Java Programming Language
Table of content:
- History Of Java Programming Langauge
- Infographic For History Of Java
- What’s In The Name | History Of Java
- Key Features Of Java
- Advantages And Disadvantages Of Java
- The Version History Of Java Langauge
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is JDK?
- How To Download Java Development Kit (JDK) For Windows, MacOS, and Linux?
- Set Environment Variables In Java
- How To Install Java (JDK) On Windows 64-Bit Machine?
- How To Install Java (JDK) On Linux?
- How To Install Java (JDK) On macOS?
- How To Test Java Installation?
- How To Write Your First Java Program On Linux OS?
- Conclusion
- Frequently Asked Questions
Table of content:
- Java Programming Language | An Introduction
- 15 Key Features Of Java
- Write Once Run Anywhere (WORA) | Features Of Java
- Java Editions
- 5 New Features Of JAVA 8
- 5 New Features Of JAVA 11
- What Makes Java Popular?
- Conclusion
- Frequently Asked Questions
Table of content:
- What is Java?
- Advantages of Java
- Disadvantages of Java
Table of content:
- What Is Java Programming?
- Role Of Integrated Development Environments (IDEs) In Java Development
- 15 Best Java IDE For Developers
- In-Depth Comparison Table
- Conclusion
- Frequently Asked Questions
Table of content:
- Key Differences Between Java And Core Java
- What Is Java?
- What Is Core Java?
- Applications Of Java
- Applications Of Core Java
- When To Use Java?
- When To Use Core Java?
- Conclusion
- Frequently Asked Questions
Table of content:
- What Are Variables In Java Language?
- How To Declare Variables In Java Programs?
- How To Initialize Variables In Java?
- Naming Conventions For Variables In Java
- Types Of Variables In Java
- Local Variables In Java
- Instance Variables In Java
- Static Variables In Java
- Final Variables In Java
- Scope and Lifetime of Variables In Java
- Data Types Of Variables In Java (Primitive & Non-primitive)
- Java Variable Type Conversion & Type Casting
- Working With Variables In Java (Examples)
- Access Modifiers & Variables In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Are Identifiers In Java?
- Syntax Rules For Identifiers In Java
- Valid Identifiers in Java
- Invalid Identifiers in Java
- Java Reserved Keywords
- Naming Conventions & Best Practices For Identifiers In Java
- What Is An Identifier Expected Error In Java?
- Reasons The Identifier Expected Error Occurs
- How To Fix/ Resolve Identifier Expected Errors In Java?
- Conclusion
- Frequently Asked Questions
Table of content:
- What Are Data Types In Java?
- Primitive Data Types In Java
- Non-Primitive Data Types In Java
- Key Differences Between Primitive And Non-Primitive Data Types In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Are Operators In Java?
- Types Of Operators In Java
- Unary Operators In Java
- Arithmetic Operators In Java
- Assignment Operators In Java
- Relational Operators In Java
- Logical Operators In Java
- Bitwise Operators In Java
- Shift Operators In Java
- Increment & Decrement Operators In Java
- Ternary Operator In Java
- Instanceof Operator In Java
- Precedence & Associativity Of Java Operators
- Advantages & Disadvantages Of Operators In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is A Return Statement In Java?
- Use Cases Of Return Statements In Java
- Returning A Value From A Method In Java
- Returning A Class Object In Java
- Returning Void (No Value) In Java
- Advantages Of Using Return Statements In Java
- Limitations Of Using Return Statements In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Are Keywords In Java?
- List Of Keywords In Java
- Detailed Overview Of Java Keywords With Examples
- What If When Keywords In Java Are Used As Variable Names?
- Difference Between Identifiers & Keywords In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Abstract Keyword In Java?
- Use Of Abstract Keyword In Java
- Abstract Methods In Java
- Abstract Classes In Java
- Advantages Of Abstract Keyword In Java
- Disadvantages Of Abstract Keyword In Java
- Abstract Classes Vs. Interfaces In Java
- Real-World Applications Of Abstract Keyword
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is throws Keyword In Java?
- How Does The throws Keyword Work?
- Throwing A Checked Exception Using throws In Java
- Throwing Multiple Exceptions Using throws In Java
- Throwing A Custom Exception Using throws In Java
- When To Use The throws Keyword In Java
- Difference Between throw and throws Keyword In Java
- Best Practices For Using The throws Keyword In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is The Volatile Keyword In Java?
- How Does Volatile Keyword In Java Work?
- Using Volatile Keyword In Java To Control Thread Execution
- Using Volatile Keyword In Java To Signal Between Multiple Threads
- Difference Between Synchronization And Volatile Keyword
- Common Mistakes And Best Practices While Using Volatile Keyword In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding Super Keyword In Java
- Super Keyword In Java With Instance Variables
- Super Keyword In Java With Method Overriding
- Super Keyword In Java With Constructor Chaining
- Applications Of Super Keyword In Java
- Difference Between This And Super Keyword In Java
- Advantages Of Using Super Keyword In Java
- Limitations And Considerations Of Super Keyword In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding This Keyword In Java
- Uses Of This Keyword In Java
- Using This Keyword For Referencing Instance Variables
- Using This Keyword For Invoking A Constructor
- Using This Keyword For Invoking A Method
- Using This Keyword With Getters And Setters
- Difference Between This And Super Keyword In Java
- Best Practices For Using This Keyword In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is new Keyword In Java?
- Uses Of The new Keyword In Java
- Memory Management With new Keyword In Java
- Example 1: Creating An Object Of A Class Using new Keyword In Java
- Example 2: Creating An Array Using The new Keyword In Java
- Best Practices For Using new Keyword In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is The Transient Keyword In Java?
- Real-Life Example Of The Transient Keyword In Java
- When To Use The Transient Keyword In Java
- Example 1: Effect Of Transient Keyword On Serialization In Java
- Example 2: Skipping Sensitive Data During Serialization With Transient Keyword In Java
- Using Transient With Final Keyword In Java
- Using Transient With Static Keyword
- Difference Between Transient And Volatile Keyword In Java
- Advantages And Disadvantages Of Transient Keyword In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Static Keyword In Java?
- Characteristics Of Static Keyword In Java
- Static Variables In Java
- Static Method In Java
- Static Blocks In Java
- Static Classes In Java
- Static Variables Vs Instance Variables In Java
- Advantages Of Static Keyword In Java
- Disadvantages Of Static Keyword In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is A Static Method In Java?
- Use Cases Of Static Method In Java
- Using Static Method In Java To Create A Utility Class
- Using Static Method In Java To Implement The Singleton Design Pattern
- Difference Between Static And Instance Methods In Java
- Limitations Of Static Method In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding Final Keyword In Java
- Final Variables In Java
- Final Methods In Java
- Final Classes In Java
- Difference Between Static And Final Keyword In Java
- Uses Of Final Keyword In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Key Difference Between final, finally, And finalize In Java
- What Is final Keyword In Java?
- What Is finally Keyword In Java?
- What Is finalize Keyword In Java?
- When To Use Which Keyword In Java?
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding The extends Keyword In Java?
- Use Of extends Keyword In Java
- Using Java extends To Implement Single Inheritance
- Using Java extends With Interfaces (Default Methods)
- Overriding Using extends Keyword In Java
- Difference Between extends And implements In Java
- Real World Applications Of Extends Keyword In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Decision Making Statement In Java?
- If Statement In Java
- If-Else Statement In Java
- Else-If Ladder In Java
- Switch Statement In Java
- Ternary/Conditional Operator (?:) In Java
- Best Practices For Writing Decision Making Statements In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Are Control Statements in Java?
- Decision-Making Control Statements In Java
- Looping Control Statements In Java
- Jump (Branching) Control Statements In Java
- Application Of Control Statements In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is The Break Statement In Java?
- Working Of The Break Statement In Java
- Using Java Break Statement With Loops
- Using Java Break Statement With Switch Statement
- Using Java Break Statement With Infinite Loops
- Common Pitfalls While Using Break Statements In Java
- Best Practices For Using The Break Statement In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Switch Statement In Java?
- Working Of The Switch Statement In Java
- Example Of Switch Statement In Java
- Java Switch Statement With String
- Java Nested Switch Statements
- Java Enum In Switch Statement
- Java Wrapper Classes In Switch Statements
- Uses Of Switch Statement In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Syntax Of main() Method In Java
- public Specifier – Main Method In Java
- static Keyword – Main Method In Java
- void Return Type Of Main Method In Java
- The main Identifier – Main Method In Java
- String[] args In Main Method In Java
- The Role Of Java Virtual Machine (JVM)
- Running Java Programs Without The Main Method
- Variations In Declaration Of Main Method In Java
- Overloading The Main Method In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Method Overriding In Java?
- Example Of Method Overriding In Java
- Ideal Use Cases Of Method Overriding In Java
- Rules For Method Overriding In Java
- Super Keyword & Method Overriding In Java
- Constructor & Method Overriding In Java
- Exception Handling In Method Overriding In Java
- Access Modifiers In Method Overriding In Java
- Advantages & Disadvantages Of Method Overriding In Java
- Difference Between Method Overloading Vs. Method Overriding In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Method Overloading In Java?
- Different Ways Of Method Overloading In Java
- Overloading The main() Method In Java
- Type Promotion & Method Overloading In Java
- Null Error & Method Overloading In Java
- Advantages Of Method Overloading In Java
- Disadvantages Of Method Overloading In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Difference Between Overloading And Overriding In Java (Comparison Table)
- What Is Method Overloading In Java?
- What Is Method Overriding In Java?
- Key Differences Between Overloading & Overriding In Java Explained
- Difference Between Overloading And Overriding In Java Code Example
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is A One-Dimensional Array In Java?
- Key Characteristics Of One-Dimensional Arrays In Java
- Declaration Of One-Dimensional Array In Java
- Initialization Of One-Dimensional Array In Java
- Common Operations On One-Dimensional Array In Java
- Advantages Of One-Dimensional Arrays In Java
- Disadvantages Of One-Dimensional Arrays In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is A Multidimensional Array In Java?
- Difference Between Single-Dimensional And Multidimensional Arrays In Java
- Declaring Multidimensional Arrays In Java
- Initializing Multidimensional Arrays In Java
- Accessing And Manipulating Elements In Multidimensional Arrays In Java
- Working Of Multidimensional Arrays With Jagged Arrays In Java
- Why Use Multidimensional Arrays In Java?
- Limitations Of Multidimensional Arrays In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Are Jagged Arrays In Java?
- Comparison With Regular Multi-Dimensional Arrays
- Declaring Jagged Arrays In Java
- Initialization Of Jagged Arrays In Java
- Printing Elements Of A Jagged Array In Java
- Accessing And Modifying Elements Of A Jagged Array In Java
- Advantages Of Jagged Arrays In Java
- Disadvantages Of Jagged Arrays In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Array Of Objects In Java?
- Declare And Initialize An Array Of Object In Java
- Example Of An Array Of Objects In Java
- Sorting An Array Of Objects In Java
- Passing Arrays Of Objects To Methods In Java
- Returning Arrays Of Objects From Methods In Java
- Advantages Of Arrays Of Objects In Java
- Disadvantages Of Arrays Of Objects In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is A Dynamic Array In Java?
- Why Use Dynamic Array In Java?
- What Is The Size And Capacity Of A Dynamic Array In Java?
- How To Create A Dynamic Array In Java?
- Managing Dynamic Data Input In Java
- Storing And Processing Real-Time Data In Java
- Use Cases Of Dynamic Arrays In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Why Return An Array In Java?
- How To Return An Array In Java
- Example 1: Returning An Array Of First N Squares
- Example 2: Doubling the Values of an Array
- Common Scenarios For Returning Arrays In Java
- Points To Remember When Returning Arrays In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding ArrayList In Java
- Differences Between Arrays And ArrayList In Java
- Returning An ArrayList In Java
- Common Use Cases For Returning An ArrayList In Java
- Pitfalls To Avoid When Returning An ArrayList In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is A Thread In Java?
- Thread Vs Process
- What is a Thread Life Cycle In Java?
- What Are Thread Priorities?
- Creating Threads In Java
- Java Thread Methods
- Commonly Used Constructors In Thread Class
- Thread Synchronization In Java
- Common Challenges Faced While Using Threads In Java
- Best Practices For Using Threads In Java
- Real-World Applications Of Threads In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding Multithreading In Java
- Methods Of Multithreading In Java (Examples)
- Difference Between Multithreading And Multitasking In Java
- Handling Exceptions In Multithreading
- Best Practices For Multithreading In Java
- Real-World Use Cases of Multithreading In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Thread Priority In Java?
- Built-In Thread Priority Constants In Java
- Thread Priority: Setter & Getter Methods
- Limitations Of Thread Priority In Java
- Best Practices For Using Thread Priority In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Thread Synchronization In Java?
- The Need For Thread Synchronization In Java
- Types Of Thread Synchronization In Java
- Mutual Exclusion In Thread Synchronization In Java
- Coordination Synchronization (Thread Communication) In Java
- Advantages Of Thread Synchronization In Java
- Disadvantages Of Thread Synchronization In Java
- Alternatives To Synchronization In Java
- Deadlock And Thread Synchronization In Java
- Real-World Use Cases Of Thread Synchronization In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is A Daemon Thread In Java?
- User Threads Vs. Daemon Threads In Java
- Methods For Daemon Threads In The Thread Class
- Creating Daemon Threads In Java
- Checking The Daemon Status Of A Thread
- Exceptions In Daemon Threads
- Limitations Of Daemon Threads In Java
- Practical Applications Of Daemon Threads In Java
- Common Mistakes To Avoid When Working With Daemon Threads In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Why Do Threads Need To Communicate?
- Understanding Inter Thread Communication In Java
- The wait() Method In Inter-Thread Communication
- The notify() Method In Inter-Thread Communication
- The notifyAll() Method In Inter-Thread Communication
- Difference Between wait() And sleep() Methods In Java
- Best Practices For Inter Thread Communication In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding The Factorial Concept
- Approaches To Implementing Factorial In Java
- Find Factorial In Java Using Iterative Approach (Using a Loop)
- Find Factorial In Java Using Recursive Approach
- Complexity Analysis Of Factorial Programs In Java
- Applications Of Factorial Program In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding The Leap Year Concept
- Approach To Check A Leap Year In Java
- Alternative Approach To Check A Leap Year In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is The Difference Between JDK, JRE, and JVM?
- What Is JVM (Java Virtual Machine)?
- What Is JRE (Java Runtime Environment)?
- What Is JDK (Java Development Kit)?
- Understanding The Difference Between JDK, JRE, And JVM
- Comparison Table For Difference Between JDK, JRE, And JVM
- Conclusion
- Frequently Asked Questions
Table of content:
- Difference Between Abstraction And Encapsulation In Java
- Understanding Abstraction In Java
- Understanding Encapsulation In Java
- When To Use Abstraction And Encapsulation?
- Conclusion
- Frequently Asked Questions
Table of content:
- Differences Between Abstract Class And Interface In Java
- What Is An Abstract Class In Java?
- What Is An Interface In Java?
- When To Use An Abstract Class?
- When To Use Interface?
- Compatibility Between Abstract Class And Interface In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Error Vs. Exception In Java
- What Is Error In Java?
- What Is Exception In Java?
- Best Practices For Handling Exceptions In Java
- Why Errors Should Not Be Handled In Java?
- Conclusion
- Frequently Asked Questions
Table of content:
- Key Differences: Java Vs. JavaScript
- What Is Java?
- What Is JavaScript?
- Difference Between Java And JavaScript Explained
- Conclusion
- Frequently Asked Questions
Table of content:
- Brief Introduction To C++
- Brief Introduction To Java
- Difference Between C++ and Java
- Overview & Features Of C++ Language
- Overview & Features of Java Language
- Example of C++ and Java Program
- Key Difference Between C++ And Java Explained
- Similarities Between Java Vs. C++
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- Basic Java interview questions and answers
- Intermediate Java interview questions and answers
- Advanced Java interview questions and answers
Table of content:
- Difference between core Java and advanced Java
- Important Core Java Questions
- Tips for Preparing for Core Java
Variables In Java | Declare, Initialise & Types (+Code Examples)
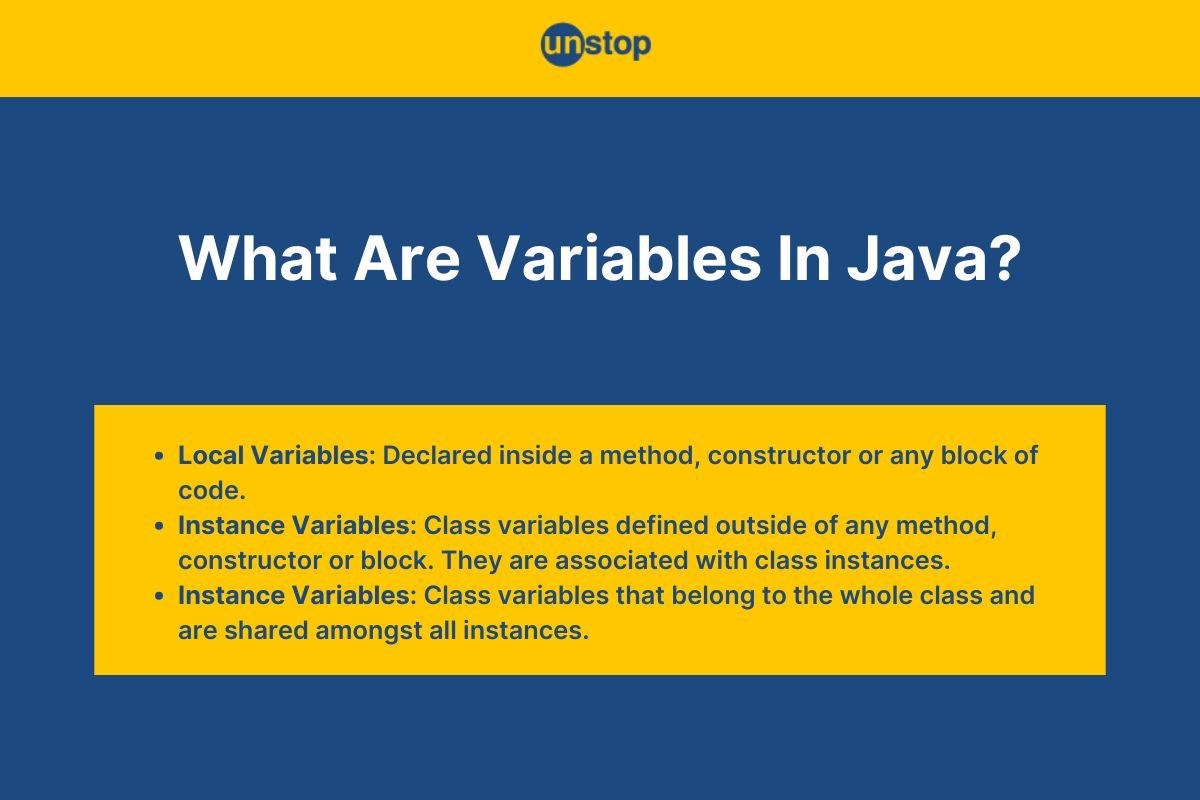
In programming, variables are essential for managing data during execution, making them fundamental to any project, big or small. Naturally, variables are like the backbone of any Java program. Just as data types define what kind of information a program can handle, variables determine how that information is stored and manipulated. In this article, we will explore different types of variables in Java, their applications, scope, and behavior with examples.
What Are Variables In Java Language?
Variables in Java programming are named memory locations or placeholders used to store data values. They act like containers that hold values, which can be changed or manipulated throughout the program.
Picture this: you have a box labelled "age,” and you can place different values (like 25, 30, or 42) into this box as needed. Similarly, in Java programming language, you can declare a variable called age (name of the memory location) and assign it a specific value, like so:
int age = 25;
Here:
- The keyword int indicates that age is an integer variable, meaning it will hold integer values.
- Value 25 is the initial value assigned to it.
The key thing to understand is that variables allow us to refer to data by name, and this is essential for writing reusable, flexible code. In the following sections, we will discuss how to declare and initialize variables in Java in detail, along with examples.
How To Declare Variables In Java Programs?
Variable declaration in Java means introducing it to the program by specifying the data type and the variable name/ identifier but not necessarily giving it a value at that point.
Key Steps To Declare A Variable In Java:
- Data Type Selection: Choose the type of data the variable will hold. The Java data types include many primitive types (e.g., int, double, String) and non-primitive types (arrays, enum, interface, etc).
- Variable Name: Use a meaningful, descriptive name that reflects the purpose or type of data. We generally use the camelCase convention in variable names.
- End with a Semicolon: Don’t forget to terminate the statement!
Syntax For Declaration Of Variables In Java:
dataType variableName;
Here,
- The dataType represents the type of data the variable will hold (e.g., int, double, String).
- The variableName refers to the name/ identifier we give to the variable, which we can use to access it across the program.
Example:
int age; // Declaration only, no value assigned
String name; // Declaration of a String variable
Here, we have simply declared one integer variable and a string variable without assigning values. The program now knows about these variables and their declaration type but doesn’t yet know what values they hold.
How To Initialize Variables In Java?
Variable initialization in Java involves assigning an initial value during or after the declaration. Doing so ensures that the variable has a meaningful value and is ready for use in the program. Once a variable has been declared, you can set its value using the simple assignment operator (=).
Syntax For Initialization Of Variables In Java:
dataType variableName = initialValue;
Here, the first part of the syntax (before =) is the same as in the declaration. After that, we use the assignment operator represented by an equals sign (=) and assign it a value given by initialValue. The simple Java program example below illustrates how to declare, initialize and print variables.
Code Example:
public class Main {
public static void main(String[] args) {
// Declare and initialize variables
int number = 9;
double price = 29.99;
String name = "Unstop";
// Display initialized variables
System.out.println("Number: " + number);
System.out.println("Price: " + price);
System.out.println("Name: " + name);
}
}
Output:
Number: 9
Price: 29.99
Name: Unstop
Code Explanation:
In the simple Java code example,
- We begin by declaring a public class named Main. Every Java program must have at least one class, which serves as the blueprint for objects, encapsulating variables and methods that perform actions within the program.
- Inside Main, we define a public method called static void main(), which is the entry point of any Java application/ the first method that gets executed.
- The public keyword means that it is accessible from outside of the class, and the static keyword means that the method belongs to the class itself and not an instance. So, it can be called without creating a class object.
- It is of type void, meaning there is no return value, and the parameter String[] args allows it to accept command-line arguments as an array of strings.
- Inside main(), we declare three variables—number, price, and name—using different data types.
- Variable number is an integer variable which we initialize with the value 9.
- Then, we have the double variable price, initialized with the value 29.99.
- And the String variable called name is assigned the value "Unstop".
- As you can see, the data type used for each variable corresponds to the kind of value we want to store in it.
- Initialization is important because variables in Java must be assigned values before they are used. If we tried to use a variable without assigning it a value, the program would not compile.
- After that, we use the System.out.println() method to print their values to the console. Here, we use the addition/ concatenation operator (+) for string concatenation. It combines the text (inside the quotes) with the value of the variable, allowing us to print both in one statement.
- The program terminates once the main() method finishes executing.
Naming Conventions For Variables In Java
There are well-defined naming conventions for variables in Java to ensure code clarity and consistency. Following these conventions helps in making code more readable and easier to maintain. In the table below, we have listed the key naming rules, along with examples and explanations:
Naming Convention | Example | Permissible? | Explanation |
---|---|---|---|
CamelCase | myVariableName | Yes | Used for naming variables and methods. Start with a lowercase letter, and capitalize each subsequent word. |
Descriptive Names | studentAge | Yes | Variable names should be meaningful and describe their purpose (e.g., studentAge indicates the age of a student). |
Underscores | my_variable | Yes, but discouraged | Although allowed, the use of underscores in Java variable names is discouraged. Use camelCase instead for better readability. |
Numbers | number1 | Yes |
Avoid using numbers in variable names unless absolutely necessary. Use more meaningful names like studentCount rather than student1. |
PascalCase | MyClass | Yes (for classes and interfaces) |
Used for naming classes and interfaces. Each word starts with an uppercase letter. If the name consists of multiple words, each subsequent word is capitalized (e.g., MyClass). |
UPPER_CASE | MAX_SIZE | Yes (for constants) |
Used for constants and final static variables. Words are separated by underscores and are all uppercase. |
Abbreviations | numStudents | Yes | Use abbreviations only if they are widely understood and clear (e.g., num for numbers in numStudents). |
Package Naming | com.example.project | yes | Packages are named in lowercase, often prefixed with a reversed domain name to ensure uniqueness. |
Types Of Variables In Java
In Java, variables can be categorized based on their scope and usage within a program. Understanding these types is essential for managing data effectively and writing clean code. The four main types of variables in Java are:
- Local Variables: Variables declared within a method, constructor, or block of code and exist only in that context.
- Instance Variables: Variables defined at the class level that belong to an instance of the class.
- Static Variables: Variables shared among all instances of a class, defined with the static keyword.
- Final Variables: Variables whose values cannot be changed once assigned, making them constants.
Want to expand your knowledge of Java programming? Check out this amazing course and become the best version of Java developer you can be.
Local Variables In Java
As mentioned above, local variables are those that are declared within a method, constructor, or any block of code. Their characteristics and behaviors make them essential for managing data specific to a particular execution context.
- Scope: Local variables in Java are accessible only within the method, constructor, or block in which they are declared. They cannot be accessed from outside this scope, ensuring that they are used only for their intended purpose.
- Lifetime: Local variables are created when the method, constructor, or block is entered and destroyed when it exits. This means they exist only during the execution of that particular block of code.
- Initialization: Unlike instance or static variables, local variables in Java must be explicitly initialized before they can be used. If you try to access a local variable without initializing it, the compiler will throw an error.
- Memory Management: Because local variables are created and destroyed dynamically, they are typically stored on the stack. This leads to efficient memory usage, especially in recursive functions.
Syntax For Local Variables In Java:
dataType variableName;
dataType variableName = initial_value
This is the same as the processes of declaration and initialization we discussed in the sections above. We revisit the declaration and initialization of local variables in the Java program example below.
Code Example:
public class Main {
public static void main(String[] args) {
// Declare and initialize a local variable
int number = 10;
// Print the value of the local variable
System.out.println("Number: " + number);
}
}
Output:
Number: 10
Code Explanation:
In this Java code example,
- We declare a variable number (of type int) inside the main() method and assign the value 10 to it. This is the local variable inside main().
- We then print its value to the console using the System.out.println() method. Since number is a local variable, it is created when the main() method is called and destroyed when the method completes execution.
Instance Variables In Java
Instance variables are those class variables defined outside of any method, constructor, or block. They are associated with instances (or objects) of the class and provide a way to store object-specific data. Each instance of a class has its own copy of the instance variables, making them unique to each object.
- Scope: Instance variables in Java have class-level scope and are accessible throughout the class in which they are declared. This means they can be used in any method of the class, allowing for easy data management related to the object's state.
- Lifetime: The lifetime of instance class variables is tied to the lifespan of the object they belong to. They are created when the object is instantiated and are destroyed when the object is no longer referenced, usually when it is garbage collected.
- Initialization: If not explicitly initialized, instance variables in Java are automatically assigned default values. For example, numeric types (e.g., int, double) default to 0, boolean types default to false, and object references default to null.
Syntax For Instance Variables In Java:
accessModifier dataType variableName;
Here,
- The accessModifier controls the accessibility of the variable, which can be public, private, or protected.
- The dataType specifies the type of data the variable whose name is given by variableName will hold (e.g., int, double, String).
Code Example:
public class Main {
// Instance variables
private String model;
private int year;
// Constructor
public Main(String model, int year) {
this.model = model; // Assign the parameter model to the instance variable
this.year = year; // Assign the parameter year to the instance variable
}
// Method to display car details
public void displayDetails() {
System.out.println("Model: " + model);
System.out.println("Year: " + year);
}
public static void main(String[] args) {
// Create an instance of the Main class
Main myCar = new Main("Toyota", 2022);
// Call the displayDetails method to print car details
myCar.displayDetails();
}
}
Output:
Model: Toyota
Year: 2022
Code Explanation:
In the example Java code,
- We declare two instance variables: model of type String and year of type int, inside the Main class. These variables are associated with instances (objects) of the Main class, meaning that each object of the Main class has its own copy of these variables, allowing different instances to hold different values.
- Then, we define a constructor Main() that initializes the instance variables with the values provided as arguments when an object is created. The this keyword is used to differentiate between the instance variables and the parameters.
- Next, we define displayDetails() method to print the details of the car. It accesses the instance variables model and year to display their values using System.out.println().
- After that, we define the main() method and create an instance of the Main class named myCar using the constructor. We pass arguments "Toyota" and 2022 to the constructor to initialize the model and year instance variables of the myCar object, respectively.
- Then, we call the displayDetails() method on the myCar object to print its details. This method call results in the output displaying the model as "Toyota" and the year as 2022.
This example shows how we can use an instance/ object of the class to initialize and access the instance variables in the class.
Static Variables In Java
Static variables are those that are defined at the class level and are shared among all instances of the class. Unlike instance variables, static variables belong to the class itself rather than to any individual object. This means that there is only one copy of a static variable, regardless of how many instances of the class are created.
- Scope: Static variables have class-level scope and are accessible throughout the class in which they are declared. They can also be accessed from outside the class using the class name, making them visible across all instances of that class.
- Lifetime: The lifetime of static variables in Java extends throughout the execution of the program. They are created when the class is loaded into memory and exist until the program terminates. Static variables retain their values across method calls and instances.
- Initialization: Static variables in Java are initialized only once, either explicitly (with a value) or implicitly (to their default values). If not explicitly initialized, static variables take on default values: numeric types default to 0, boolean types default to false, and object references default to null.
Syntax For Static Variables In Java:
static dataType variableName;
The syntax for declaration remains the same as local variables, only here we use the static keyword to indicate that the variable belongs to the class itself, rather than to any particular instance.
Code Example:
public class Counter {
// Static variable
static int count;
// Method to increment count
public static void increment() {
count++;
}
// Method to display count
public static void displayCount() {
System.out.println("Count: " + count);
}
public static void main(String[] args) {
// Increment count multiple times
increment(); // count becomes 1
increment(); // count becomes 2
increment(); // count becomes 3
// Display the final count
displayCount(); // Output: Count: 3
}
}
Output:
Count: 3
Code Explanation:
In the Java example code,
- We create a class called Counter and then declare a static integer variable count inside. Since count is static, all instances of Counter class will share the same variable, allowing it to keep track of the total number of times it has been incremented across all instances.
- Then, we define a static method increment() that increases the value of the static variable count by 1 each time it is called. This method can be invoked without creating an instance of the class, demonstrating the class-level nature of static variables.
- Next, we define the displayCount() method that outputs the current value of the static variable count to the console using System.out.println().
- In the main() method, we call the increment() method three times, causing the count to increase from its default value of 0 to 3.
- After that, we call the displayCount() to print the final value of the count, which results in the output displaying "Count: 3."
Final Variables In Java
In Java, final variables are variables that can only be assigned once. Once a final variable is initialized, its value cannot be changed, making it act like a constant throughout the program. These variables are typically used when you want to create constants or ensure that a particular variable's value remains the same after initialization.
- Immutability: Once initialized, the value of a final variable cannot be altered. Trying to reassign a value to a final variable will result in a compilation error.
- Initialization: A final variable must be initialized either at the time of declaration or within the constructor of the class. This ensures that the variable has a value before it's used, but once assigned, that value is locked.
- Usage: The final variables in Java are commonly used to define constants (e.g., PI, MAX_VALUE) or to mark variables whose values should remain constant throughout the program’s execution. These variables are often used in mathematical calculations or when dealing with fixed configurations.
Syntax For Final Variables In Java:
final dataType variableName = initialValue;
The general syntax for declaration and initialization remains the same as variables in Java. The difference is, here we use the final keyword to indicate that the variable is constant and its value cannot be changed.
Also, the name for such variables must follow the naming convention for constants, i.e., all caps with words connected using underscore, like MAX_VALUE.
Code Example:
public class Main {
// Final variable
final double PI = 3.14;
// Method to calculate area of a circle
public void calculateArea(double radius) {
double area = PI * radius * radius;
System.out.println("Area of the circle: " + area);
}
public static void main(String[] args) {
Main circle = new Main();
circle.calculateArea(5);
}
}
Output:
Area of the circle: 78.5
Code Explanation:
In this Java code sample,
- Inside the Main class, we declare a final variable named PI of type double and initialized with the value 3.14. Since PI is marked as final, its value cannot be modified anywhere else in the program.
- Then, we define a calculateArea() method to compute the area of a circle based on its radius. It uses the formula PI * radius * radius, where PI is the final variable representing the mathematical constant π.
- In the main() method, we create an instance of the Main class named circle using the new keyword.
- Next, we call the calculateArea() method using the circle object and passing a radius of 5 as an argument. The function calculates and prints the area.
The key point here is that the value of PI remains unchanged throughout the program, reinforcing the concept of immutability that comes with the final variables. Even if the program attempted to modify the value of PI, it would result in a compilation error, ensuring the integrity of the constant value.
Scope and Lifetime of Variables In Java
In Java, variables have both scope and lifetime, which determine where they can be accessed and how long they exist during program execution. Understanding these concepts is essential to manage memory effectively and avoid errors.
Scope: It defines the region of the program where a variable is visible and can be accessed.
- Local Variables: These have a narrow scope and are only accessible within the block of code (like a method or a loop) where they are declared. Once the program exits this block, the local variables are no longer accessible.
- Instance Variables: These variables in Java are associated with a specific instance (or object) of a class. They have class-level scope, meaning they can be accessed anywhere within the class in which they are declared. However, each instance of the class has its own copy of the instance variables.
- Static Variables: These variables in Java also have a class-level scope, but they are shared among all instances of the class. They can be accessed anywhere within the class and across all objects of that class without needing an object reference.
Lifetime: It refers to how long a variable exists in memory during the execution of the program.
- Local Variables: These are created when the block of code (method or loop) in which they are declared is entered and are destroyed when the block is exited. They only live as long as the block of code runs.
- Instance Variables: These are created when an object of the class is instantiated. They exist for as long as the object exists, and they are destroyed when the object is no longer in use and gets garbage collected by the Java Virtual Machine (JVM).
- Static Variables: These are initialized when the class is loaded into memory (even before any objects are created). Static variables persist for as long as the class remains loaded and are destroyed only when the class is unloaded, typically when the program ends.
Real-life Example:
Imagine you're in a classroom, and your teacher (the Java program) gives you a piece of paper (a variable) to write down answers to a quiz.
- Local Variable: If the paper is only passed around within your small group during the quiz, it’s like a local variable. It can only be accessed by those in the group (block of code), and it disappears when the quiz ends (when the block finishes executing).
- Instance Variable: If each student in the class receives their own sheet of paper, it’s like an instance variable. Each student (instance) has their own copy, and they can keep using it until the class ends (until the object is garbage collected).
- Static Variable: If the teacher posts the paper on the classroom wall for everyone to see, it’s like a static variable. It’s accessible to all students (all instances), and it stays on the wall for the entire school year (for the lifetime of the class).
Data Types Of Variables In Java (Primitive & Non-primitive)
Data types in Java define the kind of data a variable can hold and the operations that can be performed on it. They are broadly classified into two categories: Primitive and Non-primitive data types.
Primitive Data Types: These are the most basic types of data and store simple values. For example:
- int (e.g., int age = 25;) for integers,
- double (e.g., double price = 19.99;) for decimal numbers,
- char (e.g., char grade = 'A';) for characters,
- boolean (e.g., boolean isAvailable = true;) for true/false values.
Non-primitive (Reference) Data Types: These reference objects rather than storing data directly. For example:
- Arrays (e.g., int[] numbers = {1, 2, 3};) for collections of similar types,
- Classes (e.g., Scanner input = new Scanner(System.in);) for user-defined or built-in objects.
Understanding these types is essential for effectively working with variables in Java. For more, read: Data Types In Java | Primitive & Non-Primitive With Code Examples
Java Variable Type Conversion & Type Casting
In Java, converting variables from one data type to another is a common operation. This process can happen either automatically (type conversion) or manually (type casting).
Type Conversion (Implicit): Also known as type coercion, this is automatically handled by the compiler when performing operations involving different data types. For example, if you add an int and a double, the int is automatically converted to a double before the operation.
Type Casting (Explicit): Type casting is when the Java programmer explicitly converts a variable from one data type to another. It’s necessary when narrowing (converting a larger type to a smaller type), as there’s potential for data loss. There are two kinds of casting:
- Widening (implicit): Converts smaller data types to larger ones, automatically handled by the compiler.
- Narrowing (explicit): Converts larger data types to smaller ones, requiring manual casting by the programmer.
Here is your chance to top the leaderboard while practising your coding skills: Particiapte in the 100-Day Coding Sprint at Unstop.
Working With Variables In Java (Examples)
In this section, we'll explore how to access, change, and read values of variables in Java through practical examples.
Accessing Variables In Java
To access a variable means to retrieve its value and use it in expressions or statements. Variables can be accessed within their scope using their names.
Code Example:
public class VariableAccessExample {
public static void main(String[] args) {
// Declare and initialize variables
int number = 10;
String message = "Hello, Unstoppable!";
// Access and print variable values
System.out.println("Number: " + number);
System.out.println("Message: " + message);
}
}
Output:
Number: 10
Message: Hello, Unstoppable!
Code Explanation:
- In the sample Java code, we declare and initialize two variables: number of type int with value 10 and message of type String with value "Hello, Unstoppable".
- We then access the values of these variables using their names (number and message) and print them to the console using the System.out.println() method.
Changing The Value Of A Variable In Java
Variables can be updated during program execution to reflect new data.
Code Example:
public class VariableChangeExample {
public static void main(String[] args) {
// Declare and initialize a variable
int number = 5;
// Change the value of the variable
number = 10;
// Print the updated value
System.out.println("Number: " + number);
}
}
Output:
Number: 10
Code Explanation:
- In this example, we first declare and initialize an integer variable number with the value 5. Then, we reassign the value 10 to it using the simple assignment operator.
- We then print the value to the console, highlighting how variable values can be modified during program execution.
Reading User Input For Different Types Of Variables In Java
Java allows us to read different types of input from users, such as integers, doubles, and strings, using the Scanner class.
Code Example:
import java.util.Scanner;
public class VariableInputExample {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in); // Create a Scanner object for user input
// Prompt user for input and read an integer
System.out.print("Enter an integer: ");
int intValue = scanner.nextInt();
// Prompt user for input and read a double
System.out.print("Enter a double: ");
double doubleValue = scanner.nextDouble();
// Consume newline character and read a string
scanner.nextLine();
System.out.print("Enter a string: ");
String stringValue = scanner.nextLine();
// Display the input values
System.out.println("\nUser Input:");
System.out.println("Integer: " + intValue);
System.out.println("Double: " + doubleValue);
System.out.println("String: " + stringValue);
// Close the scanner
scanner.close();
}
}
Output:
Enter an integer: 5
Enter a double: 3.14
Enter a string: Hello, Unstoppable!User Input:
Integer: 5
Double: 3.14
String: Hello, Unstoppable!
Code Explanation:
- In this example, we create a Scanner object named scanner to read user input from the console.
- We prompt the user to enter an integer, a double, and a string using the System.out.print() method individually.
- As use provides input, we use the nextInt(), nextDouble(), and nextLine() methods of the Scanner class to read integer, double, and string input. We also store them in the respective variables (intValue, doubleValue, stringValue) for further processing or display.
- After that, we use the System.out.println() method to display the values to the console.
- It is important to close the Scanner object using the close() method to release system resources after use.
This example demonstrates reading different types of input from the user using a Scanner. It reads an integer, a double, and a string, stores them in variables, and then prints them. The key takeaway is how Java handles user input for various data types.
Access Modifiers & Variables In Java
Access modifiers in Java are keywords that define the accessibility of classes, methods, and variables within a program. They play a crucial role in encapsulation and security by controlling which parts of the program can access certain elements.
Access Specifiers:
- public: Allows access from any other class. Use this when you want a variable to be universally accessible.
- private: Restricts access to within the same class. This is ideal for variables that should not be exposed outside the class.
- protected: Allows access within the same class and its subclasses. This is useful for allowing inheritance while maintaining some level of encapsulation.
- Default (no modifier specified): Restricts access to within the same package. This is useful for package-private access, allowing classes within the same package to interact while hiding variables from classes in other packages.
Syntax:
accessModifier dataType variableName;
Here, the accessModifier refers to the specifier we use, and the rest of the syntax remains the same for variable declaration. You must have seen the use of public modifiers in the examples above.
Need more guidance on how to become a Java developer? You can now select an expert to be your mentor here.
Conclusion
In this article, we explored the fundamental concepts of variables in Java. Variables serve as containers that store various data types, enabling us to manipulate and manage information effectively. When declaring variables, we must specify both the data type and the variable name, and we can initialize them by assigning a value using the assignment operator.
There are three primary types of variables: local variables that are defined within a function/ block of code, instance variables that belong to an instance of a class, and static variables that belong to the whole class. It is important to understand the difference between these variable types, as well as the nuances of data types. In addition, we discussed access modifiers, whose main role is to control the visibility and accessibility of variables, enhancing encapsulation and security in our code. By mastering these concepts, we lay a strong foundation for building sophisticated software that is both functional and adaptable to user needs.
Also read: Top 100+ Java Interview Questions And Answers (2024)
Frequently Asked Questions
Q. Difference between the instance and static variables In Java.
Instance Variables |
Static Variables |
|
Association |
Associated with instances (objects) of a class. |
Associated with the class itself rather than instances. |
Number of Copies |
Each instance has its own copy of instance variables. |
Only one copy is shared among all instances of the class. |
Initialization |
Initialized when an object is created. |
Initialized only once when the class is loaded into memory. |
Accessing |
Accessed using object references. |
Accessed using the class name. |
Q. What is the difference between public, private, protected, and default access modifiers?
Access Modifier | Visibility | Description |
---|---|---|
public | Any class | Accessible from any class. Used for methods and variables that need to be widely available. |
private | Same class | Restricted to the same class. Used for encapsulating implementation details. |
protected | Same class and subclasses | Accessible within the same class and subclasses. Allows inheritance while enforcing encapsulation. |
Default | Same package | Accessible only within the same package. Useful for package-level encapsulation. |
Q. What are the benefits of using different data types in Java variables?
- Efficient Memory Allocation: Different data types occupy varying amounts of memory, allowing for optimal storage.
- Precision and Accuracy: Using specific types, like for floating-point calculations, ensures higher precision.
- Type Safety: Enforces compatibility between operations and data types, preventing runtime errors.
Q. What is the difference between local variables and instance variables?
- Local Variables: Declared within a method and exist only within that method's scope. It must be initialized before use.
- Instance Variables: Declared within a class but outside any method. Initialized when an object is created and exists as long as the object exists.
Q. How do you read user input of different data types in Java?
User input can be read using the Scanner class. For example:
- To read an integer: int num = scanner.nextInt();
- To read a double: double value = scanner.nextDouble();
- To read a string: String text = scanner.nextLine();
Q. How do variables with different access modifiers impact code organization and security in Java?
Variables with different access modifiers significantly influence code organization and security in Java:
- Encapsulation and Information Hiding: Access modifiers such as private restrict access to variables within the same class, promoting encapsulation and information hiding. This prevents external classes from being directly able to access or modify the internal state of an object, enhancing data integrity and security.
- Code Maintainability and Readability: By using appropriate access modifiers with variables in Java programs, developers can clearly define their scope and visibility, making the codebase easier to understand and maintain. For example, using public for variables intended to be accessed by other classes and private for internal implementation details enhances code readability and maintainability.
By now, you must know all about variables in Java and how to use them appropriately. Here are a few more topics you must explore:
- Key Features Of Java, Java 8 & Java 11 Explained (+Code Examples)
- Advantages And Disadvantages of Java Programming Language
- List Of 50 Core Java Interview Questions With Answers (2022)
- Top 15+ Difference Between C++ And Java Explained! (+Similarities)
- Difference Between Java And JavaScript Explained In Detail
An economics graduate with a passion for storytelling, I thrive on crafting content that blends creativity with technical insight. At Unstop, I create in-depth, SEO-driven content that simplifies complex tech topics and covers a wide array of subjects, all designed to inform, engage, and inspire our readers. My goal is to empower others to truly #BeUnstoppable through content that resonates. When I’m not writing, you’ll find me immersed in art, food, or lost in a good book—constantly drawing inspiration from the world around me.
Comments
Add commentLogin to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
Esuru Pooja.C 2 hours ago
Uma Maheshwari 1 day ago
Ankita Das 2 days ago
Nirdesh Sharma 3 days ago
Amar kumar Giri 6 days ago
Divyansh Shrivastava 1 week ago