Operators In Java | 9 Types, Precedence & More (+ Code Examples)
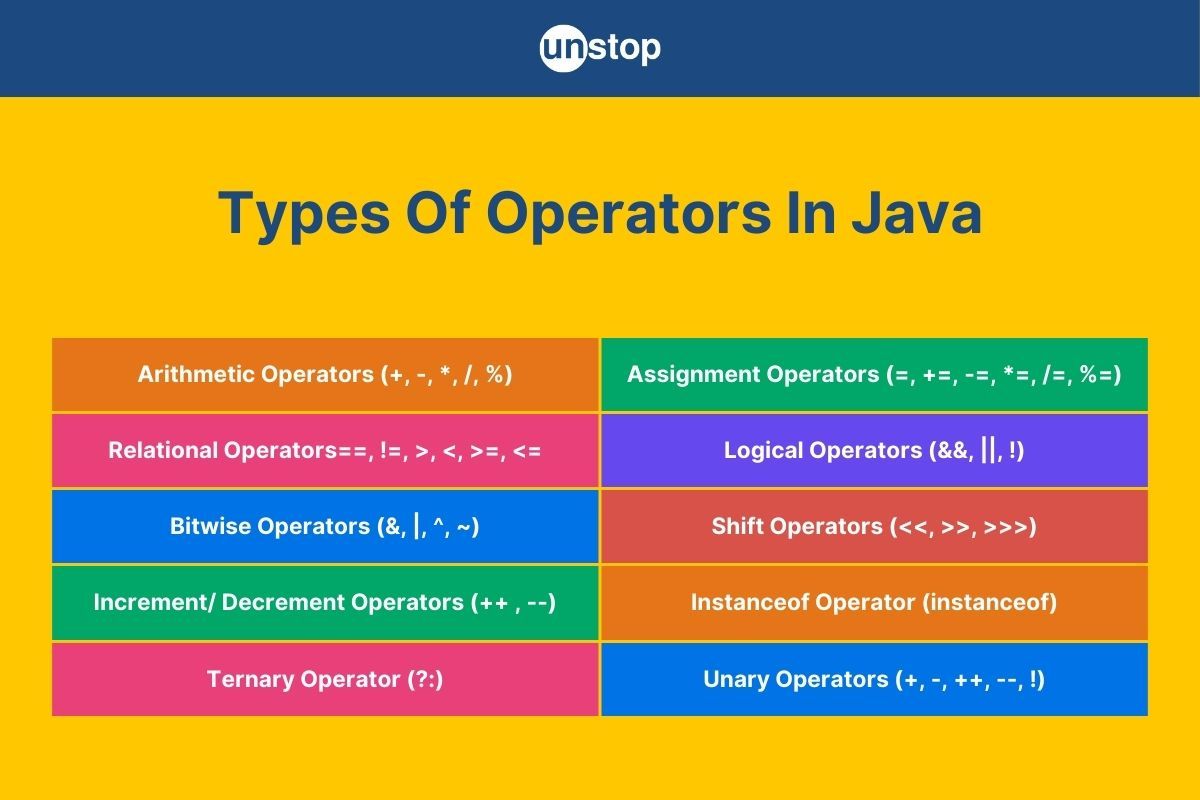
Operators are the building blocks that make your code dynamic and functional in all programming languages. Whether you're performing arithmetic operations, making logical comparisons, or controlling program flow, operators are essential to manipulating data and variables effectively.
In this article, we will discuss all the types of operators in Java and how to work with them with the help of detailed code examples. You’ll see how these operators help streamline coding tasks, improve program efficiency, and ensure your code works as expected. Let’s dive in and explore the different operator categories in Java with clear examples and real-world use cases.
What Are Operators In Java?
Java operators are symbols or keywords that perform specific operations on one or more operands and return a result. They allow you to manipulate variables, make calculations, compare values, and control the flow of your program. Operators are crucial for writing efficient and effective Java code, as they serve as the tools you use to interact with the data in your application.
For example:
int a = 5;
int b = 10;
int sum = a + b; // sum is 15
boolean result = a > b; // result is false
Here, the addition arithmetic operator (+) adds two values together. And the greater than relational operator (>) compares two values to check if one is greater than the other.
Importance of Operators in Java
Operators are crucial as they allow developers to manipulate data, control program flow, and perform calculations—all of which are essential for building functional and efficient applications. Let’s look at a few key reasons why we need operators in Java programming language:
- Efficient Data Manipulation: Operators enable easy manipulation of variables and values, allowing developers to perform arithmetic operations, assign values, and compare data in a concise and readable manner.
- Control Flow Management: Operators such as logical (&&, ||, !) and relational (>, <, ==) are key to controlling the flow of a program. They help in making decisions, repeating tasks, and ensuring that code executes correctly based on specific conditions.
- Optimization and Performance: Operators like bitwise operators allow for low-level operations on data, which can be critical for optimizing memory usage and performance, especially in systems where resources are limited.
- Code Readability and Simplicity: Using operators makes code more compact and expressive. Instead of writing long, complex statements, developers can use simple operators to perform tasks like checking conditions or performing calculations, making the code more readable and maintainable.
Operators in Java are, hence, indispensable for performing core functions within any application. They help streamline tasks, improve code efficiency, and ensure the smooth execution of the program. In the next section, we will look at the various types of operators in Java programming.
Types Of Operators In Java
Java operators are categorized based on their functionality, and understanding these categories is essential for mastering the language. The table below provides an overview of the main types of operators in Java language.
Operator Type |
Symbol/ Syntax |
Description |
Unary Operators |
+, -, ++, --, ! |
Work with a single operand to perform operations. |
Arithmetic Operators |
+, -, *, /, % |
Perform basic arithmetic/mathematical operations. |
Assignment Operators |
=, +=, -=, *=, /=, %=, &= |
Used to assign values to variables. |
Relational Operators |
==, !=, >, <, >=, <= |
Compare two values and return a boolean result (True or False) |
Logical Operators |
&&, ||, ! |
Combine boolean expressions to form complex conditions. |
Ternary Operator |
? : |
A shorthand for if-else statements. |
Bitwise Operators |
&, |, ^, ~ |
Perform operations on bits and bit values. |
Shift Operators |
<<, >>, >>> |
Shift bits of a value to the left or right, by a sepcified number. |
Increment/Decrement Operators |
++ , -- |
Increase or decrease a variable's value by 1. |
Instanceof Operator |
object instanceof ClassName |
Tests whether an object is an instance of a specified class. |
Now that you know about the different types of operators in Java, let’s discuss each of these types in detail with the help of code examples.
Unary Operators In Java
Unary operators are those that operate on a single operand to alter its value or state. For example, the logical complement operator (!) inverts a boolean value, while the unary minus (-) changes the sign of a number. These Java operators are applied directly to the operand, streamlining operations like sign reversal and logical inversion. The table below summarizes unary operators in Java.
Operator |
Symbol |
Description |
Unary Plus |
+ |
Indicates a positive value (usually redundant). |
Unary Minus |
- |
Negates an expression. |
Increment |
++ |
Increases a value by one. |
Decrement |
-- |
Decreases a value by one. |
Logical Complement |
! |
Inverts the value of a boolean |
Note: Increment (++) and Decrement (--) operators are part of unary operators but will be explained in detail in a separate section.
Below is a basic Java program example illustrating the use of these operators.
Code Example:
Output:
+x = 10
-x = -10
!flag = false
Explanation:
In the basic Java code example,
- We first declare an integer variable x and a boolean variable flag, initializing them with the values 10 and true.
- Next, we use the System.out.println() method to display the results of the unary operations applied to these variables.
- Unary plus operator (+x) explicitly denotes a positive value but does not change the value of x, resulting in 10.
- The unary minus operator (-x) negates the value of x, changing 10 to -10.
- Finally, the logical complement operator (!flag) inverts the boolean value of flag, turning true to false.
Important Note: While unary operators work on a single operand, most other operators in Java are binary, meaning they require two operands to perform operations like arithmetic, comparison, or logical combinations.
Arithmetic Operators In Java
Arithmetic operators, as the name suggests, are used for performing basic mathematical operations like addition, subtraction, multiplication, division, and modulus (remainder). In shot, these Java operators are essential for handling numerical data. They are widely used in a variety of applications, ranging from simple arithmetic computations/ mathematical manipulations to formulating complex algorithms, and more.
In the table below, we have listed all the arithmetic operators in Java, along with the respective symbols and a short description.
Types Of Arithmetic Operators In Java
Operator Name |
Symbol |
Description |
Example (int a = 10; int b = 5) |
Addition |
+ |
Adds two operands. |
int sum = a + b; // 15 |
Subtraction |
- |
Subtracts the second operand from the first. |
int difference = a - b; // 5 |
Multiplication |
* |
Multiplies two operands. |
int product = a * b; // 50 |
Division |
/ |
Divides the numerator by the denominator. |
int quotient = a / b; // 2 |
Modulus |
% |
Returns the remainder of a division operation. |
int remainder = a % b; // 0 |
Now that you are familiar with the types of arithmetic operators in Java, look at the example below. The simple Java program example illustrates how to use these operators in action.
Code Example:
Output:
a + b = 15
a - b = 5
a * b = 50
a / b = 2
a % b = 0
Explanation:
In the simple Java code example,
- We first create the Main class and define the main() method, which serves as the program’s entry point.
- Inside main(), we declare two integer variables, a and b, and initialize them with the values 10 and 5, respectively.
- Next, we use the arithmetic operators (+, -, *, /, and %) to perform various calculations.
- We print the outcome of each operation to the console using the System.out.println() method. Here, we combine a descriptive string message with the result using the string concatenation operator (+), which has the same symbol as the addition arithmetic operator.
- The operations are as follows:
- The addition operator (+) computes the sum of a and b, resulting in 15.
- The subtraction operator (-) calculates their difference, which is 5.
- The multiplication operator (*) gives the product 50.
- The division operator (/) performs integer division, returning 2.
- Finally, the modulus operator (%) calculates the remainder when a is divided by b, which is 0.
This example demonstrates the basic usage of arithmetic operators in Java, showcasing how they enable simple mathematical computations within a Java program.
Want to expand your knowledge of Java programming? Check out this amazing course and become the Java developer of your dreams.
Assignment Operators In Java
Assignment operators are vital for assigning and updating variable values in Java. These can further be classified into two types.
- The basic assignment operator, denoted by a single equals sign (=), assigns a value to a variable.
- The combined or compound assignment operators (like +=, -=, etc.) perform arithmetic operations and assignments in one step, simplifying code and improving efficiency.
The table below provides an overview of these Java operators, along with the respective symbols and descriptions.
Types Of Assignment Operators In Java
Operator |
Syntax |
Description |
Assignment |
= |
Assigns the value of the right operand to the left operand. |
Addition Assignment |
+= |
Adds the right operand to the left operand, then assigns the result (sum) to the left operand. |
Subtraction Assignment |
-= |
Subtracts the right operand from the left operand, then assigns the result (difference) to the left operand. |
Multiplication Assignment |
*= |
Multiplies the left operand by the right operand and then assigns the result (product) to the left operand. |
Division Assignment |
/= |
Divides the left operand by the right operand and then assigns the result (quotient) to the left operand. |
Modulus Assignment |
%= |
Computes the remainder of dividing the left operand by the right operand and then assigns the result (remainder) to the left operand. |
Below is an example illustrating the use of the Java assignment operators listed above.
Code Example:
Output:
a += b: 15
a -= b: 10
a *= b: 50
a /= b: 10
a %= b: 0
Explanation:
In the example,
- We start by declaring and initializing two integer variables, a and b. Here, we use the simple assignment operator to assign the values 10 and 5, respectively.
- Then, we use the compound assignment operators to update the value of the left-hand operand, here a, in place, as follows:
- Addition assignment operator (a += b) adds b to a and assigns the result to a, updating it to 15.
- Subtraction assignment operator (a -= b) subtracts b from a and assigns the result to a, reverting it to 10.
- Multiplication assignment operator (a *= b) multiplies a by b and assigns the result to a, increasing it to 50.
- Division assignment operator (a /= b) divides a by b and assigns the result to a, bringing it back to 10.
- Modulus assignment operator (a %= b) computes the remainder of a divided by b and assigns it to a, reducing it to 0.
- In each operation, we concatenate the descriptive message and the result using the string concatenation operator (+) before printing it with System.out.println().
Relational Operators In Java
As the name suggests, the relational operators compare two values to determine the relationship between them based on the purpose of the respective operator type. The result of this comparison is a boolean value: true if the relationship holds and false otherwise. These operators are often used in decision-making and to control program flow based on conditions. Look at the table below to understand the purpose of different relational operators.
Operator |
Syntax |
Description |
Equal to |
== |
Compares two values for equality. |
Not Equal to |
!= |
Compares two values for inequality. |
Greater than |
> |
Checks if the left operand is greater than the right operand. |
Less than |
< |
Checks if the left operand is less than the right operand. |
Greater than or Equal to |
>= |
Checks if the left operand is greater than or equal to the right operand. |
Less than or Equal to |
<= |
Checks if the left operand is less than or equal to the right operand. |
Code Example:
Output:
a == b: false
a != b: true
a > b: true
a < b: false
a >= b: true
a <= b: false
Explanation:
In the Java code example,
- We begin by declaring and initializing two integer variables, a and b, with values 10 and 5, respectively.
- Then, we use relational operators to evaluate comparisons/ relationships between these variables, returning true or false. Here’s how each operator works:
- Equality Check (a == b): Compares a and b for equality. Since 10 is not equal to 5, the result is false.
- Inequality Check (a != b): Checks if a and b are not equal. Since 10 is not equal to 5, the result is true.
- Greater Than (a > b): Verifies if a is greater than b. Since 10 is greater than 5, the result is true.
- Less Than (a < b): Checks if a is less than b. Since 10 is not less than 5, the result is false.
- Greater Than or Equal To (a >= b): Checks if a is greater than or equal to b. Since 10 is greater than 5, the result is true.
- Less Than or Equal To (a <= b): Verifies if a is less than or equal to b. Since 10 is not less than or equal to 5, the result is false.
- We display the outcome of each operation with descriptive text using the System.out.println() method.
Logical Operators In Java
Logical operators are used to combine or invert boolean values to perform logical operations. They evaluate expressions based on boolean logic and return a boolean result. These operators help construct complex conditions and control program flow based on multiple criteria. The table below lists the three types of logical operators with a short description of their functionality.
Operator |
Syntax |
Description |
AND |
&& |
Returns true if both operands are true. |
OR |
|| |
Returns true if either of the operands is true. |
NOT |
! |
Inverts the value of a boolean operand. |
Code Example:
Output:
a && b: false
a || b: true
!a: false
Explanation:
In the example Java code,
- We initialize two boolean variables, a and b, with the values true and false, respectively. Here, the boolean data type indicates that a and b will hold only boolean values (true or false).
- Next, we use logical operators to perform logical operations on the values of a and b.
- The logical AND operator (a && b) checks if both a and b are true. Since a is true and b is false, the result is false.
- The logical OR operator (a || b) checks if either a or b is true. Since a is true, the result is true.
- The logical NOT operator (!a) negates the value of a. Since a is true, the result is false.
- We print the outcome to the console using the System.out.println() method.
Bitwise Operators In Java
Bitwise operators allow you to manipulate data at the binary level, operating on individual bits of integer values. These operators are often used for tasks that require precise control over binary data, like optimization or hardware-level programming. Look at the table below to learn how to use the different bitwise operators.
Operator |
Syntax |
Description |
AND |
& |
Performs a bitwise AND operation, where the result is 1 only if both bits are 1. |
OR |
| |
Performs a bitwise OR operation, where it returns 1 if at least one of the corresponding bits is 1; otherwise, it returns 0. |
XOR |
^ |
Performs a bitwise XOR operation, where the result is 1 if exactly one bit is 1. |
Complement |
~ |
Inverts all bits of the operand. |
Code Example:
Output:
a & b: 1
a | b: 7
a ^ b: 6
~a: -6
Explanation:
In the example Java program,
- We declare two integer variables, a and b, initialized to 5 and 3, respectively. These integers are represented in binary as 0101 and 0011.
- Then, we use different bitwise operators to perform operations on the binary representations of a and b, returning an integer result for each.
- The bitwise AND operation (a & b) compares each corresponding bit of a and b. It returns 1 only if both bits are 1. In this case:
0101 (a)
0011 (b)
----
0001 (result)
- The bitwise OR operation (a | b) compares each corresponding bit of a and b. It returns 1 if at least one of the bits is 1. Here:
0101 (a)
0011 (b)
----
0111 (result)
- The bitwise XOR operation (a ^ b) compares each corresponding bit of a and b. It returns 1 if only one of the bits is 1. In this case:
0101 (a)
0011 (b)
----
0110 (result)
- The bitwise complement operation (~a) inverts all bits of a. The two's complement of a is calculated, resulting in -6. The result is printed as ~a: -6.
- We print the outcome of each of these operations to the console.
Here is your chance to top the leaderboard while practising your coding skills: Participate in the 100-Day Coding Sprint at Unstop.
Shift Operators In Java
Shift operators in Java are used to shift bits of an integer value to the left or right. These operations enable efficient bit-level manipulation and are commonly used in tasks like mathematical optimizations, binary data encoding, or in situations requiring direct control over bits. The shift operations are different from the bitwise operators as they specifically work by shifting bits in a specified direction rather than performing logical operations on them.
Types Of Shift Operators In Java
Operator |
Syntax |
Description |
Left Shift |
<< |
Shifts the bits of the left operand to the left by the number of positions specified by the right operand. Zeros are added to the right. |
Right Shift |
>> |
Shifts the bits of the left operand to the right by the number of positions specified by the right operand. The leftmost bits are filled with the sign bit (for signed integers). |
Unsigned Right Shift |
>>> |
Shifts the bits of the left operand to the right by the number of positions specified by the right operand. Zeros are added to the left, regardless of the sign. |
Code Example:
Output:
a << b: 32
a >> b: 2
a >>> b: 2
Explanation:
In the Java example code,
- Inside main(), we declare two integer variables, a and b and assign values 8 (1000 in binary), and 2 (0010 in binary), respectively.
- Next, we perform the shift operations on a using b to specify the number of positions to shift.
- The left shift operator shifts the bits of a (binary 1000) to the left by b (2) positions.
- When you shift 1000 two places to the left, you get 100000, which equals 32 in decimal.
- The left shift operation essentially multiplies the value by 2^b (in this case, 2^2 = 4). So, the result of a << b is 32.
- The right shift operator shifts the bits of a (binary 1000) to the right by b (2) positions.
- Shifting 1000 two places to the right results in 0010, which equals 2 in decimal.
- The right shift operation essentially divides the value by 2^b (in this case, 2^2 = 4). So, the result of a >> b is 2.
- The unsigned right shift works similarly to the regular right shift but with a key difference.
- That is, it fills the leftmost bits with 0 instead of replicating the sign bit.
- This is particularly useful for handling unsigned integers.
- In this case, since a = 8 and b = 2, shifting 1000 two places to the right gives 0010, which is 2 in decimal. The result of the unsigned right shift is also 2.
- We print the outcome of each of these bitwise operations to the console using the println() method.
Increment & Decrement Operators In Java
Increment and decrement operators in Java are used to increase or decrease the value of a variable by 1, respectively. These operators are widely used in loops and other control structures where the value of a variable needs to be updated frequently.
- Increment Operator (++) increases the value of a variable by 1.
- Decrement Operator (--) decreases the value of a variable by 1.
There are two variations of these Java operators: pre-increment/decrement and post-increment/decrement. The difference lies in when the value is modified in relation to the expression. For prefixed operators, the value is increased/ decreased before being evaluated in the expression, and for postfix, the value is increased/ decreased after being evaluated in the expression.
Types Of Increment & Decrement Operators In Java
Operator |
Syntax |
Description |
Pre-Increment |
++var |
Increments the value of the variable by 1 before it is used in the expression. |
Post-Increment |
var++ |
Increments the value of the variable by 1 after it is used in the expression. |
Pre-Decrement |
--var |
Decrements the value of the variable by 1 before it is used in the expression. |
Post-Decrement |
var-- |
Decrements the value of the variable by 1 after it is used in the expression. |
Code Example:
Output:
Pre-Increment: 6
Post-Increment: 6
Value of x after Post-Increment: 7
Pre-Decrement: 4
Post-Decrement: 4
Value of y after Post-Decrement: 3
Explanation:
In this example, we declare a variable, x and assign the value 5 to it.
- Then, we use the increment operators and its pre and post variations to perform operations on x.
- We first use the pre-increment operator (++x), which increases the value of x by 1 before it is used in the expression. So, x is incremented from 5 to 6, and the new value (6) is printed.
- Then, the post-increment operator (x++) increases the value of x by 1 after the current value is used in the expression. Initially, x is still 6 (from the previous operation), and its current value 6 is used in the output.
- After this, x is incremented to 7, and we print the updated value of x as “Value of x after Post-Increment”.
- Next, we declare another integer variable y and assign the value 5 to it.
- After that, we use the pre and post variations of the decrement operator to perform operations on y.
- We first use the pre-decrement operator (--y) which decreases the value of y by 1 before it is used in the expression. Here, y is first decremented from 5 to 4 and the new value (4) is printed as output.
- Then, we use the post-decrement operator (y--), which decreases the value of y by 1 after its current value is used in the expression. Initially, y is still 4 (from the previous operation), and its current value (4) is printed as Post-Decrement.
- After this, y is decremented to 3, and we print the updated value of y as “Value of y after Post-Decrement:”.
Ternary Operator In Java
The ternary operator offers a shorthand for conditional logic in Java. It evaluates a boolean expression and returns one of two values based on the result. This operator acts as a compact version of the if-else statement, making code more concise.
Operator |
Syntax |
Description |
Ternary |
? : |
Evaluates a boolean expression and returns one of two values based on the result. |
Code Example:
Output:
Max of a and b: 10
Explanation:
In the example Java program,
- We declare and initialize two integer variables, a and b, with the values 10 and 5, respectively.
- Next, we use the ternary operator to check if the value of a is greater than b, i.e., condition a > b.
- If the condition evaluates to true, the value before the colon (a) is assigned to the variable max.
- If the condition evaluates to false, the value after the colon (b) is assigned to max.
- In this case, since a is greater than b (i.e., 10 > 5), the condition is true, and a is assigned to max variable.
- Finally, we print the value of max to the console.
Instanceof Operator In Java
The instanceof operator checks if an object is an instance of a particular class or implements a specific interface.
- It returns true if the object is of the specified type or subclass, and false otherwise.
- This operator is particularly useful for type-checking, ensuring that objects conform to expected types before performing operations on them.
Syntax:
object instanceof ClassName
Here, the object is the operand being checked, and ClassName refers to the class or interface you want to verify the object against.
Code Example:
Output:
Is str an instance of String? true
Explanation:
In the sample Java code,
- We first declare a variable str and initialize it with the string value "Hello, world!".
- Then, we use the instanceof operator to check if str is an instance of the String class. This is done using the expression str instanceof String.
- We store the outcome in the boolean variable result since the outcome will be true or false.
- Finally, we print the result to the console, which confirms that str is indeed an instance of the String class.
Precedence & Associativity Of Java Operators
When working with multiple operators in a single expression, the precedence and associativity of the operators play a key role in determining how the expression will be evaluated as a whole.
- Operator precedence determines the order in which operators are evaluated in an expression. Operators with higher precedence are evaluated before those with lower precedence.
- On the other hand, associativity defines the direction in which operators of the same precedence level are evaluated within the same expression. Associativity can either be left-to-right (LTR) or right-to-left (RTL).
Let’s look at the rules governing these two aspects of operators before discussing where the operators are placed on the scale of precedence.
Precedence Rules:
- Operators with higher precedence are evaluated first.
- Parentheses () can be used to override the default precedence.
Associativity Rules:
- Left-to-right associativity means that operators are evaluated from left to right.
- Right-to-left associativity means that operators are evaluated from right to left.
Table Of Operator Precedence (Decreasing Order) With Associativity
Precedence Level |
Operator(s) |
Associativity |
Category |
1 |
[] . () :: |
Left-to-right |
Postfix, Member Access, Calls |
2 |
++ -- |
Right-to-left |
Unary Unary (Post-Increment/ Decrement) |
3 |
++ -- + - ~ ! |
Right-to-left |
Unary (Pre-Increment/Decrement, Negation) |
4 |
new |
Left-to-right |
Creation |
5 |
* / % |
Left-to-right |
Multiplicative |
6 |
+ - |
Left-to-right |
Additive |
7 |
<< >> >>> |
Left-to-right |
Shift |
8 |
< <= > >= instanceof |
Left-to-right |
Relational, Type-checking |
9 |
== != |
Left-to-right |
Equality |
10 |
& |
Left-to-right |
Bitwise AND |
11 |
^ |
Left-to-right |
Bitwise XOR |
12 |
&& |
Left-to-right |
Logical AND |
13 |
?: |
Right-to-left |
Ternary |
14 |
= += -= *= /= %= &= ^= <<= >>= >>>= |
Right-to-left |
Assignment, Compound Assignment |
15 |
, |
Left-to-right |
Comma (used to separate expressions) |
Need more guidance on how to become a Java developer? You can now select an expert to be your mentor here.
Advantages & Disadvantages Of Operators In Java
Java operators play a vital role in simplifying operations, but like all Java features, they come with their own set of advantages and disadvantages.
Advantages |
Disadvantages |
|
|
Conclusion
Understanding Java operators is essential for writing clear, effective code. Operators facilitate a wide range of operations, from basic arithmetic to complex bitwise manipulations. They play a key role in condition testing, decision-making, and data manipulation.
- The extensive set of operators in Java—arithmetic, unary, relational, logical, bitwise, and ternary—serves various purposes.
- However, it is important to be aware of their behavior, especially with regard to precedence and associativity.
- Ensuring correct evaluation order is critical for avoiding logical errors and unexpected results.
With a proper understanding of operator precedence and associativity, one can avoid common pitfalls and make the best use of the operators in Java.
Frequently Asked Questions
Q. What are the 7 Arithmetic Operators in Java?
The 7 types of arithmetic operators in Java are listed in the table below.
Operator |
Description |
+ (Addition) |
Adds two operands. |
- (Subtraction) |
Subtracts the second operand from the first. |
* (Multiplication) |
Multiplies two operands. |
/ (Division) |
Divides the numerator by the denominator. |
% (Modulus) |
Returns the remainder of the division. |
++ (Increment) |
Increases the value of a variable by 1. |
-- (Decrement) |
Decreases the value of a variable by 1. |
Q. What is the & Operator in Java?
The ampersand symbol (&) denotes the bitwise AND operator in Java. It performs a bitwise AND operation between two integer operands. That is,
- The result is 1 if both corresponding bits of the operands are 1
- Otherwise, the result is 0.
For Example:
int a = 5; // 0101 in binary
int b = 3; // 0011 in binary
int result = a & b; // 0001 in binary, which is 1 in decimal
Q. Which Java operator has only one operand?
The unary operators in Java operate on a single operand. They are listed in the table below with a short example.
Operator |
Description |
Example |
Unary Plus (+) |
Indicates a positive value (often redundant). |
int a = +5; |
Unary Minus (-) |
Negates the operand. |
int a = -5; |
Increment (++) |
Increases the operand’s value by 1. |
int a = 5; a++; |
Decrement (--) |
Decreases the operand’s value by 1. |
int a = 5; a--; |
Logical NOT (!) |
Inverts the boolean value of the operand. |
boolean flag = true; flag = !flag; |
Bitwise NOT (~) |
Inverts all bits of the operand. |
int a = 5; int b = ~a; // Result is -6 |
Q. What is the == Operator in Java?
The double equal to symbol (==) represents the equality relational operator in Java. It checks if two values or references are equal. It compares both the value and the memory address (for references). It is commonly used for both primitive data types and object references.
Code Example:
Output:
x and y are equal
Q. What are the three types of operands?
In programming, operands are data, values or variables on which operators perform operations. The table below lists the three types of operands:
Type of Operand |
Description |
Example |
Constants |
Fixed values that do not change during the execution of a program. They are directly used in expressions. |
5, 3.14, 'A', "Hello" |
Variables |
Named storage locations in memory that can hold different values during the execution of a program. They must be declared before use. |
int x = 5;, double pi = 3.14; |
Expressions |
Combinations of variables, constants, and operators that are evaluated to produce a result/ new value. These can be arithmetic, logical, or other types. |
a + b, x * y - 7, a > b |
Q. What is the relationship between data types and operators in Java?
Data types specify the type of value a variable can hold while operators perform operations on these values. The type of operand determines which operators can be used with it. For instance:
- Arithmetic operators can only be applied to numeric data types (int, float, etc.).
- Logical operators operate on boolean types.
- Bitwise operators work on integer types.
A mismatch between data types might require type conversion or can lead to compilation errors.
Q. Differentiate between operation, operator and operand?
Term |
Definition |
Example |
Operation |
A procedure that produces a result from one or more operands. |
5 + 3 (addition operation) |
Operator |
A symbol that tells the compiler or interpreter to perform a specific action/ operation on the operands. |
+ (addition operator) |
Operand |
The values or variables on which the operator acts/performs the operation. |
5 and 3 in the expression 5 + 3 |
This compiles our discussion on operators in Java. Do check out the following:
- Identifiers In Java | Types, Conventions, Errors & More (+Examples)
- Advantages And Disadvantages of Java Programming Language
- Top 15+ Difference Between C++ And Java Explained (+Similarities)
- Method Overriding In Java | Rules, Use-Cases & More (+Examples)
- Dynamic Method Dispatch In Java (Runtime Polymorphism) + Examples
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment