Super Keyword In Java | Definition, Applications & More (+Examples)
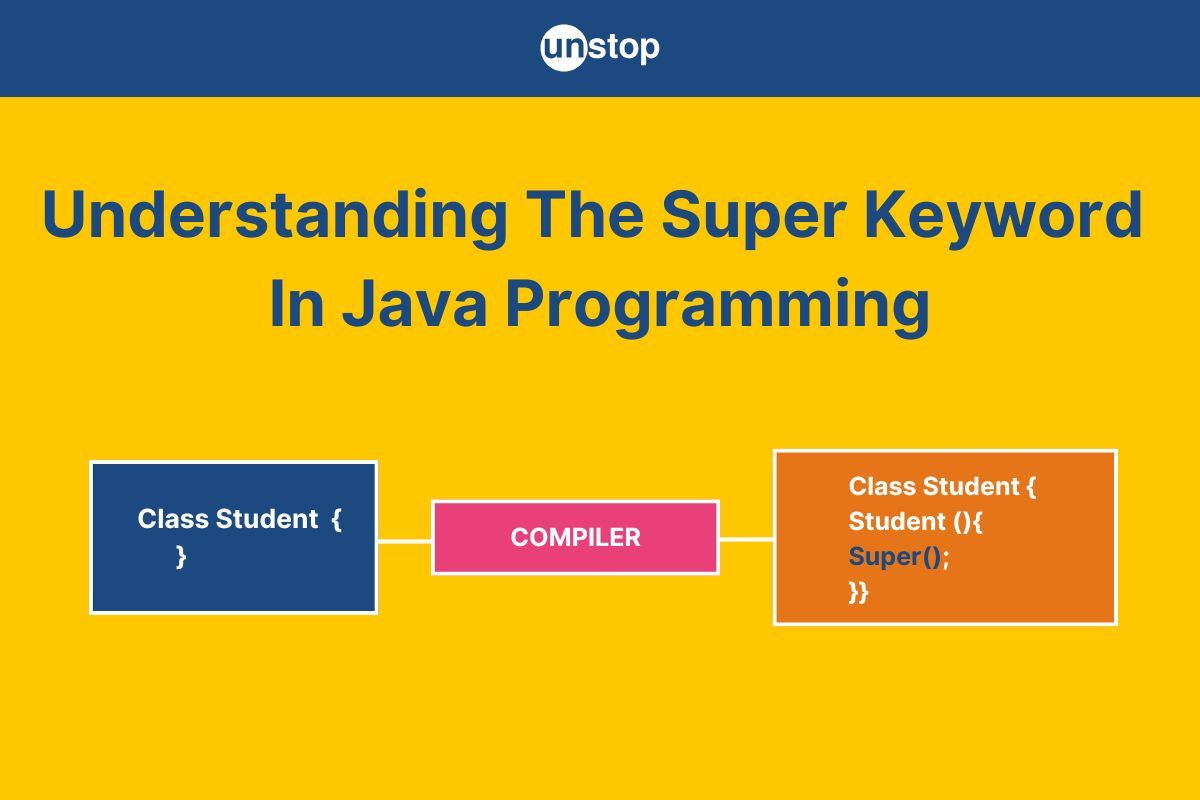
The super keyword in Java plays a crucial role in enabling inheritance, one of the core principles of object-oriented programming. It provides a way for subclasses to interact with their superclass, allowing them to access and manipulate inherited members (fields, methods) and constructors. This functionality is essential for code reuse and maintaining an organized, hierarchical structure in your codebase.
Whether you're overriding methods, calling superclass constructors, or accessing hidden fields, understanding super is key to mastering Java's inheritance model. In this article, we’ll understand the many facets of super and explore its applications, limitations and practical examples.
Understanding Super Keyword In Java
The super keyword refers to the immediate parent class object in Java programming. It allows a subclass to access members of its parent class. This access includes methods and variables that might be hidden or overridden in the child class.
- When a subclass is instantiated, super is automatically invoked. This means the constructor of the parent class runs first, ensuring that the parent’s properties and methods are set up properly before the subclass can use them.
- It also supports method overriding and polymorphism. Subclasses can provide specific implementations of methods defined in their parent classes. This flexibility helps create dynamic applications that can respond differently based on the object type.
- Moreover, super simplifies complex inheritance hierarchies. Developers can navigate through multiple levels of inheritance easily. They can call parent class methods directly using super, which makes debugging and understanding code easier.
Syntax Of Super Keyword In Java
super.memberName; // Accessing a superclass member
super(arguments); // Calling a superclass constructor
Here:
- super: It refers to the parent class object/ It is also used to call the parent class constructor.
- memberName: It can be a variable or method name.
- arguments: They are the arguments passed to the parent class constructor.
Explore this amazing course and master all the key concepts of Java programming effortlessly!
Super Keyword In Java With Instance Variables
Super plays a crucial role in distinguishing between parent and child class variables. When a child class has a variable with the same name as a parent class variable, it causes variable shadowing. This means the child class variable hides the parent class variable. Using super helps to resolve this issue.
Code Example:
Output:
Superclass name: Animal
Subclass name: Dog
Explanation:
In the above code example-
- We start by defining a class named Animal that has a single instance variable, name, initialized to the string "Animal." This variable is part of the superclass.
- Next, we create a subclass called Dog that extends Animal. This subclass also has an instance variable named name, but it is initialized to the string "Dog." Here, we notice that both classes have instance variables with the same name.
- Within the Dog class, we define a method called printNames(). This method prints two different names to the console.
- To access the name variable from the superclass Animal, we use super.name within the printNames() method. This allows us to retrieve the value "Animal."
- We also access the subclass's instance variable name directly by simply using name, which gives us "Dog."
- In the Main class, we have the main() method, which serves as the entry point of the program.
- We create an instance of the Dog class and store it in a variable called dog.
- Finally, we call the printNames() method on the dog object, which executes the code inside the method, printing both the superclass name and the subclass name to the console.
Super Keyword In Java With Method Overriding
Super is essential in method overriding. It allows a child class to call methods from its parent class. This is important for implementing polymorphic behavior. Polymorphism enables objects to be treated as instances of their parent class.
Code Example:
Output:
Animal makes a sound
Dog barks
Explanation:
In the above code example-
- We define a class named Animal that includes a method called sound(). This method, when called, prints "Animal makes a sound" to the console, representing a general behavior for all animals.
- We then create a subclass called Dog that extends Animal. This subclass overrides the sound() method to provide a specific implementation for dogs.
- In the overridden sound() method of the Dog class, we first call the superclass's sound() method using super.sound(). This executes the sound() method from the Animal class, which prints "Animal makes a sound."
- After calling the superclass method, we add additional behavior specific to the Dog class by printing "Dog barks." This illustrates how we can extend the functionality of the superclass method.
- In the Main class, we have the main() method. We now create an instance of the Dog class and store it in a variable called dog.
- When we call the sound() method on the dog object, it executes the overridden method in the Dog class, which first calls the sound() method from Animal and then prints "Dog barks," showcasing both the general and specific behaviors.
Super Keyword In Java With Constructor Chaining
Constructor chaining occurs when a constructor calls another constructor in the same or superclass using super(). This approach initializes parent class properties before executing the child class constructor. One benefit of this is ensuring that all necessary properties are set up correctly. Here's how constructor chaining works:
- The child constructor calls super() with required parameters.
- The parent constructor executes first.
- Then control returns to the child constructor.
This sequence is vital in complex inheritance scenarios where multiple levels of inheritance exist.
Code Example:
Output:
Animal type: Mammal
Dog name: Buddy
Explanation:
In the above code example-
- We start by defining a class called Animal, which has an instance variable named type to store the type of the animal.
- The Animal class includes a constructor that takes a string parameter called type. Inside this constructor, we assign the value of the parameter to the instance variable type and print a message to the console that includes the animal's type.
- Next, we create a subclass called Dog that extends Animal. In addition to inheriting properties from Animal, it has its own instance variable called name to store the name of the dog.
- The Dog class includes its own constructor that takes a string parameter called name. Inside this constructor, we first call the superclass constructor using super("Mammal"). This initializes the type variable in the Animal class with the value "Mammal" and triggers the corresponding print statement in the Animal constructor.
- After calling the superclass constructor, we assign the value of the name parameter to the instance variable name and print a message that includes the dog's name.
- In the Main class, we have the main() method. We now create an instance of the Dog class by calling the constructor with the argument "Buddy."
- This not only initializes the Dog object but also triggers the superclass constructor, resulting in both the animal type and the dog name being printed to the console.
Sharpen your coding skills with Unstop's 100-Day Coding Sprint and compete now for a top spot on the leaderboard!
Applications Of Super Keyword In Java
The super keyword in Java is a powerful tool that enables you to interact with the parent class's members. It's particularly useful in inheritance scenarios, where you want to access or override methods and variables defined in the superclass.
-
Accessing Superclass Members: When a subclass has a member with the same name as a member in the superclass, you can use the super keyword to access the superclass's version. For Example-
class Parent {
int x = 10;
}
class Child extends Parent {
int x = 20;
void printX() {
System.out.println("Child's x: " + x);
System.out.println("Parent's x: " + super.x);
}
}
-
Invoking Superclass Constructors: The super keyword can be used to explicitly call a superclass constructor, which is often needed when a subclass extends a superclass that doesn’t have a no-argument constructor or when you want to initialize superclass properties with specific values. For Example-
class Animal {
String type;
Animal(String type) {
this.type = type;
}
}
class Dog extends Animal {
Dog() {
super("Mammal"); // Calls the superclass constructor
}
}
-
Managing Overridden Methods: When methods in the superclass need additional functionality, super can ensure that both superclass and subclass logic are executed. This is common in frameworks where parent methods carry essential setup logic, and subclasses handle additional, specific operations. For Example-
class Logger {
void log(String message) {
System.out.println("Logging: " + message);
}
}
class ErrorLogger extends Logger {
@Override
void log(String message) {
super.log("Error: " + message); // Calls superclass log method
}
}
-
Refining Polymorphism: When using polymorphism, sometimes a subclass may need to access a superclass version of a method to ensure consistent behaviour. Using super, developers can fine-tune polymorphic behaviours by invoking superclass methods directly, adding clarity to code.
Difference Between This And Super Keyword In Java
Super and this keyword serve different purposes in Java. The super keyword targets the parent class, while this refers to the current class instance. Each has its own context of usage. Here's a table highlighting the key differences between this and super keyword in Java:
Aspect | super keyword | this keyword |
---|---|---|
Primary Use | Refers to the superclass (parent class) of the current class | Refers to the current instance of the class |
Accessing Superclass Members | Used to access superclass methods, fields, and constructors | Cannot access superclass members, only current class members |
Constructor Invocation | Can call the superclass constructor using super(); must be the first statement in the subclass constructor | Can call another constructor within the same class using this(); also must be the first statement |
Overridden Methods | Can be used to invoke an overridden method from the superclass | Cannot invoke an overridden method; only calls the current class’s version |
Distinguishing Fields | Used when subclass and superclass have fields with the same name to differentiate superclass fields | Used to refer to instance fields when they have the same name as parameters or local variables |
Static Context | Cannot be used in a static context since it refers to superclass instance members | Cannot be used in a static context since it refers to the current instance |
Access Modifiers | Only accessible to protected or public superclass members; cannot access private members directly | Can access all members of the current class, including private members |
Polymorphism Support | Primarily used in inheritance to refer to superclass behaviors | Primarily used within the class to refer to instance-specific data |
Advantages Of Using Super Keyword In Java
The super keyword in Java is a valuable tool that offers several advantages when working with inheritance hierarchies:
1. Encourages Code Reusability
- The super keyword helps subclasses reuse code from the superclass rather than duplicating it. Subclasses can leverage methods and fields already defined in the superclass, reducing redundancy and keeping the code DRY (Don’t Repeat Yourself).
- This reusability is particularly helpful in large projects where common functionality can be defined once in the superclass and shared across multiple subclasses.
2. Enables Polymorphism And Method Overriding
- In Java, polymorphism allows objects to be treated as instances of their superclass rather than their specific subclass. Using super, subclasses can override superclass methods and add unique behavior while still retaining access to the superclass’s version of the method.
- This allows for extended functionality without compromising the original behavior of the superclass, which is essential in many object-oriented designs.
3. Simplifies Constructor Chaining
- Constructor chaining is common in inheritance hierarchies, where a subclass constructor needs to initialize superclass fields. Using super, you can invoke a specific superclass constructor, passing any necessary parameters to initialize inherited fields.
- This is particularly useful when the superclass lacks a default (no-argument) constructor or when fields in the superclass need specific values.
4. Promotes Organized Code Structure
- By explicitly calling super to access superclass methods or fields, the dependencies within the inheritance hierarchy become clearer. This makes it easier for developers to understand the flow of a program and to see where certain behaviors come from.
- The super keyword visually signifies that a method or field is inherited, making the structure and flow of code more understandable and organized.
5. Allows Controlled Access To Superclass Members
- With super, developers can control which superclass methods and fields are accessed in a subclass. This enables fine-grained management of inherited behaviors, allowing subclasses to selectively call superclass methods only where they are needed.
- This selective access is particularly helpful in large or complex inheritance hierarchies where only certain inherited behaviors should be used.
6. Supports Encapsulation And Flexibility
- The super keyword allows subclasses to interact with encapsulated fields and methods of the superclass in a controlled manner. While a subclass can access protected members or public methods in the superclass, super ensures that private fields and methods remain hidden.
- This balance preserves the encapsulation of the superclass and promotes modular design, allowing for flexible extension without risking the integrity of the superclass’s internals.
7. Improves Debugging And Code Maintainability
- By specifying super in overridden methods, it becomes clear when both the superclass and subclass methods are executed, which aids in tracking the flow of the program. During debugging, super helps clarify which class’s version of a method or field is being accessed.
- This is especially valuable in complex applications where multiple classes in a hierarchy interact, as it allows for easier tracing and understanding of the inheritance structure.
Are you looking for someone to answer all your programming-related queries? Let's find the perfect mentor here.
Limitations And Considerations Of Super Keyword In Java
When using the super keyword in Java, there are several limitations and considerations to keep in mind, particularly around its scope, inheritance behavior, and best practices. Here are some key points:
1. Restricted To Immediate Superclass
- The super keyword can only access members of the immediate superclass. If a class has a multi-level inheritance hierarchy, super won’t reach beyond the direct parent class.
- Example: If class C extends class B, which in turn extends class A, then C can only use super to access members of B, not A.
2. Cannot Bypass Access Modifiers
- Even with super, access modifiers like private apply. If a superclass field or method is private, it cannot be accessed directly by the subclass, even with super.
- Consideration: Use protected or package-private access levels to allow subclass access where needed.
3. Not Applicable For Static Members
- The super keyword cannot access static fields or methods of a superclass because super refers to an instance, and static members belong to the class, not an instance.
- Example: To call a static method of a superclass, you must use SuperclassName.methodName().
4. Cannot Call Multiple Constructors
- Java does not allow chaining multiple superclass constructors in the same subclass constructor. Only one superclass constructor can be invoked with super, and it must be the first statement in the constructor.
- Consideration: If a subclass constructor needs to initialize the superclass in different ways, consider overloading constructors.
5. Code Readability Concerns
- Overusing super can make code harder to follow, especially in deep inheritance chains where subclasses frequently override superclass methods. This can make tracing the flow of a program challenging.
- Consideration: Use super sparingly and with careful documentation, particularly in complex inheritance structures.
6. Cannot Override Final Methods
- If a superclass method is marked as final, a subclass cannot override it, nor can it use super to modify its behavior. The super keyword cannot circumvent this restriction.
- Example: final void display() in a superclass cannot be overridden or altered by a subclass.
7. Inheritance Limitations With Composition
- If a class is designed for composition rather than inheritance, super becomes irrelevant because there is no parent-child relationship.
- Consideration: Favor composition over inheritance for complex or deeply nested class hierarchies where super might add unnecessary complexity.
Conclusion
The super keyword is a powerful tool in Java that enhances your programming capabilities. It allows you to access parent class methods and variables easily, improving code efficiency and readability. By understanding its applications and advantages, you can write cleaner, more maintainable code. Remember the key points discussed, as they will guide you in utilizing super effectively.
Now that you've grasped the essentials of the super keyword, it’s time to put your knowledge into action. Experiment with practical examples and tackle common questions to reinforce your learning. Dive deeper into Java programming and unlock your potential. Happy coding!
Frequently Asked Questions
Q. What is the purpose of the super keyword in Java?
The super keyword in Java is primarily used within a subclass to refer to its immediate superclass (parent class). Its main purposes include:
- Accessing Superclass Members: It allows a subclass to access methods and instance variables defined in the superclass, particularly when they are overridden or shadowed in the subclass.
- Invoking Superclass Constructors: It enables the subclass to call a specific constructor of the superclass, which is essential for initializing inherited fields.
Using super, you can ensure that the subclass can utilize and extend the functionality of the superclass without duplicating code.
Q. Can you use super to access private members of a superclass?
No, you cannot use the super keyword to access private members (fields or methods) of a superclass. Private members are not accessible outside the class in which they are defined, even to subclasses. For Example-
class Animal {
private String secret = "This is a secret";
private void secretMethod() {
System.out.println("Secret method in Animal");
}
}
class Dog extends Animal {
void accessSecret() {
// This will cause a compilation error
// System.out.println(super.secret);
// super.secretMethod(); // This will also cause a compilation error
}
}
To access superclass members in a subclass, you should use protected or public access modifiers.
Q. Can I use super in static methods?
No, the super keyword cannot be used in static methods because super refers to instance members of the superclass, and static methods do not belong to any specific instance of a class. Static methods belong to the class itself, not to any instance.
Q. What is the difference between super and this keywords?
The this and super keywords are essential in Java object oriented programming. Keyword this refers to the current object instance, allowing access to its members. It's used to differentiate between instance variables and parameters with the same name, pass the current object as an argument, and return the current object itself.
On the other hand, super refers to the parent class object. It's used to access members of the parent class method when there's a name conflict, call the parent class constructor, and access its methods and variables. By understanding these keywords, you can write well-structured and efficient Java code.
Q. Is super necessary in Java?
The super keyword in Java is not strictly necessary, but it plays a crucial role in specific contexts. It is essential when you need to access overridden methods from a superclass, as it allows a subclass to call the original implementation while adding its functionality.
- Additionally, super is necessary for invoking a super constructor, ensuring proper initialization of inherited fields.
- It also helps resolve naming conflicts when a subclass has a field with the same name as one in the superclass, allowing you to explicitly refer to the superclass's field. However, in situations where a method is not overridden or when accessing subclass-specific fields, java keyword super can be omitted.
- Overall, while not always required, super significantly enhances code clarity and maintainability in complex inheritance structures.
With this, we conclude our discussion on the super keyword in Java. Here are a few other topics that you might be interested in reading:
- 15 Popular JAVA Frameworks Use In 2023
- What Is Scalability Testing? How Do You Test Application Scalability?
- Top 50+ Java Collections Interview Questions
- 10 Best Books On Java In 2024 For Successful Coders
- Difference Between Java And JavaScript Explained In Detail
- Top 15+ Difference Between C++ And Java Explained! (+Similarities)
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment