Java Programming Language
Table of content:
- History Of Java Programming Langauge
- Infographic For History Of Java
- What’s In The Name | History Of Java
- Key Features Of Java
- Advantages And Disadvantages Of Java
- The Version History Of Java Langauge
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is JDK?
- How To Download Java Development Kit (JDK) For Windows, MacOS, and Linux?
- Set Environment Variables In Java
- How To Install Java (JDK) On Windows 64-Bit Machine?
- How To Install Java (JDK) On Linux?
- How To Install Java (JDK) On macOS?
- How To Test Java Installation?
- How To Write Your First Java Program On Linux OS?
- Conclusion
- Frequently Asked Questions
Table of content:
- Java Programming Language | An Introduction
- 15 Key Features Of Java
- Write Once Run Anywhere (WORA) | Features Of Java
- Java Editions
- 5 New Features Of JAVA 8
- 5 New Features Of JAVA 11
- What Makes Java Popular?
- Conclusion
- Frequently Asked Questions
Table of content:
- What is Java?
- Advantages of Java
- Disadvantages of Java
Table of content:
- What Is Java Programming?
- Role Of Integrated Development Environments (IDEs) In Java Development
- 15 Best Java IDE For Developers
- In-Depth Comparison Table
- Conclusion
- Frequently Asked Questions
Table of content:
- Key Differences Between Java And Core Java
- What Is Java?
- What Is Core Java?
- Applications Of Java
- Applications Of Core Java
- When To Use Java?
- When To Use Core Java?
- Conclusion
- Frequently Asked Questions
Table of content:
- What Are Variables In Java Language?
- How To Declare Variables In Java Programs?
- How To Initialize Variables In Java?
- Naming Conventions For Variables In Java
- Types Of Variables In Java
- Local Variables In Java
- Instance Variables In Java
- Static Variables In Java
- Final Variables In Java
- Scope and Lifetime of Variables In Java
- Data Types Of Variables In Java (Primitive & Non-primitive)
- Java Variable Type Conversion & Type Casting
- Working With Variables In Java (Examples)
- Access Modifiers & Variables In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Are Identifiers In Java?
- Syntax Rules For Identifiers In Java
- Valid Identifiers in Java
- Invalid Identifiers in Java
- Java Reserved Keywords
- Naming Conventions & Best Practices For Identifiers In Java
- What Is An Identifier Expected Error In Java?
- Reasons The Identifier Expected Error Occurs
- How To Fix/ Resolve Identifier Expected Errors In Java?
- Conclusion
- Frequently Asked Questions
Table of content:
- What Are Data Types In Java?
- Primitive Data Types In Java
- Non-Primitive Data Types In Java
- Key Differences Between Primitive And Non-Primitive Data Types In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Are Operators In Java?
- Types Of Operators In Java
- Unary Operators In Java
- Arithmetic Operators In Java
- Assignment Operators In Java
- Relational Operators In Java
- Logical Operators In Java
- Bitwise Operators In Java
- Shift Operators In Java
- Increment & Decrement Operators In Java
- Ternary Operator In Java
- Instanceof Operator In Java
- Precedence & Associativity Of Java Operators
- Advantages & Disadvantages Of Operators In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is A Return Statement In Java?
- Use Cases Of Return Statements In Java
- Returning A Value From A Method In Java
- Returning A Class Object In Java
- Returning Void (No Value) In Java
- Advantages Of Using Return Statements In Java
- Limitations Of Using Return Statements In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Are Keywords In Java?
- List Of Keywords In Java
- Detailed Overview Of Java Keywords With Examples
- What If When Keywords In Java Are Used As Variable Names?
- Difference Between Identifiers & Keywords In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Abstract Keyword In Java?
- Use Of Abstract Keyword In Java
- Abstract Methods In Java
- Abstract Classes In Java
- Advantages Of Abstract Keyword In Java
- Disadvantages Of Abstract Keyword In Java
- Abstract Classes Vs. Interfaces In Java
- Real-World Applications Of Abstract Keyword
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is throws Keyword In Java?
- How Does The throws Keyword Work?
- Throwing A Checked Exception Using throws In Java
- Throwing Multiple Exceptions Using throws In Java
- Throwing A Custom Exception Using throws In Java
- When To Use The throws Keyword In Java
- Difference Between throw and throws Keyword In Java
- Best Practices For Using The throws Keyword In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is The Volatile Keyword In Java?
- How Does Volatile Keyword In Java Work?
- Using Volatile Keyword In Java To Control Thread Execution
- Using Volatile Keyword In Java To Signal Between Multiple Threads
- Difference Between Synchronization And Volatile Keyword
- Common Mistakes And Best Practices While Using Volatile Keyword In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding Super Keyword In Java
- Super Keyword In Java With Instance Variables
- Super Keyword In Java With Method Overriding
- Super Keyword In Java With Constructor Chaining
- Applications Of Super Keyword In Java
- Difference Between This And Super Keyword In Java
- Advantages Of Using Super Keyword In Java
- Limitations And Considerations Of Super Keyword In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding This Keyword In Java
- Uses Of This Keyword In Java
- Using This Keyword For Referencing Instance Variables
- Using This Keyword For Invoking A Constructor
- Using This Keyword For Invoking A Method
- Using This Keyword With Getters And Setters
- Difference Between This And Super Keyword In Java
- Best Practices For Using This Keyword In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is new Keyword In Java?
- Uses Of The new Keyword In Java
- Memory Management With new Keyword In Java
- Example 1: Creating An Object Of A Class Using new Keyword In Java
- Example 2: Creating An Array Using The new Keyword In Java
- Best Practices For Using new Keyword In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is The Transient Keyword In Java?
- Real-Life Example Of The Transient Keyword In Java
- When To Use The Transient Keyword In Java
- Example 1: Effect Of Transient Keyword On Serialization In Java
- Example 2: Skipping Sensitive Data During Serialization With Transient Keyword In Java
- Using Transient With Final Keyword In Java
- Using Transient With Static Keyword
- Difference Between Transient And Volatile Keyword In Java
- Advantages And Disadvantages Of Transient Keyword In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Static Keyword In Java?
- Characteristics Of Static Keyword In Java
- Static Variables In Java
- Static Method In Java
- Static Blocks In Java
- Static Classes In Java
- Static Variables Vs Instance Variables In Java
- Advantages Of Static Keyword In Java
- Disadvantages Of Static Keyword In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is A Static Method In Java?
- Use Cases Of Static Method In Java
- Using Static Method In Java To Create A Utility Class
- Using Static Method In Java To Implement The Singleton Design Pattern
- Difference Between Static And Instance Methods In Java
- Limitations Of Static Method In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding Final Keyword In Java
- Final Variables In Java
- Final Methods In Java
- Final Classes In Java
- Difference Between Static And Final Keyword In Java
- Uses Of Final Keyword In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Key Difference Between final, finally, And finalize In Java
- What Is final Keyword In Java?
- What Is finally Keyword In Java?
- What Is finalize Keyword In Java?
- When To Use Which Keyword In Java?
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding The extends Keyword In Java?
- Use Of extends Keyword In Java
- Using Java extends To Implement Single Inheritance
- Using Java extends With Interfaces (Default Methods)
- Overriding Using extends Keyword In Java
- Difference Between extends And implements In Java
- Real World Applications Of Extends Keyword In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Decision Making Statement In Java?
- If Statement In Java
- If-Else Statement In Java
- Else-If Ladder In Java
- Switch Statement In Java
- Ternary/Conditional Operator (?:) In Java
- Best Practices For Writing Decision Making Statements In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Are Control Statements in Java?
- Decision-Making Control Statements In Java
- Looping Control Statements In Java
- Jump (Branching) Control Statements In Java
- Application Of Control Statements In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is The Break Statement In Java?
- Working Of The Break Statement In Java
- Using Java Break Statement With Loops
- Using Java Break Statement With Switch Statement
- Using Java Break Statement With Infinite Loops
- Common Pitfalls While Using Break Statements In Java
- Best Practices For Using The Break Statement In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Switch Statement In Java?
- Working Of The Switch Statement In Java
- Example Of Switch Statement In Java
- Java Switch Statement With String
- Java Nested Switch Statements
- Java Enum In Switch Statement
- Java Wrapper Classes In Switch Statements
- Uses Of Switch Statement In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Syntax Of main() Method In Java
- public Specifier – Main Method In Java
- static Keyword – Main Method In Java
- void Return Type Of Main Method In Java
- The main Identifier – Main Method In Java
- String[] args In Main Method In Java
- The Role Of Java Virtual Machine (JVM)
- Running Java Programs Without The Main Method
- Variations In Declaration Of Main Method In Java
- Overloading The Main Method In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Method Overriding In Java?
- Example Of Method Overriding In Java
- Ideal Use Cases Of Method Overriding In Java
- Rules For Method Overriding In Java
- Super Keyword & Method Overriding In Java
- Constructor & Method Overriding In Java
- Exception Handling In Method Overriding In Java
- Access Modifiers In Method Overriding In Java
- Advantages & Disadvantages Of Method Overriding In Java
- Difference Between Method Overloading Vs. Method Overriding In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Method Overloading In Java?
- Different Ways Of Method Overloading In Java
- Overloading The main() Method In Java
- Type Promotion & Method Overloading In Java
- Null Error & Method Overloading In Java
- Advantages Of Method Overloading In Java
- Disadvantages Of Method Overloading In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Difference Between Overloading And Overriding In Java (Comparison Table)
- What Is Method Overloading In Java?
- What Is Method Overriding In Java?
- Key Differences Between Overloading & Overriding In Java Explained
- Difference Between Overloading And Overriding In Java Code Example
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is A One-Dimensional Array In Java?
- Key Characteristics Of One-Dimensional Arrays In Java
- Declaration Of One-Dimensional Array In Java
- Initialization Of One-Dimensional Array In Java
- Common Operations On One-Dimensional Array In Java
- Advantages Of One-Dimensional Arrays In Java
- Disadvantages Of One-Dimensional Arrays In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is A Multidimensional Array In Java?
- Difference Between Single-Dimensional And Multidimensional Arrays In Java
- Declaring Multidimensional Arrays In Java
- Initializing Multidimensional Arrays In Java
- Accessing And Manipulating Elements In Multidimensional Arrays In Java
- Working Of Multidimensional Arrays With Jagged Arrays In Java
- Why Use Multidimensional Arrays In Java?
- Limitations Of Multidimensional Arrays In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Are Jagged Arrays In Java?
- Comparison With Regular Multi-Dimensional Arrays
- Declaring Jagged Arrays In Java
- Initialization Of Jagged Arrays In Java
- Printing Elements Of A Jagged Array In Java
- Accessing And Modifying Elements Of A Jagged Array In Java
- Advantages Of Jagged Arrays In Java
- Disadvantages Of Jagged Arrays In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Array Of Objects In Java?
- Declare And Initialize An Array Of Object In Java
- Example Of An Array Of Objects In Java
- Sorting An Array Of Objects In Java
- Passing Arrays Of Objects To Methods In Java
- Returning Arrays Of Objects From Methods In Java
- Advantages Of Arrays Of Objects In Java
- Disadvantages Of Arrays Of Objects In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is A Dynamic Array In Java?
- Why Use Dynamic Array In Java?
- What Is The Size And Capacity Of A Dynamic Array In Java?
- How To Create A Dynamic Array In Java?
- Managing Dynamic Data Input In Java
- Storing And Processing Real-Time Data In Java
- Use Cases Of Dynamic Arrays In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Why Return An Array In Java?
- How To Return An Array In Java
- Example 1: Returning An Array Of First N Squares
- Example 2: Doubling the Values of an Array
- Common Scenarios For Returning Arrays In Java
- Points To Remember When Returning Arrays In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding ArrayList In Java
- Differences Between Arrays And ArrayList In Java
- Returning An ArrayList In Java
- Common Use Cases For Returning An ArrayList In Java
- Pitfalls To Avoid When Returning An ArrayList In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is A Thread In Java?
- Thread Vs Process
- What is a Thread Life Cycle In Java?
- What Are Thread Priorities?
- Creating Threads In Java
- Java Thread Methods
- Commonly Used Constructors In Thread Class
- Thread Synchronization In Java
- Common Challenges Faced While Using Threads In Java
- Best Practices For Using Threads In Java
- Real-World Applications Of Threads In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding Multithreading In Java
- Methods Of Multithreading In Java (Examples)
- Difference Between Multithreading And Multitasking In Java
- Handling Exceptions In Multithreading
- Best Practices For Multithreading In Java
- Real-World Use Cases of Multithreading In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Thread Priority In Java?
- Built-In Thread Priority Constants In Java
- Thread Priority: Setter & Getter Methods
- Limitations Of Thread Priority In Java
- Best Practices For Using Thread Priority In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Thread Synchronization In Java?
- The Need For Thread Synchronization In Java
- Types Of Thread Synchronization In Java
- Mutual Exclusion In Thread Synchronization In Java
- Coordination Synchronization (Thread Communication) In Java
- Advantages Of Thread Synchronization In Java
- Disadvantages Of Thread Synchronization In Java
- Alternatives To Synchronization In Java
- Deadlock And Thread Synchronization In Java
- Real-World Use Cases Of Thread Synchronization In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is A Daemon Thread In Java?
- User Threads Vs. Daemon Threads In Java
- Methods For Daemon Threads In The Thread Class
- Creating Daemon Threads In Java
- Checking The Daemon Status Of A Thread
- Exceptions In Daemon Threads
- Limitations Of Daemon Threads In Java
- Practical Applications Of Daemon Threads In Java
- Common Mistakes To Avoid When Working With Daemon Threads In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Why Do Threads Need To Communicate?
- Understanding Inter Thread Communication In Java
- The wait() Method In Inter-Thread Communication
- The notify() Method In Inter-Thread Communication
- The notifyAll() Method In Inter-Thread Communication
- Difference Between wait() And sleep() Methods In Java
- Best Practices For Inter Thread Communication In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding The Factorial Concept
- Approaches To Implementing Factorial In Java
- Find Factorial In Java Using Iterative Approach (Using a Loop)
- Find Factorial In Java Using Recursive Approach
- Complexity Analysis Of Factorial Programs In Java
- Applications Of Factorial Program In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding The Leap Year Concept
- Approach To Check A Leap Year In Java
- Alternative Approach To Check A Leap Year In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is The Difference Between JDK, JRE, and JVM?
- What Is JVM (Java Virtual Machine)?
- What Is JRE (Java Runtime Environment)?
- What Is JDK (Java Development Kit)?
- Understanding The Difference Between JDK, JRE, And JVM
- Comparison Table For Difference Between JDK, JRE, And JVM
- Conclusion
- Frequently Asked Questions
Table of content:
- Difference Between Abstraction And Encapsulation In Java
- Understanding Abstraction In Java
- Understanding Encapsulation In Java
- When To Use Abstraction And Encapsulation?
- Conclusion
- Frequently Asked Questions
Table of content:
- Differences Between Abstract Class And Interface In Java
- What Is An Abstract Class In Java?
- What Is An Interface In Java?
- When To Use An Abstract Class?
- When To Use Interface?
- Compatibility Between Abstract Class And Interface In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Error Vs. Exception In Java
- What Is Error In Java?
- What Is Exception In Java?
- Best Practices For Handling Exceptions In Java
- Why Errors Should Not Be Handled In Java?
- Conclusion
- Frequently Asked Questions
Table of content:
- Key Differences: Java Vs. JavaScript
- What Is Java?
- What Is JavaScript?
- Difference Between Java And JavaScript Explained
- Conclusion
- Frequently Asked Questions
Table of content:
- Brief Introduction To C++
- Brief Introduction To Java
- Difference Between C++ and Java
- Overview & Features Of C++ Language
- Overview & Features of Java Language
- Example of C++ and Java Program
- Key Difference Between C++ And Java Explained
- Similarities Between Java Vs. C++
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- Basic Java interview questions and answers
- Intermediate Java interview questions and answers
- Advanced Java interview questions and answers
Table of content:
- Difference between core Java and advanced Java
- Important Core Java Questions
- Tips for Preparing for Core Java
Static Method In Java | Syntax, Uses, Limitations (With Examples)
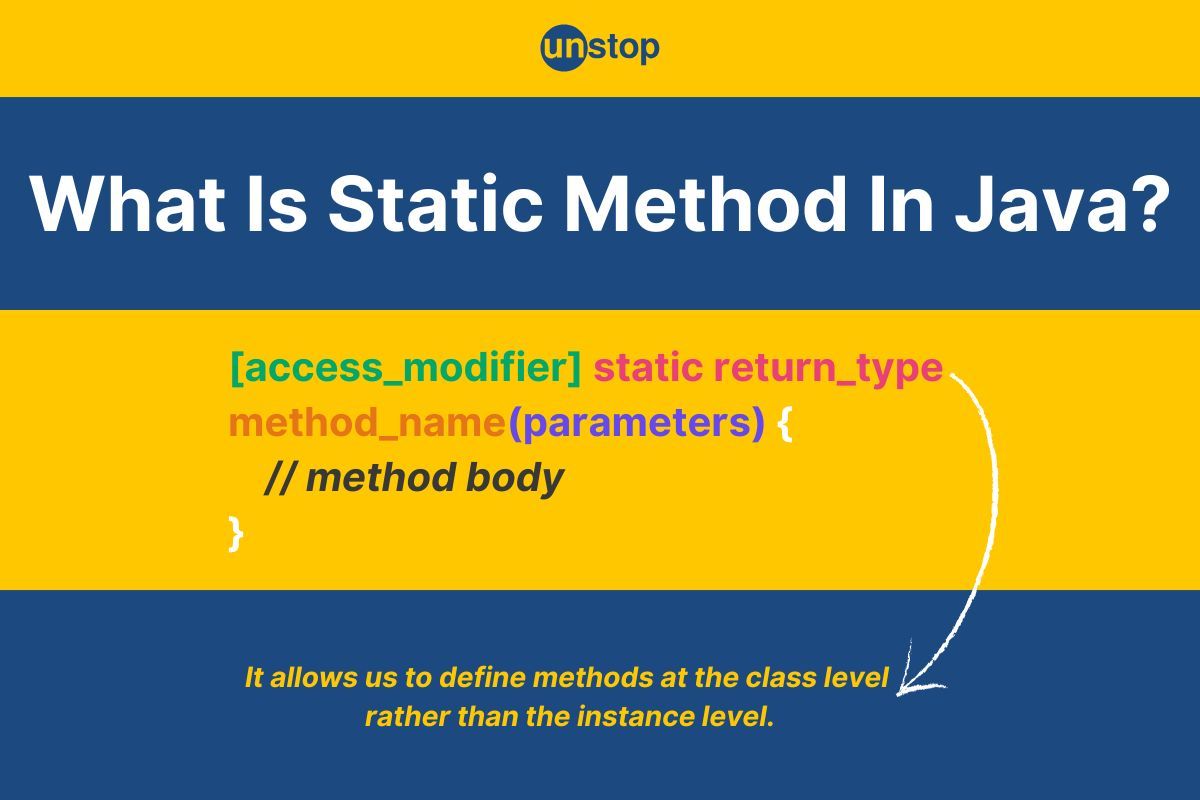
Static methods in Java are a fundamental concept that allows us to define methods at the class level rather than the instance level. These methods can be invoked without creating an object of the class, making them an efficient choice for tasks like utility operations, mathematical calculations, or accessing static variables.
In this article, we'll explore the essence of static methods in Java, their syntax, use cases, limitations, and best practices. By the end, you'll have a clear understanding of how to utilize static methods effectively in your programs.
What Is A Static Method In Java?
A static method in Java programming belongs to the class rather than an instance of the class. This means it can be called directly using the class name, without needing to create an object of the class. Static methods are often used for utility or helper methods that don’t require access to instance-specific data.
Static method in Java has the following characteristics:
- They cannot access instance variables or instance methods directly.
- They can only access other static data members and methods.
- They are invoked using the class name, making them accessible without creating objects.
For example, the Math class in Java uses static methods like Math.sqrt() and Math.pow().
Syntax Of Static Method In Java
[access_modifier] static return_type method_name(parameters) {
// method body
}
Here:
- [access_modifier]: Defines the visibility of the method. Common access modifiers are public, private, or protected. If no modifier is specified, it has package-private access by default.
- static: Indicates that the method is static and belongs to the class.
- return_type: Specifies the type of value the method returns. Use void if no value is returned.
- method_name: The name of the method, which follows Java naming conventions (e.g., camelCase).
- parameters: The input data the method accepts, enclosed in parentheses. It can be empty if no inputs are needed.
- method body: The block of code executed when the method is called.
Explore this amazing course and master all the key concepts of Java programming effortlessly!
Use Cases Of Static Method In Java
Static methods are versatile and commonly used in various scenarios. Below are the key use cases where static methods are most effective:
- Utility and Helper Methods: Static methods are ideal for utility functions that do not rely on instance-specific data. Examples include mathematical calculations, string manipulations, and other generic operations. For example-
public class MathUtils {
public static int max(int a, int b) {
return (a > b) ? a : b;
}
}
- Accessing and Modifying Static Variables: Static methods can access and modify static variables of a class. This is useful for managing shared data across all instances of a class. For example-
public class Counter {
private static int count = 0;public static void increment() {
count++;
}public static int getCount() {
return count;
}
}
- Factory Methods: Static methods are often used to create and return instances of a class. This approach is common in design patterns such as the Factory Pattern or Singleton Pattern. For example-
public class DatabaseConnection {
private static DatabaseConnection instance;private DatabaseConnection() {}
public static DatabaseConnection getInstance() {
if (instance == null) {
instance = new DatabaseConnection();
}
return instance;
}
}
- Accessing Static Constants: Static methods are used to access constant values defined in the class. This is particularly useful for organizing shared or immutable data. For example-
public class Constants {
public static final double PI = 3.14159;
public static double getPi() {
return PI;
}
}
- Entry Point of the Program (main Method): The main method in Java is static because it serves as the entry point of the program and is invoked by the Java Virtual Machine (JVM) without creating an object of the class. For example-
public static void main(String[] args) {
System.out.println("Hello, World!");
}
- Avoiding Overhead of Object Creation: For frequently called methods that do not depend on object state, static methods eliminate the overhead of object creation. For example-
public static double calculateSquareRoot(double number) {
return Math.sqrt(number);
}
- Utility Classes in Java: Standard Java libraries like java.util and java.lang use static methods extensively. For example-
- Math.sqrt(): For square root calculation.
- Arrays.sort(): For sorting arrays.
- Collections.max(): For finding the maximum element in a collection.
- Shared Resources: Static methods are used to manage shared resources, such as logging or database connections, which are common to all objects of a class. For example-
public class Logger {
public static void log(String message) {
System.out.println("Log: " + message);
}
}
- Performance-Critical Code: For performance-critical operations, static methods can be inlined by the JVM, avoiding the overhead of virtual method calls.
Using Static Method In Java To Create A Utility Class
A Utility Class is a class that contains a collection of static methods, which are usually designed to perform general, reusable operations that don’t rely on any object-specific data. These utility methods often provide basic functionality that can be called directly without needing to instantiate the class.
- In Java, we can create a utility class by declaring a class with static methods only.
- A good example of a utility class is the Math class in Java, which provides a variety of static methods for mathematical operations.
Let’s create a simple utility class called StringUtils that provides static methods for common string operations such as checking if a string is a palindrome, converting a string to uppercase, and reversing a string.
Code Example:
public class StringUtils {
// Static method to check if a string is a palindrome
public static boolean isPalindrome(String str) {
String reversed = new StringBuilder(str).reverse().toString();
return str.equals(reversed);
}
// Static method to convert a string to uppercase
public static String toUpperCase(String str) {
return str.toUpperCase();
}
// Static method to reverse a string
public static String reverseString(String str) {
return new StringBuilder(str).reverse().toString();
}
public static void main(String[] args) {
// Test the utility methods
String testString = "madam";
// Checking palindrome
boolean isPalindrome = StringUtils.isPalindrome(testString);
System.out.println(testString + " is palindrome: " + isPalindrome); // Output: true
// Converting to uppercase
String upperCase = StringUtils.toUpperCase(testString);
System.out.println("Uppercase: " + upperCase); // Output: MADAM
// Reversing the string
String reversed = StringUtils.reverseString(testString);
System.out.println("Reversed: " + reversed); // Output: madam
}
}
Output (set code file name as StringUtils.java):
madam is palindrome: true
Uppercase: MADAM
Reversed: madam
Explanation:
In the above code example-
- We start with a class called StringUtils that contains three static utility methods.
- The first method, isPalindrome(), takes a string str as input. It creates a reversed version of the string using StringBuilder and compares it with the original string to check if it’s a palindrome. If both are the same, it returns true; otherwise, it returns false.
- The second method, toUpperCase(), converts the input string str to uppercase using the built-in toUpperCase() method of the String class and returns the uppercase version of the string.
- The third method, reverseString(), reverses the input string str by utilizing StringBuilder's reverse method and then converts it back to a string before returning it.
- Inside the main() method, we test these utility methods using a string testString set to "madam".
- We check if the string is a palindrome by calling StringUtils.isPalindrome(testString) and print the result, which will output true since "madam" is a palindrome.
- We convert the string to uppercase using StringUtils.toUpperCase(testString) and print the result, which will output MADAM.
- We reverse the string using StringUtils.reverseString(testString) and print the result, which will output madam, the same as the original string because "madam" is a palindrome.
Sharpen your coding skills with Unstop's 100-Day Coding Sprint and compete now for a top spot on the leaderboard!
Using Static Method In Java To Implement The Singleton Design Pattern
The Singleton Design Pattern ensures that a class has only one instance and provides a global point of access to that instance. A key characteristic of the Singleton pattern is that it restricts the instantiation of the class to a single object.
To implement the Singleton pattern, we can use a static method that controls the creation of the object. The class will have a private static instance that is only created once and is returned every time the static method is called. This prevents multiple instances of the class from being created.
Here’s a simple example of how to implement the Singleton pattern in Java using a static method.
Code Example:
public class Singleton {
// Private static variable to hold the single instance
private static Singleton instance;
// Private constructor to prevent instantiation
private Singleton() {
// Initialization code here
}
// Public static method to provide access to the single instance
public static Singleton getInstance() {
// Create the instance if it doesn't exist
if (instance == null) {
instance = new Singleton();
}
return instance;
}
// Example method in Singleton class
public void showMessage() {
System.out.println("Hello from Singleton!");
}
public static void main(String[] args) {
// Access the Singleton instance using the static method
Singleton singleton = Singleton.getInstance();
// Calling the showMessage method on the Singleton instance
singleton.showMessage(); // Output: Hello from Singleton!
}
}
Output (set code file name as Singleton.java):
Hello from Singleton!
Explanation:
In the above code example-
- We have a class called Singleton, which implements the Singleton design pattern to ensure only one instance of the class can be created.
- The class contains a private static variable instance, which holds the single instance of the class.
- The constructor of the class is private, preventing direct instantiation of the Singleton class from outside. This ensures that no more than one instance can be created.
- The getInstance() method is a public static method that provides access to the single instance of the class. It checks if the instance is null, and if so, it creates a new instance of Singleton. If the instance already exists, it simply returns the existing instance.
- The showMessage() method is an example method inside the Singleton class that prints a message, "Hello from Singleton!", when called.
- In the main() method, we access the Singleton instance by calling Singleton.getInstance() and store it in the singleton variable.
- We then call the showMessage() method on the singleton object, which prints "Hello from Singleton!" to the console.
Difference Between Static And Instance Methods In Java
Static and instance methods in Java differ primarily in their association—static methods belong to the class, while instance methods operate on individual objects. Here’s a detailed comparison between the two:
Aspect |
Static Method |
Instance Method |
Belongs To |
The class itself. |
A specific object (instance) of the class. |
Invocation |
Can be called using the class name without creating an object. |
Requires an object of the class to be called. |
Access to Members |
Can only access static variables and methods directly. |
Can access both instance and static variables/methods directly. |
Keyword Availability |
Cannot use this or super keywords as they are instance-specific. |
Can use this and super to refer to current or parent objects. |
Polymorphism |
Does not support runtime polymorphism (method overriding). |
Supports runtime polymorphism (method overriding). |
Purpose |
Typically used for utility functions, constants, or shared operations. |
Used to define behaviors or operations that act on object-specific data. |
Memory Allocation |
Loaded into memory once, shared across all objects. |
Each object has its own copy of instance methods. |
Overriding |
Can be redefined in a subclass (method hiding). |
Can be overridden in a subclass to provide specific behavior. |
Example of Use Case |
Math utility functions like Math.sqrt() or factory methods. |
Object-specific operations like updating account balance in a bank application. |
Thread Safety |
Can introduce thread safety issues if modifying static variables. |
Typically safer since they act on instance-specific data. |
Access Modifier Flexibility |
Same access modifier rules apply. |
Same access modifier rules apply. |
Abstract Modifier |
Cannot be abstract. |
Can be abstract, requiring subclasses to implement them. |
Performance |
Slightly faster as no object reference is required for invocation. |
May involve additional overhead due to the use of object references. |
Syntax |
static return_type methodName(parameters) |
return_type methodName(parameters) |
Limitations Of Static Method In Java
While static methods are powerful and versatile, they come with several limitations. Here’s a detailed look at the constraints of using static methods in Java:
- Cannot Access Instance Members Directly: Static methods belong to the class, not to any specific object. As a result:
- They cannot access instance variables or call instance methods directly.
- They can only interact with static variables or methods. For Example-
public class Example {
private int instanceVar = 10; // Instance variable
public static void staticMethod() {
// instanceVar++; // Error: Non-static field cannot be referenced
}
}
- Lack of Polymorphism: Static methods are not part of the object’s instance and do not participate in runtime polymorphism (method overriding). They are resolved at compile time, making them less flexible. For example-
class Parent {
static void display() {
System.out.println("Parent");
}
}class Child extends Parent {
static void display() {
System.out.println("Child");
}
}public class Main {
public static void main(String[] args) {
Parent obj = new Child();
obj.display(); // Output: Parent (not Child)
}
}
- Limited to Static Context: Static methods can only use static context, which restricts their scope. They cannot use this or super keywords since these are instance-specific. For example-
public class Example {
public static void staticMethod() {
// System.out.println(this); // Error: Cannot use 'this' in static context
}
}
- Tight Coupling: Frequent use of static methods can lead to tight coupling between classes, making the code harder to maintain or test. Static methods lack the flexibility that instance-based methods provide for inheritance and dependency injection.
- Cannot Be Overridden: Although a static method can be redefined in a subclass, this is method hiding, not true method overriding. This reduces the effectiveness of dynamic behavior in object-oriented programming.
- Cannot Be Abstract: Static methods cannot be abstract, which means they cannot enforce implementation in derived classes. This limits their usability in abstract classes or interfaces. For example-
abstract class AbstractClass {
// abstract static void method(); // Error: Static methods cannot be abstract
}
- Reduces Flexibility: Static methods are tied to the class, making them less flexible for dynamic or object-specific behaviors. For instance, you cannot override them to change behavior per object.
- Thread Safety Concerns: If a static method modifies shared static data, it can lead to thread safety issues. Proper synchronization is required to handle concurrent access, which adds complexity.
- Testing Challenges: Testing static methods can be difficult because they are tied to the class and not an instance. Mocking or replacing their behavior during unit testing is more complex compared to instance methods.
- Harder to Extend: Static methods cannot take advantage of polymorphism, making it difficult to extend or customize their behavior in subclasses.
Are you looking for someone to answer all your programming-related queries? Let's find the perfect mentor here.
Conclusion
In Java, static methods offer a powerful way to design methods that belong to a class rather than instances of the class. By using the static keyword, we can create methods that can be called without the need to instantiate the class. This provides convenience and efficiency, especially for utility methods, constant values, and managing global state.
- Static methods play a key role in design patterns like the Singleton Pattern, where they help maintain a single instance of a class throughout the application's lifecycle.
- They also allow for utility classes that provide common functionality, like mathematical calculations or string manipulations, to be accessed globally without creating unnecessary objects.
- However, it's important to be mindful of their limitations, such as the inability to access instance-specific data and the potential for reduced flexibility in certain object-oriented designs.
In conclusion, while static methods are a valuable tool in Java, they should be used judiciously to ensure they align with the overall design and functionality of your application. When used correctly, static methods can enhance code efficiency, reusability, and clarity.
Frequently Asked Questions
Q. What is the purpose of using static methods in Java?
Static methods in Java are used to define methods that belong to the class rather than to instances of the class. They are useful for operations that do not require access to instance variables or methods. Common use cases include utility functions, constants, and managing global states. Static methods can be called directly using the class name, without creating an object of the class.
Q. Can a static method access instance variables?
No, a static method cannot directly access instance variables or instance methods because it is not tied to any particular instance of the class. Static methods can only interact with static variables and static methods of the class. If a static method needs to access instance variables, an object of the class must be created within the static method or passed as an argument.
Q. Can we override static methods in Java?
No, static methods cannot be overridden in Java. This is because static methods are resolved at compile-time, not runtime, and they are bound to the class rather than to an instance. If a static method with the same name is declared in a subclass, it hides (or "overrides" in a sense) the method in the parent class, but this is not true method overriding, which requires runtime polymorphism.
Q. What are the limitations of static methods in Java?
The key limitations of static methods include:
- No access to instance data: Static methods cannot access instance variables or non-static methods, limiting their ability to interact with object-specific data.
- No polymorphism: Since static methods are bound to the class, they don't exhibit polymorphic behavior like instance methods do.
- Limited to static context: They can only access other static members of the class, which can reduce flexibility in certain scenarios, especially in object-oriented designs that rely on instance-specific behavior.
Q. Can we call a static method from a non-static method?
Yes, a static method can be called from a non-static (instance) method. Since a non-static method belongs to an instance of the class, it has access to both static and instance members of the class. Therefore, within an instance method, you can directly call static methods using the class name or simply by referring to the method (since they are already accessible).
Q. What is the difference between a static method and an instance method?
The primary difference between static and instance methods is that:
- Static methods belong to the class and can be called without creating an instance of the class. They can only access static members of the class and cannot access instance variables or methods.
- Instance methods belong to an instance of the class and require an object to be created. They can access both instance and static variables/methods of the class. Instance methods are typically used for operations that require data specific to an object.
Q. How does a static method work in the Singleton Design Pattern?
In the Singleton Design Pattern, the static method plays a crucial role in ensuring that only one instance of the class exists. The static method (getInstance()) is responsible for creating the instance the first time it is called and returning the same instance for subsequent calls. This method prevents multiple instantiations of the class by controlling the creation of the object using the static keyword, ensuring a single, global point of access to the instance.
Q. Can static methods be accessed from other classes?
Yes, static methods can be accessed from other classes, as long as they are public or have an appropriate access modifier. Since static methods belong to the class and not to any instance, they can be called using the class name. For example, if MyClass has a static method doSomething(), you can access it from another class like this: MyClass.doSomething(). This allows static methods to be used as utility functions or helper methods that can be accessed globally across different parts of the program.
Q. What happens if a static method tries to access an instance variable directly?
If a static method tries to access an instance variable directly, it will result in a compilation error. This is because static methods are not bound to a particular instance of the class and thus do not have access to instance-specific data. Instance variables belong to an object, and since static methods don't operate on instances, they cannot access instance variables unless an object of the class is created and used within the static method. To fix this, you would need to pass an object of the class into the static method or access the instance variable through a reference to the class's object.
With this, we conclude our discussion on the static method in Java. Here are a few other topics that you might be interested in reading:
- Convert String To Date In Java | 3 Different Ways With Examples
- Final, Finally & Finalize In Java | 15+ Differences With Examples
- How To Find LCM Of Two Numbers In Java? Simplified With Examples
- How To Find GCD Of Two Numbers In Java? All Methods With Examples
- Volatile Keyword In Java | Syntax, Working, Uses & More (+Examples)
- Throws Keyword In Java | Syntax, Working, Uses & More (+Examples
I’m a Computer Science graduate with a knack for creative ventures. Through content at Unstop, I am trying to simplify complex tech concepts and make them fun. When I’m not decoding tech jargon, you’ll find me indulging in great food and then burning it out at the gym.
Comments
Add commentLogin to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
Esuru Pooja.C 2 hours ago
Ankita Das 2 days ago
Sunita Bhat 4 days ago
Mihir Kumar Ranjan 1 week ago