Thread In Java | Lifecycle, Methods, Priority & More (+Examples)
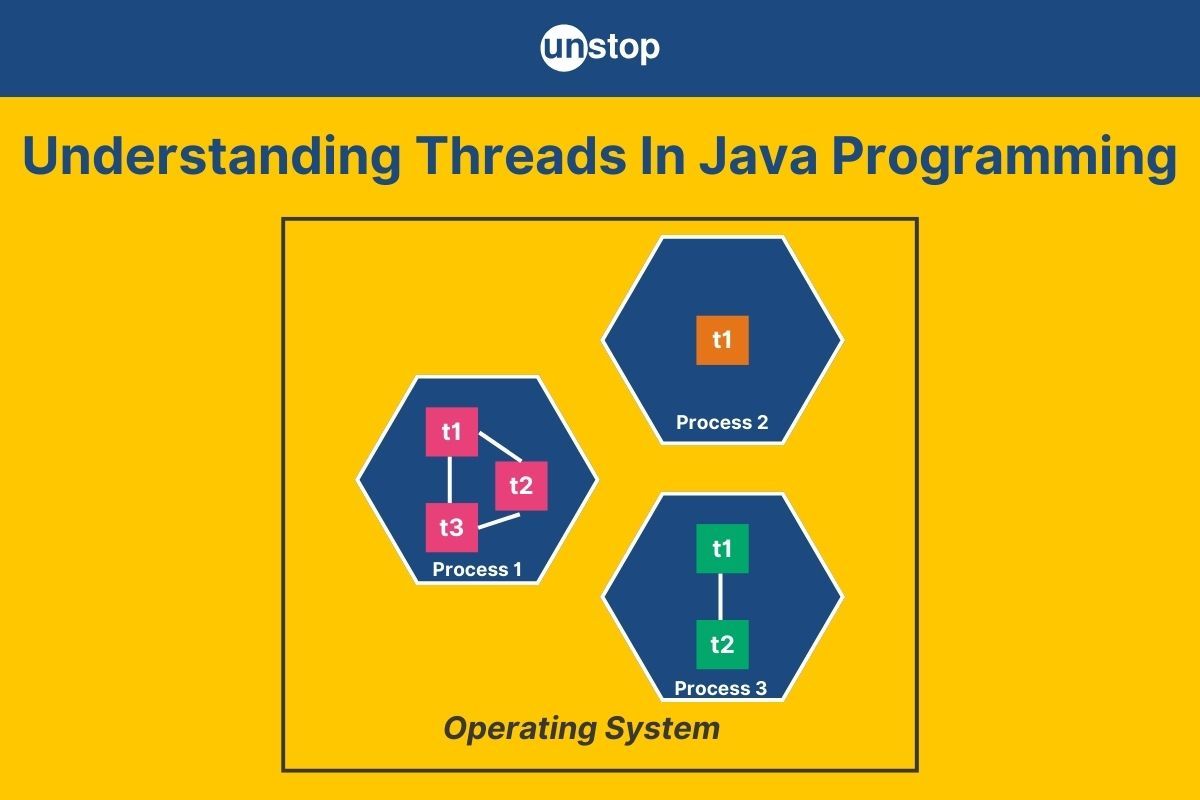
Java threads form the backbone of multitasking in Java, allowing programs to perform multiple operations simultaneously. Whether it's handling background tasks, managing user interactions, or processing large datasets, threads make Java applications more efficient and responsive.
In this article, we will explore the world of Java threads, starting with an understanding of their lifecycle, key methods, and how to create and manage them. Additionally, we’ll explore synchronization techniques to avoid concurrency issues and discuss common challenges when working with threads.
What Is A Thread In Java?
A thread in Java is a lightweight and smallest unit of execution within a program. Think of a program as a big job, and threads as smaller workers inside that job, each handling a specific task. Multiple threads can execute independently, yet share the same memory space, which makes them powerful for tasks like downloading files, processing user input, or performing calculations simultaneously.
Java programming provides robust support for threads through the java.lang.Thread class and the java.util.concurrent package. Here's how it works:
- Creating Threads: You can define a thread by either extending the Thread class or implementing the Runnable interface.
- Managing Threads: Java offers methods like start(), run(), sleep(), join(), and more to control thread execution.
- Concurrency Utilities: The java.util.concurrent package adds advanced features like thread pools and locks.
Key Features Of Threads In Java
Some of the key features of thread in java are as follows:
- Lightweight Nature: Threads are lighter compared to processes because they share the same memory and resources of their parent process.
- Independent Execution: Each thread executes independently but can work collaboratively by accessing shared resources.
- Efficient Multitasking: Threads are ideal for scenarios where tasks need to execute concurrently, such as in a multi-user server or GUI applications.
Thread Vs Process
Threads and processes are fundamental concepts in concurrent programming, but they serve distinct purposes and operate differently. Here are the key differences between the two:
Feature |
Thread |
Process |
Definition |
A lightweight sub-task within a process. |
A heavy-weight, independent execution unit. |
Memory Sharing |
Shares memory and resources with other threads in the same process. |
Has its own dedicated memory and resources. |
Communication |
Inter-thread communication is faster since they share memory. |
Requires Inter-Process Communication (IPC), which is slower. |
Overhead |
Low overhead; threads are lightweight. |
Higher overhead; processes are heavier. |
Creation Time |
Threads are quicker to create and manage. |
Processes take longer to create and manage. |
Fault Tolerance |
If one thread crashes, it may affect the entire process. |
Processes are isolated; one crashing doesn’t affect others. |
Execution |
Multiple threads can run concurrently within a single process. |
Processes run independently of each other. |
Use Case |
Used for tasks that require frequent communication and shared memory, like a multi-threaded server. |
Used for isolating tasks that need separate execution, like running multiple applications. |
Real-Life Analogy
Think of a company:
- Process: The company itself is a process. It has its own budget, departments, and resources, operating independently from other companies.
- Thread: Employees in the company are threads. They perform individual tasks but share the company’s resources, such as office space and equipment. Communication between employees (threads) is easier and faster, but if one employee makes a major mistake, it could impact the entire company.
What is a Thread Life Cycle In Java?
The thread life cycle refers to the various states a thread goes through during its lifetime, from its creation to its termination. In Java, threads are represented by the Thread class, and their life cycle includes five main states:
1. New (Created)
- When a thread is created using the Thread class or by implementing the Runnable interface, it is in the New state.
- At this stage, the thread exists as an object but hasn’t started running yet.
- Action Required: You need to call the start() method to move the thread to the next stage. For Example-
Thread t = new Thread(); // Thread is in the New state
2. Runnable (Ready to Run)
- After calling the start() method, the thread enters the Runnable state.
- In this state, the thread is ready to run but is waiting for the CPU to assign it time for execution.
- The thread scheduler determines which thread gets CPU time based on factors like thread priority. For Example-
t.start(); // Moves thread to Runnable state
3. Running (Executing)
- When the CPU allocates time to the thread, it transitions from Runnable to Running.
- In this state, the thread executes the code inside its run() method.
- A thread remains in the Running state until it either completes its task or is paused. For Example-
public void run() {
System.out.println("Thread is running...");
}
4. Blocked/Waiting (Temporarily Paused)
- A thread enters the Blocked/Waiting state when it cannot proceed with execution temporarily.
- This can happen due to reasons like:
- The thread is waiting for a resource (Blocked).
- The thread is explicitly made to wait (Waiting).
- Methods like sleep(), wait(), or synchronization can move a thread to this state. For Example-
synchronized(obj) {
obj.wait(); // Thread enters the Waiting state
}
5. Terminated (Dead)
- When a thread completes its task or the run() method finishes execution, the thread enters the Terminated state.
- A terminated thread cannot be restarted. If further execution is needed, you must create a new thread object. For Example-
public void run() {
System.out.println("Thread task completed!");
}
What Are Thread Priorities?
In Java, thread priorities determine the relative importance of a thread compared to others. The thread scheduler uses these priorities as a hint to decide which thread should run next when multiple threads are in the Runnable state. However, thread priorities don't guarantee execution order—they merely influence it.
- Priority Range: Each thread in Java has a priority represented by an integer. The priority values range from:
- Thread.MIN_PRIORITY (1) - Lowest priority.
- Thread.NORM_PRIORITY (5) - Default priority.
- Thread.MAX_PRIORITY (10) - Highest priority.
- Default Priority: By default, a thread inherits the priority of the thread that created it. Usually, this is Thread.NORM_PRIORITY unless explicitly changed.
- Setting Priorities: The setPriority() method is used to set a thread's priority, and the getPriority() method retrieves it.
Explore this amazing course and master all the key concepts of Java programming effortlessly!
Creating Threads In Java
In Java, threads can be created in two primXary ways:
- By extending the Thread class.
- By implementing the Runnable interface.
Both methods allow us to define the task to be performed in the run() method.
1. Using the Thread Class
In this method, we create a class that extends the Thread class and override its run() method to define the thread's task. After creating an instance of the class, we call the start() method to initiate the thread.
Working Steps:
- Extend the Thread class using extends keyword in Java.
- Override the run() method to define the task.
- Create an object of the class.
- Call the start() method to initiate the thread.
Code Example:
Output:
Thread running using Thread class!
Explanation:
In the above code example-
- We define a class, MyThread, that extends the Thread class to create our own thread functionality.
- Inside the MyThread class, we override the run() method, which serves as the entry point for our thread. Here, it prints a simple message: "Thread running using Thread class!".
- In the Main class, within the main() method, we create an object of MyThread, representing a new thread.
- We call the start() method on the thread object, which internally triggers the run() method to execute in a separate thread.
- As a result, we see the message printed, demonstrating that our thread is running independently of the main thread.
2. Using the Runnable Interface
This method involves implementing the Runnable interface and defining the task in its run() method. The Runnable object is then passed to a Thread object to create and start the thread.
Working Steps:
- Implement the Runnable interface.
- Define the task in the run() method.
- Create an object of the class.
- Pass the object to a Thread constructor.
- Call the start() method on the Thread object.
Code Example:
Output:
Thread running using Runnable interface!
Explanation:
In the above code example-
- We create a class, MyRunnable, that implements the Runnable interface, which requires us to define the run() method. Inside this method, we print a message: "Thread running using Runnable interface!".
- In the Main class, within the main() method, we create an object of MyRunnable. This object represents the task that we want to run in a separate thread.
- We then pass the MyRunnable object to a Thread object by using the Thread constructor, which allows the thread to execute the run() method of the MyRunnable class.
- We start the thread by calling the start() method on the Thread object. This triggers the execution of the run() method in a new thread, printing the message.
Therefore:
- Use Thread class for simpler tasks where you don't need the flexibility of multiple inheritance or sharing the task across threads.
- Use Runnable interface for more complex applications where you want better design, reusability, or need to work with thread pools and shared tasks.
Java Thread Methods
Java thread methods are functions provided by the Thread class that allow us to control and manage thread behavior and execution in a multi-threaded environment. These methods enable us to perform various operations like starting a thread, pausing its execution, checking its status, managing priorities, and synchronizing threads.
They are essential for thread management, providing control over when and how threads run, wait, and interact with each other in a program. Here’s a table explaining the commonly used Java thread methods:
Method |
Description |
start() |
Starts the execution of a thread by invoking the run() method in a new thread of execution. |
run() |
Contains the code that constitutes the thread's task. It is executed when the thread is started. |
sleep(long millis) |
Pauses the thread’s execution for the specified number of milliseconds. |
join() |
Allows one thread to wait for the completion of another thread. It blocks the current thread until the other thread finishes. |
interrupt() |
Interrupts the thread's execution. If the thread is blocked in sleep(), wait(), or join(), it will throw an InterruptedException. |
isAlive() |
Checks whether the thread is still alive (i.e., still running or waiting to run). |
setPriority(int priority) |
Sets the priority of the thread. The priority is a number between Thread.MIN_PRIORITY and Thread.MAX_PRIORITY. |
getPriority() |
Returns the current priority of the thread. |
setName(String name) |
Sets the name of the thread, which can help in debugging and identifying threads. |
getName() |
Returns the name of the thread. |
yield() |
Suggests to the thread scheduler that the current thread is willing to yield its current use of the CPU, allowing other threads to execute. |
stop() |
Deprecated. It attempts to stop a thread immediately. It's unsafe and can lead to resource issues or inconsistencies. |
wait() |
Causes the current thread to wait until it is notified (using notify() or notifyAll()) or interrupted. |
notify() |
Wakes up a single thread that is waiting on the current object’s monitor. |
notifyAll() |
Wakes up all threads that are waiting on the current object’s monitor. |
Commonly Used Constructors In Thread Class
The Thread class in Java provides multiple constructors to create and initialize threads in different ways. Below are the most commonly used constructors of the Thread class along with their purposes and examples:
1. Thread()
It creates a thread object without assigning any task to it. The thread will need to override the run() method before starting. For Example-
Thread t = new Thread() {
public void run() {
System.out.println("Thread is running!");
}
};
t.start();
2. Thread(Runnable target)
It creates a thread object and associates it with a task (a class implementing the Runnable interface). The Runnable object provides the task logic through its run() method. For Example-
Runnable task = () -> System.out.println("Task executed by thread!");
Thread t = new Thread(task);
t.start();
3. Thread(String name)
It creates a thread object with a custom name but without any assigned task. The run() method must still be overridden or implemented later. For Example-
Thread t = new Thread("CustomThreadName") {
public void run() {
System.out.println(Thread.currentThread().getName() + " is running!");
}
};
t.start();
4. Thread(Runnable target, String name)
It creates a thread object, assigns a task (Runnable), and gives it a custom name. Combines the functionality of the Thread(Runnable target) and Thread(String name) constructors. For Example-
Runnable task = () -> System.out.println(Thread.currentThread().getName() + " executing task!");
Thread t = new Thread(task, "NamedThread");
t.start();
5. Thread(ThreadGroup group, Runnable target)
It creates a thread within a specific thread group and assigns a task (Runnable). Useful for grouping threads logically for management and control. For Example-
ThreadGroup group = new ThreadGroup("CustomGroup");
Runnable task = () -> System.out.println(Thread.currentThread().getName() + " in group: " + group.getName());
Thread t = new Thread(group, task);
t.start();
6. Thread(ThreadGroup group, String name)
It creates a thread in a specific thread group with a custom name but without assigning a task. For Example-
ThreadGroup group = new ThreadGroup("CustomGroup");
Thread t = new Thread(group, "GroupThread") {
public void run() {
System.out.println(Thread.currentThread().getName() + " in group: " + group.getName());
}
};t.start();
Thread Synchronization In Java
Thread synchronization in Java is a mechanism to control the access of multiple threads to shared resources. It ensures that only one thread can access a critical section of code or resource at a time, preventing data inconsistency and race conditions.
When multiple threads access shared resources (e.g., variables, files, databases) simultaneously, their operations might overlap and cause unpredictable results. Synchronization ensures:
- Data Consistency: Prevents corruption of shared data.
- Mutual Exclusion: Allows only one thread to execute a synchronized block at a time.
- Thread Safety: Ensures the program behaves correctly when accessed by multiple threads.
Java provides several ways to synchronize threads: Synchronized Methods, Synchronized Blocks, Static Synchronization, Locks (from java.util.concurrent package)
For Example:
Explanation:
In the above code snippet-
- We define a class, SharedResource, which includes a method printNumbers(int n) to print the first five multiples of a given number n.
- Inside the printNumbers method, we use a synchronized block to ensure that only one thread at a time can access the code within this block when using the same object of SharedResource.
- The method iterates from 1 to 5, calculates the multiples of n, and prints them one by one.
- After printing each multiple, we pause the thread execution for 500 milliseconds using Thread.sleep(500) to simulate a delay.
- If the thread is interrupted during the sleep period, it throws an InterruptedException, which we handle by printing the exception details.
- This design helps maintain thread safety when multiple threads try to access the same instance of SharedResource, avoiding conflicts or unexpected results.
Common Challenges Faced While Using Threads In Java
Using threads in Java can significantly enhance application performance, but it comes with its own set of challenges. Here are some common issues developers face:
- Race Conditions: Multiple threads accessing shared resources simultaneously can lead to unpredictable results. For Example- Two threads updating the same variable may produce incorrect values.
- Deadlocks: Two or more threads waiting for each other indefinitely, preventing further execution. For Example- Thread A locks Resource 1 and waits for Resource 2, while Thread B locks Resource 2 and waits for Resource 1.
- Thread Starvation: Threads with lower priorities may be unable to execute if higher-priority threads dominate CPU time.
- Thread Interference: Incorrect behavior due to threads overwriting each other’s operations on shared resources. For Example- Inconsistent intermediate states during reads and writes.
- Resource Contention: Threads competing for limited resources (e.g., memory, CPU) can degrade performance.
- Difficult Debugging: Debugging multithreaded applications is complex due to non-deterministic thread scheduling.
- Memory Consistency Issues: Threads may not have updated views of shared variables due to caching.
- Overhead: Excessive creation and destruction of threads can introduce significant performance overhead.
Sharpen your coding skills with Unstop's 100-Day Coding Sprint and compete now for a top spot on the leaderboard!
Best Practices For Using Threads In Java
Below are some best practices for working with threads in Java:
- Use Synchronization Wisely: Synchronize only critical sections of code to prevent race conditions. Avoid over-synchronizing to reduce thread contention and improve performance.
- Avoid Nested Locks: Acquire locks in a consistent order to prevent deadlocks. Minimize the use of multiple locks to simplify the design.
- Use Thread Pools: Prefer ExecutorService or thread pools instead of manually creating threads to manage resources efficiently. This prevents excessive thread creation and improves scalability.
- Minimize Shared Resources: Reduce dependency on shared resources to limit contention among threads. Favor thread-local variables if possible.
- Prefer volatile for Flags: Use the volatile keyword for simple shared variables, ensuring visibility across threads without synchronization overhead.
- Handle Exceptions Gracefully: Catch exceptions within threads to prevent unexpected terminations. Log or handle errors appropriately for debugging and stability.
- Implement Graceful Shutdowns: Provide clean termination mechanisms for threads, such as shutdown() for thread pools. Avoid abrupt terminations that can leave resources in an inconsistent state.
- Avoid Busy Waiting: Use synchronization primitives like wait(), notify(), or Condition instead of continuously checking a condition in a loop.
- Use Higher-Level APIs: Leverage java.util.concurrent classes like ReentrantLock, Semaphore, and CyclicBarrier for robust thread synchronization and management.
- Test Concurrently: Simulate high-concurrency scenarios during testing to identify issues like race conditions, deadlocks, or thread starvation. Use tools like thread analyzers to detect potential threading issues.
- Document Multithreaded Code: Clearly document synchronization logic, shared resources, and thread interactions to aid future maintenance.
- Optimize Thread Priorities Cautiously: Use thread priorities sparingly, as their behavior can vary across platforms.
- Avoid Excessive Thread Creation: Limit the number of threads to match the available system resources, especially CPU cores.
- Favor Immutable Objects: Use immutable objects where possible, as they are inherently thread-safe and reduce synchronization needs.
- Be Cautious with Thread.sleep(): Avoid using Thread.sleep() for synchronization; instead, use proper synchronization mechanisms like CountDownLatch or wait().
Real-World Applications Of Threads In Java
Threads in Java play a vital role in building high-performance, scalable applications. Here are some common real-world applications where threads are widely used:
1. Web Servers and Application Servers
- Purpose: Handle multiple client requests simultaneously.
- Example: A web server like Apache Tomcat uses threads to serve multiple users by spawning a thread for each incoming request.
2. Real-Time Systems
- Purpose: Execute time-critical tasks concurrently.
- Example: Real-time monitoring systems like traffic control, flight booking systems, or healthcare equipment where multiple sensors send data simultaneously.
3. Multimedia Applications
- Purpose: Perform concurrent tasks like video playback, audio decoding, and user interactions.
- Example: Media players or video conferencing software rely on threads for smooth audio-video synchronization.
4. Asynchronous Tasks
- Purpose: Execute background tasks without blocking the main program.
- Example: Chat applications use threads for real-time message updates while allowing the user to perform other actions.
5. Gaming Applications
- Purpose: Handle various aspects like rendering graphics, processing player input, and managing AI behavior concurrently.
- Example: Multiplayer games use threads to ensure a seamless experience for all players.
6. File Processing
- Purpose: Read or write multiple files concurrently to improve efficiency.
- Example: Log file analysis tools process large datasets in parallel using threads.
7. Background Services
- Purpose: Run recurring or long-running tasks in the background.
- Example: Java threads are used for scheduled tasks, such as sending emails, data backups, or database cleanup.
8. Data-Intensive Applications
- Purpose: Perform computationally intensive tasks like sorting, searching, and data transformation across large datasets.
- Example: Threads are employed in data analytics platforms and machine learning pipelines for parallel processing.
9. IoT and Embedded Systems
- Purpose: Manage multiple connected Iot devices simultaneously.
- Example: Smart home systems use threads to communicate with sensors, lights, and appliances in parallel.
10. Financial Applications
- Purpose: Handle concurrent transactions securely and efficiently.
- Example: Online banking systems use threads to process multiple user transactions simultaneously while ensuring data consistency.
11. Parallel Programming and Multithreading Libraries
- Purpose: Perform tasks in parallel to optimize performance on multicore processors.
- Example: Java threads are often utilized in frameworks like Fork/Join or ExecutorService for parallel task execution.
Are you looking for someone to answer all your programming-related queries? Let's find the perfect mentor here.
Conclusion
Threads are a powerful feature in Java that enable developers to build responsive, efficient, and scalable applications by leveraging concurrent execution. Whether it’s managing multiple client requests in a web server, processing large datasets in parallel, or handling real-time user interactions in games, threads play a pivotal role in modern software development.
By understanding the lifecycle of a thread, proper synchronization techniques, and best practices, developers can harness the full potential of multithreading while avoiding pitfalls like race conditions and deadlocks. With tools like ExecutorService and classes from the java.util.concurrent package, Java simplifies thread management, making it easier to design robust multithreaded applications.
Frequently Asked Questions
Q. What is a thread in Java?
A thread in Java is the smallest unit of a process that can run independently within a program. Java threads allow multiple tasks to execute concurrently, sharing the same memory space, which makes applications more efficient and responsive. Threads are part of Java's java.lang.Thread class.
Q. What is the difference between a thread and a process?
- Thread: A lightweight subunit of a process. Threads share the same memory space and resources, making context switching faster.
- Process: An independent program with its own memory and resources. Processes are heavier than threads, and context switching between processes is slower.
Q. How do we create threads in Java?
Threads can be created in two ways:
- Using the Thread class: Extend the Thread class and override the run() method.
- Using the Runnable interface:Implement the Runnable interface and pass the instance to a Thread object. The start() method is used to begin the thread's execution.
Q. What is thread synchronization, and why is it important?
Thread synchronization ensures that multiple threads do not interfere with each other while accessing shared resources, preventing race conditions and ensuring data consistency. In Java, synchronization can be achieved using:
- The synchronized keyword (methods or blocks).
- Higher-level constructs like ReentrantLock or Semaphore from java.util.concurrent.
Q. What are the different states in the thread lifecycle?
The thread lifecycle in Java includes:
- New: The thread object is created but not yet started.
- Runnable: The thread is ready to run but waiting for CPU allocation.
- Running: The thread is actively executing.
- Blocked/Waiting: The thread is paused, waiting for a resource or signal.
- Terminated: The thread has finished execution.
Q. What are thread priorities, and how do they work?
Each thread in Java has a priority ranging from 1 (lowest) to 10 (highest). Thread priorities help the thread scheduler determine the order in which threads are executed, though the actual behavior depends on the underlying OS. The priority can be set using the setPriority() method.
Q. What are common challenges when working with threads, and how can they be mitigated?
Common challenges include:
- Race Conditions: Avoided using synchronization or volatile variables.
- Deadlocks: Prevented by acquiring locks in a consistent order and minimizing nested locks.
- Resource Contention: Minimized by reducing shared resource dependency or using thread-local variables.
- Thread Starvation: Ensured by fair scheduling using higher-level constructs like ReentrantLock.
With this, we conclude our discussion on the concept of threads in Java. Here are a few other topics that you might be interested in reading:
- Final, Finally & Finalize In Java | 15+ Differences With Examples
- Super Keyword In Java | Definition, Applications & More (+Examples)
- How To Find LCM Of Two Numbers In Java? Simplified With Examples
- How To Find GCD Of Two Numbers In Java? All Methods With Examples
- Throws Keyword In Java | Syntax, Working, Uses & More (+Examples)
- 10 Best Books On Java In 2024 For Successful Coders
- Difference Between Java And JavaScript Explained In Detail
- Top 15+ Difference Between C++ And Java Explained! (+Similarities)
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment