Table of content:
- What Is Static Keyword In Java?
- Characteristics Of Static Keyword In Java
- Static Variables In Java
- Static Method In Java
- Static Blocks In Java
- Static Classes In Java
- Static Variables Vs Instance Variables In Java
- Advantages Of Static Keyword In Java
- Disadvantages Of Static Keyword In Java
- Conclusion
- Frequently Asked Questions
Static Keyword In Java | Types, Characteristics & More (+Examples)
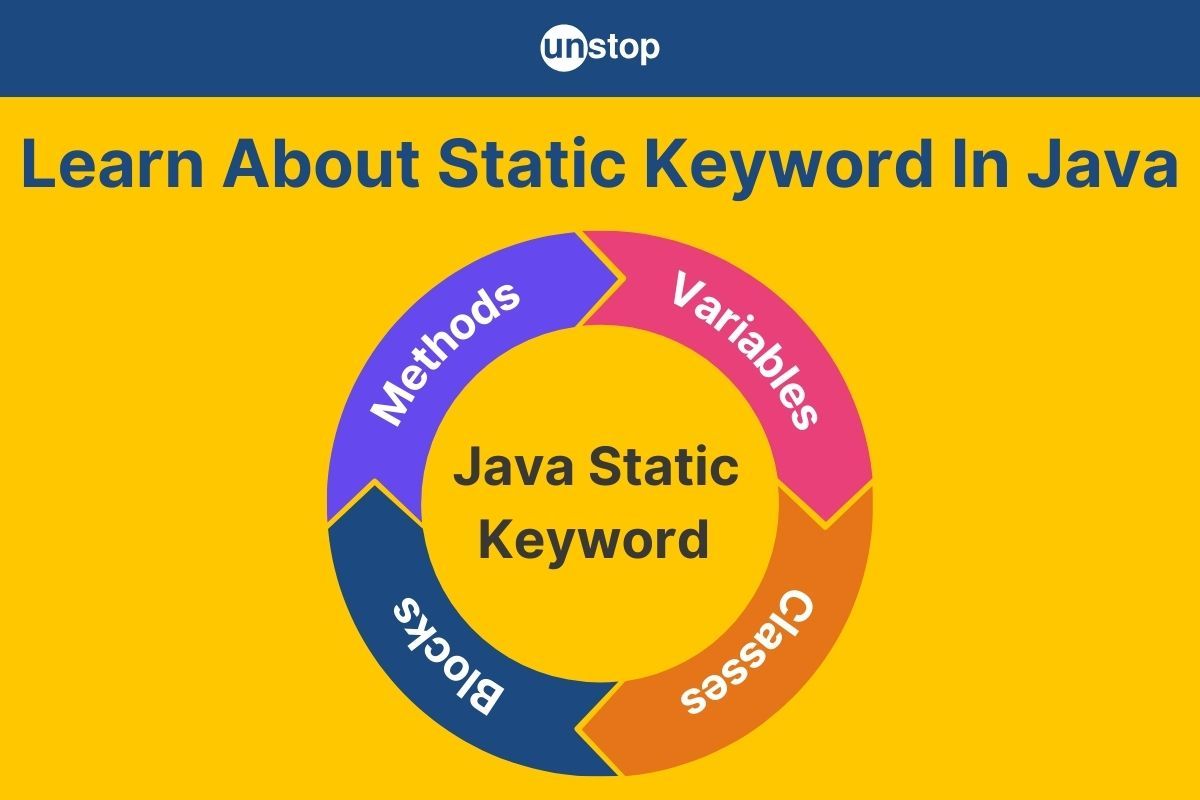
The static keyword in Java is a fundamental concept that plays a key role in managing memory and controlling the behavior of variables, methods, and inner classes. When a member (variable or method) is declared as static, it belongs to the class rather than to instances of the class. This means that a static member is shared across all instances of the class, making it useful for scenarios where we need a single shared value or method.
In this article, we will explore the different uses of the static keyword, its impact on memory management, and practical examples to demonstrate its functionality.
What Is Static Keyword In Java?
The static keyword in Java programming is used to indicate that a particular field, method, or block belongs to the class itself, rather than to instances of the class. This means that the static member is shared among all instances of the class.
Static can be of four types in Java:
- Static Variables: A static variable is shared by all objects of the class. It is initialized only once, at the start, and retains its value across all instances.
- Static Methods: A static method can be called without creating an instance of the class. It can access only static variables and static methods in the class.
- Static Blocks: A static block is used to initialize static variables. It runs once when the class is loaded into memory.
- Static Classes: A nested static class is associated with the outer class itself, not with instances of the outer class.
Characteristics Of Static Keyword In Java
The static keyword in Java has several important characteristics that define its behavior and usage. Here are the key characteristics of the static keyword:
1. Belongs to the Class, Not Instances
- Class-level access: Static members (variables, methods, or blocks) belong to the class itself rather than to any particular instance of the class.
- This means all instances of the class share the same static members.
2. Shared Among All Instances
- Common resource: A static variable is shared among all objects of the class. This means that if one object changes the value of a static variable, the change is reflected across all other instances of the class.
- For example, a static counter in a class would count the total number of instances created, and this count is the same for all objects of that class.
3. Memory Allocation
- One-time memory allocation: Static variables are allocated memory only once, when the class is loaded into memory. This makes them memory-efficient when compared to instance variables, which are created every time an object is instantiated.
4. Access Without Instantiation
- Direct access: Static methods and variables can be accessed without creating an instance of the class. This is useful when the functionality does not depend on instance-specific data but rather on class-level data.
- For example: You can call a static method like Math.sqrt() directly without creating an object of the Math class.
5. Static Methods
- No access to instance members: Static methods cannot access non-static instance variables or methods directly. They can only access other static members of the class.
- Calling convention: Static methods are typically called using the class name (e.g., ClassName.methodName()), although they can also be called using an object reference.
6. Static Blocks
- Initialization: Static blocks are used to initialize static variables. They are executed only once, when the class is first loaded into memory.
- Syntax:
static {
// Static initialization block
}
7. Static Nested Classes
- Independent of outer class instances: A static nested class can exist without an instance of the outer class. It can only access static members of the outer class.
- Usage: Static nested classes are often used for logical grouping of classes in cases where the nested class does not require access to the instance variables or methods of the outer class.
8. Can Be Used with Final Variables
- Constants: Static final variables are often used to define constants. Since the value of a static final variable cannot be changed after initialization, they are typically written in uppercase letters.
- For Example:
class MyClass {
static final int MAX_SIZE = 100;
}
9. Can Be Inherited
- Inherited by subclasses: Static variables and methods are inherited by subclasses, but they are not overridden by instance methods in the subclass. The subclass inherits the static method, but it cannot override it in the same way as instance methods.
10. Performance Improvement
- Efficiency: Static methods can be called without creating an instance, which can lead to performance improvements in certain situations. Since static members are loaded into memory once and accessed by all instances, it saves memory and improves access speed compared to instance-level members.
Explore this amazing course and master all the key concepts of Java programming effortlessly!
Static Variables In Java
Static variables in Java are variables declared with the static keyword. They are also known as class variables because they belong to the class rather than any specific instance of the class. This means that the value of a static variable is shared among all instances of the class, making it a common resource.
Syntax Of Static Variables In Java
static data_type variable_name;
Here:
- static Keyword: Indicates that the variable is shared and belongs to the class.
- Data Type: Specifies the type of the variable (e.g., int, float, String).
- Variable Name: The name used to reference the static variable.
Characteristics Of Static Variables In Java
- Shared Resource: The variable is shared across all instances of the class.
- Memory Allocation: Allocated memory only once, during class loading.
- Default Values: Static variables are initialized to their default values if not explicitly initialized (e.g., 0 for integers, null for objects).
- Class-level Access: Can be accessed directly using the class name (e.g., ClassName.variableName).
Uses Of Static Variables In Java
- Common Counters: To keep track of values like the total number of objects created.
- Constants: In combination with the final keyword, static variables are used to define constants.
- Shared Data: Useful for sharing data across all instances of a class.
- Global Configuration: To store configuration values or settings that are consistent for the application.
Code Example:
Y2xhc3MgU3RhdGljRXhhbXBsZSB7CgogICAgLy8gU3RhdGljIHZhcmlhYmxlIHRvIGNvdW50IHRoZSBudW1iZXIgb2Ygb2JqZWN0cyBjcmVhdGVkCiAgICBzdGF0aWMgaW50IG9iamVjdENvdW50ID0gMDsKCiAgICAvLyBJbnN0YW5jZSB2YXJpYWJsZQogICAgU3RyaW5nIG5hbWU7CgogICAgLy8gQ29uc3RydWN0b3IKICAgIFN0YXRpY0V4YW1wbGUoU3RyaW5nIG5hbWUpIHsKICAgICAgICB0aGlzLm5hbWUgPSBuYW1lOwogICAgICAgIG9iamVjdENvdW50Kys7IC8vIEluY3JlbWVudCBzdGF0aWMgdmFyaWFibGUKICAgIH0KCiAgICAvLyBTdGF0aWMgbWV0aG9kIHRvIGRpc3BsYXkgdGhlIG9iamVjdCBjb3VudAogICAgc3RhdGljIHZvaWQgZGlzcGxheUNvdW50KCkgewogICAgICAgIFN5c3RlbS5vdXQucHJpbnRsbigiVG90YWwgb2JqZWN0cyBjcmVhdGVkOiAiICsgb2JqZWN0Q291bnQpOwogICAgfQoKICAgIC8vIEluc3RhbmNlIG1ldGhvZCB0byBkaXNwbGF5IGluc3RhbmNlIGRldGFpbHMKICAgIHZvaWQgZGlzcGxheURldGFpbHMoKSB7CiAgICAgICAgU3lzdGVtLm91dC5wcmludGxuKCJOYW1lOiAiICsgbmFtZSk7CiAgICB9Cn0KCnB1YmxpYyBjbGFzcyBNYWluIHsKICAgIHB1YmxpYyBzdGF0aWMgdm9pZCBtYWluKFN0cmluZ1tdIGFyZ3MpIHsKICAgICAgICAvLyBDcmVhdGUgb2JqZWN0cwogICAgICAgIFN0YXRpY0V4YW1wbGUgb2JqMSA9IG5ldyBTdGF0aWNFeGFtcGxlKCJPYmplY3QxIik7CiAgICAgICAgU3RhdGljRXhhbXBsZSBvYmoyID0gbmV3IFN0YXRpY0V4YW1wbGUoIk9iamVjdDIiKTsKCiAgICAgICAgLy8gRGlzcGxheSBvYmplY3QgZGV0YWlscwogICAgICAgIG9iajEuZGlzcGxheURldGFpbHMoKTsKICAgICAgICBvYmoyLmRpc3BsYXlEZXRhaWxzKCk7CgogICAgICAgIC8vIEFjY2VzcyBzdGF0aWMgdmFyaWFibGUgYW5kIG1ldGhvZAogICAgICAgIFN0YXRpY0V4YW1wbGUuZGlzcGxheUNvdW50KCk7CiAgICB9Cn0K
Output:
Name: Object1
Name: Object2
Total objects created: 2
Explanation:
In the above code example-
- We start by defining a class StaticExample. Inside it, we declare a static variable objectCount initialized to 0. This variable is shared among all objects of the class and tracks the number of objects created.
- We also define an instance variable name to store a unique name for each object. Since it is not static, each object will have its own copy of this variable.
- The constructor StaticExample(String name) is designed to initialize the name variable with the given parameter and increment the objectCount static variable by 1. This ensures that each time an object is created, the count of total objects increases.
- A static method displayCount() is defined to print the total number of objects created using the objectCount variable. Being static, this method can be called without creating an instance of the class.
- The instance method displayDetails() prints the name of the specific object. It operates on the instance variable name and is unique to each object.
- In the Main class, we define the main() method, where the execution begins. We create two objects, obj1 and obj2, of the StaticExample class, passing "Object1" and "Object2" as their names, respectively.
- Each time we create an object, the constructor runs, assigning the name value to the respective object and incrementing the objectCount static variable.
- We use obj1.displayDetails() and obj2.displayDetails() to print the name of each object, demonstrating the use of the instance method and how each object maintains its own name.
- Finally, we call StaticExample.displayCount() to access the static method and print the total number of objects created. Since objectCount is static, it reflects the shared value across all objects, showing the correct total of 2 objects.
Static Method In Java
A static method in Java is a method declared with the static keyword. Unlike instance methods, a static method belongs to the class itself rather than any specific instance. It can be called without creating an object of the class and is primarily used when the behavior does not depend on instance-specific data.
Syntax Of Static Methods In Java
static return_type method_name(parameters) {
// Method body
}
Here:
- static Keyword: Indicates that the method belongs to the class and not to instances of the class.
- Return Type: Specifies the type of value the method will return (e.g., void, int, String).
- Method Name: The name used to call the method.
- Parameters (optional): Inputs that the method accepts (e.g., int a, int b).
- Method Body: Contains the logic or operations performed by the method.
Characteristics Of Static Methods In Java
- Class-level Access: Can be called using the class name without creating an object.
- Cannot Access Non-static Members: Static methods can only access static variables or other static methods directly.
- Direct Invocation: Often used for utility or helper functions like Math.sqrt() or Arrays.sort().
- Cannot Use this or super: Since static methods are not tied to any instance, they cannot use instance-specific keywords like this or super.
Uses Of Static Methods In Java
- Utility Methods: For common operations like calculations, formatting, or conversions.
- Factory Methods: To create objects in a controlled manner.
- Accessing Static Variables: To operate on or retrieve static variables.
- Entry Points: The main method is static, as it serves as the entry point for Java programs.
Code Example:
Y2xhc3MgU3RhdGljTWV0aG9kRXhhbXBsZSB7CgogICAgLy8gU3RhdGljIHZhcmlhYmxlCiAgICBzdGF0aWMgaW50IGNvdW50ID0gMDsKCiAgICAvLyBTdGF0aWMgbWV0aG9kIHRvIGRpc3BsYXkgY291bnQKICAgIHN0YXRpYyB2b2lkIGRpc3BsYXlDb3VudCgpIHsKICAgICAgICBTeXN0ZW0ub3V0LnByaW50bG4oIkN1cnJlbnQgY291bnQ6ICIgKyBjb3VudCk7CiAgICB9CgogICAgLy8gSW5zdGFuY2UgbWV0aG9kCiAgICB2b2lkIGluY3JlbWVudENvdW50KCkgewogICAgICAgIGNvdW50Kys7IC8vIEluY3JlbWVudCBzdGF0aWMgdmFyaWFibGUKICAgIH0KfQoKcHVibGljIGNsYXNzIE1haW4gewogICAgcHVibGljIHN0YXRpYyB2b2lkIG1haW4oU3RyaW5nW10gYXJncykgewogICAgICAgIC8vIENhbGwgc3RhdGljIG1ldGhvZCBkaXJlY3RseSB1c2luZyB0aGUgY2xhc3MgbmFtZQogICAgICAgIFN0YXRpY01ldGhvZEV4YW1wbGUuZGlzcGxheUNvdW50KCk7CgogICAgICAgIC8vIENyZWF0ZSBvYmplY3RzCiAgICAgICAgU3RhdGljTWV0aG9kRXhhbXBsZSBvYmoxID0gbmV3IFN0YXRpY01ldGhvZEV4YW1wbGUoKTsKICAgICAgICBTdGF0aWNNZXRob2RFeGFtcGxlIG9iajIgPSBuZXcgU3RhdGljTWV0aG9kRXhhbXBsZSgpOwoKICAgICAgICAvLyBJbmNyZW1lbnQgY291bnQgdXNpbmcgaW5zdGFuY2UgbWV0aG9kcwogICAgICAgIG9iajEuaW5jcmVtZW50Q291bnQoKTsKICAgICAgICBvYmoyLmluY3JlbWVudENvdW50KCk7CgogICAgICAgIC8vIENhbGwgc3RhdGljIG1ldGhvZCBhZ2FpbiB0byBkaXNwbGF5IHVwZGF0ZWQgY291bnQKICAgICAgICBTdGF0aWNNZXRob2RFeGFtcGxlLmRpc3BsYXlDb3VudCgpOwogICAgfQp9Cg==
Output:
Current count: 0
Current count: 2
Explanation:
In the above code example-
- We begin by defining a class StaticMethodExample, where a static variable count is declared and initialized to 0. This static variable is shared among all instances of the class and keeps track of a common count.
- A static method displayCount() is defined to print the current value of the count variable. Being static, this method can be called without creating an instance of the class, making it accessible through the class name.
- The instance method incrementCount() increments the static variable count. Even though this method is non-static and tied to specific objects, it modifies the shared static variable, affecting its value globally.
- In the Main class, the main() method begins execution. First, we call the static method StaticMethodExample.displayCount() directly using the class name, which prints the initial value of count as 0.
- Next, we create two objects, obj1 and obj2, of the StaticMethodExample class. These objects are independent, but they share access to the same static variable count.
- Using obj1.incrementCount() and obj2.incrementCount(), we invoke the instance method to increment the static variable. Each call to this method increases the shared count value by 1, making its new value 2 after both increments.
- We call StaticMethodExample.displayCount() again to display the updated value of count. Since it is a static variable, the method reflects the cumulative changes made by both objects, showing the updated value as 2.
Static Blocks In Java
Static blocks in Java are code blocks declared with the static keyword. They are executed when the class is loaded into memory, before the execution of the main method or any other methods. Static blocks are mainly used for initializing static variables or executing logic that needs to be performed only once for the entire class.
Syntax Of Static Blocks In Java
static {
// Code to initialize static variables or perform operations
}
Characteristics Of Static Blocks In Java
- Executed Once: Static blocks run only once when the class is loaded into memory by the JVM.
- Initialization of Static Members: Often used to initialize static variables with more complex logic than a simple assignment.
- Run Before Any Method: Static blocks are executed before the main method or any constructor.
- Multiple Static Blocks: A class can have multiple static blocks, which are executed in the order they appear in the class.
- No Return Value: Unlike methods, static blocks do not return any value and cannot be called directly.
Uses Of Static Blocks In Java
- Static Variable Initialization: To initialize static variables with non-trivial logic.
- Static Resource Loading: Load external resources like files, configurations, or database connections when the class is loaded.
- Logging or Debugging: Print information during class loading for debugging purposes.
- Class-level Preprocessing: Perform operations that should only be executed once, such as caching or registering services.
Code Example:
Y2xhc3MgU3RhdGljQmxvY2tFeGFtcGxlIHsKCiAgICAvLyBTdGF0aWMgdmFyaWFibGUKICAgIHN0YXRpYyBpbnQgdmFsdWU7CgogICAgLy8gU3RhdGljIGJsb2NrIGZvciBpbml0aWFsaXphdGlvbgogICAgc3RhdGljIHsKICAgICAgICBTeXN0ZW0ub3V0LnByaW50bG4oIlN0YXRpYyBibG9jayBpcyBleGVjdXRlZC4iKTsKICAgICAgICB2YWx1ZSA9IDQyOyAvLyBJbml0aWFsaXppbmcgc3RhdGljIHZhcmlhYmxlCiAgICB9CgogICAgLy8gU3RhdGljIG1ldGhvZCB0byBhY2Nlc3MgdGhlIHN0YXRpYyB2YXJpYWJsZQogICAgc3RhdGljIHZvaWQgZGlzcGxheVZhbHVlKCkgewogICAgICAgIFN5c3RlbS5vdXQucHJpbnRsbigiVmFsdWU6ICIgKyB2YWx1ZSk7CiAgICB9Cn0KCnB1YmxpYyBjbGFzcyBNYWluIHsKICAgIHB1YmxpYyBzdGF0aWMgdm9pZCBtYWluKFN0cmluZ1tdIGFyZ3MpIHsKICAgICAgICBTeXN0ZW0ub3V0LnByaW50bG4oIk1haW4gbWV0aG9kIHN0YXJ0cy4iKTsKCiAgICAgICAgLy8gQWNjZXNzaW5nIHN0YXRpYyBtZXRob2QKICAgICAgICBTdGF0aWNCbG9ja0V4YW1wbGUuZGlzcGxheVZhbHVlKCk7CiAgICB9Cn0K
Output:
Main method starts.
Static block is executed.
Value: 42
Explanation:
In the above code example-
- We define a class StaticBlockExample that contains a static variable value. This variable is shared among all instances of the class and will hold a common value.
- A static block is included in the class. The static block is executed automatically when the class is loaded into memory, even before any objects are created or methods are called. It is used here to initialize the static variable value.
- Inside the static block, we print a message "Static block is executed." to indicate when the block is executed. We then assign the value 42 to the static variable value.
- A static method displayValue() is defined to print the value of the static variable value. Since the method is static, we can call it without creating an instance of the class.
- In the Main class, the main() method begins execution. The first line in the method prints "Main method starts." to indicate the start of the program.
- We then call the static method StaticBlockExample.displayValue() directly using the class name. This method prints the value of the static variable value, which was initialized to 42 by the static block.
- The static block ensures that the variable value is initialized before any static methods are called or any objects are created. This guarantees proper setup of shared resources during class loading.
Static Classes In Java
In Java, static classes are nested classes (also known as inner classes) that are declared with the static keyword. These classes are associated with the enclosing class rather than any instance of the enclosing class. Static nested classes have access to the static members of their outer class, but they cannot directly access the non-static members (unless through an instance of the outer class).
Syntax Of Static Classes In Java
class OuterClass {
static class StaticNestedClass {
// Methods and fields of the static nested class
}
}
Here:
- Outer Class: The enclosing class that contains the static nested class.
- Static Nested Class: Declared with the static keyword inside the outer class.
- Methods and Fields: Static nested classes can have their own fields and methods, including static ones.
- Access Scope: Can access the static members of the outer class but not non-static members directly.
Characteristics Of Static Classes In Java
- Independent of Outer Class Instance: A static nested class does not need an instance of the outer class to be instantiated.
- Access to Static Members: Static nested classes can directly access the static variables and methods of the enclosing class.
- Encapsulation: Static nested classes are used to logically group classes that are only used by the enclosing class.
- Memory Efficiency: Static nested classes reduce memory overhead because they do not retain a reference to the outer class's instance.
Uses Of Static Classes In Java
- Logical Grouping: To group related classes together for better code organization.
- Utility Classes: When a nested class is used only for providing utility methods or logic relevant to the outer class.
- Avoiding Unnecessary Outer Class References: To avoid holding references to the outer class when it’s not needed.
Code Example:
Y2xhc3MgT3V0ZXJDbGFzcyB7CiAgICAvLyBTdGF0aWMgdmFyaWFibGUgaW4gdGhlIG91dGVyIGNsYXNzCiAgICBzdGF0aWMgaW50IG91dGVyU3RhdGljVmFyID0gMTA7CgogICAgLy8gU3RhdGljIG5lc3RlZCBjbGFzcwogICAgc3RhdGljIGNsYXNzIFN0YXRpY05lc3RlZENsYXNzIHsKICAgICAgICB2b2lkIGRpc3BsYXkoKSB7CiAgICAgICAgICAgIFN5c3RlbS5vdXQucHJpbnRsbigiQWNjZXNzaW5nIHN0YXRpYyB2YXJpYWJsZSBvZiBvdXRlciBjbGFzczogIiArIG91dGVyU3RhdGljVmFyKTsKICAgICAgICB9CiAgICB9Cn0KCnB1YmxpYyBjbGFzcyBNYWluIHsKICAgIHB1YmxpYyBzdGF0aWMgdm9pZCBtYWluKFN0cmluZ1tdIGFyZ3MpIHsKICAgICAgICAvLyBDcmVhdGUgYW4gb2JqZWN0IG9mIHRoZSBzdGF0aWMgbmVzdGVkIGNsYXNzCiAgICAgICAgT3V0ZXJDbGFzcy5TdGF0aWNOZXN0ZWRDbGFzcyBuZXN0ZWRPYmogPSBuZXcgT3V0ZXJDbGFzcy5TdGF0aWNOZXN0ZWRDbGFzcygpOwoKICAgICAgICAvLyBDYWxsIG1ldGhvZCBvZiB0aGUgc3RhdGljIG5lc3RlZCBjbGFzcwogICAgICAgIG5lc3RlZE9iai5kaXNwbGF5KCk7CiAgICB9Cn0K
Output:
Accessing static variable of outer class: 10
Explanation:
In the above code example-
- We start by defining a class OuterClass that includes a static variable outerStaticVar initialized to 10. This variable is shared among all instances of the class and is accessible without creating an object.
- Inside the OuterClass, we define a static nested class called StaticNestedClass. Because it is static, this nested class does not depend on an instance of the OuterClass for its creation.
- The StaticNestedClass contains an instance method display(). In this method, we access the static variable outerStaticVar of the enclosing OuterClass and print its value. Since the nested class is static, it can directly access static members of the outer class.
- In the Main class, we start execution in the main() method. To use the StaticNestedClass, we create an object of it using the syntax OuterClass.StaticNestedClass. This demonstrates how we can instantiate a static nested class without needing an instance of the enclosing class.
- After creating the object nestedObj, we call its display() method. This method accesses and prints the value of the static variable outerStaticVar from the OuterClass, displaying "Accessing static variable of outer class: 10".
Sharpen your coding skills with Unstop's 100-Day Coding Sprint and compete now for a top spot on the leaderboard!
Static Variables Vs Instance Variables In Java
Static variables are shared across all instances of a class, while instance variables are unique to each object. Understanding the differences between them is essential for efficient memory management and designing well-structured Java programs.
Here’s a detailed comparison table explaining the key differences between static variables and instance variables in Java:
Aspect |
Static Variables |
Instance Variables |
Definition |
A variable declared with the static keyword, belonging to the class rather than to any instance. |
A variable that is defined inside a class but outside any method, constructor, or block, and belongs to a specific instance of the class. |
Memory Allocation |
Allocated memory once, when the class is loaded. It is shared among all instances of the class. |
Memory is allocated each time a new object (instance) of the class is created. |
Access |
Can be accessed using the class name or through an instance (though class name is preferred). |
Must be accessed through an instance of the class. |
Default Value |
Static variables are initialized to default values (e.g., 0 for integers, null for objects, etc.) when the class is loaded. |
Instance variables are also initialized to default values when an object is created, but these values can vary depending on the type of the variable. |
Scope |
Has class-level scope; shared by all instances of the class. |
Has instance-level scope; each instance of the class gets its own copy of the instance variable. |
Lifetime |
The lifetime of static variables is tied to the class, so they exist as long as the class is loaded in memory. |
The lifetime of instance variables is tied to the lifecycle of the object they belong to. They are destroyed when the object is garbage collected. |
Accessing through Object |
Static variables can be accessed via objects, but it's generally recommended to access them through the class name. |
Instance variables must be accessed through an instance of the class. |
Usage |
Used for values or properties that should be shared across all instances of the class (e.g., counters, constants). |
Used for values or properties that are specific to each instance (e.g., object attributes). |
Initialization |
Static variables are initialized only once when the class is loaded, regardless of how many instances of the class are created. |
Instance variables are initialized each time an instance of the class is created. |
Modification |
Any modification to a static variable affects all instances of the class, since it is shared. |
Modifying an instance variable only affects the specific object it belongs to. |
Example |
class Counter { |
class Person { |
Thread Safety |
Static variables may pose a risk in multi-threaded applications, as they are shared among all instances. Synchronization may be needed for thread safety. |
Instance variables are independent for each object, so there is less risk of thread interference for each individual object. |
Therefore:
- Static variables are class-level variables that are shared among all instances, and their value remains consistent across instances.
- Instance variables are object-level variables that hold data unique to each instance of the class.
- Static variables should be used when we need a common property across all instances, while instance variables are best for properties unique to each object.
Advantages Of Static Keyword In Java
The static keyword in Java provides several advantages that enhance the efficiency, maintainability, and clarity of code. Here are the key advantages:
1. Memory Efficiency
- Shared Memory: Static variables are shared across all instances of a class, meaning only one copy is created and maintained in memory.
- Reduced Overhead: Saves memory compared to creating separate copies for each instance.
2. Global Access
- Accessible Without Objects: Static members (methods and variables) can be accessed directly using the class name, reducing the need to create objects.
- Simplifies Usage: Useful for constants and utility functions that don’t depend on object state.
3. Easy Initialization
- Static Blocks: Allow one-time initialization of static variables or resources when the class is loaded into memory.
4. Logical Grouping
- Static Nested Classes: Enable grouping helper classes or related functionality logically within the outer class without creating unnecessary dependencies on the outer class's instance.
5. Code Reusability
- Utility Classes: Static methods in utility classes (like Math and Collections) can be reused without the need for instantiation.
6. Improved Performance
- Direct Access: Static members are resolved at compile time (for methods) and class-level loading (for variables), which can be faster compared to instance-based access.
7. Consistency
- Shared State: Static variables ensure a consistent value shared across all objects, which is ideal for counters, configuration constants, or maintaining state globally.
8. Encapsulation of Related Data
- Static Constants: Static fields marked as final are used for constants, ensuring they remain encapsulated at the class level and cannot be modified.
9. Simplifies Implementation of Singleton Pattern
- The static keyword is commonly used to implement the Singleton Design Pattern, where a static variable holds the single instance of the class.
For Example:
class Singleton {
private static Singleton instance;private Singleton() { }
public static Singleton getInstance() {
if (instance == null) {
instance = new Singleton();
}
return instance;
}
}
10. Facilitates Static Import
- Static Import: Allows importing static members from other classes to simplify syntax and avoid repetitive class references.
11. Better Organization
- Static Blocks and Classes: Helps organize code logically by grouping related functionality, which improves readability and maintainability.
Are you looking for someone to answer all your programming-related queries? Let's find the perfect mentor here.
Disadvantages Of Static Keyword In Java
While the static keyword in Java offers several advantages, it also comes with some disadvantages that developers should be mindful of. Here are the key disadvantages of using static in Java:
1. Lack of Flexibility
- No Polymorphism: Static methods cannot be overridden in subclasses, limiting polymorphic behavior. They are bound at compile time rather than runtime, reducing flexibility in object-oriented design. For Example-
class Parent {
static void display() {
System.out.println("Parent display");
}
}class Child extends Parent {
// Cannot override static method, will hide the method instead
static void display() {
System.out.println("Child display");
}
}
2. Difficulty in Unit Testing
- Global State: Static variables maintain global state across the application, which can make unit testing difficult. Shared state across different parts of the application can lead to unintended side effects.
- Example: If a static variable is modified in one test, it may affect other tests.
3. Limited Object-Oriented Design
- Reduced Encapsulation: Static members (variables and methods) are tied to the class rather than individual objects. This breaks the concept of encapsulation, as static variables are shared by all instances of a class.
- Example: A static variable holds the same value for all instances, which can be problematic when each object needs its own unique state.
4. Can Cause Tight Coupling
- Dependency on Static Members: Overuse of static members (especially static methods) can lead to tight coupling between classes, as classes will depend on each other at the class level rather than through instance-level interactions.
- Example: Static methods can make it difficult to change the behavior of a class since the class is hardcoded into other classes through static references.
5. Hard to Manage Resources
- Static Variables Persist in Memory: Static variables exist as long as the class is loaded, which can lead to potential memory leaks if static references are not properly managed, especially when dealing with large applications.
- Example: Static objects might hold resources (like database connections or large data structures) that aren’t released until the program exits, potentially causing resource management issues.
6. Static Members Cannot Access Instance Members
- Limited Interaction: Static methods cannot access instance variables or instance methods directly. They can only interact with other static members of the class, which limits their usefulness in certain scenarios. For Example-
class Example {
int instanceVar = 10;
static void staticMethod() {
// Cannot access instanceVar directly
// System.out.println(instanceVar); // Error
}
}
7. Inconsistent Behavior with Inheritance
- Static Methods and Inheritance: Static methods are not inherited in the traditional sense. Instead, they are hidden if a subclass declares a static method with the same name, leading to potentially confusing behavior for developers.
8. Makes Code Less Modular
- Code Reusability Issues: Static methods and variables, because of their global nature, can be harder to reuse in different contexts without potentially altering the class's global state.
- Example: A static method that modifies a shared static variable may introduce bugs when used in multiple contexts, reducing modularity and reusability.
9. Increases Complexity in Multi-threaded Applications
- Thread Safety: Since static variables are shared among all instances, they can introduce synchronization issues in multi-threaded applications. If two or more threads modify the same static variable concurrently, it may lead to inconsistent or unexpected results. For Example-
class Counter {
static int count = 0;
public void increment() {
count++; // Potential issue in multi-threaded environments
}
}
10. Overuse Leads to Poor Design
- Anti-Pattern: Overusing the static keyword can lead to poor design decisions, such as using static variables to maintain state across instances or relying too heavily on static methods for behavior. This violates object-oriented principles like encapsulation, inheritance, and polymorphism.
- Example: Relying on static variables instead of passing data through object instances.
Conclusion
The static keyword in Java language is a powerful feature that allows developers to define class-level members, providing shared access to variables, methods, and even nested classes. Its primary advantages include memory efficiency, global access, and the ability to create utility classes and constants. However, like all powerful tools, it must be used carefully. Overuse of static members can lead to tight coupling, difficulties in testing, and complications in managing resources, especially in multi-threaded environments.
By understanding both the advantages and disadvantages of the static keyword, Java developers can make informed decisions about when and how to use it. It is most beneficial for situations where class-level access is required, such as utility methods, constants, or managing shared state. With thoughtful application, the static keyword can significantly improve the design, efficiency, and clarity of Java programs.
Frequently Asked Questions
Q. When should we use static methods in Java?
Static methods should be used in the following scenarios:
- Utility Methods: When the method does not depend on object state and performs a task that is related to the class, such as mathematical calculations or string manipulations (e.g., Math.sqrt(), Collections.sort()).
- Factory Methods: When you need to create and return instances of a class, particularly in patterns like Singleton or Factory.
- Access to Static Data: When the method needs to operate on static variables of the class.
- Initialization: Static methods can be used for initialization purposes in static blocks or static initialization methods.
Q. Can we access static methods without creating an object in Java?
Yes, static methods can be accessed directly using the class name, without needing to instantiate an object. This is one of the key advantages of static methods. Static methods belong to the class itself rather than to any particular instance, which allows them to be called without creating an object. For Example-
class MyClass {
static void myMethod() {
System.out.println("Static method called");
}
}public class Test {
public static void main(String[] args) {
MyClass.myMethod(); // Accessing static method directly via class name
}
}
Q. Can static methods access instance variables and methods in Java?
No, static methods cannot directly access instance variables or instance methods. Since static methods are tied to the class and not any specific instance, they don’t have access to the instance-level data. They can only interact with other static variables or static methods. For Example-
class MyClass {
int instanceVar = 10;
static int staticVar = 20;static void staticMethod() {
// System.out.println(instanceVar); // Error: Cannot access instance variable in static method
System.out.println(staticVar); // Valid: Static variable can be accessed
}
}
Q. What is the purpose of static blocks in Java?
A static block in Java is used for initialization of static variables or performing one-time setup operations when a class is loaded. The static block is executed only once, when the class is first loaded into memory. It is useful for tasks like initializing complex static variables or performing one-time operations that are necessary for the class.
Q. What are the drawbacks of using the static keyword in Java?
Some potential drawbacks of using the static keyword in Java include:
- Reduced Flexibility: Static methods cannot be overridden, and static variables are shared across all instances of the class, which can make code harder to maintain.
- Testing Challenges: Static members are tightly coupled to the class, which can make unit testing difficult as they introduce global state that is hard to isolate.
- Resource Management: Static variables remain in memory for the lifetime of the program, which can lead to memory leaks if not managed properly.
- Tight Coupling: Overuse of static methods and variables can create dependencies between classes, making the system harder to modify and extend.
Q. What is a static inner class in Java? How is it different from a non-static inner class?
A static inner class is a nested class that is declared with the static keyword. Unlike non-static inner classes, static inner classes do not have access to the instance variables or methods of the outer class. They can only access static members of the outer class.
Key Differences:
- Static Inner Class: Can be instantiated without an instance of the outer class and can only access static members of the outer class.
- Non-static Inner Class: Requires an instance of the outer class to be instantiated and can access both static and instance members of the outer class.
With this, we can conclude our discussion on static keywords in Java. Here are a few other topics that you might be interested in reading:
- Convert String To Date In Java | 3 Different Ways With Examples
- Final, Finally & Finalize In Java | 15+ Differences With Examples
- Super Keyword In Java | Definition, Applications & More (+Examples)
- How To Find LCM Of Two Numbers In Java? Simplified With Examples
- How To Find GCD Of Two Numbers In Java? All Methods With Examples
- Volatile Keyword In Java | Syntax, Working, Uses & More (+Examples)
I’m a Computer Science graduate with a knack for creative ventures. Through content at Unstop, I am trying to simplify complex tech concepts and make them fun. When I’m not decoding tech jargon, you’ll find me indulging in great food and then burning it out at the gym.
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
Comments
Add comment