Table of content:
- What Is Abstract Keyword In Java?
- Use Of Abstract Keyword In Java
- Abstract Methods In Java
- Abstract Classes In Java
- Advantages Of Abstract Keyword In Java
- Disadvantages Of Abstract Keyword In Java
- Abstract Classes Vs. Interfaces In Java
- Real-World Applications Of Abstract Keyword
- Conclusion
- Frequently Asked Questions
Abstract Keyword In Java | Classes, Methods & More (+Examples)
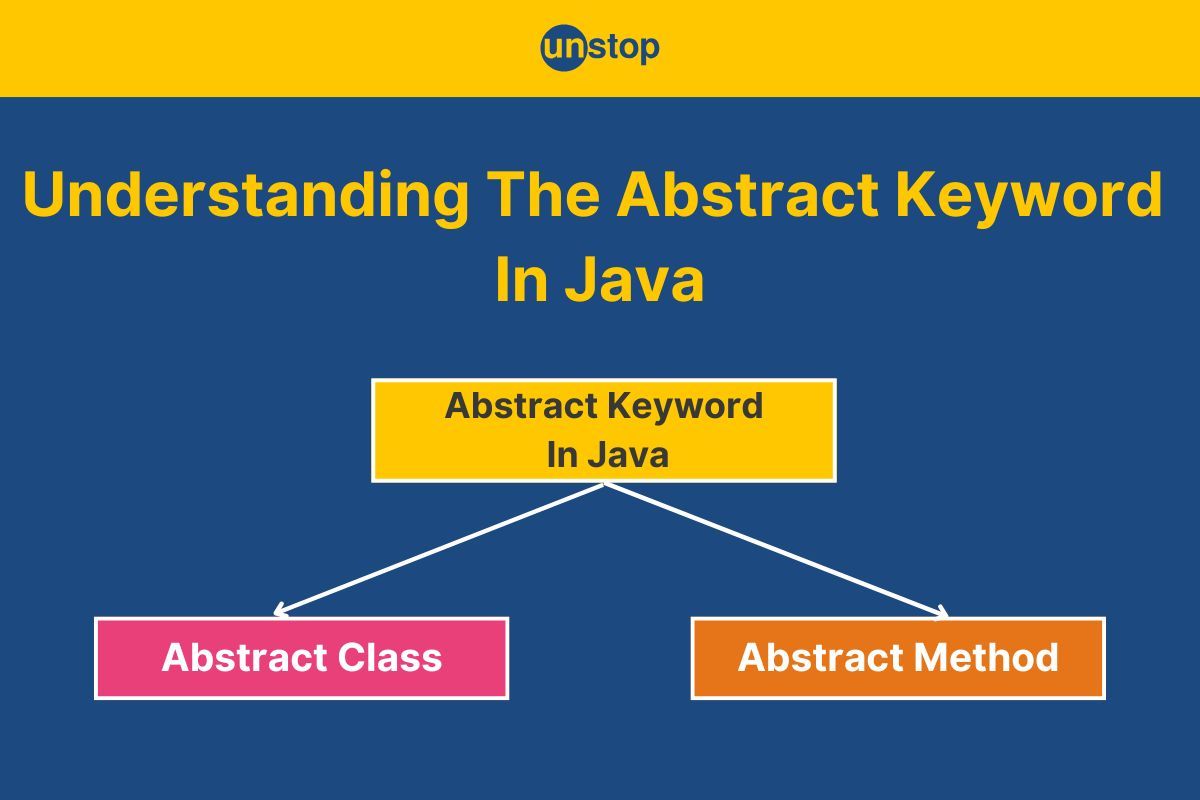
As budding software developers stepping into the world of object-oriented programming, understanding the core concepts of abstraction is crucial. In Java, the abstract keyword is a powerful tool that allows us to design flexible and reusable code by focusing on what an object does rather than how it does it.
In this article, we will explore the essence of the abstract keyword in Java, explaining how it enables the creation of abstract classes and methods. We'll break down its role in achieving abstraction, its implementation, and the benefits it offers in real-world programming scenarios. By the end, you'll have a solid grasp of how to utilize the abstract keyword to design more effective and structured Java programs.
What Is Abstract Keyword In Java?
The abstract keyword in Java programming is used to define abstract classes and abstract methods. It is a fundamental part of object-oriented programming that facilitates abstraction, allowing you to define the structure of a class without implementing all its functionality.
It is used to declare:
- Abstract Classes: Classes that cannot be instantiated and may contain abstract methods.
- Abstract Methods: Methods without a body, meant to be implemented by subclasses.
Abstraction focuses on "what" an object does rather than "how" it does it, making the abstract keyword a crucial element in designing flexible and reusable code.
Explore this amazing course and master all the key concepts of Java programming effortlessly!
Real-Life Analogy Of Abstract In Programming
Imagine a blueprint for a car.
- The blueprint outlines that every car must have basic functionalities like a steering wheel, brakes, and an engine.
- However, the specific details (e.g., type of engine, number of gears) are left to the car manufacturers to define based on the type of car (sports, SUV, sedan).
Here:
- Abstract class = Blueprint of the car.
- Abstract methods = Common functionalities required in all cars, but implementation depends on the specific car model.
Use Of Abstract Keyword In Java
The abstract keyword in Java is used for various purposes to define abstract classes and methods. Here’s how and where it can be used:
- Declaring Abstract Classes: The abstract keyword is used to declare a class as abstract, meaning it cannot be instantiated directly. Abstract classes serve as blueprints for other classes, outlining required methods without full implementation. For Example-
abstract class Shape {
abstract void draw(); // Abstract method
}
- Defining Abstract Methods: Abstract methods are declared using the abstract keyword within an abstract class. These methods do not have a body and must be implemented by subclasses. For Example-
abstract class Vehicle {
abstract void startEngine(); // Abstract method
}
- Enforcing Subclass Implementation: When an abstract method is declared in an abstract class, all subclasses inheriting the class must implement the abstract method (unless the subclass is also abstract). This ensures that certain behaviors are defined consistently across all subclasses. For Example-
abstract class Animal {
abstract void makeSound();
}class Dog extends Animal {
@Override
void makeSound() {
System.out.println("Woof!");
}
}
- Combining Abstract and Concrete Methods: Abstract classes can have both abstract methods (without implementation) and concrete methods (with implementation). This allows a mix of enforced behavior and reusable functionality. For Example-
abstract class BankAccount {
abstract void calculateInterest(); // Abstract methodvoid displayAccountType() { // Concrete method
System.out.println("This is a bank account.");
}
}
- Supporting Polymorphism: Abstract classes enable polymorphism by allowing a base class reference to refer to objects of its subclasses. This is useful when dealing with collections of related objects.
- Designing Frameworks and APIs: Abstract classes are heavily used in frameworks and APIs to define generic behaviors that subclasses must implement. For example, in Java’s Swing framework, classes like AbstractButton and AbstractListModel are abstract.
- Creating a Contract for Derived Classes: Abstract classes provide a structured "contract" by outlining what methods a derived class must define. This is useful in designing systems with a consistent interface. For Example-
abstract class Employee {
abstract void calculateSalary();
abstract void displayDetails();
}
- Avoiding Object Instantiation: Abstract classes ensure that certain base classes are never instantiated directly. Instead, they serve as templates for subclasses. For Example-
abstract class Appliance {
abstract void operate();
}class WashingMachine extends Appliance {
@Override
void operate() {
System.out.println("Washing clothes.");
}
}
Abstract Methods In Java
An abstract method in Java is a method that is declared in an abstract class but does not have any implementation (i.e., no method body). It serves as a placeholder for methods that must be implemented by the subclasses (derived classes). The abstract method forces the subclasses to provide their own implementation of the method.
Key Characteristics Of Abstract Methods
- No Method Body: Abstract methods are only declared, but they don’t have a body. The actual functionality of the method is provided by the subclass.
- Defined in Abstract Class: Abstract methods can only exist within abstract classes. They cannot be present in regular (non-abstract) classes.
- Must Be Overridden: Any subclass that inherits an abstract class must override and provide an implementation for all abstract methods unless the subclass is also abstract.
- Enforces a Contract: Abstract methods act as a contract, ensuring that all subclasses implement the required method(s).
Syntax Of Abstract Method
abstract returnType methodName(); // Abstract method
Here:
- abstract keyword: It indicates that the method is abstract and does not have a body.
- Return Type: It specifies the type of value the method returns (e.g., int, void).
- Method Name: The name of the method that follows normal naming conventions.
Code Example:
Ly8gQWJzdHJhY3QgY2xhc3Mgd2l0aCBhbiBhYnN0cmFjdCBtZXRob2QKYWJzdHJhY3QgY2xhc3MgQW5pbWFsIHsKCiAgICAvLyBBYnN0cmFjdCBtZXRob2QgKG5vIGltcGxlbWVudGF0aW9uKQogICAgYWJzdHJhY3Qgdm9pZCBtYWtlU291bmQoKTsgIC8vIEV2ZXJ5IGFuaW1hbCB3aWxsIG1ha2UgYSBzb3VuZAoKICAgIC8vIENvbmNyZXRlIG1ldGhvZCAoaW1wbGVtZW50ZWQgaW4gdGhlIGFic3RyYWN0IGNsYXNzKQogICAgdm9pZCBzbGVlcCgpIHsKICAgICAgICBTeXN0ZW0ub3V0LnByaW50bG4oIkFuaW1hbCBpcyBzbGVlcGluZy4iKTsKICAgIH0KfQoKLy8gQ29uY3JldGUgc3ViY2xhc3MgdGhhdCBwcm92aWRlcyBpbXBsZW1lbnRhdGlvbiBmb3IgdGhlIGFic3RyYWN0IG1ldGhvZApjbGFzcyBEb2cgZXh0ZW5kcyBBbmltYWwgewoKICAgIEBPdmVycmlkZQogICAgdm9pZCBtYWtlU291bmQoKSB7CiAgICAgICAgU3lzdGVtLm91dC5wcmludGxuKCJXb29mISBXb29mISIpOyAgLy8gRG9nJ3MgaW1wbGVtZW50YXRpb24gb2YgbWFrZVNvdW5kKCkKICAgIH0KfQoKY2xhc3MgTWFpbiB7CgogICAgcHVibGljIHN0YXRpYyB2b2lkIG1haW4oU3RyaW5nW10gYXJncykgewogICAgICAgIEFuaW1hbCBteURvZyA9IG5ldyBEb2coKTsgICAgICAgICAgIC8vIFBvbHltb3JwaGlzbQogICAgICAgIG15RG9nLm1ha2VTb3VuZCgpOyAgICAgICAgICAgICAgICAgIC8vIE91dHB1dDogV29vZiEgV29vZiEKICAgICAgICBteURvZy5zbGVlcCgpOyAgICAgICAgICAgICAgICAgICAgICAvLyBPdXRwdXQ6IEFuaW1hbCBpcyBzbGVlcGluZy4KICAgIH0KfQo=
Output:
Woof! Woof!
Animal is sleeping.
Explanation:
In the above code example-
- We begin with an abstract class Animal, which contains an abstract method makeSound(). This method is declared but not implemented in the Animal class, meaning that every subclass of Animal must provide its own implementation of makeSound().
- The sleep() method is a concrete method in the Animal class. It is fully implemented and outputs "Animal is sleeping." when called.
- The Dog class is a concrete subclass of Animal. It provides an implementation for the abstract makeSound() method. In this case, the makeSound() method is overridden to output "Woof! Woof!".
- In the Main class, we use polymorphism by creating an Animal reference, myDog, and assigning it an instance of the Dog class.
- When we call myDog.makeSound(), the Dog's version of makeSound() is invoked, printing "Woof! Woof!".
- We also call myDog.sleep(), which invokes the inherited sleep() method from the Animal class, outputting "Animal is sleeping.".
Sharpen your coding skills with Unstop's 100-Day Coding Sprint and compete now for a top spot on the leaderboard!
Abstract Classes In Java
An abstract class in Java is a class that is declared with the abstract keyword.It serves as a blueprint for other classes and is used when a base class defines common properties or methods that must be shared by its subclasses but should not be instantiated on its own.
Key Characteristics Of Abstract Classes
- Cannot be instantiated: You cannot create objects of an abstract class directly.
- May contain abstract methods: These are methods without a body (implementation), intended to be overridden in derived classes.
- Can contain concrete methods: Abstract classes can have fully implemented methods.
- Used for common functionality: They provide a base structure and enforce certain behaviors in derived classes.
- Can have constructors: Unlike interfaces, abstract classes can have constructors, but they are only called during object creation of derived classes.
Syntax Of Abstract Classes
abstract class ClassName {
abstract returnType methodName(); // Abstract method
returnType methodName() { // Concrete method
// method body
}
}
Here:
- abstract keyword: It is used to declare the class or method as abstract.
- Abstract Class: A class declared with the abstract keyword, which cannot be instantiated directly.
- Abstract Method: A method declared without a body, using the abstract keyword. Must be implemented in subclasses.
- Concrete Method: A method with a body in the abstract class. Subclasses can inherit or override it.
- Subclass: A class that extends the abstract class and provides implementations for abstract methods.
Code Example:
Ly8gQWJzdHJhY3QgY2xhc3MKYWJzdHJhY3QgY2xhc3MgQW5pbWFsIHsKCiAgICAvLyBBYnN0cmFjdCBtZXRob2QKICAgIGFic3RyYWN0IHZvaWQgc291bmQoKTsKCiAgICAvLyBDb25jcmV0ZSBtZXRob2QKICAgIHZvaWQgc2xlZXAoKSB7CiAgICAgICAgU3lzdGVtLm91dC5wcmludGxuKCJTbGVlcGluZy4uLiIpOwogICAgfQp9CgovLyBDb25jcmV0ZSBzdWJjbGFzcyAxCmNsYXNzIERvZyBleHRlbmRzIEFuaW1hbCB7CgogICAgQE92ZXJyaWRlCiAgICB2b2lkIHNvdW5kKCkgewogICAgICAgIFN5c3RlbS5vdXQucHJpbnRsbigiRG9nIHNheXM6IFdvb2YgV29vZiEiKTsKICAgIH0KfQoKLy8gQ29uY3JldGUgc3ViY2xhc3MgMgpjbGFzcyBDYXQgZXh0ZW5kcyBBbmltYWwgewoKICAgIEBPdmVycmlkZQogICAgdm9pZCBzb3VuZCgpIHsKICAgICAgICBTeXN0ZW0ub3V0LnByaW50bG4oIkNhdCBzYXlzOiBNZW93ISIpOwogICAgfQp9CgpwdWJsaWMgY2xhc3MgTWFpbiB7CgogICAgcHVibGljIHN0YXRpYyB2b2lkIG1haW4oU3RyaW5nW10gYXJncykgewoKICAgICAgICAvLyBVc2luZyBwb2x5bW9ycGhpc20KICAgICAgICBBbmltYWwgbXlEb2cgPSBuZXcgRG9nKCk7CiAgICAgICAgQW5pbWFsIG15Q2F0ID0gbmV3IENhdCgpOwoKICAgICAgICAvLyBDYWxsIG1ldGhvZHMKICAgICAgICBteURvZy5zb3VuZCgpOyAgLy8gT3V0cHV0OiBEb2cgc2F5czogV29vZiBXb29mIQogICAgICAgIG15RG9nLnNsZWVwKCk7ICAvLyBPdXRwdXQ6IFNsZWVwaW5nLi4uCgogICAgICAgIG15Q2F0LnNvdW5kKCk7ICAvLyBPdXRwdXQ6IENhdCBzYXlzOiBNZW93IQogICAgICAgIG15Q2F0LnNsZWVwKCk7ICAvLyBPdXRwdXQ6IFNsZWVwaW5nLi4uCiAgICB9Cn0K
Output:
Dog says: Woof Woof!
Sleeping…
Cat says: Meow!
Sleeping...
Explanation:
In the above code example-
- We start with an abstract class Animal, which cannot be instantiated directly.
- Inside the Animal class, we declare an abstract method sound(). This method is not defined in the Animal class, meaning it must be implemented by any subclass of Animal.
- The sleep() method is a concrete method in the Animal class, meaning it has an implementation. This method simply prints "Sleeping..." when called.
- We then define two concrete subclasses of Animal: Dog and Cat. Each subclass provides its own implementation of the sound() method, making the sound() method specific to each animal.
- In the Dog class, the sound() method is overridden to print "Dog says: Woof Woof!".
- Similarly, in the Cat class, the sound() method is overridden to print "Cat says: Meow!".
- In the Main class, we use polymorphism by declaring myDog and myCat as Animal type variables, but instantiate them as Dog and Cat, respectively.
- When we call myDog.sound(), the Dog's version of sound() is invoked, outputting "Dog says: Woof Woof!".
- We also call myDog.sleep(), which uses the inherited sleep() method from Animal, printing "Sleeping...".
- Similarly, calling myCat.sound() invokes the Cat's version of sound(), outputting "Cat says: Meow!".
- Finally, myCat.sleep() calls the inherited sleep() method from Animal, printing "Sleeping...".
Advantages Of Abstract Keyword In Java
The abstract keyword is a powerful feature in Java that enables developers to define abstract classes and methods. Here are the key advantages:
- Facilitates Code Reusability: Abstract classes allow shared functionality (concrete methods) to be reused across subclasses, reducing code duplication. Subclasses can inherit and build upon the common methods provided by the abstract class.
- Enforces Consistency: Abstract methods enforce that all subclasses implement specific behaviors or functionality. It ensures a consistent interface across different implementations of the same base class.
- Enables Abstraction: Abstract classes help to focus on the essential features and behaviors of objects rather than implementation details. This promotes cleaner and more understandable code.
- Encourages Polymorphism: Abstract classes support polymorphism by allowing the use of a base class reference to point to different subclass objects. This helps in writing more flexible and extensible code.
- Bridges the Gap Between Interface and Concrete Class: Abstract classes can have both abstract methods (like an interface) and concrete methods (with implementation). This allows developers to define common functionality while still requiring subclasses to implement specific methods.
- Provides a Blueprint for Derived Classes: Abstract classes act as a blueprint, outlining the structure and behavior that subclasses must follow. This helps in designing systems with a clear hierarchy and defined responsibilities.
- Supports Partial Implementation: Unlike interfaces, abstract classes allow partial implementation by defining some methods as concrete while leaving others abstract. This strikes a balance between complete abstraction and full implementation.
Disadvantages Of Abstract Keyword In Java
While the abstract keyword offers significant advantages in structuring and designing code, it comes with some limitations and challenges. Here are the key disadvantages:
- Cannot Be Instantiated: Abstract classes cannot be instantiated directly, which might lead to additional overhead in creating subclasses, even for simple functionalities.
- Limited to Single Inheritance: Java allows only one class to be extended at a time, including abstract classes. This restricts flexibility when multiple inheritance is required.
- Overhead in Implementation: Subclasses must provide implementations for all abstract methods, which can lead to redundancy if multiple subclasses share similar implementations.
- Increased Complexity: Using abstract classes in a class hierarchy can make the design more complex and harder to understand, especially for large systems with multiple layers of abstraction.
- Reduced Flexibility Compared to Interfaces: With interfaces, a class can implement multiple behaviors by adhering to multiple contracts, but an abstract class limits a class to inherit only from one parent.
- May Lead to Code Duplication: Abstract classes can include shared code, but the lack of multiple inheritance may force developers to duplicate code across unrelated hierarchies.
- Less Adaptable to Changes: Changes in an abstract class may require modifications across all its subclasses, increasing the risk of introducing bugs or errors in the system.
- Partial Implementation May Be Misused: Abstract classes allow partial implementation, which can lead to inconsistent behavior if not carefully designed or documented.
Are you looking for someone to answer all your programming-related queries? Let's find the perfect mentor here.
Abstract Classes Vs. Interfaces In Java
When it comes to designing the structure of your Java application, both abstract classes and interfaces are powerful tools that serve distinct purposes. While abstract classes allow you to define a blueprint with shared functionality, interfaces provide a way to enforce a strict contract that multiple classes can adhere to. The key differences between these two are as follows:
Feature |
Abstract Class |
Interface |
Declaration |
Declared using the abstract keyword. |
Declared using the interface keyword. |
Methods |
Can have both abstract and concrete methods. |
Can have abstract methods (implicitly public and abstract) and default or static methods (from Java 8). |
Variables |
Can have instance variables (with access modifiers). |
Can only have constants (public static final by default). |
Multiple Inheritance |
A class can extend only one abstract class. |
A class can implement multiple interfaces. |
Access Modifiers for Methods |
Methods can have public, protected, or private access modifiers. |
All methods are public by default. |
Constructors |
Can have constructors. |
Cannot have constructors. |
Use Case |
Used when classes share common functionality and need some abstract behavior. |
Used to define a completely abstract contract for classes to implement. |
Speed of Development |
Supports partial implementation, allowing faster development. |
Requires all abstract methods to be implemented unless default methods are used. |
Inheritance |
Can inherit from a single class (abstract or not). |
Can inherit from multiple interfaces. |
Keyword for Usage |
All methods are public by default. |
Implementing classes use the implements keyword. |
Java Version Dependency |
Available since Java 1.0. |
Default and static methods added in Java 8. |
Therefore:
- Use abstract classes when you need partial implementation, shared state (instance variables), or constructors.
- Use interfaces when you want to enforce a strict contract for unrelated classes or enable multiple inheritance.
Real-World Applications Of Abstract Keyword
The abstract keyword is widely used in software development to create flexible, scalable, and maintainable systems. Here are some common real-world applications where it proves invaluable:
1. Framework and API Design
- Abstract classes are heavily utilized in frameworks (e.g., Java Swing, JavaFX) and APIs to define shared behaviors while leaving specific implementations to the developer.
- Example: The AbstractList class in the Java Collections Framework provides a skeletal implementation of the List interface.
2. Template Method Pattern
- Abstract classes are key in implementing the Template Method Design Pattern, where a base class provides a template of operations, and subclasses override specific steps.
- Example: A ReportGenerator abstract class could have an abstract generateReport() method, with subclasses defining specific report formats (PDF, Excel, etc.).
3. Defining Domain-Specific Blueprints
- Abstract classes act as blueprints for domain-specific objects, ensuring subclasses adhere to the business logic.
- Example: In a banking system, an Account abstract class could define methods like calculateInterest() and getAccountType().
4. Game Development
- Abstract classes are used to define base behaviors for game development objects, such as GameCharacter or Enemy, while allowing subclasses to specialize.
- Example: An abstract GameObject class with methods like render() and update() could be implemented differently for characters, obstacles, and projectiles.
5. Software Architecture Layers
- Abstract classes are used to create modular architectures by separating core logic from specific implementations.
- Example: In a service-oriented architecture, an abstract Service class can define common methods like start() and stop().
6. Polymorphic Behavior in Applications
- Abstract classes help implement polymorphism, allowing base class references to manage objects of various subclasses.
- Example: In a billing system, a Payment abstract class can represent different payment methods (Credit Card, PayPal, etc.).
7. Enhancing Security in Applications
- Abstract classes can encapsulate sensitive logic, ensuring that specific details are hidden while exposing necessary operations to subclasses.
- Example: A UserAuthentication abstract class might define methods like login() and logout(), ensuring consistent implementation across authentication types.
8. Plugin and Extension Systems
- Abstract classes provide the foundation for creating extensible systems where plugins or modules implement specific functionality.
- Example: A Plugin abstract class in an IDE or media player can define the structure for all plugins.
9. Test Automation Frameworks
- Abstract classes are used to define reusable testing logic while allowing customization for specific test cases.
- Example: An abstract TestCase class can include methods like setup() and teardown(), implemented by concrete test cases.
Conclusion
The abstract keyword in Java language is a cornerstone of object-oriented programming, enabling developers to design flexible and reusable code by emphasizing abstraction. It allows us to define blueprints for related classes through abstract classes and methods, enforcing structure while leaving room for specific implementations.
Whether used in frameworks, enterprise applications, or real-world systems like payment processing and game development, the abstract keyword fosters clean, modular, and maintainable code. While it has limitations, such as restricted inheritance and complexity in large systems, its advantages far outweigh these challenges when used thoughtfully. By mastering abstraction, developers can craft scalable and robust Java applications that adhere to sound design principles.
Frequently Asked Questions
Q. What is the purpose of the abstract keyword in Java?
The abstract keyword in Java is used to achieve abstraction, which is the process of hiding implementation details and showing only the essential features of an object. It is applied to:
- Abstract Classes: To define a class that cannot be instantiated directly and serves as a blueprint for subclasses.
- Abstract Methods: To declare methods that must be implemented by subclasses.
Q. Can we have a constructor in an abstract class?
Yes, an abstract class can have a constructor. While an abstract class itself cannot be instantiated, its constructor is called when a subclass object is created. This allows the abstract class to initialize common properties for its subclasses.
Q. What happens if a subclass does not implement all abstract methods of an abstract class?
If a subclass fails to implement all abstract methods of its parent abstract class, the subclass must itself be declared as abstract. Otherwise, the code will not compile because Java enforces the implementation of all abstract methods in a concrete subclass.
Q. How does an abstract class differ from an interface?
Abstract classes allow a mix of abstract and concrete methods and can have instance variables, constructors, and access modifiers for methods. In contrast, interfaces (pre-Java 8) could only contain abstract methods and constants. Starting with Java 8, interfaces can also include default and static methods, but they still cannot have constructors or instance variables.
Q. Can an abstract class extend another abstract class or implement an interface?
Yes, an abstract class can:
- Extend another abstract class: It inherits all the abstract and concrete methods of the parent abstract class.
- Implement an interface: It must provide implementations for the interface’s abstract methods or remain abstract itself.
Q. What are the limitations of using the abstract keyword?
Some of the key limitations include:
- Abstract classes cannot be instantiated directly.
- They are restricted to single inheritance, meaning a class can only extend one abstract class at a time.
- Subclasses are forced to implement all abstract methods, which might lead to redundancy if multiple subclasses require similar implementations.
- Overuse of abstraction can increase design complexity, making the system harder to understand and maintain.
With this, we come to an end of our discussion about the abstract keyword in Java. Here are a few other topics that you might be interested in reading:
- Convert String To Date In Java | 3 Different Ways With Examples
- Final, Finally & Finalize In Java | 15+ Differences With Examples
- Super Keyword In Java | Definition, Applications & More (+Examples)
- How To Find LCM Of Two Numbers In Java? Simplified With Examples
- How To Find GCD Of Two Numbers In Java? All Methods With Examples
- Volatile Keyword In Java | Syntax, Working, Uses & More (+Examples)
I’m a Computer Science graduate with a knack for creative ventures. Through content at Unstop, I am trying to simplify complex tech concepts and make them fun. When I’m not decoding tech jargon, you’ll find me indulging in great food and then burning it out at the gym.
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
Comments
Add comment