Java Programming Language
Table of content:
- History Of Java Programming Langauge
- Infographic For History Of Java
- What’s In The Name | History Of Java
- Key Features Of Java
- Advantages And Disadvantages Of Java
- The Version History Of Java Langauge
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is JDK?
- How To Download Java Development Kit (JDK) For Windows, MacOS, and Linux?
- Set Environment Variables In Java
- How To Install Java (JDK) On Windows 64-Bit Machine?
- How To Install Java (JDK) On Linux?
- How To Install Java (JDK) On macOS?
- How To Test Java Installation?
- How To Write Your First Java Program On Linux OS?
- Conclusion
- Frequently Asked Questions
Table of content:
- Java Programming Language | An Introduction
- 15 Key Features Of Java
- Write Once Run Anywhere (WORA) | Features Of Java
- Java Editions
- 5 New Features Of JAVA 8
- 5 New Features Of JAVA 11
- What Makes Java Popular?
- Conclusion
- Frequently Asked Questions
Table of content:
- What is Java?
- Advantages of Java
- Disadvantages of Java
Table of content:
- What Is Java Programming?
- Role Of Integrated Development Environments (IDEs) In Java Development
- 15 Best Java IDE For Developers
- In-Depth Comparison Table
- Conclusion
- Frequently Asked Questions
Table of content:
- Key Differences Between Java And Core Java
- What Is Java?
- What Is Core Java?
- Applications Of Java
- Applications Of Core Java
- When To Use Java?
- When To Use Core Java?
- Conclusion
- Frequently Asked Questions
Table of content:
- What Are Variables In Java Language?
- How To Declare Variables In Java Programs?
- How To Initialize Variables In Java?
- Naming Conventions For Variables In Java
- Types Of Variables In Java
- Local Variables In Java
- Instance Variables In Java
- Static Variables In Java
- Final Variables In Java
- Scope and Lifetime of Variables In Java
- Data Types Of Variables In Java (Primitive & Non-primitive)
- Java Variable Type Conversion & Type Casting
- Working With Variables In Java (Examples)
- Access Modifiers & Variables In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Are Identifiers In Java?
- Syntax Rules For Identifiers In Java
- Valid Identifiers in Java
- Invalid Identifiers in Java
- Java Reserved Keywords
- Naming Conventions & Best Practices For Identifiers In Java
- What Is An Identifier Expected Error In Java?
- Reasons The Identifier Expected Error Occurs
- How To Fix/ Resolve Identifier Expected Errors In Java?
- Conclusion
- Frequently Asked Questions
Table of content:
- What Are Data Types In Java?
- Primitive Data Types In Java
- Non-Primitive Data Types In Java
- Key Differences Between Primitive And Non-Primitive Data Types In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Are Operators In Java?
- Types Of Operators In Java
- Unary Operators In Java
- Arithmetic Operators In Java
- Assignment Operators In Java
- Relational Operators In Java
- Logical Operators In Java
- Bitwise Operators In Java
- Shift Operators In Java
- Increment & Decrement Operators In Java
- Ternary Operator In Java
- Instanceof Operator In Java
- Precedence & Associativity Of Java Operators
- Advantages & Disadvantages Of Operators In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is A Return Statement In Java?
- Use Cases Of Return Statements In Java
- Returning A Value From A Method In Java
- Returning A Class Object In Java
- Returning Void (No Value) In Java
- Advantages Of Using Return Statements In Java
- Limitations Of Using Return Statements In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Are Keywords In Java?
- List Of Keywords In Java
- Detailed Overview Of Java Keywords With Examples
- What If When Keywords In Java Are Used As Variable Names?
- Difference Between Identifiers & Keywords In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Abstract Keyword In Java?
- Use Of Abstract Keyword In Java
- Abstract Methods In Java
- Abstract Classes In Java
- Advantages Of Abstract Keyword In Java
- Disadvantages Of Abstract Keyword In Java
- Abstract Classes Vs. Interfaces In Java
- Real-World Applications Of Abstract Keyword
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is throws Keyword In Java?
- How Does The throws Keyword Work?
- Throwing A Checked Exception Using throws In Java
- Throwing Multiple Exceptions Using throws In Java
- Throwing A Custom Exception Using throws In Java
- When To Use The throws Keyword In Java
- Difference Between throw and throws Keyword In Java
- Best Practices For Using The throws Keyword In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is The Volatile Keyword In Java?
- How Does Volatile Keyword In Java Work?
- Using Volatile Keyword In Java To Control Thread Execution
- Using Volatile Keyword In Java To Signal Between Multiple Threads
- Difference Between Synchronization And Volatile Keyword
- Common Mistakes And Best Practices While Using Volatile Keyword In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding Super Keyword In Java
- Super Keyword In Java With Instance Variables
- Super Keyword In Java With Method Overriding
- Super Keyword In Java With Constructor Chaining
- Applications Of Super Keyword In Java
- Difference Between This And Super Keyword In Java
- Advantages Of Using Super Keyword In Java
- Limitations And Considerations Of Super Keyword In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding This Keyword In Java
- Uses Of This Keyword In Java
- Using This Keyword For Referencing Instance Variables
- Using This Keyword For Invoking A Constructor
- Using This Keyword For Invoking A Method
- Using This Keyword With Getters And Setters
- Difference Between This And Super Keyword In Java
- Best Practices For Using This Keyword In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is new Keyword In Java?
- Uses Of The new Keyword In Java
- Memory Management With new Keyword In Java
- Example 1: Creating An Object Of A Class Using new Keyword In Java
- Example 2: Creating An Array Using The new Keyword In Java
- Best Practices For Using new Keyword In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is The Transient Keyword In Java?
- Real-Life Example Of The Transient Keyword In Java
- When To Use The Transient Keyword In Java
- Example 1: Effect Of Transient Keyword On Serialization In Java
- Example 2: Skipping Sensitive Data During Serialization With Transient Keyword In Java
- Using Transient With Final Keyword In Java
- Using Transient With Static Keyword
- Difference Between Transient And Volatile Keyword In Java
- Advantages And Disadvantages Of Transient Keyword In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Static Keyword In Java?
- Characteristics Of Static Keyword In Java
- Static Variables In Java
- Static Method In Java
- Static Blocks In Java
- Static Classes In Java
- Static Variables Vs Instance Variables In Java
- Advantages Of Static Keyword In Java
- Disadvantages Of Static Keyword In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is A Static Method In Java?
- Use Cases Of Static Method In Java
- Using Static Method In Java To Create A Utility Class
- Using Static Method In Java To Implement The Singleton Design Pattern
- Difference Between Static And Instance Methods In Java
- Limitations Of Static Method In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding Final Keyword In Java
- Final Variables In Java
- Final Methods In Java
- Final Classes In Java
- Difference Between Static And Final Keyword In Java
- Uses Of Final Keyword In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Key Difference Between final, finally, And finalize In Java
- What Is final Keyword In Java?
- What Is finally Keyword In Java?
- What Is finalize Keyword In Java?
- When To Use Which Keyword In Java?
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding The extends Keyword In Java?
- Use Of extends Keyword In Java
- Using Java extends To Implement Single Inheritance
- Using Java extends With Interfaces (Default Methods)
- Overriding Using extends Keyword In Java
- Difference Between extends And implements In Java
- Real World Applications Of Extends Keyword In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Decision Making Statement In Java?
- If Statement In Java
- If-Else Statement In Java
- Else-If Ladder In Java
- Switch Statement In Java
- Ternary/Conditional Operator (?:) In Java
- Best Practices For Writing Decision Making Statements In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Are Control Statements in Java?
- Decision-Making Control Statements In Java
- Looping Control Statements In Java
- Jump (Branching) Control Statements In Java
- Application Of Control Statements In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is The Break Statement In Java?
- Working Of The Break Statement In Java
- Using Java Break Statement With Loops
- Using Java Break Statement With Switch Statement
- Using Java Break Statement With Infinite Loops
- Common Pitfalls While Using Break Statements In Java
- Best Practices For Using The Break Statement In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Switch Statement In Java?
- Working Of The Switch Statement In Java
- Example Of Switch Statement In Java
- Java Switch Statement With String
- Java Nested Switch Statements
- Java Enum In Switch Statement
- Java Wrapper Classes In Switch Statements
- Uses Of Switch Statement In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Syntax Of main() Method In Java
- public Specifier – Main Method In Java
- static Keyword – Main Method In Java
- void Return Type Of Main Method In Java
- The main Identifier – Main Method In Java
- String[] args In Main Method In Java
- The Role Of Java Virtual Machine (JVM)
- Running Java Programs Without The Main Method
- Variations In Declaration Of Main Method In Java
- Overloading The Main Method In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Method Overriding In Java?
- Example Of Method Overriding In Java
- Ideal Use Cases Of Method Overriding In Java
- Rules For Method Overriding In Java
- Super Keyword & Method Overriding In Java
- Constructor & Method Overriding In Java
- Exception Handling In Method Overriding In Java
- Access Modifiers In Method Overriding In Java
- Advantages & Disadvantages Of Method Overriding In Java
- Difference Between Method Overloading Vs. Method Overriding In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Method Overloading In Java?
- Different Ways Of Method Overloading In Java
- Overloading The main() Method In Java
- Type Promotion & Method Overloading In Java
- Null Error & Method Overloading In Java
- Advantages Of Method Overloading In Java
- Disadvantages Of Method Overloading In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Difference Between Overloading And Overriding In Java (Comparison Table)
- What Is Method Overloading In Java?
- What Is Method Overriding In Java?
- Key Differences Between Overloading & Overriding In Java Explained
- Difference Between Overloading And Overriding In Java Code Example
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is A One-Dimensional Array In Java?
- Key Characteristics Of One-Dimensional Arrays In Java
- Declaration Of One-Dimensional Array In Java
- Initialization Of One-Dimensional Array In Java
- Common Operations On One-Dimensional Array In Java
- Advantages Of One-Dimensional Arrays In Java
- Disadvantages Of One-Dimensional Arrays In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is A Multidimensional Array In Java?
- Difference Between Single-Dimensional And Multidimensional Arrays In Java
- Declaring Multidimensional Arrays In Java
- Initializing Multidimensional Arrays In Java
- Accessing And Manipulating Elements In Multidimensional Arrays In Java
- Working Of Multidimensional Arrays With Jagged Arrays In Java
- Why Use Multidimensional Arrays In Java?
- Limitations Of Multidimensional Arrays In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Are Jagged Arrays In Java?
- Comparison With Regular Multi-Dimensional Arrays
- Declaring Jagged Arrays In Java
- Initialization Of Jagged Arrays In Java
- Printing Elements Of A Jagged Array In Java
- Accessing And Modifying Elements Of A Jagged Array In Java
- Advantages Of Jagged Arrays In Java
- Disadvantages Of Jagged Arrays In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Array Of Objects In Java?
- Declare And Initialize An Array Of Object In Java
- Example Of An Array Of Objects In Java
- Sorting An Array Of Objects In Java
- Passing Arrays Of Objects To Methods In Java
- Returning Arrays Of Objects From Methods In Java
- Advantages Of Arrays Of Objects In Java
- Disadvantages Of Arrays Of Objects In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is A Dynamic Array In Java?
- Why Use Dynamic Array In Java?
- What Is The Size And Capacity Of A Dynamic Array In Java?
- How To Create A Dynamic Array In Java?
- Managing Dynamic Data Input In Java
- Storing And Processing Real-Time Data In Java
- Use Cases Of Dynamic Arrays In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Why Return An Array In Java?
- How To Return An Array In Java
- Example 1: Returning An Array Of First N Squares
- Example 2: Doubling the Values of an Array
- Common Scenarios For Returning Arrays In Java
- Points To Remember When Returning Arrays In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding ArrayList In Java
- Differences Between Arrays And ArrayList In Java
- Returning An ArrayList In Java
- Common Use Cases For Returning An ArrayList In Java
- Pitfalls To Avoid When Returning An ArrayList In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is A Thread In Java?
- Thread Vs Process
- What is a Thread Life Cycle In Java?
- What Are Thread Priorities?
- Creating Threads In Java
- Java Thread Methods
- Commonly Used Constructors In Thread Class
- Thread Synchronization In Java
- Common Challenges Faced While Using Threads In Java
- Best Practices For Using Threads In Java
- Real-World Applications Of Threads In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding Multithreading In Java
- Methods Of Multithreading In Java (Examples)
- Difference Between Multithreading And Multitasking In Java
- Handling Exceptions In Multithreading
- Best Practices For Multithreading In Java
- Real-World Use Cases of Multithreading In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Thread Priority In Java?
- Built-In Thread Priority Constants In Java
- Thread Priority: Setter & Getter Methods
- Limitations Of Thread Priority In Java
- Best Practices For Using Thread Priority In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Thread Synchronization In Java?
- The Need For Thread Synchronization In Java
- Types Of Thread Synchronization In Java
- Mutual Exclusion In Thread Synchronization In Java
- Coordination Synchronization (Thread Communication) In Java
- Advantages Of Thread Synchronization In Java
- Disadvantages Of Thread Synchronization In Java
- Alternatives To Synchronization In Java
- Deadlock And Thread Synchronization In Java
- Real-World Use Cases Of Thread Synchronization In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is A Daemon Thread In Java?
- User Threads Vs. Daemon Threads In Java
- Methods For Daemon Threads In The Thread Class
- Creating Daemon Threads In Java
- Checking The Daemon Status Of A Thread
- Exceptions In Daemon Threads
- Limitations Of Daemon Threads In Java
- Practical Applications Of Daemon Threads In Java
- Common Mistakes To Avoid When Working With Daemon Threads In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Why Do Threads Need To Communicate?
- Understanding Inter Thread Communication In Java
- The wait() Method In Inter-Thread Communication
- The notify() Method In Inter-Thread Communication
- The notifyAll() Method In Inter-Thread Communication
- Difference Between wait() And sleep() Methods In Java
- Best Practices For Inter Thread Communication In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding The Factorial Concept
- Approaches To Implementing Factorial In Java
- Find Factorial In Java Using Iterative Approach (Using a Loop)
- Find Factorial In Java Using Recursive Approach
- Complexity Analysis Of Factorial Programs In Java
- Applications Of Factorial Program In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding The Leap Year Concept
- Approach To Check A Leap Year In Java
- Alternative Approach To Check A Leap Year In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is The Difference Between JDK, JRE, and JVM?
- What Is JVM (Java Virtual Machine)?
- What Is JRE (Java Runtime Environment)?
- What Is JDK (Java Development Kit)?
- Understanding The Difference Between JDK, JRE, And JVM
- Comparison Table For Difference Between JDK, JRE, And JVM
- Conclusion
- Frequently Asked Questions
Table of content:
- Difference Between Abstraction And Encapsulation In Java
- Understanding Abstraction In Java
- Understanding Encapsulation In Java
- When To Use Abstraction And Encapsulation?
- Conclusion
- Frequently Asked Questions
Table of content:
- Differences Between Abstract Class And Interface In Java
- What Is An Abstract Class In Java?
- What Is An Interface In Java?
- When To Use An Abstract Class?
- When To Use Interface?
- Compatibility Between Abstract Class And Interface In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Error Vs. Exception In Java
- What Is Error In Java?
- What Is Exception In Java?
- Best Practices For Handling Exceptions In Java
- Why Errors Should Not Be Handled In Java?
- Conclusion
- Frequently Asked Questions
Table of content:
- Key Differences: Java Vs. JavaScript
- What Is Java?
- What Is JavaScript?
- Difference Between Java And JavaScript Explained
- Conclusion
- Frequently Asked Questions
Table of content:
- Brief Introduction To C++
- Brief Introduction To Java
- Difference Between C++ and Java
- Overview & Features Of C++ Language
- Overview & Features of Java Language
- Example of C++ and Java Program
- Key Difference Between C++ And Java Explained
- Similarities Between Java Vs. C++
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- Basic Java interview questions and answers
- Intermediate Java interview questions and answers
- Advanced Java interview questions and answers
Table of content:
- Difference between core Java and advanced Java
- Important Core Java Questions
- Tips for Preparing for Core Java
Volatile Keyword In Java | Syntax, Working, Uses & More (+Examples)
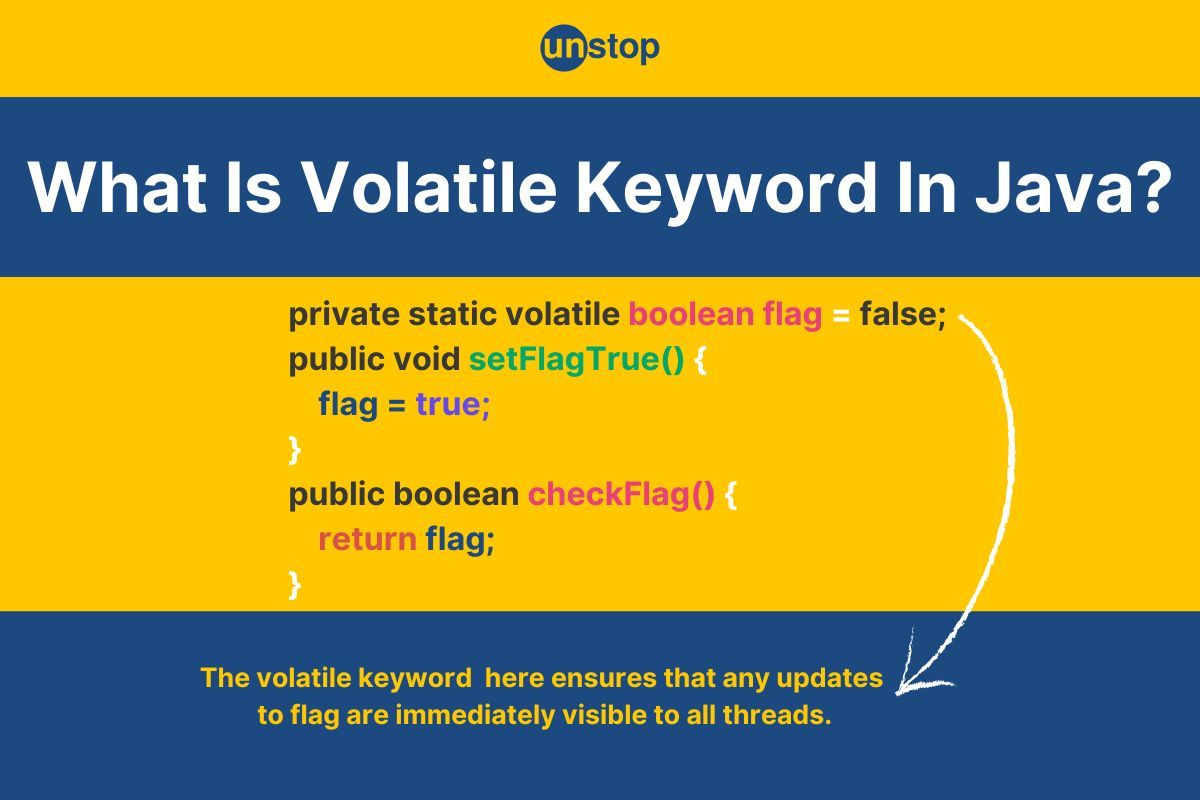
The volatile keyword in Java might sound a bit intimidating, but it’s actually a simple tool to help make your programs thread-safe. Imagine two or more people trying to share the same notebook—it can get messy if they don’t see the latest changes! Similarly, volatile ensures that every thread in your program sees the most up-to-date value of a shared variable.
In this article, we’ll break down what volatile does, how it works, best practices for its use, and provide examples you can easily understand. By the end, you’ll see how it helps keep your programs running smoothly, without unexpected behavior!
What Is The Volatile Keyword In Java?
The volatile keyword is a modifier in Java programming that is used with variables to ensure their visibility across threads. A volatile variable is always read from and written to the main memory, bypassing the thread's local cache. This is important in multithreaded programming, where different threads might be accessing and modifying the same variable. The volatile keyword ensures that any changes made to the variable by one thread are immediately visible to other threads.
Syntax Of Volatile Keyword In Java
volatile dataType variableName;
Here:
- volatile: It is a keyword that ensures visibility of updates across threads.
- dataType: It represents the data type of the variable.
- variableName: It is the name of the variable.
Real-Life Example To Understand This Better:
Imagine you have two threads in a program. One thread is responsible for updating a flag, and the other thread needs to check this flag to decide whether it should keep running or stop.
- Without volatile, the thread that checks the flag may not see the updated value because of caching in each thread. This could lead to the thread checking the flag repeatedly and not noticing the change made by the other thread.
- With the volatile keyword, when one thread changes the value of the flag, the update is immediately visible to the other thread. This ensures that the second thread always reads the most recent value.
How Does Volatile Keyword In Java Work?
The volatile keyword in Java works by ensuring visibility and ordering of variable updates across threads:
- Ensures Visibility: Changes to a volatile variable by one thread are immediately visible to all other threads. Threads read the value directly from main memory instead of using cached copies.
- Prevents Caching: The variable is not stored in thread-local caches, ensuring all threads work with the same value.
- Establishes a Happens-Before Relationship: A write to a volatile variable happens-before any subsequent reads of that variable by other threads. This ensures a consistent order of operations.
- Prevents Instruction Reordering: The compiler and CPU are prevented from reordering instructions involving volatile variables. This guarantees predictable behavior in multithreaded programs.
- Does Not Ensure Atomicity: The volatile keyword in Java does not make compound actions (e.g., counter++) atomic. Use synchronization for such operations to avoid race conditions.
- Use Case: Ideal for flags, status indicators, and variables shared across threads where only visibility (not atomicity) is required.
Explore this amazing course and master all the key concepts of Java programming effortlessly!
Using Volatile Keyword In Java To Control Thread Execution
Here’s a simple example demonstrating the use of the volatile keyword in Java for controlling thread execution:
Code Example:
public class VolatileExample {
private volatile boolean stopRequested = false;
public void start() {
new Thread(() -> {
while (!stopRequested) {
// Do some work
}
}).start();
}
public void stop() {
stopRequested = true;
}
}
Output:
The code itself doesn't directly produce any visible output. Its primary purpose is to create and manage a thread that runs until a stopRequested flag is set to true.
Explanation:
In the above code example-
- First, in the VolatileExample class, we declare a volatile boolean variable stopRequested, initially set to false.
- The start() method creates a new thread that continuously checks the value of stopRequested in a while loop. As long as stopRequested remains false, the thread keeps executing some work (represented by a comment).
- The stop() method sets stopRequested to true, signaling the thread to stop its execution.
- The volatile keyword ensures that any update to stopRequested made by one thread (such as calling stop()) is immediately visible to the other thread (which is checking stopRequested), allowing the thread to exit the loop and stop its work.
Using Volatile Keyword In Java To Signal Between Multiple Threads
Here’s a simple example demonstrating how the volatile keyword is used to signal between multiple threads in Java.
Code Example:
public class VolatileExample {
// Declare a volatile variable
private static volatile boolean flag = false;
public static void main(String[] args) throws InterruptedException {
// Thread 1: Sets the flag to true after some delay
Thread thread1 = new Thread(() -> {
try {
Thread.sleep(1000); // Simulate some work
flag = true; // Set flag to true
System.out.println("Flag set to true by Thread 1");
} catch (InterruptedException e) {
e.printStackTrace();
}
});
// Thread 2: Checks the flag and reacts when it becomes true
Thread thread2 = new Thread(() -> {
while (!flag) {
// Busy-wait until the flag is set to true
}
System.out.println("Flag checked by Thread 2: Flag is now " + flag);
});
// Start both threads
thread1.start();
thread2.start();
// Wait for both threads to finish
thread1.join();
thread2.join();
}
}
Output (set code file name as VolatileExample.java):
Flag set to true by Thread 1
Flag checked by Thread 2: Flag is now true
Explanation:
In the above code example-
- We declare a volatile boolean variable flag to ensure that its value is consistently visible to all threads.
- In the main() method, we create and start two threads:
- Thread 1 simulates some work by sleeping for 1000 milliseconds. After that, it sets flag to true and prints a message indicating that the flag has been set.
- Thread 2 enters a busy-wait loop, repeatedly checking the value of flag. It continues waiting until flag becomes true, at which point it prints a message showing that the flag is now true.
- Both threads are started using start() function, and then we use join() to ensure that the main thread waits for both threads to finish before completing the program.
- The volatile keyword ensures that when flag is set to true by Thread 1, Thread 2 immediately sees the change and exits the loop, allowing it to print the updated flag value.
Sharpen your coding skills with Unstop's 100-Day Coding Sprint and compete now for a top spot on the leaderboard!
Difference Between Synchronization And Volatile Keyword
Both the synchronization and volatile keywords play crucial roles in Java’s multithreading environment, but they serve different purposes. While synchronization ensures thread safety for complex operations by providing mutual exclusion, the volatile keyword guarantees visibility of changes to variables across threads without the overhead of locking.
Here's a detailed comparison between the synchronization and volatile keywords in Java:
Aspect |
Synchronization |
Volatile |
Purpose |
Ensures mutual exclusion to prevent race conditions in critical sections. |
Ensures visibility of changes made to a variable by one thread to other threads. |
Visibility |
Ensures visibility of changes to variables across threads within a synchronized block. |
Guarantees that updates to a volatile variable are immediately visible to all threads. |
Atomicity |
Provides atomicity for compound actions (e.g., x++, x = x + 1). |
Does not provide atomicity; individual reads and writes are atomic, but compound actions require additional synchronization. |
Locking Mechanism |
Uses locks (monitor) to control access to the synchronized block or method. |
Does not use locks. It ensures visibility but does not block or synchronize threads. |
Performance |
May cause performance overhead due to locking, especially with high contention. |
Lightweight and faster than synchronization, as it does not involve acquiring locks. |
Thread Safety |
Ensures thread safety for complex operations within the synchronized block. |
Ensures thread safety only for simple reads and writes; not for complex operations. |
Usage |
Used for protecting critical sections, ensuring only one thread can access the resource at a time. |
Used for flags or simple variables shared across threads where visibility is the main concern. |
Memory Consistency |
Guarantees that all changes made by one thread within a synchronized block are visible to other threads after the lock is released. |
Guarantees that the most recent value of the volatile variable is visible to all threads. |
Impact on Instruction Reordering |
Prevents instruction reordering inside a synchronized block. |
Prevents reordering of reads and writes to the volatile variable but not for other instructions. |
Use Case |
Suitable for managing complex, multi-step operations where atomicity and synchronization are needed. |
Best for simple variables like flags, counters, or state indicators where visibility is required but atomicity is not. |
Code Example:
// Using Synchronization
class SharedResource {
private int counter = 0;
public synchronized void increment() {
counter++; // Thread-safe increment due to synchronization
}
public synchronized int getCounter() {
return counter;
}
}
// Using Volatile
class SharedFlag {
private volatile boolean flag = false;
public void setFlag() {
flag = true; // Immediate visibility to other threads
}
public boolean checkFlag() {
return flag; // Always reads the latest value of flag
}
}
Output:
There are no actual threads or method calls in the code itself, so it does not directly produce any output.
Explanation:
In the above code example-
- We have a private integer variable counter in the SharedResource class that is initialized to 0.
- We then mark the increment() method as synchronized, ensuring that only one thread can execute it at a time, preventing concurrent modifications to the counter.
- Inside the synchronized method, the counter is incremented safely, making it thread-safe.
- The getCounter() method is also synchronized, allowing only one thread to access the counter's value at a time, ensuring consistency when reading the value.
- Now, in the SharedFlag class, we have a private boolean variable flag that is marked as volatile.
- The setFlag() method sets flag to true, and the volatile keyword ensures that this change is immediately visible to all threads, preventing caching of the variable in thread-local memory.
- The checkFlag() method always returns the latest value of flag, as it directly reads from the main memory, ensuring consistency across threads.
Common Mistakes And Best Practices While Using Volatile Keyword In Java
Here are some common mistakes and best practices when using the volatile keyword in Java:
Common Mistakes:
- Using volatile for Complex Synchronization:The volatile ensures visibility but does not guarantee atomicity, so using it for complex operations like incrementing a counter can lead to race conditions. For Example-
private volatile int counter = 0;
counter++; // Not atomic, could lead to race conditions
- Assuming volatile Handles Thread Communication: The volatile only ensures visibility, not proper coordination or mutual exclusion. For inter-thread communication, additional mechanisms like wait(), notify(), or synchronized blocks are necessary.
- Believing volatile Makes All Operations Thread-Safe: The volatile ensures that the value of a variable is visible across threads, but it doesn't guarantee thread safety for complex operations (e.g., checking and updating a variable together).
- Using volatile for Non-Primitive Types: The volatile can be used with primitive types or references to simple objects, but not with complex objects. It does not ensure atomicity for mutable objects like collections. For Example-
private volatile MyObject obj; // obj may still not be thread-safe
- Misunderstanding volatile Semantics: The volatile only affects visibility. It doesn’t synchronize access to a variable, leading to potential issues if combined with other operations on the variable. For Example-
private volatile boolean flag;
// The flag being volatile ensures visibility, but not atomic updates like checking and setting it together.
Best Practices:
- Use volatile for Simple Variables: Use volatile for flags or state indicators (like boolean, int, etc.) that are only read or written in simple operations. For Example-
private volatile boolean flag = false;
- Use Atomic Classes for Non-Atomic Operations: For operations like increments or complex reads and writes, prefer atomic classes like AtomicInteger from java.util.concurrent.atomic. For Example-
private AtomicInteger counter = new AtomicInteger(0);
counter.incrementAndGet(); // Atomic operation
- Combine volatile with Proper Synchronization: Use volatile in combination with synchronized blocks or Locks for thread safety when performing complex operations on shared data. For Example-
private volatile boolean flag = false;
public synchronized void toggleFlag() {
flag = !flag;
}
- Avoid volatile for Complex Object Synchronization: For mutable objects (e.g., collections), use synchronization mechanisms like synchronized or Lock to ensure thread safety, rather than volatile. For Example-
private final List<String> list = new ArrayList<>();
public synchronized void addToList(String item) {
list.add(item); // Use synchronization for complex objects
}
- Use volatile for Flags or State Indicators: The best use of volatile is for simple flags that indicate thread execution status or cancellation, ensuring immediate visibility across threads. For Example-
private volatile boolean stopRequested = false;
- Ensure Proper Visibility Across Threads: Remember that volatile ensures visibility of the variable’s latest value but does not manage atomicity or mutual exclusion, so use it when only visibility is needed.
Are you looking for someone to answer all your programming-related queries? Let's find the perfect mentor here.
Conclusion
The volatile keyword is a valuable tool for maintaining visibility and consistency of shared variables in multithreaded Java applications. However, it is not a silver bullet. While it addresses visibility issues, it falls short in providing atomicity and coordination. Use it wisely for simple use cases like flags or status variables, and combine it with synchronization mechanisms for more complex scenarios. By understanding its strengths and limitations, you can write safer and more efficient multithreaded code in Java.
Frequently Asked Questions
Q. What is the volatile keyword in Java, and when should it be used?
The volatile keyword in Java is used to ensure that a variable’s value is always visible to all threads. When a variable is declared as volatile, any read or write operation on that variable is directly performed on the main memory rather than cached in local thread memory. This is particularly useful in multithreaded programming when one thread modifies a variable and other threads need to see the latest value.
When to use: You should use volatile for flags or state variables where visibility is critical (e.g., flags to stop threads, shared status variables).
Q. Does volatile guarantee thread safety in Java?
No, volatile does not guarantee thread safety. It only ensures that changes made to a volatile variable are visible across all threads. However, it does not ensure atomicity. For example, incrementing a volatile counter (counter++) is not atomic, and it can still lead to race conditions.
Thread safety: To ensure thread safety for complex operations (e.g., incrementing a counter, checking and updating a value), you should use synchronization or atomic classes like AtomicInteger from java.util.concurrent.atomic.
Q. Can volatile be used for all types of variables in Java?
While volatile can be used with primitive data types (int, boolean, etc.) and object references, it is not suitable for complex data types or mutable objects like collections or user-defined objects.
Limitations: For complex or mutable objects, using volatile does not ensure atomicity or thread safety for object manipulations. In these cases, you should rely on synchronization (e.g., synchronized blocks or Lock objects) to manage thread-safe access to the object.
Q. What is the difference between volatile and synchronized in Java?
- Volatile: Guarantees visibility of changes to a variable across threads but does not guarantee atomicity or thread safety for composite operations. It is suitable for simple flags or state indicators.
- Synchronized: Ensures that only one thread at a time can access the synchronized block or method, providing mutual exclusion. It also guarantees visibility of changes, along with atomicity.
- Use case: Use volatile for flags or simple variables and synchronized for more complex operations where atomicity is required (e.g., read-modify-write operations).
Q. What happens if I use volatile incorrectly in Java?
Using volatile incorrectly can lead to subtle bugs. For instance, it does not solve the problem of thread synchronization for composite operations or mutable objects. If you use it for non-atomic operations, such as incrementing a counter (counter++), it can result in race conditions, leading to inconsistent or incorrect values.
Example: Using volatile on a counter or performing a complex calculation will not guarantee that the operation is atomic or thread-safe. For such operations, consider using AtomicInteger or synchronizing the method.
Q. How does the volatile keyword work with multiple threads in Java?
When a variable is marked as volatile, any write to that variable by one thread is immediately visible to other threads. This ensures that each thread accesses the most recent value of the variable, avoiding inconsistencies due to local caches.
Usage in multi-threading: The volatile keyword in Java is often used for controlling thread execution, signaling between threads (e.g., flags for stopping threads), and ensuring immediate visibility of updates without synchronization. However, it does not synchronize access to the variable, so it is not suitable for complex operations that require atomicity or mutual exclusion.
With this, we conclude our discussion on the volatile keyword in Java. Here are a few other topics that you might be interested in reading:
- 15 Popular JAVA Frameworks Use In 2023
- What Is Scalability Testing? How Do You Test Application Scalability?
- Top 50+ Java Collections Interview Questions
- 10 Best Books On Java In 2024 For Successful Coders
- Difference Between Java And JavaScript Explained In Detail
- Top 15+ Difference Between C++ And Java Explained! (+Similarities)
I’m a Computer Science graduate with a knack for creative ventures. Through content at Unstop, I am trying to simplify complex tech concepts and make them fun. When I’m not decoding tech jargon, you’ll find me indulging in great food and then burning it out at the gym.
Comments
Add commentLogin to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
Esuru Pooja.C 2 hours ago
Ankita Das 2 days ago
Siddhi Raney 4 days ago
Sunita Bhat 6 days ago
SIVANI Immidi 1 week ago
Mihir Kumar Ranjan 1 week ago