90+ Java Collections Interview Questions (+Answers) You Must Know
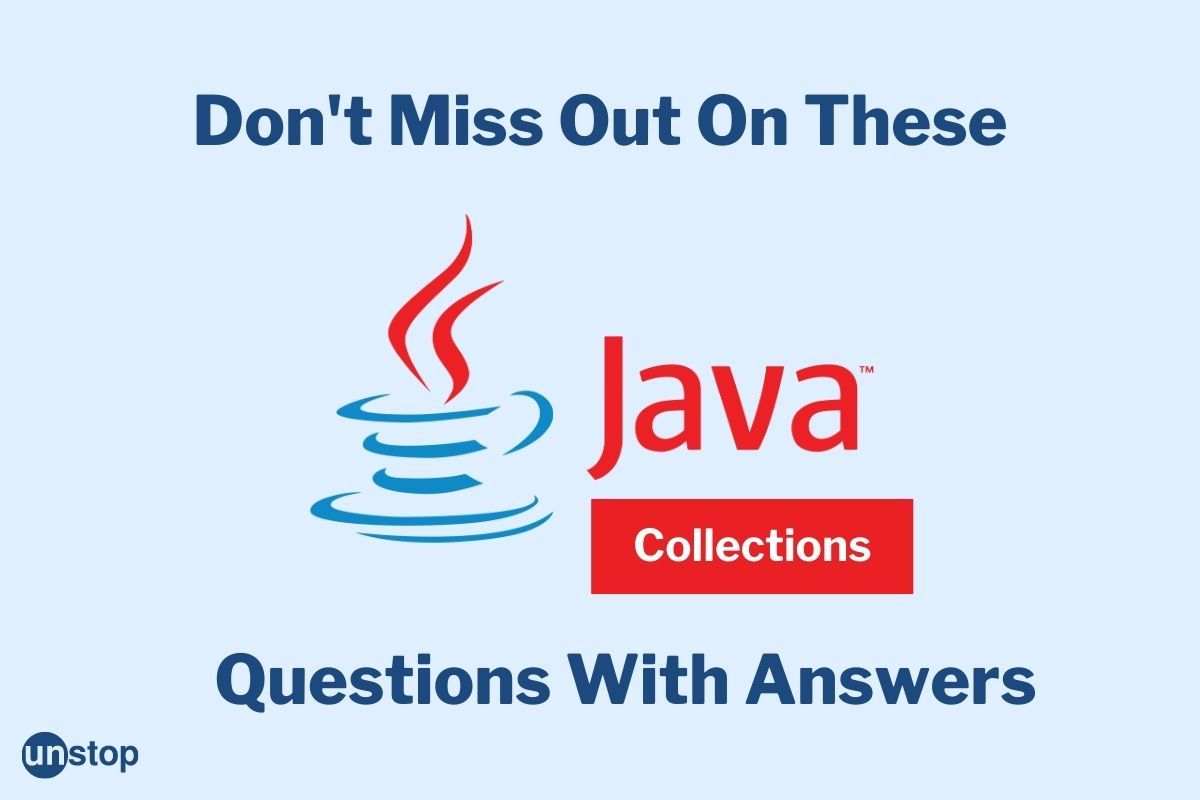
The Java Collections framework, a group of classes and interfaces, is the essential building element for creating data structures in Java. Given this significance, it is extremely important for you to prepare well when appearing for a job interview in this segment. And to help you in the preparations, we have listed the 93 Java Collections interview questions with answers that you must know.
To begin with, note that Java Collections offers robust data structures, like lists, maps, sets, stacks, and queues, allowing programmers to store and handle enormous amounts of data quickly. Algorithms for quickly searching, sorting, and modifying these collections are also included in the framework. Its effective implementations can be utilized to build applications ranging from easily distributed systems to complicated programs.
List Of Java Collections Interview Questions
Q1. What are collections in Java?
Java's Collections framework offers an architecture for storing and managing a collection of objects. It contains interfaces, implementations, and algorithms for using data structures like lists, sets, maps, etc.
In other words, the Java Collections framework provides a unified interface for accessing and manipulating different types of data structures, such as lists, maps, sets, etc. The collection of objects offers efficient implementation of several algorithms to manipulate these collections efficiently, like sorting, searching, or merging them optimally.
Q2. How do collections differ from the collections in Java?
Collections in Java are the classes and interfaces related to the collection framework for grouping elements. Collections differ from the Collection in Java as follows:
Collection: An interface is referred to as a collection in the Java.util.package.
- It is used to depict various objects as a single entity.
- It is comparable to the C++ programming language's container.
- The collection interface is the fundamental interface of the collection framework.
- It offers a variety of classes and interfaces for collectively representing a group of different items. List, Set, and Queue are the collection interface's main sub-interfaces.
- Despite being a component of the Java collection framework, the interface's Collection is not passed down to the map interface.
- The most important operations available through the Collection interface are add(), delete(), clear(), size(), and contains().
Collections: A utility class found in the Java.util.package.
- It defines a number of practical collection-related methods, including sorting and searching.
- The methods are all static.
- These methods provide much-needed simplicity for developers, enhancing their ability to work with the Collection framework.
- The collection elements can be sorted using the sort() function, which also offers the min() and max() methods for retrieving the minimum and maximum values, respectively.
Q3. Describe the hierarchy of the Collection framework.
The hierarchy of the Java Collection framework is-
Interfaces -> Abstract Classes-> Concrete Implementation Classes (i.e., ArrayList, Vector, LinkedList).
The Collection framework in Java defines a set of interfaces and classes that allow a programmer to collect and manipulate data efficiently.
The main hierarchy starts with the interfaces, such as collection, list, queue, set, etc., at its top. This is followed by abstract classes, then concrete implementation classes. It is the interfaces that define the operations that must be supported by all their sub-types, including both general-purpose implementations like ArrayList or LinkedLists and specialized ones like PriorityQueue or HashSet, respectively.
Q4. Give me four advantages of Java Collections.
The most important advantages of using Java Collections frameworks include-
- Improved performance and increased efficiency due to reusable code. And better memory utilization through efficient design.
- More robust programs by leveraging powerful tools provided by JDK.
- Flexibility over APIs, thus allowing easy use and modification according to structural changes within system components.
- Thread safety and easier development with readily available objects/ collections classes.
Q5. What are the features of Java Collections?
Some of the most prominent features of Java Collections are-
- Iterators - This feature allows one to traverse through elements of collections regardless of their type.
- Generics- This implies that only allowed types are accepted into collections to achieve type safety during compile time.
- Lambda Expressions –It provides a concise way for implementing functional programming paradigms on top of existing API libraries.
- Streams – They facilitate operations like filtering, mapping, etc., to be carried out on collection elements in a parallel manner.
- Serialization –It enables collections to be serializable for reliable storage and ease of transfer.
Q6. What is the function of a List Interface in Java Collections?
The List Interface in Java Collections stores and retrieves elements using indices and provides a range of useful methods for operating on those elements. It contains sequence-related operations such as size, add, remove, search, etc.
Q7. How does a fail-fast iterator work in Java Collections?
A fail-fast iterator is used to traverse elements of a collection in Java. It throws a Concurrent Modification Exception if the structure of the underlying collection has been modified outside the scope of iterators methods such as remove or add. This functionality helps guard against unpredictable results due to potential conflicts between multiple threads accessing and modifying a shared data structure concurrently. The idea behind this technique is that instead of allowing errors to go unnoticed, it fails early by throwing an exception at runtime rather than after some indeterminate period leading up to wrong behavior or incorrect output within our application logic.
Q8. Can duplicate elements be stored within a Collection interface in Java?
Yes, duplicate elements can be stored within a Collection interface, provided all their characteristics, like type, value, and identity, are different from one another. However, having similar values will not make them unique enough, so duplicate elements should never be added to Collections interfaces. If added, this would lead to unpredictable behavior when accessing these collections via APIs based on common conventions.
Duplicate elements may break the semantic correctness of a data structure and lead to unpredictable results when used in applications or algorithms. In duplicate elements, multiple copies of the same elements don’t add value but increase memory consumption unnecessarily. In many cases, duplicate elements can be replaced with better alternatives like sets which make sure every element is unique, thereby ensuring efficiency as well as accuracy
Q9. Are duplicate values allowed within an unordered collection such as HashMap or LinkedHashMap using the Java Collection framework?
No duplicate values are allowed within any unordered collection, such as HashMap or LinkedHashMap. In other words, duplicates are not allowed in any Collection framework based on Java. All values must be unique, a requirement imposed by the APIs embedded within implementations of Java collections. Duplicate values are not allowed in the Java Collection framework, as they would violate API constraints.
So, unordered collections such as HashMap or LinkedHashMap must contain unique values only, thus restricting duplicate values within these data structures.
Q10. What are some examples of static methods implemented with the help of the Java Collection framework?
Some examples of static methods that can be implemented with the help of the Java Collection framework include addAll(), sort(), and replaceAll().
Q11. How do you create and manipulate a dynamic array using Java Collections?
Creating a dynamic array using Java Collections is relatively easy.
- The most effective way to do this is using an ArrayList. An ArrayList is a dynamic array that can hold elements of any type and grows as more elements are added.
- To create an empty, dynamic array, you simply invoke the constructor by writing:
ArrayList<Type> list = new Arraylist<>(), where Type will be replaced with any valid data type such as int, double or String, etc.
- After creating the empty list, you can add items one by one using .add().
For example, if we were adding Strings to our list, we would write:
list.add("String1"); //Adds "String1" to position 0 in the List
list.add("String2"); //Adds "String2" to position 1 in the List
To manipulate these values once they have been added, you can access them by referring to their index within your array (the same way arrays work).
Q12. Explain how the Map interface works differently from others in the Java collection framework.
The Map interface in the Java Collection differs from other interfaces because it does not extend the collection interface like List or Set do. Instead, Map creates a key-value mapping relationship where each unique Key stores an associated Value.
Maps also have special methods that provide additional functionality, such as retrieving keys and values separately, removing entries based on their Keys, checking for membership by Keys only (not Values), etc. Additionally, since maps are typically unordered collections of entries, they contain no guarantees about ordering elements when iterating over them with an Iterator object.
Q13. What is a Doubly-Linked List, and why is it important in Java Collections?
A Doubly-Linked List is a data structure whose elements contain pointers to the next and the previous elements and their value. This list allows quick access to any item on it without having to search through an entire list of items. One can find an element by following the links from one node to another.
Furthermore, this structure facilitates insertion/deletion operations since one only needs two pointer updates (to or from the deleted/inserted item) instead of shifting all subsequent memory blocks within an array or vector-type arrangement. In Java collections, doubly linked lists are used in various implementations, such as the LinkedList class or AbstractSequentialList subclass. This is because they offer fast traversal capabilities in comparison to other types of linear structures like arrays and vectors that require iterating over their content sequentially when locating specific values.
Q14. How does the hash code for an object affect how objects are stored and retrieved within a collection?
The hash code for an object helps determine where objects are stored within a collection based on their computed hashes relative to each other's similarities, like, if two objects have similar input fields. Such objects would be mapped closer together than those that differ substantially in terms of field patterns, thus ensuring better overall performance via a more efficient lookup table query process.
Q15. What roles do Root interfaces play in Java Collections?
Root interfaces are command patterns used by Java Collections to establish a consistent API structure for all types of collection classes. This, in turn, enables developers to access common methods like add() or remove() without having to worry about implementation details related to different data structures.
Q16. How has the original collection been extended to produce better ways of storing data using more modern methods?
The original collection of data storage methods, such as paper-based filing systems and microfilm/microfiche archives, have been extended to include more modern methods like digital databases, cloud computing services, Optical Character Recognition (OCR) technologies for digitizing text documents, and Electronic Document Management Systems (EDMS).
These new methods allow for more precise searching and retrieval capabilities, easier sharing of information across multiple locations and devices, secure encryption technologies to protect the data from unauthorized access, automated backups, and archiving services that can store terabytes or petabytes of data.
Other ways that the original collection of data storage has been extended include:
- Automated Storage and Retrieval Systems (ASRS): The systems manage large amounts of inventory in warehouses, hospitals, and factories. ASRS uses robotic arms with sorting trays or shelves to store and categorize items quickly while providing increased safety for employees without direct access to hazardous materials.
- Big Data Analytics Platforms: Combining powerful database technologies like Hadoop, these platforms can process complex queries quickly across huge datasets. This type of analysis can help organizations detect patterns useful for making more informed decisions.
- Magnetic Storage Media: Today’s magnetic media, such as USB sticks, hard drives, and tapes, provide a reliable way for companies to retain records indefinitely if stored correctly in secure locations away from extreme temperatures or environmental factors that could damage them over time.
- Cloud Services: Cloud-based services like Amazon Web Services (AWS), Google Compute Engine (GCE), and Microsoft Azure offer scalability on demand at reduced costs compared with local hardware solutions. Businesses today can leverage virtualized environments where they only pay for what is being used at any given moment rather than investing heavily upfront in infrastructure investments without knowing how it will grow over time.
Q17. Can you explain the main characteristics of Collection Objects, including their purpose and functionality?
- They are data structures that store and group multiple items of the same or different types, such as strings, numbers, objects, etc.
- Their main purpose is to organize large amounts of data efficiently so it can be easily accessed for manipulation.
- Some common operations performed on collections include adding/removing elements from them, searching through them for specific items, etc.
- Different collection objects have different characteristics depending on your language; some may come with useful methods, while others offer more flexibility when dealing with their contents.
- Examples of collection objects include arrays, stacks, queues, linked lists, etc.
Q18. What advantages do key-value pairs offer when using Java Collections?
The advantages of using key-value pairs are-
- Key-value pairs offer an efficient way to store and retrieve data from a collection by using the key as an identifier for that specific item. This ensures no duplication of values with different keys since each value can only be associated with one unique key.
- Key-value pairs also allow for quick access and retrieval times since they provide direct access based on the key rather than having to loop through each element or search linearly until it finds something that matches the criteria you are searching for.
- They also enable easier manipulation of items within collections because operations like adding, deleting, or updating elements can all be done quickly and easily at any given time without requiring changes in the existing codebase related to traversing through collections manually when making modifications.
Q19. Explain some common operations that can be performed on list objects, such as add(), remove(), etc.
List objects have several main methods which can be used to perform various tasks ranging from sorting elements to adding/removing them from the list. Some of these are-
- The add() method: This is probably the most commonly used way of inserting new items into a pre-existing arrangement of values,
- The remove() method: The remove() lets us delete an object by its index position within the collection, for instance.
- Other operations, such as contains(), clear() getIndexOf (), lastIndexOf (), etc., are also available for list objects in the collections framework, depending upon the type of implementation being leveraged.
Q20. What challenges may arise when working with Legacy Class while trying to implement new features into them utilizing Java Collections?
When working with Legacy Classes within the Java Collections framework, there could be difficulties in maintaining older codebases. This is due to unstructured design philosophies implemented during earlier versions that make it difficult to implement modern practices without risking breaking existing functionality already present before changes are made. To limit this risk, large portions of the codebase may need to be rewritten for full compatibility between old and new editions before any further improvements can be safely added.
Q21. How does the equals method work when comparing two objects within a collection?
The equals method compares two objects based on their hash codes (or sometimes other properties), checking whether there's at least partial agreement between them. If so, the two items are considered equal otherwise, they'll be marked as different entities. This serves an important purpose when analyzing whether certain elements exist inside collections or not.
Q22. What benefits come from having Random Access capabilities within your Java Collections framework?
Random Access capabilities let users quickly access stored values by jumping directly to their position rather than scanning all content linearly searching for the desired items, which may produce worse performance results under larger datasets than expected.
- Random Access provides a fast, constant-time lookup for the desired data, regardless of its location in the collection.
- Random Access allows users to implement quick search algorithms and create efficient sorting strategies without traversing through all elements within a list or array.
- The Random Access capability also enables weaker forms of memory locality optimization. It does so by avoiding unnecessary object copying when accessing multiple objects from an unsorted list containing references directly into that list structure's nodes at random locations.
Q23. When creating new instances of a collection class, what should one consider regarding initial capacity requirements?
When creating new instances of a collection class, it is important to consider initial capacity requirements since this defines how much memory will be allocated to accommodate the requested information. That is, setting too low a value could lead to insufficient storing resources, whereas an overly excessive one might incur unnecessary costs depending on the application context. It is advisable to set the initial capacity according to the estimated size of data that will be stored, in order to optimize space usage and performance.
Q24. Can you give an example where multiple entities would need to be collected via one single entity reference point implemented by means of Java Collections?
An example where multiple entities need to be collected via a single entity reference point implemented through Java Collections would be using Map objects such as HashMap. Here, each element can have its unique key index that represents a particular object, making it easy to iterate over the entire dataset and extract specified data points without issues.
Q25. What advantages does implementing the serializable interfaces in a collection bring, and what are some drawbacks?
The advantages of implementing serializable interfaces in Java Collections include better portability and the ability to transfer across different platforms with minimal effort. However, some drawbacks come into play related to increased size units per operation performed during serialization/deserialization processes reducing overall efficiency levels compared to non-serialized approaches. Serializable interfaces also provide improved security as sensitive data can be encrypted during serialization.
Q26. How can Enum types be effectively stored within Java Collections, enabling easy retrieval and manipulation of data?
Enum types can be effectively stored in Java Collections by mapping their values into suitable key indices. This allows for easy retrieval manipulation of data and reduction of any type of casting errors when referencing individual elements from within collections.
Q27. How can Legacy Classes be compatible with modern-day Collections frameworks such as Java?
Strategies to make Legacy Classes compatible with modern-day collections frameworks such as Java involve refactoring the codebase according to newer programming styles and patterns. Also, introducing more object-oriented based paradigms, deleting old unneeded methods, and replacing them with equivalent commands written using more contemporary syntaxes.
Q28. Explain how Immutable classes work when being incorporated into Java Collection-based programs.
Immutable classes guarantee certain integrity principles related to the objects themselves when incorporated into Java Collection-based programs. This means that no change will be applied after an instance's initialization phase, allowing better control over how elements behave when using multiple threads simultaneously without risking undesirable side effects due to concurrent modification issues.
Q29. Describe the process for safely iterating through a collection without risking any unforeseeable errors or exceptions occurring.
Fail-safe iterators are a technique designed to traverse collections safely without risking any unexpected exceptions from occurring. By using the iterators' combined guarantee clauses (such as while-loops), data points can be checked multiple times before deciding whether they conform to expected criteria or otherwise stop execution prematurely. Fail-safe iterators also work well in preventing race conditions during multi-threaded operations when dealing with read/write accesses.
Q30. What is the difference between fail-fast and fail-safe collections in Java?
The primary difference between fail-fast and fail-safe collections in Java is:
- The fail-fast collection is a type of collection that throws ConcurrentModificationException when its structure gets modified while iterating over it.
- This type of collection cannot be used for multi-threaded applications, as once a thread modifies its structure, all other threads trying to modify or use this collection will get an exception during iteration.
- On the contrary, fail-safe collections are not tied to any underlying data source like an array.
Q31. How do you ensure the thread safety of a collection in Java?
To maintain thread safety on any Java Collections object, we must-
- Ensure proper synchronization whenever accessing or modifying elements inside such objects by using synchronized blocks/methods or using aspects defined by util class methods like Collections#synchronizedMap etc.
- Using the Atomic classes from Java 5 instead of non-thread safety mutable classes like ArrayList, HashMap, etc.
- Synchronizing access to shared resources using Lock and ReentrantLock objects ensures thread safety.
These are a few ways to ensure the thread safety of a collection in Java.
Q32. What primitive data types are supported by the Java Collection framework?
The primitive data types supported by the Java Collections framework are int, long, boolean, float, char, byte, and short. These can store values in collection classes like List and Set. However, wrapping is required when passing a primitive value as an object into any of these collections.
Q33. What is the default capacity for an ArrayList when no other parameters are specified while creating it?
Default capacity for ArrayList when no other parameters are specified while creating it:
When we create an instance of ArrayList without providing any initial capacity, then the default capacity size allocated is ten elements where each element occupies 8 bytes plus a 4-byte chunk dedicated to maintaining the index information associated with this list object, thus making total requirement per instance at least 42 bytes along with additional memory required for object header portion allotted as per JVM configurations.
Q34. Explain what Weak References mean with respect to garbage collection in Java.
When we talk about weak references within the context of garbage collection in Java, then it means that such objects can be reclaimed from memory even when they haven't been explicitly referenced by our application code directly. And hence making them available for future reallocations if required as per JVM memory management operations helps us avoid serious out-of-memory type issues caused due to poor management of allocated resources.
Q35. Describe how Priority Heap works internally within a Priority Queue implemented using the Java Collections library.
Here is how the priority heap works internally within the context of Priority Queue implemented using the Java Collections library-
- The priority queue is realized through a special form of the binary heap called an 'implicit' heap implementation.
- Here the elements are prioritized by their natural ordering or comparator defined earlier under respective collections utility classes used for sorting purposes.
- The priority queue enables efficient removal operation at each step towards achieving the desired result set, depending on query filter conditions submitted during the time frame initiation phase, along with additional parameters such as page size and limit clause.
Q36. What utility classes are available in the Java Collections framework?
There are several utility classes available with Java Collections frameworks like Collection, List, Set, and Map. They form the core foundation for implementing various data structure types and help from utility methods associated with these base class implementations, such as Arrays#sort() or comparator-based sorting utilities, to maintain order in respective objects while storing them inside collection-type object instances.
Q37. Is it possible to make a List or Set read-only? If yes, how can we do that in Java?
Yes! We can make a list or set an instance in Java read only by wrapping that particular array under an unmodifiable wrapper provided by util APIs such as Collections#unmodifiableList(), Collections#unmodifiableSet(). This would prevent external modifications to our original underlying source. Further eliminating the chances of losing consistency across multiple threads working simultaneously upon the same resource during parallel processing scenarios.
Q38. What is the actual class used by Java Collections framework for implementing Queue data structure?
The actual class used by the Java Collections framework for implementing queue data structure is called PriorityQueue. It uses a priority heap supporting the same set of operations like insert, delete, etc., as provided in linear linked list implementations. But note that here all elements are internally stored and evaluated based on natural ordering or custom comparator logic provided earlier while creating respective instances, ensuring that every step towards retrieving a top element from this collection follows O(log n) time complexity. This provides efficient implementation with respect to memory utilization.
Q39. How can we use the read-only list in Java Collections?
The read-only lists in Java Collections can be used to provide a view of an existing list structure that cannot change. That is, the users may traverse the elements and retrieve data if they desire but cannot modify the contents. Thus creating an immutable collection in the read-only list.
Q40. What are the different collection implementations available in Java?
Several implementations are available for use when working with Collections classes in Java. This includes ArrayList, HashSet, LinkedHashSet, and TreeMap structures. Some specialized variations also exist, such as PriorityQueue or ConcurrentSkipListSet, which serve specific purposes under certain circumstances, such as sorting operations on threads or access optimization.
Q41. What basic operations of Collections can be performed in Java?
Basic operations performed within the Collection classes include adding items (add), replacing items (replace), removing items (remove), checking the size/length of the collection(length or Size() method ), traversing through each element(for loop/iterator) tracking position respective to other objects stored inside it, etc.
Code example on Basic operations:
Output:
Printing all elements using for loop:
School
Books
Students
Q42. What is the queue construction time when using a single sorting sequence within a multi-threaded environment?
The queue construction time largely depends on what underlying single sorting sequence logic has been applied before accessing elements from it. Usually, either Insertion Sort or Merge Sort algorithms offer increased performance when dealing with large multi-threaded environments where multiple queues are accessed simultaneously due to their relative complexities & speed during execution stages associated with division-based sorting operations.
Example of single sorting sequence:
Output:
Given array: 10 15 4 6
Sorted Array: 4 6 1015
Q43. How does a Red Black Tree affect positional access and extra memory usage compared to Hash Table structures?
When compared to Hash Table structures, a Red Black Tree offers improved positional access performance as elements inserted into them are stored according to their relative order. This makes the speed of retrieval & traversal when attempting to locate specific data points within large collections much faster than would otherwise be possible with regular hash table implementations. This is because regular hash table implementations rely on randomness for storing knowledge.
Red Black Trees also require extra memory than Hash Tables, as the additional overhead of storing and maintaining the relative data order involves a set of extra structures to be allocated in memory for each node within it. In Red Black Tree, it also requires extra memory for rebalancing occur, as any inserted node is expected to adhere to a certain set of rules and conditions. This also requires extra logic for successful insertion & maintenance.
Q44. What is a Java Class in the context of Collections?
A Java Class is a basic type of object-oriented programming used to define and store collections objects in Collections. It contains fields, methods, and constructors for creating an instance or collecting object instances.
Q45. How are Collection utility classes used to support manipulating collections?
Collection utility classes provide support for manipulating collections by providing several methods, which are often implemented as static utility functions such as sort(), binarySearch(), and reverse(). These classes also have various other features like iterators, comparators, etc.
Q46. Explain what fail-fast and fail-safe iterators are.
Fail-fast iterators will throw a ConcurrentModificationException if they detect that an element in the underlying collection has been changed without the iterator’s knowledge. This is because fail-fast iterators do not use locks to monitor changes, so they can never guarantee correctness if elements are modified during iteration.
A fail-safe iterator uses some locking mechanism to ensure it detects concurrent modifications and does not suffer from this problem. It also guarantees correct behavior even when elements are modified while looping through them. However, performance may be worse than a fail-fast implementation since extra synchronization overhead must be used for each access operation on the Collection object being iterated.
Q47. Can you explain how flow control works in relation to collections objects?
Flow control in relation to collections objects is used to efficiently iterate over a collection structure and perform operations on its elements without having to manually keep track of the position within it. For example, using while-loop, for-each loop, iterators, etc.
Q48. Describe how to store elements within a Collection object instance.
Elements are stored within a Collection object instance through internal data structures such as ArrayList, HashTable, etc. Alternatively, they can be wrapped in a custom Java class that implements a Collection interface offering direct access.
Q49. What benefits come from implementing a read-only interface on top of your underlying data structure or algorithm that stores your actual objects or key values?
Implementing a read-only interface provides benefits like allowing encapsulation so users cannot change underlying actual objects or key values directly. Also, when dealing with complex algorithms in the read-only interfaces, these interfaces allow separating their logic from actual implementations. Thus making them more modular and maintainable, plus providing safer concurrency threading scenarios.
An example of a read-only interface algorithm that stores your actual objects or key objects is-
Output:
Returns the value of Map object associated with the key.
Q50. How can one leverage Iterable Interface as part of their implementation strategy while dealing with Java Collections framework?
We can leverage Iterable Interface while developing our own custom Java Collection type classes because this interface extends trait traversable. This provides basic functionality for traversing elements inside the container plus methods for each (f:(A) -> B): Unit/map(f: A->B ): Collection[B], which allows you to apply transformations across existing collection's elements and then combine the results into another collection if desired.
Example of Iterable interface:
Output:
Student Marks: 30,40,50
Q51. What techniques must be considered for internal implementation of natural sorting based on an internal iterator's implementation strategy while designing their own custom Java Collection type classes?
If one wants to carry out the internal implementation of natural sorting on their own custom Java Collections framework, one can use techniques such as the comparator interface. It offers useful functions such as compare (Object o1, Object o2 ). Additionally, they can also use the Iterator interface, which also has several methods that help during the implementation process, like next(), hasNext(), etc. making the job much easier plus efficient if done right.
Q52. What is a collection of elements in Java?
A collection of elements in Java is a group of objects that are stored and accessed using the same interface. For example, a List or a Map. Grouping the collection of elements that are related to objects together allows developers to write code more efficiently by manipulating all its elements at once instead of looping through them individually.
Q53. What are the features of the collections utility class in Java?
Some features of the Java Collection's utility class are-
1. It provides algorithms to manipulate the collections like sorting, randomizing, reversing, etc.
2. It implements several interfaces such as List, Set, Map, and more for the manipulation of data
3. Provides methods to operate on collections such as element searching, filtering elements from a collection, etc.
4 .it helps overcome any limitations while dealing with arrays, making it easier for developers since they don't need external libraries or classes while working with them.
Q54. Can you explain what is meant by read-only collections?
In simple words, a read-only collection is something that cannot be altered after its creation. And any attempts to modify them will throw an error since they are considered immutable data structures that do not allow for changes once created. However, new versions could be added via copy-constructors. Read-only collections help ensure data consistency when sharing between threads, as nobody can change values while others may still need access to original content within the collection.
In other words, read-only collections refer to a situation where you do not modify data within the existing structure. Instead, you only allow operations with read access, such as getting elements from it without updating or changing their contents (e.g., get(Object o) ).
Q55. How can we ensure concurrency of retrievals when using Java Collections?
To achieve concurrency of retrievals when dealing with Java Collections, we should use synchronization techniques such as locks or thread pools where available/applicable. This is how multiple requests can be processed concurrently without interfering with each other's results due to race conditions caused by accessing shared resources simultaneously from different threads.
Q56. Could you describe some of the basic interfaces available for use in Java Collections?
The basic interfaces available in Java Collections are Iterable, Collection, List, Set, and Map - each provides a different way to store objects which can then be manipulated together as a group or individually by providing certain operations over them (such as sorting).
Q57. What types of objects are used as keys to store and access values within a collection?
The key objects used within collections uniquely identify an item or element stored in them. They tend to be small enough, so they don't take up too much memory but are still distinct enough to allow easy lookup and access when needed. Examples of key objects include Strings (for indexing) or ints (as IDs), depending on the structure required/desired from your collection's contents.
Q58. How is an Array List used to store a list of objects in Java?
An Array List in Java stores a list of objects by allowing index-based access to data stored within the structure. The array stores each object as an element. It allows users to add, remove or modify elements using various methods provided by the collection framework, such as add(), get(), set(), etc.
Q59. What are the major differences between the original class and the vector class in Java Collections?
Original class and vector class are distinct classes from the Java Collections Framework designed especially for storing sequences of objects (lists). One significant difference between them is that while the original class uses an Object[] array internally, which resizes whenever it runs out of space, the vector class creates new arrays when full, copying all existing elements into newly created ones.
Q60. Describe the pop operation method for manipulating elements from a Collection data structure in Java.
The pop operation method manipulates collection structures by removing the last element from a given container and returning its value so we can further process it or discard depending upon our requirements. The pop operation basically works like stack pushing/popping operations, but instead of the topmost part, we’re dealing with the last item inside the container.
Q61. Can you explain how a dequeue operation works with regard to collections in Java?
The dequeue operation allows us to manipulate containers by inserting and removing elements from both ends of a given container. It hence permits faster access in case of queues or stack-like behaviors when dealing with priority-based systems such as thread pools. It also provides us with more control over these operations.
Q62. What advantages does an enqueue operation offer when dealing with Collections' data structures in Java?
Enqueue operation offers advantages like adding new items at the end of the queue while taking out existing ones from the start. Thus allowing users to carry out various FIFO/LIFO type tasks simultaneously and quickly by using this operation on data structures. Moreover, due to their uniformity, enqueues offer better throughput than other methods, allowing additional items inside the collection within a shorter time.
Q63. What are the benefits of using an unbounded queue within the concurrent environment programs written in Java?
Unbounded queues within the concurrent environment programs written in Java language provide benefits like less memory consumption since there is no need for prearranging larger than the required array size. Also, improved performance via lower waiting time between operations and higher throughput since the use of data structure of unbounded queue type act as an automatic synchronization mechanism.
Q64. Explain what types of errors can occur while in an operating environment data structures using Java Collections.
A ConcurrentModificationException can occur when modifying a data structure in an operating environment using Java Collections if the sequence of operations performed is not thread-safe. This type of error typically occurs when multiple threads attempt to modify a shared data structure simultaneously, and one or more threads attempt to change the size or content of that shared object while another thread is also trying to access it concurrently.
If two simultaneous modifications happen, then this exception will be thrown because concurrent modification has occurred and could lead to unpredictable results.
Other errors that may occur with concurrency include deadlock (two processes waiting for each other), race conditions (different parts updating different pieces without ensuring consistency), and starvation (one process hogging resources).
Q65. What is the purpose of concurrent collections in Java?
Concurrent collections are used to construct thread-safe data structures that can be shared amongst multiple threads in a concurrent application.
Q66. What does a ConcurrentHashMap Classwork do, and how can it be used?
The ConcurrentHashMap class efficiently implements a hash map, allowing multiple threads to safely modify the contents without the need of additional synchronization or locking mechanisms that are generally required by other implementations such as Hashtable and Collections synchronizedMap(). ConcurrentHashMap class implements the Map interface, its parent interface, and additional Java 6 features like atomic putIfAbsent(), etc. It also supports another feature called ‘Computing on Entries,’ which allows you to compute values based on existing entries in a ConcurrentHashMap class.
Q67. What are some methods available to use with the Queue Interface in Java?
Some methods available for use with the Queue Interface include add(), offer(), remove(), poll(), element(), and peek().
Q68. How do we handle an exception in a thread thrown while running in Java?
The ideal approach to handle an exception thrown in a thread is to use a try/catch block or the UncaughtExceptionHandler interface. You can respond appropriately to certain exceptions that could be thrown while running your code by using a try/catch block. With its uncaughtException() method, the UncaughtExceptionHandler adds a second level of exception handling. This method can provide more advanced behaviors, such as reporting details about the error before quitting your program if necessary.
Q69. Can you explain what the implementation of HashCode looks like when programming with Java?
Implementation of Hashcode involves overriding the object class' hashCode() method and returning a unique integer value, which should be derived from any distinctive features of the object in question. For instance, its properties values, if applicable, etc.
Q70. What would happen if you set up a public int Hashcode for your application in Java's programming model?
When programming in Java, it is important to ensure that the public int Hashcode variable defined for an application does not result in any conflicting instances or duplicates. This can be done by having multiple entities share matching hash codes within their respective initialization stages before processing can occur concurrently inside a memory boundary.
Q71. What is meant by the thread-safe variant? What kind of specialized behavior does it bring into our applications?
The thread-safe variants of concurrent collections such as ConcurrentHashMap, queue interfaces, and other implementations help to provide specialized behavior in our applications. They protect the data from unexpected errors due to race conditions when multiple threads simultaneously modify shared states without prior warnings or indications. Thread-safe variants help improve performance compared to traditional approaches, allowing us to meet our targets faster and more efficiently with minimal risks involved along the way.
Q72. Can you highlight the differences between arrays and using single Null Key concepts in our programming environments?
The differences between arrays and single Null Key concepts in programming environments are-
Arrays: -An array is a data structure that stores multiple values of the same or different type. An ordered list can hold any value, number, string, object, etc. Each array item has its index number, which can be accessed quickly and easily. Arrays allow for efficient storage and retrieval of information when compared to single null vital concepts.
Single Null Keys Concepts: -A single null key concept is a programming method used to store data as keys with associated values without repeating any keys during storage (only one 'null' key). When attempting to retrieve this information on demand, only one query needs to be made since all elements are stored under separate unique identifiers rather than grouped into arrays like typical databases. This allows for faster access times but may require more coding effort making them less common in some environments than using arrays.
Q73. Which child interface should we implement or consider while working with the Java Collection Framework after certain prerequisite settings are set or applied correctly to accomplish the desired result from our codebase?
The most common child interface to consider when working with the Java Collection Framework is the List Interface.
- This interface provides an ordered collection of objects that can be manipulated using various methods such as add, remove, clear, and sort.
- It also supports index-based positioning so that elements may be added or removed from specific positions in a list.
- Additionally, Lists support operations like searching for particular items and iterating through them.
- Therefore, this makes it very useful for accomplishing desired results from a codebase since it provides easy access to many powerful functions that can manipulate data collections quickly and efficiently.
Q74. What is the specialized behavior of a Java Collection?
Specialized behavior of a Java Collection includes the ability to contain heterogeneous data types, sorting elements in different ways depending on the type of collection (e.g., LinkedList vs. Vector), iterating over them sequentially and randomly, etc.
Q75. How does the garbage collector manage memory for collections in Java?
The garbage collector manages memory for collections by tracking objects that are no longer needed or referenced within a program. And also, deleting these objects so they do not take up any more system resources than necessary. Garbage collector allows programs to run efficiently without needing manual intervention from developers to free unused memory themselves when it is no longer required.
Q76. Can you explain a marker interface and how it affects collections in Java programming?
A marker interface is an empty parent class that provides certain features, such as serialization and compression capabilities, that child classes can use. Implementing this marker interface will allow implementations specific benefits when working with collections in Java programming environments due special set of methods included inside marker interfaces allowing better maintainability over time, etc.
Q77. What legacy interfaces exist to aid working with Java Collections that may be obsolete by modern standards?
Legacy interfaces like Enumeration, Iterator, and Comparable are still available. Still, older versions lack some powerful features in comparison to built-in modern object-oriented languages today, limiting their employment outside classic cases, which come across now & then.
Q78. Could you describe an enumeration interface and its purpose associated with collections within Java language operations?
An enumeration interface provides functionality similar to iteration, specifically going through each element individually within a collection while maintaining some order. The enumeration interface can also provide additional features such as filtering, sorting, and retrieving specific elements from the collection.
Example of Enumeration interface:
Output:
Vector Elements [1, 2, 3, 4, 5] 1 2 3 4 5
Q79. How are type of objects differentiated yet managed effectively using Java Collection classes?
The type of objects can be differentiated based on their properties like visibility (public/private), mutability (immutable/mutable), or memory requirements, which play an important role in how they are managed inside Java Collections classes. For instance, when using `List` interface implementations, all its element types must be defined beforehand unwaveringly during operations involving adding new individual items dynamically in the type of objects.
Q80. What processes determine single object equality between elements within various Java Collection constructions?
Single object equality between different elements is determined by comparing various fields that define each object type, including whether two references point to the exact location in memory. This helps distinguish multiple copies of similar item components taking part in many Java Collections construction methods available today.
Q81. What are some common patterns used when creating collection pipelines?
Commonly-used patterns include streaming APIs, map/reduce operations, the declarative composition of multiple functions into one pipeline system, and more advanced features like parallelism for optimization purposes.
Q82. Are any generic type parameters included in newer versions of the core library?
Yes, libraries like the Java Collection Framework have added generic type parameters to facilitate better collections pipelines for customizations on existing persistent data structures. These include Func<T, U>, IEnumerable<T>, and List<T>.
Q83. Are heterogeneous elements allowed off common storage types found so far from past examples? Or rather just primitive or wrapper ones as likely best practice implementations instead?
Heterogeneous objects are permitted within Java collections off common storage types to guarantee maximum flexibility for developers when operations require so. Like using wrapper classes such as `Integer,` `Boolean` etc., can be used to represent primitive ones found normally during any DDA mapping operation seen among many implementations typically located inside big (Java) Collection architectures implemented currently.
Q84. What if the processing requires sorting of elements of these current elements before retrieval will occur correctly for proper operation of the Collection in question?
Sorting of elements of these current elements can be done with various strategies depending on the type of collection. For example, sorting via a custom comparator object is sometimes necessary. At the same time, the Collections class contains several static sort methods that take care of most basic situations like alphabetical order by default without further ado.
Q85. What is the difference between heterogeneous elements and homogenous elements in Java Collections?
1. Heterogeneous elements are those that contain different types of data, while homogenous elements have the same kind of data present in them.
2. Heterogeneous elements allow greater flexibility when accessing data, while homogenous elements are limited regarding what can be done with the data.
3. Heterogeneous elements require more memory than homogenous elements, containing various types and subtypes.
Q86. How can you create an array of strings in Java?
An array of strings can be created using the String[] keyword followed by a set of square brackets containing list items separated by commas.
The basic syntax for this is-
String[] myArray = {"item 1"," item 2"," item 3"};
Example of an array of strings:
Output:
book[a] : Java Programming
book[b] : Data Structures and Algorithms
book[c] : Database Systems Design & Implementation
book [d]: Artificial Intelligence
Q87. Can we use a string key for mapping values using collections classes?
Yes, if desired, we can use a string key for mapping values using collections classes such as Maps and HashMaps. The value associated with each string key varies between each pair within these structures and not necessarily the keys themselves, which must remain unique identifiers when used as part of this structure design pattern.
Q88. What is the purpose of a universal iterator in the Java Collections framework?
Universal iterators allow us to traverse through all objects stored within Java Collection frameworks regardless of their implementation or underlying data model represented therein (e.g., ArrayList). Universal iterators facilitate easy looping operations over multiple blocks without having to code any additional logic specifically tailored toward particular interface implementations.
Q89. Which concurrent utility classes are available to help with thread-safe operations on collections data structures?
There are several concurrent utility classes available to help with thread-safe operations on collections data structures, such as CopyOnWriteArrayList and ConcurrentHashMap. Both provide optimized read/write processes for multiple threads accessing the same collection concurrently, preventing the risk of deadlock issues occurring within their execution pathways and offering other benefits.
Q90. How do we create an initial list and then convert the list into another form using collection class methods?
To create an initial list, we can use a List interface (or its implementation classes) like ArrayList. We can then convert it into another form using methods from various Collection class types, such as sort() or sublist(). This will allow us to manipulate our lists more efficiently by sorting elements / splitting them up based upon specific criteria inputted beforehand into our program logic. After transforming these values accordingly, thenceforth throughout each's respective lifecycle onward, indeed, indeed ad infinitum.
Q91. What are some methods available to retrieve elements from different types of lists of values?
Methods that can be used to retrieve elements from different lists of values within Java Collections are getting (int index) and removing (Object o). These will allow us to access the item in question by its given numerical position or object representation, respectively. They can be combined with other methods like forEach() loop so that we can iterate over our collections more efficiently as desired/required.
Q92. Is there any functionality between collections and standards that allow interchangeability among them?
Yes, some functionality allows interchange between collections and standards, such as importing and exporting data in different formats, querying multiple databases simultaneously, or using APIs to integrate with other systems. Some tools and frameworks also allow developers to convert between collections and standards, such as JSON Schema, Microdata, or RDF.
Q93. Which Java Collection implementation supports legacy interfaces and custom object storage?
- The Java Collections framework's Vector class allows for the storage of custom objects and supports legacy interfaces.
- The ArrayList class also supports legacy interfaces and allows for the storage of custom objects.
- The Java Collections framework's LinkedList class additionally supports the storage of custom objects compatible with legacy interfaces.
This compiles our list of Java Collections interview questions and answers. Here are a few more articles that will help you prepare for that job interview:
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Blogs you need to hog!
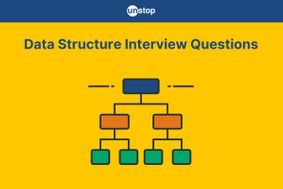
55+ Data Structure Interview Questions For 2024 (Detailed Answers)
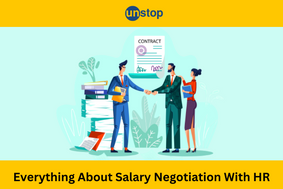
How To Negotiate Salary With HR: Tips And Insider Advice
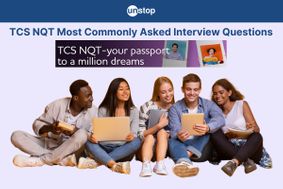
Best 80+ TCS NQT Most Commonly Asked Interview Questions for 2025
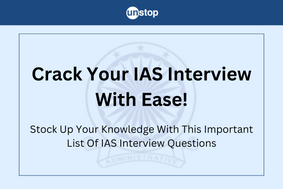
Comments
Add comment