Difference Between JDK, JRE, And JVM | Comparison + Real Analogy
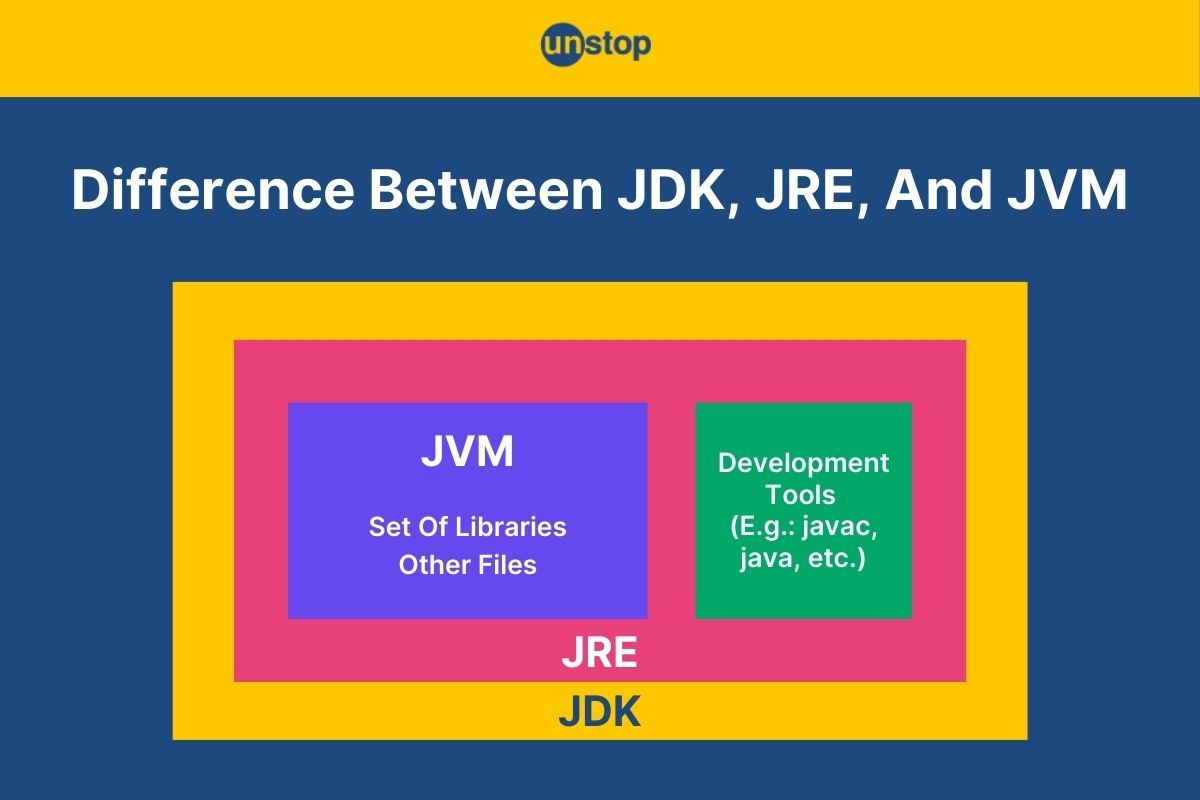
Java is one of the most popular programming languages in the world, known for its portability and "write once, run anywhere" promise. But how does it achieve that? Enter the dynamic trio of JDK, JRE, and JVM. These three essential components form the backbone of Java’s ecosystem, each playing a distinct role in Java development and execution.
In this article, we’ll break down the difference between JDK, JRE, and JVM, beginning with what they are, how they interconnect, and how each contributes to the seamless operation of Java applications.
What Is The Difference Between JDK, JRE, and JVM?
At first glance, JDK, JRE, and JVM might seem like interchangeable terms, but each one serves a distinct purpose in the Java ecosystem. To put it simply:
- JVM (Java Virtual Machine) is responsible for running Java bytecode. It’s the engine that executes your Java programs, regardless of the platform.
- JRE (Java Runtime Environment) is the package that includes JVM along with everything needed to run Java applications (such as libraries and supporting files). It’s like the environment where your Java program lives and runs.
- JDK (Java Development Kit) is the full toolkit for developing Java applications. It contains everything in the JRE, plus development tools like a compiler and debugger that are necessary to write, compile, and debug Java code.
While JDK provides the tools for development, JRE is more focused on running Java applications, and JVM is the engine that makes it all work. In the following sections, we’ll dive into the specific roles and components of each to clarify how they all interconnect.
What Is JVM (Java Virtual Machine)?
The Java Virtual Machine (JVM) is a virtual machine that runs Java bytecode, translating it into machine code that a specific system’s hardware can understand. This process enables Java applications to run on any platform that has a compatible JVM without modification to the source code. In short, JVM makes Java "platform-independent."
- It acts as an intermediary between Java bytecode and the system’s hardware.
- When a Java program is compiled, it’s converted into bytecode, which is a set of instructions that the JVM can interpret and execute.
- The JVM ensures that the same Java program can run on any machine, regardless of its operating system or architecture.
- This is a key feature of Java's "write once, run anywhere" slogan.
Key Components of JVM
JVM is not just a single entity but a system made up of several essential components:
- Class Loader: This component loads class files (compiled Java programs) into memory when the program is executed. It also verifies the bytecode for correctness.
- Bytecode Verifier: Ensures that the bytecode loaded by the Class Loader follows the Java language's security constraints.
- Execution Engine: This is the core part of JVM, which executes the bytecode. It does this by either interpreting the bytecode or using Just-In-Time (JIT) compilation to convert the bytecode into native machine code for faster execution.
- Garbage Collector: Manages memory by automatically deleting objects that are no longer in use, freeing up memory space for new objects.
Platform Independence: How JVM Makes It Happen
The JVM allows Java to be platform-independent because it abstracts the underlying system architecture. A program written in Java is compiled into bytecode that can be run on any JVM, regardless of the system it's running on. This means developers don’t have to worry about tailoring code to specific hardware or operating systems.
Imagine writing a book (your Java program) and printing copies of it (bytecode). The book doesn’t need to be rewritten for every reader (different operating systems); you just need to print it in a format (bytecode) that all readers (JVMs) can understand.
What Is JRE (Java Runtime Environment)?
The Java Runtime Environment (JRE) provides everything needed to run Java programs, acting as the link between the compiled Java bytecode and the underlying operating system.
- The JRE is a software package that creates an environment for Java applications to run.
- It includes the JVM (Java Virtual Machine), along with the class libraries and supporting files required to execute Java programs.
- In simple terms, if JVM is the engine, then JRE is the entire vehicle that ensures a smooth ride.
When you install Java on your computer, you’re usually installing the JRE. This allows you to run Java applications, but it doesn’t provide the tools for developing them. Developers, therefore, rely on the JDK, which we’ll discuss shortly.
Components of JRE
The JRE is made up of several critical components that work together to run Java applications:
- Java Virtual Machine (JVM): Executes Java bytecode and handles tasks like memory management and garbage collection.
- Class Libraries: A set of pre-written code libraries that Java programs can use. These include essential packages for input/output, networking, GUI development, and more.
- Deployment Technologies: Tools and APIs, such as Java Web Start and Java Plug-In, that help launch Java applications.
- Runtime Libraries: Provide common functionality that developers don’t need to implement from scratch, like file operations, data structures, and mathematical operations.
Use Case: JRE is perfect for users who just want to run Java programs. For instance:
- A college student running a Java-based project on their laptop.
- An enterprise application deployed on a server using Java technologies.
However, it lacks the development tools like a compiler or debugger, which are necessary for writing and building Java programs.
Want to expand your knowledge of Java programming? Check out this amazing course and become the Java developer of your dreams.
What Is JDK (Java Development Kit)?
The Java Development Kit (JDK) is the powerhouse toolkit for Java developers. It equips you with everything you need to create, compile, and debug Java applications, making it the foundation of Java development.
- It is a comprehensive software package that includes the tools and libraries needed for Java development.
- JDK contains the JRE, which allows you to run Java applications, along with additional tools like the Java compiler and debugger for building and testing your code.
Simply put, if JRE lets you enjoy the movie, the JDK is the studio where the movie is made.
Key Components of JDK
- JRE: Includes the JVM, class libraries, and other resources to run Java applications.
- Java Compiler (javac): Converts Java source code into bytecode that the JVM can execute.
- Debugger (jdb): Helps developers identify and fix errors in their code.
- Development Tools: Utilities like jar (for packaging Java applications) and javadoc (for generating documentation).
Use Case: The JDK is essential for anyone writing Java code, whether you’re a student learning Java basics or a professional building enterprise-grade applications.
Understanding The Difference Between JDK, JRE, And JVM
JDK, JRE, and JVM form a layered architecture, each fulfilling a specific role in the Java ecosystem. Let’s see where these layers lie and their role in the execution of Java programs.
- JVM (Java Virtual Machine): The Execution Engine
- At the core of the Java architecture, the JVM translates platform-independent Java bytecode into machine-specific instructions.
- It handles runtime tasks like memory management, garbage collection, and exception handling.
- JVM ensures Java’s “write once, run anywhere” promise by abstracting the underlying operating system.
- JRE (Java Runtime Environment): The Execution Platform
- Building on the JVM, the JRE provides the environment needed to run Java applications.
- It includes the JVM, essential libraries, and other runtime components like Java APIs.
- While the JVM handles execution, the JRE ensures the application has access to pre-built functionality (e.g., handling input/output operations or GUI elements).
- JDK (Java Development Kit): The Development Suite
- The JDK goes beyond the runtime capabilities of JRE by including tools to create, compile, and debug Java applications.
- It provides developers with javac (compiler) to convert source code into bytecode, and jdb (debugger) to troubleshoot applications.
- With the JDK, you get the full toolkit required to develop and test Java programs from scratch.
Hierarchical Relationship
- The JVM is the lowest level and is indispensable for running Java applications.
- The JRE builds on the JVM by packaging it with libraries and other tools needed for execution.
- The JDK is the most comprehensive, containing the JRE and adding development tools.
This interdependence highlights how each component contributes to Java’s lifecycle—from writing and compiling code to executing it across diverse environments.
Here is your chance to top the leaderboard while practicing your coding skills: Participate in the 100-Day Coding Sprint at Unstop.
Comparison Table For Difference Between JDK, JRE, And JVM
The table below provides a snapshot of the core differences between JDK, JRE, and JVM.
Feature |
JDK (Java Development Kit) |
JRE (Java Runtime Environment) |
JVM (Java Virtual Machine) |
Purpose |
To develop and run Java applications |
To run precompiled Java programs |
To execute Java bytecode into machine-specific code |
Includes |
JRE + development tools (compiler, debugger, etc.) |
JVM + libraries and runtime files |
Core engine for running Java programs |
Main Role |
Creation and testing of Java applications |
Execution of Java applications |
Platform-independent execution of bytecode |
Users |
Developers |
End users and application deployers |
N/A (a background component for both JDK and JRE) |
Development Tools |
Yes (e.g., javac, jdb, jar) |
No |
No |
Dependency |
Cannot exist without JRE and JVM |
Depends on JVM |
Standalone runtime engine |
Output |
Produces bytecode from source code |
Runs Java bytecode |
Translates bytecode into native machine code |
Let’s understand the difference between JDK, JRE, and JVM with the help of a real-world example.
The Coffee Shop– Let’s imagine Java is a coffee shop business, then:
- JVM is the coffee machine (execution). It processes the raw ingredients (bytecode) to produce the final coffee (executed program). It doesn’t care where the coffee beans (bytecode) come from as long as they meet the required specifications.
- JRE is the entire setup of the coffee shop (runtime), including the coffee machine (JVM), the cups, the tables, and the baristas (libraries and runtime). It’s everything needed to serve coffee to the customers (users).
- JDK is the warehouse and workshop (development + runtime). This is where you store coffee beans (source code), roasters (compiler), packaging materials (debugger, tools), and even an extra coffee machine for testing (JRE). It’s where the magic of creating new coffee blends (Java programs) happens.
Need more guidance on how to become a Java developer? You can now select an expert to be your mentor here.
Conclusion
Understanding the difference between JDK, JRE, and JVM is crucial for mastering Java and its ecosystem. These components work in harmony to create, run, and manage Java applications:
- The JVM is the heart of Java, ensuring programs run seamlessly across platforms by translating bytecode into machine-specific instructions. In short, JVM ensures portability.
- The JRE extends this functionality by providing a runtime environment complete with essential libraries and resources to execute Java programs. In short, JRE provides usability.
- The JDK, in turn, is a developer’s toolbox, combining the JRE with tools like the compiler and debugger to enable the creation of Java applications. In short, JDK empowers productivity.
By distinguishing their roles, you can appreciate Java’s efficiency and versatility, whether you're developing new applications or simply running existing ones.
Frequently Asked Questions
Q1. How do JVM, JRE, and JDK relate and work together in the Java development process?
JVM, JRE, and JDK form a hierarchical structure in the Java ecosystem:
- JVM is the core engine that executes Java bytecode.
- JRE packages the JVM with libraries and resources needed to run Java programs.
- JDK includes the JRE, along with tools like a compiler and debugger, enabling developers to create and test Java applications.
Together, they ensure seamless development and execution of Java programs.
Q2. Do I need both JDK and JRE?
Whether it is possible to work with just either JDK or JRE, or you need both depends on your role:
- If you're developing Java programs, you need the JDK as it includes the tools required to write, compile, and debug code.
- If you're only running Java applications, the JRE alone is sufficient. However, installing the JDK automatically provides the JRE.
Q3. Is JVM part of JRE or JDK?
The JVM is part of both:
- It resides within the JRE to execute Java bytecode.
- The JRE itself is included in the JDK, making the JVM indirectly a part of the JDK.
Think of it as the engine shared by both the runtime environment (JRE) and the development toolkit (JDK).
Q4. What are JDK, JRE, JVM, and JIT in Java?
- JDK (Java Development Kit): A toolkit for developing Java programs, including a compiler and debugger.
- JRE (Java Runtime Environment): Provides the environment and resources to run Java programs.
- JVM (Java Virtual Machine): Executes Java bytecode by converting it to machine-specific instructions.
- JIT (Just-In-Time Compiler): A component of the JVM that optimizes bytecode execution by compiling frequently used code into native machine code at runtime.
Q5. Can I install JDK without JRE?
No, the JDK comes bundled with the JRE. Installing the JDK provides you with both the development tools and the runtime environment. However, JRE can be installed separately if you only need to run Java programs.
Q6. Why is JVM considered platform-independent but not JRE?
The JVM executes bytecode in a platform-independent manner, as the bytecode is the same across all systems. However, the JRE includes platform-specific libraries and native resources tailored to the operating system, making it platform-dependent.
By now, you must have a clear view of the difference between JDK, JRE, and JVM. Here are some more topics you must explore:
- Operators In Java | 9 Types, Precedence & More (+ Code Examples)
- Java Keywords | List Of 54 Keywords With Description +Code Examples
- Variables In Java | Declare, Initialise & Types (+Code Examples)
- Identifiers In Java | Types, Conventions, Errors & More (+Examples)
- Data Types In Java | Primitive & Non-Primitive With Code Examples
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment