Control Statements In Java | Types & Applications (+Code Examples)
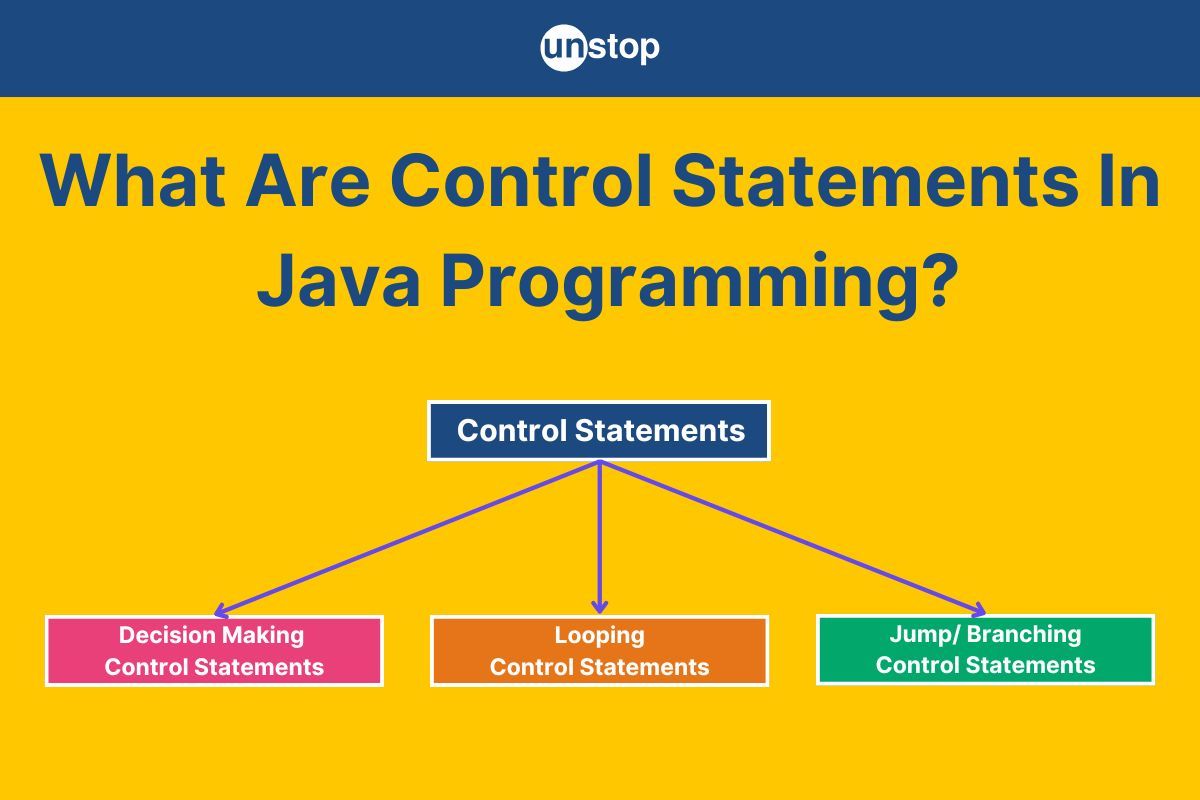
Control statements in Java are the backbone of decision-making and flow control within a program. They enable us to dictate how the program executes, allowing it to respond dynamically to various conditions. By using control statements, we can direct the program's execution path, either through decisions, loops, or jumps, making our applications more robust and versatile.
In this article, we’ll explore the different types of control statements in Java, including decision-making statements like if, else, and switch, looping constructs such as for, while, and do-while, and jump statements like break, continue, and return. Each section will explore their syntax, use cases, and practical examples to help you master the art of controlling program flow in Java effectively.
What Are Control Statements in Java?
Control statements in Java programming are the building blocks of any program that dictate the flow of execution. They enable us to control how, when, and under what conditions different parts of the program are executed. By utilizing control statements, we can make decisions, repeat actions, or execute specific parts of the code based on certain conditions.
Types Of Control Structures In Java
Control statements in Java can be categorized into three main types:
- Decision-Making Statements
- It allows the program to choose between different paths based on a condition.
- Examples: if, if-else, if-else-if, switch
- Looping Statements
- It enables the program to execute a block of code multiple times as long as a condition is met.
- Examples: for, while, do-while
- Jump/Branching Statements
- It controls the flow by altering the normal sequence of execution.
- Examples: break, continue, return
Real-Life Example
Let’s relate control statements in Java to something we do daily, i.e. planning your morning routine:
- Decision-Making (if-else): You wake up and check the time-
- If it’s early, you decide to make a full breakfast.
- Else, you grab a quick snack to avoid being late.
- Looping (for/while): You prepare your lunch-
- You repeatedly pack items (sandwich, fruit, drink) until your lunchbox is full.
- Branching (break/continue): While walking to work, you meet a friend-
- If you’re running late, you quickly say goodbye and continue walking.
- If you’re early, you stop to chat (break your walk briefly).
Now that we have understood how the control statements in Java work, let's discuss their types in detail in the sections ahead.
Explore this amazing course and master all the key concepts of Java programming effortlessly!
Decision-Making Control Statements In Java
Decision-making statements in Java allow the program to choose a path of execution based on conditions. They enable conditional logic, where different blocks of code are executed depending on whether certain conditions are true or false.
Types of Decision-Making Statements in Java
- If Statement: Executes a block of code if the condition evaluates to true.
- If-else Statement: Executes one block of code if the condition is true, and another block if the condition is false.
- If-else-if Ladder: Checks multiple conditions in sequence; the first true condition's block is executed.
- Switch Statement: Allows execution of different blocks of code based on the value of a single variable or expression.
Let’s imagine you're deciding what to wear based on the weather and temperature-
Code Example:
Output (set code file name as DecisionMakingExample.java):
Wear casual clothing and sunglasses.
Don't forget sunscreen!
Explanation:
In the above code example-
- First, we define a class DecisionMakingExample, which contains the main() method. This is where our program starts execution.
- Inside the main() method, we declare and initialize two variables:
- First, weather is a String variable with a value of "sunny", representing current weather conditions. It can take values such as "sunny", "rainy", or "cloudy".
- Second, temperature is an int variable set to 25, representing the temperature in degrees Celsius.
- We then use an if-else structure to handle decision-making based on the weather and temperature values. If weather equals "rainy", we further check the temperature:
- If the temperature is less than 20, we print the message "Wear a raincoat and carry an umbrella.", indicating the need for both warm and rain-protective gear.
- Otherwise, if the temperature is 20 or higher, we print "Carry an umbrella and wear light clothing.", suggesting lighter attire while still preparing for rain.
- If weather equals "sunny", we again evaluate the temperature:
- If the temperature is greater than 30, we print "Wear light clothing and sunglasses.", highlighting the need to stay cool in hot weather.
- Otherwise, for temperatures 30 or below, we print "Wear casual clothing and sunglasses.", as the weather is sunny but not extremely hot.
- If weather equals "cloudy", we print "Carry a jacket just in case.", anticipating the possibility of cooler or unpredictable weather.
- If weather doesn’t match any of these values, we print "Weather unknown, dress comfortably!", a default fallback message for unknown conditions.
- After the if-else structure, we use a switch statement to provide additional advice based solely on the weather:
- For "sunny", we print "Don't forget sunscreen!", a reminder to protect ourselves from UV rays.
- For "rainy", we print "Avoid slippery paths.", encouraging safety during wet conditions.
- For "cloudy", we print "Keep an eye on the sky for rain.", suggesting vigilance for potential rain.
- For any other value of weather, the default case prints "Stay prepared for any situation.", a general advice statement for uncertain conditions.
Looping Control Statements In Java
Looping control statements in Java are used to execute a block of code repeatedly, as long as a specified condition is met. These statements are essential for automating repetitive tasks, processing data, or iterating through collections.
Types Of Looping Control Statements In Java
The different types of looping control statements in java are:
- For Loop: It is used when the number of iterations is known beforehand. It’s syntax is as follows:
for (initialization; condition; update) {
// Code block to be executed
}
- While Loop: It executes a block of code as long as the condition remains true. The condition is checked before each iteration. It’s syntax is as follows:
while (condition) {
// Code block to be executed
}
- Do-while Loop: Similar to the while loop, but the code block is executed at least once, as the condition is checked after execution. It’s syntax is as follows:
do {
// Code block to be executed
} while (condition);
Let’s demonstrate all three types of loops by calculating the sum of the first n natural numbers-
Code Example:
Output (set code file name as LoopingExample.java):
Sum using for loop: 15
Sum using while loop: 15
Sum using do-while loop: 15
Explanation:
In the above code example-
- We start by defining a class LoopingExample, which contains the main() method. This is where the execution of our program begins.
- Inside the main() method, we declare and initialize an integer variable n with the value 5. This represents the number of natural numbers we want to sum, i.e., 1 + 2 + 3 + 4 + 5.
- We calculate the sum using three different loops: a for loop, a while loop, and a do-while loop.
- First, we use the for loop:
- We initialize an integer variable sumFor to 0 to store the running total of the sum.
- The loop starts with i = 1 and runs while i is less than or equal to n. In each iteration, we add i to sumFor and increment i.
- When the loop completes, sumFor contains the total sum of the first 5 natural numbers.
- We then print the result with the message "Sum using for loop: ", followed by the value of sumFor.
- Next, we use the while loop:
- We initialize an integer variable sumWhile to 0 to store the running total and a separate variable i to 1 to act as the loop counter.
- The loop continues to execute as long as i is less than or equal to n. Inside the loop, we add i to sumWhile and increment i by 1.
- After the loop finishes, sumWhile holds the total sum.
- We print the result with the message "Sum using while loop: ", followed by the value of sumWhile.
- Finally, we use the do-while loop:
- We initialize another variable sumDoWhile to 0 for the running total and a loop counter j to 1.
- Unlike the while loop, the do-while loop ensures that the body of the loop executes at least once, even if the condition is false.
- Inside the loop, we add j to sumDoWhile and increment j by 1. The loop condition is checked after each iteration and continues as long as j is less than or equal to n.
- When the loop ends, sumDoWhile contains the total sum.
- We print the result with the message "Sum using do-while loop: ", followed by the value of sumDoWhile.
Sharpen your coding skills with Unstop's 100-Day Coding Sprint and compete now for a top spot on the leaderboard!
Jump (Branching) Control Statements In Java
Jump or branching control statements in Java are used to alter the flow of execution by transferring control to another part of the program. These statements can either skip the rest of the current iteration, exit a loop, or terminate the method entirely. They are crucial for controlling program flow dynamically based on conditions.
Types Of Jump/Branching Control Statements In Java:
- Break Statement: It is used to exit a loop or a switch statement prematurely. Stops the execution of the current loop and proceeds to the next statement after the loop.
- Example Use Case: Exit a loop when a specific condition is met.
- Continue Statement: It skips the rest of the code in the current iteration and proceeds with the next iteration of the loop.
- Example Use Case: Skip processing for specific conditions within a loop.
- Return Statement: It exits from the current method and optionally returns a value to the method caller.
- Example Use Case: Stop execution in a method once a result is determined.
Let’s combine these branching statements in a program that processes a list of numbers-
Code Example:
Output (set code file name as BranchingExample.java):
Processing numbers:
Processing 1
2 is even, skipping further operations.
Processing 3
4 is even, skipping further operations.
Processing 5
Negative number encountered, stopping processing.
Sum of first positive numbers:
Result: 15
Explanation:
In the above code example-
- We define a class BranchingExample with a main() method, where the program execution starts.
- Inside the main() method, we initialize an array numbers with a mix of positive integers and one negative number (-1). This array serves as the input for processing.
- We start processing the numbers using a for-each loop that iterates over each element in the numbers array:
- First, we check if the current number (num) is negative using if (num < 0). If true, we print a message indicating that a negative number has been encountered and stop the loop using the break statement. This ensures no further numbers are processed after a negative value.
- If the number is even, determined by the condition num % 2 == 0, we print a message stating that it is even and skip further processing for that iteration using the continue statement. The loop moves to the next number in the array without executing the remaining code for the current iteration.
- For all other cases (odd and non-negative numbers), we print a message stating that the number is being processed. This is where the actual processing logic would go.
- After completing the loop, we move on to calculate the sum of the positive numbers in the array until a negative number is encountered.
- We call the method calculateSum(numbers) and print the result with the message "Sum of first positive numbers:". The calculateSum method works as follows:
- We initialize a variable sum to 0 to store the running total of positive numbers.
- We use a for-each loop to iterate through the numbers array.
- If a negative number is found (num < 0), we immediately return the current sum using the return statement, stopping further calculations.
- For each positive number, we add it to sum. If no negative number is encountered, the loop completes, and the method returns the total sum of all the numbers in the array.
- The combined logic in the main() method and calculateSum ensures we efficiently process the numbers and handle cases like encountering negative numbers or skipping specific conditions (e.g., even numbers).
Are you looking for someone to answer all your programming-related queries? Let's find the perfect mentor here.
Application Of Control Statements In Java
Here are some key applications of control statements in Java:
- User Authentication and Authorization: Control statements like if-else are used in authentication systems to verify user credentials. When users enter their credentials, the system checks if the input matches the stored values. If the credentials are valid, the user is granted access; otherwise, they are denied access. For Example-
if (enteredUsername.equals(storedUsername) && enteredPassword.equals(storedPassword)) {
System.out.println("Login successful.");
} else {
System.out.println("Invalid credentials.");
}
- Menu-Driven Programs: Control statements such as switch are used in menu-driven applications where users choose from a set of predefined options. The program executes specific code depending on the user's selection. For Example-
switch (choice) {
case 1: // Addition
break;
case 2: // Subtraction
break;
default:
System.out.println("Invalid choice");
}
- Input Validation: Loops and conditional statements (like while and if) are used to ensure that the user's input meets certain criteria. For example, if the user enters a non-positive number, the system will keep asking until a valid number is entered. For Example-
while (true) {
if (number > 0) {
break;
} else {
System.out.println("Enter a positive number.");
}
}
- Looping through Data (Arrays, Lists): Control statements like for loops are used to iterate through arrays or lists to process or display each element. This is useful in situations like summing values in an array or processing a list of items. For Example-
for (int i = 0; i < numbers.length; i++) {
sum += numbers[i]; // Summing array elements
}
- Search Algorithms: Control statements such as for and if are used in search algorithms (like linear search). The program checks each element in the array or list to find the target value and stops once it is found. For Example-
if (numbers[i] == searchValue) {
found = true;
break; // Exit the loop if the value is found
}
- Game Loops: In game development, loops (especially while loops) are used to continuously update the game state, process user inputs, and display changes until the user chooses to quit the game. For Example-
while (gameRunning) {
if (choice == 1) { // User chooses to play
playGame();
} else if (choice == 2) { // User chooses to quit
gameRunning = false;
}
}
- Billing or Payment Systems: Control statements like if-else are used to apply conditional logic in systems that calculate prices. For example, a discount might be applied based on the total amount, using a simple if condition to check the purchase total. For Example-
if (totalAmount > 200) {
discount = 0.1; // 10% discount for large orders
}
finalAmount = totalAmount - (totalAmount * discount);
- Data Processing (Filtering Data): Control statements such as for loops combined with if conditions are used to filter data based on specific criteria. This is common in applications that need to process large datasets and extract relevant information. For Example-
for (int num : numbers) {
if (num % 2 == 0) {
System.out.println(num); // Print even numbers
}
}
Conclusion
In Java, control statements are crucial building blocks that empower developers to manage the flow of execution within a program. From making decisions with if-else and switch, to controlling repetition through loops (for, while), to handling errors with try-catch, these statements provide flexibility, responsiveness, and efficiency in application development. They enable the program to make dynamic choices, validate user input, process data, and manage exceptions gracefully.
Whether it's for controlling the flow of execution, optimizing performance, or creating interactive and adaptable applications, control statements are essential for writing effective, reliable, and maintainable Java programs. Understanding and utilizing them proficiently is key to mastering Java programming and solving complex problems in real-world applications.
Frequently Asked Questions
Q. Why are control statements important for flow control in Java?
Control statements are fundamental to managing the flow of execution in a program. Without them, the program would execute instructions sequentially without making any decisions or repeating tasks, making it highly inflexible and inefficient. In Java, control statements like if-else, loops, and switch allow the program to:
- Make decisions based on certain conditions.
- Repeat operations multiple times when necessary.
- Skip or jump over code blocks, which makes the program more dynamic.
For example, using an if statement helps a program check whether a condition is met (like if a user is logged in), and if the condition is true, it can take a specific action (like granting access). Similarly, loops allow repetitive actions, and break or continue statements control loop behavior, improving efficiency by minimizing unnecessary steps.
- What is the difference between if and switch statements in Java?
- If statement: The if statement is used to execute a block of code if a specified condition is true. It can be used for checking multiple conditions with if-else and else-if.
- Use case: When you have conditions that aren't limited to a specific set of discrete values (like comparing ranges or multiple variables).
- Switch statement: The switch statement is used to execute one of many possible code blocks based on the value of a single expression. It is typically used when you need to test a variable against multiple possible constant values.
- Use case: When you have a fixed set of possible values for a single variable, and you want a more readable and efficient way of handling them.
For Example:
// if statement
if (score > 90) {
System.out.println("Excellent");
} else if (score > 80) {
System.out.println("Good");
} else {
System.out.println("Needs Improvement");
}// switch statement
switch (day) {
case 1:
System.out.println("Monday");
break;
case 2:
System.out.println("Tuesday");
break;
default:
System.out.println("Invalid Day");
}
Q. What is the purpose of break and continue statements in Java?
- Break statement: The break statement is used to immediately exit from a loop or switch statement. It is typically used when a certain condition is met and there's no need to continue further iterations.
- Use case: Exiting from a loop early when a condition is satisfied (e.g., search for an element in a list and break out of the loop once found).
- Continue statement: The continue statement skips the current iteration of a loop and proceeds with the next iteration. It’s useful when you want to skip over certain steps in a loop.
- Use case: Skipping even numbers in a loop, or continuing a loop when a certain condition isn't met.
Q. How do looping control statements enhance the flexibility of Java programs?
Looping control statements in Java (for, while, and do-while) enhance the flexibility and efficiency of a program by enabling repetitive tasks to be automated. Rather than writing the same code repeatedly, loops allow you to execute a block of code multiple times based on a condition or a set number of iterations.
- Efficiency: Loops reduce redundancy and save lines of code. For example, if you need to perform an operation on each element of a list or array, a loop allows you to iterate through all elements without manually specifying each one.
- Flexibility: Java provides different types of loops, each suited for different situations:
- for loop: Ideal when you know the exact number of iterations.
- while loop: Useful when the number of iterations is unknown and depends on a condition.
- do-while loop: Ensures that the loop body executes at least once, regardless of the condition.
This flexibility is key when processing data, handling user input, or implementing repeated tasks within the program.
Q. How does the do-while loop differ from the while loop in Java?
The do-while loop and while loop in Java both perform repeated actions, but they differ in their execution behavior:
- while loop: The condition is evaluated before each iteration. If the condition is false initially, the body of the loop may not execute at all. This makes it suitable for situations where you want to repeat an action as long as a condition holds true, but the loop should not execute if the condition is false at the outset.
- do-while loop: The condition is evaluated after each iteration. This guarantees that the body of the loop is executed at least once, even if the condition is false from the start. It’s useful when the loop body must be executed at least once, regardless of the condition (e.g., prompting the user for input until they provide a valid answer).
In summary, choose a while loop when the condition needs to be checked before execution and a do-while loop when at least one iteration of the loop is required before evaluating the condition.
Q. How do nested control statements work in Java?
Nested control statements in Java occur when one control statement (like a loop or an if statement) is placed inside another control statement. These are commonly used in situations where a decision depends on multiple conditions or when loops need to perform tasks within other loops.
- Nested if statements: These are used when there are multiple conditions that need to be checked in a hierarchical manner. For example, checking if a number is both positive and even can be handled by nesting an if statement inside another if statement.
- Nested loops: This is used when a task involves performing repeated actions on data structures like 2D arrays or matrices. For example, to iterate through a matrix, a for loop can be nested inside another for loop.
While nesting control statements can provide more powerful logic, it's important to be cautious of creating deeply nested structures, as they can make the code difficult to read and maintain.
With this, we conclude our discussion on the control statements in Java programming language. Here are a few other topics that you might be interested in reading:
- Throws Keyword In Java | Syntax, Working, Uses & More (+Examples)
- Final, Finally & Finalize In Java | 15+ Differences With Examples
- Volatile Keyword In Java | Syntax, Working, Uses & More (+Examples)
- Super Keyword In Java | Definition, Applications & More (+Examples)
- How To Find GCD Of Two Numbers In Java? All Methods With Examples
- How To Find LCM Of Two Numbers In Java? Simplified With Examples
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment