Java Programming Language
Table of content:
- History Of Java Programming Langauge
- Infographic For History Of Java
- What’s In The Name | History Of Java
- Key Features Of Java
- Advantages And Disadvantages Of Java
- The Version History Of Java Langauge
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is JDK?
- How To Download Java Development Kit (JDK) For Windows, MacOS, and Linux?
- Set Environment Variables In Java
- How To Install Java (JDK) On Windows 64-Bit Machine?
- How To Install Java (JDK) On Linux?
- How To Install Java (JDK) On macOS?
- How To Test Java Installation?
- How To Write Your First Java Program On Linux OS?
- Conclusion
- Frequently Asked Questions
Table of content:
- Java Programming Language | An Introduction
- 15 Key Features Of Java
- Write Once Run Anywhere (WORA) | Features Of Java
- Java Editions
- 5 New Features Of JAVA 8
- 5 New Features Of JAVA 11
- What Makes Java Popular?
- Conclusion
- Frequently Asked Questions
Table of content:
- What is Java?
- Advantages of Java
- Disadvantages of Java
Table of content:
- What Is Java Programming?
- Role Of Integrated Development Environments (IDEs) In Java Development
- 15 Best Java IDE For Developers
- In-Depth Comparison Table
- Conclusion
- Frequently Asked Questions
Table of content:
- Key Differences Between Java And Core Java
- What Is Java?
- What Is Core Java?
- Applications Of Java
- Applications Of Core Java
- When To Use Java?
- When To Use Core Java?
- Conclusion
- Frequently Asked Questions
Table of content:
- What Are Variables In Java Language?
- How To Declare Variables In Java Programs?
- How To Initialize Variables In Java?
- Naming Conventions For Variables In Java
- Types Of Variables In Java
- Local Variables In Java
- Instance Variables In Java
- Static Variables In Java
- Final Variables In Java
- Scope and Lifetime of Variables In Java
- Data Types Of Variables In Java (Primitive & Non-primitive)
- Java Variable Type Conversion & Type Casting
- Working With Variables In Java (Examples)
- Access Modifiers & Variables In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Are Identifiers In Java?
- Syntax Rules For Identifiers In Java
- Valid Identifiers in Java
- Invalid Identifiers in Java
- Java Reserved Keywords
- Naming Conventions & Best Practices For Identifiers In Java
- What Is An Identifier Expected Error In Java?
- Reasons The Identifier Expected Error Occurs
- How To Fix/ Resolve Identifier Expected Errors In Java?
- Conclusion
- Frequently Asked Questions
Table of content:
- What Are Data Types In Java?
- Primitive Data Types In Java
- Non-Primitive Data Types In Java
- Key Differences Between Primitive And Non-Primitive Data Types In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Are Operators In Java?
- Types Of Operators In Java
- Unary Operators In Java
- Arithmetic Operators In Java
- Assignment Operators In Java
- Relational Operators In Java
- Logical Operators In Java
- Bitwise Operators In Java
- Shift Operators In Java
- Increment & Decrement Operators In Java
- Ternary Operator In Java
- Instanceof Operator In Java
- Precedence & Associativity Of Java Operators
- Advantages & Disadvantages Of Operators In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is A Return Statement In Java?
- Use Cases Of Return Statements In Java
- Returning A Value From A Method In Java
- Returning A Class Object In Java
- Returning Void (No Value) In Java
- Advantages Of Using Return Statements In Java
- Limitations Of Using Return Statements In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Are Keywords In Java?
- List Of Keywords In Java
- Detailed Overview Of Java Keywords With Examples
- What If When Keywords In Java Are Used As Variable Names?
- Difference Between Identifiers & Keywords In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Abstract Keyword In Java?
- Use Of Abstract Keyword In Java
- Abstract Methods In Java
- Abstract Classes In Java
- Advantages Of Abstract Keyword In Java
- Disadvantages Of Abstract Keyword In Java
- Abstract Classes Vs. Interfaces In Java
- Real-World Applications Of Abstract Keyword
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is throws Keyword In Java?
- How Does The throws Keyword Work?
- Throwing A Checked Exception Using throws In Java
- Throwing Multiple Exceptions Using throws In Java
- Throwing A Custom Exception Using throws In Java
- When To Use The throws Keyword In Java
- Difference Between throw and throws Keyword In Java
- Best Practices For Using The throws Keyword In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is The Volatile Keyword In Java?
- How Does Volatile Keyword In Java Work?
- Using Volatile Keyword In Java To Control Thread Execution
- Using Volatile Keyword In Java To Signal Between Multiple Threads
- Difference Between Synchronization And Volatile Keyword
- Common Mistakes And Best Practices While Using Volatile Keyword In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding Super Keyword In Java
- Super Keyword In Java With Instance Variables
- Super Keyword In Java With Method Overriding
- Super Keyword In Java With Constructor Chaining
- Applications Of Super Keyword In Java
- Difference Between This And Super Keyword In Java
- Advantages Of Using Super Keyword In Java
- Limitations And Considerations Of Super Keyword In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding This Keyword In Java
- Uses Of This Keyword In Java
- Using This Keyword For Referencing Instance Variables
- Using This Keyword For Invoking A Constructor
- Using This Keyword For Invoking A Method
- Using This Keyword With Getters And Setters
- Difference Between This And Super Keyword In Java
- Best Practices For Using This Keyword In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is new Keyword In Java?
- Uses Of The new Keyword In Java
- Memory Management With new Keyword In Java
- Example 1: Creating An Object Of A Class Using new Keyword In Java
- Example 2: Creating An Array Using The new Keyword In Java
- Best Practices For Using new Keyword In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is The Transient Keyword In Java?
- Real-Life Example Of The Transient Keyword In Java
- When To Use The Transient Keyword In Java
- Example 1: Effect Of Transient Keyword On Serialization In Java
- Example 2: Skipping Sensitive Data During Serialization With Transient Keyword In Java
- Using Transient With Final Keyword In Java
- Using Transient With Static Keyword
- Difference Between Transient And Volatile Keyword In Java
- Advantages And Disadvantages Of Transient Keyword In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Static Keyword In Java?
- Characteristics Of Static Keyword In Java
- Static Variables In Java
- Static Method In Java
- Static Blocks In Java
- Static Classes In Java
- Static Variables Vs Instance Variables In Java
- Advantages Of Static Keyword In Java
- Disadvantages Of Static Keyword In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is A Static Method In Java?
- Use Cases Of Static Method In Java
- Using Static Method In Java To Create A Utility Class
- Using Static Method In Java To Implement The Singleton Design Pattern
- Difference Between Static And Instance Methods In Java
- Limitations Of Static Method In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding Final Keyword In Java
- Final Variables In Java
- Final Methods In Java
- Final Classes In Java
- Difference Between Static And Final Keyword In Java
- Uses Of Final Keyword In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Key Difference Between final, finally, And finalize In Java
- What Is final Keyword In Java?
- What Is finally Keyword In Java?
- What Is finalize Keyword In Java?
- When To Use Which Keyword In Java?
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding The extends Keyword In Java?
- Use Of extends Keyword In Java
- Using Java extends To Implement Single Inheritance
- Using Java extends With Interfaces (Default Methods)
- Overriding Using extends Keyword In Java
- Difference Between extends And implements In Java
- Real World Applications Of Extends Keyword In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Decision Making Statement In Java?
- If Statement In Java
- If-Else Statement In Java
- Else-If Ladder In Java
- Switch Statement In Java
- Ternary/Conditional Operator (?:) In Java
- Best Practices For Writing Decision Making Statements In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Are Control Statements in Java?
- Decision-Making Control Statements In Java
- Looping Control Statements In Java
- Jump (Branching) Control Statements In Java
- Application Of Control Statements In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is The Break Statement In Java?
- Working Of The Break Statement In Java
- Using Java Break Statement With Loops
- Using Java Break Statement With Switch Statement
- Using Java Break Statement With Infinite Loops
- Common Pitfalls While Using Break Statements In Java
- Best Practices For Using The Break Statement In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Switch Statement In Java?
- Working Of The Switch Statement In Java
- Example Of Switch Statement In Java
- Java Switch Statement With String
- Java Nested Switch Statements
- Java Enum In Switch Statement
- Java Wrapper Classes In Switch Statements
- Uses Of Switch Statement In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Syntax Of main() Method In Java
- public Specifier – Main Method In Java
- static Keyword – Main Method In Java
- void Return Type Of Main Method In Java
- The main Identifier – Main Method In Java
- String[] args In Main Method In Java
- The Role Of Java Virtual Machine (JVM)
- Running Java Programs Without The Main Method
- Variations In Declaration Of Main Method In Java
- Overloading The Main Method In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Method Overriding In Java?
- Example Of Method Overriding In Java
- Ideal Use Cases Of Method Overriding In Java
- Rules For Method Overriding In Java
- Super Keyword & Method Overriding In Java
- Constructor & Method Overriding In Java
- Exception Handling In Method Overriding In Java
- Access Modifiers In Method Overriding In Java
- Advantages & Disadvantages Of Method Overriding In Java
- Difference Between Method Overloading Vs. Method Overriding In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Method Overloading In Java?
- Different Ways Of Method Overloading In Java
- Overloading The main() Method In Java
- Type Promotion & Method Overloading In Java
- Null Error & Method Overloading In Java
- Advantages Of Method Overloading In Java
- Disadvantages Of Method Overloading In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Difference Between Overloading And Overriding In Java (Comparison Table)
- What Is Method Overloading In Java?
- What Is Method Overriding In Java?
- Key Differences Between Overloading & Overriding In Java Explained
- Difference Between Overloading And Overriding In Java Code Example
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is A One-Dimensional Array In Java?
- Key Characteristics Of One-Dimensional Arrays In Java
- Declaration Of One-Dimensional Array In Java
- Initialization Of One-Dimensional Array In Java
- Common Operations On One-Dimensional Array In Java
- Advantages Of One-Dimensional Arrays In Java
- Disadvantages Of One-Dimensional Arrays In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is A Multidimensional Array In Java?
- Difference Between Single-Dimensional And Multidimensional Arrays In Java
- Declaring Multidimensional Arrays In Java
- Initializing Multidimensional Arrays In Java
- Accessing And Manipulating Elements In Multidimensional Arrays In Java
- Working Of Multidimensional Arrays With Jagged Arrays In Java
- Why Use Multidimensional Arrays In Java?
- Limitations Of Multidimensional Arrays In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Are Jagged Arrays In Java?
- Comparison With Regular Multi-Dimensional Arrays
- Declaring Jagged Arrays In Java
- Initialization Of Jagged Arrays In Java
- Printing Elements Of A Jagged Array In Java
- Accessing And Modifying Elements Of A Jagged Array In Java
- Advantages Of Jagged Arrays In Java
- Disadvantages Of Jagged Arrays In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Array Of Objects In Java?
- Declare And Initialize An Array Of Object In Java
- Example Of An Array Of Objects In Java
- Sorting An Array Of Objects In Java
- Passing Arrays Of Objects To Methods In Java
- Returning Arrays Of Objects From Methods In Java
- Advantages Of Arrays Of Objects In Java
- Disadvantages Of Arrays Of Objects In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is A Dynamic Array In Java?
- Why Use Dynamic Array In Java?
- What Is The Size And Capacity Of A Dynamic Array In Java?
- How To Create A Dynamic Array In Java?
- Managing Dynamic Data Input In Java
- Storing And Processing Real-Time Data In Java
- Use Cases Of Dynamic Arrays In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Why Return An Array In Java?
- How To Return An Array In Java
- Example 1: Returning An Array Of First N Squares
- Example 2: Doubling the Values of an Array
- Common Scenarios For Returning Arrays In Java
- Points To Remember When Returning Arrays In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding ArrayList In Java
- Differences Between Arrays And ArrayList In Java
- Returning An ArrayList In Java
- Common Use Cases For Returning An ArrayList In Java
- Pitfalls To Avoid When Returning An ArrayList In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is A Thread In Java?
- Thread Vs Process
- What is a Thread Life Cycle In Java?
- What Are Thread Priorities?
- Creating Threads In Java
- Java Thread Methods
- Commonly Used Constructors In Thread Class
- Thread Synchronization In Java
- Common Challenges Faced While Using Threads In Java
- Best Practices For Using Threads In Java
- Real-World Applications Of Threads In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding Multithreading In Java
- Methods Of Multithreading In Java (Examples)
- Difference Between Multithreading And Multitasking In Java
- Handling Exceptions In Multithreading
- Best Practices For Multithreading In Java
- Real-World Use Cases of Multithreading In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Thread Priority In Java?
- Built-In Thread Priority Constants In Java
- Thread Priority: Setter & Getter Methods
- Limitations Of Thread Priority In Java
- Best Practices For Using Thread Priority In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Thread Synchronization In Java?
- The Need For Thread Synchronization In Java
- Types Of Thread Synchronization In Java
- Mutual Exclusion In Thread Synchronization In Java
- Coordination Synchronization (Thread Communication) In Java
- Advantages Of Thread Synchronization In Java
- Disadvantages Of Thread Synchronization In Java
- Alternatives To Synchronization In Java
- Deadlock And Thread Synchronization In Java
- Real-World Use Cases Of Thread Synchronization In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is A Daemon Thread In Java?
- User Threads Vs. Daemon Threads In Java
- Methods For Daemon Threads In The Thread Class
- Creating Daemon Threads In Java
- Checking The Daemon Status Of A Thread
- Exceptions In Daemon Threads
- Limitations Of Daemon Threads In Java
- Practical Applications Of Daemon Threads In Java
- Common Mistakes To Avoid When Working With Daemon Threads In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Why Do Threads Need To Communicate?
- Understanding Inter Thread Communication In Java
- The wait() Method In Inter-Thread Communication
- The notify() Method In Inter-Thread Communication
- The notifyAll() Method In Inter-Thread Communication
- Difference Between wait() And sleep() Methods In Java
- Best Practices For Inter Thread Communication In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding The Factorial Concept
- Approaches To Implementing Factorial In Java
- Find Factorial In Java Using Iterative Approach (Using a Loop)
- Find Factorial In Java Using Recursive Approach
- Complexity Analysis Of Factorial Programs In Java
- Applications Of Factorial Program In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding The Leap Year Concept
- Approach To Check A Leap Year In Java
- Alternative Approach To Check A Leap Year In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is The Difference Between JDK, JRE, and JVM?
- What Is JVM (Java Virtual Machine)?
- What Is JRE (Java Runtime Environment)?
- What Is JDK (Java Development Kit)?
- Understanding The Difference Between JDK, JRE, And JVM
- Comparison Table For Difference Between JDK, JRE, And JVM
- Conclusion
- Frequently Asked Questions
Table of content:
- Difference Between Abstraction And Encapsulation In Java
- Understanding Abstraction In Java
- Understanding Encapsulation In Java
- When To Use Abstraction And Encapsulation?
- Conclusion
- Frequently Asked Questions
Table of content:
- Differences Between Abstract Class And Interface In Java
- What Is An Abstract Class In Java?
- What Is An Interface In Java?
- When To Use An Abstract Class?
- When To Use Interface?
- Compatibility Between Abstract Class And Interface In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Error Vs. Exception In Java
- What Is Error In Java?
- What Is Exception In Java?
- Best Practices For Handling Exceptions In Java
- Why Errors Should Not Be Handled In Java?
- Conclusion
- Frequently Asked Questions
Table of content:
- Key Differences: Java Vs. JavaScript
- What Is Java?
- What Is JavaScript?
- Difference Between Java And JavaScript Explained
- Conclusion
- Frequently Asked Questions
Table of content:
- Brief Introduction To C++
- Brief Introduction To Java
- Difference Between C++ and Java
- Overview & Features Of C++ Language
- Overview & Features of Java Language
- Example of C++ and Java Program
- Key Difference Between C++ And Java Explained
- Similarities Between Java Vs. C++
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- Basic Java interview questions and answers
- Intermediate Java interview questions and answers
- Advanced Java interview questions and answers
Table of content:
- Difference between core Java and advanced Java
- Important Core Java Questions
- Tips for Preparing for Core Java
New Keyword In Java | Syntax, Uses And More (+Code Examples)
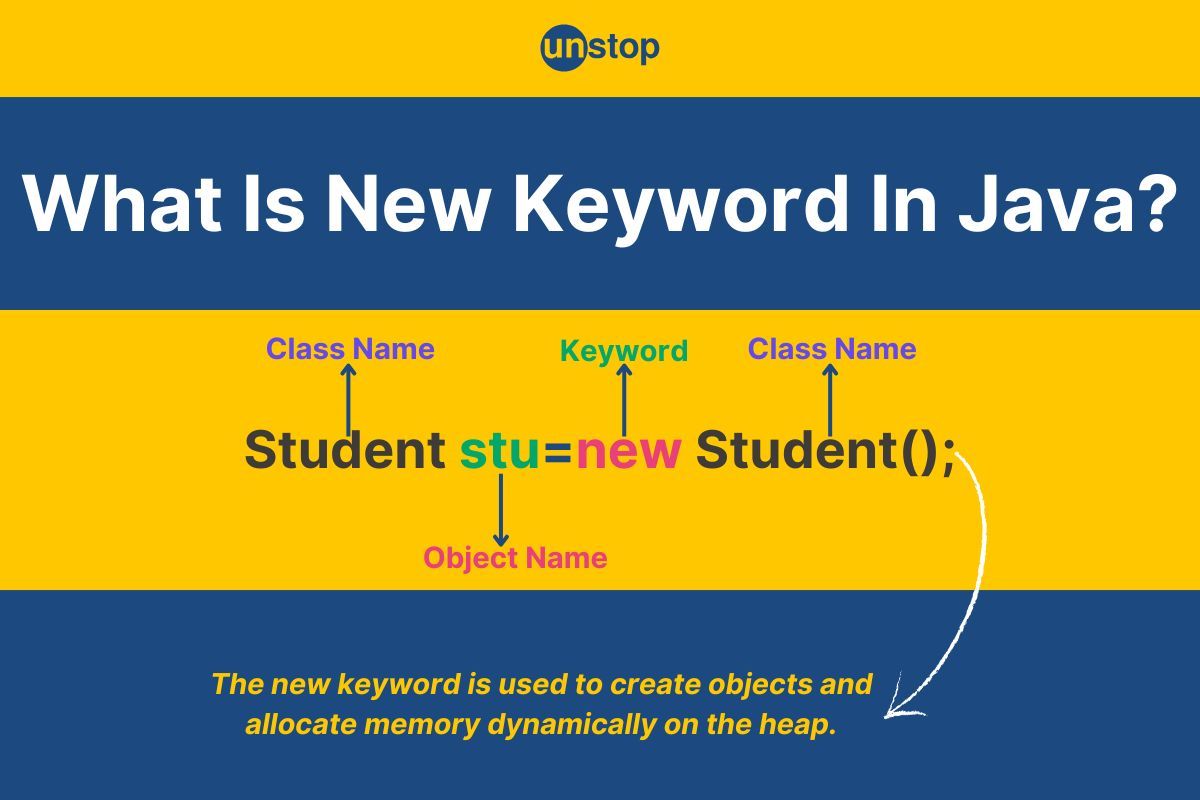
The new keyword in Java is a powerful tool that enables us to create new objects and allocate memory dynamically. It's the cornerstone of Java's object-oriented programming approach, allowing developers to instantiate classes and access their methods and properties. Without it, working with objects—one of the core aspects of Java—wouldn't be possible.
In this article, we'll explore the role of the new keyword in Java, how it works, and its significance in memory management and object creation. We'll also examine examples to demonstrate its use in real-world scenarios.
What Is new Keyword In Java?
The new keyword in Java programming is used to create objects and allocate memory dynamically during program execution. It is an integral part of Java's object-oriented design, allowing us to instantiate classes, initialize objects, and allocate memory on the heap.
When used, the new keyword calls the constructor of the class, which sets up the initial state of the object. Additionally, it can also be used to create arrays. This memory allocation is managed by Java's Garbage Collector, ensuring efficient memory usage by automatically reclaiming unused objects.
The new keyword is essential for object creation in Java, as it makes sure objects are properly initialized and ready for use.
Syntax Of new Keyword In Java
ClassName objectName = new ClassName(arguments);
Here:
- ClassName: Represents the type of object being created.
- objectName: A reference variable that stores the memory address of the newly created object.
- new: The Java keyword that allocates memory dynamically for the object.
- ClassName(arguments): A constructor call to initialize the object. The arguments provided depend on the constructor defined in the class.
Explore this amazing course and master all the key concepts of Java programming effortlessly!
Uses Of The new Keyword In Java
Below are the key uses of the new keyword in Java:
- Creating Objects: The primary use of the new keyword is to create objects of a class. It dynamically allocates memory for the object and initializes it.
MyClass obj = new MyClass(); // Creates an object of MyClass
- Invoking Constructors: When used, the new keyword calls the constructor of the class. It can invoke default or parameterized constructors.
MyClass obj = new MyClass(); // Invokes default constructor
MyClass obj2 = new MyClass(10); // Invokes parameterized constructor
- Allocating Memory for Arrays: The new keyword is used to allocate memory for arrays dynamically.
int[] numbers = new int[5]; // Allocates memory for an integer array of size 5
String[] names = new String[3]; // Allocates memory for a String array
- Creating Anonymous Objects: It is used to create objects without assigning them to a reference variable, often for one-time use.
new MyClass().someMethod(); // Calls someMethod on an anonymous object
- Instantiating Inner Classes: The new keyword is used to create instances of inner or nested classes.
OuterClass.InnerClass obj = new OuterClass().new InnerClass();
- Dynamic Class Loading via Reflection: The newInstance() method in the Reflection API uses the new keyword internally to create objects dynamically.
Class<?> cls = Class.forName("MyClass");
Object obj = cls.getDeclaredConstructor().newInstance(); // Uses `new` internally
- Creating Wrapper Objects: The new keyword is used to create objects of wrapper classes for primitive types (although autoboxing is preferred in modern Java versions).
Integer num = new Integer(10); // Deprecated in Java 9 but still valid
- Implementing Custom Data Structures: Java developers can use the new keyword to instantiate objects required for data structures like linked lists, trees, and graphs.
Node node = new Node(5); // Node of a linked list or tree
- Allocating Memory for Multidimensional Arrays: It is used for creating multidimensional arrays dynamically.
int[][] matrix = new int[3][3]; // 2D array
- With Polymorphism: The new keyword helps in creating objects of derived classes while storing references in a base class type, supporting polymorphism.
Parent obj = new Child(); // Polymorphic behavior
Memory Management With new Keyword In Java
The new keyword plays a crucial role in Java's memory management by dynamically allocating memory for objects and arrays on the heap memory. Java ensures efficient use of memory through its automatic Garbage Collection (GC) mechanism, which deallocates memory used by objects that are no longer referenced.
How Memory Management Works With The new Keyword
- Heap Memory Allocation: When we use the new keyword, Java allocates memory for the object or array on the heap. This memory includes space for the object’s fields (variables) and metadata (like the class of the object).
- Object Initialization: The new keyword invokes the class’s constructor to initialize the object with the required values or default settings.
- Reference Variables: A reference variable stores the address of the object in memory, allowing us to interact with the object. If the reference variable is reassigned or goes out of scope, the object becomes eligible for garbage collection.
- Garbage Collection: Objects created using the new keyword are managed by Java's Garbage Collector. When an object is no longer reachable by any reference, the Garbage Collector automatically deallocates its memory, freeing it for future use.
- Efficient Memory Use: Java's memory management, combined with the new keyword, ensures that memory is allocated only as needed and reclaimed when objects are no longer used.
Code Example:
class Car {
String model;
Car(String model) {
this.model = model; // Constructor initializes the object
}
}
public class Main {
public static void main(String[] args) {
Car car1 = new Car("Sedan"); // Memory allocated for car1
Car car2 = new Car("SUV"); // Memory allocated for car2
car1 = null; // car1 reference removed, object is now eligible for garbage collection
// car2 still points to the "SUV" object, which is not garbage collected
}
}
Output:
Since the code does not include any System.out.println statements (except the constructor initialization), it produces no visible output when run.
Explanation:
In the above code example-
- We define a class named Car that represents a blueprint for creating Car objects.
- Inside the Car class, we declare an instance variable model of type String to store the car's model name.
- We create a constructor Car(String model) that takes a model name as a parameter and assigns it to the model variable using this.model = model. This ensures that each Car object is initialized with a specific model name when created.
- In the main method of the Main class, we first create a Car object named car1 and initialize it with the model "Sedan". This allocates memory for the car1 object and assigns it a reference.
- Similarly, we create another Car object named car2 and initialize it with the model "SUV". Now car2 references this new object in memory.
- We set car1 to null, which removes the reference to the "Sedan" object. The "Sedan" object is no longer accessible, making it eligible for garbage collection by the Java runtime.
- Meanwhile, car2 still references the "SUV" object, so it remains accessible and is not garbage collected.
Example 1: Creating An Object Of A Class Using new Keyword In Java
In this example, we create an object of a class Person using the new keyword, and initialize it with a constructor.
Code Example:
class Person {
String name;
int age;
// Constructor
Person(String name, int age) {
this.name = name;
this.age = age;
}
// Method to display information
void display() {
System.out.println("Name: " + name);
System.out.println("Age: " + age);
}
}
public class Main {
public static void main(String[] args) {
// Creating a new object using the 'new' keyword
Person person1 = new Person("Alia", 25);
// Displaying the information
person1.display();
}
}
Output:
Name: Alia
Age: 25
Explanation:
In the above code example-
- We define a class called Person, which represents an individual with two attributes: name (a String) and age (an int).
- Inside the Person class, we define a constructor Person(String name, int age), which takes two parameters—name and age. This constructor initializes the instance variables this.name and this.age with the provided values, ensuring that each Person object has a specific name and age.
- We also define a method display() in the Person class, which prints the name and age of the Person object to the console.
- In the main method of the Main class, we create a new Person object called person1 using the new keyword. This initializes the object with the name "Alia" and age 25.
- Finally, we call the display() method on the person1 object, which prints Alia's name and age to the console.
Sharpen your coding skills with Unstop's 100-Day Coding Sprint and compete now for a top spot on the leaderboard!
Example 2: Creating An Array Using The new Keyword In Java
In this example, we create an array of integers using the new keyword and initialize its elements.
Code Example:
public class Main {
public static void main(String[] args) {
// Creating an array of integers using the 'new' keyword
int[] numbers = new int[5];
// Initializing the array elements
numbers[0] = 10;
numbers[1] = 20;
numbers[2] = 30;
numbers[3] = 40;
numbers[4] = 50;
// Displaying the array elements
System.out.println("Array elements:");
for (int num : numbers) {
System.out.println(num);
}
}
}
Output:
Array elements:
10
20
30
40
50
Explanation:
In the above code example-
- We begin by defining the Main class, where the execution of the program starts in the main method.
- Inside the main method, we create an array of integers called numbers using the new keyword. This array has a size of 5, meaning it can hold 5 integer elements. Initially, all elements in the array are set to 0.
- We then initialize the array elements by assigning values to each index in the array:
- numbers[0] = 10;
- numbers[1] = 20;
- numbers[2] = 30;
- numbers[3] = 40;
- numbers[4] = 50;
- After assigning values to all the elements, we print a message "Array elements:" to indicate that we are about to display the array's contents.
- We then use a for-each loop to iterate over the elements of the numbers array. For each element (referred to as num), we print its value using System.out.println(num);. This will display each integer in the array on a new line.
Best Practices For Using new Keyword In Java
The new keyword plays a central role in Java programming, especially when creating objects and managing memory dynamically. To use it effectively, it is essential to follow best practices that ensure code efficiency, clarity, and proper memory management.
- Initialize Objects Immediately: Always initialize reference variables when they are declared to avoid NullPointerException.
- Use Constructor Overloading Appropriately: Select the appropriate constructor for the specific use case to avoid unnecessary object creation.
- Avoid Overusing Anonymous Objects: Use anonymous objects only when you don't need to reuse the object later.
- Prefer Factory Methods Over Direct Instantiation: Use factory methods or design patterns like Singleton instead of directly using new for better maintainability and flexibility.
- Use Autoboxing for Wrapper Classes: Take advantage of autoboxing to avoid manually using new with wrapper classes, which reduces memory overhead.
- Avoid Memory Leaks by Nullifying References: Explicitly nullify references to objects when they are no longer needed, helping garbage collection to reclaim memory.
- Use Reflection with Caution: When using reflection, handle exceptions properly and avoid overusing it to prevent runtime errors.
- Use new Only When Necessary: Avoid creating objects unnecessarily, especially when they are only needed temporarily.
- Leverage new for Array Creation: Use new for dynamically creating arrays with a size determined at runtime.
- Be Careful with Inner Class Instantiation: Ensure that when instantiating inner classes, the correct syntax is used and the outer class instance is properly referenced.
Are you looking for someone to answer all your programming-related queries? Let's find the perfect mentor here.
Conclusion
The new keyword in Java is a vital tool for object creation and memory management. By dynamically allocating memory on the heap, it enables us to instantiate objects and initialize them using constructors. Whether we are working with classes or arrays, the new keyword ensures that objects are properly initialized and ready for use. Additionally, Java's automatic memory management system, particularly through Garbage Collection, helps reclaim memory occupied by objects that are no longer needed.
Understanding how the new keyword works in Java empowers developers to write efficient, object-oriented code while relying on Java's memory management to handle cleanup tasks. Overall, the new keyword is indispensable for creating objects and managing memory in Java applications.
Frequently Asked Questions
Q. What does the new keyword do in Java?
The new keyword in Java is used to create objects and allocate memory dynamically. When the new keyword is used, it performs two actions:
- Memory Allocation: It allocates memory for the object on the heap, where all objects in Java are stored.
- Object Initialization: It invokes the constructor of the class, initializing the object's fields with the values provided (if any).
For example, when we create an object of a class using the new keyword, the memory for the object is reserved on the heap, and the constructor is called to set the initial state of the object.
Q. Can we use the new keyword for primitive data types in Java?
No, we cannot use the new keyword with primitive data types in Java. Primitive data types such as int, char, float, etc., are directly stored in stack memory, and they are not objects. The new keyword is only used for creating instances of classes (objects) and arrays. For example:
int num = 10; // No 'new' keyword needed for primitive types
In contrast, for arrays and objects, the new keyword is required to allocate memory dynamically.
Q. How does Garbage Collection work with objects created using the new keyword in Java?
In Java, objects created with the new keyword are stored in the heap memory. Once an object is no longer referenced, it becomes eligible for Garbage Collection. Java’s Garbage Collector automatically identifies objects that are no longer reachable from any active part of the program and reclaims the memory they occupy.
For example, if we assign null to an object reference or the reference goes out of scope, the object can be garbage collected, and its memory is freed for reuse. The new keyword does not directly handle memory deallocation; that responsibility lies with the Garbage Collector.
Person person = new Person("John"); // object created
person = null; // object becomes eligible for garbage collection
Q. What is the difference between using new keyword for creating an object and creating an array in Java?
While the new keyword is used for both creating objects and arrays, the way memory is allocated differs slightly:
- Objects: When an object is created using the new keyword, memory is allocated on the heap for the instance variables defined in the class.
- Arrays: When an array is created with the new keyword, memory is allocated for the entire array, and the array elements are initialized to their default values (e.g., 0 for int, null for objects).
For example:
Person person = new Person("Alice"); // Creates an object
int[] numbers = new int[5]; // Creates an array of integers with 5 elements, all initialized to 0
Q. Can we create an array of objects using the new keyword?
Yes, we can create an array of objects using the new keyword in Java. When we create an array of objects, the array itself is created first, and then each element of the array can reference an object created using the new keyword.
For example:
Person[] people = new Person[3]; // Creates an array of 3 Person objects (null references initially)
people[0] = new Person("Alia"); // Allocates memory for the first Person object
people[1] = new Person("Bhaskar"); // Allocates memory for the second Person object
people[2] = new Person("Chandra"); // Allocates memory for the third Person object
In this case, the array is created first, and then the individual objects are instantiated within that array using the new keyword.
With this, we conclude our discussion on the new keyword in Java. Here are a few other topics that you might be interested in reading:
- Convert String To Date In Java | 3 Different Ways With Examples
- Final, Finally & Finalize In Java | 15+ Differences With Examples
- Super Keyword In Java | Definition, Applications & More (+Examples)
- How To Find LCM Of Two Numbers In Java? Simplified With Examples
- How To Find GCD Of Two Numbers In Java? All Methods With Examples
- Volatile Keyword In Java | Syntax, Working, Uses & More (+Examples)
I’m a Computer Science graduate with a knack for creative ventures. Through content at Unstop, I am trying to simplify complex tech concepts and make them fun. When I’m not decoding tech jargon, you’ll find me indulging in great food and then burning it out at the gym.
Comments
Add commentLogin to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
Esuru Pooja.C 2 hours ago
Ankita Das 2 days ago
Sunita Bhat 5 days ago
SIVANI Immidi 1 week ago
Mihir Kumar Ranjan 1 week ago