Java Programming Language
Table of content:
- History Of Java Programming Langauge
- Infographic For History Of Java
- What’s In The Name | History Of Java
- Key Features Of Java
- Advantages And Disadvantages Of Java
- The Version History Of Java Langauge
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is JDK?
- How To Download Java Development Kit (JDK) For Windows, MacOS, and Linux?
- Set Environment Variables In Java
- How To Install Java (JDK) On Windows 64-Bit Machine?
- How To Install Java (JDK) On Linux?
- How To Install Java (JDK) On macOS?
- How To Test Java Installation?
- How To Write Your First Java Program On Linux OS?
- Conclusion
- Frequently Asked Questions
Table of content:
- Java Programming Language | An Introduction
- 15 Key Features Of Java
- Write Once Run Anywhere (WORA) | Features Of Java
- Java Editions
- 5 New Features Of JAVA 8
- 5 New Features Of JAVA 11
- What Makes Java Popular?
- Conclusion
- Frequently Asked Questions
Table of content:
- What is Java?
- Advantages of Java
- Disadvantages of Java
Table of content:
- What Is Java Programming?
- Role Of Integrated Development Environments (IDEs) In Java Development
- 15 Best Java IDE For Developers
- In-Depth Comparison Table
- Conclusion
- Frequently Asked Questions
Table of content:
- Key Differences Between Java And Core Java
- What Is Java?
- What Is Core Java?
- Applications Of Java
- Applications Of Core Java
- When To Use Java?
- When To Use Core Java?
- Conclusion
- Frequently Asked Questions
Table of content:
- What Are Variables In Java Language?
- How To Declare Variables In Java Programs?
- How To Initialize Variables In Java?
- Naming Conventions For Variables In Java
- Types Of Variables In Java
- Local Variables In Java
- Instance Variables In Java
- Static Variables In Java
- Final Variables In Java
- Scope and Lifetime of Variables In Java
- Data Types Of Variables In Java (Primitive & Non-primitive)
- Java Variable Type Conversion & Type Casting
- Working With Variables In Java (Examples)
- Access Modifiers & Variables In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Are Identifiers In Java?
- Syntax Rules For Identifiers In Java
- Valid Identifiers in Java
- Invalid Identifiers in Java
- Java Reserved Keywords
- Naming Conventions & Best Practices For Identifiers In Java
- What Is An Identifier Expected Error In Java?
- Reasons The Identifier Expected Error Occurs
- How To Fix/ Resolve Identifier Expected Errors In Java?
- Conclusion
- Frequently Asked Questions
Table of content:
- What Are Data Types In Java?
- Primitive Data Types In Java
- Non-Primitive Data Types In Java
- Key Differences Between Primitive And Non-Primitive Data Types In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Are Operators In Java?
- Types Of Operators In Java
- Unary Operators In Java
- Arithmetic Operators In Java
- Assignment Operators In Java
- Relational Operators In Java
- Logical Operators In Java
- Bitwise Operators In Java
- Shift Operators In Java
- Increment & Decrement Operators In Java
- Ternary Operator In Java
- Instanceof Operator In Java
- Precedence & Associativity Of Java Operators
- Advantages & Disadvantages Of Operators In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is A Return Statement In Java?
- Use Cases Of Return Statements In Java
- Returning A Value From A Method In Java
- Returning A Class Object In Java
- Returning Void (No Value) In Java
- Advantages Of Using Return Statements In Java
- Limitations Of Using Return Statements In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Are Keywords In Java?
- List Of Keywords In Java
- Detailed Overview Of Java Keywords With Examples
- What If When Keywords In Java Are Used As Variable Names?
- Difference Between Identifiers & Keywords In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Abstract Keyword In Java?
- Use Of Abstract Keyword In Java
- Abstract Methods In Java
- Abstract Classes In Java
- Advantages Of Abstract Keyword In Java
- Disadvantages Of Abstract Keyword In Java
- Abstract Classes Vs. Interfaces In Java
- Real-World Applications Of Abstract Keyword
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is throws Keyword In Java?
- How Does The throws Keyword Work?
- Throwing A Checked Exception Using throws In Java
- Throwing Multiple Exceptions Using throws In Java
- Throwing A Custom Exception Using throws In Java
- When To Use The throws Keyword In Java
- Difference Between throw and throws Keyword In Java
- Best Practices For Using The throws Keyword In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is The Volatile Keyword In Java?
- How Does Volatile Keyword In Java Work?
- Using Volatile Keyword In Java To Control Thread Execution
- Using Volatile Keyword In Java To Signal Between Multiple Threads
- Difference Between Synchronization And Volatile Keyword
- Common Mistakes And Best Practices While Using Volatile Keyword In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding Super Keyword In Java
- Super Keyword In Java With Instance Variables
- Super Keyword In Java With Method Overriding
- Super Keyword In Java With Constructor Chaining
- Applications Of Super Keyword In Java
- Difference Between This And Super Keyword In Java
- Advantages Of Using Super Keyword In Java
- Limitations And Considerations Of Super Keyword In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding This Keyword In Java
- Uses Of This Keyword In Java
- Using This Keyword For Referencing Instance Variables
- Using This Keyword For Invoking A Constructor
- Using This Keyword For Invoking A Method
- Using This Keyword With Getters And Setters
- Difference Between This And Super Keyword In Java
- Best Practices For Using This Keyword In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is new Keyword In Java?
- Uses Of The new Keyword In Java
- Memory Management With new Keyword In Java
- Example 1: Creating An Object Of A Class Using new Keyword In Java
- Example 2: Creating An Array Using The new Keyword In Java
- Best Practices For Using new Keyword In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is The Transient Keyword In Java?
- Real-Life Example Of The Transient Keyword In Java
- When To Use The Transient Keyword In Java
- Example 1: Effect Of Transient Keyword On Serialization In Java
- Example 2: Skipping Sensitive Data During Serialization With Transient Keyword In Java
- Using Transient With Final Keyword In Java
- Using Transient With Static Keyword
- Difference Between Transient And Volatile Keyword In Java
- Advantages And Disadvantages Of Transient Keyword In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Static Keyword In Java?
- Characteristics Of Static Keyword In Java
- Static Variables In Java
- Static Method In Java
- Static Blocks In Java
- Static Classes In Java
- Static Variables Vs Instance Variables In Java
- Advantages Of Static Keyword In Java
- Disadvantages Of Static Keyword In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is A Static Method In Java?
- Use Cases Of Static Method In Java
- Using Static Method In Java To Create A Utility Class
- Using Static Method In Java To Implement The Singleton Design Pattern
- Difference Between Static And Instance Methods In Java
- Limitations Of Static Method In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding Final Keyword In Java
- Final Variables In Java
- Final Methods In Java
- Final Classes In Java
- Difference Between Static And Final Keyword In Java
- Uses Of Final Keyword In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Key Difference Between final, finally, And finalize In Java
- What Is final Keyword In Java?
- What Is finally Keyword In Java?
- What Is finalize Keyword In Java?
- When To Use Which Keyword In Java?
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding The extends Keyword In Java?
- Use Of extends Keyword In Java
- Using Java extends To Implement Single Inheritance
- Using Java extends With Interfaces (Default Methods)
- Overriding Using extends Keyword In Java
- Difference Between extends And implements In Java
- Real World Applications Of Extends Keyword In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Decision Making Statement In Java?
- If Statement In Java
- If-Else Statement In Java
- Else-If Ladder In Java
- Switch Statement In Java
- Ternary/Conditional Operator (?:) In Java
- Best Practices For Writing Decision Making Statements In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Are Control Statements in Java?
- Decision-Making Control Statements In Java
- Looping Control Statements In Java
- Jump (Branching) Control Statements In Java
- Application Of Control Statements In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is The Break Statement In Java?
- Working Of The Break Statement In Java
- Using Java Break Statement With Loops
- Using Java Break Statement With Switch Statement
- Using Java Break Statement With Infinite Loops
- Common Pitfalls While Using Break Statements In Java
- Best Practices For Using The Break Statement In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Switch Statement In Java?
- Working Of The Switch Statement In Java
- Example Of Switch Statement In Java
- Java Switch Statement With String
- Java Nested Switch Statements
- Java Enum In Switch Statement
- Java Wrapper Classes In Switch Statements
- Uses Of Switch Statement In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Syntax Of main() Method In Java
- public Specifier – Main Method In Java
- static Keyword – Main Method In Java
- void Return Type Of Main Method In Java
- The main Identifier – Main Method In Java
- String[] args In Main Method In Java
- The Role Of Java Virtual Machine (JVM)
- Running Java Programs Without The Main Method
- Variations In Declaration Of Main Method In Java
- Overloading The Main Method In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Method Overriding In Java?
- Example Of Method Overriding In Java
- Ideal Use Cases Of Method Overriding In Java
- Rules For Method Overriding In Java
- Super Keyword & Method Overriding In Java
- Constructor & Method Overriding In Java
- Exception Handling In Method Overriding In Java
- Access Modifiers In Method Overriding In Java
- Advantages & Disadvantages Of Method Overriding In Java
- Difference Between Method Overloading Vs. Method Overriding In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Method Overloading In Java?
- Different Ways Of Method Overloading In Java
- Overloading The main() Method In Java
- Type Promotion & Method Overloading In Java
- Null Error & Method Overloading In Java
- Advantages Of Method Overloading In Java
- Disadvantages Of Method Overloading In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Difference Between Overloading And Overriding In Java (Comparison Table)
- What Is Method Overloading In Java?
- What Is Method Overriding In Java?
- Key Differences Between Overloading & Overriding In Java Explained
- Difference Between Overloading And Overriding In Java Code Example
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is A One-Dimensional Array In Java?
- Key Characteristics Of One-Dimensional Arrays In Java
- Declaration Of One-Dimensional Array In Java
- Initialization Of One-Dimensional Array In Java
- Common Operations On One-Dimensional Array In Java
- Advantages Of One-Dimensional Arrays In Java
- Disadvantages Of One-Dimensional Arrays In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is A Multidimensional Array In Java?
- Difference Between Single-Dimensional And Multidimensional Arrays In Java
- Declaring Multidimensional Arrays In Java
- Initializing Multidimensional Arrays In Java
- Accessing And Manipulating Elements In Multidimensional Arrays In Java
- Working Of Multidimensional Arrays With Jagged Arrays In Java
- Why Use Multidimensional Arrays In Java?
- Limitations Of Multidimensional Arrays In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Are Jagged Arrays In Java?
- Comparison With Regular Multi-Dimensional Arrays
- Declaring Jagged Arrays In Java
- Initialization Of Jagged Arrays In Java
- Printing Elements Of A Jagged Array In Java
- Accessing And Modifying Elements Of A Jagged Array In Java
- Advantages Of Jagged Arrays In Java
- Disadvantages Of Jagged Arrays In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Array Of Objects In Java?
- Declare And Initialize An Array Of Object In Java
- Example Of An Array Of Objects In Java
- Sorting An Array Of Objects In Java
- Passing Arrays Of Objects To Methods In Java
- Returning Arrays Of Objects From Methods In Java
- Advantages Of Arrays Of Objects In Java
- Disadvantages Of Arrays Of Objects In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is A Dynamic Array In Java?
- Why Use Dynamic Array In Java?
- What Is The Size And Capacity Of A Dynamic Array In Java?
- How To Create A Dynamic Array In Java?
- Managing Dynamic Data Input In Java
- Storing And Processing Real-Time Data In Java
- Use Cases Of Dynamic Arrays In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Why Return An Array In Java?
- How To Return An Array In Java
- Example 1: Returning An Array Of First N Squares
- Example 2: Doubling the Values of an Array
- Common Scenarios For Returning Arrays In Java
- Points To Remember When Returning Arrays In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding ArrayList In Java
- Differences Between Arrays And ArrayList In Java
- Returning An ArrayList In Java
- Common Use Cases For Returning An ArrayList In Java
- Pitfalls To Avoid When Returning An ArrayList In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is A Thread In Java?
- Thread Vs Process
- What is a Thread Life Cycle In Java?
- What Are Thread Priorities?
- Creating Threads In Java
- Java Thread Methods
- Commonly Used Constructors In Thread Class
- Thread Synchronization In Java
- Common Challenges Faced While Using Threads In Java
- Best Practices For Using Threads In Java
- Real-World Applications Of Threads In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding Multithreading In Java
- Methods Of Multithreading In Java (Examples)
- Difference Between Multithreading And Multitasking In Java
- Handling Exceptions In Multithreading
- Best Practices For Multithreading In Java
- Real-World Use Cases of Multithreading In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Thread Priority In Java?
- Built-In Thread Priority Constants In Java
- Thread Priority: Setter & Getter Methods
- Limitations Of Thread Priority In Java
- Best Practices For Using Thread Priority In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Thread Synchronization In Java?
- The Need For Thread Synchronization In Java
- Types Of Thread Synchronization In Java
- Mutual Exclusion In Thread Synchronization In Java
- Coordination Synchronization (Thread Communication) In Java
- Advantages Of Thread Synchronization In Java
- Disadvantages Of Thread Synchronization In Java
- Alternatives To Synchronization In Java
- Deadlock And Thread Synchronization In Java
- Real-World Use Cases Of Thread Synchronization In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is A Daemon Thread In Java?
- User Threads Vs. Daemon Threads In Java
- Methods For Daemon Threads In The Thread Class
- Creating Daemon Threads In Java
- Checking The Daemon Status Of A Thread
- Exceptions In Daemon Threads
- Limitations Of Daemon Threads In Java
- Practical Applications Of Daemon Threads In Java
- Common Mistakes To Avoid When Working With Daemon Threads In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Why Do Threads Need To Communicate?
- Understanding Inter Thread Communication In Java
- The wait() Method In Inter-Thread Communication
- The notify() Method In Inter-Thread Communication
- The notifyAll() Method In Inter-Thread Communication
- Difference Between wait() And sleep() Methods In Java
- Best Practices For Inter Thread Communication In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding The Factorial Concept
- Approaches To Implementing Factorial In Java
- Find Factorial In Java Using Iterative Approach (Using a Loop)
- Find Factorial In Java Using Recursive Approach
- Complexity Analysis Of Factorial Programs In Java
- Applications Of Factorial Program In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding The Leap Year Concept
- Approach To Check A Leap Year In Java
- Alternative Approach To Check A Leap Year In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is The Difference Between JDK, JRE, and JVM?
- What Is JVM (Java Virtual Machine)?
- What Is JRE (Java Runtime Environment)?
- What Is JDK (Java Development Kit)?
- Understanding The Difference Between JDK, JRE, And JVM
- Comparison Table For Difference Between JDK, JRE, And JVM
- Conclusion
- Frequently Asked Questions
Table of content:
- Difference Between Abstraction And Encapsulation In Java
- Understanding Abstraction In Java
- Understanding Encapsulation In Java
- When To Use Abstraction And Encapsulation?
- Conclusion
- Frequently Asked Questions
Table of content:
- Differences Between Abstract Class And Interface In Java
- What Is An Abstract Class In Java?
- What Is An Interface In Java?
- When To Use An Abstract Class?
- When To Use Interface?
- Compatibility Between Abstract Class And Interface In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Error Vs. Exception In Java
- What Is Error In Java?
- What Is Exception In Java?
- Best Practices For Handling Exceptions In Java
- Why Errors Should Not Be Handled In Java?
- Conclusion
- Frequently Asked Questions
Table of content:
- Key Differences: Java Vs. JavaScript
- What Is Java?
- What Is JavaScript?
- Difference Between Java And JavaScript Explained
- Conclusion
- Frequently Asked Questions
Table of content:
- Brief Introduction To C++
- Brief Introduction To Java
- Difference Between C++ and Java
- Overview & Features Of C++ Language
- Overview & Features of Java Language
- Example of C++ and Java Program
- Key Difference Between C++ And Java Explained
- Similarities Between Java Vs. C++
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- Basic Java interview questions and answers
- Intermediate Java interview questions and answers
- Advanced Java interview questions and answers
Table of content:
- Difference between core Java and advanced Java
- Important Core Java Questions
- Tips for Preparing for Core Java
Extends Keyword In Java | Syntax, Applications & More (+Examples)
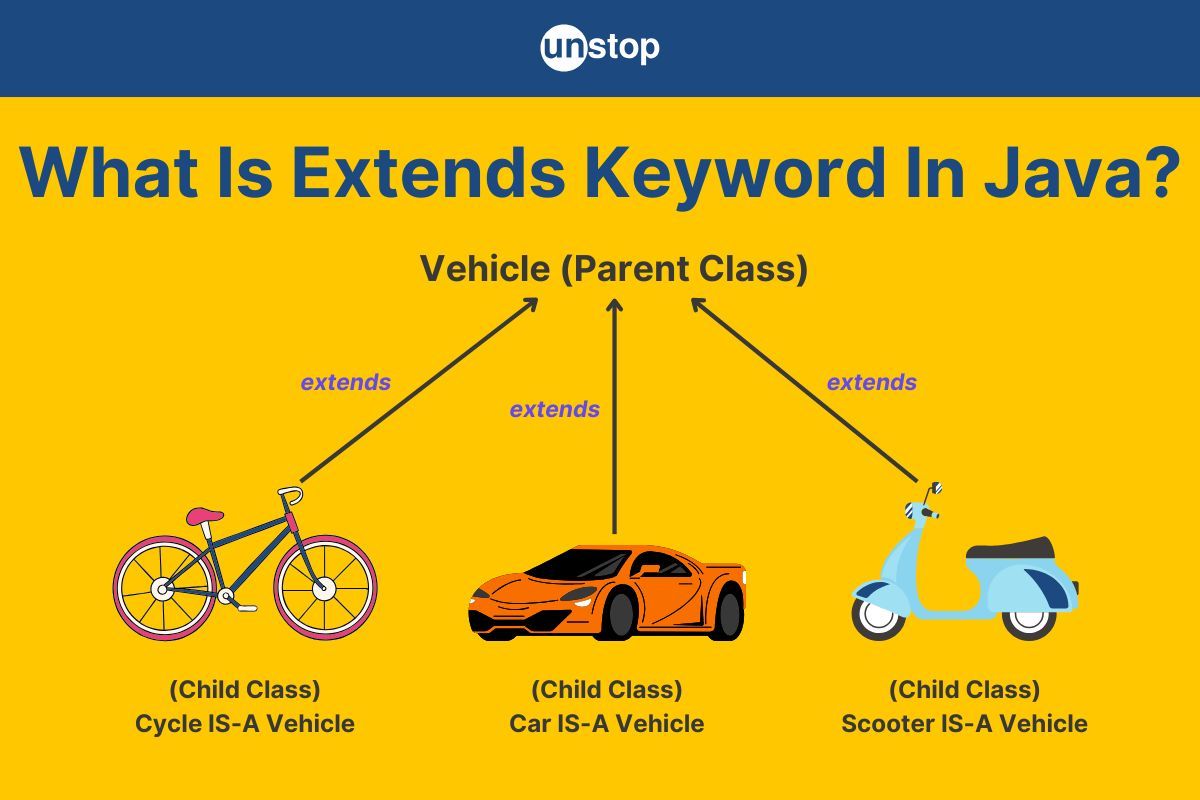
In Java, inheritance is a powerful concept that allows one class to acquire the properties and methods of another. At the heart of this lies the extends keyword, which plays a crucial role in defining relationships between classes. By using extends, we can create a subclass that inherits from a parent class, promoting code reuse and modularity.
In this article, we'll explore the extends keyword in Java, its syntax, usage, and significance in object-oriented programming. From basic examples to advanced scenarios, we will uncover how extends enhances code structure and flexibility while adhering to the principles of inheritance.
Understanding The extends Keyword In Java?
The extends keyword in Java programming is used to establish inheritance between two classes. When a class uses extends, it means it is inheriting (or "borrowing") properties and methods from another class, called the parent or superclass. This allows the new class, called the child or subclass, to reuse existing code and add its own features.
Real-Life Analogy:
Imagine a general blueprint for a car. This blueprint defines common features like wheels, engine, and seats. Now, if you want to design a sports car, you don't need to start from scratch. You can extend the car's blueprint to inherit its features and add new ones, like a turbo engine or a sporty design.
Thus:
- The general car blueprint is the superclass.
- The sports car blueprint is the subclass that extends the superclass.
Syntax Of extends Keyword In Java
class Superclass {
// Fields and methods of the superclass
}class Subclass extends Superclass {
// Additional fields and methods of the subclass
}
Here:
- Extends: It establishes an inheritance relationship between two classes. Indicates that the subclass is inheriting from the superclass.
- Superclass: The base class that provides common features to be inherited.
- Subclass: The derived class that inherits from the superclass using extends.
Use Of extends Keyword In Java
The extends keyword in Java is primarily used to create an inheritance relationship between two classes or interfaces. It allows one class or interface to inherit the properties and methods of another, promoting code reuse and enhancing modularity. Some of the key uses of the extends keyword in Java are as follows:
- Single Inheritance (Class Inheritance): A class can inherit from another class using the extends keyword. This allows the derived (child) class to reuse the code in the base (parent) class. Java only supports single inheritance for classes, meaning one class can extend only one other class directly.
- Multiple Inheritance through Interfaces: Interfaces can extend multiple interfaces using the extends keyword, allowing for multiple inheritance of behavior (methods). This helps in achieving multiple inheritance in Java (which is not possible with classes directly).
- Method Overriding: When a subclass inherits a method from the superclass, it can override that method to provide a more specific implementation. The extends keyword establishes the inheritance link, making overriding possible.
Using Java extends To Implement Single Inheritance
Single Inheritance occurs when one class (the derived or child class) inherits from another class (the base or parent class). The extends keyword in Java is used to establish this relationship, allowing the child class to access the properties and methods of the parent class.
Code Example:
// Base Class (Parent)
class Animal {
void eat() {
System.out.println("This animal eats food.");
}
}
// Derived Class (Child)
class Dog extends Animal {
void bark() {
System.out.println("The dog barks.");
}
}
public class Main {
public static void main(String[] args) {
Dog myDog = new Dog(); // Create an object of the derived class
// Calling inherited method from the base class
myDog.eat();
// Calling method from the derived class
myDog.bark();
}
}
Output:
This animal eats food.
The dog barks.
Explanation:
In the above code example-
- We start by defining a base class Animal that represents a generic parent class with a method eat() that outputs "This animal eats food."
- Then, we create a derived class Dog that extends the Animal class using the extends keyword. This establishes an inheritance relationship.
- In the Dog class, we add a new method bark() that outputs "The dog barks." This method is specific to the Dog class.
- Inside the Main class, we define the main() method, which is the entry point of our program.
- We then create an object myDog of the Dog class, which allows us to access methods from both the Dog class and the inherited methods from the Animal class.
- By calling myDog.eat(), we invoke the eat() method from the base Animal class, showcasing inheritance.
- By calling myDog.bark(), we invoke the bark() method specific to the Dog class, demonstrating the addition of child-specific behavior.
Explore this amazing course and master all the key concepts of Java programming effortlessly!
Using Java extends With Interfaces (Default Methods)
In Java, interfaces allow you to define abstract behavior that classes can implement. Starting from Java 8, interfaces can also have default methods, which are methods with a default implementation. This allows you to provide common functionality across multiple classes without requiring them to implement the method themselves.
The extends keyword is used to allow one interface to inherit from another interface, and interfaces can also extend other interfaces. With default methods, interfaces can provide a default behavior, while still allowing implementing classes to override or extend that behavior.
Key Points To Remember:
- Interfaces can inherit from other interfaces using extends.
- Default methods allow interfaces to provide method implementations, which can be optionally overridden by classes.
- Multiple interfaces can be extended, and default methods help avoid conflicts when the same method is defined in multiple interfaces.
Code Example:
// Base Interface with a Default Method
interface Animal {
// Default method
default void eat() {
System.out.println("This animal eats food.");
}
}
// Another Interface extending the first one, with a Default Method
interface Pet extends Animal {
// Default method
default void play() {
System.out.println("This pet loves to play.");
}
}
// Derived Class implementing both interfaces
class Dog implements Pet {
// Override the default method from Pet (which comes from Animal)
@Override
public void eat() {
System.out.println("The dog eats dog food.");
}
// Can use the default play() method from Pet or override it
}
public class Main {
public static void main(String[] args) {
Dog myDog = new Dog(); // Create an object of the Dog class
// Calling the overridden method from the Dog class
myDog.eat();
// Calling the default method from Pet interface
myDog.play();
}
}
Output:
The dog eats dog food.
This pet loves to play.
Explanation:
In the above code example-
- We start with an interface Animal, which defines a default method eat(). This method outputs "This animal eats food." By using the default keyword, we allow the method to have a body, meaning it can be inherited by any class that implements this interface without needing to override it.
- We then define another interface Pet that extends the Animal interface using the extends keyword. This means that Pet inherits all methods from Animal, including the default eat() method. In addition, we add a new default method play() in the Pet interface, which outputs "This pet loves to play."
- Next, we create the Dog class that implements the Pet interface. By implementing the Pet interface, Dog must either override the default methods from Pet (and Animal) or use them as they are.
- In the Dog class, we choose to override the eat() method. Thus eat() outputs "The dog eats dog food." This overrides the inherited eat() method from the Animal interface, providing specific behavior for the Dog class.
- The Dog class doesn't need to override the play() method from the Pet interface because it can use the default implementation provided by the Pet interface. The method outputs "This pet loves to play." If we wanted to, we could override this method in the Dog class to give it a specific implementation.
- In the Main class, we create an object myDog of type Dog, which allows us to call methods defined in the Dog class as well as those inherited from the interfaces.
- By calling myDog.eat(), we invoke the overridden version of eat() in the Dog class, which outputs "The dog eats dog food."
- By calling myDog.play(), we invoke the default play() method from the Pet interface, which outputs "This pet loves to play."
Overriding Using extends Keyword In Java
Method overriding is a feature in Java that allows a subclass to provide a specific implementation for a method that is already defined in its superclass. This is done using the extends keyword to create an inheritance relationship, allowing the subclass to "override" the inherited method.
When a subclass overrides a method, it provides a new version of the method that matches the method signature of the parent class. Overriding is an essential concept in polymorphism and allows the behavior of inherited methods to be customized.
Code Example:
// Parent Class (Superclass)
class Animal {
void makeSound() {
System.out.println("The animal makes a sound.");
}
}
// Child Class (Subclass)
class Dog extends Animal {
// Overriding the makeSound() method
@Override
void makeSound() {
System.out.println("The dog barks.");
}
}
public class Main {
public static void main(String[] args) {
Animal myAnimal = new Animal();
myAnimal.makeSound(); // Calls Animal's method
Dog myDog = new Dog();
myDog.makeSound(); // Calls Dog's overridden method
}
}
Output:
The animal makes a sound.
The dog barks.
Explanation:
In the above code example-
- We start with a parent class Animal, which contains a method makeSound(). This method outputs "The animal makes a sound." It represents a general behavior for animals in the Animal class.
- We then create a child class Dog, which extends the Animal class using the extends keyword. This establishes an inheritance relationship where the Dog class inherits the makeSound() method from Animal.
- In the Dog class, we override the makeSound() method to provide a specific implementation for dogs. The overridden method outputs "The dog barks," which is more appropriate for a dog compared to the general message in the Animal class.
- In the Main class, we create two objects: myAnimal of type Animal and myDog of type Dog.
- When we call myAnimal.makeSound(), the program uses the method from the Animal class, which outputs "The animal makes a sound."
- When we call myDog.makeSound(), the program uses the overridden method from the Dog class, which outputs "The dog barks." This demonstrates how a subclass can modify or specialize the behavior inherited from the superclass.
Sharpen your coding skills with Unstop's 100-Day Coding Sprint and compete now for a top spot on the leaderboard!
Difference Between extends And implements In Java
Both extends and implements are used to create relationships between classes and interfaces, but they serve different purposes and are applied in different contexts. Here are the key differences between the two:
Aspect |
Java extends |
Java implements |
Purpose |
Used for inheritance — to establish a relationship between a class and another class or between an interface and another interface. |
Used by a class to implement an interface, meaning the class agrees to provide concrete implementations for the abstract methods defined in the interface. |
Used By |
- A class can extend another class. - An interface can extend another interface. |
- A class implements one or more interfaces. |
Multiple Inheritance |
No (A class can only extend one class due to single inheritance). |
Yes (A class can implement multiple interfaces, which allows multiple inheritance). |
Inheritance Type |
- Class to class: A subclass inherits fields and methods from its superclass. - Interface to interface: An interface inherits method signatures from another interface. |
A class must implement all methods declared in the interface. |
Method Implementation |
Inherits methods from the superclass. If the method is abstract in the superclass, the subclass must provide an implementation. |
Must provide implementations for all abstract methods defined in the interface. |
Fields Inherited |
A class inherits fields (variables) from its superclass. The subclass can access them if they are public or protected. |
A class does not inherit fields from an interface. It only implements the method signatures defined in the interface. |
Constructor Inheritance |
A subclass can inherit constructors from its superclass (indirectly, using super). |
No constructors are inherited. A class implementing an interface does not inherit constructors from the interface. |
Abstract Methods |
- A subclass inherits abstract methods if the superclass has abstract methods. - The subclass must provide an implementation (unless the subclass is also abstract). |
A class must provide concrete implementations for all abstract methods of the interface. |
Access Modifiers for Methods |
A subclass can override the methods of its superclass, but the access modifier of the overridden method cannot be more restrictive than the method in the superclass. |
A class implementing an interface can implement the interface's methods with any access modifier, but it must provide implementations for all abstract methods. |
Class vs Interface Relationship |
Establishes a relationship between a class and its superclass (or subclass). |
Establishes a relationship between a class and an interface. |
Type of Inheritance |
Single inheritance for classes, but multiple inheritance for interfaces. |
Multiple inheritance (A class can implement multiple interfaces). |
Example |
class Animal { } |
interface Animal { class Dog implements Animal { |
Abstract Methods in Superclass/Interface |
If the superclass has abstract methods, the subclass must implement them. |
A class must implement all abstract methods of an interface. |
Default Methods |
Not applicable. A class extending another class cannot directly inherit default methods. |
An interface can have default methods with implementations, and classes implementing the interface can inherit them. |
Supports Method Overriding |
A subclass can override methods from the superclass. |
A class can override methods from an interface (implementations of the interface's methods). |
Real-World Analogy |
Like a child inheriting traits (behavior) from their parents. |
Like a worker following the rules set by an organization (the interface), but also bringing their own work skills (method implementations). |
Therefore:
- Java extends is used to create a subclass from a superclass (inheritance), where the subclass inherits both fields and methods from the superclass.
- Java implements is used when a class adopts the behavior of an interface and provides concrete implementations for its abstract methods.
Are you looking for someone to answer all your programming-related queries? Let's find the perfect mentor here.
Real World Applications Of Extends Keyword In Java
Below are some real-world scenarios where the extends keyword is commonly used in Java language:
1. Building a Hierarchical System (Employee Management System)
In an Employee Management System, we might have a general class Employee that is extended by more specialized classes like Manager, Developer, and Intern. The Manager class might have extra functionality, like the ability to assign tasks, while the Developer and Intern classes have different specific methods. For Example-
// Base Class
class Employee {
void work() {
System.out.println("Employee is working.");
}
}// Derived Class
class Manager extends Employee {
void assignTask() {
System.out.println("Manager is assigning tasks.");
}
}
2. Vehicle Inheritance (Vehicle Management System)
In a Vehicle Management System, the extends keyword can model different types of vehicles like Car, Truck, and Motorcycle by creating a base class Vehicle and extending it for each type of vehicle. Each vehicle class may have specific methods related to its type. For Example-
// Base Class
class Vehicle {
void start() {
System.out.println("Vehicle is starting.");
}
}// Derived Class
class Car extends Vehicle {
void honk() {
System.out.println("Car is honking.");
}
}
3. Banking System (Account Types)
In a Banking System, we can use inheritance to define various account types like SavingsAccount, CheckingAccount, or BusinessAccount. These subclasses can extend a base class BankAccount that holds common properties and methods for all account types. For Example-
// Base Class
class BankAccount {
void deposit(double amount) {
System.out.println("Depositing: " + amount);
}
}// Derived Class
class SavingsAccount extends BankAccount {
void applyInterest() {
System.out.println("Applying interest to savings account.");
}
}
4. E-commerce System (Product Categories)
In an E-commerce System, different product categories can extend a base class Product. For example, Electronics, Clothing, and Books can be subclasses that have specific details, like warranty for electronics or size for clothing. For Example-
// Base Class
class Product {
void displayProductInfo() {
System.out.println("Product information.");
}
}// Derived Class
class Electronics extends Product {
void displayWarranty() {
System.out.println("Displaying warranty details.");
}
}
5. Game Development (Character Types)
In Game Development, we often need to model different types of characters, such as Warrior, Mage, and Archer, which all inherit from a common base class Character. Each class can have specific abilities or stats, but they all share common actions like attack() or move(). For Example-
// Base Class
class Character {
void move() {
System.out.println("Character is moving.");
}
}// Derived Class
class Warrior extends Character {
void useSword() {
System.out.println("Warrior is using a sword.");
}
}
Conclusion
The extends keyword in Java is a powerful tool that enables classes to inherit properties and methods from other classes, promoting code reuse and simplifying software design. Whether you're working with single or multilevel inheritance, abstract classes, or interfaces, extends plays a crucial role in building flexible and maintainable code.
However, it's important to use it wisely, keeping in mind best practices like avoiding deep inheritance hierarchies and knowing when to prefer composition over inheritance. By understanding and applying the extends keyword effectively, you can enhance your object-oriented programming skills and create more efficient, scalable Java applications.
Frequently Asked Questions
Q. What does the extends keyword do in Java?
The extends keyword in Java is used to create a subclass (child class) from a superclass (parent class), enabling the subclass to inherit methods and fields from the superclass. It allows for code reuse, making it easier to create a new class that builds upon an existing class, while adding or overriding functionalities. For example, if you have a Vehicle class, you can create a Car class that extends Vehicle, inheriting its properties and methods.
Q. Can a class extend more than one class in Java?
No, Java does not support multiple inheritance for classes. A class can only extend one other class at a time. This means that a class can inherit from only one superclass. However, Java allows multiple inheritance through interfaces. A class can implement multiple interfaces, thus achieving some benefits of multiple inheritance, but with more flexibility and less ambiguity.
Q. How does the extends keyword work with abstract classes?
When a class extends an abstract class, it inherits the abstract class's fields and methods, but it must implement all of the abstract methods defined in the parent class (unless the subclass is also abstract). The subclass is required to provide concrete implementations for these abstract methods to become a fully functional class. This makes abstract classes useful for creating a blueprint that must be followed by subclasses.
Q. What is the difference between extends and implements in Java?
The difference between them is as follows:
- extends: Used when a class inherits from another class (either concrete or abstract). A class can only extend one class, and the subclass inherits the methods and fields of the superclass.
- implements: Used when a class implements an interface. A class can implement multiple interfaces, and it must provide concrete implementations for all methods declared in the interface. Interfaces are used to define a contract that a class must fulfill, but they do not provide method implementations.
Q. Can a subclass call a constructor of the superclass using extends?
Yes, a subclass can call a constructor from its superclass using the super() keyword. This is particularly useful when the superclass has a parameterized constructor. If the superclass does not have a default constructor (i.e., no constructor with no parameters), the subclass must explicitly call one of the superclass's constructors using super(). If the superclass has a no-argument constructor, it is automatically called, unless another constructor is explicitly invoked in the subclass.
Q. What happens if a subclass does not override a method from the superclass?
If a subclass does not override a method from the superclass, the subclass inherits the method as it is from the superclass. This means that the subclass will use the version of the method provided by the superclass, unless it needs to be customized by overriding it. If a method is not overridden and it is called on an object of the subclass, the superclass's version of the method will be executed.
With this, we conclude our discussion on the extends keyword in Java. Here are a few other topics that you might be interested in reading:
- Convert String To Date In Java | 3 Different Ways With Examples
- Final, Finally & Finalize In Java | 15+ Differences With Examples
- Throws Keyword In Java | Syntax, Working, Uses & More (+Examples)
- How To Find LCM Of Two Numbers In Java? Simplified With Examples
- How To Find GCD Of Two Numbers In Java? All Methods With Examples
- Volatile Keyword In Java | Syntax, Working, Uses & More (+Examples)
I’m a Computer Science graduate with a knack for creative ventures. Through content at Unstop, I am trying to simplify complex tech concepts and make them fun. When I’m not decoding tech jargon, you’ll find me indulging in great food and then burning it out at the gym.
Comments
Add commentLogin to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
Esuru Pooja.C 2 hours ago
Sunita Bhat 2 days ago
Ankita Das 2 days ago
Mihir Kumar Ranjan 1 week ago