Multithreading In Java - Complete Guide With Uses & Code Examples
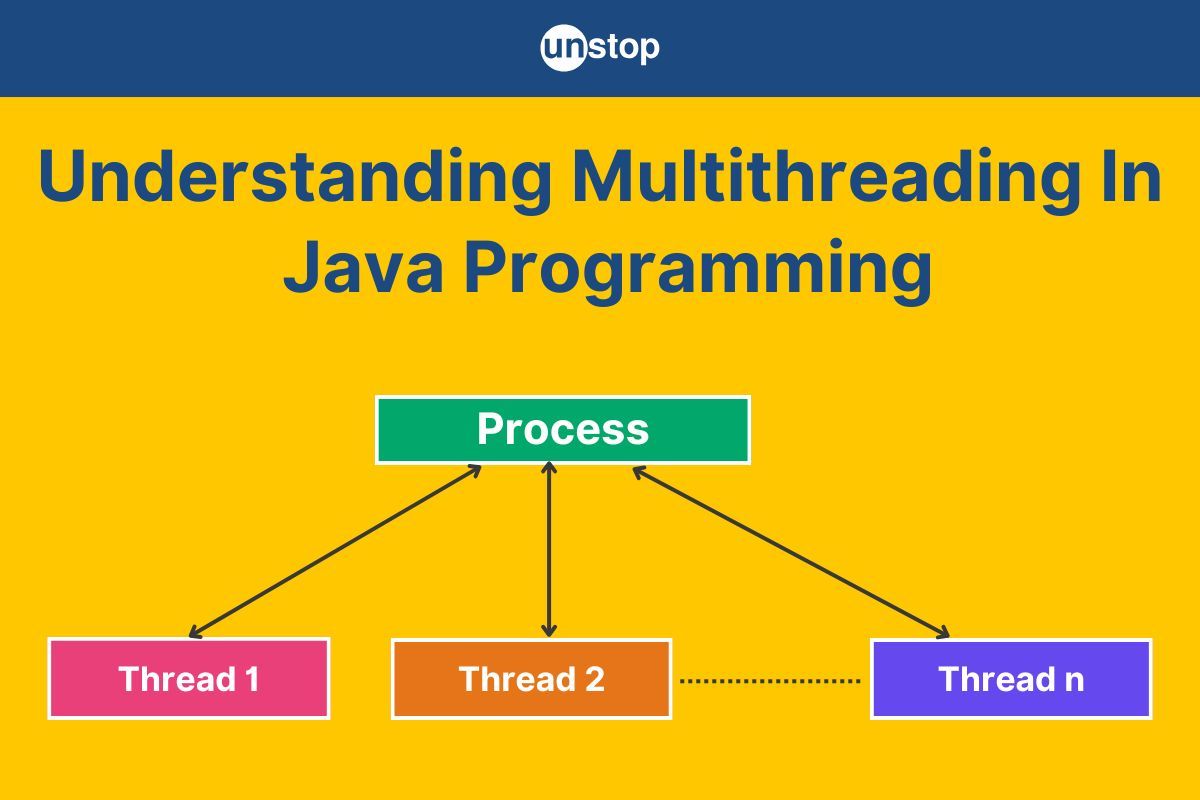
Multithreading is a powerful feature in Java that allows concurrent execution of two or more threads, providing better resource utilization and enhancing the performance of applications. By enabling multiple tasks to run simultaneously, multithreading helps in improving efficiency, especially in CPU-bound or I/O-bound processes.
In this article, we will explore the concept of multithreading in Java, discussing its benefits, implementation, and the various techniques available for managing threads. We will also understand the core components such as the Thread class and the Runnable interface, as well as explore practical use cases and best practices for writing thread-safe code.
Understanding Multithreading In Java
Multithreading is a programming technique where multiple threads are executed concurrently within a single process. A thread is the smallest unit of a program that can be scheduled for execution. Multithreading enables better utilization of CPU resources, allows for simultaneous operations, and improves the performance of applications, especially on systems with multiple processors or cores.
Key Benefits Of Multithreading In Java:
- Efficient Resource Use: Utilizes CPU more effectively by performing multiple tasks in parallel.
- Responsiveness: Keeps applications responsive by running background tasks (e.g., downloading a file) while the main program continues.
- Scalability: Leverages multi-core processors for better performance.
Real-Life Example: Online Food Ordering System:
Imagine an app where a user places an order, the payment is processed, and the delivery status is updated simultaneously. Multithreading can handle these tasks efficiently:
- Thread 1: Fetch and display menu items.
- Thread 2: Process payment.
- Thread 3: Track delivery status in real-time.
Methods Of Multithreading In Java (Examples)
In Java programming, multithreading can be achieved using various methods. These methods provide flexibility in implementing threads to run tasks concurrently. Here's an overview of the primary methods:
1. Extending The Thread Class
In this approach, you create a new class that extends the Thread class and override the run() method to define the task to be performed by the thread.
Code Example:
Output:
Thread is running: 1
Thread is running: 2
Thread is running: 3
Thread is running: 4
Thread is running: 5
Explanation:
In the above code example-
- We create a custom thread by extending the Thread class and overriding its run() method, which contains the code executed when the thread runs.
- Inside the run() method, we use a for loop that iterates from 1 to 5, printing a message with the iteration number to simulate some work.
- To mimic a time-consuming task, we use Thread.sleep(500) to introduce a delay of 500 milliseconds between iterations.
- We handle any InterruptedException that might occur during sleep by catching it in a try-catch block and printing an interruption message.
- In the main() method, we instantiate our custom thread class MyThread, creating a thread object.
- When we call start() on the thread object, it triggers the thread's run() method to execute in parallel with the main program.
Explore this amazing course and master all the key concepts of Java programming effortlessly!
2. Implementing The Runnable Interface
Here, you create a class that implements the Runnable interface and define the task inside the run() method. You then pass an instance of this class to a Thread object.
Code Example:
Output:
Runnable thread is running: 1
Runnable thread is running: 2
Runnable thread is running: 3
Runnable thread is running: 4
Runnable thread is running: 5
Explanation:
In the above code example-
- We define a custom task by implementing the Runnable interface and overriding its run() method, which contains the code executed when the thread runs.
- Inside the run method, we use a for loop that iterates from 1 to 5, printing a message with the iteration number to simulate some work.
- To mimic a time-consuming task, we use Thread.sleep(500) to introduce a delay of 500 milliseconds between iterations.
- We handle any InterruptedException during sleep with a try-catch block, printing an interruption message if it occurs.
- In the main() method, we create an instance of MyRunnable, representing the task we want to run in a thread.
- We pass the MyRunnable instance to a Thread object, associating the task with the thread.
- Calling start on the Thread object begins execution of the thread, invoking the run() method of the MyRunnable instance in parallel with the main program.
Difference Between Multithreading And Multitasking In Java
Multithreading refers to the ability of a CPU or a single process to manage multiple threads within it. Multitasking is the ability of an operating system to execute multiple processes simultaneously. Here are the key differences between the two:
Feature |
Multithreading |
Multitasking |
Definition |
Managing multiple threads within a single process. |
Managing multiple processes simultaneously. |
Granularity |
Threads are lightweight sub-units of a process. |
Processes are independent, heavier units. |
Memory Usage |
Threads share the same memory space. |
Each process has its own memory space. |
Context Switching |
Faster as threads share resources. |
Slower due to the overhead of switching memory spaces. |
Communication |
Easier between threads since they share memory. |
More complex due to separate memory spaces. |
Overhead |
Minimal, as threads are smaller and share resources. |
Higher, as each process requires its own resources. |
Example |
A browser downloading files, rendering pages, and running scripts in parallel. |
Running a browser, media player, and text editor simultaneously. |
Use Case |
Optimizing tasks within a single application. |
Running multiple applications on a system. |
Dependency |
Threads are part of the same process, sharing dependencies. |
Processes are independent and isolated. |
Think of multithreading as multiple cooks (threads) in the same kitchen (process) sharing the same set of ingredients and tools to prepare different dishes. Meanwhile, multitasking is like multiple restaurants (processes) operating independently, each with its own kitchen and resources.
Handling Exceptions In Multithreading
When working with multithreading, exceptions in a thread do not propagate to other threads or the main thread. Instead, they are confined to the thread where they occur. To handle exceptions properly in multithreading:
- Wrap the code in the run() method or the task's logic in a try-catch block.
- Ensure exceptions are logged or managed appropriately to prevent silent failures.
Code Example:
Output:
Thread started.
Exception in thread: / by zero
Thread finished.
Main thread continues execution.
Explanation:
In the above code example-
- We create a custom thread by extending the Thread class and overriding the run() method, which defines the thread's behavior.
- Inside the run() method, we print a message indicating the thread has started.
- We then perform a calculation that causes an ArithmeticException by attempting to divide 10 by 0.
- Since an exception occurs, the subsequent statement to print the result does not execute.
- We handle the ArithmeticException in a try-catch block, printing the exception message when it occurs.
- The finally block ensures that the message "Thread finished." is printed regardless of whether an exception occurred or not.
- In the main() method, we create an instance of our MyThread class and start the thread, invoking its run() method in parallel with the main program.
- The main thread continues its execution independently and prints "Main thread continues execution." while the custom thread handles its task.
Sharpen your coding skills with Unstop's 100-Day Coding Sprint and compete now for a top spot on the leaderboard!
Best Practices For Multithreading In Java
Multithreading can enhance performance and responsiveness, but improper handling can lead to complex bugs like deadlocks, race conditions, and unpredictable behavior. Here are some best practices:
- Minimize Shared Data: It reduces the chance of race conditions. Prefer local variables over shared variables. If sharing is unavoidable, use synchronization mechanisms.
- Use High-Level Concurrency APIs: Use the java.util.concurrent package for thread-safe collections, locks, thread pools, and other utilities. Example: Use ExecutorService for managing threads instead of manually creating them.
- Avoid Busy Waiting: It wastes CPU cycles. Use proper synchronization tools like wait(), notify(), or modern alternatives like CountDownLatch or Semaphore.
- Properly Handle Exceptions: Catch and handle exceptions within threads using a try-catch block. For centralized handling, use Thread.UncaughtExceptionHandler.
- Use Thread Pools: Efficiently reuses threads, avoiding the overhead of thread creation. Use Executors.newFixedThreadPool() or Executors.newCachedThreadPool().
- Avoid Nested Locks: Prevents deadlocks. Always acquire locks in the same order across threads to avoid circular dependencies.
- Leverage Synchronization Wisely: Use synchronization (synchronized blocks, ReentrantLock) to ensure thread safety, but minimize its scope to reduce contention.
- Use Volatile for Shared Variables: Use the volatile keyword for variables accessed by multiple threads to ensure visibility across threads.
- Prefer Immutable Objects: Immutable objects are inherently thread-safe. Design classes with final fields and no setters.
- Test for Thread Safety: Simulate concurrent environments using tools like java.util.concurrent.ThreadLocalRandom or testing frameworks such as Concurrency Test Framework.
- Avoid Thread Priority Manipulation: Thread priority may not behave consistently across platforms; rely on proper thread design instead.
- Use Daemon Threads for Background Tasks: Daemon threads are automatically terminated when the main program exits. Use them for tasks like monitoring and logging.
- Graceful Shutdown: Always shut down threads or thread pools gracefully using methods like shutdown() or shutdownNow().
- Monitor Performance: Use profiling tools like JVisualVM or Java Mission Control to monitor thread activity and identify bottlenecks.
- Keep Code Readable: Document threading logic and use meaningful names for threads to make the code maintainable.
Real-World Use Cases of Multithreading In Java
Multithreading is widely used to improve the performance, responsiveness, and scalability of applications. Below are some common real-world scenarios where multithreading is essential:
1. Web Servers and Application Servers
- Servers need to handle multiple client requests simultaneously. Each request can be processed in a separate thread.
- Example: A web server like Apache or Tomcat processes HTTP requests concurrently.
- Benefit: Allows hundreds or thousands of users to interact with the server without waiting for others.
2. Background Tasks in Desktop Applications
- Applications often perform background tasks like file saving, loading, or data syncing. Multithreading ensures these tasks don’t block the UI.
- Example: An IDE like IntelliJ or Eclipse uses threads to index files and keep the UI responsive.
- Benefit: Enhances user experience by avoiding freezes or delays.
3. Real-Time Gaming
- Games rely on multithreading to handle rendering, physics calculations, and user input concurrently.
- Example: A 3D game renders graphics in one thread while handling player input and physics simulation in others.
- Benefit: Achieves smooth gameplay and responsiveness.
4. Parallel Data Processing
- Large datasets can be processed in parallel using threads, especially in big data and analytics applications.
- Example: A program processes logs or performs matrix operations using multithreading for speed.
- Benefit: Speeds up data analysis and computation-intensive tasks.
5. Chat Applications
- Chat servers use threads to handle multiple users sending and receiving messages concurrently.
- Example: Applications like WhatsApp or Slack use multithreading for real-time message delivery.
- Benefit: Ensures smooth communication and fast response times.
6. Asynchronous File Download and Upload
- Download managers or cloud storage systems use threads to handle multiple file transfers simultaneously.
- Example: A download manager splits a large file into parts and downloads them using multiple threads.
- Benefit: Speeds up downloads and improves resource utilization.
7. Real-Time Monitoring and Alerts
- Monitoring systems use threads to collect, analyze, and respond to data streams in real time.
- Example: Network monitoring tools analyze multiple data sources simultaneously.
- Benefit: Provides timely alerts and insights.
8. Multimedia Applications
- Multimedia applications play audio and video streams using separate threads for decoding and rendering.
- Example: A media player decodes video in one thread and audio in another for seamless playback.
- Benefit: Ensures smooth audio-video synchronization.
9. Financial Systems
- Stock trading platforms and banking systems use multithreading to process transactions and updates concurrently.
- Example: A stock trading application updates stock prices in real time while processing buy/sell orders.
- Benefit: Handles high transaction volumes efficiently.
10. Machine Learning and AI
- Multithreading is used to train machine learning models by parallelizing tasks like gradient computation and matrix operations.
- Example: A Java-based ML library parallelizes matrix multiplication for faster model training.
- Benefit: Speeds up computation-heavy tasks.
Are you looking for someone to answer all your programming-related queries? Let's find the perfect mentor here.
Conclusion
Multithreading in Java is a powerful feature that enables efficient execution of concurrent tasks, making applications more responsive, scalable, and capable of handling complex operations. By utilizing threads effectively, developers can improve performance in areas like web servers, real-time gaming, data processing, and more. However, multithreading also introduces challenges like race conditions, deadlocks, and resource contention, which require careful handling and adherence to best practices.
With proper understanding and implementation, multithreading can transform applications, ensuring they are optimized for today's multi-core systems and diverse use cases. Whether building responsive user interfaces, processing massive datasets, or managing real-time systems, multithreading is an essential skill for Java developers, enabling them to tackle complex problems with efficiency and elegance.
Frequently Asked Questions
Q. What is the difference between Multithreading and Multitasking?
- Multithreading is the ability of a program to execute multiple threads simultaneously within the same process. Threads share the same memory and resources, making it lightweight.
- Multitasking refers to the execution of multiple independent processes by the operating system. Each process has its own memory space, and switching between them is more resource-intensive compared to threads.
Multithreading is used to optimize performance within a single application, while multitasking manages multiple applications running concurrently.
Q. What are the main advantages of using Multithreading in Java?
The main advantage of using multithreading in java are:
- Improved Performance: Threads can perform multiple tasks concurrently, making efficient use of CPU resources.
- Responsiveness: Keeps applications responsive by performing long-running tasks in the background (e.g., file downloads, data syncing).
- Resource Sharing: Threads share memory and resources, reducing overhead compared to processes.
- Scalability: Makes applications scalable for multi-core processors by parallelizing tasks.
- Cost Efficiency: Reduces the cost of creating separate processes for each task.
Q. What are the main challenges in implementing Multithreading?
The main challenges encountered during the implementation of multithreading are:
- Race Conditions: Multiple threads accessing shared resources simultaneously can lead to inconsistent results.
- Deadlocks: Threads waiting indefinitely for resources held by each other.
- Thread Safety: Ensuring that shared resources are accessed in a synchronized manner.
- Complex Debugging: Multithreaded applications are harder to debug due to non-deterministic behavior.
- Overhead: Creating and managing threads involves overhead, which can negate the benefits if not used judiciously.
Q. What are the common methods to create threads in Java? Which one is preferred?
Some of the preferred common methods are:
- Extending Thread Class: Override the run() method of the Thread class and start the thread using start().
- Implementing Runnable Interface: Implement the run() method of the Runnable interface and pass it to a Thread object.
- Using Callable and Future: For tasks that return results or throw exceptions.
- Using ExecutorService: For managing thread pools and reusing threads.
Preferred Approach: The ExecutorService framework is recommended as it provides better control, scalability, and ease of management compared to manual thread creation.
Q. How does Java handle thread synchronization?
Java provides several mechanisms for thread synchronization to prevent race conditions:
- The synchronized Keyword: Used to lock methods or code blocks, ensuring that only one thread can execute the block at a time.
- ReentrantLock Class: Provides advanced synchronization features like try-lock and fairness policies.
- Volatile Variables: Ensures visibility of changes to variables across threads.
- Atomic Variables (e.g., AtomicInteger): Provides thread-safe operations without explicit synchronization.
- Wait/Notify Mechanism: Coordinates thread communication within synchronized blocks.
Q. What is the role of the java.util.concurrent package in Multithreading?
The java.util.concurrent package simplifies multithreading by providing high-level abstractions for managing threads and concurrent tasks:
- Thread Pools: Classes like ExecutorService and ForkJoinPool allow efficient thread management.
- Locks: Provides ReentrantLock, ReadWriteLock, and other locking mechanisms for advanced control.
- Synchronizers: Tools like Semaphore, CountDownLatch, and CyclicBarrier help coordinate thread execution.
- Concurrent Collections: Thread-safe collections like ConcurrentHashMap and CopyOnWriteArrayList.
- Atomic Variables: For lock-free thread-safe operations on single variables.
Q. What are the differences between start() and run() methods in the Thread class?
1. The start() method:
- Starts a new thread and invokes the run() method in that thread.
- Creates a separate call stack for the new thread.
- Proper way to initiate a thread.
2. The run() method:
- Executes the run() method in the current thread, not a new thread.
- Used for defining the thread's behavior but does not start a new thread.
- Key Difference: Always use start() to execute multithreading; calling run() directly executes the code in the main thread, defeating the purpose of threading.
With this, we conclude our discussion on the concept of multithreading in Java. Here are a few other topics that you might be interested in reading:
- Final, Finally & Finalize In Java | 15+ Differences With Examples
- Super Keyword In Java | Definition, Applications & More (+Examples)
- How To Find LCM Of Two Numbers In Java? Simplified With Examples
- How To Find GCD Of Two Numbers In Java? All Methods With Examples
- Throws Keyword In Java | Syntax, Working, Uses & More (+Examples)
- 10 Best Books On Java In 2024 For Successful Coders
- Difference Between Java And JavaScript Explained In Detail
- Top 15+ Difference Between C++ And Java Explained! (+Similarities)
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment