Java Keywords | List Of 54 Keywords With Description +Code Examples
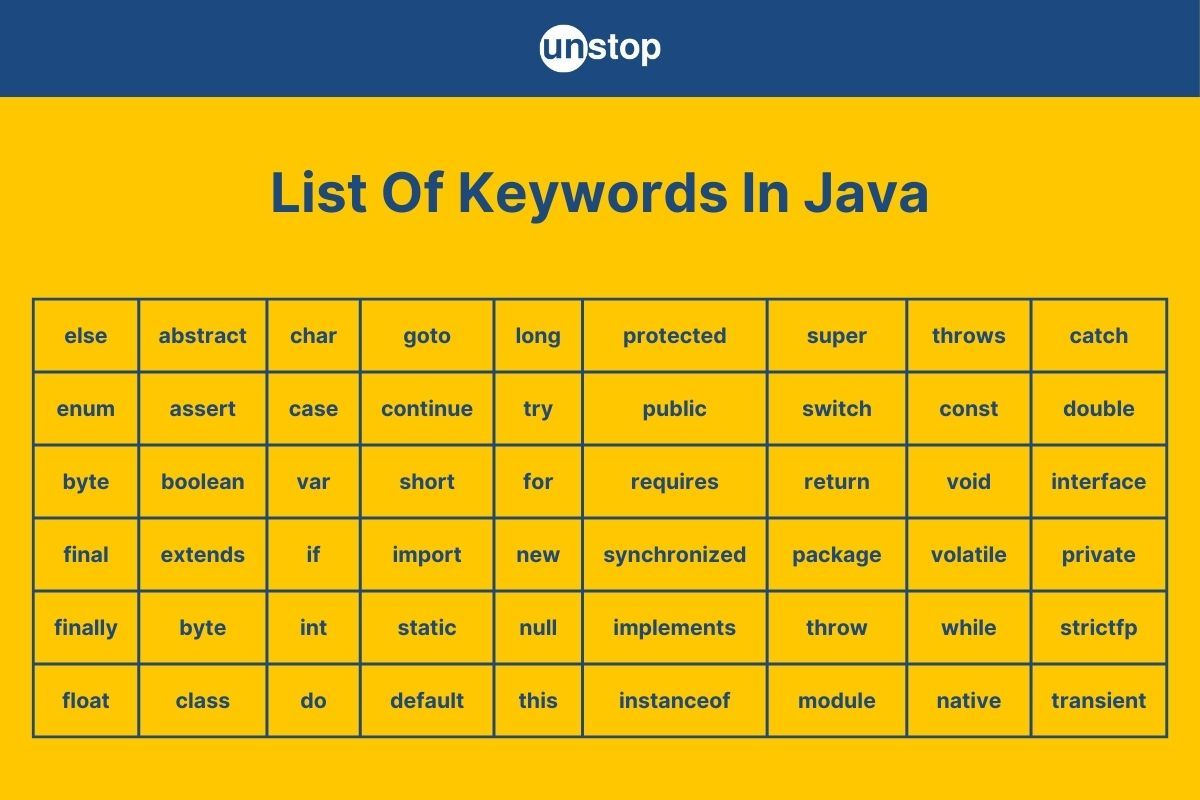
Keywords in Java are reserved words with predefined meanings, forming the backbone of the language’s syntax. They cannot be used as identifiers (e.g., variable names or class names), ensuring consistency in how the Java compiler interprets code. In this article, we will discuss the various Java keywords, along with their purpose, usage, and behavior, with examples.
What Are Keywords In Java?
Keywords in Java are special reserved words that serve specific purposes/ functionality in the language. They act as directives for the compiler, shaping how code is structured and executed. Because they are fundamental to Java’s syntax, keywords cannot be used as identifiers/ names for variables, methods, or classes.
Characteristics/ Properties Of Keywords In Java
Keywords in Java programming are the building blocks of the language, with these key characteristics and properties:
- Reserved Words: Keywords cannot be redefined or reused as identifiers (e.g., variable names, class names).
- Case Sensitivity: Keywords are lowercase only (if is valid, If is not). This distinguishes them from user-defined identifiers, which can have mixed cases.
- Predefined Functionality: Each keyword has a specific role, such as defining structures (class), controlling flow (if, for), or specifying data types (int, boolean).
- Integral to Syntax: Keywords are recognized by the Java compiler during compilation to ensure code integrity.
- Context-Specific Use: Some keywords have context-specific usage and restrictions. For instance, extends is specific to inheritance in class declarations. Certain keywords
- For example, extends can only be used in class declarations to specify inheritance.
- Unused Keywords: Reserved words like const and goto exist but are not actively used.
- No Unicode Variants: Keywords must be written in standard ASCII characters and no Unicode variants. For instance, class must be written exactly as that without any accents (like cláss).
Importance Of Keywords In Java
Keywords play a critical role in defining and managing:
- Control Flow: Keywords like if, else, for, while, and switch help in creating control flow structures that dictate the execution of code blocks.
- Object-Oriented Features: Keywords in Java enable class creation and inheritance, such as class, extends, and interface.
- Access Control: Java keywords such as public, private, and protected manage the accessibility of classes, methods, and variables, ensuring encapsulation and security in code.
- Error Handling: Keywords like try, catch, finally, and throw are used for exception handling, enabling robust and error-resistant code.
- Data Types: Keywords such as int, double, boolean, and char define the data types of variables, specifying the kind of data they can hold.
By leveraging these keywords effectively, developers can write robust, maintainable, and efficient code.
List Of Keywords In Java
As mentioned, Java keywords are foundational to the language's syntax. Wondering how many keywords are there in Java? The table below provides a complete list of keywords in Java language, along with their descriptions.
Keyword |
Description |
abstract |
Indicates that a class or method is abstract and cannot be instantiated directly or is incomplete. |
assert |
Used to perform debugging checks, asserting that a condition is true. |
boolean |
Declares a variable that can only hold values– true or false. |
break |
Used to terminate the execution of a loop or a switch statement. |
byte |
Declares an 8-bit integer variable. |
case |
Defines a block of code in a switch statement. |
catch |
Define how to handle exceptions thrown by a try block. |
char |
Declares a variable for holding a single 16-bit Unicode character. |
class |
Declares a class, which is a blueprint for creating objects. |
const |
Reserved keyword, but it is not used in Java. |
continue |
Used to skip the current loop iteration and move to the next iteration. |
default |
Specifies the default block of code in a switch statement or defines default methods in interfaces. |
do |
Starts a do-while loop, which executes the block at least once. |
double |
Declares a variable for holding a 64-bit double-precision floating point number. |
else |
Defines a block of code to execute if an if-condition is false. |
enum |
Declares an enumerated type, a set of named constants. |
extends |
Indicates that a class is extending a superclass (inheritance). |
final |
Used to define constants, prevent method overriding, or inheritance of a class. |
finally |
Defines a block of code that always executes after a try block, regardless of an exception being thrown. |
float |
Declares a variable for holding a 32-bit floating point number. |
for |
Starts a for loop for iterating over a range of values or a collection. |
goto |
Reserved but not used in Java. |
if |
Starts a conditional statement to execute code based on a condition. |
implements |
Indicates that a class implements an interface. |
import |
Allows classes or packages to be used from other Java files. |
instanceof |
Tests whether an object is an instance of a specific class or subclass. |
int |
Declares a variable for holding a 32-bit integer. |
interface |
Declares an interface– which contains method signatures. |
long |
Declares a variable for holding a 64-bit integer. |
module |
Declares a module for the modularization introduced in Java 9. |
native |
Specifies that a method is implemented in native code via the JNI (Java Native interpreter). |
new |
Creates new objects or arrays. |
null |
Represents the absence of a reference to any object. |
package |
Declares a package to group related classes and interfaces. |
private |
An access modifier indicating that a member is only accessible within its own class. |
protected |
An access modifier indicating that a member is accessible within its package and by subclasses. |
public |
An access modifier that grants access to a member from any other class. |
requires |
Specifies module dependencies (introduced in Java 9). |
return |
Exits from the current method, optionally returning a value. |
short |
Declares a variable for holding a 16-bit integer. |
static |
Declares a member that belongs to the class, rather than any instance. |
strictfp |
Used to ensure floating-point calculations are consistent across different platforms. |
super |
Refers to the superclass of the current object, often used to access superclass methods and constructors. |
switch |
Starts a switch statement for branching logic. |
synchronized |
Ensures thread-safe access to a block of code or object. |
this |
Refers to the current object instance. |
throw |
Explicitly throws an exception. |
throws |
Declares exceptions a method might throw. |
transient |
Marks a variable to be skipped during serialization. |
try |
Starts a block of code that is tested for exceptions. |
var |
Allows implicit type inference for variables (introduced in Java 10). |
void |
Specifies that a method does not return a value. |
volatile |
Indicates that a variable may be modified asynchronously by different threads. |
while |
Starts a while loop that continues as long as the condition is true. |
Detailed Overview Of Java Keywords With Examples
Java keywords are reserved words that form the foundation of the language. They carry predefined meanings and cannot be used for variable names or identifiers. In this section, we will discuss the usage of these keywords with examples.
abstract, class, extends, implements Keywords In Java
These keywords are vital in defining the backbone of object-oriented programming (OOP) in Java. They facilitate the creation of abstract classes, inheritance, and interfaces.
Key Functions:
- abstract: Marks a class or method as abstract, meaning it cannot be instantiated directly and requires subclasses to provide implementations for abstract methods.
- class: Declares a class, the basic building block of OOP in Java.
- extends: Establishes inheritance, allowing one class to acquire properties and methods from another.
- implements: Enables a class to adhere to an interface by providing concrete implementations of its methods.
Code Example:
Output:
Bark
Feeding...
Breathing...
Explanation:
In the Java program example,
- We use the interface keyword to define a blueprint for class structure, which must be implemented by other classes.
- Then, we used the class keyword to define all the classes: Mammal, Dog, and Main.
- In these, we used the abstract keyword to mark Mammal class abstract, indicating it cannot be instantiated directly.
- Also, we use the implements keyword in Mammal class definition to commit to the Animal interface. This means that the Mammal class will implement the blueprint provided by the Animal interface.
- We use the extends keyword in Dog class definition, indicating that it inherits from the Mammal class.
- In the main() method, we call all the methods (abstract, concrete and interface) using the dog object. Everything runs without a glitch, indicating that the keywords used get their job done.
boolean, if, else Keywords In Java
These keywords enable decision-making in Java programs.
Key Functions:
- boolean: This keyword is used to declare variables that can hold one of two values: true or false. It's fundamental for decision-making in Java, allowing programs to make choices based on conditions.
- if: It is used in if conditional statements, that executes a block of code if the specified condition is true.
- else: Used in combination with the if-keyword, to provide an alternate block of code to execute when the if condition evaluates to false.
Code Example:
Output:
Java is fun!
Explanation:
In the example,
- We use the boolean keyword to declare a boolean variable isJavaFun and initialize it to true (because it stores true or false values only).
- Then, we use the if and else keywords to define an if-else conditional statement.
- Here, the if condition checks the value of isJavaFun. If true, the associated block executes, printing "Java is fun!".
- If the condition were false, the else block would execute instead, printing "Java is not fun.".
- In the main() method, since isJavaFun is true, the program prints the message from the if block, demonstrating conditional decision-making in Java.
Want to expand your knowledge of Java programming? Check out this amazing course and become the Java developer of your dreams.
byte, short, int, long Keywords In Java
These keywords are used to declare integer variables, each offering different ranges of values and memory usage:
Key Functions:
- byte: Declares an 8-bit integer, with values from -128 to 127. Ideal for memory-efficient arrays.
- short: Declares a 16-bit integer, ranging from -32,768 to 32,767. Typically used when you need a variable that takes less space than int, but more than byte.
- int: Declares a 32-bit integer, ranging from values from -2^31 to 2^31-1, commonly used for whole numbers. The default choice for integer variables unless a specific range or memory size is required.
- long: Declares a 64-bit integer, for values beyond the range of int, i.e., from -2^63 to 2^63-1.
Code Example:
Output:
byte: 10
short: 1000
int: 100000
long: 100000000
Explanation:
- We declare four integer variables using the byte, short, int, and long keywords to represent values of different sizes and ranges.
- Then, we initialize these variables demonstrating their respective capacities:
- byte for small numbers (smallNumber = 10),
- short for slightly larger/ medium-sized values (mediumNumber = 1000),
- int for standard 32-bit integers (number = 100000), and
- long for very large numbers (largeNumber = 100000000L).
- In the main() method, we print the values of each variable using System.out.println.
- This demonstrates how these keywords allow memory-efficient storage based on the size of the data.
char, double, float Keywords In Java
These keywords declare variables for storing characters and floating-point numbers.
Key Functions:
- char: Stores a single 16-bit Unicode character, such as letters or symbols.
- double: Declares 64-bit double-precision floating-point numbers, suitable for precise calculations such as scientific computations
- float: Declares 32-bit single-precision floating-point numbers, ideal for memory-constrained contexts. While it uses less memory than double, it has less precision.
Code Example:
Output:
char: A
double: 9.99
float: 36.6
Explanation:
In the Java program example,
- We declare variables using the char, double, and float keywords, as follows:
- The char keywords defined a variable to hold a single character (letter = 'A'),
- The double-type variable stores a high-precision floating-point number (price = 9.99), and
- The variable defined using the float keyword stores a less precise, memory-efficient floating-point number (temperature = 36.6F).
- In the main() method, we print these values, illustrating how char represents single characters and double and float handle numeric data of varying precision.
for, while, do Keywords In Java
These keywords create loops that repeatedly execute a block of code.
- for: This keyword initiates the for loop that executes a block of code a specified number of times, typically used when the number of iterations is known.
- while: The keyword defined a while loop that runs a block of code as long as a specified condition is true, useful when the number of iterations are not known in advance.
- do: Is used to define a do-while loop. Similar to while, this loop ensures the block executes at least once since the condition is checked after execution.
Code Example:
Output:
For loop iteration: 0
For loop iteration: 1
For loop iteration: 2
While loop iteration: 0
While loop iteration: 1
While loop iteration: 2
Do-while loop iteration: 0
Do-while loop iteration: 1
Do-while loop iteration: 2
Explanation:
In the main() method of the Java code example,
- We use the for keyword to define a for loop that initializes i to 0 and increments it until it is less than 3, printing each iteration.
- Then, we initialize a variable j with value 0, to be used as the counter variable in the while loop (where we use the while keyword).
- The loop begins iteration with j at 0, and increments it until it is less than 3, printing each iteration.
- Next, we initialize another variable k with the value 0, to be used as the counter variable.
- Then, we use the do and while keywords to define a do-while loop that begins with k at 0, increments it until it is less than 3, printing each iteration, but ensures the block executes at least once regardless of the condition.
assert Keyword In Java
The assert keyword is used for debugging purposes. It enables you to test assumptions in your code. If the assumption is false, the program will throw an AssertionError.
Code Example:
Output:
Assertion passed!
Explanation:
- In the main() method, we first declare and initialize an integer variable x with the value 1.
- Then, we use the assert keyword to define an assertion/ assert statement that checks if x is greater than 0.
- Since the condition is true, the assertion passes, printing the string message– "Assertion passed!”.
- If the condition were false, an AssertionError with the message "x should be greater than 0" would be thrown.
break, switch case, default Keywords In Java
These keywords are commonly used to control program flow in structures like switch statements and loops.
- break: This keyword is used to exit a loop or switch block prematurely. For example, in loops, it leads to immediate termination, and in switch-case, it helps exit the matched case block after execution, thus preventing fall-through to subsequent cases.
- switch: This keyword initiates a switch statement to define how to evaluate a variable and branches to matching case.
- case: It is used to define the block of code for a specific value in a switch.
- default: This keyword defines a block of code within a switch statement to be executed if none of the specified case values match the switch expression.
Code Example:
Output:
Wednesday
Explanation:
- In this example, we first initialize the variable day with the value 3.
- Then, we use the switch and case keywords to define the switch-case statement.
- Here, the statement checks the value of day against multiple cases. So, when the variable value matches a case, the respective code block is executed.
- Since here, the value of day matches case 3, the program prints – "Wednesday".
- Once that is done, the break statement (defined using the break keyword) ensures that the flow exits the switch block.
- We also use the default keyword to define a fallback block of code to be executed if none of the cases match the day variable.
continue Keyword In Java
The continue keyword is used to define what is known as the continue statement. It is often used in iterative/ control statements like loops to skip the current iteration and move to the next one.
Code Example:
Output:
0
1
2
4
Explanation:
In the example Java program,
- We define a for loop that runs for the range 0 to 5. In every iteration, the if-statement first checks if the value of i is equal to 3.
- If the condition is not met, the loop continues with printing the value of the counter variable and increments it by 1.
- If the condition is true, the if-block is executed. It contains the continue statement (defined using the continue keyword) which makes the flow jump to the beginning of the next iteration.
- As shown in the output, the number 3 is not printed.
enum Keyword In Java
The enum keyword is used to declare/ define a fixed set of constants. Look at the Java example code below to see how this works.
Code Example:
Output:
Today is: WEDNESDAY
Explanation:
In the example above,
- We use the enum keyword to define the Day enum to contain constants for the days of the week.
- Then, we create today variable, set it to Day.WEDNESDAY and print it to the console.
try, catch, finally, final Keywords In Java
These keywords serve various purposes in Java, primarily revolving around exception handling and immutability.
- try-catch-finally: The try block contains code that might throw exceptions. The catch block handles those exceptions if they occur. The finally block executes regardless of exceptions, ensuring cleanup or additional actions.
- final keyword: Used to declare constants or prevent inheritance and method overriding.
Code Example:
Output:
Max value: 100
Cannot divide by zero!
This block always executes.
Explanation:
- We define a constant MAX_VALUE using the final keyword, which ensures that its value cannot be modified.
- Also, we assign the value 100 to the constant and print it to the console.
- Next, we use the try, catch, and finally keywords to create a structure where:
- The try block contains code that can throw an error, which here is– dividing by 0.
- The catch block catches that specific type of error and defines how to handle it, which is done here by printing the message– “Cannot divide by zero!”.
- Then, we have the finally block, which runs regardless of exceptions, guaranteeing the message "This block always executes." is printed.
Here is your chance to top the leaderboard while practising your coding skills: Participate in the 100-Day Coding Sprint at Unstop.
throw, throws Keywords In Java
These keywords define how exceptions are explicitly thrown and declared in Java programs.
- throw: Used to explicitly throw an exception from a block of code or method. It allows for the creation of a new exception object and its passing to the runtime system.
- throws: Declares in a method signature the exceptions that the method might throw, ensuring the caller handles or declares them further.
Code Example:
Output:
Age must be 18 or older.
Explanation:
- We define a try-catch block where the try block reviews the checkAge() method, and any exception thrown is handled by the catch block.
- Then, we define the checkAge() method. In the signature, we use the throws keyword to declare that it might throw an Exception.
- Also, we use the throw keyword to create and throw a new exception if the age parameter is less than 18.
- In the main() method, the try-catch block calls the checkAge method.
- When the exception is thrown, it is caught by the catch block, and the exception's message is printed.
goto Keyword In Java
The goto keyword is reserved but not used in Java. It serves no practical application and is included only for future use or compatibility with other programming languages.
import, instanceof Keywords In Java
These keywords streamline class dependencies and type-checking in Java.
- import: Simplifies code by allowing developers to include other Java packages or classes into the current file, making their functionalities accessible without needing to specify their full package paths each time.
- instanceof: Checks whether an object is an instance of a specified class or subclass, ensuring type safety. It is a type-checking operator in Java that returns true if the object is an instance of the specified type, helping to ensure type safety and avoid ClassCastException.
Code Example:
Output:
List: [Java]
list is an instance of ArrayList
Explanation:
- We use the import keyword/ statement to include the ArrayList class from the java.util package, making it accessible without needing the full package path.
- Then, we create an ArrayList object list and populate it with a string "Java".
- Next, we use the instanceof keyword inside an if-statement which checks if list is an instance of ArrayList. If true, it prints "list is an instance of ArrayList" as shown in the output.
interface Keyword In Java
The interface keyword is used to declare an interface, a blueprint for classes that defines abstract methods and constants. Interfaces promote abstraction by specifying what a class should do, leaving the "how" to implement classes.
Code Example:
Output:
Toyota starts!
Explanation:
- We use the interface keyword to define the Car interface containing a start() method (which is void and has no body).
- Then, we define the Toyota class, which implements the Car interface and provides a concrete implementation of the start() method.
- In the main() method, we create an object of Toyota type and assign it to a Car reference (myCar), demonstrating polymorphism.
- We then use the myCar object to call the start() method. This example demonstrates that the interface defined is implemented by classes.
native Keyword In Java
The native keyword marks a method as implemented in native (non-Java) code through the Java Native Interface (JNI). It bridges Java with libraries written in C, C++, or other languages.
Code Example:
Output:
Hello from the simulated native method!
Explanation:
- Normally, a native method would execute non-Java code. Since this example is simplified, it mimics a native method using a standard Java method. In real-world scenarios, native methods are linked via System.loadLibrary.
- Since online compilers don't support JNI, we replaced the native method with a regular Java method that prints "Hello from simulated native method!".
new, null Keywords In Java
These keywords are used for object creation and reference management.
- new: This keyword is used to create new objects in Java as it allocates memory for the object and initializes it using the corresponding class constructor. It is essential for instantiating classes and accessing their properties and methods.
- null: This keyword represents an empty reference or the absence of an object. It is often used to check whether a reference variable points to a valid object or not. It can also be assigned explicitly to deallocate an object reference.
Code Example:
Output:
Dog's name: Buddy
myDog is null
Explanation:
- We use the new keyword to create a new Car object and initialize its brand property.
- Also, we use the null keyword to remove the reference to the object, making myCar point to nothing.
package keyword in Java
The package keyword is used to organize classes and interfaces into namespaces, making the code more manageable and reusable.
Code Example:
Output:
Hello from MyClass!
Explanation:
In this example, we use the package keyword to declare the mypackage namespace, grouping related classes together. The MyClass class is part of the mypackage package and contains a method display that prints a message.
private, protected, public Keywords In Java
These access modifiers that control how fields, methods, and constructors can be accessed across classes and packages.
- private: Limits access to the same class. Ensures encapsulation and data protection.
- protected: Permits access within the same package and subclasses, enabling inheritance while maintaining some restriction.
- public: Allows access from any class or package, facilitating full visibility.
Code Example:
Output:
Name: Shivani
Explanation:
- The name field is declared using the private keyword, making it accessible only within the Person class.
- The getName() method is declared with the public keyword, making it accessible from outside the Person class.
- It provides controlled access to the name field, demonstrating how encapsulation works—data is hidden, and external access is allowed only through predefined methods.
- In the main() method, we create an instance of Person and use it to call getName() method, printing the name.
return Keyword In Java
The return keyword is used to define the return statement, which marks the exit from the current method and optionally returns a value.
Code Example:
Output:
Sum: 8
Explanation:
- In the example, we define an add() function, which takes integers a and b as arguments.
- The return keyword in the add() method helps exit the method and provides the result of a + b back to the caller.
- This returned value is stored in sum and printed as "Sum: 8."
static, strictfp keywords in Java
The static keyword indicates that a class member (variable or method) belongs to the class itself rather than any specific object instance. This allows access to the member without needing to create an instance of the class.
The strictfp keyword enforces strict floating-point calculations across all platforms, ensuring consistent results regardless of the underlying hardware or software.
Code Example:
Output:
Static method called!
Explanation:
- We define a class MyClass containing a display() method to print a message.
- The display() method is declared as static using the keyword, so it can be called directly using the class name MyClass.
- It also contains a calculate() method, which we define using the strictfp keyword to ensure consistent floating-point behavior.
- In the main() method, we call display() directly using the class name, printing "Static method called!".
super Keyword In Java
The super keyword provides a way to refer directly to the superclass of the current object. It is commonly used to access superclass methods, constructors, or variables, often in cases of method overriding or constructor chaining.
Code Example:
Output:
Animal display method
Dog display method
Explanation:
- In this example, we have an Animal class with a display() method. The Dog class extends the Animal class and overrides the display() method.
- Within the Dog class, we use the super keyword to call the display() method from the superclass (Animal). This ensures that both the Animal class’s display() method and the Dog class’s overridden display() method are executed.
- In the main() method, we create an instance of the Dog class and invoke the display() method. As a result, both the superclass (Animal) and subclass (Dog) methods are executed.
synchronized Keyword In Java
The synchronized keyword is a concurrency control mechanism that prevents multiple threads from accessing a critical section of code simultaneously. This ensures thread safety and prevents issues like race conditions or data inconsistency.
Code Example:
Output:
Thread-0: 1
Thread-0: 2
Thread-0: 3
Thread-1: 4
Thread-1: 5
Thread-1: 6
Explanation:
- In this example, we create a Counter class implementing the Runnable interface. It contains two threads (t1 and t2) to run the same instance of the Counter class.
- The run() method in the class, contains the synchronized keyword.
- As a result, when the run() method is executed, the synchronized (this) block ensures that only one thread can execute the code inside the block at a time, even though both threads (t1 and t2) are trying to increment the count variable simultaneously.
this keyword in Java
The this keyword is a reference to the current object. It is used to distinguish between instance variables and parameters with the same name, as well as to invoke other constructors or methods of the same object.
Code Example:
Output:
Name: Sahil
Age: 25
Explanation:
- The this keyword is used in the constructor to distinguish instance variables from parameters.
- It is also used in the display() method to access and print instance variables.
transient keyword in Java
The transient keyword marks a variable to be excluded from the serialization process. When an object is serialized, transient fields are not included in the byte stream, making them ideal for sensitive or temporary data.
Code Example:
Output:
Username: Adit
Password: null
Explanation:
- The password field is declared as transient, preventing it from being serialized.
- The username field is serialized and deserialized correctly.
- The password field is not serialized, resulting in null when deserialized.
void Keyword In Java
The void keyword specifies that a method does not return any value. It is used when a method performs an action, such as printing or modifying data, without producing a result that needs to be returned.
Code Example:
Output:
Hello, World!
Explanation:
- In the example, we have defined the greet() method with the void keyword, indicating it does not return a value.
- It performs an action (prints the string message– Hello, World!) but does not return any value.
volatile Keyword In Java
The volatile keyword ensures that changes to a variable are immediately visible to all threads. It is used for variables that can be modified by multiple threads, guaranteeing the latest value is read directly from memory.
Code Example:
Output:
Thread-1: false
Thread-1: true
Thread-0: true
Thread-0: false
Explanation:
- The flag variable in the SharedData class is declared as volatile.
- The volatile keyword ensures the flag variable's updates are visible across threads, providing consistency.
Need more guidance on how to become a Java developer? You can now select an expert to be your mentor here.
What If When Keywords In Java Are Used As Variable Names?
In Java, keywords are reserved words with predefined meanings in the language's syntax. They cannot be used as identifiers, such as variable names, class names, or method names. Attempting to do so results in compilation errors, as the compiler recognizes these keywords as special terms defining Java's structure and behavior.
Explanation Of Various Scenarios With Examples
Let's look at scenarios where a keyword is mistakenly used as a variable name and how to correct these mistakes:
Example 1: Using Keyword Reserved for Data Type (int) as a Variable Name
The int keyword represents the integer data type and when used as the name/ identifier for a variable, it will lead to an error.
Incorrect Code:
Output:
error: not a statement
int int = 10;
Correct Code:
Output:
10
Explanation: In the incorrect code, int is used both as a keyword and as a variable name, causing a compilation error. The correct code replaces int with myInt, avoiding the conflict and compiling successfully.
Example 2: Using class Keyword as a Variable Name
The class keyword is reserved for declaring/ defining classes in Java programs. Using it as a variable name results in a similar error. Below is a concise explanation with a snippet.
Incorrect Code:
String class = "Hello"; // Error: 'class' is a keyword
Correct Code:
String myClass = "Hello"; // Using a non-keyword variable name
System.out.println(myClass);
Explanation:
In the incorrect code, the class keyword is used as the variable name, causing a compilation error. The correct code uses myClass instead, ensuring the code compiles and runs as expected.
Example 3: Using for Keywrod as a Variable Name
The for keyword is reserved to define for loop, an iterative control statement that executes a block of code repeatedly. Using it as a variable name will lead to a compilation error.
Incorrect Code:
int for = 5; // Error: 'for' is a keyword
Correct Code:
int loopCounter = 5; // Using a non-keyword variable name
System.out.println(loopCounter);
Explanation:
In the incorrect code, for keyword is used as the variable name, leading to a compilation error. In the correct code, changing the variable name to loopCounter avoids the conflict, allowing the program to compile and execute properly.
Using Java keywords as variable names leads to compilation errors because of their reserved nature. Always use alternative names that do not conflict with keywords. This practice not only ensures successful compilation but also enhances code readability and maintainability.
Difference Between Identifiers & Keywords In Java
In Java programming, keywords are reserved words with predefined meanings in the language, while identifiers are user-defined names for elements such as variables, methods, classes, and objects. Understanding their differences is essential for avoiding syntax errors and writing clear, structured code.
Feature |
Keywords |
Identifiers |
Definition |
Reserved words predefined by the language |
Names defined by the programmer |
Usage |
Define the structure and behavior of the program |
Identify variables, methods, classes, etc. |
Examples |
if, for, class, int, boolean |
myVariable, calculateSum, Student |
Restrictions |
Cannot be used as identifiers |
Must start with a letter/underscore, can contain letters, digits, and underscores |
Case Sensitivity |
Case-sensitive (e.g., class ≠ Class) |
Case-sensitive (e.g., myVar ≠ MyVar) |
Count |
Fixed: Java has 51 keywords |
Unlimited: Defined by the programmer |
Special Characters |
Cannot include special characters |
Cannot include special characters (except underscores) |
Example Of Identifiers In Java
Identifiers are used to name variables, methods, classes, and objects. Below is an example illustrating the concept for better understanding.
Code Example:
Output:
Number: 10
Name: Shivani
Explanation:
In this example,
- We declare two variables, where myNumber and myName are identifiers representing the variable names.
- Identifiers allow you to reference these variables like we do when printing their values.
- They are chosen by the programmer and must follow Java's naming rules (e.g., starting with a letter or underscore and avoiding keywords).
While keywords serve as the building blocks of Java's syntax with predefined meanings, identifiers allow programmers to name and reference program elements flexibly. Understanding this distinction ensures error-free and well-structured code.
Conclusion
Java keywords are the backbone of the language, shaping its structure and defining its behavior. Each keyword in Java serves a specific purpose, whether it’s controlling program flow (if, while), managing inheritance (extends, implements), or defining data types (int, boolean). By understanding how these reserved words work, you can unlock the full potential of Java’s syntax.
These keywords act as the universal language Java understands, ensuring consistency and clarity in your programs. Misusing them, like trying to use a keyword as a variable name, leads to errors, emphasizing their importance in creating error-free code. Mastering the use of keywords in Java is essential for writing clear, maintainable, and efficient programs.
Frequently Asked Questions
Q. What do you mean by keyword?
In programming, a keyword is a reserved word with a predefined meaning and specific functionality within the language. Keywords are integral to the language's syntax, defining control structures, data types, and operations. Since they are part of the language's core vocabulary, they cannot be used as identifiers like variable or method names. For instance, in Java, keywords such as if, else, and while control program flow, while int and boolean define data types.
Q. Is void a keyword in Java?
Yes, void is a keyword in Java. It indicates that a method does not return any value.
Code Example:
Here, the main method uses void because it performs an action (printing to the console) without returning any value.
Q. What is this keyword with an example in Java?
The this keyword refers to the current object and is used to access instance variables and methods within a class.
Code Example:
Output:
Name: Shivani
Explanation: In the constructor, this.name refers to the instance variable name, differentiating it from the parameter name. The this keyword ensures the correct variable is accessed.
Q. Can keywords be used as variable names in Java?
No, keywords cannot be used as variable names. This is because keywords are words reserved by a programming language, which have special meanings and are utilized to carry out particular functions in the code. Using them as variable names would cause a syntax error because the compiler would not be able to distinguish between the intended use as a keyword or as an identifier.
Code Example:
Q. How are keywords different from identifiers in Java?
- Keywords: Reserved words with predefined meanings used for specific purposes in Java. For example, public, class, if.
- Identifiers: User-defined names for variables, methods, classes, and objects. For example, myVariable, calculateSum.
Keywords cannot be used as identifiers. This means identifiers must follow naming conventions, and one should not use keywords in Java for this purpose.
Do check out the following:
- Dynamic Method Dispatch In Java (Runtime Polymorphism) + Examples
- Top 15+ Difference Between C++ And Java Explained! (+Similarities)
- Method Overloading In Java | Ways, Rules & Type Promotion (+Codes)
- 20+ Differences Between C And Java (Everything You Need To Know)
- Advantages And Disadvantages of Java Programming Language
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment