Java Programming Language
Table of content:
- History Of Java Programming Langauge
- Infographic For History Of Java
- What’s In The Name | History Of Java
- Key Features Of Java
- Advantages And Disadvantages Of Java
- The Version History Of Java Langauge
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is JDK?
- How To Download Java Development Kit (JDK) For Windows, MacOS, and Linux?
- Set Environment Variables In Java
- How To Install Java (JDK) On Windows 64-Bit Machine?
- How To Install Java (JDK) On Linux?
- How To Install Java (JDK) On macOS?
- How To Test Java Installation?
- How To Write Your First Java Program On Linux OS?
- Conclusion
- Frequently Asked Questions
Table of content:
- Java Programming Language | An Introduction
- 15 Key Features Of Java
- Write Once Run Anywhere (WORA) | Features Of Java
- Java Editions
- 5 New Features Of JAVA 8
- 5 New Features Of JAVA 11
- What Makes Java Popular?
- Conclusion
- Frequently Asked Questions
Table of content:
- What is Java?
- Advantages of Java
- Disadvantages of Java
Table of content:
- What Is Java Programming?
- Role Of Integrated Development Environments (IDEs) In Java Development
- 15 Best Java IDE For Developers
- In-Depth Comparison Table
- Conclusion
- Frequently Asked Questions
Table of content:
- Key Differences Between Java And Core Java
- What Is Java?
- What Is Core Java?
- Applications Of Java
- Applications Of Core Java
- When To Use Java?
- When To Use Core Java?
- Conclusion
- Frequently Asked Questions
Table of content:
- What Are Variables In Java Language?
- How To Declare Variables In Java Programs?
- How To Initialize Variables In Java?
- Naming Conventions For Variables In Java
- Types Of Variables In Java
- Local Variables In Java
- Instance Variables In Java
- Static Variables In Java
- Final Variables In Java
- Scope and Lifetime of Variables In Java
- Data Types Of Variables In Java (Primitive & Non-primitive)
- Java Variable Type Conversion & Type Casting
- Working With Variables In Java (Examples)
- Access Modifiers & Variables In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Are Identifiers In Java?
- Syntax Rules For Identifiers In Java
- Valid Identifiers in Java
- Invalid Identifiers in Java
- Java Reserved Keywords
- Naming Conventions & Best Practices For Identifiers In Java
- What Is An Identifier Expected Error In Java?
- Reasons The Identifier Expected Error Occurs
- How To Fix/ Resolve Identifier Expected Errors In Java?
- Conclusion
- Frequently Asked Questions
Table of content:
- What Are Data Types In Java?
- Primitive Data Types In Java
- Non-Primitive Data Types In Java
- Key Differences Between Primitive And Non-Primitive Data Types In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Are Operators In Java?
- Types Of Operators In Java
- Unary Operators In Java
- Arithmetic Operators In Java
- Assignment Operators In Java
- Relational Operators In Java
- Logical Operators In Java
- Bitwise Operators In Java
- Shift Operators In Java
- Increment & Decrement Operators In Java
- Ternary Operator In Java
- Instanceof Operator In Java
- Precedence & Associativity Of Java Operators
- Advantages & Disadvantages Of Operators In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is A Return Statement In Java?
- Use Cases Of Return Statements In Java
- Returning A Value From A Method In Java
- Returning A Class Object In Java
- Returning Void (No Value) In Java
- Advantages Of Using Return Statements In Java
- Limitations Of Using Return Statements In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Are Keywords In Java?
- List Of Keywords In Java
- Detailed Overview Of Java Keywords With Examples
- What If When Keywords In Java Are Used As Variable Names?
- Difference Between Identifiers & Keywords In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Abstract Keyword In Java?
- Use Of Abstract Keyword In Java
- Abstract Methods In Java
- Abstract Classes In Java
- Advantages Of Abstract Keyword In Java
- Disadvantages Of Abstract Keyword In Java
- Abstract Classes Vs. Interfaces In Java
- Real-World Applications Of Abstract Keyword
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is throws Keyword In Java?
- How Does The throws Keyword Work?
- Throwing A Checked Exception Using throws In Java
- Throwing Multiple Exceptions Using throws In Java
- Throwing A Custom Exception Using throws In Java
- When To Use The throws Keyword In Java
- Difference Between throw and throws Keyword In Java
- Best Practices For Using The throws Keyword In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is The Volatile Keyword In Java?
- How Does Volatile Keyword In Java Work?
- Using Volatile Keyword In Java To Control Thread Execution
- Using Volatile Keyword In Java To Signal Between Multiple Threads
- Difference Between Synchronization And Volatile Keyword
- Common Mistakes And Best Practices While Using Volatile Keyword In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding Super Keyword In Java
- Super Keyword In Java With Instance Variables
- Super Keyword In Java With Method Overriding
- Super Keyword In Java With Constructor Chaining
- Applications Of Super Keyword In Java
- Difference Between This And Super Keyword In Java
- Advantages Of Using Super Keyword In Java
- Limitations And Considerations Of Super Keyword In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding This Keyword In Java
- Uses Of This Keyword In Java
- Using This Keyword For Referencing Instance Variables
- Using This Keyword For Invoking A Constructor
- Using This Keyword For Invoking A Method
- Using This Keyword With Getters And Setters
- Difference Between This And Super Keyword In Java
- Best Practices For Using This Keyword In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is new Keyword In Java?
- Uses Of The new Keyword In Java
- Memory Management With new Keyword In Java
- Example 1: Creating An Object Of A Class Using new Keyword In Java
- Example 2: Creating An Array Using The new Keyword In Java
- Best Practices For Using new Keyword In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is The Transient Keyword In Java?
- Real-Life Example Of The Transient Keyword In Java
- When To Use The Transient Keyword In Java
- Example 1: Effect Of Transient Keyword On Serialization In Java
- Example 2: Skipping Sensitive Data During Serialization With Transient Keyword In Java
- Using Transient With Final Keyword In Java
- Using Transient With Static Keyword
- Difference Between Transient And Volatile Keyword In Java
- Advantages And Disadvantages Of Transient Keyword In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Static Keyword In Java?
- Characteristics Of Static Keyword In Java
- Static Variables In Java
- Static Method In Java
- Static Blocks In Java
- Static Classes In Java
- Static Variables Vs Instance Variables In Java
- Advantages Of Static Keyword In Java
- Disadvantages Of Static Keyword In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is A Static Method In Java?
- Use Cases Of Static Method In Java
- Using Static Method In Java To Create A Utility Class
- Using Static Method In Java To Implement The Singleton Design Pattern
- Difference Between Static And Instance Methods In Java
- Limitations Of Static Method In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding Final Keyword In Java
- Final Variables In Java
- Final Methods In Java
- Final Classes In Java
- Difference Between Static And Final Keyword In Java
- Uses Of Final Keyword In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Key Difference Between final, finally, And finalize In Java
- What Is final Keyword In Java?
- What Is finally Keyword In Java?
- What Is finalize Keyword In Java?
- When To Use Which Keyword In Java?
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding The extends Keyword In Java?
- Use Of extends Keyword In Java
- Using Java extends To Implement Single Inheritance
- Using Java extends With Interfaces (Default Methods)
- Overriding Using extends Keyword In Java
- Difference Between extends And implements In Java
- Real World Applications Of Extends Keyword In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Decision Making Statement In Java?
- If Statement In Java
- If-Else Statement In Java
- Else-If Ladder In Java
- Switch Statement In Java
- Ternary/Conditional Operator (?:) In Java
- Best Practices For Writing Decision Making Statements In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Are Control Statements in Java?
- Decision-Making Control Statements In Java
- Looping Control Statements In Java
- Jump (Branching) Control Statements In Java
- Application Of Control Statements In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is The Break Statement In Java?
- Working Of The Break Statement In Java
- Using Java Break Statement With Loops
- Using Java Break Statement With Switch Statement
- Using Java Break Statement With Infinite Loops
- Common Pitfalls While Using Break Statements In Java
- Best Practices For Using The Break Statement In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Switch Statement In Java?
- Working Of The Switch Statement In Java
- Example Of Switch Statement In Java
- Java Switch Statement With String
- Java Nested Switch Statements
- Java Enum In Switch Statement
- Java Wrapper Classes In Switch Statements
- Uses Of Switch Statement In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Syntax Of main() Method In Java
- public Specifier – Main Method In Java
- static Keyword – Main Method In Java
- void Return Type Of Main Method In Java
- The main Identifier – Main Method In Java
- String[] args In Main Method In Java
- The Role Of Java Virtual Machine (JVM)
- Running Java Programs Without The Main Method
- Variations In Declaration Of Main Method In Java
- Overloading The Main Method In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Method Overriding In Java?
- Example Of Method Overriding In Java
- Ideal Use Cases Of Method Overriding In Java
- Rules For Method Overriding In Java
- Super Keyword & Method Overriding In Java
- Constructor & Method Overriding In Java
- Exception Handling In Method Overriding In Java
- Access Modifiers In Method Overriding In Java
- Advantages & Disadvantages Of Method Overriding In Java
- Difference Between Method Overloading Vs. Method Overriding In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Method Overloading In Java?
- Different Ways Of Method Overloading In Java
- Overloading The main() Method In Java
- Type Promotion & Method Overloading In Java
- Null Error & Method Overloading In Java
- Advantages Of Method Overloading In Java
- Disadvantages Of Method Overloading In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Difference Between Overloading And Overriding In Java (Comparison Table)
- What Is Method Overloading In Java?
- What Is Method Overriding In Java?
- Key Differences Between Overloading & Overriding In Java Explained
- Difference Between Overloading And Overriding In Java Code Example
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is A One-Dimensional Array In Java?
- Key Characteristics Of One-Dimensional Arrays In Java
- Declaration Of One-Dimensional Array In Java
- Initialization Of One-Dimensional Array In Java
- Common Operations On One-Dimensional Array In Java
- Advantages Of One-Dimensional Arrays In Java
- Disadvantages Of One-Dimensional Arrays In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is A Multidimensional Array In Java?
- Difference Between Single-Dimensional And Multidimensional Arrays In Java
- Declaring Multidimensional Arrays In Java
- Initializing Multidimensional Arrays In Java
- Accessing And Manipulating Elements In Multidimensional Arrays In Java
- Working Of Multidimensional Arrays With Jagged Arrays In Java
- Why Use Multidimensional Arrays In Java?
- Limitations Of Multidimensional Arrays In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Are Jagged Arrays In Java?
- Comparison With Regular Multi-Dimensional Arrays
- Declaring Jagged Arrays In Java
- Initialization Of Jagged Arrays In Java
- Printing Elements Of A Jagged Array In Java
- Accessing And Modifying Elements Of A Jagged Array In Java
- Advantages Of Jagged Arrays In Java
- Disadvantages Of Jagged Arrays In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Array Of Objects In Java?
- Declare And Initialize An Array Of Object In Java
- Example Of An Array Of Objects In Java
- Sorting An Array Of Objects In Java
- Passing Arrays Of Objects To Methods In Java
- Returning Arrays Of Objects From Methods In Java
- Advantages Of Arrays Of Objects In Java
- Disadvantages Of Arrays Of Objects In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is A Dynamic Array In Java?
- Why Use Dynamic Array In Java?
- What Is The Size And Capacity Of A Dynamic Array In Java?
- How To Create A Dynamic Array In Java?
- Managing Dynamic Data Input In Java
- Storing And Processing Real-Time Data In Java
- Use Cases Of Dynamic Arrays In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Why Return An Array In Java?
- How To Return An Array In Java
- Example 1: Returning An Array Of First N Squares
- Example 2: Doubling the Values of an Array
- Common Scenarios For Returning Arrays In Java
- Points To Remember When Returning Arrays In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding ArrayList In Java
- Differences Between Arrays And ArrayList In Java
- Returning An ArrayList In Java
- Common Use Cases For Returning An ArrayList In Java
- Pitfalls To Avoid When Returning An ArrayList In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is A Thread In Java?
- Thread Vs Process
- What is a Thread Life Cycle In Java?
- What Are Thread Priorities?
- Creating Threads In Java
- Java Thread Methods
- Commonly Used Constructors In Thread Class
- Thread Synchronization In Java
- Common Challenges Faced While Using Threads In Java
- Best Practices For Using Threads In Java
- Real-World Applications Of Threads In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding Multithreading In Java
- Methods Of Multithreading In Java (Examples)
- Difference Between Multithreading And Multitasking In Java
- Handling Exceptions In Multithreading
- Best Practices For Multithreading In Java
- Real-World Use Cases of Multithreading In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Thread Priority In Java?
- Built-In Thread Priority Constants In Java
- Thread Priority: Setter & Getter Methods
- Limitations Of Thread Priority In Java
- Best Practices For Using Thread Priority In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Thread Synchronization In Java?
- The Need For Thread Synchronization In Java
- Types Of Thread Synchronization In Java
- Mutual Exclusion In Thread Synchronization In Java
- Coordination Synchronization (Thread Communication) In Java
- Advantages Of Thread Synchronization In Java
- Disadvantages Of Thread Synchronization In Java
- Alternatives To Synchronization In Java
- Deadlock And Thread Synchronization In Java
- Real-World Use Cases Of Thread Synchronization In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is A Daemon Thread In Java?
- User Threads Vs. Daemon Threads In Java
- Methods For Daemon Threads In The Thread Class
- Creating Daemon Threads In Java
- Checking The Daemon Status Of A Thread
- Exceptions In Daemon Threads
- Limitations Of Daemon Threads In Java
- Practical Applications Of Daemon Threads In Java
- Common Mistakes To Avoid When Working With Daemon Threads In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Why Do Threads Need To Communicate?
- Understanding Inter Thread Communication In Java
- The wait() Method In Inter-Thread Communication
- The notify() Method In Inter-Thread Communication
- The notifyAll() Method In Inter-Thread Communication
- Difference Between wait() And sleep() Methods In Java
- Best Practices For Inter Thread Communication In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding The Factorial Concept
- Approaches To Implementing Factorial In Java
- Find Factorial In Java Using Iterative Approach (Using a Loop)
- Find Factorial In Java Using Recursive Approach
- Complexity Analysis Of Factorial Programs In Java
- Applications Of Factorial Program In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding The Leap Year Concept
- Approach To Check A Leap Year In Java
- Alternative Approach To Check A Leap Year In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is The Difference Between JDK, JRE, and JVM?
- What Is JVM (Java Virtual Machine)?
- What Is JRE (Java Runtime Environment)?
- What Is JDK (Java Development Kit)?
- Understanding The Difference Between JDK, JRE, And JVM
- Comparison Table For Difference Between JDK, JRE, And JVM
- Conclusion
- Frequently Asked Questions
Table of content:
- Difference Between Abstraction And Encapsulation In Java
- Understanding Abstraction In Java
- Understanding Encapsulation In Java
- When To Use Abstraction And Encapsulation?
- Conclusion
- Frequently Asked Questions
Table of content:
- Differences Between Abstract Class And Interface In Java
- What Is An Abstract Class In Java?
- What Is An Interface In Java?
- When To Use An Abstract Class?
- When To Use Interface?
- Compatibility Between Abstract Class And Interface In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Error Vs. Exception In Java
- What Is Error In Java?
- What Is Exception In Java?
- Best Practices For Handling Exceptions In Java
- Why Errors Should Not Be Handled In Java?
- Conclusion
- Frequently Asked Questions
Table of content:
- Key Differences: Java Vs. JavaScript
- What Is Java?
- What Is JavaScript?
- Difference Between Java And JavaScript Explained
- Conclusion
- Frequently Asked Questions
Table of content:
- Brief Introduction To C++
- Brief Introduction To Java
- Difference Between C++ and Java
- Overview & Features Of C++ Language
- Overview & Features of Java Language
- Example of C++ and Java Program
- Key Difference Between C++ And Java Explained
- Similarities Between Java Vs. C++
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- Basic Java interview questions and answers
- Intermediate Java interview questions and answers
- Advanced Java interview questions and answers
Table of content:
- Difference between core Java and advanced Java
- Important Core Java Questions
- Tips for Preparing for Core Java
This Keyword In Java | Syntax, Best Practices & More (+Examples)
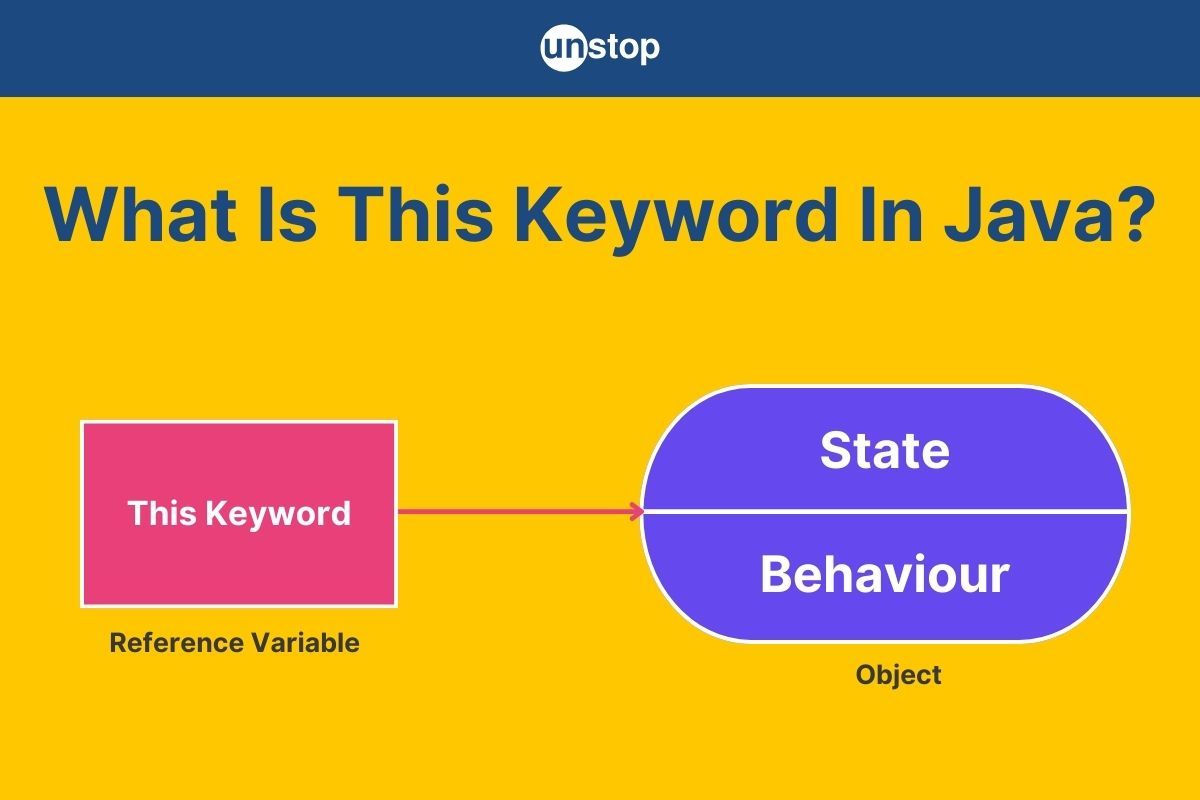
In Java, the this keyword is a powerful and versatile tool used to represent the current instance of a class. It allows us to differentiate between class attributes and parameters when they share the same name, invoke other constructors in the same class, and pass the current object as a parameter. Understanding the use of this is essential for mastering object-oriented programming in Java.
In this article, we'll explore the various applications of the this keyword, understand how it works, and see practical examples to enhance our knowledge.
Understanding This Keyword In Java
The this keyword in Java programming is a reference variable that refers to the current instance of a class. It plays a crucial role in object-oriented programming by helping us address ambiguity, reuse constructors, and achieve better code clarity. It’s automatically available in all non-static methods and constructors of a class.
Syntax Of This Keyword In Java
The this keyword has versatile uses in Java, and its syntax depends on the context where it is applied:
- Referencing the Current Object
- The this keyword refers to the object that is currently being operated upon.
- It’s commonly used when instance variables and parameters share the same name.
this.instanceVariable;
- Invoking Current Class Constructor
- We can call one constructor from another in the same class using this(). This is known as constructor chaining.
- It must be the first statement in the constructor.
this(parameters);
- Calling Current Class Methods
- The this keyword can call other methods of the same class explicitly.
this.methodName(parameters);
Uses Of This Keyword In Java
The this keyword is a fundamental part of Java, used to refer to the current instance of a class. It is versatile and plays a critical role in various aspects of object-oriented programming. Below are the primary uses of the this keyword:
- Referencing Current Class Instance Variables: Resolves ambiguity between instance variables and method parameters with the same name.
- Invoking Current Class Methods: Explicitly calls other methods within the same class.
- Invoking Current Class Constructors (Constructor Chaining): Allows calling one constructor from another within the same class.
- Passing Current Instance as an Argument: Passes the current object as a parameter to another method or constructor.
- Returning the Current Instance: Returns the current object from a method to enable method chaining.
- Avoiding Ambiguity: Differentiates between instance variables and local variables or parameters when they have the same name.
Explore this amazing course and master all the key concepts of Java programming effortlessly!
Using This Keyword For Referencing Instance Variables
The this keyword helps resolve ambiguity between instance variables and method parameters when they have the same name. By using this, we explicitly refer to the instance variable of the current object. This ensures the correct assignment or access to the instance variable.
Code Example:
class Employee {
private String name;
// Constructor to initialize name
public Employee(String name) {
this.name = name; // 'this.name' refers to the instance variable
}
// Method to display name
public void display() {
System.out.println("Employee Name: " + this.name);
}
}
public class Main {
public static void main(String[] args) {
Employee emp = new Employee("Alia"); // Create object
emp.display(); // Call display method
}
}
Output:
Employee Name: Alia
Explanation:
In the above code example-
- We begin by defining a class named Employee, which represents an employee in our program.
- Inside the Employee class, there’s a private member variable, name, to store the name of the employee. We make it private to ensure it’s only accessible within the Employee class.
- A constructor, Employee(String name), is provided to initialize the name variable when we create an object of the Employee class.
- To avoid ambiguity between the parameter name and the instance variable name, we use the this keyword to refer explicitly to the instance variable.
- We define a display() method to print the employee's name. This method uses System.out.println to display a message containing the name variable.
- In the Main class, we have the main() method, which serves as the entry point of our program.
- Inside the main method, we create an Employee object named emp by calling the constructor and passing "Alia" as the name.
- Finally, we call the display method on the emp object, which prints "Employee Name: Alia" to the console.
Using This Keyword For Invoking A Constructor
The this keyword can be used to invoke another constructor of the same class, enabling constructor chaining. This is helpful when multiple constructors need to share common initialization code. The this() call must always be the first statement in the constructor.
Code Example:
class Car {
private String brand;
private int year;
// Constructor 1: Default constructor
public Car() {
this("Unknown", 0); // Invoking parameterized constructor
}
// Constructor 2: Parameterized constructor
public Car(String brand, int year) {
this.brand = brand;
this.year = year;
}
// Method to display car details
public void displayDetails() {
System.out.println("Car Brand: " + this.brand + ", Year: " + this.year);
}
}
public class Main {
public static void main(String[] args) {
Car car1 = new Car(); // Calls default constructor
Car car2 = new Car("Toyota", 2021); // Calls parameterized constructor
car1.displayDetails();
car2.displayDetails();
}
}
Output:
Car Brand: Unknown, Year: 0
Car Brand: Toyota, Year: 2021
Explanation:
In the above code example-
- We start by defining a class named Car to represent a car, with private fields brand and year to store its brand and manufacturing year.
- Keeping these fields private ensures they can only be accessed and modified through the methods defined in the class.There are two constructors in the Car class.
- The first constructor is a default constructor, which doesn't take any parameters. It initializes the car's brand to "Unknown" and the year to 0 by invoking the second constructor using the this keyword.
- The second constructor is a parameterized constructor that accepts a brand and a year as arguments, allowing us to initialize a car with specific details.
- In the parameterized constructor, we use the this keyword to differentiate between the constructor parameters and the instance variables of the same name.
- The displayDetails() method prints the car's brand and manufacturing year in a user-friendly format. It uses System.out.println for output.
- In the Main class, we define the main() method, which is the entry point of our program.
- Within main, we create two Car objects. The first, car1, uses the default constructor, while the second, car2, uses the parameterized constructor with "Toyota" and 2021 as values.
- Finally, we call the displayDetails method on both car1 and car2, displaying their respective details. The default constructor initializes car1 with "Unknown" and 0", while car2displays "Toyota" and2021".
Using This Keyword For Invoking A Method
The this keyword is used to explicitly invoke another method of the current class. It ensures clarity when chaining methods or when there is potential ambiguity in accessing methods within the same class.
Code Example:
class Calculator {
private int result;
// Method to add numbers
public void add(int a, int b) {
this.result = a + b; // Set result
this.display(); // Invoking display() method
}
// Method to display the result
public void display() {
System.out.println("Result: " + this.result);
}
}
public class Main {
public static void main(String[] args) {
Calculator calc = new Calculator();
calc.add(5, 10); // Call add method
}
}
Output:
Result: 15
Explanation:
In the above code example-
- We define a class named Calculator to perform basic arithmetic operations, with a private field result to store the calculation result.
- The result field is private to ensure it can only be accessed and modified through the methods within the class.
- The add() method takes two integers, a and b, as parameters and computes their sum. The result of the addition is stored in the result field.
- Inside the add method, we use the this keyword to refer to the result instance variable explicitly.
- After calculating the result, the add method calls the display method to output the result. This demonstrates how methods within the class can interact.
- The display method prints the current value of the result field using System.out.println.
- In the Main() class, we define the main() method, which serves as the program's entry point.
- Within the main method, we create an instance of the Calculator class named calc.
- We then call the add() method on the calc object with arguments 5 and 10. The add method computes the sum and displays the result, which is printed as "Result: 15".
Sharpen your coding skills with Unstop's 100-Day Coding Sprint and compete now for a top spot on the leaderboard!
Using This Keyword With Getters And Setters
The this keyword is often used in setters to resolve ambiguity between instance variables and method parameters when they share the same name. It ensures the correct assignment of values to instance variables. In getters, this can be used optionally to refer to the current object's variables explicitly.
Code Example:
class Student {
private String name;
private int age;
// Setter for name
public void setName(String name) {
this.name = name; // Resolves ambiguity
}
// Getter for name
public String getName() {
return this.name; // Refers to the instance variable
}
// Setter for age
public void setAge(int age) {
this.age = age; // Resolves ambiguity
}
// Getter for age
public int getAge() {
return this.age; // Refers to the instance variable
}
// Display method
public void display() {
System.out.println("Name: " + this.name + ", Age: " + this.age);
}
}
public class Main {
public static void main(String[] args) {
Student student = new Student();
// Using setters
student.setName("John");
student.setAge(20);
// Display details using getters
System.out.println("Name: " + student.getName());
System.out.println("Age: " + student.getAge());
// Alternatively, display all details
student.display();
}
}
Output:
Name: John
Age: 20
Name: John, Age: 20
Explanation:
In the above code example-
- We define a class named Student to represent a student, with private fields name and age to store the student’s details.
- The fields are private to enforce encapsulation, ensuring they are accessed and modified only through methods in the class.
- The setName() method is a setter that assigns a value to the name field. The this keyword resolves ambiguity between the parameter and the instance variable with the same name.
- The getName() method is a getter that returns the value of the name field. It ensures controlled access to the private variable.
- Similarly, the setAge() method sets a value for the age field, and the getAge() method retrieves the value of the age field.
- The display method outputs the student’s details in a single statement using System.out.println.
- In the Main() class, the main() method serves as the program's entry point.
- Within main, we create an instance of the Student class named student.
- We use the setName and setAge methods to assign values to the name and age fields.
- The getName and getAge methods are called to retrieve and display the name and age values individually.
- Alternatively, we call the display method to print both details together in a single statement, demonstrating the flexibility of the class methods.
Difference Between This And Super Keyword In Java
The key differences between this and super keyword in Java are as follows:
Feature |
This Keyword |
Super Keyword |
Reference |
Refers to the current object (instance) of the class. |
Refers to the immediate parent class (superclass). |
Use in Constructor |
Can be used to call another constructor in the same class (constructor chaining). |
Cannot be used for constructor chaining in the same class. It is used to call the parent class constructor. |
Access to Variables |
Accesses instance variables of the current class. |
Accesses instance variables of the parent class. |
Access to Methods |
Can be used to call methods of the current class. |
Used to call methods of the parent class, especially when overridden in the subclass. |
Use in Inheritance |
Refers to the current object of the class where this is used. |
Refers to the parent class object in an inheritance hierarchy. |
Scope |
Limited to the current class or subclass if used in a subclass. |
Limited to the parent class and can be used in subclasses to refer to the superclass members. |
Ambiguity Resolution |
Resolves ambiguity between instance variables and method parameters with the same name. |
Cannot resolve ambiguity in the same way. It is used to refer to the parent class's members when they are hidden by the subclass. |
Best Practices For Using This Keyword In Java
Here are some best practices to ensure the effective and clean use of this keyword in Java:
- Use this for Clarity: Always use this in setters and constructors to avoid ambiguity between instance variables and parameters with the same name. For Example-
public void setName(String name) {
this.name = name; // Improves clarity
}
- Ensure Proper Use in Constructor Chaining: Use this() only to chain constructors within the same class. Always place the this() call as the first statement in the constructor. For Example-
public Student() {
this("Unknown", 0); // Chaining to parameterized constructor
}
- Avoid Redundancy: Do not use this unnecessarily in situations where it is implied or obvious. For Example-
// Avoid unnecessary usage:
System.out.println(this.name); // Instead of just `name` if no ambiguity exists.
- Use this for Method Chaining: Return this from methods when implementing fluent APIs or method chaining. For Example-
public Student setName(String name) {
this.name = name;
return this; // Supports method chaining
}
- Be Mindful When Passing this: Pass this only when the current object needs to be passed, such as to another method or constructor. Avoid exposing an incomplete object (e.g., in a constructor before initialization is complete). For Example-
public void register(Registry registry) {
registry.add(this);
}
- Use in Inner Classes: Use this to explicitly refer to the outer class instance when working with inner classes. For Example-
OuterClass.this.method(); // Refers to the method in the outer class
- Avoid Overusing this in Getters: The use of this in getters is optional but can be omitted if it doesn't enhance clarity. For Example-
public String getName() {
return name; // Simple and sufficient
}
- Follow Coding Standards: Ensure consistent usage of this across the codebase to improve readability and maintainability.
By adhering to these best practices, you can effectively utilize the this keyword to write clean, efficient, and unambiguous Java code.
Are you looking for someone to answer all your programming-related queries? Let's find the perfect mentor here.
Conclusion
In Java, the this keyword is like a trusty sidekick for the current object, helping it stand out in a crowd of variables and methods. It’s there to clear up confusion, especially when method parameters and instance variables share the same name. It’s also the key to calling other constructors, chaining methods, and making your code more fluent. When used properly, this helps maintain clarity and organization, making your code easier to read and debug.
So, whether you’re passing the object to another method, referring to instance variables, or just keeping things neat, remember that this is always there, ensuring that your code stays as smooth as possible.
Frequently Asked Questions
Q. What does the this keyword refer to in Java?
The this keyword in Java refers to the current instance of the class in which it is used. It is a reference variable that points to the object invoking the method or constructor. In essence, it helps to distinguish between instance variables (fields) and method parameters when they have the same name, or when we want to pass the current object to another method or constructor.
Q. Can I use the this keyword in a static method?
No, you cannot use the this keyword inside a static method. This is because static methods belong to the class itself, not to any specific instance of the class. Since this refers to the current instance of a class, using it in a static context (where no instance exists) would cause a compilation error.
Q. What is constructor chaining with this?
Constructor chaining refers to the practice of calling one constructor from another within the same class using this(). It is typically used to avoid code duplication and ensure that multiple constructors share common initialization logic. The this() call must be the first statement in the constructor. For Example-
class Car {
private String model;
private int year;
// Default constructor
public Car() {
this("Unknown", 0); // Calls the parameterized constructor
}
// Parameterized constructor
public Car(String model, int year) {
this.model = model;
this.year = year;
}
}
Q. What happens if this() is used incorrectly in a constructor?
If this() is used incorrectly in a constructor, such as not being the first statement, it will result in a compilation error. The this() call is specifically meant for constructor chaining, where one constructor calls another constructor in the same class. It must be the very first statement in the constructor to ensure proper chaining and initialization. Using it elsewhere in the constructor will lead to the error: "Call to this() must be the first statement in constructor".
Q. Can I use this to call another method within the same class?
Yes, you can use this to explicitly call another method within the same class. While it’s not strictly necessary (as Java will implicitly call methods without this), it can be useful for clarity, especially when dealing with complex code or method chaining. For Example-
class Calculator {
private int result;
public void add(int a, int b) {
this.result = a + b; // Assign the sum to the result
this.display(); // Explicitly call another method
}
public void display() {
System.out.println("Result: " + this.result);
}
}
Q. Why is the this keyword needed in Java?
The this keyword is needed in Java to refer to the current instance of the class within its methods and constructors.
- It helps resolve ambiguity when the instance variables and method parameters share the same name, making it clear which variable is being referred to.
- Additionally, this allows calling other constructors within the same class (constructor chaining) and can be used to pass the current object to other methods or constructors.
- It essentially acts as a reference to the object that is invoking the method or constructor.
With this we conclude our discussion on the this keyword in Java. Here are a few other topics that you might be interested in reading:
- 15 Popular JAVA Frameworks Use In 2023
- What Is Scalability Testing? How Do You Test Application Scalability?
- Top 50+ Java Collections Interview Questions
- 10 Best Books On Java In 2024 For Successful Coders
- Difference Between Java And JavaScript Explained In Detail
- Top 15+ Difference Between C++ And Java Explained! (+Similarities)
I’m a Computer Science graduate with a knack for creative ventures. Through content at Unstop, I am trying to simplify complex tech concepts and make them fun. When I’m not decoding tech jargon, you’ll find me indulging in great food and then burning it out at the gym.
Comments
Add commentLogin to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
Esuru Pooja.C 2 hours ago
Ankita Das 2 days ago
Ragini yadav 3 days ago
Sunita Bhat 5 days ago
SIVANI Immidi 1 week ago
Mihir Kumar Ranjan 1 week ago