Table of content:
- What Are Data Types In Java?
- Primitive Data Types In Java
- Non-Primitive Data Types In Java
- Key Differences Between Primitive And Non-Primitive Data Types In Java
- Conclusion
- Frequently Asked Questions
Data Types In Java | Primitive & Non-Primitive With Code Examples
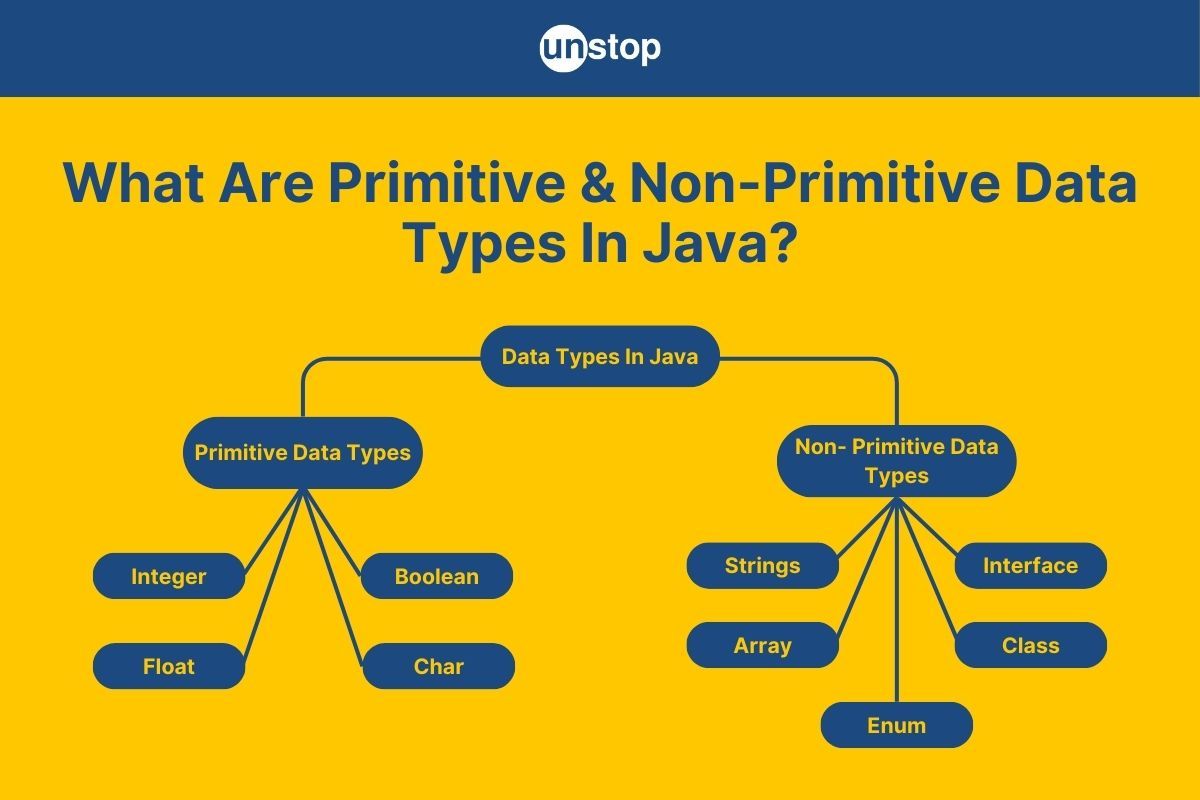
Data types are basic components of any programming language as they define the different kinds of data that can be stored in a variable. In other words, they stipulate the kind of information that our programs can work with. In this article, we will explore data types in Java, as understanding them is crucial for writing code that not only works but also works efficiently. Throughout this article, we will discuss various data types in Java programming language, their use cases, applications, and behaviour.
What Are Data Types In Java?
When we talk about data types in Java, we're essentially talking about the fundamental units that define the type of data we can work with. They also stipulate the different sizes of values to be stored in a variable.
Mentioning the data type for a variable or function sends a signal to the compiler indicating how the programmer intends to store, manage, and manipulate the respective data variable or method. Data types in Java can be categorized into two groups: primitive and non-primitive types (also called reference data types).
- Java Primitive Data Types: These are the building blocks of Java programming, as they define the basic types. We can use them to define non-primitive data types, but not the other way around.
- Non-primitive Data Types: These are more complex data types in Java that act more like organizers, helping us manage complex data structures.
With that basic understanding of these datatypes in Java, let us explore their types individually in depth.
Primitive Data Types In Java
As mentioned above, primitive data types in Java serve as the building blocks of data manipulation and are not objects, meaning they hold simple values. They are predefined by the language and named by a keyword.
- They define the basic types of values/ data/ information that we can work with, in a program. Think of them as the sturdy foundation upon which our digital creations stand.
- In other words, they help indicate the kind of values we are storing in memory.
- Some examples of primitive types include integer type (int) for whole numbers, floating-point data type (float) for decimals, character type (char) for individual/ single characters, boolean type representing true and false, etc.
- Each of these primitive data types in Java has a specific size and range, allowing developers to choose the most appropriate type based on the nature of the data and memory constraints.
Hence, the primitive data types in Java make it easier to define the type of data and the memory space a variable storing the data can occupy.
Key Characteristics Of Primitive Data Types In Java
- Simplicity: Primitive types are the basic building blocks of data in Java. They are not objects and do not have methods associated with them. Their simplicity allows for efficient storage and manipulation of fundamental data values.
- Fixed Size: Each primitive data type has a predefined size and range. This ensures that the memory allocation for these types is consistent and predictable, which is important for performance and memory management.
- Direct Value Storage: Primitive data types in Java directly store their values, unlike non-primitive types which store references to objects. This means operations on primitive types are performed directly on the data they contain.
- No Methods: Primitive data types in Java do not have methods. They are used purely for storing and manipulating simple values. Operations on primitive types are performed using operators and built-in language constructs.
- Default Values: Each primitive data type in Java has a default value assigned to it when it is declared but not explicitly initialized. For example, the default value for int is 0, and for boolean, it is false.
Now that we know what primitive data types are, let's look at their subtypes and understand them with the help of code examples.
Integer Types/ Data Type In Java
Integer data types in Java encompass all types used to depict whole numbers, such as int, byte, short, etc.
- Each of these integer primitive data types in Java varies in size, memory usage, and range, catering to different needs in programming.
- Whenever declaring a variable of a specific type, we use the respective keyword (like int, byte, short, long, etc.) before the variable name.
- This data type refers to the type of the variable values.
- The same applies to functions/ methods. When we want to specify the data type of a method's return value, we use the respective keyword before the function name.
- Another thing to note is, when we say that data type is signed, it means that it can store both positive and negative values.
Let's look at the subtypes of the integer data type in Java, followed by a basic Java program example.
The Byte Data Type: The byte data type is an 8-bit signed two's complement integer. This primtive data type in Java is denoted by the byte keyword and has a memory consumption of 1 byte. The byte data type stores signed values used when memory conservation is crucial, such as in large arrays or streams. Syntax:
byte variableName = value;
The Integer Data Type: The int data type is a 32-bit signed two's complement integer with a memory size of 4 bytes. The 32-bit integer type is the most commonly used integral type, suitable for general-purpose integer arithmetic. Syntax:
int variableName = value;
The Short Data Type: This is a 16-bit signed two's complement integer equivalent to a memory size of 2 bytes. This integer type stores/ allows a shorter range of integral values than the traditional int type. It is especially useful for saving memory in large arrays, where the memory savings from using shorts instead of ints are significant. Syntax:
short variableName = value;
The Long Data Type: This type is a signed 64-bit two's complement integer that uses memory of 8 bytes. It is the opposite of the short type, as it covers a wider range than the traditional int type. In other words, the long type is used when a wider range of values than those provided by int is needed. Syntax:
long variableName = value;
Let's look at a Java program example that demonstrates how to declare, initialize, and then print variables of these basic integer types.
Code Example:
cHVibGljIGNsYXNzIEludGVnZXJUeXBlc0V4YW1wbGUgewpwdWJsaWMgc3RhdGljIHZvaWQgbWFpbihTdHJpbmdbXSBhcmdzKSB7CgpieXRlIGEgPSAxMDsKaW50IGIgPSA1MDAwMDsKc2hvcnQgYyA9IDEwMDA7CmxvbmcgZCA9IDEwMDAwMDAwMDBMOwoKU3lzdGVtLm91dC5wcmludGxuKCJieXRlOiAiICsgYSk7ClN5c3RlbS5vdXQucHJpbnRsbigiaW50OiAiICsgYik7ClN5c3RlbS5vdXQucHJpbnRsbigic2hvcnQ6ICIgKyBjKTsKU3lzdGVtLm91dC5wcmludGxuKCJsb25nOiAiICsgZCk7Cn0KfQ==
Output:
byte: 10
int: 50000
short: 1000
long: 1000000000
Explanation:
- We begin by declaring a public class named IntegerTypesExample. In Java, a class is a blueprint for creating objects, and it can contain methods to define the behavior of these objects.
- Then, we define the main method, which is the entry point of any Java application. It is public (i.e., is accessible from outside the class), static (i.e., can be called without creating an instance of the class), and returns void (no return value).
- It takes a single argument, an array of String objects (String[] args), which can store command-line arguments.
- Inside the main, we declare four variables of different integer data types and initialize them as follows:
- Variable a is of type byte, an 8-bit signed integer type that can hold values from -128 to 127. Here, a is assigned the value 10.
- Variable b is of type int, a 32-bit signed integer type that holds values from -2,147,483,648 to 2,147,483,647. Here, b is assigned the value 50000.
- Variable c is of type short, a 16-bit signed integer type that can hold values from -32,768 to 32,767. Here, c is assigned the value 1000.
- Variable d is of type long, a 64-bit signed integer type that can hold values from -9,223,372,036,854,775,808 to 9,223,372,036,854,775,807. Here, d is assigned the value 1,000,000,000. Note the L suffix, which indicates that the value is of type long.
- Then, we use the System.out.println() statements to print the values of the variables to the console.
- The formatted string inside println() concatenates a string message (the name of the type) with the value.
The Float Data Types In Java
The float types represent single-precision 32-bit floating-point numbers. These primitive data types in Java are used when decimal precision is required, but memory conservation is also a concern. In other words, they should not be used to store whole/ precise values with no decimal places. There are two subtypes of float with varied ranges and sizes.
The Float Data Type: The float type is a single-precision 32-bit IEEE 754 floating-point number with memory usage of 4 bytes. The float data type is used for applications that require smaller decimal values and where memory conservation is crucial. Syntax:
float variableName = value;
The Double Data Type: This is a double-precision 64-bit IEEE 754 floating-point number that takes up to 8 bytes of space in memory. The double type is most commonly used floating-point type, offering higher precision than float. Syntax:
double variableName = value;
Let's look at an example Java program that illustrates the use of the float and double data type.
Code Example:
cHVibGljIGNsYXNzIEZsb2F0RG91YmxlRXhhbXBsZSB7CnB1YmxpYyBzdGF0aWMgdm9pZCBtYWluKFN0cmluZ1tdIGFyZ3MpIHsKCmZsb2F0IGEgPSAxMC41ZjsKZG91YmxlIGIgPSAxMjMuNDU2OwoKU3lzdGVtLm91dC5wcmludGxuKCJmbG9hdDogIiArIGEpOwpTeXN0ZW0ub3V0LnByaW50bG4oImRvdWJsZTogIiArIGIpOwp9Cn0=
Output:
float: 10.5
double: 123.456
Explanation:
In the example Java code-
- We begin by defining a public class named FloatDoubleExample and then the public main method.
- Inside the main method, we declare two variables of float and double data types and initialize them as follows:
- Variable a is of type float, a 32-bit floating-point data type that can represent fractional numbers with single precision. It is assigned the value 10.5, where the suffix f is required to indicate that the literal is of type float.
- Variable b is of type double, a 64-bit floating-point data type that can represent fractional numbers with double the precision of float. Here, b is assigned the value 123.456
- Then, we use the System.out.println() statements to print the values of these variables.
The Boolean Data Types In Java
The boolean types represents a data type that can have one of two possible values: true or false. It is commonly used as a flag variable to track true or false conditions in logical operations and conditional statements, thus controlling the flow of a program.
The size of the type boolean is not defined precisely but it typically uses 1 bit of memory. However, this may vary as per the various JVM implementations.
Syntax:
boolean variableName = value;
Here, boolean is the keyword used to indicate that the value stored in the variable identified by the name variableName is of type boolean. Below is a sample Java program illustrating the use of this data type.
Code Example:
cHVibGljIGNsYXNzIEJvb2xlYW5FeGFtcGxlIHsKcHVibGljIHN0YXRpYyB2b2lkIG1haW4oU3RyaW5nW10gYXJncykgewoKYm9vbGVhbiBpc0phdmFGdW4gPSB0cnVlOwpib29sZWFuIGlzQ29kaW5nSGFyZCA9IGZhbHNlOwoKU3lzdGVtLm91dC5wcmludGxuKCJJcyBKYXZhIEZ1bj8gIiArIGlzSmF2YUZ1bik7ClN5c3RlbS5vdXQucHJpbnRsbigiSXMgQ29kaW5nIEhhcmQ/ICIgKyBpc0NvZGluZ0hhcmQpOwp9Cn0=
Output:
Is Java Fun? true
Is Coding Hard? false
Explanation:
- We first define a public class called BooleanExample and initiate the main method.
- Inside main, we declare two boolean variables, isJavaFun and isCodingHard, and initialize them with the values true and false, respectively.
- Then, we use the System.out.println() statements to print the values of these variables along with descriptive text.
Char Data Type In Java
The char type represents a single 16-bit Unicode character that takes up to 2 bytes of memory space. This data type can store any character (single character) from the Unicode character set, including letters, digits, and special symbols.
Syntax:
char variableName = 'value';
Here, the char keyword indicates the type of the value stored inside the variable by the name variableName. All values stored in this type is enclosed in single quotes, as shown in the Java program sample below.
Code Example:
cHVibGljIGNsYXNzIENoYXJFeGFtcGxlIHsKcHVibGljIHN0YXRpYyB2b2lkIG1haW4oU3RyaW5nW10gYXJncykgewovLyBEZWNsYXJhdGlvbiBhbmQgaW5pdGlhbGl6YXRpb24gb2YgY2hhciB2YXJpYWJsZXMKY2hhciBncmFkZSA9ICdBJzsKY2hhciBzeW1ib2wgPSAnJCc7CmNoYXIgbGV0dGVyID0gJ1onOwoKLy8gUHJpbnRpbmcgdGhlIHZhbHVlcyBvZiB0aGUgY2hhciB2YXJpYWJsZXMKU3lzdGVtLm91dC5wcmludGxuKCJHcmFkZTogIiArIGdyYWRlKTsKU3lzdGVtLm91dC5wcmludGxuKCJTeW1ib2w6ICIgKyBzeW1ib2wpOwpTeXN0ZW0ub3V0LnByaW50bG4oIkxldHRlcjogIiArIGxldHRlcik7Cn0KfQ==
Output:
Is Java Fun? true
Grade: A
Explanation:
- We declare a public class named CharExample and then the main method, which is the program's entry point for execution.
- Inside main, we declare three variables of char type (it can hold a single 16-bit Unicode character) and initialize them as follows:
- The variable grade is assigned the character value A.
- Variable symbol is assigned the character value $, which is a special symbol belonging to the character set.
- Variable letter is assigned the value Z, which is an alphabet (just like A).
- Then, we use System.out.println() statements to print the values of these variables along with descriptive text.
Why Is The Size Of char Data Type In Java 2 Bytes?
In Java, the size of the char data type is 2 bytes because it is designed to store Unicode characters.
- Each Unicode character is represented using a 16-bit encoding scheme, which means it requires 2 bytes of memory.
- This allows Java to support a wide range of characters from different languages and writing systems worldwide.
- By allocating 2 bytes (16 bits) for each char, Java ensures that it can accommodate the entire Unicode character set, providing support for internationalization and multilingual applications.
- This larger size, compared to some other data types, allows Java to handle a diverse range of characters without sacrificing compatibility or precision.
Non-Primitive Data Types In Java
Non-primitive data types, also known as reference types, are more complex than primitive data types in Java. Unlike primitive types, which directly store values, non-primitive types store references (memory address) to the objects they represent.
- This distinction allows non-primitive types to be used to model complex data structures and to encapsulate data and behavior in a more organized manner.
- In this sense, non-primitive types are user-defined data types and are not defined by Java itself (except for String).
- These types store references to the actual data rather than the data itself, allowing for the creation of more complex structures of data and enabling the use of methods (functions) to perform operations on the data.
- Non-primitive types are essential for object-oriented programming, providing the foundation for building sophisticated applications.
When you're dealing with a non-primitive type, you're not just storing the value directly like you do with primitive types. Instead, it's more like you're leaving a little breadcrumb trail- a reference, pointing to where the actual object lives (memory location) in the computer's memory.
Key Characteristics Of Non-Primitive Data Types In Java
- Reference-Based Storage: Non-primitive types store references to objects rather than the actual data. When you assign a non-primitive variable to another variable, you are copying the reference, not the actual object. Changes made to one reference affect all references pointing to the same object.
- Customizable: Non-primitive data types in Java can be defined by users, allowing for the creation of custom data structures and classes tailored to specific needs. This is in contrast to primitive types, which are predefined and fixed in size and behavior.
- Support for Methods: Non-primitive types can contain methods that define behaviors and operations on the data they encapsulate. This is central to object-oriented programming, where data and functions are bundled together in classes.
- Dynamic Size: Unlike primitive types, which have a fixed size, non-primitive data types in Java can dynamically change in size. For example, arrays can grow or shrink in size depending on the implementation or data added to them.
- Inheritance and Polymorphism: Non-primitive data types in Java support inheritance and polymorphism, which are key features of object-oriented programming. Classes can inherit from other classes, and objects can be treated as instances of their parent classes.
String Non-Primitive Data Type In Java (Character Array)
The string type is a non-primitive data type in Java used to represent a sequence of characters. To be more precise, unlike primitive data types, strings are objects of the String class, which is part of java.lang package. This class provides a rich set of methods for manipulating and processing textual data.
- A string is essentially an array of characters, i.e., a sequence of characters, which may include letters, digits, symbols, and whitespace.
- Strings are enclosed within double quotes (" "). For example, the phrase "Hello, Unstop!" is a string literal.
- Note that the String keyword in the declaration of the string variable must be in uppercase since Java is a case-sensitive language.
- Once created, the content of a string object cannot be changed, meaning they are immutable. Any operation that modifies a string actually creates a new string object.
- The strings non-primitive data type in Java are widely used for handling and manipulating textual data, including user input, file processing, and data exchange.
Understanding the string data type is essential for Java developers to handle and manipulate textual data with ease, leveraging the extensive functionality provided by the String class.
Syntax:
string message = "character array";
Here, the string keyword indicates that the variable given by the name message is a character string type variable. And the value inside double quotes is refers to the string value stored in the variable.
Code Example:
cHVibGljIGNsYXNzIFN0cmluZ0V4YW1wbGUgewpwdWJsaWMgc3RhdGljIHZvaWQgbWFpbihTdHJpbmdbXSBhcmdzKSB7CgpTdHJpbmcgZ3JlZXRpbmcgPSAiSGVsbG8sIFdvcmxkISI7ClN5c3RlbS5vdXQucHJpbnRsbihncmVldGluZyk7Cn0KfQ==
Output:
Hello, World!
Explanation:
- We begin by defining a public class called StringExample followed by the main method.
- Inside main, we declare a String variable named greeting and initialize it with the value "Hello, World!".
- Then, we use the System.out.println() statement to print the value of the greeting variable.
Array Non-Primitive Data Type In Java
Arrays are non-primitive data types in Java that allow you to store multiple values of the same type in a single variable. This means, that the values stored inside an array must be of homogenous type.
- These non-primitive types also have a fixed size, i.e., once created, its size cannot be changed.
- They are ordered collection of elements where we use the index values to access the elements. Indices start from 0, i.e., the first element has an index of 0.
- We use the square brackets for index notation [], as a part of array declaration and also when we want to access its elements.
- They are considered non-primitive data types because they are objects that can dynamically allocate memory for storing elements.
- They are extremely useful for storing and manipulating multiple values of the same type
Basic Array Syntax:
data_type[] array_name = {value1, value2, value3, value4};
Here,
- The terms data_type and array_name refer to the data type of the values stored inside the array and its name, respectively.
- Square brackets [] indicate that it is an array. Unlike the syntax for array declaration in other languages like C/ C++, we do not put the size of the array inside these brackets.
- The value1, value2,... inside the curly braces {} refer to the values stored inside the array.
Code Example:
cHVibGljIGNsYXNzIEFycmF5RXhhbXBsZSB7CnB1YmxpYyBzdGF0aWMgdm9pZCBtYWluKFN0cmluZ1tdIGFyZ3MpIHsKCmludFtdIG51bWJlcnMgPSB7MSwgMiwgMywgNCwgNX07Cgpmb3IgKGludCBpID0gMDsgaSA8IG51bWJlcnMubGVuZ3RoOyBpKyspIHsKU3lzdGVtLm91dC5wcmludGxuKCJFbGVtZW50IGF0IGluZGV4ICIgKyBpICsgIjogIiArIG51bWJlcnNbaV0pOwp9Cn0KfQ==
Output:
Element at index 0: 1
Element at index 1: 2
Element at index 2: 3
Element at index 3: 4
Element at index 4: 5
Explanation:
In the sample Java code-
- We begin by defining a class named ArrayExample, which contains the main method.
- Inside the main, we declare an array of integers called numbers and initialize it with five integer values {1, 2, 3, 4, 5}.
- Then, we initiate a for loop to iterate over the elements of the array using the index variable i. Note that arrays are indexed collections of elements, starting from index 0.
- In every iteration, the loop prints the element with the respective index position along with a descriptive message using the System.out.println() statement.
- As seen in the console, the output displays each element of the array along with its corresponding index, ranging from index 0 to index 4.
Interface Non-Primitive Data Types In Java
Interfaces are a critical component of the type system, classified as non-primitive data types in Java. They define a contract of methods that a class must implement and are essential for achieving abstraction and enabling multiple inheritance, allowing developers to design flexible and modular applications.
- An interface in Java is a reference type, similar to a class, but it can contain only method signatures and constants. That is, they are used to define methods that other classes must implement.
- Starting from Java 8, interfaces can also include default and static methods with implementations.
- By using interfaces, Java provides a way to ensure that different classes adhere to the same method specifications, facilitating polymorphism and code reusability.
- They contain abstract method declarations without implementations. Interfaces can declare constants, which are implicitly public, static, and final.
Interface non-primitive data types in Java are like blueprints or contracts for classes. These define a set of methods that a class must implement when it wants to use the interface.
Syntax:
access_modifier interface interfaceName {
// methods
//default methods
}
Here,
- The access_modifier refers to the visibility of the interface given by the name interfaceName.
- The interface term is the keyword for definition/ declaration and the curly braces contain the methods for implementation.
Code Example:
Ly8gRGVmaW5lIGFuIGludGVyZmFjZQppbnRlcmZhY2UgRHJhd2FibGUgewp2b2lkIGRyYXcoKTsKfQoKLy8gSW1wbGVtZW50IHRoZSBpbnRlcmZhY2UgaW4gYSBjbGFzcwpjbGFzcyBDaXJjbGUgaW1wbGVtZW50cyBEcmF3YWJsZSB7CnB1YmxpYyB2b2lkIGRyYXcoKSB7ClN5c3RlbS5vdXQucHJpbnRsbigiRHJhd2luZyBhIGNpcmNsZSIpOwp9Cn0KCnB1YmxpYyBjbGFzcyBJbnRlcmZhY2VFeGFtcGxlIHsKcHVibGljIHN0YXRpYyB2b2lkIG1haW4oU3RyaW5nW10gYXJncykgewovLyBDcmVhdGUgYW4gb2JqZWN0IG9mIHRoZSBjbGFzcyBpbXBsZW1lbnRpbmcgdGhlIGludGVyZmFjZQpDaXJjbGUgY2lyY2xlID0gbmV3IENpcmNsZSgpOwovLyBDYWxsIHRoZSBtZXRob2QgZGVmaW5lZCBpbiB0aGUgaW50ZXJmYWNlCmNpcmNsZS5kcmF3KCk7Cn0KfQ==
Output:
Drawing a circle
Explanation:
In the Java code sample-
- We declare a Drawable interface using the interface keyword. It contains an abstract method called draw() without a body.
- Any class that implements this interface must provide an implementation for the draw method.
- Next, we create a class Circle using the class and implement keywords. Here, the implements keyword indicates that the class will provide an implementation for the Drawable interface.
- Inside the class, the draw() method uses the System.out.println() statement to display a message- "Drawing a circle" to the console.
- After that, we define the main method, which is the entry point of the program.
- Inside main, we create an instance of the Circle class called circle using the new keyword.
- Then, we call the draw() method on the circle object using the dot operator (.). This call executes the implementation provided in the Circle class, printing "Drawing a circle" to the console.
Enumeration (Enum) Data Type In Java
Enumerations, commonly known as Enums, are a special type of non-primitive data type in Java used to define collections of constants. They allow you to create a set of named constants that represent possible values.
- In other words, Enums provide a way to create a group of named values, which are known as enumeration constants.
- They are used to represent fixed sets of related items, such as days of the week, directions, or states in a program.
- Enums in Java are more powerful than their counterparts in other programming languages, offering features like type safety, methods, and the ability to implement interfaces.
- By using Enums, developers can write more readable and maintainable code, reducing the risk of errors associated with using literal constants.
Syntax:
public enum EnumName {
Constant1, // Enum constants
Constant2,
Constant3;
}
Here,
- Keywords public and enum refer to the visibility mode and the data type enumerations given by the name EnumName.
- The values inside the curly braces, separated by commas (ending a semicolon), i.e., Constant1, Constant2, and so on, refer to the constant values stored inside the enum type.
Code Example:
Ly8gRGVmaW5lIGFuIGVudW0KZW51bSBEYXkgewpNT05EQVksIFRVRVNEQVksIFdFRE5FU0RBWSwgVEhVUlNEQVksIEZSSURBWSwgU0FUVVJEQVksIFNVTkRBWQp9CgpwdWJsaWMgY2xhc3MgRW51bUV4YW1wbGUgewpwdWJsaWMgc3RhdGljIHZvaWQgbWFpbihTdHJpbmdbXSBhcmdzKSB7CgovLyBVc2UgdGhlIGVudW0gY29uc3RhbnRzCkRheSB0b2RheSA9IERheS5TQVRVUkRBWTsKU3lzdGVtLm91dC5wcmludGxuKCJUb2RheSBpcyAiICsgdG9kYXkpOwp9Cn0=
Output:
Today is SATURDAY
Explanation:
In this Java example-
- We first define an enum called Day containing constants MONDAY, TUESDAY, WEDNESDAY, THURSDAY, FRIDAY, SATURDAY, and SUNDAY.
- Then, we define a class named EnumExample, which contains the main method.
- Inside main, we create an enum variable called today of type Day and initialize it the value Saturday.
- In other words, the Day enum is used to declare a variable today, which is assigned one of the enum constants.
- Then, we use the System.out.println() statement to display the value of the today variable along with a descriptive message.
- Note that, when printing an enum constant, Java automatically calls the toString() method, which returns the name of the constant.
Classes And Objects Non-Primitive Data Types In Java
The classes and objects are a fundamental part of object-oriented programming (OOP) and are categorized as non-primitive data types in Java. They provide a blueprint for creating complex data types that encapsulate both data and behavior, allowing for modular and reusable code.
- A class in Java is a blueprint for creating objects.
- It defines a set of properties (fields/ attributes) and methods (functions/ behaviors) that the objects created from the class can have.
- Classes allow you to model real-world entities and define their characteristics and behaviors.
- An object is an instance of a class that realizes the class with actual values assigned to its fields.
- Objects interact with each other through their methods and can represent real-world entities or abstract concepts.
Syntax For Class Declaration: | Syntax For Object Creation: |
class ClassName { // Defining the class // Define attributes // Define methods |
// Create an object of the class // Access attributes & methods of the object objectName.methodName(); // Call method
|
Let's look at an example Java program illustrating the concept of these non-primitive data types.
Code Example:
Y2xhc3MgQ2FyIHsKU3RyaW5nIGNvbG9yOwoKdm9pZCBkcml2ZSgpIHsKU3lzdGVtLm91dC5wcmludGxuKCJDYXIgaXMgZHJpdmluZy4uLiIpO30KfQoKcHVibGljIGNsYXNzIE1haW4gewpwdWJsaWMgc3RhdGljIHZvaWQgbWFpbihTdHJpbmdbXSBhcmdzKSB7CkNhciBteUNhciA9IG5ldyBDYXIoKTsKbXlDYXIuY29sb3IgPSAiUmVkIjsKbXlDYXIuZHJpdmUoKTsKfQp9
Output:
Car is driving...
Explanation:
In the Java example code-
- We define a class named Car using the class keyword. Inside the class-
- We have a field (or member variable/ instance variable) color of String type that holds the color of the car. This is a non-static variable and will be different for each object of the class.
- A void method drive() which prints the string message- "Car is driving..." to the console using System.out.println() statement. It represents the behavior of the Car class.
- Then, we define another class Main containing the main method which serves as the entry point of the program.
- Inside the main method, we create a new instance/ object of the Car class referred to by the variable myCar.
- Next, we use the dot operator (.) to access and assign value Red to the field color of the object myCar, i.e., myCar.color = "Red".
- We then call the drive() method on myCar object, i.e., myCar.drive(), which executes the code inside the method and prints "Car is driving..." to the console.
Key Differences Between Primitive And Non-Primitive Data Types In Java
All the data types in Java are categorized into two primary groups: primitive and non-primitive data types. Understanding the differences between them is crucial for effective programming and efficient memory management.
- Primitive data types in Java represent simple values and are directly stored in memory. They include fundamental types such as integers, booleans, and characters.
- Non-primitive data types, also known as reference types, are more complex structures like arrays, strings, and objects. These types store references to their data rather than the actual data itself.
The table below highlights the key distinctions between non-primitive and primitive data types in Java.
Category |
Primitive Data Types |
Non-Primitive Data Types |
Definition |
Basic data types directly stored in memory |
Complex data types that store references to objects |
Memory Allocation |
Memory allocated on the stack |
Memory allocated on the heap |
Size |
Fixed-size |
Variable size |
Mutability |
Primitive data types in Java are immutable, meaning their values cannot be changed once assigned. |
Non-primitive data types are generally mutable, allowing modification of their state. For example, you can modify the contents of a string or the elements of an array. |
Default Values |
Have default values (e.g., int defaults to 0, boolean defaults to false) |
Non-primitive data types default to null if not explicitly initialized. |
Operations |
Support basic operations such as arithmetic operations (for numeric types), logical, and bitwise operations. |
Support a wide range of operations depending on their type (e.g., concatenation for strings, manipulation for arrays) |
Memory Usage |
Generally require less memory compared to non-primitive types. |
Often require more memory due to their dynamic nature and the overhead of storing references. |
Equality |
Primitive data types compare values directly using their content. |
Compare references by default, , meaning they check whether two variables refer to the same object in memory. It requires custom methods or utilities for comparing contents |
Examples |
int, float, char, boolean |
String, Arrays, Classes, Interfaces |
The table highlights the primary differences between non-primitive and primitive data types in Java. Now, let's look at an example providing a comparative view of these types.
Code Example:
cHVibGljIGNsYXNzIERhdGFUeXBlQ29tcGFyaXNvbiB7CnB1YmxpYyBzdGF0aWMgdm9pZCBtYWluKFN0cmluZ1tdIGFyZ3MpIHsKCi8vIFByaW1pdGl2ZSBEYXRhIFR5cGVzCmludCBwcmltaXRpdmVOdW1iZXIgPSAxMDsKY2hhciBwcmltaXRpdmVDaGFyID0gJ0EnOwoKLy8gTm9uLVByaW1pdGl2ZSBEYXRhIFR5cGVzClN0cmluZyBub25QcmltaXRpdmVTdHJpbmcgPSAiSGVsbG8sIFdvcmxkISI7CmludFtdIG5vblByaW1pdGl2ZUFycmF5ID0gezEsIDIsIDMsIDQsIDV9OwoKLy8gT3V0cHV0ClN5c3RlbS5vdXQucHJpbnRsbigiUHJpbWl0aXZlIE51bWJlcjogIiArIHByaW1pdGl2ZU51bWJlcik7ClN5c3RlbS5vdXQucHJpbnRsbigiUHJpbWl0aXZlIENoYXJhY3RlcjogIiArIHByaW1pdGl2ZUNoYXIpOwpTeXN0ZW0ub3V0LnByaW50bG4oIk5vbi1QcmltaXRpdmUgU3RyaW5nOiAiICsgbm9uUHJpbWl0aXZlU3RyaW5nKTsKU3lzdGVtLm91dC5wcmludCgiTm9uLVByaW1pdGl2ZSBBcnJheTogIik7CmZvciAoaW50IGkgPSAwOyBpIDwgbm9uUHJpbWl0aXZlQXJyYXkubGVuZ3RoOyBpKyspIHsKU3lzdGVtLm91dC5wcmludChub25QcmltaXRpdmVBcnJheVtpXSArICIgIik7Cn0KfQp9
Output:
Primitive Number: 10
Primitive Character: A
Non-Primitive String: Hello, World!
Non-Primitive Array: 1 2 3 4 5
Explanation:
We begin by creating a class DataTypeComparison to show the comparison between the different data types in Java.
- Inside the class, we have the main method, where we declare two primitive data type variables and initialize them as follows:
- First we declare a simple integer type variable called primitiveNumber and assign a value 10 to it. This represents a simple numeric value stored directly in memory.
- Second, we declare and initialize a char type variable primitiveChar with the value 'A'. It represents a single character stored directly in memory.
- After that, we declare two non-primitive type variables and initialize them as follows:
- First we have a string type variable called nonPrimitiveString initialized with the value "Hello, World!".
- Note that a String is an object that holds a sequence of characters and is stored on the heap, with the variable nonPrimitiveString holding a reference to this object.
- Then, we have a non-primitive array of integers nonPrimitiveArray initialized with five integer values {1, 2, 3, 4, 5}.
- Note that an array is an object that holds multiple integer values stored on the heap, with nonPrimitiveArray holding a reference to the array object.
- Next, we use a set of System.out.println() statements to directly print the value of the two primitive variable and the non-primitive string.
- When printing the non-primitive array type, we use a for loop with a System.out.println() statement to iterate over each element of the array before printing.
- Upon execution, the program displays the values of these variables, distinguishing between primitive and non-primitive data types effectively.
Conclusion
In this article, we explored the fundamental concepts of data types in Java, which are essential for defining the nature of variables and memory they will occupy. They are classified into two main types, i.e., primitive and non-primitive data types.
- Primitive data types in Java, such as int and char, are the basic building blocks directly storing values and occupying fixed amounts of memory.
- On the other hand, non-primitive types, including String, arrays, and classes, store references to objects and offer greater flexibility and complexity.
- The key differences between the non-primitive and primitive data types in Java include memory allocation, mutability, and default values.
Mastering these concepts is crucial for writing efficient and effective code in Java. Understanding how to utilize both primitive and non-primitive data types allows you to make informed decisions about memory management and program design, leading to more optimized and robust solutions.
Also read: Top 100+ Java Interview Questions And Answers (2024)
Frequently Asked Questions
Q. How does Java manage character and boolean data types?
The character and boolean types as primitive data types in Java.
- Character Data Type (char): This type represents single characters, such as letters, digits, and symbols. Characters are stored as 16-bit Unicode characters, allowing Java to support a wide range of international characters. For example: char letter = 'A';
- Boolean Data Type (boolean): This type is used to store one of two possible values: true or false. They are commonly used for decision-making and control flow in programs. For example: Boolean isJavaFun = true;
Both char and boolean are primitive data types and are managed directly by the Java Virtual Machine (JVM), which handles their storage and operations efficiently.
Q. What are primitive value and non-primitive data types in Java?
Primitive Data Types: These types are building blocks of data, i.e., basic data types built into Java, including int, float, char, and boolean. They represent simple values like numbers and characters and are stored directly in memory.
Non-Primitive Data Types: These types, also known as reference types, are more complex and can represent objects rather than just values. Meaning, they store references to objects or data structures and offer more complex functionality compared to primitive types. They include classes, interfaces, arrays, and enums. Unlike primitive data types, non-primitive data types are mutable, allowing for dynamic changes to their state.
Q. Why is String a non-primitive data type in Java?
A String is considered a non-primitive data type in Java because it is an object that holds a sequence/ array of characters as against a simple value like primitive types. Here are two more characteristics of the string type that make it a non-primitive data type in java:
- Dynamic Nature: They are dynamic, meaning their length and contents can change at runtime. This dynamic behavior aligns with the characteristics of non-primitive data types, which can be mutable and flexible in handling complex data.
- Object-oriented Design: They are instances of the built-in String class, which provides various methods for manipulating and working with strings. Being an object, strings inherit properties and behaviors from this class, making them more versatile and powerful than simple primitive values.
For example:
String greeting = "Hello";
greeting = greeting + ", World!";
In this example, the string greeting is concatenated with the string- ", World!" to form the complete greeting "Hello, World!". This dynamic modification of the string illustrates its non-primitive nature and object-oriented characteristics.
Q. What are the default values of primitive data types in Java?
The default values of primitive data types in Java depend on their type:
- Int/ Numeric Types: The default value for numeric types (byte, short, int, long, float, double) is 0.
- Boolean Type: The default value for the boolean type (boolean) is false.
- Character Type: The default value for the character type (char) is the null character '\u0000'.
Example:
cHVibGljIGNsYXNzIERlZmF1bHRWYWx1ZUV4YW1wbGUgewoKLy8gSW5zdGFuY2UgdmFyaWFibGVzIHRvIGRlbW9uc3RyYXRlIGRlZmF1bHQgdmFsdWVzCmludCBkZWZhdWx0VmFsdWVGb3JJbnQ7CmJvb2xlYW4gZGVmYXVsdFZhbHVlRm9yQm9vbGVhbjsKY2hhciBkZWZhdWx0VmFsdWVGb3JDaGFyOwoKcHVibGljIHN0YXRpYyB2b2lkIG1haW4oU3RyaW5nW10gYXJncykgewovLyBDcmVhdGUgYW4gaW5zdGFuY2Ugb2YgdGhlIGNsYXNzIHRvIGFjY2VzcyBpbnN0YW5jZSB2YXJpYWJsZXMKRGVmYXVsdFZhbHVlRXhhbXBsZSBleGFtcGxlID0gbmV3IERlZmF1bHRWYWx1ZUV4YW1wbGUoKTsKCi8vIERlZmF1bHQgdmFsdWVzClN5c3RlbS5vdXQucHJpbnRsbigiRGVmYXVsdCB2YWx1ZSBmb3IgaW50OiAiICsgZXhhbXBsZS5kZWZhdWx0VmFsdWVGb3JJbnQpOwpTeXN0ZW0ub3V0LnByaW50bG4oIkRlZmF1bHQgdmFsdWUgZm9yIGJvb2xlYW46ICIgKyBleGFtcGxlLmRlZmF1bHRWYWx1ZUZvckJvb2xlYW4pOwpTeXN0ZW0ub3V0LnByaW50bG4oIkRlZmF1bHQgdmFsdWUgZm9yIGNoYXI6IFsiICsgZXhhbXBsZS5kZWZhdWx0VmFsdWVGb3JDaGFyICsgIl0iKTsKfQp9
Output:
Default value for int: 0
Default value for boolean: false
Default value for char: [ ]
Explanation:
- We first define a class DefaultValueExample to demonstrate default values for primitive data types in Java.
- Then, we declare three instance variables of different data types: defaultValueForInt (int type), defaultValueForBoolean (bool type), and defaultValueForChar (char type).
- Note that we have not initialized these variables, so they will automatically be initialized with default values.
- Next, we define the main method, which is the program's entry point of execution.
- Inside main, we create an instance of the DefaultValueExample class called example using new keyword. This allows us to access the instance variables of the class.
- After that, we use the System.out.println() statement to print the values of the instance variables (which will be default values).
- As seen in the output window, the default values of the instance variables are 0 for int, false for boolean, and an empty space representing the null character for char.
Q. Why class is a non-primitive data type in Java
A class is a non-primitive data type in Java because it defines a template/ blueprint for creating objects.
- Objects are instances of classes that are created at runtime and can have states and behaviour associated with them.
- Unlike primitive data types, which store simple values directly, classes define the structure, behaviour, and attributes of objects.
- Classes are used to encapsulate data and methods into a single unit and are stored on the heap.
Q. What is a primitive data type in Java (in simple words)?
In simple words, a primitive data type in Java is a basic type of data that holds simple values directly, such as numbers or characters.
- These types are the building blocks of data in Java and are managed directly by the Java Virtual Machine (JVM).
- Imagine you are building a LEGO castle, and each type of LEGO brick represents a different primitive data type in Java. Just like each brick has a specific size and shape, each primitive data type has a specific size and type of value it can hold.
The types integer for while numbers (short, long, byte, etc), float type for floating-point values (float and double), char type (for single characters), and boolean type (for true or false values), are subtypes of the primitive data types in Java.
Here are a few other articles you must read:
An economics graduate with a passion for storytelling, I thrive on crafting content that blends creativity with technical insight. At Unstop, I create in-depth, SEO-driven content that simplifies complex tech topics and covers a wide array of subjects, all designed to inform, engage, and inspire our readers. My goal is to empower others to truly #BeUnstoppable through content that resonates. When I’m not writing, you’ll find me immersed in art, food, or lost in a good book—constantly drawing inspiration from the world around me.
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
Comments
Add comment