Table of content:
- Understanding GCD In Mathematics
- How To Find The Greatest Common Divisor
- GCD Of Two Numbers In Java Using For Loop
- GCD Of Two Numbers In Java Using Recursion
- GCD Of Two Numbers In Java Using While Loop
- GCD Of Two Numbers In Java Using Java's Built-in gcd() Method (Java 8+)
- Complexity Analysis Of All Methods
- Practical Applications of GCD (Greatest Common Divisor)
- Conclusion
- Frequently Asked Questions
How To Find GCD Of Two Numbers In Java? All Methods With Examples
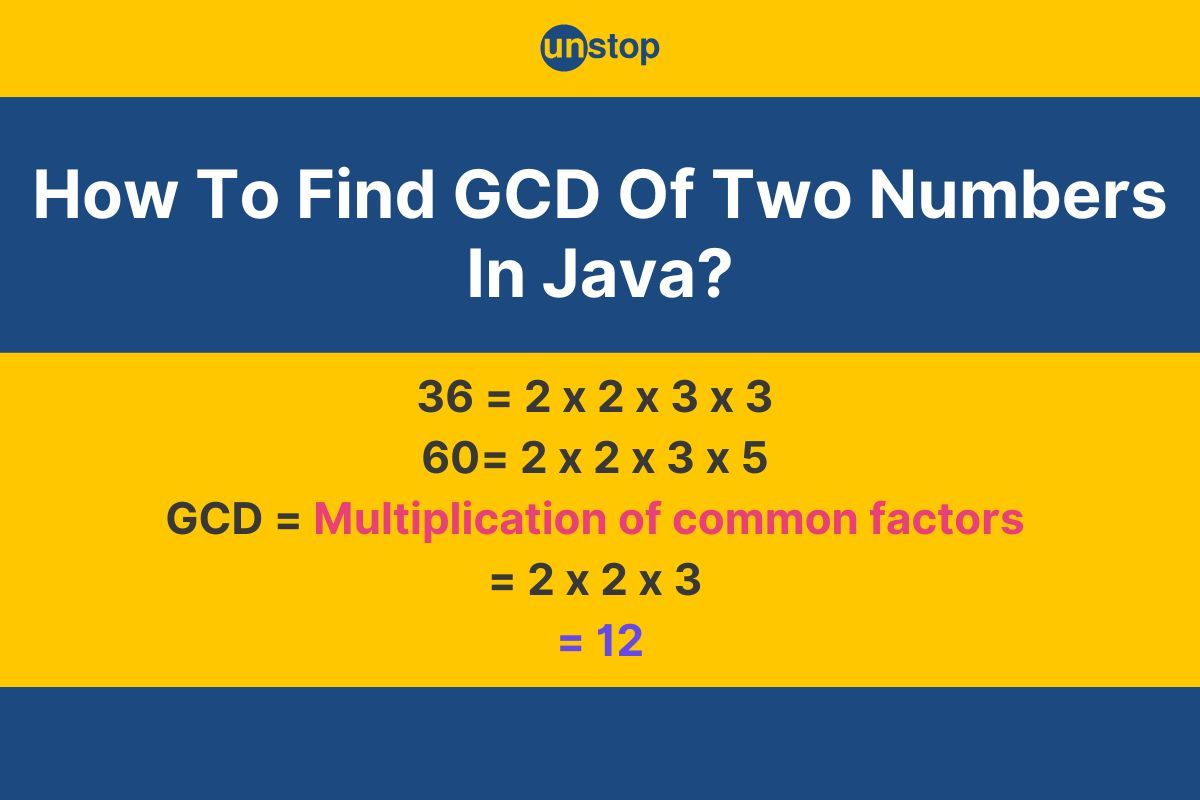
The Greatest Common Divisor (GCD) of two numbers is a crucial concept in mathematics and computer science. It represents the largest positive integer that divides both numbers without leaving a remainder. Understanding how to calculate the GCD is essential for various mathematical operations and algorithms, including simplifying fractions and solving problems in number theory.
In this article, we will explore the concept of finding the Greatest Common Divisor (GCD) of two numbers in Java programming. We will walk through the different methods to compute the GCD, focusing on the practical implementations and efficient approaches.
Understanding GCD In Mathematics
As discussed above, the Greatest Common Divisor (GCD), also known as the Greatest Common Factor (GCF), is a fundamental concept in number theory. It refers to the largest positive integer that divides two or more integers without leaving a remainder.
- For example, the GCD of 36 and 60 is 12, because 12 is the greatest number that can divide both 36 and 60 exactly.
- The GCD plays a crucial role in various mathematical operations, such as simplifying fractions, solving Diophantine equations, and in algorithms used in computer science, such as cryptography and number theory problems.
- GCD helps in reducing fractions to their simplest form and is involved in many problems requiring the identification of the greatest divisor between numbers.
Mathematical Properties Of GCD
Some of the important mathematical properties of GCD are as follows:
- Euclidean Algorithm: One of the most efficient methods for finding the GCD of two numbers is the Euclidean algorithm. This algorithm is based on the principle that the GCD of two numbers also divides their difference. If a and b are two numbers, then:
GCD(a,b)=GCD(b,a%b)
where a%b is the remainder when a is divided by b. This process continues recursively until the remainder is zero, and the GCD is the non-zero divisor at that point.
- Property of GCD with Respect to Multiples: If a is divisible by b, then GCD(a,b)=b. For example, the GCD of 50 and 10 is 10 because 50 is a multiple of 10.
- GCD and LCM Relationship: There is a mathematical relationship between GCD and the Least Common Multiple (LCM) of two numbers:
LCM(a,b)=∣a×b∣/GCD(a,b)
This relationship is useful in problems where both GCD and LCM need to be computed, such as finding the smallest common denominator in fractions.
How To Find The Greatest Common Divisor
The Greatest Common Divisor (GCD), also known as Greatest Common Factor (GCF), of two numbers is the largest number that divides both of them exactly without leaving a remainder. Here are the steps to find the GCF of two numbers:
- List the Factors of Each Number: Start by listing all the factors (divisors) of each number. A factor is any number that divides evenly into the given number.
- Identify the Common Factors: Look for the factors that are common to both numbers.
- Choose the Greatest Common Factor: The largest number among the common factors is the GCF.
Alternatively, you can use the Euclidean algorithm, which is a more efficient way to find the GCF, especially for larger numbers. Let’s look at an example:
Example: Finding The GCF of 36 and 60 (Using Factor Listing)
- List the factors of 36: Factors of 36 are- 1, 2, 3, 4, 6, 9, 12, 18, 36
- List the factors of 60: Factors of 60 are- 1, 2, 3, 4, 5, 6, 10, 12, 15, 20, 30, 60
- Identify the common factors: Common factors of 36 and 60 are- 1, 2, 3, 4, 6, 12
- Choose the greatest common factor: The greatest common factor is 12.
Thus, GCF(36, 60) = 12.
GCD Of Two Numbers In Java Using For Loop
To find the Greatest Common Divisor (GCD) of two numbers using a for loop, we can follow a simple method of iterating through all possible divisors and checking which is the largest one that divides both numbers without leaving a remainder.
Working Algorithm:
- Input the Two Numbers: First, we need two numbers for which we want to find the GCD. Let's call them a and b.
- Identify the Smaller Number: The GCD will always be less than or equal to the smaller of the two numbers, so we start our loop from 1 to the smaller of the two numbers.
- Check Divisibility: For each number from 1 to the smaller number, check if it divides both a and b without leaving a remainder. If it does, we know that it's a common divisor.
- Track the Largest Divisor: As we find common divisors, we keep track of the largest one, which will be the GCD.
- Return the GCD: Once the loop completes, the largest common divisor will be the GCD.
Code Example:
import java.util.Scanner;
public class GCDUsingForLoop {
public static void main(String[] args) {
// Create a scanner object to read input
Scanner scanner = new Scanner(System.in);
// Input two numbers
System.out.print("Enter the first number: ");
int a = scanner.nextInt();
System.out.print("Enter the second number: ");
int b = scanner.nextInt();
// Initialize the GCD variable
int gcd = 1;
// Find the smaller of the two numbers
int min = (a < b) ? a : b;
// Loop from 1 to the smaller number
for (int i = 1; i <= min; i++) {
// Check if 'i' is a divisor of both numbers
if (a % i == 0 && b % i == 0) {
gcd = i; // Update gcd to the current divisor
}
}
// Output the GCD
System.out.println("The GCD of " + a + " and " + b + " is: " + gcd);
// Close the scanner
scanner.close();
}
}
Output (set the code file name as GCDUsingForLoop.java):
Enter the first number: 36
Enter the second number: 24
The GCD of 36 and 24 is: 12
Explanation:
In the above code example-
- We start by importing the Scanner class from the java.util package to allow user input.
- In the main() method, we create a Scanner object named scanner to capture user input from the console.
- We prompt the user to enter two integers, which we store in the variables a and b.
- We initialize a variable gcd to 1, which will hold the greatest common divisor (GCD) of the two numbers.
- To optimize the loop, we find the smaller of the two numbers (a and b) using a conditional operator and store it in min.
- We then start a for loop that runs from 1 to min, checking each number to see if it divides both a and b without a remainder.
- If a number divides both numbers evenly, we update the value of gcd to that number, ensuring we always keep the latest common divisor.
- After the loop ends, we print the value of gcd, which is the greatest common divisor of a and b.
- Finally, we close the Scanner object to release the resource.
Time Complexity: O(min(a, b))
Space Complexity: O(1)
GCD Of Two Numbers In Java Using Recursion
In this approach, we use recursion to calculate the Greatest Common Divisor (GCD) of two numbers. Recursion is a method where a function calls itself to break down the problem into smaller subproblems. The key idea behind the recursive approach to finding the GCD is based on the Euclidean algorithm, which states that:
GCD(a,b)=GCD(b,a mod b)
The algorithm continues recursively until the second number (b) becomes zero, at which point the first number (a) will be the GCD. Here-
- Base Case: If b == 0, then the GCD is a. This is the termination condition for the recursion.
- Recursive Case: If b != 0, we recursively call the gcd() function with b and a % b as arguments. This reduces the problem size at each step.
- Termination: When b becomes 0, the recursion stops, and the GCD is a.
Code Example:
import java.util.Scanner;
public class GCDUsingRecursion {
// Recursive function to find GCD
public static int gcd(int a, int b) {
// Base case: if b is 0, return a
if (b == 0) {
return a;
}
// Recursive case: gcd(b, a % b)
return gcd(b, a % b);
}
public static void main(String[] args) {
// Create a scanner object to read input
Scanner scanner = new Scanner(System.in);
// Input two numbers
System.out.print("Enter the first number: ");
int a = scanner.nextInt();
System.out.print("Enter the second number: ");
int b = scanner.nextInt();
// Call the recursive GCD function and display the result
int result = gcd(a, b);
System.out.println("The GCD of " + a + " and " + b + " is: " + result);
// Close the scanner
scanner.close();
}
}
Output (set code file name as GCDUsingRecursion.java):
Enter the first number: 15
Enter the second number: 25
The GCD of 15 and 25 is: 5
Explanation:
In the above code example-
- We begin by importing the Scanner class from the java.util package to handle user input.
- Inside the GCDUsingRecursion class, we define a recursive function gcd that takes two integers, a and b, as parameters.
- In the gcd() function, we first check if b is equal to 0, which is the base case of the recursion.
- If b is 0, we return a as the GCD.
- If b is not 0, we proceed with the recursive case, calling gcd with the values b and a % b. This step reduces the problem by applying the Euclidean algorithm.
- In the main() method, we create a Scanner object to read input from the user.
- We prompt the user to input two integers, storing them in the variables a and b.
- We then call the recursive gcd function, passing a and b as arguments, and store the result in the result variable.
- After the recursive call completes, we print the result, displaying the greatest common divisor of a and b.
- Finally, we close the Scanner object to free up resources.
Time Complexity: O(log(min(a, b)))
Space Complexity: O(log(min(a, b)))
Explore this amazing course and master all the key concepts of Java programming effortlessly!
GCD Of Two Numbers In Java Using While Loop
In this approach, we calculate the Greatest Common Divisor (GCD) of two numbers using a while loop. This method is similar to the Euclidean algorithm used in recursion, but instead of recursive function calls, we use a loop to repeatedly reduce the problem.
Working Algorithm:
- Input the Two Numbers: We need two numbers, a and b, for which we want to find the GCD.
- Use a While Loop: The loop continues as long as b is not zero. In each iteration, we compute the remainder of a divided by b and set a to b and b to a % b.
- Termination: The loop stops when b becomes zero, at which point a will hold the GCD.
- Return the GCD: After the loop finishes, a contains the GCD of the two numbers.
Code Example:
import java.util.Scanner;
public class GCDUsingWhileLoop {
// Function to find GCD using a while loop
public static int gcd(int a, int b) {
// While loop to continue until b becomes 0
while (b != 0) {
int temp = b;
b = a % b; // Calculate remainder
a = temp; // Assign b to a for the next iteration
}
// When b becomes 0, a holds the GCD
return a;
}
public static void main(String[] args) {
// Create a scanner object to read input
Scanner scanner = new Scanner(System.in);
// Input two numbers
System.out.print("Enter the first number: ");
int a = scanner.nextInt();
System.out.print("Enter the second number: ");
int b = scanner.nextInt();
// Call the gcd function and display the result
int result = gcd(a, b);
System.out.println("The GCD of " + a + " and " + b + " is: " + result);
// Close the scanner
scanner.close();
}
}
Output (set code file name as GCDUsingWhileLoop):
Enter the first number: 6
Enter the second number: 18
The GCD of 6 and 18 is: 6
Explanation:
In the above code example-
- We start by importing the Scanner class from the java.util package to handle user input.
- Inside the GCDUsingWhileLoop class, we define a function gcd() that takes two integers, a and b, as parameters to find their greatest common divisor.
- In the gcd() function, we use a while loop that continues executing as long as b is not 0.
- Inside the loop, we first store the value of b in a temporary variable temp.
- We then calculate the remainder of a divided by b using the modulus operator (a % b) and assign it to b.
- We assign the previous value of b (stored in temp) to a for the next iteration, effectively applying the Euclidean algorithm.
- The loop continues until b becomes 0, at which point a holds the GCD of the two numbers.
- In the main() method, we create a Scanner object to read input from the user.
- We prompt the user to input two integers, which are stored in the variables a and b.
- We call the gcd() function, passing a and b as arguments, and store the result in the result variable.
- After receiving the result, we print the GCD of a and b.
- Finally, we close the Scanner object to release the resources.
Time Complexity: O(log(min(a, b)))
Space Complexity: O(1)
Sharpen your coding skills with Unstop's 100-Day Coding Sprint and compete now for a top spot on the leaderboard!
GCD Of Two Numbers In Java Using Java's Built-in gcd() Method (Java 8+)
In Java 8 and later, the Math class provides a built-in method gcd() to compute the Greatest Common Divisor (GCD) of two numbers. This method simplifies the process of calculating the GCD as it directly implements the efficient Euclidean algorithm internally, offering a quick and reliable solution.
Working Algorithm:
- Input the Two Numbers: The two integers for which we need to find the GCD are taken as input.
- Use Math.gcd(a, b): Java provides the gcd() method in the Math class, which takes two integers a and b as arguments and returns their GCD. This method internally uses the Euclidean algorithm.
- Output the GCD: The result is returned directly by the method and can be printed or used in further calculations.
Code Example:
import java.util.Scanner;
public class GCDUsingMathGCD {
public static void main(String[] args) {
// Create a scanner object to read input
Scanner scanner = new Scanner(System.in);
// Input two numbers
System.out.print("Enter the first number: ");
int a = scanner.nextInt();
System.out.print("Enter the second number: ");
int b = scanner.nextInt();
// Call Math.gcd() and display the result
int result = Math.gcd(a, b);
System.out.println("The GCD of " + a + " and " + b + " is: " + result);
// Close the scanner
scanner.close();
}
}
Output (set code file name as GCDUsingMathGCD):
Enter the first number: 36
Enter the second number: 60
The GCD of 36 and 60 is: 12
Explanation:
In the above code example-
- We begin by importing the Scanner class from the java.util package to handle user input.
- In the main() method, we create a Scanner object named scanner to read data from the console.
- We prompt the user to enter two integers, which we store in the variables a and b.
- We then call the Math.gcd() method, which is a built-in Java function to compute the greatest common divisor of two numbers. The result is stored in the variable result.
- After calculating the GCD, we print the result to the console, displaying the GCD of a and b.
- Finally, we close the Scanner object to free up resources.
Time Complexity: O(log(min(a, b)))
Space Complexity: O(1)
Note: The above code will work in Java 8 and later versions because the Math.gcd() method was introduced in Java 8. In earlier versions of Java, this method does not exist, and you would need to implement the GCD logic manually or use BigInteger.gcd() for large numbers.
Complexity Analysis Of All Methods
Here is the complexity analysis of all the methods that we have discussed above:
Method |
Time Complexity |
Space Complexity |
For Loop (Iterative) |
O(min(a, b)) |
O(1) |
Recursion (Euclidean Algorithm) |
O(log(min(a, b))) |
O(log(min(a, b))) |
While Loop (Euclidean Algorithm) |
O(log(min(a, b))) |
O(1) |
Java's Built-in gcd() Method (Java 8+) |
O(log(min(a, b))) |
O(1) |
Therefore:
- For large numbers, the Euclidean algorithm (whether implemented recursively or iteratively) is the most efficient method in terms of both time and space complexity.
- The For Loop method is the least efficient and should be avoided for large values due to its O(min(a, b)) time complexity.
- Java’s built-in gcd() method is a convenient and optimized choice for GCD computation, offering logarithmic time and constant space complexity.
Practical Applications of GCD (Greatest Common Divisor)
The Greatest Common Divisor (GCD) plays a crucial role in many mathematical and real-world applications. Below are some practical examples where GCD is used:
1. Simplifying Fractions
- Application: GCD is used to simplify fractions to their lowest terms.
- Example: Suppose you have a fraction like 36/60. To simplify it, find the GCD of 36 and 60 (which is 12) and divide both the numerator and denominator by 12.
- Why it works: Simplifying a fraction reduces it to its simplest form, making calculations easier.
2. Cryptography (RSA Algorithm)
- Application: GCD is used in cryptography, specifically in the RSA encryption algorithm, which relies on modular arithmetic.
- Example: When generating public and private keys, the two keys must be coprime (i.e., their GCD must be 1). The GCD function is used to ensure this condition is met.
- Why it works: The RSA algorithm uses the properties of prime numbers and GCD to securely encrypt and decrypt data.
3. Finding Least Common Multiple (LCM)
- Application: GCD is used to find the Least Common Multiple (LCM) of two numbers. The relationship is:
LCM(a,b)=∣a×b∣/GCD(a,b)
- Example: If you want to find the LCM of 12 and 18, first compute their GCD (which is 6), and then use the formula: LCM(12,18)=∣12×18∣/6=36
- Why it works: LCM helps in solving problems related to synchronizing events, scheduling, or finding repeating patterns.
4. Dividing Resources or Items Evenly
- Application: GCD can be used to divide resources, such as splitting items or distributing goods evenly.
- Example: If you have 36 apples and 60 oranges, and you want to distribute them evenly into baskets such that each basket has the same number of apples and oranges, the maximum number of items per basket will be the GCD of 36 and 60, which is 12.
- Why it works: The GCD tells you the largest possible number of baskets that can be formed while keeping the number of items per basket the same.
5. Scheduling and Synchronizing Cyclic Events
- Application: GCD is used in scheduling algorithms to determine when two cyclic events will coincide.
- Example: If two events occur every 12 minutes and 18 minutes, the GCD of 12 and 18 will tell you when both events will occur at the same time. In this case, the GCD is 6, so both events will coincide every 6 minutes.
- Why it works: The GCD helps identify the interval at which multiple events with different periodicities will coincide.
6. Computer Graphics and Image Processing
- Application: In digital image processing, GCD is used to find the greatest common factor when resizing images or manipulating pixels, ensuring consistent ratios.
- Example: When scaling down an image to fit into a smaller display or screen, the GCD can help determine the scaling factor to maintain the image's aspect ratio.
- Why it works: By using the GCD, you ensure that both the width and height are scaled down by the greatest possible factor without distorting the image.
7. Network Addressing (CIDR Notation)
- Application: GCD is used in Classless Inter-Domain Routing (CIDR) for efficient IP address allocation and subnetting.
- Example: When subnetting a network, you can use GCD to divide the available address space into smaller subnets by finding common divisors.
- Why it works: GCD helps in efficiently partitioning a network's address space into smaller, manageable subnets.
8. Solving Diophantine Equations
- Application: In number theory, Diophantine equations (equations that seek integer solutions) often involve the GCD.
- Example: A linear Diophantine equation like ax + by = c has integer solutions if and only if the GCD of a and b divides c.
- Why it works: The GCD provides the necessary condition for finding integer solutions to equations of this form.
9. Efficient Memory Allocation
- Application: In systems programming, GCD can help in optimizing memory allocation, ensuring that blocks of memory are efficiently divided.
- Example: When allocating memory for different applications or modules, GCD is used to determine the largest common block size to avoid fragmentation and ensure efficient usage.
- Why it works: Using the GCD for memory block sizes ensures that each block is a multiple of the GCD, making memory allocation more efficient and preventing wastage.
10. Game Development (Leveling or Resource Allocation)
- Application: In games, GCD can be used to allocate resources or scale levels of difficulty in such a way that the game remains balanced.
- Example: In a strategy game, if you want to allocate units or resources such that each group gets an equal share, the GCD of the total number of units and groups can help determine the fair distribution.
- Why it works: GCD ensures an even and optimal distribution of resources, enhancing game balance.
Are you looking for someone to answer all your programming-related queries? Let's find the perfect mentor here.
Conclusion
Finding the GCD of two numbers is a fundamental concept in mathematics, and understanding how to implement it efficiently in Java can be valuable for solving various problems. We've explored multiple methods to calculate the GCD, from simple loops and recursion to the optimized Euclidean algorithm and Java's built-in gcd() method. Each method has its own advantages depending on the context, and knowing when to use the right approach is key to writing efficient code. By mastering the different techniques, you can enhance your problem-solving skills and apply these methods to a wide range of algorithms and real-world applications.
Frequently Asked Questions
Q. What is the Greatest Common Divisor (GCD)?
The Greatest Common Divisor (GCD), also known as the Greatest Common Factor (GCF), is the largest positive integer that divides two or more numbers without leaving a remainder. For example, for the numbers 36 and 60, the GCD is 12 because 12 is the largest number that divides both 36 and 60 without leaving any remainder.
The GCD is important in many mathematical operations, especially in simplifying fractions and finding the least common multiple (LCM). The Euclidean algorithm is one of the most efficient ways to compute the GCD.
Q. How does the Euclidean algorithm work to find the GCD?
The Euclidean algorithm is based on the principle that the GCD of two numbers a and b (where a > b) is the same as the GCD of b and a % b (the remainder when a is divided by b). This process is repeated until the remainder is 0, at which point the GCD is the last non-zero remainder.
For example, to find the GCD of 60 and 48:
- First, divide 60 by 48, the remainder is 12 (60 % 48 = 12).
- Next, divide 48 by 12, the remainder is 0 (48 % 12 = 0).
- Since the remainder is 0, the GCD is 12.
This algorithm is efficient and reduces the problem size quickly, making it ideal for large numbers.
Q. What is the difference between using a for loop, while loop, and recursion to find the GCD?
- For Loop: In the for loop approach, we iterate through all integers from 1 to the smaller of the two numbers, checking if each divides both numbers. This method is less efficient, especially for larger numbers, as it can require many iterations.
- While Loop (Euclidean Algorithm): The while loop method uses the Euclidean algorithm, where we iteratively update the two numbers by replacing the larger number with the remainder of the division (a % b) until one of the numbers becomes 0. This is much faster than the for loop approach and operates in O(log(min(a, b))) time complexity.
- Recursion (Euclidean Algorithm): The recursive method is conceptually similar to the while loop but uses function calls instead of a loop. Each recursive call reduces the problem size by computing the remainder of the two numbers. The time complexity is the same as the while loop approach, O(log(min(a, b))), but recursion requires more space due to the call stack.
Q. Can the GCD of two prime numbers be anything other than 1?
No, the GCD of two prime numbers is always 1, as prime numbers have no divisors other than 1 and themselves. Since prime numbers do not share any common divisors, the only integer that can divide both of them is 1.
For example:
- The GCD of 7 and 11 is 1, because the only common divisor is 1.
- The GCD of 5 and 13 is also 1 for the same reason.
This property is essential in number theory, especially when dealing with prime factorization and cryptographic algorithms.
Q. Why is finding the GCD important in real-world applications?
Finding the GCD has practical applications in various fields such as:
- Simplifying Fractions: When you reduce a fraction to its simplest form, you divide both the numerator and the denominator by their GCD.
- Cryptography: In algorithms like RSA, the GCD is used to compute the private key by finding the inverse of numbers modulo a given value.
- Computer Science: GCD plays a role in tasks like load balancing, network optimization, and distributed systems.
- Signal Processing: The GCD can be used to synchronize multiple periodic signals or determine the period of combined signals.
In these and many other fields, the GCD is a critical operation to ensure efficiency, simplicity, and correctness in computations and algorithms.
Q. Can the GCD of two numbers be greater than either of the numbers?
No, the GCD of two numbers cannot be greater than either of the numbers. By definition, the GCD is the largest integer that divides both numbers exactly, so it must always be less than or equal to the smaller of the two numbers.
For example, for the numbers 20 and 30, the GCD is 10, which is less than both 20 and 30.
Q. How can I use the GCD to find the Least Common Multiple (LCM)?
The Least Common Multiple (LCM) of two numbers can be calculated using the GCD. The relationship between GCD and LCM is given by the formula:
LCM(a,b)=∣a×b∣/GCD(a,b)
This formula works because the product of two numbers is equal to the product of their GCD and LCM. By using the GCD, we can avoid redundant calculations, making the process of finding the LCM more efficient.
For example, to find the LCM of 12 and 18:
- First, find the GCD, which is 6.
- Then, calculate the LCM: ∣12×18∣/6=216/6=36
- Thus, the LCM of 12 and 18 is 36.
With this, we conclude our discussion on how to find the GCD of two numbers in Java. Here are a few other topics that you might be interested in reading:
- 15 Popular JAVA Frameworks Use In 2023
- What Is Scalability Testing? How Do You Test Application Scalability?
- Top 50+ Java Collections Interview Questions
- 10 Best Books On Java In 2024 For Successful Coders
- Difference Between Java And JavaScript Explained In Detail
- Top 15+ Difference Between C++ And Java Explained! (+Similarities)
I’m a Computer Science graduate with a knack for creative ventures. Through content at Unstop, I am trying to simplify complex tech concepts and make them fun. When I’m not decoding tech jargon, you’ll find me indulging in great food and then burning it out at the gym.
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
Comments
Add comment