Method Overloading In Java | Ways, Rules & Type Promotion (+Codes)
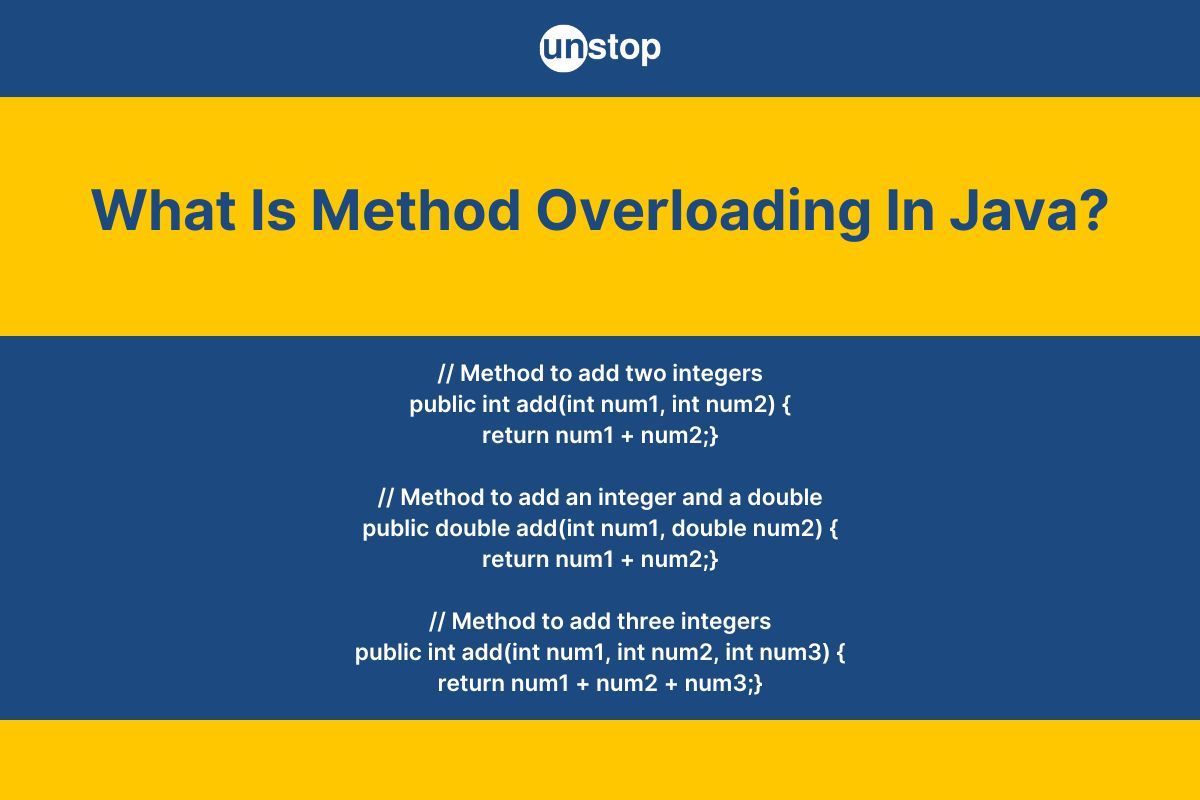
Imagine defining multiple methods with the same name, each tailored to handle different types or numbers of parameters. This is called method overloading—a feature that adds flexibility and streamlines code, making it more readable and maintainable.
In this article, we’ll explore the concept of method overloading in Java, covering its rules, examples, and various implementation strategies. We'll also discuss common pitfalls and best practices to help you master this essential Java feature.
What Is Method Overloading In Java?
Say you walk into a coffee shop and ask the barista to give you a coffee. Now, this could be any type–espresso, cappuccino, or latte, and any size–small, regular, or large. Even with so much variation, we are still talking about the same thing– Coffee, with the same basic process but different ingredients or methods.
This is similar to method overloading in Java, where you can have multiple methods with the same name/identifier but different parameter lists or signatures. This feature enables methods to perform similar tasks but on different types or numbers of inputs, making the code more flexible and easier to maintain. In short, it allows the Java program to handle different situations using the same method name.
Method overloading is a type of compile-time polymorphism where the compiler decides which method to invoke based on the method signature (the name and parameter list) at compile time/ during compilation. This allows developers to use the same method name for different operations, enhancing code readability and reducing clutter.
Syntax For Method Overloading In Java
accessModifier returnType methodName(parameterType1 parameter1, parameterType2 parameter2, ...) {
// Method implementation
}
Here,
- The accessModifier (optional component) specifies the visibility or accessibility of the method, which means from where all the given methods can be accessed from. It can be public, private, protected, or package-private (default).
- returnType specifies the type of data that the method will return after it has completed its execution. It can be any valid data type in Java, but if the method does not return any value, we should mark the return type as void.
- methodName refers to the name of the method. In method overloading, we can have multiple methods with the same name within the same class.
- The terms parameter1, parameter2... are the list of parameters the method accepts and parameterType1, parameterType2... are the data types of these parameters. We can have zero or more parameters, and they all are enclosed in parentheses. If there are multiple parameters, we should separate them by commas.
- Following all the components, we have the curly braces {} that contain the logical implementation part of the method/ lines of code.
Rules For Method Overloading In Java
Method overloading enhances code flexibility and readability, but it follows certain rules that help ensure the correct implementation. These rules are as follows:
- Same Class Rule: Method overloading in Java must occur within the same class. This means that all methods with the same name and different parameters should be defined in a single class.
- Parameter Change Rule: To overload a method, there must be a change in the method’s parameters. This could mean changing:
- The number of parameters.
- The types of parameters.
- The order of the parameters.
- Return Type Flexibility: While method overloading in Java allows flexibility in return types, it’s important to note that changing only the return type will not overload a method. The return type must be accompanied by a change in the data type of the method’s parameters.
- Access Modifier Change: You are free to modify the method’s access level (e.g., public, private, protected, or package-private). However, access modifiers alone cannot be used to differentiate overloaded methods.
- Exception Handling: Overloaded methods can throw different types of exceptions, depending on their implementation. You can modify the exception-handling behavior in overloaded methods to address specific errors.
Examples Of Method Overloading In Java
Now that we've got some idea about the syntax for method overloading in Java let's look at an example that illustrates this concept for better understanding. In the simple Java program example, we will define a Calculator class wherein we will overload a function called add() to perform the addition function on varied numbers and types of variables/ values.
Code Example:
Output:
Addition (int): 12
Addition (double): 6.2
Addition (int + double): 8.5
Addition (three integers): 6
Explanation:
In this example Java program,
- We first define a class Calculator, which contains methods to perform the addition arithmetic operation on various values, all with the public access modifier.
- Inside the class, we have used the method overloading concept to define four variations of the add() method. We overload it as follows:
- The first add() method takes two integer values as input, calculates their sum using the addition operator and returns an integer to the program.
- The second add() method takes two double type values as parameters, calculates and returns the sum of type double.
- The third add() method takes one integer and one double type value as input, calculates and returns their sum of type double.
- The fourth add() method takes three integer values as parameters, calculates their sum and returns the integer value.
- Next, we define the Main class containing the main() function, which is the entry point for the program’s execution.
- Inside, we first create an instance of the Calculator class, called calculator, using the new operator and the class constructor Calculator().
- Then, we call the add() method on the calculator object four times, with different types and number of arguments, as follows:
- First, we call the add() method by passing values 5 and 7 as arguments and store the outcome in the integer variable sumInt. This invokes the first method, as its definition matches the call.
- Second, we call add() by passing values 3.5 and 2.7 (both double) and store the outcome in the sumDouble variable. This invokes the second add() method.
- Third, we call add() by passing values 5 and 3.5 as arguments and store the outcome in the sumMixed variable, invoking the third version of the overloaded method.
- Lastly, we call add() by passing values 1, 2, and 3 arguments and store the outcome in the sumThreeIntegers variable, invoking the last version of the add() method.
- Finally, we print the outcomes stored in respective variables using the System.out println() method.
This example demonstrates how method overloading can be used to handle different numbers and types of parameters. The main idea is that each method version has a distinct signature, which allows the compiler to determine which method to call based on the provided arguments.
Want to expand your knowledge of Java programming? Check out this amazing course and become the Java developer of your dreams.
Different Ways Of Method Overloading In Java
As you must know by now, method overloading in Java allows you to create methods with the same name but different parameter lists. It enhances code flexibility and readability. There are three ways to achieve method overloading in Java.
You have already gotten a sneak peek into these methods in the example above, but here, we will discuss each of them in detail with the help of examples.
Changing The Number Of Parameters For Method Overloading In Java
In this approach, you create multiple methods within the same class, using the same method name but varying the number of parameters. Java distinguishes between these methods by their "method signature"—a unique combination of the method name and its parameter types and count.
The Syntax for Method Overloading in Java by Changing the Number of Parameters:
public class MyClass {
// Method with a specific number of parameters
public returnType methodName(parameterType1 param1, parameterType2 param2) {
// Method implementation
}
// Overloaded method with a different number of parameters
public returnType methodName(parameterType1 param1) {
// Method implementation
}
// Additional overloaded methods with different parameter counts
}
Here, we have overloaded the method methodName() by varying the number of parameters it takes. Each method version can perform similar actions but with flexibility in input.
Real-Life Example: Bookstore Cart
Consider a Bookstore class where customers can add either a single book or multiple books to their cart. In the Java code example below, we have created two overloaded addToCart methods: one for a single book and one for multiple books. Let’s see how this works.
Code Example:
Output:
Added the book: The Great Gatsby to the cart.
Added the book: To Kill a Mockingbird to the cart.
Added the book: 1984 to the cart.
Added the book: Brave New World to the cart.
Explanation:
In this example, we define a Bookstore class with two overloaded methods for adding books to a customer's cart. The methods are designed to handle different scenarios based on the number of books the customer wants to add.
- The first addToCart() method adds a single book to the cart. It takes one string-type object (book title) as input and prints a message to the console, indicating that a book was added.
- The second addToCart() method takes an array of strings (multiple book titles) as input and uses a for loop to print a series of messages, one for each book, indicating that a book was added to the cart.
- Then, we define the Main class with the main() method. Inside, we create an instance of the Bookstore class, bookstore.
- We then call the addToCart() method twice, with a different number of arguments.
- The first call with a single book name invokes the first version of the method, and the second call with an array containing 3 book titles invokes the second version.
Changing Data Types Of Parameters For Method Overloading In Java
In this method, you define multiple versions of a method with the same name, but each accepts different data types for parameters. This technique is useful for operations that apply to different types of input, enhancing flexibility and code reuse.
Syntax for Method Overloading in Java by Changing the Data Types of Parameters:
public class ClassName {
// Method with different data types
public ReturnType methodName(DataType1 parameter1, DataType2 parameter2) {
// Method implementation
// Return statement
}
// Overloaded method with different data types
public ReturnType methodName(DataType3 parameter1, DataType4 parameter2) {
// Method implementation
// Return statement
}
}
Here, the methodName method is overloaded by varying the data types of its parameters, enabling the same method to handle different types of data. Note that the returns type must be compatible with the type of parameters.
Real-Life Example: Temperature Converter
Here's an example to demonstrate method overloading by changing the data types of the arguments. We'll create a Converter class with overloaded methods to convert temperatures from Celsius to Fahrenheit and vice versa.
Code Example:
Output:
25.0 Celsius is equal to 77.0 Fahrenheit.
68 Fahrenheit is equal to 20.0 Celsius.
Explanation:
In this example, as mentioned, we create a Converter class with a method called convertTemprature() with two variations.
- The first convertTemprature() takes a double type input ( temperature in Celsius), uses a formula to convert it to Fahrenheit (integer type) and returns the same.
- The second convertTemprature() takes an integer type input (temperature in Fahrenheit), converts it to Celcius (type double) and returns the same.
- Then, in the Main class, we create an instance of the Converter class called converter.
- We then declare a double-type variable celsius and assign the value 25.0 to it. After that, we call the convertTemprature() method and store the outcome in the fahrenheit variable.
- This call invokes the method, which takes a double type as input, and we print the value to the console.
- Similarly, we initialize a variable fahrenheitTemp with value 68 and call convertTemprature() method on it.
The output confirms that our overloaded methods in the Converter class work correctly, providing accurate temperature conversions in both directions.
Changing Order Of Parameters For Method Overloading In Java
In this technique, the methods have the same name but differ by the order of their parameters. This subtle difference in parameter order creates unique method signatures, allowing for multiple methods with the same name.
Syntax for Method Overloading in Java by Changing the Order of Parameters of Methods:
public class ClassName {
// Method with changed parameter order (Version 1)
public returnType methodName(type1 parameter1, type2 parameter2) {
// Method implementation
}
// Method with changed parameter order (Version 2)
public returnType methodName(type2 parameter2, type1 parameter1) {
// Method implementation
}
}
Here, the same method name (methodName) is used, but the parameters are arranged differently, creating unique method signatures.
Real-Life Example: Transaction Processor
We will create a TransactionProcessor class in the Java example that allows for transactions either by specifying the amount first or the description first, showing how parameter order can influence functionality. When we call the method, Java will determine which version of the method to execute based on the order of arguments you pass when invoking it.
Code Example:
Output:
Processing transaction (amount first):
Amount: INR500.0
Description: Purchase of booksProcessing transaction (description first):
Description: Meal expenses
Amount: INR300.0
Explanation:
In the sample Java code,
- We define a TransactionProcessor class with a method processTransaction() that we hav is overloaded by differing the sequence of parameters it takes.
- The first processTransaction() method expects the amount (double) first, followed by the description (String).
- The second processTransaction() method accepts the description first and the amount second.
- In the Main class, we create an instance of the TransactionProcessor class named processor.
- Then, we call the processTransaction() method twice, first by passing an number followed by a string as arguments, and then by passing a string followed by a number as arguments.
This code demonstrates method overloading by having two methods with the same name (processTransaction) but different parameter orders.
Overloading The main() Method In Java
In Java, the main() method plays a critical role as the program's entry point. Its signature is specific and non-modifiable: public static void main(String[] args). This often raises the question– can we create multiple main() methods with varying parameter lists and, thus, overload the main() method itself?
The answer is yes — we can overload the main() method in Java. According to the rules of method overloading, we can create multiple methods with the same name, main, as long as each has a different parameter list. However, the JVM (Java Virtual Machine), which provides the runtime environment for executing Java bytecode, will always look specifically for the standard public static void main(String[] args) signature as the entry point when running a Java program.
Syntax (Overloaded main() Method):
public class ClassName {
// Overloaded main() method
public static void main(DataType1 arg1, DataType2 arg2, ...) {
// Method implementation
}
}
Here,
- The public specifier indicates that the main() method is accessible from anywhere, meaning it can be called from outside the class.
- The static keyword means the method belongs to the class, not instances of the class. This allows it to be called without creating an object of the class.
- The void keyword means the method does not return any value.
- DataType1, DataType2, ... represent the types of parameters the overloaded main() method can accept. This differs from the standard main() method, which accepts a String[] args array.
Each overloaded main() method can have a different parameter list, allowing you to pass different types and numbers of arguments. Multiple overloaded main() methods with different parameters can exist in the same class. Look at the simple example Java program below for a better understanding of how to overload the main() method.
Code Example:
Output:
Standard main() method
Explanation:
In the Java code example,
- We begin by defining the Main class that includes the standard public main() method which takes String[] args as parameter.
- When we run this class, only the standard main() method is triggered as the program’s entry point. This method iterates over any command-line arguments (stored in args) using a for loop and prints each one.
- In addition to the entry-point method, we’ve defined three other main() methods to demonstrate overloading:
- The second method is an overloaded main() that takes an integer (int x) as a parameter.
- The third method is another overloaded main() that accepts a single string (String name).
- The fourth method is an overloaded main() that accepts a double (double value).
- Each overloaded method prints a unique message when called directly.
- While the standard main() prints "Standard main() method" and iterates through command-line arguments, the other main() versions display custom messages when they receive their respective parameters.
- As shown in the output, when the class is run, the additional methods don’t act as entry points.
- However, they can be explicitly called from within the standard main() or elsewhere in the program.
Here is your chance to top the leaderboard while practising your coding skills: Participate in the 100-Day Coding Sprint at Unstop.
Type Promotion & Method Overloading In Java
Type promotion refers to the automatic promotion of lower members of a data type hierarchy to upper members in order to find the best match when passing parameters to an overloaded method. This ensures that the method that fits the provided arguments most accurately is selected. Java follows specific rules to determine the most suitable overloaded method.
Type Promotion Hierarchy For Method Overloading In Java
Java uses a hierarchy for type promotion when selecting the best matching overloaded method, as follows:
- Exact Match: The method with the exact parameter type match is selected first.
- Widening Primitive Conversion: If an exact match isn’t found, Java attempts to find the closest match by applying widening primitive conversions. For instance, if a method expects an int, but a short is provided, Java promotes the short to an int.
- Autoboxing: If neither an exact match nor a widening conversion is available, Java checks for autoboxing, where primitive types like int can be converted to their corresponding wrapper classes (e.g., Integer).
- Varargs: If none of the above rules work, Java checks for methods that accept varargs (variable-length argument lists) as a last resort.
When we invoke an overloaded method with arguments, the Java compiler applies these promotion rules to determine the most appropriate method to call based on the provided argument data types.
Syntax for Type Promotion in Method Overloading in Java:
class ClassName {
// Method with a specific parameter type
returnType methodName(dataType1 parameterName1) {
// Method implementation for dataType1
}
// Overloaded method with a different parameter type
returnType methodName(dataType2 parameterName2) {
// Method implementation for dataType2
}
// Additional overloaded methods with different parameter types
// ...
}
// Main class to execute the program
public class Main {
public static void main(String[] args) {
ClassName object = new ClassName();
// Call overloaded methods with arguments that may undergo type promotion
object.methodName(argument1); // Calls the appropriate overloaded method
object.methodName(argument2); // Calls the appropriate overloaded method
// ...
}
}
Here,
- ClassName represents the name of the class containing the overloaded methods.
- The methodName is the name of the method being overloaded and returnType specifies the data type of the value it returns.
- Terms dataType1, dataType2,… represent different parameter data types for the overloaded methods where parameterName1, parameterName2,... are the names of the method's parameters.
- The terms argument1, argument2,... are the actual values or variables passed as arguments to the overloaded methods.
In the main method, the Java compiler will decide which overloaded method to call based on the arguments passed and the method’s parameter types. If no exact match is found, it will apply type promotion according to the rules above to select the best match.
Examples Of Type Promotion With Method Overloading In Java
Let's explore method overloading in Java with type promotion through a real-life scenario. In this case, we will consider two examples that showcase how overloaded methods handle different types of arguments, including when type promotion happens implicitly.
Example 1: Normal Type Promotion With Method Overloading In Java
Imagine you're writing a Java program to calculate electricity bills. You want to create overloaded methods for calculating the bill based on different parameters:
- Method 1: Calculate the bill based on units consumed (integer input).
- Method 2: Calculate the bill based on the number of days the electricity was used (integer input).
- Method 3: Calculate the bill based on both units consumed and the number of days (integer inputs).
We’ll define overloaded methods to accommodate these scenarios and demonstrate how type promotion works when calling these methods.
Code Example:
Output:
Bill based on units consumed: Rs. 502.5
Bill based on days of usage: Rs. 14.0
Bill based on units and days: Rs. 773.75
Explanation:
In this example Java code, we have created an ElectricityBillCalculator class with three overloaded methods to calculate electricity bills based on units consumed, days of usage, or a combination of both.
- As mentioned in the code comments, the first calculateBill() method expects the units of electricity (float type parameter) consumed and calculates the bill based on that.
- The second calculateBill() method expects the number of days of usage (integer type parameter) and calculates the bill.
- The third calculateBill() method expects units consumed and days of consumption (one float and one integer parameter) and calculates the bill based on both.
- Then, we define the Main class, with the main() function, inside which we create an instance of the ElectricityBillCalculator class.
- We then call the calculateBill() method thrice, as follows
- The first call with a float value (100.5f) invokes the first method, and the Java compiler promotes float in the calculation to double.
- The second call with an integer value (7) invokes the second method, where Java promotes int to double type for the calculation.
- In the third call, we pass one int (10) and one float value (150.75f), invoking the last method, where Java promotes the types to meet the function definition.
- In this example, type promotion happens naturally when float or int arguments interact with double constants in the methods.
Example 2: Type Promotion & Method Overloading In Java With Ambiguity
Imagine you're developing a ShippingCalculator for an international e-commerce platform. You want to create overloaded methods to calculate shipping costs based on the weight of items, which can be provided in either kilograms or pounds. However, ambiguity arises when customers provide weights using both data types, leading to a compilation error. This ambiguity can occur due to type promotion in overloaded methods.
Code Example:
Output:
Main.java:15: error: reference to calculateCost is ambiguous
calculator.calculateCost(10, 2); // Ambiguity: Could be kilograms or pounds
^
both method calculateCost(int,long) in ShippingCalculator and method calculateCost(long,int) in ShippingCalculator match
Main.java:16: error: reference to calculateCost is ambiguous
calculator.calculateCost(15, 5); // Ambiguity: Could be kilograms or pounds
^
both method calculateCost(int,long) in ShippingCalculator and method calculateCost(long,int) in ShippingCalculator match
2 errors
Explanation:
In this example,
- We create a ShippingCalculator class containing two overloaded methods for calculating shipping costs.
- Each method calculates the cost based on the weight and quantity of items, but each accepts different data types for the arguments.
- The first calculateCost() method accepts an int for weight in kilograms and a long for quantity.
- The second calculateCost() definition of the method accepts a long for weight in pounds and an int for quantity.
- Then, we define the Main class with the main() method.
- Inside, we attempt to call the calculateCost() method twice, but both calls cause an ambiguity.
- In the first call calculator.calculateCost(10, 2), the arguments 10 and 2 could be interpreted in two ways:
- 10 as weight in kilograms (int) and 2 as quantity (long), which would match Method 1.
- Or 10 as weight in pounds (long) and 2 as quantity (int), which would match Method 2.
- The compiler can’t decide which method to invoke because the argument types are ambiguous and match both method signatures.
- Similarly, in the second call calculator.calculateCost(15, 5,) the arguments could also be interpreted as either kilograms and quantity (int, long) or pounds and quantity (long, int), leading to the same ambiguity.
- As shown in the output, when you compile this code, it throws a compiler error with a message. This error message indicates that the compiler is unable to determine which method to call because the argument types are too similar to resolve the ambiguity automatically.
Resolving the Ambiguity:
To resolve the ambiguity, we can make the argument types distinct enough for the compiler to identify which method to invoke. One way to do this is by passing explicit type information (using type casting) or adjusting the arguments in a way that each overloaded method matches uniquely. The Java code example below is the modified and corrected version of the previous one.
Code Example:
Output:
Calculating shipping cost based on weight in pounds: 20 lbs
Calculating shipping cost based on weight in kilograms: 75 kg
Null Error & Method Overloading In Java
We already know that method overloading in Java programs is a common technique that allows multiple methods within a class to share the same name but have different parameter lists. This improves code readability and flexibility.
- However, when overloaded methods can both accept null as an argument, it can lead to a compile-time ambiguity.
- This ambiguity arises because the compiler is unable to determine which method to call when null is passed as an argument.
To understand this issue and how it can be mitigated, let’s walk through a real-life scenario.
Suppose we’re building an online booking system for a theater. Here, you can overload the methods to reserve seats based either on seat numbers or customer names. However, if null is passed as an argument, the compiler encounters ambiguity when trying to decide which method to invoke. Let’s see how this issue occurs and how we can resolve it.
Code Example:
Output:
java: reference to reserveSeat is ambiguous both method reserveSeat(java.lang.Integer) in TheaterBookingSystem and method reserveSeat(java.lang.String) in TheaterBookingSystem match
Explanation:
In this example,
- We have a TheaterBookingSystem class with two overloaded methods to reserve seats:
- Method 1 (reserveSeat(Integer seatNumber)): Reserves a seat based on a seat number (an Integer argument).
- Method 2 (reserveSeat(String customerName)): Reserves a seat for a customer based on their name (a String argument).
- In the main() method, we call bookingSystem.reserveSeat(null). However, since both methods can accept null (either as an Integer or a String), the compiler doesn’t know which method to choose, leading to an ambiguity error.
Compiler Error:
The error message shown in the output above indicates that both overloaded methods match the null argument, making it unclear which method should be called.
Resolving the Ambiguity:
We can resolve this ambiguity by explicitly specifying the type of the null argument. By casting null to a specific type, we provide the necessary information for the compiler to choose the correct overloaded method. The sample Java code below illustrates how we can make the call unambiguous.
Code Example:
Output:
Reserving seat number: null
Explanation:
In this revised example,
- We declare an Integer variable seatNumber and set it to null. This explicitly specifies the type of the argument, making it clear that we intend to call the method that accepts an Integer.
- When we pass seatNumber to bookingSystem.reserveSeat(), the compiler now selects the reserveSeat(Integer seatNumber) method without any ambiguity.
- The program executes successfully, and we see the output: Reserving seat number: null.
Note: When multiple overloaded methods can accept null as an argument, ambiguity may arise. To resolve this, explicitly specify the argument type by casting or by using variables of specific types. This approach ensures that the compiler can unambiguously choose the correct method.
Need more guidance on how to become a Java developer? You can now select an expert to be your mentor here.
Advantages Of Method Overloading In Java
Method Overloading is a powerful feature in Java that brings several significant benefits to coding practices, enhancing code readability, reusability, and maintainability. Here are the major advantages of method overloading in Java programming:
- Easy to Understand and Flexible: Overloaded methods make code more readable, like having different tools with the same purpose but customized for specific needs. For example, you can have a calculate method that handles different types of data, making it easier to understand the purpose without introducing new method names.
- Polymorphism and Code Reusability: Method overloading in Java allows us to use the same method name for similar actions with different parameters. This avoids the need for separate methods for each variation, making the code more compact and reusable. Instead of creating distinct methods for slight differences, we can define multiple versions of the same method, enhancing flexibility and reducing code duplication.
- Simplified Maintenance: When updates are needed, method overloading in Java programs lets us modify a specific overloaded method rather than adding new ones. This simplifies code maintenance since all similar functionality is consolidated within one method name.
- Optimized for Better Performance: By avoiding redundant methods, overloading reduces the overall method count in a class, resulting in more compact, efficient code. With fewer redundant calls and smaller method lists, the program runs more efficiently, potentially boosting performance.
Disadvantages Of Method Overloading In Java
While method overloading offers many benefits, it also comes with some challenges:
- Potential for Ambiguity: When multiple overloaded methods can accept similar argument types (e.g., int and long or String and Integer), ambiguity can arise, leading to compilation errors. This occurs because the compiler may be unable to determine which overloaded method to call, especially when null or similar ambiguous values are passed.
- Increased Complexity with Many Overloads: Having too many overloaded methods can make the code harder to read and maintain, as it can be difficult to keep track of which method corresponds to which parameters. This is particularly true in large classes with several overloaded methods, leading to potential confusion for developers.
- Reduced Code Clarity: Method overloading in Java can sometimes obscure the intent of a method call. If different overloads perform slightly different tasks, it can make the code less intuitive, especially for new Java developers or those unfamiliar with the specific overloads in use.
- Compiler Overhead: Overloaded methods introduce additional work for the compiler, which needs to resolve the correct method to invoke based on the parameters. In some cases, this may introduce a slight overhead, impacting compilation time, though this is usually minimal.
Conclusion
Method overloading in Java basically allows multiple methods to share the same name but with different parameter configurations, enabling us to write code that is both adaptable and intuitive. There are three ways of overloading methods, i.e., by changing the number of parameters, their type or the order in which a function expects them.
Also, one must be mindful of the essential rules that govern method overloading in Java language, such as defining all overloaded methods in the same class and more. We also discussed nuances such as type promotion and handling potential pitfalls, like ambiguity with null values. We’ve also tackled a common question—whether the main() method can be overloaded—along with examining the many benefits that overloading brings, including improved readability and minimized redundancy.
Whether you're a beginner or an experienced Java developer, understanding method overloading can significantly enhance your Java coding skills, equipping you to create more elegant, efficient solutions to a wide range of real-world challenges.
Also read: Top 100+ Java Interview Questions And Answers (2024)
Frequently Asked Questions
Q. Does Java support Operator Overloading?
No, the Java programming language does not support operator overloading. In languages that allow it, operator overloading redefines or extends the behavior of operators (like +, -, *, /) for user-defined data types.
In Java, the behavior of operators is fixed and predefined for the built-in data types (integers, floating-point numbers, etc.), and we cannot change this behavior or define custom operator behaviors for our classes. Java does not provide operator overloading for safety and simplicity reasons, as it helps avoid potential confusion and ambiguities in code.
Q. Can we overload methods in the same class with the same parameters but different return types?
No, Java does not allow method overloading based on return type alone. Overloaded methods must differ in their parameter list (number, types, or order of parameters). If two methods have identical parameter lists but different return types, the compiler throws an error as it cannot distinguish between them based solely on the return type.
Code Example:
Output:
Main.java:7: error: method add(int,int) is already defined in class MethodOverloadingDemo
public double add(int a, int b) {
^
1 error
Explanation:
Here, we attempt to overload a method named add() in the MethodOverloadingDemo class. However, both methods have identical names and parameters, which leads to a compilation error since the return type alone cannot differentiate overloaded methods.
Q. Can we overload the static method in Java?
Yes, static methods can be overloaded based on their parameter list. Overloading is determined by the method's name and its parameters, and static methods follow the same rule.
Code Example:
Output:
Message: Hello, world!
Number: 42
Message: Overloading, Number: 123
Explanation:
In this example, each printMessage method accepts different parameter types, demonstrating method overloading with static methods.
Q. How does method overloading differ from method overriding in Java?
The table below highlights the key differences between method overriding and method overloading in Java.
Aspect |
Method Overloading |
Method Overriding |
Parameter List |
Must differ in type, number, or order. |
Must be the same (name, types, and order). |
Return Type |
Can be different, but return type alone doesn’t distinguish them. |
Must be the same as the overridden method. |
Access Modifiers |
Can vary between overloaded methods. |
Must be the same or more permissive than the superclass. |
Polymorphism |
Compile-time (static) polymorphism. |
Runtime (dynamic) polymorphism. |
Usage |
Multiple ways to perform similar operations within a class. |
Customizing superclass behavior in a subclass. |
Q. Is there any performance impact when using method overloading in Java?
No, method overloading does not introduce significant performance overhead. Since method selection occurs at compile time (known as compile-time polymorphism), there’s no runtime cost associated with resolving overloaded methods. The compiler selects the appropriate method version based on the number, types, and order of parameters, ensuring efficient byte-code execution.
This means that the Java Virtual Machine (JVM) does not need to perform any further runtime method resolution or lookup to decide which method to execute. Hence, method overloading in Java doesn't introduce extra runtime costs related to its execution.
Q. What is the relationship between method overloading and the "this" keyword in Java?
The 'this' keyword is used to reference the current instance of the class. In the context of method overloading, it helps distinguish between instance variables and method parameters, especially when they have the same names.
Code Example:
Output:
Name: Sachin
Name: Virat Kohli
Explanation:
In this code, we have a Person class with two setName methods that are overloaded based on the number of parameters. We use the "this" keyword to refer to the instance variable name when setting its value inside these methods. In the main method, we demonstrate how to use the overloaded methods to set and display the person's name.
The "this" keyword helps us distinguish between the instance variable name and the method parameter name. It makes the code more readable and avoids ambiguity, especially in the cases of overloading methods with similar parameter names.
This compiles our discussion on method overloading in Java. Here are a few other interesting Java topics you must explore:
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment