Table of content:
- What Is A Return Statement In Java?
- Use Cases Of Return Statements In Java
- Returning A Value From A Method In Java
- Returning A Class Object In Java
- Returning Void (No Value) In Java
- Advantages Of Using Return Statements In Java
- Limitations Of Using Return Statements In Java
- Conclusion
- Frequently Asked Questions
Return Statement In Java | Syntax, Uses & More (+Code Examples)
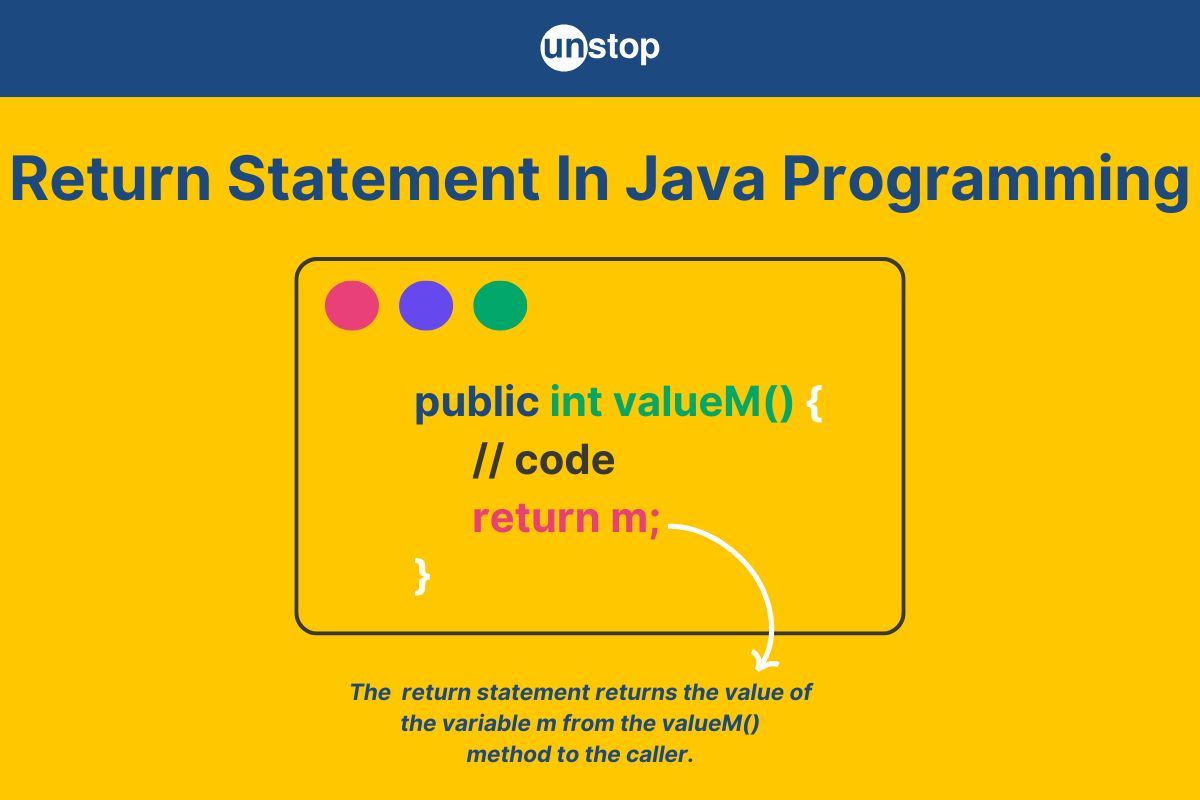
In Java, the return statement plays a crucial role in controlling the flow of a program. It is primarily used to exit from a method and optionally pass a value back to the method caller. Whether it’s returning the result of a calculation, a message, or simply ending a void method, the return statement provides flexibility and functionality.
In this article, we will understand the significance of the return statement in Java, its syntax, usage with different return types, and best practices for writing efficient and readable code. Let’s explore how this simple yet powerful keyword enhances the structure and logic of Java programs.
What Is A Return Statement In Java?
In Java programming, a return statement is used to exit a method and optionally send a value back to the caller. It tells the program, "I’m done here, and here’s the result (if any)." The value being returned must match the method’s declared return type. If a method is declared as void, it doesn’t return a value, but the return statement can still be used to exit the method early.
Syntax Of Return Statement
return value; // For methods with a return type.
return; // For void methods.
Here:
- return: It is a keyword that signals the end of method execution. Used in all methods (with or without a return value).
- value (Optional): The result being sent back to the method caller. It must match the return type of the method (e.g., int, String, or double).
Real-Life Analogy Of Return Statement In Java
Imagine ordering a pizza online. When the delivery person arrives, they "return" the pizza to you.
- The pizza is the "value" being returned, while the delivery process is like the method in a program.
- If you only ordered a service, like repairing something, the technician may simply leave after the task (like a void method) with no "return value."
Sharpen your coding skills with Unstop's 100-Day Coding Sprint and compete now for a top spot on the leaderboard!
Use Cases Of Return Statements In Java
The return statement in Java is versatile and serves several critical purposes in a program. Its use depends on the context and the type of method in which it is employed. Below are the primary use cases:
- Returning a Value from a Method: Used to return results (like calculations or data) back to the caller. For Example-
public int add(int a, int b) {
return a + b; // Returns the sum of two numbers
}
- Exiting a Method Early: The return statement can also be used to exit a method early before it reaches its end. This is useful when a condition is met, and there’s no need to continue the method's execution. For Example-
public void checkNumber(int num) {
if (num < 0) {
System.out.println("Negative number detected.");
return; // Exit early
}
System.out.println("Number is valid.");
}
- Returning Objects or Complex Data: When a method needs to return more than just simple data (like a number or string), you can use the return statement to return objects of a class or objects implementing an interface. For Example-
public Car getCar() {
return new Car("Sedan", "Blue"); // Return a Car object
}
- Returning Boolean Values for Conditions: The return statement can return true or false based on a condition. This is often used for validating inputs or checking whether a condition is met. For Example-
public boolean isEven(int number) {
return number % 2 == 0; // Return true if number is even
}
- Returning Multiple Values Using Collections: In Java, you can use collections like arrays or lists to return multiple values from a method. This is useful when you need to return more than one result without using multiple return statements. For Example-
public List<Integer> getScores() {
return Arrays.asList(85, 90, 95); // Return a list of scores
}
- Default Return in Recursion: In recursive methods, the return statement is essential for defining a base case. This base case stops the recursion when a certain condition is met, preventing an infinite loop. For Example-
public int factorial(int n) {
if (n == 0) return 1; // Base case
return n * factorial(n - 1);
}
- Void Methods for Task Completion: In void methods (methods that don’t return a value), the return statement can still be used to exit the method early. This is useful for tasks like printing, logging, or checking conditions. For Example-
public void printMessage(String message) {
if (message == null) {
return; // Exit early for invalid input
}
System.out.println(message);
}
- Used in Lambda Expressions (Java 8+): In Java 8 and later, lambda expressions allow methods to return values concisely. This is especially useful for functional programming-style tasks like mapping, filtering, and reducing data. For Example-
Function<Integer, String> checkOddEven = num -> {
return num % 2 == 0 ? "Even" : "Odd";
};
Returning A Value From A Method In Java
In Java, methods can be designed to perform specific tasks and return a result to the caller. The return statement facilitates this process. When a method is declared with a specific return type (e.g., int, double, String), it must use a return statement to provide a value of that type to the caller.
- Method Declaration with a Return Type: The method signature includes a return type that defines the data type of the value the method will return. For Example-
public int calculateSum() { ... }
- Return Statement: The return statement ends the method execution and provides a value to the caller. The value must match the method's declared return type.
- Purpose: Commonly used to return calculated results, retrieved data, or processed values. For Example- Returning the sum of two numbers.
We'll now look at a Java program to calculate and return the area of a rectangle.
Code Example:
cHVibGljIGNsYXNzIFJlY3RhbmdsZUFyZWEgewoKICAgIC8vIE1ldGhvZCB0byBjYWxjdWxhdGUgdGhlIGFyZWEgb2YgYSByZWN0YW5nbGUKICAgIHB1YmxpYyBzdGF0aWMgZG91YmxlIGNhbGN1bGF0ZUFyZWEoZG91YmxlIGxlbmd0aCwgZG91YmxlIHdpZHRoKSB7CiAgICAgICAgZG91YmxlIGFyZWEgPSBsZW5ndGggKiB3aWR0aDsgLy8gQ2FsY3VsYXRlIGFyZWEKICAgICAgICByZXR1cm4gYXJlYTsgICAgICAgICAgICAgICAgICAgLy8gUmV0dXJuIHRoZSBjYWxjdWxhdGVkIHZhbHVlCiAgICB9CgogICAgcHVibGljIHN0YXRpYyB2b2lkIG1haW4oU3RyaW5nW10gYXJncykgewogICAgICAgIGRvdWJsZSBsZW5ndGggPSA1LjU7IC8vIExlbmd0aCBvZiB0aGUgcmVjdGFuZ2xlCiAgICAgICAgZG91YmxlIHdpZHRoID0gMy4yOyAgLy8gV2lkdGggb2YgdGhlIHJlY3RhbmdsZQoKICAgICAgICAvLyBDYWxsIHRoZSBtZXRob2QgYW5kIHN0b3JlIHRoZSByZXR1cm5lZCB2YWx1ZQogICAgICAgIGRvdWJsZSBhcmVhID0gY2FsY3VsYXRlQXJlYShsZW5ndGgsIHdpZHRoKTsKCiAgICAgICAgLy8gRGlzcGxheSB0aGUgcmVzdWx0CiAgICAgICAgU3lzdGVtLm91dC5wcmludGxuKCJUaGUgYXJlYSBvZiB0aGUgcmVjdGFuZ2xlIGlzOiAiICsgYXJlYSArICIgc3F1YXJlIHVuaXRzIik7CiAgICB9Cn0K
Output (set code file name as RectangleArea.java):
The area of the rectangle is: 17.6 square units
Explanation:
In the above code example-
- We start by defining a public class named RectangleArea, which acts as a container for all the logic and methods we need to calculate the area of a rectangle.
- Inside the class, we create a static method called calculateArea() that takes two parameters: length and width. These parameters represent the dimensions of the rectangle.
- In the calculateArea() method, we multiply the length by the width to calculate the area. This calculated value is then stored in a variable named area. Finally, we return this value to the caller.
- Moving to the main() method, which is the starting point of our program, we declare two variables: length and width. We assign the values 5.5 and 3.2 to these variables, representing the dimensions of the rectangle.
- Next, we call the calculateArea() method and pass the length and width as arguments. The returned result, which is the area of the rectangle, is stored in a variable named area.
- After calculating the area, we use the System.out.println() function to display the result. This prints the area of the rectangle along with the appropriate units, making the output clear and informative.
- The program is designed for precision, as we use the double data type to handle dimensions that might include decimal values. By making the calculateArea method static, we ensure that it can be used without creating an instance of the class, which simplifies our code and makes it reusable.
Explore this amazing course and master all the key concepts of Java programming effortlessly!
Returning A Class Object In Java
In Java, a method can return an object of a class or an interface type. This allows methods to return more complex data structures or encapsulate functionality, making the code modular and reusable. When a method returns an object, it essentially provides a reference to that object for further use.
- Method Declaration: The return type in the method signature is specified as the class or interface type. For Example-
public MyClass createObject() { ... }
- Returning an Object: The method can create an object, perform operations, and then return it using the return statement.
- Returning an Interface: A method can return an object that implements a specified interface. This promotes abstraction by ensuring the caller interacts with the object via the interface, not its concrete implementation.
- Purpose: Used for object creation, factory methods, or returning polymorphic behavior via interfaces. For Example- Returning a database connection object or a customized instance.
Here’s an example of a method that returns an object of the Rectangle class.
Code Example:
Ly8gQ2xhc3MgcmVwcmVzZW50aW5nIGEgUmVjdGFuZ2xlCmNsYXNzIFJlY3RhbmdsZSB7CgogICAgcHJpdmF0ZSBkb3VibGUgbGVuZ3RoOwogICAgcHJpdmF0ZSBkb3VibGUgd2lkdGg7CgogICAgLy8gQ29uc3RydWN0b3IKICAgIHB1YmxpYyBSZWN0YW5nbGUoZG91YmxlIGxlbmd0aCwgZG91YmxlIHdpZHRoKSB7CiAgICAgICAgdGhpcy5sZW5ndGggPSBsZW5ndGg7CiAgICAgICAgdGhpcy53aWR0aCA9IHdpZHRoOwogICAgfQoKICAgIC8vIE1ldGhvZCB0byBjYWxjdWxhdGUgYXJlYQogICAgcHVibGljIGRvdWJsZSBnZXRBcmVhKCkgewogICAgICAgIHJldHVybiBsZW5ndGggKiB3aWR0aDsKICAgIH0KCiAgICBAT3ZlcnJpZGUKICAgIHB1YmxpYyBTdHJpbmcgdG9TdHJpbmcoKSB7CiAgICAgICAgcmV0dXJuICJSZWN0YW5nbGVbbGVuZ3RoPSIgKyBsZW5ndGggKyAiLCB3aWR0aD0iICsgd2lkdGggKyAiXSI7CiAgICB9Cn0KCnB1YmxpYyBjbGFzcyBDbGFzc1JldHVybkV4YW1wbGUgewoKICAgIC8vIE1ldGhvZCB0byBjcmVhdGUgYW5kIHJldHVybiBhIFJlY3RhbmdsZSBvYmplY3QKICAgIHB1YmxpYyBzdGF0aWMgUmVjdGFuZ2xlIGNyZWF0ZVJlY3RhbmdsZShkb3VibGUgbGVuZ3RoLCBkb3VibGUgd2lkdGgpIHsKICAgICAgICByZXR1cm4gbmV3IFJlY3RhbmdsZShsZW5ndGgsIHdpZHRoKTsgLy8gUmV0dXJuaW5nIHRoZSBvYmplY3QKICAgIH0KCiAgICBwdWJsaWMgc3RhdGljIHZvaWQgbWFpbihTdHJpbmdbXSBhcmdzKSB7CiAgICAgICAgLy8gQ3JlYXRlIGEgUmVjdGFuZ2xlIHVzaW5nIHRoZSBtZXRob2QKICAgICAgICBSZWN0YW5nbGUgcmVjdCA9IGNyZWF0ZVJlY3RhbmdsZSg0LjAsIDMuNSk7CgogICAgICAgIC8vIERpc3BsYXkgdGhlIHJlY3RhbmdsZSBkZXRhaWxzIGFuZCBhcmVhCiAgICAgICAgU3lzdGVtLm91dC5wcmludGxuKCJDcmVhdGVkOiAiICsgcmVjdCk7CiAgICAgICAgU3lzdGVtLm91dC5wcmludGxuKCJBcmVhIG9mIHRoZSByZWN0YW5nbGU6ICIgKyByZWN0LmdldEFyZWEoKSk7CiAgICB9Cn0K
Output (set code file name as ClassReturnExample.java):
Created: Rectangle[length=4.0, width=3.5]
Area of the rectangle: 14.0
Explanation:
In the above code example-
- We define a class named Rectangle that represents a rectangle with two attributes: length and width. These attributes are declared as private to enforce encapsulation, ensuring that they can only be accessed or modified through the class's methods.
- A constructor is provided in the Rectangle class to initialize the length and width when a new rectangle object is created. This constructor takes two parameters, assigns them to the instance variables, and ensures the object is set up properly.
- The getArea() method is defined to calculate the area of the rectangle. It multiplies the length by the width and returns the result. This method provides a simple way to retrieve the calculated area for any rectangle object.
- We override the toString() method in the Rectangle class to provide a readable string representation of the rectangle object. This method returns a string containing the rectangle's dimensions in a clear format.
- Moving to the ClassReturnExample class, we define a static method named createRectangle. This method takes the length and width as parameters, creates a new Rectangle object using these values, and then returns the created object.
- In the main() method, which is the program's entry point, we call the createRectangle method with specific dimensions (4.0 and 3.5). This method call returns a new Rectangle object, which we store in a variable named rect.
- We use the System.out.println() function to print details about the created rectangle. This triggers the overridden toString method, displaying the rectangle's dimensions in a descriptive format.
- We then call the getArea() method on the rect object to calculate and display the rectangle's area. The result is printed using System.out.println(), providing a clear and user-friendly output.
Returning Void (No Value) In Java
In Java, when a method has a return type of void, it means the method does not return any value to the caller. Instead of providing a result or data, the method performs a task, such as printing a message, modifying an object, or performing some action, but does not give anything back.
- The return; statement in a void method marks the end of the method's execution.
- No value is returned to the caller.
- It is optional to include a return in a void method; it’s only needed if you want to exit early.
Let’s look at an example where we have a void method that checks whether a number is positive, negative, or zero. The method does not return any value, but it prints the result directly.
Code Example:
cHVibGljIGNsYXNzIE51bWJlckNoZWNrIHsKCiAgICAvLyBWb2lkIG1ldGhvZCB0aGF0IGNoZWNrcyBpZiB0aGUgbnVtYmVyIGlzIHBvc2l0aXZlLCBuZWdhdGl2ZSwgb3IgemVybwogICAgcHVibGljIHZvaWQgY2hlY2tOdW1iZXIoaW50IG51bSkgewogICAgICAgIAogICAgICAgIGlmIChudW0gPiAwKSB7CiAgICAgICAgICAgIFN5c3RlbS5vdXQucHJpbnRsbigiVGhlIG51bWJlciBpcyBwb3NpdGl2ZS4iKTsKICAgICAgICAgICAgcmV0dXJuOyAvLyBFYXJseSByZXR1cm4gYWZ0ZXIgcHJpbnRpbmcKICAgICAgICB9CiAgICAgICAgCiAgICAgICAgZWxzZSBpZiAobnVtIDwgMCkgewogICAgICAgICAgICBTeXN0ZW0ub3V0LnByaW50bG4oIlRoZSBudW1iZXIgaXMgbmVnYXRpdmUuIik7CiAgICAgICAgICAgIHJldHVybjsgLy8gRWFybHkgcmV0dXJuIGFmdGVyIHByaW50aW5nCiAgICAgICAgfQogICAgICAgIAogICAgICAgIFN5c3RlbS5vdXQucHJpbnRsbigiVGhlIG51bWJlciBpcyB6ZXJvLiIpOwogICAgfQoKICAgIHB1YmxpYyBzdGF0aWMgdm9pZCBtYWluKFN0cmluZ1tdIGFyZ3MpIHsKICAgICAgICBOdW1iZXJDaGVjayBjaGVja2VyID0gbmV3IE51bWJlckNoZWNrKCk7CiAgICAgICAgCiAgICAgICAgLy8gQ2FsbGluZyB0aGUgY2hlY2tOdW1iZXIgbWV0aG9kIHdpdGggZGlmZmVyZW50IHZhbHVlcwogICAgICAgIGNoZWNrZXIuY2hlY2tOdW1iZXIoNSk7ICAgIC8vIE91dHB1dDogVGhlIG51bWJlciBpcyBwb3NpdGl2ZS4KICAgICAgICBjaGVja2VyLmNoZWNrTnVtYmVyKC0zKTsgICAvLyBPdXRwdXQ6IFRoZSBudW1iZXIgaXMgbmVnYXRpdmUuCiAgICAgICAgY2hlY2tlci5jaGVja051bWJlcigwKTsgICAgLy8gT3V0cHV0OiBUaGUgbnVtYmVyIGlzIHplcm8uCiAgICB9Cn0K
Output (set code file name as NumberCheck.java):
The number is positive.
The number is negative.
The number is zero.
Explanation:
In the above code example-
- We start by defining a class named NumberCheck, which contains a method checkNumber(). This method checks whether a given number is positive, negative, or zero.
- The checkNumber() method takes an integer parameter num and uses a series of if and else if statements to determine the number's sign.
- First, we check if num is greater than zero. If it is, we print "The number is positive." and return immediately from the method using the return statement. The return statement ensures that the method exits as soon as we know the number is positive, without checking further conditions.
- If the number is not positive, we then check if it is less than zero using the else if condition. If this condition is true, we print "The number is negative." and return from the method, ending the check.
- If neither of the previous conditions is true, it means the number must be zero, so we print "The number is zero."
- In the main() method, we create an instance of the NumberCheck class called checker. We then call the checkNumber method on this object three times, passing different values to check the output for positive, negative, and zero cases.
- The first call to checkNumber(5) prints "The number is positive.", the second call to checkNumber(-3) prints "The number is negative.", and the third call to checkNumber(0) prints "The number is zero."
Advantages Of Using Return Statements In Java
Here are some of the key advantages of using the return statement effectively:
- Passes Data Between Methods: The return statement allows methods to pass data back to the caller. This is useful when a method performs a task, such as a calculation, and needs to send the result back to the calling method for further processing or decision-making.
- Improves Code Clarity: By using return statements in methods, it’s clear what value is being returned to the caller. This improves the overall readability of the code, as the purpose and behavior of the method are immediately understood by other Java developers or reviewers.
- Controls Method Execution: The return statement allows for early termination of a method. This is particularly useful when a condition is met, such as an error or when a result is already found, preventing unnecessary further computation or logic in the method.
- Supports Recursion: In recursive methods, the return statement is used to define a base case, which ensures the recursion ends. Without a proper return statement to stop the recursion, it can lead to infinite loops or stack overflow errors.
- Returns Complex Data: Methods can return objects or more complex data types, like arrays, collections, or custom classes, allowing methods to provide more meaningful results. This enables better organization and handling of more sophisticated data structures.
- Enhances Lambda Expressions: In Java 8 and later, the return statement is crucial for lambda expressions. It allows for concise, functional programming-style operations, such as returning values in functions used with streams, mapping, or filtering.
- Improves Error Handling: The return statement can be used for better error handling and flow control. For instance, a method can return an error code or message when it encounters invalid input, allowing the calling code to handle errors gracefully without crashing.
- Encourages Reusability: By using the return statement, methods can return values that can be reused across different parts of the program. This supports modular programming by allowing methods to serve as building blocks that can be called and reused multiple times with different inputs.
Are you looking for someone to answer all your programming-related queries? Let's find the perfect mentor here.
Limitations Of Using Return Statements In Java
While the return statement is a fundamental part of method execution in Java, it does come with certain limitations and considerations that developers should be aware of. Here are some of the main limitations:
- Cannot Be Used Outside a Method: The return statement can only be used inside methods. It cannot be used in constructors, instance initializers, or class-level code blocks.
Reason: return is designed to terminate a method's execution and return control to the calling code. Outside a method, there's no context of returning a value or exiting a block, which makes the statement invalid.
- Unreachable Code After Return Statement: Any code placed after a return statement in the same method is unreachable and will result in a compilation error.
Reason: Once a return statement is executed, the control flow of the method ends, and any subsequent code is never reached. The compiler detects this and flags it as an error.
- Methods with Non-Void Return Type Must Always Return a Value: If a method is declared with a return type other than void, it must always return a value of the correct type. Failing to do so results in a compile-time error.
Reason: The method's return type defines what the method is expected to return. If no return value is provided, the method is incomplete, and the Java compiler cannot compile the code. For Example-
public int sum(int a, int b) {
// No return statement here, will result in compile-time error
}
- Multiple Return Statements Can Lead to Confusion: Using multiple return statements in a single method, especially in complex logic, can reduce code clarity and lead to confusion or errors in understanding the control flow.
Reason: While returning early can be useful in some cases, excessive use of multiple return points makes it difficult to follow the method's logic, especially when the method is large or has nested conditions.
- Return Statement in Loops and Recursion Can Lead to Early Exit: Using a return statement inside loops or recursive methods can cause an early exit, which may not always be desired.
Reason: A return statement terminates the method immediately, which can halt further loop iterations or prevent the continuation of recursive calls. For Example-
public void findEvenNumber(int[] arr) {
for (int num : arr) {
if (num % 2 == 0) {
System.out.println("Found even number: " + num);
return; // Exits the loop and method early
}
}
System.out.println("No even numbers found.");
}
- Returning Complex Objects Requires Proper Memory Management: When returning complex objects (like arrays, collections, or custom objects), developers must be cautious about managing memory and ensuring that returned objects are not unexpectedly modified outside the method.
Reason: Returning mutable objects means that any changes to those objects in the calling code will affect the original object, which could lead to bugs or unexpected behavior. For Example-
public List<String> getNames() {
List<String> names = new ArrayList<>();
names.add("John");
names.add("Alice");
return names;
}
// The returned list can be modified by the calling code, affecting the original list.
Conclusion
The return statement in Java language is an essential tool that enhances the functionality, readability, and maintainability of code. It plays a pivotal role in passing data between methods, controlling method execution, and handling recursion effectively. By enabling methods to return values, objects, or even complex data types, it allows for cleaner, more modular code that can be easily reused and maintained. Additionally, the return statement supports better error handling, optimizes program flow, and fosters the use of modern programming techniques like lambda expressions in Java 8 and beyond. Understanding and utilizing the return statement effectively is key to writing efficient and clean Java code, making it a fundamental concept for both beginners and experienced developers.
Frequently Asked Questions
Q. What is the purpose of the return statement in Java?
The return statement in Java is used to exit a method and optionally return a value to the caller. It serves two primary purposes:
- Returning a result or data from a method, which can be used elsewhere in the Java program.
- Ending the method execution early when a certain condition is met, avoiding unnecessary computation.
- In methods with a non-void return type, the return statement must be followed by a value of the specified type.
Q. What is the difference between return value; and return; in Java?
Here’s a table that clearly explains the difference between return value; and return; in Java:
Aspect |
return value; |
return; |
Usage |
Used in methods that have a return type (e.g., int, String, etc.) |
Used in void methods that do not return any value |
Purpose |
To return a value of the specified type to the caller |
To exit the method early without returning any value |
Return Type |
Must match the method's declared return type (e.g., int, String) |
No return type needed since it's a void method |
Example Method Type |
public int add(int a, int b) |
public void printMessage() |
Example Code |
return 10; |
return; |
What it returns |
A value of the specified type (e.g., integer, string) |
No value is returned |
When to Use |
When the method is designed to compute or provide a result |
When you want to terminate the method without returning anything |
Q. Can a method have multiple return statements?
Yes, a method can have multiple return statements, but only one will be executed in a single method call. This is often used in conditional statements to exit the method early based on specific conditions. For Example-
public int findMax(int a, int b) {
if (a > b) return a; // Returns if condition is true
else return b; // Returns if condition is false
}
Q. What happens if a method with a return type does not have a return statement?
If a method is defined to return a value (e.g., int, String, etc.), it must include a return statement that provides a value of the expected type. Failing to include a return statement in such methods will result in a compile-time error. For Example- A method defined as public int getAge() must return an integer, and if no return is provided, the code will not compile.
Q. How does the return statement work in recursion?
In recursive methods, the return statement is essential for defining the base case and stopping the recursion. If a method continues to call itself indefinitely, it can result in a stack overflow error. By using return in the base case, we ensure that the recursion halts and returns a result to the previous method calls. For Example-
public int factorial(int n) {
if (n == 0) return 1; // Base case
return n * factorial(n - 1); // Recursive call
}
Q. Can I return an object from a method in Java?
Yes, methods in Java can return objects. You can return instances of classes or even complex data types, such as collections or arrays. When returning an object, the method’s return type must be declared as the type of the object. For Example-
public Person getPerson() {
return new Person("John", 30);
}
Q. What happens if a return statement is placed after the method's return type is already met?
If a return statement is placed after the method has already returned a value, the code will not compile. A method can only return once during its execution, and once a return statement is executed, the method's flow is terminated. Any code, including additional return statements after the first one, is considered unreachable and will result in a compile-time error.
With this, we have come to an end in our discussion of the return statement in Java. Here are a few other topics that you might be interested in reading:
- Final, Finally & Finalize In Java | 15+ Differences With Examples
- Identifiers In Java | Types, Conventions, Errors & More (+Examples)
- Top 15+ Difference Between C++ And Java Explained! (+Similarities)
- Method Overloading In Java | Ways, Rules & Type Promotion (+Codes)
- This Keyword In Java | Syntax, Best Practices & More (+Examples)
- 90+ Java Collections Interview Questions (+Answers) You Must Know
I’m a Computer Science graduate with a knack for creative ventures. Through content at Unstop, I am trying to simplify complex tech concepts and make them fun. When I’m not decoding tech jargon, you’ll find me indulging in great food and then burning it out at the gym.
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
Comments
Add comment