Final Keyword In Java | Variables, Methods & Classes (+Examples)
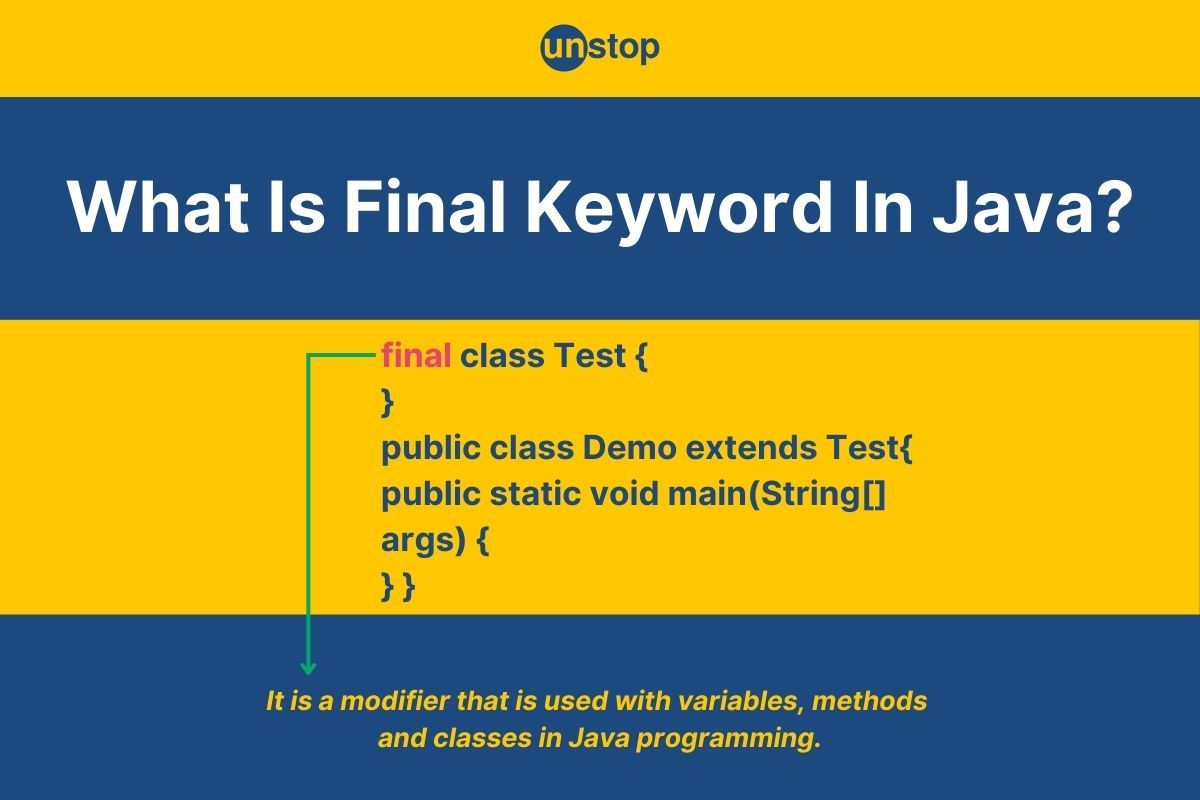
The final keyword in Java is a powerful tool that helps developers enforce restrictions on variables, methods, and classes, making code more predictable and secure. When used appropriately, final can prevent accidental modification of variables, prevent method overriding, and even make classes immutable, all of which can contribute to more efficient and bug-free code.
In this article, we’ll understand the various uses of the final keyword in Java, exploring how it can improve your code’s integrity, performance, and readability. Whether you’re a beginner or an experienced Java developer, understanding the final keyword is essential to writing robust Java applications.
Understanding Final Keyword In Java
The final keyword in Java programming language serves a specific purpose. It restricts the user from changing a variable, method, or class. For example, when a variable is declared as final, its value cannot be altered after initialization. This ensures that certain values remain constant throughout the program. In Java programming, using final can enhance code reliability and maintainability.
Key Characteristics Of Final Keyword In Java
Final keyword has distinct characteristics in Java:
- Final Variable: Once a variable is assigned a value, it cannot be changed.
- Final Method: A final method cannot be overridden by subclasses, which is useful when you want to maintain the original behavior in derived classes.
- Final Class: A final class cannot be subclassed, meaning no other class can inherit from it. This is often done for security or efficiency.
These features help programmers to create immutable classes and protect critical methods from being altered. Using the keyword final promotes better design patterns in Java programs.
Common Misconceptions About Final Keyword In Java
Many misconceptions exist about the final keyword in Java:
- One common myth is that declaring a variable as final makes it immutable. However, this is not entirely true for objects. The reference to the object cannot change, but the object's internal state can still be modified.
- Another misconception is that all classes should use final keyword to prevent inheritance. While it can be useful, overusing final may limit flexibility in code design.
Explore this amazing course and master all the key concepts of Java programming effortlessly!
Final Variables In Java
A final variable is a variable that cannot be changed once it is assigned. We declare it using the keyword final. Final variables in Java can be used in methods or classes:
- When you declare a final variable, you must initialize it.
- After initialization, any attempt to change its value results in a compile-time error.
- This ensures that the value remains constant throughout the program.
Syntax:
final dataType variableName = value;
Here:
- final: The keyword indicating that the variable cannot be reassigned.
- dataType: The type of the variable (e.g., int, String, double).
- variableName: The name of the variable.
- value: The initial value assigned to the variable (mandatory for final variables).
Code Example:
Output:
The constant value is: 100
Explanation:
In the above code example-
- We first create a class called FinalVariableExample.
- Inside this class, we define the main() method, which is the entry point for our program.
- We then declare a variable named MY_CONSTANT and use the final keyword to make it a constant, which means its value can't be changed once it's set.
- We also initialize MY_CONSTANT with the value 100.
- To display this constant, we use System.out.println() to print "The constant value is: " followed by the value of MY_CONSTANT.
- If we try to modify the value of MY_CONSTANT by assigning a new value, such as 200, the compiler will throw an error, indicating that a final variable's value cannot be reassigned.
Final Methods In Java
Final methods in Java cannot be overridden by subclasses. This means once a method is declared as final, its behaviour remains constant throughout the inheritance hierarchy. Final methods are useful in various scenarios:
- They provide security for essential operations.
- In practice, many developers use final methods when creating API libraries or frameworks.
- This ensures users follow intended usage without unexpected changes.
Syntax:
accessModifier final returnType methodName(parameters) {
// Method body
}
Here:
- accessModifier: It defines the method's visibility (e.g., public, private, protected).
- final: The keyword indicating that the method cannot be overridden.
- returnType: The type of value the method returns (e.g., void, int, String).
- methodName: The name of the method.
- parameters: The list of method parameters or method variables (if any).
Code Example:
Output:
This is a final method.
Explanation:
In the above code example-
- We create a class called ParentClass.
- Inside ParentClass, we define a method displayMessage() and mark it with the final keyword, which means it cannot be overridden by any subclasses.
- The displayMessage() method prints the message "This is a final method." to the console.
- Next, we create a subclass called ChildClass that extends ParentClass.
- In ChildClass, if we attempt to override the displayMessage() method, the compiler will throw an error since displayMessage() is declared final in ParentClass.
- In the FinalMethodExample class, we define the main() method. We then create an instance of ChildClass and call displayMessage() on it.
- This call executes the displayMessage() method from ParentClass, displaying "This is a final method." since the method cannot be overridden in ChildClass.
Sharpen your coding skills with Unstop's 100-Day Coding Sprint and compete now for a top spot on the leaderboard!
Final Classes In Java
A final class in Java cannot be subclassed. This means no other classes can extend it. It helps prevent changes to the class structure. This ensures the integrity of its methods and properties. Final classes are useful in several scenarios:
- They provide security by preventing unwanted modifications.
- Developers often use them for utility classes or constants.
- They also improve performance since the Java compiler optimizes them better than non-final classes.
Syntax:
public final class ClassName {
// Class contents
}
Here:
- public: Access modifier that defines the class’s visibility.
- final: The keyword indicating that the class cannot be subclassed.
- ClassName: The name of the class.
Code Example:
Output:
This is a final class, and it cannot be subclassed.
Explanation:
In the above code example-
- We define a class named FinalClass and mark it as final, which means it cannot be subclassed or extended by any other class.
- Inside FinalClass, we create a method called showMessage() that prints "This is a final class, and it cannot be subclassed." to the console.
- If we try to create a subclass of FinalClass, such as SubClass, the compiler will throw an error since FinalClass is marked as final.
- In the FinalClassExample class, we define the main() method. We then create an instance of FinalClass called finalClassInstance and call the showMessage() method on it.
- This displays "This is a final class, and it cannot be subclassed." to confirm that FinalClass is being used directly and cannot be extended.
Difference Between Static And Final Keyword In Java
In Java, both static and final are keywords that modify the behavior of variables, but they serve different purposes and have distinct characteristics. Here’s a detailed comparison between static and final variables:
Feature | Static Variable | Final Variable |
---|---|---|
Definition | Static keyword belongs to the class and is shared across instances. | Constant whose value cannot be changed after initialization. |
Memory Allocation | Allocated memory only once when the class is loaded. | Each instance of the class has its own copy if it’s an instance variable, but all instances share the value if it’s static. |
Initialization | Can be initialized multiple times (but typically only once in the program lifecycle). | Must be initialized exactly once. |
Mutability | Can be changed anytime after initialization. | Cannot be changed once assigned a value. |
Access | Accessed using the class name or an instance. | Accessed like a normal variable. |
Use Case | Used when a variable should be shared across all instances of a class. | Used to define constants or fixed values that should not change. |
Uses Of Final Keyword In Java
Using the final keyword in Java offers several benefits, especially in improving code stability, performance, and readability. Here’s a look at the key uses of final keyword:
- Memory Optimization: The final variables are constants, so they’re stored in a way that optimizes memory usage. The compiler can optimize these values knowing that they will not change, leading to faster access times.
- Faster Method Binding: Methods declared as final are not subject to dynamic binding (overriding). This enables the Java Virtual Machine (JVM) to perform early binding, improving performance, especially in frequently called methods.
- Data Integrity: Declaring variables as final helps to ensure that they remain constant, which can be especially useful when working with sensitive data. This makes code more secure and less error-prone.
- Method Behavior Stability: When methods are declared final, they cannot be overridden by subclasses, which preserves their intended functionality and protects important code from unintended modification.
- Thread-Safety in Multithreaded Environments: Declaring a variable as final makes it immutable once assigned, which is beneficial in concurrent programming. Final variables are thread-safe by default because their values cannot change, reducing the risk of race conditions and inconsistent data.
- Enhanced Readability and Maintainability: Using the final modifier helps to communicate the developer’s intent more clearly. A final variable or method tells others reading the code that this part is not meant to be changed, making the code easier to understand and maintain.
- Reduced Complexity in Inheritance: With final classes, inheritance is restricted. This can simplify the class hierarchy, preventing complicated dependencies or misuse in subclasses.
- Easier to Create Immutable Classes: The final keyword is essential for creating immutable classes, which are classes whose instances cannot be modified after creation. By declaring all fields final and ensuring they are initialized once, you can create truly immutable classes, which are beneficial in functional programming and concurrent applications.
Are you looking for someone to answer all your programming-related queries? Let's find the perfect mentor here.
Conclusion
The final keyword in Java language is an essential feature that enhances code reliability and maintainability. It allows developers to create constants, prevent method overriding, and restrict class inheritance, ensuring that critical components remain unchanged and predictable. By promoting immutability, final also contributes to better performance and thread safety in applications. Mastering the use of the final keyword is crucial for Java developers, as it leads to more robust and efficient software solutions while fostering clearer code intent and easier collaboration.
Frequently Asked Questions
Q. What does the final keyword do in Java?
The final keyword in Java restricts modification of variables, methods, and classes, providing a way to enforce immutability, stability, and security within the codebase. By marking a variable, method, or class as final, developers ensure that:
- Final Variables: These can only be assigned a value once. Once initialized, they cannot be reassigned, turning them into constants that improve readability and consistency.
- Final Methods: Declaring a method as final prevents subclasses from overriding it. This is especially useful when you want to lock down specific functionality that should remain consistent and unaltered across inheritance hierarchies.
- Final Classes: Declaring a class as final prevents it from being subclassed. This helps secure the class design, ensuring that its behavior and structure cannot be extended or modified in a way that might compromise its intended use.
Q. Can a final variable be changed?
No, a final variable cannot be changed once it is initialized. When you declare a variable as final, you can only assign a value to it once, either at the time of declaration or in the constructor for instance variables. This prevents any reassignment or modification afterward, ensuring that the variable remains a constant throughout the lifecycle of the application. For example-
final int MY_CONSTANT = 10;
MY_CONSTANT = 20; // This will throw a compilation error.
Using final for variables promotes immutability, which is particularly useful in multi-threaded applications where shared data must remain consistent across threads.
Q. Can we inherit a final class in Java?
No, a final class cannot be inherited. Declaring a class as final prevents any other class from subclassing it, effectively "locking" its structure and functionality. This restriction is useful when you want to:
- Preserve Class Design: By preventing inheritance, a final class ensures that no other class can alter its intended design or add potentially conflicting behavior through subclassing.
- Ensure Security and Efficiency: Many system classes, such as java.lang.String and java.lang.Math, are final. This prevents their core functionalities from being changed, ensuring reliable performance and security. For Example-
public final class FinalClass {
public void showMessage() {
System.out.println("This is a final class, and it cannot be subclassed.");
}
}
// This will throw a compilation error:
// class SubClass extends FinalClass { }
Declaring a class as final is beneficial in cases where a class represents a utility or an immutable object, where modifications via Java inheritance would compromise its intended use.
Q. Is it possible to declare an array as final in Java?
Yes, you can declare an array as final in Java. However, there’s an important distinction to note:
- Reference Immutability: Declaring an array as final prevents you from reassigning the array reference to a different array. This means that once you’ve assigned an array to a final variable, you cannot point that variable to a new array.
- Element Mutability: While you cannot change the array reference, you can still modify the individual elements within the array. For Example-
final int[] numbers = {1, 2, 3};
// numbers = new int[]{4, 5, 6}; // This line would throw a compilation error.
numbers[0] = 10; // This is allowed.
System.out.println(numbers[0]); // Output: 10
Using final on arrays is helpful in cases where the array reference should not change, but you still need flexibility in modifying the array’s content, such as dynamically updating elements.
Q. How does using the final keyword improve security in Java applications?
Using final in Java enhances application security by restricting modifications to sensitive data, methods, or classes. This control reduces the risk of accidental or malicious alterations that could compromise the application. Here’s how:
- Data Protection: Declaring variables as final ensures that critical constants or data values remain unchanged throughout the program, preserving data integrity and avoiding unintended side effects.
- Consistent Method Behavior: By marking methods as final, you prevent them from being overridden by potentially unsafe implementations in subclasses, ensuring that the method’s behavior remains secure and predictable.
- Protected Class Structure: Final classes prevent subclasses from altering the class structure, which is especially important for secure system classes. For example, making String final prevents malicious modifications or subclassing that could affect core functionalities across the application.
With this, we conclude our discussion on the final keyword in Java programming. Here are a few other topics that you might be interested in reading:
- 15 Popular JAVA Frameworks Use In 2023
- What Is Scalability Testing? How Do You Test Application Scalability?
- Top 50+ Java Collections Interview Questions
- 10 Best Books On Java In 2024 For Successful Coders
- Difference Between Java And JavaScript Explained In Detail
- Top 15+ Difference Between C++ And Java Explained! (+Similarities)
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment