Table of content:
- History Of Java Programming Langauge
- Infographic For History Of Java
- What’s In The Name | History Of Java
- Key Features Of Java
- Advantages And Disadvantages Of Java
- The Version History Of Java Langauge
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is JDK?
- How To Download Java Development Kit (JDK) For Windows, MacOS, and Linux?
- Set Environment Variables In Java
- How To Install Java (JDK) On Windows 64-Bit Machine?
- How To Install Java (JDK) On Linux?
- How To Install Java (JDK) On macOS?
- How To Test Java Installation?
- How To Write Your First Java Program On Linux OS?
- Conclusion
- Frequently Asked Questions
Table of content:
- Java Programming Language | An Introduction
- 15 Key Features Of Java
- Write Once Run Anywhere (WORA) | Features Of Java
- Java Editions
- 5 New Features Of JAVA 8
- 5 New Features Of JAVA 11
- What Makes Java Popular?
- Conclusion
- Frequently Asked Questions
Table of content:
- What is Java?
- Advantages of Java
- Disadvantages of Java
Table of content:
- What Is Java Programming?
- Role Of Integrated Development Environments (IDEs) In Java Development
- 15 Best Java IDE For Developers
- In-Depth Comparison Table
- Conclusion
- Frequently Asked Questions
Table of content:
- Key Differences Between Java And Core Java
- What Is Java?
- What Is Core Java?
- Applications Of Java
- Applications Of Core Java
- When To Use Java?
- When To Use Core Java?
- Conclusion
- Frequently Asked Questions
Table of content:
- What Are Variables In Java Language?
- How To Declare Variables In Java Programs?
- How To Initialize Variables In Java?
- Naming Conventions For Variables In Java
- Types Of Variables In Java
- Local Variables In Java
- Instance Variables In Java
- Static Variables In Java
- Final Variables In Java
- Scope and Lifetime of Variables In Java
- Data Types Of Variables In Java (Primitive & Non-primitive)
- Java Variable Type Conversion & Type Casting
- Working With Variables In Java (Examples)
- Access Modifiers & Variables In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Are Identifiers In Java?
- Syntax Rules For Identifiers In Java
- Valid Identifiers in Java
- Invalid Identifiers in Java
- Java Reserved Keywords
- Naming Conventions & Best Practices For Identifiers In Java
- What Is An Identifier Expected Error In Java?
- Reasons The Identifier Expected Error Occurs
- How To Fix/ Resolve Identifier Expected Errors In Java?
- Conclusion
- Frequently Asked Questions
Table of content:
- What Are Data Types In Java?
- Primitive Data Types In Java
- Non-Primitive Data Types In Java
- Key Differences Between Primitive And Non-Primitive Data Types In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Are Operators In Java?
- Types Of Operators In Java
- Unary Operators In Java
- Arithmetic Operators In Java
- Assignment Operators In Java
- Relational Operators In Java
- Logical Operators In Java
- Bitwise Operators In Java
- Shift Operators In Java
- Increment & Decrement Operators In Java
- Ternary Operator In Java
- Instanceof Operator In Java
- Precedence & Associativity Of Java Operators
- Advantages & Disadvantages Of Operators In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is A Return Statement In Java?
- Use Cases Of Return Statements In Java
- Returning A Value From A Method In Java
- Returning A Class Object In Java
- Returning Void (No Value) In Java
- Advantages Of Using Return Statements In Java
- Limitations Of Using Return Statements In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Are Keywords In Java?
- List Of Keywords In Java
- Detailed Overview Of Java Keywords With Examples
- What If When Keywords In Java Are Used As Variable Names?
- Difference Between Identifiers & Keywords In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Abstract Keyword In Java?
- Use Of Abstract Keyword In Java
- Abstract Methods In Java
- Abstract Classes In Java
- Advantages Of Abstract Keyword In Java
- Disadvantages Of Abstract Keyword In Java
- Abstract Classes Vs. Interfaces In Java
- Real-World Applications Of Abstract Keyword
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is throws Keyword In Java?
- How Does The throws Keyword Work?
- Throwing A Checked Exception Using throws In Java
- Throwing Multiple Exceptions Using throws In Java
- Throwing A Custom Exception Using throws In Java
- When To Use The throws Keyword In Java
- Difference Between throw and throws Keyword In Java
- Best Practices For Using The throws Keyword In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is The Volatile Keyword In Java?
- How Does Volatile Keyword In Java Work?
- Using Volatile Keyword In Java To Control Thread Execution
- Using Volatile Keyword In Java To Signal Between Multiple Threads
- Difference Between Synchronization And Volatile Keyword
- Common Mistakes And Best Practices While Using Volatile Keyword In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding Super Keyword In Java
- Super Keyword In Java With Instance Variables
- Super Keyword In Java With Method Overriding
- Super Keyword In Java With Constructor Chaining
- Applications Of Super Keyword In Java
- Difference Between This And Super Keyword In Java
- Advantages Of Using Super Keyword In Java
- Limitations And Considerations Of Super Keyword In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding This Keyword In Java
- Uses Of This Keyword In Java
- Using This Keyword For Referencing Instance Variables
- Using This Keyword For Invoking A Constructor
- Using This Keyword For Invoking A Method
- Using This Keyword With Getters And Setters
- Difference Between This And Super Keyword In Java
- Best Practices For Using This Keyword In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is new Keyword In Java?
- Uses Of The new Keyword In Java
- Memory Management With new Keyword In Java
- Example 1: Creating An Object Of A Class Using new Keyword In Java
- Example 2: Creating An Array Using The new Keyword In Java
- Best Practices For Using new Keyword In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is The Transient Keyword In Java?
- Real-Life Example Of The Transient Keyword In Java
- When To Use The Transient Keyword In Java
- Example 1: Effect Of Transient Keyword On Serialization In Java
- Example 2: Skipping Sensitive Data During Serialization With Transient Keyword In Java
- Using Transient With Final Keyword In Java
- Using Transient With Static Keyword
- Difference Between Transient And Volatile Keyword In Java
- Advantages And Disadvantages Of Transient Keyword In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Static Keyword In Java?
- Characteristics Of Static Keyword In Java
- Static Variables In Java
- Static Method In Java
- Static Blocks In Java
- Static Classes In Java
- Static Variables Vs Instance Variables In Java
- Advantages Of Static Keyword In Java
- Disadvantages Of Static Keyword In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is A Static Method In Java?
- Use Cases Of Static Method In Java
- Using Static Method In Java To Create A Utility Class
- Using Static Method In Java To Implement The Singleton Design Pattern
- Difference Between Static And Instance Methods In Java
- Limitations Of Static Method In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding Final Keyword In Java
- Final Variables In Java
- Final Methods In Java
- Final Classes In Java
- Difference Between Static And Final Keyword In Java
- Uses Of Final Keyword In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Key Difference Between final, finally, And finalize In Java
- What Is final Keyword In Java?
- What Is finally Keyword In Java?
- What Is finalize Keyword In Java?
- When To Use Which Keyword In Java?
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding The extends Keyword In Java?
- Use Of extends Keyword In Java
- Using Java extends To Implement Single Inheritance
- Using Java extends With Interfaces (Default Methods)
- Overriding Using extends Keyword In Java
- Difference Between extends And implements In Java
- Real World Applications Of Extends Keyword In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Decision Making Statement In Java?
- If Statement In Java
- If-Else Statement In Java
- Else-If Ladder In Java
- Switch Statement In Java
- Ternary/Conditional Operator (?:) In Java
- Best Practices For Writing Decision Making Statements In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Are Control Statements in Java?
- Decision-Making Control Statements In Java
- Looping Control Statements In Java
- Jump (Branching) Control Statements In Java
- Application Of Control Statements In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is The Break Statement In Java?
- Working Of The Break Statement In Java
- Using Java Break Statement With Loops
- Using Java Break Statement With Switch Statement
- Using Java Break Statement With Infinite Loops
- Common Pitfalls While Using Break Statements In Java
- Best Practices For Using The Break Statement In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Switch Statement In Java?
- Working Of The Switch Statement In Java
- Example Of Switch Statement In Java
- Java Switch Statement With String
- Java Nested Switch Statements
- Java Enum In Switch Statement
- Java Wrapper Classes In Switch Statements
- Uses Of Switch Statement In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Syntax Of main() Method In Java
- public Specifier – Main Method In Java
- static Keyword – Main Method In Java
- void Return Type Of Main Method In Java
- The main Identifier – Main Method In Java
- String[] args In Main Method In Java
- The Role Of Java Virtual Machine (JVM)
- Running Java Programs Without The Main Method
- Variations In Declaration Of Main Method In Java
- Overloading The Main Method In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Method Overriding In Java?
- Example Of Method Overriding In Java
- Ideal Use Cases Of Method Overriding In Java
- Rules For Method Overriding In Java
- Super Keyword & Method Overriding In Java
- Constructor & Method Overriding In Java
- Exception Handling In Method Overriding In Java
- Access Modifiers In Method Overriding In Java
- Advantages & Disadvantages Of Method Overriding In Java
- Difference Between Method Overloading Vs. Method Overriding In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Method Overloading In Java?
- Different Ways Of Method Overloading In Java
- Overloading The main() Method In Java
- Type Promotion & Method Overloading In Java
- Null Error & Method Overloading In Java
- Advantages Of Method Overloading In Java
- Disadvantages Of Method Overloading In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Difference Between Overloading And Overriding In Java (Comparison Table)
- What Is Method Overloading In Java?
- What Is Method Overriding In Java?
- Key Differences Between Overloading & Overriding In Java Explained
- Difference Between Overloading And Overriding In Java Code Example
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is A One-Dimensional Array In Java?
- Key Characteristics Of One-Dimensional Arrays In Java
- Declaration Of One-Dimensional Array In Java
- Initialization Of One-Dimensional Array In Java
- Common Operations On One-Dimensional Array In Java
- Advantages Of One-Dimensional Arrays In Java
- Disadvantages Of One-Dimensional Arrays In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is A Multidimensional Array In Java?
- Difference Between Single-Dimensional And Multidimensional Arrays In Java
- Declaring Multidimensional Arrays In Java
- Initializing Multidimensional Arrays In Java
- Accessing And Manipulating Elements In Multidimensional Arrays In Java
- Working Of Multidimensional Arrays With Jagged Arrays In Java
- Why Use Multidimensional Arrays In Java?
- Limitations Of Multidimensional Arrays In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Are Jagged Arrays In Java?
- Comparison With Regular Multi-Dimensional Arrays
- Declaring Jagged Arrays In Java
- Initialization Of Jagged Arrays In Java
- Printing Elements Of A Jagged Array In Java
- Accessing And Modifying Elements Of A Jagged Array In Java
- Advantages Of Jagged Arrays In Java
- Disadvantages Of Jagged Arrays In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Array Of Objects In Java?
- Declare And Initialize An Array Of Object In Java
- Example Of An Array Of Objects In Java
- Sorting An Array Of Objects In Java
- Passing Arrays Of Objects To Methods In Java
- Returning Arrays Of Objects From Methods In Java
- Advantages Of Arrays Of Objects In Java
- Disadvantages Of Arrays Of Objects In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is A Dynamic Array In Java?
- Why Use Dynamic Array In Java?
- What Is The Size And Capacity Of A Dynamic Array In Java?
- How To Create A Dynamic Array In Java?
- Managing Dynamic Data Input In Java
- Storing And Processing Real-Time Data In Java
- Use Cases Of Dynamic Arrays In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Why Return An Array In Java?
- How To Return An Array In Java
- Example 1: Returning An Array Of First N Squares
- Example 2: Doubling the Values of an Array
- Common Scenarios For Returning Arrays In Java
- Points To Remember When Returning Arrays In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding ArrayList In Java
- Differences Between Arrays And ArrayList In Java
- Returning An ArrayList In Java
- Common Use Cases For Returning An ArrayList In Java
- Pitfalls To Avoid When Returning An ArrayList In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is A Thread In Java?
- Thread Vs Process
- What is a Thread Life Cycle In Java?
- What Are Thread Priorities?
- Creating Threads In Java
- Java Thread Methods
- Commonly Used Constructors In Thread Class
- Thread Synchronization In Java
- Common Challenges Faced While Using Threads In Java
- Best Practices For Using Threads In Java
- Real-World Applications Of Threads In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding Multithreading In Java
- Methods Of Multithreading In Java (Examples)
- Difference Between Multithreading And Multitasking In Java
- Handling Exceptions In Multithreading
- Best Practices For Multithreading In Java
- Real-World Use Cases of Multithreading In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Thread Priority In Java?
- Built-In Thread Priority Constants In Java
- Thread Priority: Setter & Getter Methods
- Limitations Of Thread Priority In Java
- Best Practices For Using Thread Priority In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Thread Synchronization In Java?
- The Need For Thread Synchronization In Java
- Types Of Thread Synchronization In Java
- Mutual Exclusion In Thread Synchronization In Java
- Coordination Synchronization (Thread Communication) In Java
- Advantages Of Thread Synchronization In Java
- Disadvantages Of Thread Synchronization In Java
- Alternatives To Synchronization In Java
- Deadlock And Thread Synchronization In Java
- Real-World Use Cases Of Thread Synchronization In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is A Daemon Thread In Java?
- User Threads Vs. Daemon Threads In Java
- Methods For Daemon Threads In The Thread Class
- Creating Daemon Threads In Java
- Checking The Daemon Status Of A Thread
- Exceptions In Daemon Threads
- Limitations Of Daemon Threads In Java
- Practical Applications Of Daemon Threads In Java
- Common Mistakes To Avoid When Working With Daemon Threads In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Why Do Threads Need To Communicate?
- Understanding Inter Thread Communication In Java
- The wait() Method In Inter-Thread Communication
- The notify() Method In Inter-Thread Communication
- The notifyAll() Method In Inter-Thread Communication
- Difference Between wait() And sleep() Methods In Java
- Best Practices For Inter Thread Communication In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding The Factorial Concept
- Approaches To Implementing Factorial In Java
- Find Factorial In Java Using Iterative Approach (Using a Loop)
- Find Factorial In Java Using Recursive Approach
- Complexity Analysis Of Factorial Programs In Java
- Applications Of Factorial Program In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding The Leap Year Concept
- Approach To Check A Leap Year In Java
- Alternative Approach To Check A Leap Year In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is The Difference Between JDK, JRE, and JVM?
- What Is JVM (Java Virtual Machine)?
- What Is JRE (Java Runtime Environment)?
- What Is JDK (Java Development Kit)?
- Understanding The Difference Between JDK, JRE, And JVM
- Comparison Table For Difference Between JDK, JRE, And JVM
- Conclusion
- Frequently Asked Questions
Table of content:
- Difference Between Abstraction And Encapsulation In Java
- Understanding Abstraction In Java
- Understanding Encapsulation In Java
- When To Use Abstraction And Encapsulation?
- Conclusion
- Frequently Asked Questions
Table of content:
- Differences Between Abstract Class And Interface In Java
- What Is An Abstract Class In Java?
- What Is An Interface In Java?
- When To Use An Abstract Class?
- When To Use Interface?
- Compatibility Between Abstract Class And Interface In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Error Vs. Exception In Java
- What Is Error In Java?
- What Is Exception In Java?
- Best Practices For Handling Exceptions In Java
- Why Errors Should Not Be Handled In Java?
- Conclusion
- Frequently Asked Questions
Table of content:
- Key Differences: Java Vs. JavaScript
- What Is Java?
- What Is JavaScript?
- Difference Between Java And JavaScript Explained
- Conclusion
- Frequently Asked Questions
Table of content:
- Brief Introduction To C++
- Brief Introduction To Java
- Difference Between C++ and Java
- Overview & Features Of C++ Language
- Overview & Features of Java Language
- Example of C++ and Java Program
- Key Difference Between C++ And Java Explained
- Similarities Between Java Vs. C++
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- Basic Java interview questions and answers
- Intermediate Java interview questions and answers
- Advanced Java interview questions and answers
Table of content:
- Difference between core Java and advanced Java
- Important Core Java Questions
- Tips for Preparing for Core Java
All Decision Making Statements In Java Explained (+Code Examples)
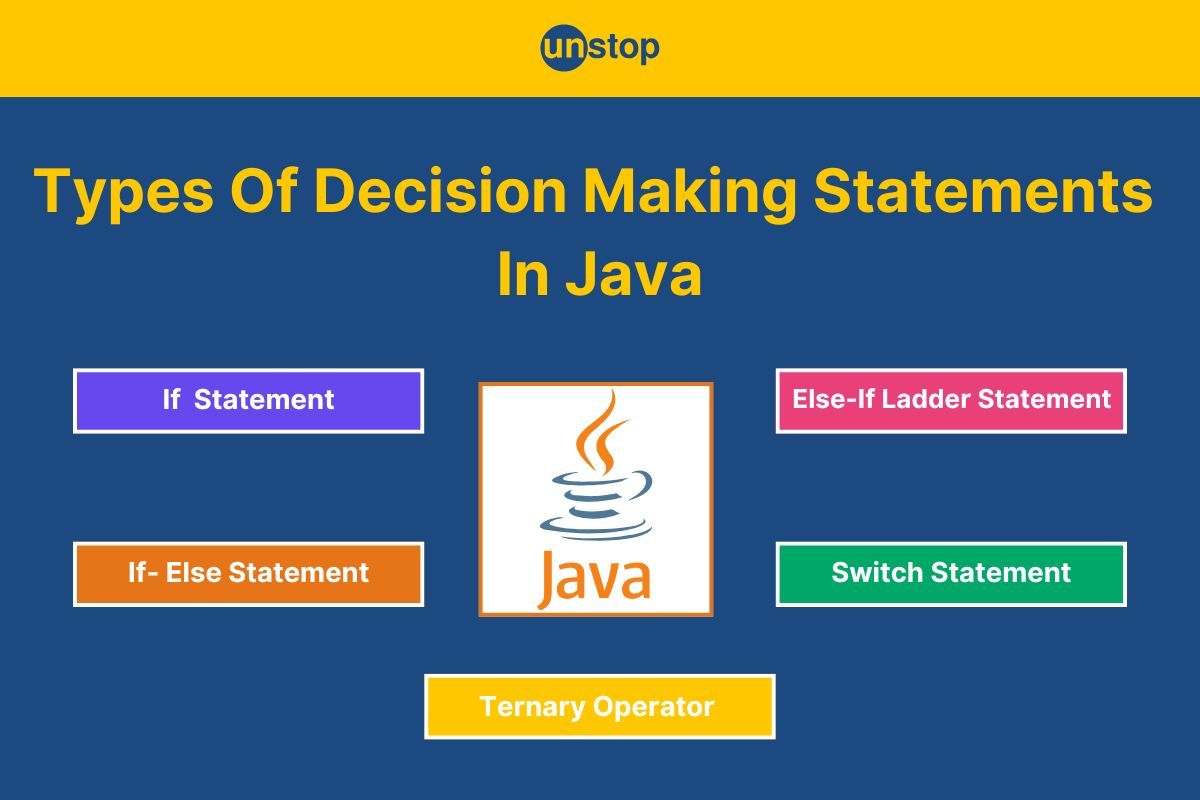
Decision-making statements in Java are constructs that allow us to control the flow of execution based on specific conditions. These statements enable programs to "decide" which block of code to execute depending on whether a condition evaluates to true or false. Essentially, they bring logic into our code, helping it respond dynamically to varying inputs or states.
In this article, we'll explore the core decision-making statements in Java, including if, if-else, else-if, switch, and more. We’ll dive into their syntax, use cases, and examples to understand how they work and when to use each of them effectively.
What Is Decision Making Statement In Java?
In Java programming, decision-making statements allow us to execute certain blocks of code based on specific conditions. They are used to control the flow of execution in a program, making it possible to decide which action to take based on whether a condition is true or false. These statements help in making decisions during runtime.
Here are the different types of decision-making statements in Java:
- If Statement: The if statement evaluates a condition and executes the block of code inside it only if the condition is true.
- If-else Statement: The if-else statement provides two options: one for when the condition is true and another for when it is false.
- Else-if Ladder: The else-if ladder is used when we have multiple conditions to check. It allows us to check for more than two conditions.
- Switch Statement: The switch statement is used when we have a variable that can take one of several values, and we want to execute different code depending on its value.
- Ternary Operator (?:): The ternary operator is a shorthand for the if-else statement. It evaluates a condition and returns one of two values based on whether the condition is true or false.
We will now discuss each of these statements in detail in the sections ahead.
If Statement In Java
The if statement in Java is a fundamental control structure that allows us to execute a block of code only when a specified condition is true. It is used for decision-making and helps in controlling the flow of a program based on conditions.
Syntax Of The If Statement In Java
if (condition) {
// code to be executed if the condition is true
}
Here:
- if: The keyword that initiates the conditional check.
- Condition: A boolean expression that is evaluated. If the condition is true, the block of code inside the if statement is executed.
- Code Block: A set of one or more statements that will execute if the condition evaluates to true. The code block is enclosed in curly braces {}.
Let’s look at an example to understand how the if statement works in Java:
Code Example:
public class IfExample {
public static void main(String[] args) {
int number = 10;
// If statement to check if the number is greater than 5
if (number > 5) {
System.out.println("The number is greater than 5");
}
}
}
Output (set code file name as IfExample.java):
The number is greater than 5
Explanation:
In the above code example-
- We start by defining a class called IfExample. This class contains the main() method, which serves as the entry point for the program.
- Inside the main method, we declare an integer variable named number and initialize it with a value of 10. This variable will be used in the conditional check.
- We then use an if statement to check if the value of number is greater than 5. The condition we are testing is number > 5.
- Since number is 10, which is indeed greater than 5, the condition evaluates to true.
- When the condition is true, the code inside the if block gets executed. This block contains the System.out.println() statement, which prints the message "The number is greater than 5" to the console.
- If the value of number were less than or equal to 5, the code inside the if block would not execute, and nothing would be printed.
Explore this amazing course and master all the key concepts of Java programming effortlessly!
If-Else Statement In Java
The if-else statement is an extension of the if statement. It allows us to execute one block of code if a condition is true, and another block of code if the condition is false. This provides a way to handle two possible scenarios and control the flow of the program based on the condition.
Syntax Of The If-Else Statement In Java
if (condition) {
// code to be executed if the condition is true
} else {
// code to be executed if the condition is false
}
Here:
- if: The keyword that starts the condition evaluation.
- Condition: A boolean expression that evaluates to true or false.
- else: The keyword that begins the alternative block of code, which runs if the condition is false.
Here’s an example using the if-else statement in Java-
Code Example:
public class IfElseExample {
public static void main(String[] args) {
int number = 3;
// If-else statement to check if the number is greater than 5
if (number > 5) {
System.out.println("The number is greater than 5");
} else {
System.out.println("The number is not greater than 5");
}
}
}
Output (set code file name as IfElseExample.java):
The number is not greater than 5
Explanation:
In the above code example-
- We define a class called IfElseExample, and within this class, we have the main() method, which is the starting point of our program.
- Inside the main method, we declare an integer variable named number and initialize it with the value 3. This variable will be used in the conditional check.
- Next, we use an if-else statement to evaluate whether the value of number is greater than 5. The condition being tested is number > 5.
- Since the value of number is 3, which is less than 5, the condition evaluates to false.
- As the condition is false, the program skips over the code inside the if block and instead executes the code in the else block.
- The else block contains a System.out.println() statement that prints "The number is not greater than 5" to the console.
- If number had been greater than 5, the program would have printed "The number is greater than 5" instead.
Else-If Ladder In Java
The else-if ladder is an extension of the if-else statement. It allows us to check multiple conditions in sequence. If the first condition is false, the program evaluates the next condition, and so on. This is useful when we have more than two possible outcomes and need to execute different blocks of code for each case.
Syntax Of The Else-If Ladder In Java
if (condition1) {
// code to be executed if condition1 is true
} else if (condition2) {
// code to be executed if condition2 is true
} else if (condition3) {
// code to be executed if condition3 is true
} else {
// code to be executed if none of the conditions are true
}
Here:
- if: Starts the evaluation of the first condition.
- else if: Checks additional conditions if the previous conditions were false.
- Condition: Each condition is a boolean expression that is checked sequentially.
- else: The default block that executes if none of the conditions evaluate to true.
Given below is an example demonstrating the else-if ladder in Java programming-
Code Example:
public class ElseIfExample {
public static void main(String[] args) {
int number = 15;
// Else-if ladder to check the value of the number
if (number > 20) {
System.out.println("The number is greater than 20");
} else if (number > 10) {
System.out.println("The number is greater than 10 but less than or equal to 20");
} else if (number > 5) {
System.out.println("The number is greater than 5 but less than or equal to 10");
} else {
System.out.println("The number is 5 or less");
}
}
}
Output (set code file name as ElseIfExample.java):
The number is greater than 10 but less than or equal to 20
Explanation:
In the above code example-
- We define a class called ElseIfExample, and within it, we have the main() method, which is where the program starts execution.
- Inside the main method, we declare an integer variable number and initialize it with the value 15. This variable will be used in a series of conditional checks.
- We then use an if-else if-else ladder to check different conditions based on the value of number.
- The first condition checks if number is greater than 20 (number > 20). Since the value of number is 15, which is not greater than 20, this condition is false, so the program moves to the next condition.
- The second condition checks if number is greater than 10 (number > 10). Since 15 is indeed greater than 10, this condition evaluates to true.
- Because this condition is true, the code inside the corresponding block gets executed, which prints "The number is greater than 10 but less than or equal to 20" to the console.
- The remaining else if and else blocks are skipped since the condition in the second else if was true.
- If none of the conditions had been true, the else block would have been executed, printing "The number is 5 or less."
Sharpen your coding skills with Unstop's 100-Day Coding Sprint and compete now for a top spot on the leaderboard!
Switch Statement In Java
The switch statement in Java is a control structure that allows us to choose one of many code blocks to execute based on the value of a variable or expression. It's an alternative to using multiple if-else statements when there are multiple possible outcomes for a given condition. This makes the code cleaner and more readable when dealing with multiple conditions.
Syntax Of Switch Statement In Java
switch (expression) {
case value1:
// code to be executed if expression == value1
break;
case value2:
// code to be executed if expression == value2
break;
case value3:
// code to be executed if expression == value3
break;
default:
// code to be executed if no cases match
}
Here:
- switch: The keyword that begins the switch block.
- Expression: The value that is evaluated. It can be of types like int, char, String, etc.
- case: Defines possible values that the expression is compared against.
- break: The statement that exits the switch block once a match is found.
- default: A fallback block of code that is executed if none of the cases match.
Here’s an example demonstrating the use of the switch statement in Java-
Code Example:
public class SwitchExample {
public static void main(String[] args) {
int day = 3;
// Switch statement to print the day of the week
switch (day) {
case 1:
System.out.println("Monday");
break;
case 2:
System.out.println("Tuesday");
break;
case 3:
System.out.println("Wednesday");
break;
case 4:
System.out.println("Thursday");
break;
case 5:
System.out.println("Friday");
break;
case 6:
System.out.println("Saturday");
break;
case 7:
System.out.println("Sunday");
break;
default:
System.out.println("Invalid day");
}
}
}
Output (set code file name as SwitchExample.java):
Wednesday
Explanation:
In the above code example-
- We define a class called SwitchExample, and within it, we have the main() method, which serves as the entry point for the program.
- Inside the main method, we declare an integer variable named day and initialize it with the value 3. This variable represents the day of the week, with 1 for Monday, 2 for Tuesday, and so on.
- We then use a switch statement to check the value of day and print the corresponding day of the week.
- The switch statement evaluates the value of day and compares it with the values in each case block.
- Since the value of day is 3, the program matches the case 3 block. This block contains the statement System.out.println("Wednesday");, so the program prints "Wednesday" to the console.
- The break statement in each case block ensures that once a match is found and the corresponding action is executed, the program exits the switch statement, preventing the execution of subsequent cases.
- If the value of day did not match any of the specified cases (for example, if it were a number outside the range of 1 to 7), the default block would execute, printing "Invalid day."
Ternary/Conditional Operator (?:) In Java
The ternary operator (also known as the conditional operator) is a shorthand for the if-else statement. It provides a concise way to evaluate a condition and choose between two expressions based on whether the condition is true or false. The ternary operator is often used for simple conditions and assignments.
Syntax Of Ternary Operator In Java
condition ? expressionIfTrue : expressionIfFalse;
Here:
- Condition: A boolean expression that is evaluated.
- ?: The operator that separates the condition from the expressions.
- Expression if true: The code or value that will be executed/returned if the condition is true.
- Expression if false: The code or value that will be executed/returned if the condition is false.
Let’s look at a code example to understand the working of ternary operator in Java-
Code Example:
public class TernaryOperatorExample {
public static void main(String[] args) {
int number = 8;
// Using the ternary operator to check if the number is even or odd
String result = (number % 2 == 0) ? "Even" : "Odd";
System.out.println("The number is: " + result);
}
}
Output (set code file name as TernaryOperatorExample.java):
The number is: Even
Explanation:
In the above code example-
- We define a class called TernaryOperatorExample, and within this class, we have the main() method, which is the entry point of the program.
- Inside the main method, we declare an integer variable number and initialize it with the value 8. This variable will be checked to determine if it is even or odd.
- We then use the ternary operator to check whether number is even or odd. The ternary operator has the following structure: condition ? valueIfTrue : valueIfFalse.
- In this case, the condition is number % 2 == 0, which checks if the number is divisible by 2 (i.e., if it is even).
- Since the value of number is 8, which is divisible by 2, the condition evaluates to true. As a result, the value "Even" is assigned to the result variable.
- If number had been an odd number (e.g., 7), the condition would have been false, and the value "Odd" would have been assigned to result.
- Finally, we use System.out.println() to print the message "The number is: Even" to the console, as result contains the string "Even."
Are you looking for someone to answer all your programming-related queries? Let's find the perfect mentor here.
Best Practices For Writing Decision Making Statements In Java
When writing decision-making statements in Java, it's important to focus on readability, maintainability, and efficiency. Here are some best practices that can help you write clean, effective decision-making logic:
- Keep Conditions Simple: Avoid complex conditions with multiple logical operators (&&, ||). If needed, break them down into simpler conditions or use intermediate variables to improve readability. For Example-
boolean isWeekend = day == 6 || day == 7;
if (isWeekend) {
// Do something
}
- Avoid Deeply Nested Statements: Excessive nesting can make code hard to read and maintain. Use early returns (in methods) or simplify logic with functions that handle complex conditions separately. For Example-
if (condition1) {
if (condition2) {
// Do something
}
}
- Use switch for Multiple Discrete Conditions: For checking the same variable against multiple possible values, switch is often more readable and efficient than an if-else ladder. For Example-
switch (day) {
case 1: System.out.println("Monday"); break;
case 2: System.out.println("Tuesday"); break;
case 3: System.out.println("Wednesday"); break;
default: System.out.println("Invalid day");
}
- Use default in switch Statements: Always include a default case in a switch statement to handle unexpected values, improving code robustness and making the logic easier to follow. For Example-
switch (day) {
case 1: // Monday
case 2: // Tuesday
default: // Handle invalid days
}
- Use Meaningful Variable Names: Ensure that the variables used in the conditions have meaningful names that clearly describe their role. This enhances readability. For Example-
int age = 25;
if (age >= 18) { // instead of "x" or "num"
System.out.println("Adult");
}
- Be Careful with Floating Point Comparisons: Avoid direct comparison of floating-point numbers (float, double) due to precision issues. Instead, use a tolerance value to compare them. For Example-
double tolerance = 0.0001;
if (Math.abs(a - b) < tolerance) {
// Consider a and b equal
}
- Avoid Complex Boolean Expressions: When dealing with complex boolean conditions, use descriptive variables for readability. For Example-
boolean isEligibleForDiscount = age > 18 && age < 60;
if (isEligibleForDiscount) {
// Apply discount
}
- Handle All Possible Conditions: Make sure that all possible conditions are handled. For example, in a switch statement, always include a default case to handle unexpected inputs.
- Document Complex Conditions: If a condition is non-trivial, consider adding comments to explain why it’s necessary. This helps future maintainers understand the reasoning behind the logic. For Example-
// Check if the user is eligible for a special offer
if (userAge > 18 && hasValidSubscription) {
// Process the offer
}
- Optimize for Performance: In performance-critical applications, evaluate conditions that are likely to be true first to reduce the number of evaluations. For Example-
if (isValid && isAvailable) {
// Proceed with operations
}
Conclusion
In Java programming language, decision-making statements are essential for directing the flow of execution based on specific conditions. Whether you're using an if-else statement for simple choices, an else-if ladder for multiple conditions, a switch statement for discrete values, or the ternary operator for compact expressions, each decision-making tool has its unique use case.
By following best practices such as keeping conditions simple, choosing the most appropriate statement, avoiding deep nesting, and ensuring readability, you can write clean, efficient, and maintainable code. Proper use of these structures ensures your Java applications are not only logical but also clear and robust, making them easier to maintain and extend in the future.
Frequently Asked Questions
Q. What is the difference between if and if-else statements in Java?
The if statement allows you to execute a block of code if a condition is true. If the condition is false, the program simply skips the block. On the other hand, the if-else statement allows you to specify an alternative block of code to execute when the condition is false. This provides a two-way choice: one block of code executes if the condition is true, and another executes if it's false.
Q. What is an else-if ladder, and when should it be used?
An else-if ladder is used when there are multiple conditions to evaluate sequentially. If the first condition is false, the program evaluates the next condition in the ladder, and so on, until a true condition is found or the else block (if present) is executed as the default.
It should be used when you have more than two conditions to check, and you need to execute a different block of code depending on which condition is true.
Q. When should I use the switch statement over if-else in Java?
A switch statement is most effective when you need to compare the same variable or expression against multiple possible constant values. It is more efficient and readable compared to a chain of if-else statements when there are many potential conditions to check. The switch is also easier to maintain, as it consolidates the multiple conditions into one structure.
Q. What are the advantages of using the ternary operator in Java?
The ternary operator provides a concise, single-line alternative to if-else statements, making your code shorter and potentially more readable for simple conditions. It is especially useful when assigning values based on a condition or performing quick decisions in expressions. However, it should be used sparingly, as overuse can lead to decreased readability in more complex conditions.
Q. Can I use multiple conditions in an if statement in Java?
Yes, you can use multiple conditions in an if statement by combining them with logical operators such as && (AND) and || (OR). This allows you to check for more complex conditions. For Example-
if (age > 18 && hasLicense) {
System.out.println("Eligible to drive");
}
Q. What are the limitations of using the switch statement in Java?
While the switch statement is great for handling multiple discrete values, it does have some limitations:
- It can only evaluate expressions that are of type byte, short, char, int, String, and their corresponding wrapper classes. You cannot use objects or boolean values.
- A switch statement evaluates values exactly as they are, so it can’t handle ranges or conditions like if statements can.
- It is limited to comparing one expression against multiple cases, and if you have conditions that require different variables to be compared, if-else or else-if might be more appropriate.
Q. How can I improve the readability of complex decision-making logic?
To improve readability:
- Break complex conditions into smaller, more descriptive variables.
- Avoid deeply nested if statements by using early returns in methods.
- Use comments to explain non-obvious conditions.
- Prefer switch when evaluating the same variable against multiple values.
- Maintain consistent formatting and indentation to make the decision-making logic clear.
- Refactor lengthy if-else chains into methods or helper functions if they involve complex logic.
With this, we come to an end of our discussion on the decision-making statements in Java. Here are a few other topics that you might be interested in reading:
- Convert String To Date In Java | 3 Different Ways With Examples
- Final, Finally & Finalize In Java | 15+ Differences With Examples
- Super Keyword In Java | Definition, Applications & More (+Examples)
- How To Find LCM Of Two Numbers In Java? Simplified With Examples
- How To Find GCD Of Two Numbers In Java? All Methods With Examples
- Volatile Keyword In Java | Syntax, Working, Uses & More (+Examples)
I’m a Computer Science graduate with a knack for creative ventures. Through content at Unstop, I am trying to simplify complex tech concepts and make them fun. When I’m not decoding tech jargon, you’ll find me indulging in great food and then burning it out at the gym.
Comments
Add commentLogin to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
Divyansh Shrivastava 2 days ago
Nirdesh Sharma 1 week ago
Esuru Pooja.C 2 weeks ago
Sunita Bhat 2 weeks ago
Ankita Das 2 weeks ago
Mihir Kumar Ranjan 3 weeks ago