Java Programming Language
Table of content:
- History Of Java Programming Langauge
- Infographic For History Of Java
- What’s In The Name | History Of Java
- Key Features Of Java
- Advantages And Disadvantages Of Java
- The Version History Of Java Langauge
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is JDK?
- How To Download Java Development Kit (JDK) For Windows, MacOS, and Linux?
- Set Environment Variables In Java
- How To Install Java (JDK) On Windows 64-Bit Machine?
- How To Install Java (JDK) On Linux?
- How To Install Java (JDK) On macOS?
- How To Test Java Installation?
- How To Write Your First Java Program On Linux OS?
- Conclusion
- Frequently Asked Questions
Table of content:
- Java Programming Language | An Introduction
- 15 Key Features Of Java
- Write Once Run Anywhere (WORA) | Features Of Java
- Java Editions
- 5 New Features Of JAVA 8
- 5 New Features Of JAVA 11
- What Makes Java Popular?
- Conclusion
- Frequently Asked Questions
Table of content:
- What is Java?
- Advantages of Java
- Disadvantages of Java
Table of content:
- What Is Java Programming?
- Role Of Integrated Development Environments (IDEs) In Java Development
- 15 Best Java IDE For Developers
- In-Depth Comparison Table
- Conclusion
- Frequently Asked Questions
Table of content:
- Key Differences Between Java And Core Java
- What Is Java?
- What Is Core Java?
- Applications Of Java
- Applications Of Core Java
- When To Use Java?
- When To Use Core Java?
- Conclusion
- Frequently Asked Questions
Table of content:
- What Are Variables In Java Language?
- How To Declare Variables In Java Programs?
- How To Initialize Variables In Java?
- Naming Conventions For Variables In Java
- Types Of Variables In Java
- Local Variables In Java
- Instance Variables In Java
- Static Variables In Java
- Final Variables In Java
- Scope and Lifetime of Variables In Java
- Data Types Of Variables In Java (Primitive & Non-primitive)
- Java Variable Type Conversion & Type Casting
- Working With Variables In Java (Examples)
- Access Modifiers & Variables In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Are Identifiers In Java?
- Syntax Rules For Identifiers In Java
- Valid Identifiers in Java
- Invalid Identifiers in Java
- Java Reserved Keywords
- Naming Conventions & Best Practices For Identifiers In Java
- What Is An Identifier Expected Error In Java?
- Reasons The Identifier Expected Error Occurs
- How To Fix/ Resolve Identifier Expected Errors In Java?
- Conclusion
- Frequently Asked Questions
Table of content:
- What Are Data Types In Java?
- Primitive Data Types In Java
- Non-Primitive Data Types In Java
- Key Differences Between Primitive And Non-Primitive Data Types In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Are Operators In Java?
- Types Of Operators In Java
- Unary Operators In Java
- Arithmetic Operators In Java
- Assignment Operators In Java
- Relational Operators In Java
- Logical Operators In Java
- Bitwise Operators In Java
- Shift Operators In Java
- Increment & Decrement Operators In Java
- Ternary Operator In Java
- Instanceof Operator In Java
- Precedence & Associativity Of Java Operators
- Advantages & Disadvantages Of Operators In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is A Return Statement In Java?
- Use Cases Of Return Statements In Java
- Returning A Value From A Method In Java
- Returning A Class Object In Java
- Returning Void (No Value) In Java
- Advantages Of Using Return Statements In Java
- Limitations Of Using Return Statements In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Are Keywords In Java?
- List Of Keywords In Java
- Detailed Overview Of Java Keywords With Examples
- What If When Keywords In Java Are Used As Variable Names?
- Difference Between Identifiers & Keywords In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Abstract Keyword In Java?
- Use Of Abstract Keyword In Java
- Abstract Methods In Java
- Abstract Classes In Java
- Advantages Of Abstract Keyword In Java
- Disadvantages Of Abstract Keyword In Java
- Abstract Classes Vs. Interfaces In Java
- Real-World Applications Of Abstract Keyword
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is throws Keyword In Java?
- How Does The throws Keyword Work?
- Throwing A Checked Exception Using throws In Java
- Throwing Multiple Exceptions Using throws In Java
- Throwing A Custom Exception Using throws In Java
- When To Use The throws Keyword In Java
- Difference Between throw and throws Keyword In Java
- Best Practices For Using The throws Keyword In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is The Volatile Keyword In Java?
- How Does Volatile Keyword In Java Work?
- Using Volatile Keyword In Java To Control Thread Execution
- Using Volatile Keyword In Java To Signal Between Multiple Threads
- Difference Between Synchronization And Volatile Keyword
- Common Mistakes And Best Practices While Using Volatile Keyword In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding Super Keyword In Java
- Super Keyword In Java With Instance Variables
- Super Keyword In Java With Method Overriding
- Super Keyword In Java With Constructor Chaining
- Applications Of Super Keyword In Java
- Difference Between This And Super Keyword In Java
- Advantages Of Using Super Keyword In Java
- Limitations And Considerations Of Super Keyword In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding This Keyword In Java
- Uses Of This Keyword In Java
- Using This Keyword For Referencing Instance Variables
- Using This Keyword For Invoking A Constructor
- Using This Keyword For Invoking A Method
- Using This Keyword With Getters And Setters
- Difference Between This And Super Keyword In Java
- Best Practices For Using This Keyword In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is new Keyword In Java?
- Uses Of The new Keyword In Java
- Memory Management With new Keyword In Java
- Example 1: Creating An Object Of A Class Using new Keyword In Java
- Example 2: Creating An Array Using The new Keyword In Java
- Best Practices For Using new Keyword In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is The Transient Keyword In Java?
- Real-Life Example Of The Transient Keyword In Java
- When To Use The Transient Keyword In Java
- Example 1: Effect Of Transient Keyword On Serialization In Java
- Example 2: Skipping Sensitive Data During Serialization With Transient Keyword In Java
- Using Transient With Final Keyword In Java
- Using Transient With Static Keyword
- Difference Between Transient And Volatile Keyword In Java
- Advantages And Disadvantages Of Transient Keyword In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Static Keyword In Java?
- Characteristics Of Static Keyword In Java
- Static Variables In Java
- Static Method In Java
- Static Blocks In Java
- Static Classes In Java
- Static Variables Vs Instance Variables In Java
- Advantages Of Static Keyword In Java
- Disadvantages Of Static Keyword In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is A Static Method In Java?
- Use Cases Of Static Method In Java
- Using Static Method In Java To Create A Utility Class
- Using Static Method In Java To Implement The Singleton Design Pattern
- Difference Between Static And Instance Methods In Java
- Limitations Of Static Method In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding Final Keyword In Java
- Final Variables In Java
- Final Methods In Java
- Final Classes In Java
- Difference Between Static And Final Keyword In Java
- Uses Of Final Keyword In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Key Difference Between final, finally, And finalize In Java
- What Is final Keyword In Java?
- What Is finally Keyword In Java?
- What Is finalize Keyword In Java?
- When To Use Which Keyword In Java?
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding The extends Keyword In Java?
- Use Of extends Keyword In Java
- Using Java extends To Implement Single Inheritance
- Using Java extends With Interfaces (Default Methods)
- Overriding Using extends Keyword In Java
- Difference Between extends And implements In Java
- Real World Applications Of Extends Keyword In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Decision Making Statement In Java?
- If Statement In Java
- If-Else Statement In Java
- Else-If Ladder In Java
- Switch Statement In Java
- Ternary/Conditional Operator (?:) In Java
- Best Practices For Writing Decision Making Statements In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Are Control Statements in Java?
- Decision-Making Control Statements In Java
- Looping Control Statements In Java
- Jump (Branching) Control Statements In Java
- Application Of Control Statements In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is The Break Statement In Java?
- Working Of The Break Statement In Java
- Using Java Break Statement With Loops
- Using Java Break Statement With Switch Statement
- Using Java Break Statement With Infinite Loops
- Common Pitfalls While Using Break Statements In Java
- Best Practices For Using The Break Statement In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Switch Statement In Java?
- Working Of The Switch Statement In Java
- Example Of Switch Statement In Java
- Java Switch Statement With String
- Java Nested Switch Statements
- Java Enum In Switch Statement
- Java Wrapper Classes In Switch Statements
- Uses Of Switch Statement In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Syntax Of main() Method In Java
- public Specifier – Main Method In Java
- static Keyword – Main Method In Java
- void Return Type Of Main Method In Java
- The main Identifier – Main Method In Java
- String[] args In Main Method In Java
- The Role Of Java Virtual Machine (JVM)
- Running Java Programs Without The Main Method
- Variations In Declaration Of Main Method In Java
- Overloading The Main Method In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Method Overriding In Java?
- Example Of Method Overriding In Java
- Ideal Use Cases Of Method Overriding In Java
- Rules For Method Overriding In Java
- Super Keyword & Method Overriding In Java
- Constructor & Method Overriding In Java
- Exception Handling In Method Overriding In Java
- Access Modifiers In Method Overriding In Java
- Advantages & Disadvantages Of Method Overriding In Java
- Difference Between Method Overloading Vs. Method Overriding In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Method Overloading In Java?
- Different Ways Of Method Overloading In Java
- Overloading The main() Method In Java
- Type Promotion & Method Overloading In Java
- Null Error & Method Overloading In Java
- Advantages Of Method Overloading In Java
- Disadvantages Of Method Overloading In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Difference Between Overloading And Overriding In Java (Comparison Table)
- What Is Method Overloading In Java?
- What Is Method Overriding In Java?
- Key Differences Between Overloading & Overriding In Java Explained
- Difference Between Overloading And Overriding In Java Code Example
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is A One-Dimensional Array In Java?
- Key Characteristics Of One-Dimensional Arrays In Java
- Declaration Of One-Dimensional Array In Java
- Initialization Of One-Dimensional Array In Java
- Common Operations On One-Dimensional Array In Java
- Advantages Of One-Dimensional Arrays In Java
- Disadvantages Of One-Dimensional Arrays In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is A Multidimensional Array In Java?
- Difference Between Single-Dimensional And Multidimensional Arrays In Java
- Declaring Multidimensional Arrays In Java
- Initializing Multidimensional Arrays In Java
- Accessing And Manipulating Elements In Multidimensional Arrays In Java
- Working Of Multidimensional Arrays With Jagged Arrays In Java
- Why Use Multidimensional Arrays In Java?
- Limitations Of Multidimensional Arrays In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Are Jagged Arrays In Java?
- Comparison With Regular Multi-Dimensional Arrays
- Declaring Jagged Arrays In Java
- Initialization Of Jagged Arrays In Java
- Printing Elements Of A Jagged Array In Java
- Accessing And Modifying Elements Of A Jagged Array In Java
- Advantages Of Jagged Arrays In Java
- Disadvantages Of Jagged Arrays In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Array Of Objects In Java?
- Declare And Initialize An Array Of Object In Java
- Example Of An Array Of Objects In Java
- Sorting An Array Of Objects In Java
- Passing Arrays Of Objects To Methods In Java
- Returning Arrays Of Objects From Methods In Java
- Advantages Of Arrays Of Objects In Java
- Disadvantages Of Arrays Of Objects In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is A Dynamic Array In Java?
- Why Use Dynamic Array In Java?
- What Is The Size And Capacity Of A Dynamic Array In Java?
- How To Create A Dynamic Array In Java?
- Managing Dynamic Data Input In Java
- Storing And Processing Real-Time Data In Java
- Use Cases Of Dynamic Arrays In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Why Return An Array In Java?
- How To Return An Array In Java
- Example 1: Returning An Array Of First N Squares
- Example 2: Doubling the Values of an Array
- Common Scenarios For Returning Arrays In Java
- Points To Remember When Returning Arrays In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding ArrayList In Java
- Differences Between Arrays And ArrayList In Java
- Returning An ArrayList In Java
- Common Use Cases For Returning An ArrayList In Java
- Pitfalls To Avoid When Returning An ArrayList In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is A Thread In Java?
- Thread Vs Process
- What is a Thread Life Cycle In Java?
- What Are Thread Priorities?
- Creating Threads In Java
- Java Thread Methods
- Commonly Used Constructors In Thread Class
- Thread Synchronization In Java
- Common Challenges Faced While Using Threads In Java
- Best Practices For Using Threads In Java
- Real-World Applications Of Threads In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding Multithreading In Java
- Methods Of Multithreading In Java (Examples)
- Difference Between Multithreading And Multitasking In Java
- Handling Exceptions In Multithreading
- Best Practices For Multithreading In Java
- Real-World Use Cases of Multithreading In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Thread Priority In Java?
- Built-In Thread Priority Constants In Java
- Thread Priority: Setter & Getter Methods
- Limitations Of Thread Priority In Java
- Best Practices For Using Thread Priority In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Thread Synchronization In Java?
- The Need For Thread Synchronization In Java
- Types Of Thread Synchronization In Java
- Mutual Exclusion In Thread Synchronization In Java
- Coordination Synchronization (Thread Communication) In Java
- Advantages Of Thread Synchronization In Java
- Disadvantages Of Thread Synchronization In Java
- Alternatives To Synchronization In Java
- Deadlock And Thread Synchronization In Java
- Real-World Use Cases Of Thread Synchronization In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is A Daemon Thread In Java?
- User Threads Vs. Daemon Threads In Java
- Methods For Daemon Threads In The Thread Class
- Creating Daemon Threads In Java
- Checking The Daemon Status Of A Thread
- Exceptions In Daemon Threads
- Limitations Of Daemon Threads In Java
- Practical Applications Of Daemon Threads In Java
- Common Mistakes To Avoid When Working With Daemon Threads In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Why Do Threads Need To Communicate?
- Understanding Inter Thread Communication In Java
- The wait() Method In Inter-Thread Communication
- The notify() Method In Inter-Thread Communication
- The notifyAll() Method In Inter-Thread Communication
- Difference Between wait() And sleep() Methods In Java
- Best Practices For Inter Thread Communication In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding The Factorial Concept
- Approaches To Implementing Factorial In Java
- Find Factorial In Java Using Iterative Approach (Using a Loop)
- Find Factorial In Java Using Recursive Approach
- Complexity Analysis Of Factorial Programs In Java
- Applications Of Factorial Program In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding The Leap Year Concept
- Approach To Check A Leap Year In Java
- Alternative Approach To Check A Leap Year In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is The Difference Between JDK, JRE, and JVM?
- What Is JVM (Java Virtual Machine)?
- What Is JRE (Java Runtime Environment)?
- What Is JDK (Java Development Kit)?
- Understanding The Difference Between JDK, JRE, And JVM
- Comparison Table For Difference Between JDK, JRE, And JVM
- Conclusion
- Frequently Asked Questions
Table of content:
- Difference Between Abstraction And Encapsulation In Java
- Understanding Abstraction In Java
- Understanding Encapsulation In Java
- When To Use Abstraction And Encapsulation?
- Conclusion
- Frequently Asked Questions
Table of content:
- Differences Between Abstract Class And Interface In Java
- What Is An Abstract Class In Java?
- What Is An Interface In Java?
- When To Use An Abstract Class?
- When To Use Interface?
- Compatibility Between Abstract Class And Interface In Java
- Conclusion
- Frequently Asked Questions
Table of content:
- Error Vs. Exception In Java
- What Is Error In Java?
- What Is Exception In Java?
- Best Practices For Handling Exceptions In Java
- Why Errors Should Not Be Handled In Java?
- Conclusion
- Frequently Asked Questions
Table of content:
- Key Differences: Java Vs. JavaScript
- What Is Java?
- What Is JavaScript?
- Difference Between Java And JavaScript Explained
- Conclusion
- Frequently Asked Questions
Table of content:
- Brief Introduction To C++
- Brief Introduction To Java
- Difference Between C++ and Java
- Overview & Features Of C++ Language
- Overview & Features of Java Language
- Example of C++ and Java Program
- Key Difference Between C++ And Java Explained
- Similarities Between Java Vs. C++
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- Basic Java interview questions and answers
- Intermediate Java interview questions and answers
- Advanced Java interview questions and answers
Table of content:
- Difference between core Java and advanced Java
- Important Core Java Questions
- Tips for Preparing for Core Java
Daemon Thread In Java | Creation, Applications & More (+Examples)
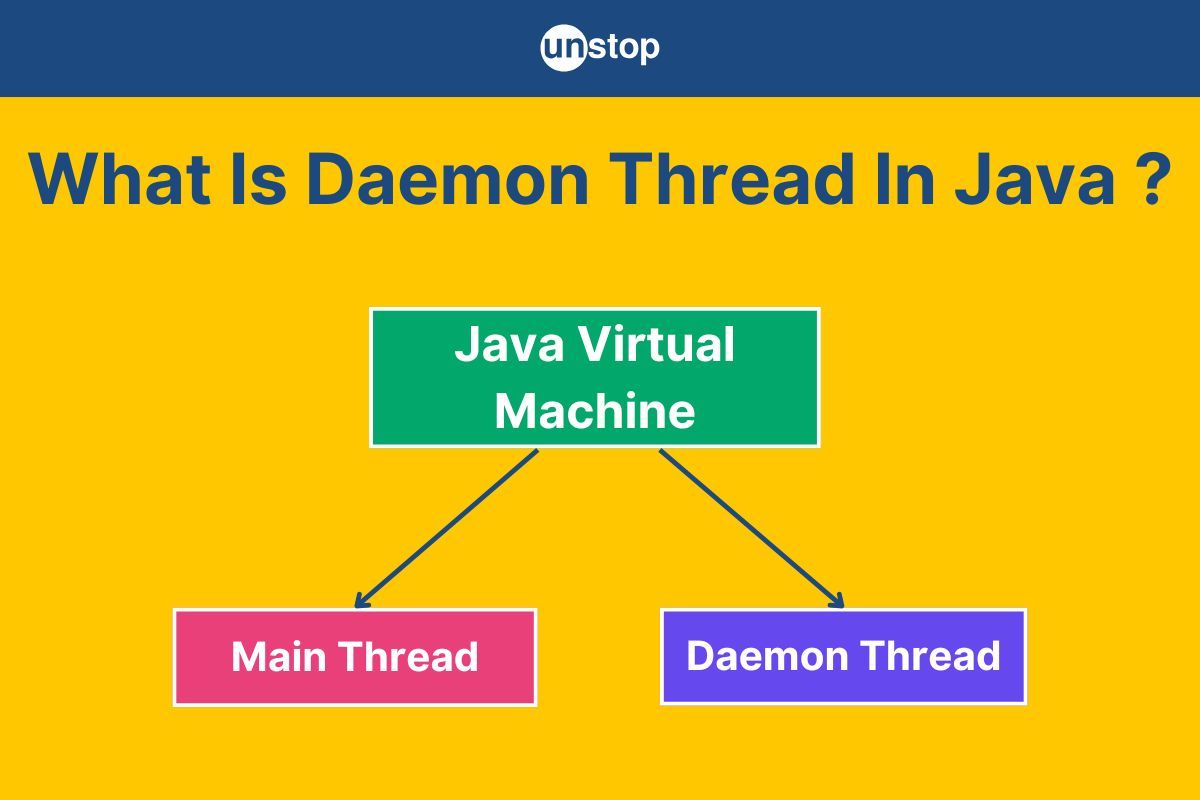
In Java, threads are the backbone of multitasking and concurrency, enabling programs to perform multiple operations simultaneously. Among the various types of threads, daemon threads hold a unique position. Unlike regular user threads that complete their tasks before the application terminates, daemon threads are designed to run in the background, providing essential services to user threads without holding up the application's lifecycle.
In this article, we will explore the concept of daemon threads, how they differ from user threads, their practical applications, and the methods to create and manage them in Java. By the end, you'll have a comprehensive understanding of how to effectively use daemon threads in your Java programs.
What Is A Daemon Thread In Java?
A daemon thread in Java programming is a background thread that supports the execution of user threads by providing auxiliary services. Unlike regular threads, daemon threads are not intended to perform tasks critical to the application; instead, they handle low-priority tasks like cleanup and maintenance. The JVM (Java Virtual Machine) does not wait for daemon threads to complete before exiting, meaning they terminate automatically once all user threads have finished execution.
Some Examples Of Daemon Threads:
- Garbage Collection (GC): Java’s garbage collector is a classic example of a daemon thread that manages memory by removing unused objects.
- Background Logging: Logging frameworks often use daemon threads to write logs to files or servers asynchronously.
Key Features Of Daemon Threads In Java
Daemon threads play a crucial role in Java's multithreading model. Some of it’s key features are:
- Background Execution: Daemon threads run silently in the background, supporting the main application without interfering with its flow.
- Non-Blocking JVM Exit: The JVM will terminate as soon as all user threads complete, even if daemon threads are still running.
- Auxiliary Role: Daemon threads are designed to assist user threads by performing non-critical tasks, such as logging or garbage collection.
- Lifecycle Dependency: The lifecycle of a daemon thread depends on user threads; they automatically end when there are no active user threads.
Default Nature Of Daemon Threads In Java
In Java, threads are non-daemon (user threads) by default, meaning that any thread created without explicitly being set as a daemon will act as a user thread. This default behavior ensures that new threads are part of the application's main tasks and do not terminate prematurely while the JVM is running.
Explore this amazing course and master all the key concepts of Java programming effortlessly!
User Threads Vs. Daemon Threads In Java
While user threads are essential for the primary execution of an application, daemon threads provide silent background support. Understanding the distinction between user and daemon threads is vital to grasp the role of each in Java applications:
Aspect |
User Threads |
Daemon Threads |
Definition |
Threads that perform the main application tasks. |
Background threads that support user threads by performing auxiliary tasks. |
Priority |
Often assigned higher priority to ensure application functionality. |
Generally have lower priority and focus on non-critical tasks. |
JVM Exit Behavior |
JVM waits for all user threads to complete before exiting. |
JVM exits even if daemon threads are still running. |
Lifecycle Control |
Controlled explicitly by the programmer. |
Lifecycle depends on the user threads; they terminate automatically when no user threads remain. |
Use Case |
Executes core application logic, such as computations, file processing, or user interactions. |
Handles background activities like garbage collection, logging, or resource monitoring. |
Examples |
Main thread, threads for file uploads/downloads, UI threads in a GUI application. |
Garbage collector, background logger, monitoring tools. |
Creation |
Created by the programmer using Thread class or Runnable interface. |
Created like user threads but explicitly set as daemon using setDaemon(true). |
Impact on Application |
Critical to the application; the program cannot function without them. |
Auxiliary; the program can function even if these threads are absent. |
Default Nature |
Threads are user threads by default. |
Must be explicitly marked as daemon using setDaemon(true). |
Termination |
Continue execution until their task is complete or explicitly stopped. |
Automatically terminate when all user threads finish execution. |
Blocking Behavior |
Can block the JVM from exiting if still running. |
Do not block the JVM from exiting. |
Methods For Daemon Threads In The Thread Class
The Thread class in Java provides specific methods to work with daemon threads. These methods allow us to set, check, and manage daemon threads effectively.
1. The setDaemon(boolean on) Method
- Description: Sets the daemon status of a thread.
- Parameters: on (boolean) — If true, marks the thread as a daemon thread; otherwise, it is a user thread.
- Usage: Must be called before starting the thread, or it throws IllegalThreadStateException.
- Example:
Thread thread = new Thread();
thread.setDaemon(true); // Mark as a daemon thread
2. The isDaemon() Method
- Description: Checks whether a thread is a daemon thread.
- Returns: A boolean — true if the thread is a daemon thread, false otherwise.
- Usage: Can be called at any point to determine the daemon status of a thread.
- Example:
Thread thread = new Thread();
System.out.println("Is thread a daemon? " + thread.isDaemon());
3. The start() Method
- Description: Starts the execution of a thread.
- Usage with Daemon Threads: Daemon threads must be marked using setDaemon(true) before calling this method.
- Example:
Thread daemonThread = new Thread(() -> System.out.println("Daemon running..."));
daemonThread.setDaemon(true);
daemonThread.start(); // Start the daemon thread
Creating Daemon Threads In Java
Unlike user threads, daemon threads automatically terminate when all user threads finish execution. To create a daemon thread, we start by creating a normal thread and then explicitly set it as a daemon using the setDaemon(true) method before starting the thread.
Steps To Create A Daemon Thread:
- Create a Thread: Use the Thread class or implement the Runnable interface to define a thread.
- Set as Daemon: Call the setDaemon(true) method on the thread object before starting it. If this is done after starting the thread, an IllegalThreadStateException is thrown.
- Start the Thread: Use the start() method to begin the execution of the daemon thread.
- Verify Daemon Status: Use the isDaemon() method to confirm whether the thread is a daemon.
Let’s look at a code example to understand the creation of daemon thread in Java-
Code Example:
public class DaemonThreadDemo {
public static void main(String[] args) {
// Create a thread
Thread daemonThread = new Thread(() -> {
while (true) {
System.out.println("Daemon thread is running...");
try {
Thread.sleep(500); // Sleep for 500 milliseconds
} catch (InterruptedException e) {
e.printStackTrace();
}
}
});
// Set the thread as a daemon
daemonThread.setDaemon(true);
// Verify if the thread is a daemon
System.out.println("Is the thread a daemon? " + daemonThread.isDaemon());
// Start the thread
daemonThread.start();
// Main thread logic
System.out.println("Main thread is running...");
try {
Thread.sleep(2000); // Sleep for 2 seconds
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println("Main thread is finishing...");
}
}
Output (set code file name as DaemonThreadDemo.java):
Is the thread a daemon? true
Main thread is running...
Daemon thread is running...
Daemon thread is running...
Daemon thread is running...
Daemon thread is running...
Main thread is finishing...
Explanation:
In the above code example-
- We begin by defining a DaemonThreadDemo class with a main() method. This serves as the entry point of our program.
- Inside the main method, we create a new thread using a lambda expression. This thread continuously prints "Daemon thread is running..." in an infinite loop.
- To avoid overwhelming the console, we make the thread pause for 500 milliseconds after each print statement using Thread.sleep(500). If interrupted, it catches the InterruptedException and prints the stack trace.
- We then set this thread as a daemon by calling daemonThread.setDaemon(true). This ensures that the thread runs in the background and terminates when the main thread finishes.
- To confirm the daemon status, we use daemonThread.isDaemon() and print the result. This helps us verify that the thread has been correctly marked as a daemon.
- We start the daemon thread using daemonThread.start(), allowing it to execute its logic concurrently with the main thread.
- Meanwhile, the main thread prints "Main thread is running..." to the console, signifying its operation.
- We make the main thread sleep for 2 seconds using Thread.sleep(2000) to simulate some processing time. During this pause, the daemon thread continues its execution.
- Finally, the main thread prints "Main thread is finishing..." before terminating. At this point, the daemon thread also stops running since it depends on the main thread's lifecycle.
Checking The Daemon Status Of A Thread
In Java, we can check whether a thread is a daemon thread using the isDaemon() method of the Thread class. This method returns a boolean value:
- true if the thread is a daemon.
- false if the thread is a user thread.
The daemon status of a thread determines its role:
- Daemon Threads: Supportive background tasks that terminate when all user threads complete.
- User Threads: Perform core application tasks and keep the JVM running until they finish execution.
Code Example:
public class CheckDaemonStatus {
public static void main(String[] args) {
// Create a user thread (default behavior)
Thread userThread = new Thread(() -> System.out.println("User thread running..."));
// Create a daemon thread
Thread daemonThread = new Thread(() -> System.out.println("Daemon thread running..."));
daemonThread.setDaemon(true); // Set as daemon
// Check and print the daemon status of each thread
System.out.println("Is userThread a daemon? " + userThread.isDaemon());
System.out.println("Is daemonThread a daemon? " + daemonThread.isDaemon());
// Start the threads
userThread.start();
daemonThread.start();
}
}
Output (set code file name as CheckDaemonStatus.java):
Is userThread a daemon? false
Is daemonThread a daemon? true
User thread running…
Daemon thread running...
Explanation:
In the above code example-
- We start by defining a CheckDaemonStatus class with a main() method as the program's entry point.
- Inside the main method, we create a thread named userThread. By default, this is a user thread, and its task is to print "User thread running..." when executed.
- Next, we create another thread named daemonThread. This thread is specifically set as a daemon thread by calling daemonThread.setDaemon(true). Its task is to print "Daemon thread running..." during execution.
- To verify the type of each thread, we use the isDaemon() method. We check and print whether userThread is a daemon thread, followed by checking daemonThread. This output helps us confirm their respective statuses.
- We then start both threads using their start() method, allowing them to execute their respective logic concurrently.
- The userThread completes its task independently of the daemonThread, which is terminated automatically once the main thread finishes execution.
Note: Threads are user threads by default, meaning isDaemon() returns false unless explicitly set otherwise. A thread can be marked as a daemon using the setDaemon(true) method before starting it.
Exceptions In Daemon Threads
Daemon threads in Java, like user threads, can throw exceptions during their execution. However, handling exceptions in daemon threads requires careful attention due to their non-critical role and the following characteristics:
Behavior Of Daemon Threads When An Exception Occurs:
- Abrupt Termination: If an uncaught exception occurs in a daemon thread, it terminates immediately, just like a user thread.
- No Impact on JVM: The JVM remains unaffected as long as user threads are running.
- Risk of Incomplete Tasks: Since daemon threads are often used for auxiliary operations, exceptions can lead to incomplete background tasks.
The different types of exceptions encountered in daemon threads in Java are:
1. Checked Exceptions
These exceptions must be explicitly declared in the method signature or handled using a try-catch block. They occur due to foreseeable and recoverable conditions.
Example Scenarios in Daemon Threads
- InterruptedException: When a daemon thread is sleeping or waiting and is interrupted.
- IOException: If a daemon thread performs I/O operations, such as logging, and encounters issues like file not found or disk write errors. For Example-
Thread daemonThread = new Thread(() -> {
try {
Thread.sleep(1000); // Checked exception
} catch (InterruptedException e) {
System.out.println("Daemon thread interrupted: " + e.getMessage());
}
});
daemonThread.setDaemon(true);
daemonThread.start();
2. Unchecked Exceptions (Runtime Exceptions)
These exceptions occur during runtime and do not require explicit handling in the code. They can terminate the daemon thread if unhandled.
Example Scenarios in Daemon Threads
- NullPointerException: Attempting to access a null object.
- ArithmeticException: Performing illegal arithmetic operations, such as division by zero. For Example-
Thread daemonThread = new Thread(() -> {
try {
int result = 10 / 0; // Unchecked exception
} catch (ArithmeticException e) {
System.out.println("ArithmeticException caught: " + e.getMessage());
}
});
daemonThread.setDaemon(true);
daemonThread.start();
3. Errors (Critical Issues)
Errors indicate severe problems that the application cannot recover from, such as memory issues or JVM crashes. These are generally not caught in a try-catch block.
Example Scenarios in Daemon Threads
- StackOverflowError: Infinite recursion in a daemon thread.
- OutOfMemoryError: When a daemon thread tries to allocate memory, but the heap is full. For Example-
Thread daemonThread = new Thread(() -> {
try {
recursiveCall(); // This will cause StackOverflowError
} catch (StackOverflowError e) {
System.out.println("Caught StackOverflowError: " + e.getMessage());
}
});daemonThread.setDaemon(true);
daemonThread.start();
// Recursive method to trigger StackOverflowError
static void recursiveCall() {
recursiveCall();
}
4. Thread-Specific Exceptions
Daemon threads may also encounter exceptions unique to threading operations:
- IllegalThreadStateException: Occurs when attempting to change the daemon status of a thread after it has started. For Example-
Thread daemonThread = new Thread(() -> System.out.println("Running daemon thread"));
daemonThread.start();
try {
daemonThread.setDaemon(true); // Will throw IllegalThreadStateException
} catch (IllegalThreadStateException e) {
System.out.println("IllegalThreadStateException caught: " + e.getMessage());
}
Best Practices for Exception Handling in Daemon Threads:
- Handle Checked Exceptions: Always use try-catch blocks for predictable issues like I/O or sleep interruptions.
- Anticipate Runtime Exceptions: Test and validate daemon thread logic to avoid unexpected runtime errors.
- Avoid Critical Operations: Do not assign tasks prone to errors or unrecoverable failures to daemon threads.
- Use Logging: Log exceptions to monitor and debug issues encountered in daemon threads effectively.
- Minimal Dependencies: Avoid critical dependencies on daemon threads for application integrity.
Therefore, by understanding and handling these exceptions, we can make daemon threads more reliable and predictable in Java applications.
Limitations Of Daemon Threads In Java
While daemon threads are useful for background operations, they come with significant limitations that make them unsuitable for critical tasks. Some of these limitations are as follows:
- Unpredictable Termination: Daemon threads are terminated abruptly when all user threads finish execution, regardless of whether the daemon thread has completed its task. This means that if a daemon thread is performing a critical operation, such as writing data to a file or completing a long-running computation, it may not finish properly, leading to potential data corruption or incomplete operations.
- No Guarantee of Completion: Since daemon threads are not essential for the application’s completion, they are subject to termination when the JVM shuts down. This means there is no guarantee that a daemon thread will finish its execution, even if it’s in the middle of important work. For example, a daemon thread that is performing periodic maintenance or logging may leave tasks unfinished if the JVM exits unexpectedly.
- Limited Control Over Execution: Daemon threads are designed to run in the background without user intervention. However, this means they do not have as much control or attention from the developer. If a daemon thread is stuck or running into issues (e.g., deadlocks), it may go unnoticed since it doesn’t block the application, making debugging and troubleshooting more difficult.
- Resource Management Challenges: Daemon threads typically do not manage resources such as database connections or file handles as effectively as user threads. If a daemon thread terminates unexpectedly, it may leave resources in an inconsistent state, such as unclosed file streams or uncommitted database transactions, leading to potential memory leaks or resource contention.
- Lack of Synchronization Guarantees: Daemon threads may not synchronize their operations with user threads, which could lead to race conditions or inconsistent states if both types of threads access shared resources concurrently. If a daemon thread is performing a task while the user thread expects the resource to be in a specific state, there could be conflicts leading to unexpected behavior.
- Inability to Handle Critical Tasks: Daemon threads are not suitable for critical tasks that must be guaranteed to complete. For example, operations like saving important user data or handling transactions cannot be safely handled by daemon threads since the JVM may terminate the daemon thread before it finishes, resulting in lost data or transactions.
- No Exception Handling by Default: In daemon threads, uncaught exceptions may not be handled as effectively as in user threads. If a daemon thread throws an exception and it's not caught within the thread, the thread may terminate, and the exception may go unnoticed. This lack of visibility makes it more difficult to identify and address issues with daemon threads.
- Performance Impact: Daemon threads, if not properly managed, can affect the overall performance of an application. For instance, if a daemon thread is running tasks like garbage collection, logging, or background updates too frequently, it may consume excessive CPU cycles or memory resources, impacting the performance of user threads and the application as a whole.
- Difficulty in Testing and Debugging: Since daemon threads run in the background, they are often challenging to test and debug. If a problem arises in a daemon thread, it may not be easy to reproduce the issue, as the thread's execution is usually invisible to the developer. This makes tracking down issues in daemon threads more complicated compared to user threads.
- Limited Usage Scenarios: Daemon threads are primarily intended for non-essential background tasks, and as such, they are not appropriate for all use cases. They should not be used for tasks that require high reliability, such as processing critical business logic or handling important transactions. Their use should be reserved for auxiliary tasks that can be safely abandoned when the JVM shuts down.
Sharpen your coding skills with Unstop's 100-Day Coding Sprint and compete now for a top spot on the leaderboard!
Practical Applications Of Daemon Threads In Java
Daemon threads are ideal for performing background tasks that support the primary (user) threads without blocking the program's execution. Below are some of their key practical applications:
1. Garbage Collection (GC)
- Purpose: Automatically manages memory by reclaiming unused objects, preventing memory leaks.
- Daemon Nature: Runs in the background without requiring explicit control, ensuring smooth execution of user threads.
- Example: The JVM's garbage collector is a classic example of a daemon thread, responsible for reclaiming heap memory occupied by unreachable objects.
2. Background Logging
- Purpose: Records application logs in the background for debugging, monitoring, and auditing purposes.
- Daemon Nature: Ensures that log collection does not interrupt the main application flow.
- Example:
Thread loggingThread = new Thread(() -> {
while (true) {
System.out.println("Logging application status...");
try {
Thread.sleep(5000); // Log every 5 seconds
} catch (InterruptedException e) {
System.out.println("Logging thread interrupted.");
}
}
});loggingThread.setDaemon(true);
loggingThread.start();
3. Task Scheduling
- Purpose: Schedules and executes tasks periodically, such as sending notifications or performing database cleanup.
- Daemon Nature: Operates in the background without blocking user threads.
- Example: A scheduler that sends reminders at regular intervals.
4. Resource Monitoring
- Purpose: Tracks resource utilization (CPU, memory, disk, etc.) and notifies administrators if thresholds are exceeded.
- Daemon Nature: Provides continuous monitoring without affecting the application's performance.
- Example:
Thread resourceMonitor = new Thread(() -> {
while (true) {
System.out.println("Monitoring resources...");
try {
Thread.sleep(1000); // Monitor every second
} catch (InterruptedException e) {
System.out.println("Resource monitoring interrupted.");
}
}
});
resourceMonitor.setDaemon(true);
resourceMonitor.start();
5. Database Connection Pooling
- Purpose: Manages a pool of database connections to optimize resource usage and improve performance.
- Daemon Nature: Handles the creation and cleanup of idle connections in the background.
6. Cache Management
- Purpose: Updates, invalidates, or cleans cache data periodically to ensure accuracy and efficiency.
- Daemon Nature: Operates behind the scenes to support the application’s caching mechanism.
7. Security and Maintenance Tasks
- Purpose: Executes security checks, backups, and other maintenance tasks without user intervention.
- Daemon Nature: Performs tasks silently in the background to avoid interrupting application workflows.
8. Chat Applications
- Purpose: Listens for incoming messages or notifications while the user interacts with the application.
- Daemon Nature: Runs in the background to support real-time communication.
- Example: A messaging app’s thread to fetch new messages continuously.
9. Automatic Updates
- Purpose: Checks for and downloads updates in the background without disrupting the user experience.
- Daemon Nature: Operates unobtrusively while the application runs.
10. System Services
- Purpose: Provides auxiliary services like printing, networking, or file indexing in an operating system or application.
- Daemon Nature: Ensures smooth operation without active user involvement.
Common Mistakes To Avoid When Working With Daemon Threads In Java
When working with daemon threads in Java, try to avoid the following:
- Using Daemon Threads for Critical Tasks: Daemon threads should not be used for essential operations like saving user data or handling transactions, as they may be terminated abruptly when the JVM shuts down.
- Not Setting Daemon Flag Before Starting: Always set the daemon flag (setDaemon(true)) before calling start() on a thread. Setting it after starting the thread results in an IllegalThreadStateException.
- Assuming Daemon Threads Will Always Complete: Since daemon threads can be terminated when the JVM shuts down, don't assume they will always finish their tasks. Design tasks to handle incomplete work.
- Ignoring Exception Handling: Daemon threads can terminate due to unhandled exceptions. Proper exception handling is crucial to prevent them from exiting unexpectedly.
- Overloading with Too Many Daemon Threads: Creating too many daemon threads can cause performance issues. Limit the number of threads based on your application's needs.
- Neglecting Thread Interruption Handling: Daemon threads can be interrupted. Ensure they handle interruptions and terminate cleanly to avoid resource leaks.
- Assuming Daemon Threads Don’t Affect Main Thread: Daemon threads may consume system resources, affecting the performance of user threads. Monitor their resource usage to avoid performance degradation.
- Failing to Synchronize Shared Resources: Daemon threads accessing shared resources must be synchronized properly to prevent race conditions or data inconsistency.
- Assuming Daemon Threads Are Always Low Priority: Daemon threads can have their priority adjusted with setPriority(). Don’t assume they always run with low priority.
- Not Accounting for JVM Shutdown Behavior: Daemon threads are terminated when the JVM shuts down. Ensure that they are used for non-essential tasks that can be safely interrupted.
- Over-Relying on Daemon Threads for Long Tasks: Daemon threads are best suited for short-lived tasks. For long-running tasks, use user threads to ensure completion.
Thus, avoiding these mistakes helps ensure daemon threads are used effectively without introducing unexpected issues.
Are you looking for someone to answer all your programming-related queries? Let's find the perfect mentor here.
Conclusion
In Java, daemon threads are useful for running background tasks that support the main application, like garbage collection or logging. They allow the JVM to exit without waiting for these threads to finish. However, they come with limitations, such as being abruptly terminated when the JVM shuts down, and they should not be used for critical tasks. By understanding their behavior, managing resources properly, and using them for non-essential operations, you can leverage daemon threads effectively without risking data loss or performance issues in your application.
Frequently Asked Questions
Q. What is a daemon thread in Java?
A daemon thread in Java is a thread that runs in the background to perform auxiliary tasks that support the main application. These threads do not prevent the JVM from exiting. When all user threads have finished execution, the JVM can terminate, and daemon threads are automatically stopped without waiting for them to complete their tasks.
Q. How do I create a daemon thread in Java?
To create a daemon thread, you must first create a Thread object, then set it as a daemon thread using the setDaemon(true) method before starting the thread. If the daemon flag is set after calling start(), it will throw an IllegalThreadStateException. For example-
Thread thread = new Thread(() -> {
System.out.println("Daemon thread running");
});
thread.setDaemon(true); // Set as daemon before starting
thread.start();
Q. Can daemon threads be interrupted?
Yes, daemon threads can be interrupted. Like any other thread, daemon threads can be stopped by calling interrupt() on them. However, since they are typically used for background tasks, you must ensure they handle interruptions properly to avoid leaving resources in an inconsistent state.
Q. What happens if a daemon thread throws an exception?
If an exception occurs in a daemon thread and is not caught, the thread will terminate immediately. Since daemon threads are non-essential, uncaught exceptions in them will not affect the main application. However, it's a good practice to handle exceptions to ensure the background tasks can recover or fail gracefully.
Q. Are daemon threads suitable for long-running tasks?
No, daemon threads are not ideal for long-running tasks. Since they are terminated when the JVM shuts down, they should only be used for tasks that can be interrupted or abandoned without critical consequences. Long-running tasks should be handled by user threads to ensure proper completion.
Q. Can I change a thread to a daemon thread after it has started?
No, you cannot change a thread to a daemon thread once it has started. The daemon flag must be set before the start() method is called. If you try to set it after the thread has started, it will result in an IllegalThreadStateException.
With this, we have come to an end in our discussion on the daemon threads in Java. Here are a few other topics that you might be interested in reading:
- Final, Finally & Finalize In Java | 15+ Differences With Examples
- Super Keyword In Java | Definition, Applications & More (+Examples)
- How To Find LCM Of Two Numbers In Java? Simplified With Examples
- How To Find GCD Of Two Numbers In Java? All Methods With Examples
- 10 Best Books On Java In 2024 For Successful Coders
- Difference Between Java And JavaScript Explained In Detail
- Top 15+ Difference Between C++ And Java Explained! (+Similarities)
I’m a Computer Science graduate with a knack for creative ventures. Through content at Unstop, I am trying to simplify complex tech concepts and make them fun. When I’m not decoding tech jargon, you’ll find me indulging in great food and then burning it out at the gym.
Comments
Add commentLogin to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
Esuru Pooja.C 2 hours ago
Ankita Das 2 days ago