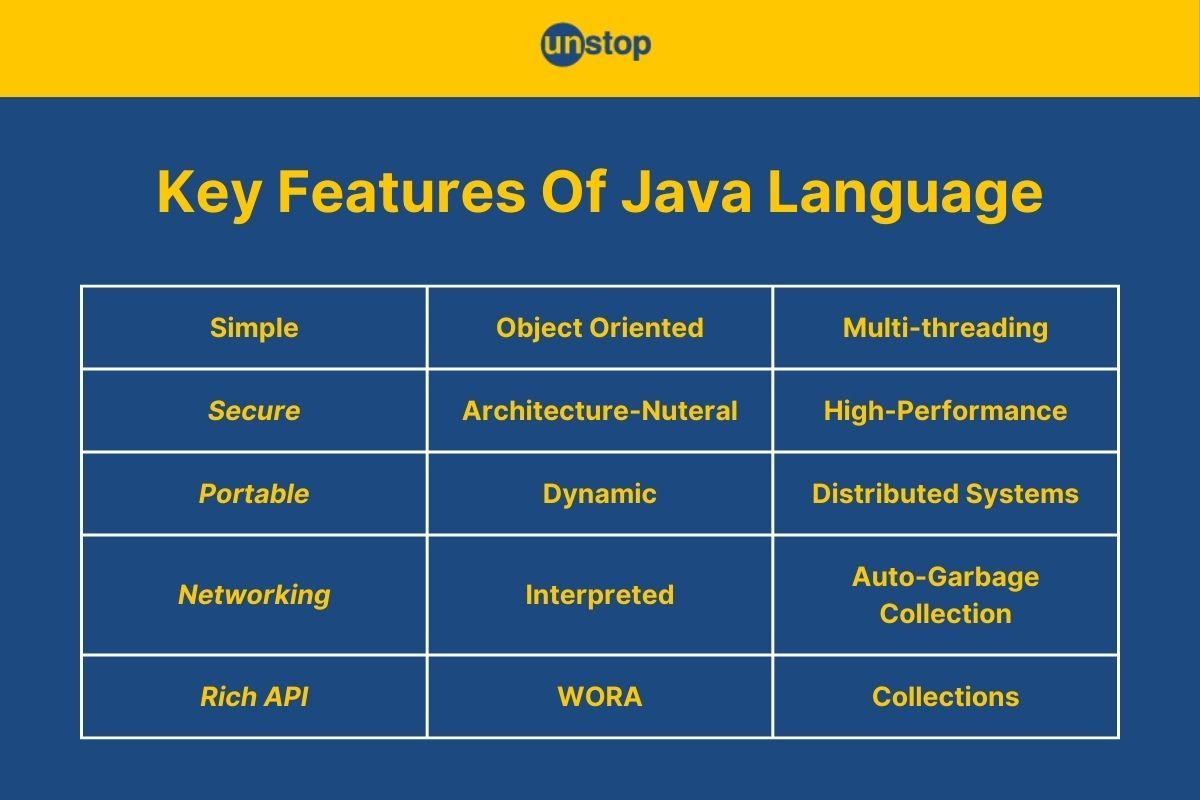
Table of content:
- Java Programming Language | An Introduction
- 15 Key Features Of Java
- Write Once Run Anywhere (WORA) | Features Of Java
- Java Editions
- 5 New Features Of JAVA 8
- 5 New Features Of JAVA 11
- What Makes Java Popular?
- Conclusion
- Frequently Asked Questions
If you are looking for coffee and have ended up here, we are sorry to disappoint. If you are looking for comprehensive info on features of Java programming language, then read till the end. Java, a purely object-oriented programming language, is one of the most reliable, flexible, and widely used languages out there. One of the basic concepts behind developing Java was to provide developers the ability to write one and run anywhere (WORA).
In this article, we will explore the key features of Java that have made it one of the most popular programming languages used by millions of developers worldwide.
Java Programming Language | An Introduction
Java is an object-oriented, class-based, high-level programming language intended to be implementation-independent. It was primarily developed by James Gosling and released in 1995 by Sun Microsystems.
- The language derives much of its syntax from C and C++, but it has fewer low-level facilities than either of them.
- Java’s greatest challenge lay in producing an architecture-neutral language that could be run over quite a wide range of hardware and software.
- Out of these notions developed the idea of “Write Once, Run Anywhere” (WORA), a capability that has since developed as a central aspect of Java philosophy.
- Java programs are compiled into bytecode, which is usually a kind of intermediate language that can run on any computer architecture, such as Java Virtual Machine (JVM) implementation.
Fun Fact: It is widely believed that Oracle developed Java and not Sun Microsystems. But this is only partially correct. The language was actually developed by a team called the Green Team, headed by James Gosling at Sun Microsystems. However, one can say that Oracle owns Java since it acquired Sun Microsystems in 2010.
15 Key Features Of Java
Java stands as a beacon in the programming world, celebrated for its robust set of features that cater to developers seeking efficiency, security, and cross-platform capabilities. Let’s delve into these defining characteristics that make Java the programming language of choice for millions.
Simplicity Feature Of Java
Java was designed with simplicity in mind, aiming to be as accessible and straightforward as possible for programmers. This simplicity is evident in several aspects of the language, from its syntax to its removal of complex features found in other languages.
Here are a few Java features/ components that highlight its simplicity:
- Syntax Similar to English: Java's syntax is clean and readable, closely resembling human language, which makes it easier to understand and write.
- No Pointers: Unlike C/C++, Java does not use pointers (variables that store the memory address of another variable), which simplifies memory management and enhances security.
- Automatic Garbage Collection: Java automatically manages memory allocation and deallocation, freeing developers from the burden of manual memory management.
- Exception Handling: Java's robust exception handling allows for managing runtime errors and exceptions systematically, reducing the chances of crashing.
Java Example: A Classic Hello World Program
Let’s begin by illustrating the simplicity of Java through a hello world program The simple program is designed to introduce the fundamental structure of a Java application and to demonstrate how straightforward it is to execute a Java program that performs a basic task—printing text to the console.
Code Example:
Output:
Hello, World!
Explanation:
- We begin by defining a public class named HelloWorld, which is a user-defined blueprint for creating objects or, in this case, a container for the application's entry point.
- Inside the class, we have a main() method, which is the entry point of any Java application. (The method is executed by the Java Virtual Machine when the program starts.)
- Inside the main() method, we have a single line of code that performs the action of the program- System.out.println("Hello, World!");.
- This line uses the System class from the Java Standard Library, and System.out is an instance of PrintStream. It is commonly used for outputting information to the console.
- The println() method prints its argument to the console and then terminates the line.
- When executed, the program outputs the phrase "Hello, World!" to the console, confirming that the Java environment's setup, compilation, and execution processes are correctly configured.
Object-Oriented Features Of Java
Java is inherently object-oriented, which means it uses classes and objects to model real-world entities. Object-oriented programming (OOP) in Java is centred around four main principles: encapsulation, inheritance, abstraction, and polymorphism. These principles/ concepts of OOPs make Java programs more modular, flexible, and maintainable.
Encapsulation | Feature Of Java
Encapsulation entails bundling the data (attributes) and methods (functions) that operate on the data into a single unit or class and restricting access to some of the object's components. This concept is often used to hide the internal representation, or state, of an object from the outside. In Java, this is achieved through access modifiers like private, protected, and public.
public class Person {
private String name; // Private variable, encapsulated
public String getName() {
return name;
}
public void setName(String newName) {
this.name = newName;}
}
The Person class has a private field name, which is encapsulated within the class. External access to name is provided through the getName() and setName() methods, allowing controlled access to the private data.
Inheritance | Feature Of Java
Inheritance allows a new class to inherit the properties and methods of an existing class. The new class is called a subclass, and the existing class is known as the superclass. This mechanism provides a way to organize and structure software.
public class Employee extends Person {
private double salary;
public double getSalary() {
return salary;
}
public void setSalary(double newSalary) {
this.salary = newSalary;}
}
Here, the Employee class inherits from the Person class, meaning it can use the name property along with its getters and setters, in addition to adding new properties like salary.
Abstraction | Feature Of Java
Abstraction means hiding the complex implementation details and showing only the necessary features of an object. In Java, abstraction is achieved using abstract classes and interfaces.
public abstract class Animal {
public abstract void sound();
}
public class Dog extends Animal {
public void sound() {
System.out.println("Bark");}
}
Here, the Animal class is abstract and includes an abstract method sound(). The Dog class extends Animal and provides an implementation for the sound() method.
Polymorphism | Feature Of Java
Polymorphism allows objects of different classes to be treated as objects of a common superclass. It's the ability of a single function or method to work in different ways depending on the context.
Animal myDog = new Dog();
myDog.sound(); // Outputs "Bark"
Here, the Animal reference myDog points to an instance of Dog. When the sound() method is called on myDog, it executes the Dog class's version of the method, demonstrating polymorphism.
These OOP Java principles are fundamental in enabling developers to write clean, modular, and reusable code.
Security Features Of Java
Security is a cornerstone of Java's design, offering a robust framework that protects applications from both internal and external threats. Java's security model includes a variety of built-in security features designed to safeguard data and systems from malicious attacks, unauthorized access, and unintended code execution. Here are a few features of Java that make it a secure language:
Bytecode Verification | Feature Of Java
The Java Virtual machine (JVM) verifies the Java bytecode before execution.
- It checks the code against a set of rules to ensure that it doesn't perform any dangerous operations, such as accessing local variables without proper initialization or violating access rights.
- This forms the first line of defence for Java code, safeguarding against many common vulnerabilities.
Sandbox Model | Feature Of Java
Java operates on a "sandbox" security model, especially in the context of Java applets (though less common today), where code from unknown sources is run in a restricted environment. This sandbox limits access to system resources and APIs, effectively isolating the execution and preventing the code from performing potentially harmful operations on the host machine.
Secure Class Loading | Feature Of Java
Java's class loader architecture plays a crucial role in its security model.
- It allows the JVM to load classes at runtime from trusted sources, ensuring that classes are not replaced by potentially malicious versions.
- Additionally, Java provides mechanisms for separating namespace hierarchies, preventing classes from unauthorized packages from accessing classes within restricted packages.
Cryptography | Feature Of Java
Java provides extensive support for cryptography through its Java Cryptography Architecture (JCA) and Java Cryptography Extension (JCE). These frameworks offer APIs for secure key generation, encryption, decryption, and other cryptographic operations, enabling the secure transmission and storage of data.
Cipher cipher = Cipher.getInstance("AES/CBC/PKCS5Padding");
cipher.init(Cipher.ENCRYPT_MODE, secretKey);
byte[] encryptedData = cipher.doFinal(plainText.getBytes());
This code snippet demonstrates how Java's Cipher class can be used to encrypt data using the AES algorithm. The Cipher class is part of Java's cryptographic framework and provides a secure way to handle encryption and decryption operations.
Robustness Features Of Java
Java's reputation for robustness is grounded in its strong emphasis on early error checking, runtime checking, safe/ strong memory management, and exception handling mechanisms. These features collectively make Java programs remarkably stable and resilient to crashes.
Early and Runtime Checking | Feature Of Java
Java performs early checks at compile-time and rigorous runtime checks, significantly reducing the chances of errors slipping through to the execution phase.
- Type-checking at compile-time ensures that all operations are type-safe.
- Array bounds-checking at runtime prevents out-of-bounds errors, a common source of security vulnerabilities in other languages.
- Example: Java automatically checks array bounds and throws an ArrayIndexOutOfBoundsException if an attempt is made to access an array element outside of its bounds, preventing undefined behavior or memory corruption.
Exception Handling | Feature Of Java
Java's exception handling framework forces developers to handle error conditions either by catching the exception and dealing with it or by declaring it and letting it propagate. This explicit handling of exceptional scenarios ensures that the program can recover from errors gracefully or fail securely without exposing the system to further risk.
try {
// Code that might throw an exception
int result = 10 / 0;
} catch (ArithmeticException e) {
// Handling division by zero error
System.out.println("Division by zero is not allowed.");
}
The code fragment above demonstrates Java's try-catch block, which is used to catch and handle the ArithmeticException that occurs when dividing by zero, thereby preventing the program from crashing unexpectedly.
Safe Memory Management | Feature Of Java
Java's garbage collection mechanism automatically reclaims memory used by objects that are no longer needed, which helps prevent memory leaks and memory corruption issues. By managing memory allocation and deallocation behind the scenes, Java eliminates the risks associated with manual memory management, such as dangling pointers and memory fragmentation.
Together, these features of Java contribute to its robustness, making it a reliable choice for developing high-quality, durable software applications that can withstand the rigours of real-world usage.
Architecture-Neutral | Features Of Java
Java's architecture-neutral nature is one of its most distinctive features, which enables Java programs to run on any hardware and operating system without modification. This is made possible by the Java Virtual Machine (JVM), which abstracts the underlying hardware and OS from Java bytecode, the intermediate form into which Java source code is compiled.
How Does This Feature Of Java Work?
- When you compile a Java program, the compiler converts your source code into bytecode.
- This bytecode is a platform-independent code that can be executed by any JVM, regardless of the underlying computer architecture.
- This means that the same Java program can run on Windows, macOS, Linux, or any other operating system that has a compatible JVM without needing to recompile the code for each platform.
- For example, a Java program compiled on a Windows machine can be executed on a Linux or macOS machine without any modifications or recompilation, provided that a JVM is installed on those machines.
Fun Fact: The language was first named Oak in reference to an oak tree that was growing just outside Gosling’s office. Later, it was called Java, derived from the kind of coffee consumed on an island of Indonesia. This is also reflected in Java’s logo, which is a steaming cup of coffee.
Portability | Features Of Java
Java's portability is a crucial feature that goes hand-in-hand with its architecture-neutral design. It ensures that Java programs can be transferred and executed across different environments with ease. This portability is largely attributed to the Java Virtual Machine (JVM), which serves as an intermediary between Java bytecode and the underlying hardware.
Core Aspects of Java's Portability Feature:
- Standardized Runtime Environment: The JVM provides a consistent runtime environment across all platforms, meaning Java programs can expect the same behavior regardless of where they're executed.
- No Platform-specific Features: Java avoids the use of platform-specific libraries or functions, which means that Java code does not have to be adapted or rewritten to run on different operating systems or architectures.
- Bytecode Universality: Java bytecode is the universal language of the JVM, and because bytecode is the same regardless of the original platform where the code was compiled, it can be executed on any device that has a JVM.
Example: A Java application developed and compiled on a Mac can be executed on Windows, Linux, or any other platform with a JVM, without needing any modifications to the source code or the compiled bytecode.
High-Performance Features Of Java
Java is designed to offer high performance for all types of applications, challenging the notion that high-level, platform-independent languages must sacrifice speed for portability and ease of use. Here are the key features of Java that make it a high performance powerful programming language:
Just-In-Time (JIT) Compilation | Feature Of Java
Java bytecode is initially interpreted, which allows for platform independence and portability. However, the Java Virtual Machine (JVM) also includes a Just-In-Time (JIT) compiler that compiles bytecode into native machine code at runtime. This means that frequently executed sections of code can run much faster, as they're executed directly by the hardware after the initial compilation.
Optimized Garbage Collection | Feature Of Java
The automatic garbage collection feature of Java is highly optimized to efficiently manage memory. Modern JVMs implement sophisticated algorithms to minimize the impact of garbage collection on application performance, ensuring that memory management is both effective and efficient.
Adaptive Optimization | Feature Of Java
The JVM monitors running Java programs and can optimize performance in real time by identifying and recompiling frequently executed paths for better performance, a process known as adaptive optimization.
Example: While the exact performance improvements depend on the specific JVM implementation and the nature of the application, Java programs can achieve performance close to native applications through JIT compilation and runtime optimizations.
Distributed | Features Of Java
Java inherently supports distributed computing, enabling developers to create applications that can run across multiple computers or devices, working together over a network. This feature is crucial for developing large-scale, network-based applications that require resources to be shared and tasks to be executed collaboratively across different systems.
Key features of Java's distributed computing support:
- Remote Method Invocation (RMI): Java's RMI allows an object on one Java virtual machine to invoke methods on an object in another JVM, even if they're located on different machines. This makes it easier to design and implement distributed applications.
- Java Naming and Directory Interface (JNDI): JNDI provides a unified interface to multiple naming and directory services, allowing Java applications to discover and look up data and resources across a network.
- Java Message Service (JMS): For applications that need to reliably send and receive messages between two or more computers, JMS offers a powerful, loosely-coupled communication mechanism.
Example: An e-commerce application that uses Java's distributed computing features can handle user requests on the front end, process transactions on server clusters, and manage inventory data stored on different databases. All this data is distributed across various locations but works seamlessly as a unified system.
Multi-Threading Feature Of Java
Java provides built-in support for multi-threaded programming, which allows for the concurrent execution of two or more parts of a program. This capability enables Java applications to perform multiple tasks simultaneously, improving performance and responsiveness, especially in applications requiring complex operations or real-time processing.
Highlights of Java's multithreading feature include:
- Thread Class and Runnable Interface: Java allows for the creation of new threads by extending the Thread class or implementing the Runnable interface, offering flexibility in how threads are managed and executed.
- Synchronization: To ensure data consistency and thread safety, Java provides synchronized blocks and methods, allowing threads to lock resources when they are being updated.
- Concurrency Utilities: Beyond basic thread management, Java offers a rich set of concurrency utilities under the java.util.concurrent package. This simplifies the development of complex concurrent applications with more advanced features like thread pools, atomic variables, and blocking queues.
Code Example:
Output:
Hello from a Unstop!
Explanation:
- The HelloThread class is defined as a subclass of Java's built-in Thread class. Here, HelloThread inherits the methods and properties of Thread. This is done by extending Thread class enabling it to behave like a thread.
- Within HelloThread, the run() method is overridden. The run method is the heart of any thread as it defines the code that constitutes the new thread's task.
- In this case, when the thread runs, it simply prints the message "Hello from Unstop!" to the standard output.
- In the TestThread class, the main() method serves as the application's entry point. Here, we create a new instance of HelloThread and then call the start() method on this instance.
- This call triggers the JVM to create a new thread of execution and further call the HelloThread object's run method. This causes the message to be printed, while the main method could potentially be doing other work.
- The creation and starting of the thread are done in one line by chaining the constructor call with the start method call.
Dynamic | Features Of Java
Java is designed to be a dynamic and versatile programming language capable of adapting at runtime to changes in the environment or application. This feature of Java is reflected in several key areas, which include the following:
- Runtime Class Loading: Java can load classes at runtime, meaning new code can be incorporated and used within a running application without needing to restart it. This is particularly useful for modular applications and plugins.
- Reflection: Java's reflection API allows programs to inspect and manipulate objects and classes at runtime. This includes the ability to discover class fields, methods, and constructors, invoke methods, and create instances dynamically.
- Dynamic Invocation: Introduced in later versions of Java, the java.lang.invoke package supports dynamic language features, allowing method calls to be resolved only at runtime. This is especially beneficial for scripting languages implemented atop the JVM.
Example:
// Using reflection to dynamically invoke a method
Class<?> clazz = Class.forName("java.lang.String");
Method method = clazz.getMethod("toUpperCase");
System.out.println(method.invoke("hello"));
This block of code demonstrates how we can use reflection to dynamically load the String class, retrieve the toUpperCase method, and invoke it on a sample string. This showcases Java's ability to perform operations that are determined at runtime rather than compile-time, reflecting its dynamic nature.
Networking | Features Of Java
Java provides extensive support for networking, allowing developers to easily create applications that communicate over the internet or local networks. With its rich set of APIs, Java simplifies the development of network applications, from low-level socket programming to high-level internet services.
Some key features of Java highlighting its networking capabilities include:
- java.net Package: This package includes classes and interfaces for implementing networking functionalities such as sockets, which are used to establish a connection between two machines over a network for data exchange.
- URL and HttpURLConnection: Java allows applications to access and manipulate data on the internet through classes like URL and HttpURLConnection, making web communication and data retrieval straightforward.
- ServerSocket and Socket: These classes enable the creation of server and client applications that communicate through TCP/IP. Developers can build robust server-side applications that listen for connections from clients, process requests, and send responses.
Code Example:
Explanation: The blocks of code demonstrate how to create a basic client that connects to a server using a Socket, sends an HTTP GET request, and prints the server's response. This illustrates Java's capability to handle low-level network communications with ease.
Interpreted | Features Of Java
Java blends compiled and interpreted programming paradigms to offer a unique advantage in terms of flexibility and performance.
- While Java programs are compiled into platform-independent bytecode, this bytecode is not directly executed by the hardware.
- Instead, it's interpreted by the Java Virtual Machine (JVM) on whichever platform the application is running on.
Example: A Java program, when compiled, generates bytecode (.class files) that is not tied to any specific type of hardware. When this program is run, the JVM on the host system interprets the bytecode into the host's machine code, allowing it to be executed.
Automatic Garbage Collection | Features Of Java
Java simplifies memory management through its automatic garbage collection (GC) mechanism.
- It systematically identifies and disposes of objects that are no longer in use, freeing up memory resources without manual intervention.
- This feature of Java is a significant boon for developers, as it reduces the risk of memory leaks and other memory-related issues that can degrade application performance and stability.
Example: In a Java program, when an object is created using the new keyword, memory is allocated. Once there are no more references to that object (meaning the object is no longer needed), the garbage collector will eventually reclaim the memory, making it available for new objects.
public class GarbageCollectionExample {
public static void main(String[] args) {
String str = new String("Example");// At this point, the object "str" is in use and cannot be collected.
str = null;
// Now, there are no references to the String object. It becomes eligible for garbage collection.
}
}
In this example, once the reference str is set to null, the String object it previously pointed to becomes eligible for garbage collection, as there are no more references to it. The garbage collector will automatically free the memory at an appropriate time determined by the JVM.
Simplified Syntax | Features Of Java
Java's syntax is designed to be clear and concise, making it easier for developers to read and write code efficiently.
- This simplicity aids in reducing the likelihood of errors, enhancing maintainability, and facilitating faster development cycles.
- Java achieves this by eliminating certain complexities found in other languages, such as pointers, operator overloading, and multiple inheritance, focusing instead on a straightforward programming model.
Code Example:
Output:
Sum of 1 to 10 is: 55
Explanation: This example demonstrates Java's clear syntax through a loop that calculates the sum of numbers from 1 to 10.
- Java's for-loop construct is easy to understand, with all loop control elements (initialization, condition, and increment) neatly placed together.
- The += operator succinctly expresses addition and assignment in a single step, while the System.out.println method clearly outputs the result.
Rich API | Features Of Java
Java boasts a comprehensive and rich Application Programming Interface (API) that spans various functionalities. This includes input/output (I/O), networking, utilities, XML parsing, database connection, and more. The extensive API provides developers with a vast toolkit, enabling them to build complex applications with minimal effort and without reinventing the wheel.
Code Example:
Output:
Java
API
Explanation: The example showcases Java's rich API and its convenience for developers.
- By utilizing the ArrayList from the Java Collections Framework, the example highlights how effortlessly one can manipulate lists.
- The addition of "Java" and "API" to the list, followed by the use of the forEach method with a method reference (System.out::println), exemplifies the seamless integration of functional programming concepts into Java.
Write Once Run Anywhere (WORA) | Features Of Java
The Write Once, Run Anywhere (WORA) principle is a hallmark feature of Java programming language, emphasizing its cross-platform capabilities.
- It ensures that Java code is written and compiled only once.
- That is, any device on which there is an installed Java Virtual Machine (JVM) can run without concern for the hardware and operating system on that device.
- This capability exists because the bytecode acts as an intermediate code for the compiled Java code and does not require machine targeting. This bytecode will have to be interpreted or compiled at runtime by the JVM into native machine code.
How Does The WORA Feature Of Java Work?
- Java Source Code: This is the code that developers write in the Java programming language. It is typically human-readable and saved with a .java extension.
- Java Compiler: When you compile your Java source code, the Java compiler (javac) translates the human-readable .java files into Java bytecode (instructions that JVM can understand). These instructions are not specific to any one processor or operating system, which is crucial to the WORA principle.
- Java Byte Code: The output of the compilation process is the bytecode contained within .class files. This bytecode is the intermediary form of your program that sits between the source code and the machine code.
- Java Runtime Environment (JRE): For the bytecode to be executed, it must be run on a Java Runtime Environment, which includes the Java Virtual Machine (JVM) along with the necessary libraries and resources to run the Java application. There are different JREs for different operating systems (Windows, Unix, Mac, and Android), but they all understand the same Java bytecode.
At runtime, bytecode instructions are either interpreted instruction by instruction by the JVM or compiled into native machine code through JIT compilation to be executed directly by the host processor. This is done on the fly and in memory, hence the high performance is exhibited.
Example: Temperature Converter
The following example illustrates a simple Java application that converts temperatures from Celsius to Fahrenheit and vice versa.
Code Example:
Output:
20.0 C = 68.0 F
68.0 F = 20.0 C
Explanation:
- We begin by declaring a public class TemperatureConverter containing two static methods. Public means it can be accessed from outside its package.
- The celsiusToFahrenheit() method takes a temperature in Celsius as an argument, convert it to Fahrenheit equivalent using the formula (celsius * 9/5) + 32 and returns the same.
- The fahrenheitToCelsius() method takes a temperature in Fahrenheit as an argument, converts it to Celsius with the formula (fahrenheit - 32) * 5/9 and returns the same.
- Static methods are used here to allow temperature conversion without instantiating the class, emphasizing their utility.
- Then, we have the main() method, which is the entry point of the program, where the program starts execution.
- Inside main(), we declare two variables of double data type. Variable celsius is initialized with value of 20, and variable fahrenheit is initialized to 68.
- We then use the print() stream function and call conversion methods with these values.
- The function calls print the converted value, concatenating the result in a string.
- The output confirms the correctness of our conversions, as 20.0 C equals 68.0 F, and conversely, 68.0 F converts back to 20.0 C.
This reaffirms the expected behavior of our program, that is runs on any system (Windows, Mac, Unix, etc.) with a compatible Java Runtime Environment.
Java Editions
Java comes in several editions, each offering different development environments and targeting various devices and applications. Each edition offers a unique set of features, libraries, and APIs meant to be used for specific tasks.
Java Standard Edition (Java SE): The Java SE Platform is the foundation for developing and running applications in general environments, from desktops to servers. It includes the full Java Runtime Environment (JRE), the Java compiler (javac), and APIs for core utilities, graphical user interface (GUI) components, networking, security, and database access, among others.
Java Enterprise Edition (Java EE): Building on Java SE, Java EE provides additional libraries and APIs for scalable, multi-tier server-side enterprise application development. It supports web services, enterprise JavaBeans (EJBs) based on component enterprise architectures, and a wide range of web technologies, from JavaServer Pages (JSP) to Servlets.
Java Micro Edition (Java ME): Java ME tailors Java SE for software development on small devices with limited capacities, such as mobile phones. It offers different configurations and profiles to accommodate classes of devices.
JavaFX: JavaFX has been introduced as a platform for developing and delivering rich internet applications that can operate across a wide range of devices, including network computers and mobile or TV devices. JavaFX applications can incorporate web view, 2D or 3D graphics, audio and video, and touchscreen support.
5 New Features Of JAVA 8
Java 8, released in March 2014, introduced several major features that enhanced the language and platform. These include:
- Lambda Expressions: This feature of Java 8 enables clearer and more concise code, especially for filtering, extracting, and sorting data.
- Streams API: Introduced a new abstraction called Stream that allows processing sequences of elements in a functional style.
- Date and Time API: Added a new API for dates and times to improve on the older, less convenient java.util.Date and java.util.Calendar.
- Default Methods: This Java feature allowed methods to be added to interfaces without breaking the existing implementation of these interfaces.
- Nashorn JavaScript Engine: A new lightweight, high-performance JavaScript runtime built into Java.
5 New Features Of JAVA 11
Java 11, released in September 2018, continued to add improvements and new features to the Java platform. These include:
- Running Java File with Single Command: Enhanced the java launcher to run a single-file source-code program directly, simplifying execution and testing of small programs.
- New HTTP Client: Standardized HTTP client API that replaced the legacy HttpURLConnection and provided support for HTTP/2.
- Local-Variable Syntax for Lambda Parameters: Introduced the var keyword to declare the formal parameters of implicitly typed lambda expressions, enhancing readability.
- Improved Collection API: Added new methods to the Collection API, such as isEmpty default method in the Collection interface.
- String Enhancements: Introduced new methods for strings, such as repeat, isBlank, strip, stripLeading, stripTrailing, and lines, to perform common string operations more efficiently.
What Makes Java Popular?
Java's enduring popularity and relevance in the programming world can be attributed to several key features and philosophical underpinnings that make it a preferred choice for developers. Here are some of those pivotal features of Java:
- Platform Independence: Java with its principle of "Write Once, Run Anywhere," allows developers to write code once and deploy it across various platforms without modification. This enables applications to run on everything from small devices to supercomputers, seamlessly bridging the gap between a variety of operating systems available out there.
- Object-Oriented Design: Java’s design is profoundly object-oriented, simplifying coding by modelling it on real-world objects.
- Security and Memory Management: Java provides robust protection against unauthorized access, making it a safe choice for running code from any part of the globe.
- Built-in Concurrency: Java natively supports multi-threading, allowing applications to perform multiple tasks simultaneously. This feature of Java is essential for modern software that demands high responsiveness and fast processing.
- Rich Library and Ecosystem: Java offers an extensive library and ecosystem providing developers with myriad tools, frameworks, and resources necessary for building various applications. Including support for graphical user interfaces, complex data structures, and network connections.
- Vibrant Community and Continuous Evolution: Java has a vibrant community of developers who share knowledge and collaborate on innovative solutions, continuously pushing the boundaries of what can be achieved with the language.
Conclusion
Java is a versatile, powerful, yet simple programming language. The popularity and efficiency of this langauge have steadily grown over the years. Today, it has a strong foothold in the world of enterprise-level application development, mobile application development (specifically Android apps), web development, server-side applications, gaming, and so much more.
- The simplicity and object-oriented features of Java streamline the development process, while its security and robustness ensure reliable and secure software.
- The architecture-neutral and portable features of Java allow developers to write code that can run on any platform, epitomizing the "Write Once, Run Anywhere" philosophy.
- Also, the high performance aspects, distributed capabilities, and multi-threading features of Java make it ideal for modern, scalable applications.
- Its dynamic nature, extensive networking support, interpreted execution, and automatic garbage collection further enhance its appeal.
- The language's simplified syntax and rich API provide a user-friendly environment for both new and experienced programmers.
Additionally, with the continual introduction of new features in Java 8 and Java 11, Java remains a cutting-edge language that evolves with the demands of the industry. Whether you're building simple applications or complex systems, Java's comprehensive features and enduring relevance make it a top choice for developers worldwide.
Also Read: Top 100+ Java Interview Questions And Answers (2024)
Frequently Asked Questions
Q. How does Java's performance compare to other programming languages, especially newer ones?
Java is naturally often compared to many old languages (like C/C++) and modern languages (like Python, Go, or Kotlin). Key features of Java that help it compete against the new languages include:
- JIT Compilation and HotSpot Optimization: The JVM will automatically improve the performance of any running Java application over time through its technique for optimizing the code running process on a real-time basis.
- Garbage Collection: Advanced garbage collection algorithms reduce overhead and enhance memory management.
- Mature Ecosystem: Over the years, Java has formed a big ecosystem of libraries and tools to improve performance, together with a great number of JVM optimizations.
Q. Can Java be used for modern web development practices and frameworks, such as microservices and serverless computing?
Yes, Java is best suited for the modern web development practices, like the emergence of microservices and serverless computing. Its powerful ecosystem, mature libraries, and frameworks make it an appealing language for engineers in building scalable and high-performance web applications.
Microservices: Java has many readily available frameworks and tools tailor-made for the microservices architecture, such as Spring Boot, Micronaut, and Quarkus.
- These help in the easy formation of independently deployable, small, modular services that can be composed to provide a complex application.
- Such capabilities include service discovery, configuration management, built-in support for RESTful communication, and much more that is required in the development of microservices.
- Further, Java is perfectly supported in Docker and Kubernetes, allowing one to easily containerize and orchestrate any kind of service when developing more complex microservices.
Serverless Computing: Technologies such as AWS Lambda, Azure Functions, Google Cloud Functions, are fully supporting Java. This means that a developer will be in a position to write functions in Java and have them execute in reaction to certain events.
- The Platform as a Service level abstracts away all the underlying infrastructure and allows developers to put their full focus only on their code.
- Deployment, scaling, and execution are then handled fully by the platform.
- Recent Java frameworks and JDK improvements target the startup time and memory consumption of serverless applications, which have been the performance and cost concerns.
These features of Java programming language make it suitable for microservices development and serverless computing.
Q. What are the security best practices when developing applications in Java?
When developing applications in Java, adhering to security best practices is crucial to safeguard against vulnerabilities and threats. These include:
- Keep Java and Libraries Updated: Regularly update the JDK and third-party libraries to their latest versions to mitigate known vulnerabilities.
- Use Secure Coding Practices: Follow secure coding guidelines, such as validating input to prevent injection attacks and escaping data when working with HTML, SQL, or LDAP to guard against cross-site scripting (XSS) and injection vulnerabilities.
- Manage Dependencies: Utilize tools like OWASP Dependency-Check to identify and remove unused or outdated libraries that might introduce security risks.
- Implement Proper Error Handling: Avoid revealing sensitive information in error messages. Use generic messages for errors and log detailed information securely for debugging purposes.
- Encrypt Sensitive Data: Use strong encryption algorithms for data at rest and in transit. Employ HTTPS for network communication and secure sensitive information using Java Cryptography Architecture (JCA) for encryption and decryption.
- Secure Session Management: Use secure session management practices, including secure cookies (with HttpOnly and Secure flags), and avoid exposing session IDs in URLs.
Q. How does Java handle the development of artificial intelligence (AI) and machine learning (ML) applications?
Java is well-equipped for developing Artificial Intelligence (AI) and Machine Learning (ML) applications thanks to its robustness, ease of use, and rich set of libraries and tools. Key features of Java that make it suitable for AI/ML tasks include:
- Libraries and Frameworks: Java offers a wide array of libraries and frameworks for AI and ML, such as Deeplearning4j for deep learning, Weka for data mining, and MOA (Massive Online Analysis) for data stream mining, making it easier to implement complex algorithms and processes.
- Platform Independence: Java’s platform independence allows AI and ML applications to be deployed across various environments without modification, facilitating the development and distribution of solutions.
- Performance: With features like automatic memory management and multithreading, Java enables efficient processing of large datasets, a common requirement in AI and ML projects.
- Community and Support: A vast and active community, along with extensive documentation and resources, provides valuable support for developers working on AI and ML projects in Java.
Q. What is the future of Java in the era of containerization and cloud-native applications?
Java's future in containerization and cloud-native applications remains bright, thanks to ongoing enhancements aimed at optimizing Java for these environments. Key developments include:
- Reduced Footprint: Efforts to minimize Java's runtime footprint and startup time, which are critical for containerized applications, are ongoing. Projects like GraalVM contribute to this by providing ahead-of-time compilation.
- Microservices and Serverless Support: Java frameworks like Spring Boot, Micronaut, and Quarkus are designed to support microservices and serverless architectures, offering fast boot times and low memory footprint.
- Cloud-Native Integration: Java continues to integrate deeply with cloud-native technologies, including Kubernetes and Docker, facilitating seamless deployment, scaling, and management of Java applications in the cloud.
Java's adaptability, combined with improvements in efficiency and cloud-native support, ensures its continued relevance and utility in developing modern, scalable, and efficient applications in the cloud era.
Q. How does Java support cross-platform mobile application development, especially compared to frameworks like Flutter and React Native?
Java itself isn’t inherently created for cross-platform mobile development but its strength in cross platform mobile delepoment comes from its frameworks. Java really shines through with frameworks such as Codename One. These put an abstraction layer in between the native mobile platform be it iOS or Android and the Java code.
We write our app logic in Java, and that is translated for each targeted platform. Listed in the table below are some advantageous and disadvantageous features of Java in this regard.
Advantages | Disadvantages |
|
|
Comparison with Flutter and React Native:
- Language: Both JavaScript (React Native) and Dart (Flutter) have their pros and cons. However, JavaScript is a lot more prevalent, making React Native an easy choice for many who are already used to working with web development.
- Performance: A performance comparison between Flutter and React Native would most probably differ, but in general, they both offer closer-to-native performance due to the use of a UI rendering engine.
- UI Customization: Flutter and React Native provide more flexibility in creating platform-specific UIs.
Q. What are the implications of the modular system introduced in Java 9 for large-scale application development?
The introduction of the modular system in Java 9, known as Project Jigsaw, brought significant benefits for large-scale application development. The key features of Java modular system include:
- Improved Scalability and Performance: Arranging code in well-defined dependency modules enabled Java applications to do well in performance and scale nicely along with reducing the overall memory footprint.
- Security and Maintainability from a Human Perspective: The encapsulation of internal APIs that modules offer is so tight that this would enhance security by avoiding any unintended access. It also supports maintainability through having well-defined interfaces between the components.
- Simplified Dependency Management: Any modular system significantly simplifies dependency management. This implies that it becomes easier to understand and manage components an application relies on, thus reducing conflicts and predictably making the building process more straightforward.
- Facilitated Decomposition of Monoliths: For legacy applications, modules enabled the gradual evolution of the monolithic codebases to reasonably sized, modular architectures, and that allows them to facilitate moving to microservices or any other modern architecture.
This compiles our discussion on the features of Java programming language. Do check out the following for more such interesting reads:
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment