Throws Keyword In Java | Syntax, Working, Uses & More (+Examples)
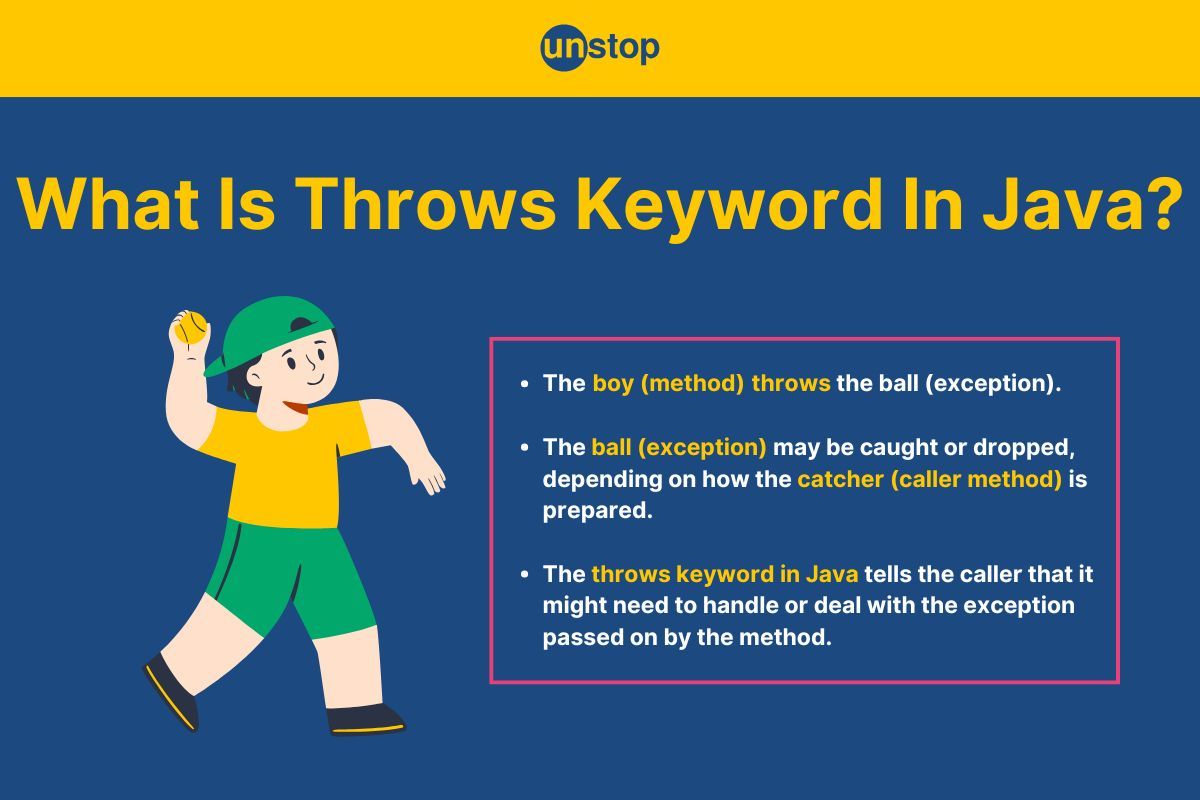
When working with Java, handling errors and exceptions is crucial to building robust and reliable applications. The throws keyword plays a key role in exception handling by allowing a method to declare the exceptions it might throw during its execution. This not only informs the developers using the method about potential errors but also ensures they handle them appropriately.
In this article, we'll explore the concept of the throws keyword, understand its syntax, and see how it works with practical examples to help you use it effectively in your Java programs.
What Is throws Keyword In Java?
The throws keyword in Java programming is used in method declarations to specify the exceptions that a method might throw during its execution. By doing so, it alerts the calling method or code block to handle these exceptions either through a try-catch block or by further propagating them with the throws keyword.
In essence, the throws keyword acts as a contract between a method and its caller, declaring the possible exceptional scenarios that may occur.
Syntax Of throws Keyword In Java
return_type method_name(parameters) throws ExceptionType1, ExceptionType2 {
// Method body
}
Here:
- return_type: The type of value the method returns (e.g., void, int).
- method_name: The name of the method being declared.
- parameters: The input parameters for the method (if any).
- throws: The keyword indicating the exceptions the method might throw.
- ExceptionType1, ExceptionType2: A comma-separated list of exception classes.
Importance Of throws In Exception Handling
The throws keyword is important because:
- Clarity and Communication: It explicitly communicates the exceptions a method might throw to its callers.
- Error Propagation: It allows exceptions to propagate up the call stack, enabling centralized error handling.
- Checked Exceptions: For checked exceptions (like IOException), Java enforces that they must be declared with throws or handled within the method.
Real-Life Analogy
Imagine a librarian issuing a book to a student but also warns the student: "This book is old and may have missing pages or damaged text."
Here, the librarian has effectively "thrown" a warning (exception) to the student. The student (caller) must decide whether to accept the book with the risk (handle the exception) or report it back to the librarian (propagate the exception).
In programming terms:
- The librarian (method) declares the possible issues (exceptions) using throws.
- The student (caller) decides how to address those issues.
Explore this amazing course and master all the key concepts of Java programming effortlessly!
How Does The throws Keyword Work?
The throws keyword in Java operates as a mechanism for exception propagation. It is used in method declarations to specify the exceptions that a method can potentially throw during its execution. These exceptions can then be handled by the calling method or further propagated up the call stack.
Here’s a step-by-step breakdown of how it works:
Step 1: Method Declares Exceptions
When a method can encounter scenarios that might lead to exceptions (e.g., file not found, invalid input, network issues), it uses the throws keyword in its method signature.
- Purpose: To indicate that the method does not handle the exception itself but leaves it to the caller.
- This declaration must be done for checked exceptions (like IOException), while unchecked exceptions (like ArithmeticException) do not require explicit declaration.
Step 2: Caller Acknowledges the Exception
The method caller has two options:
- Handle the exception using a try-catch block.
- Propagate the exception further using its own throws declaration.
Step 3: Program Flow
- If the exception is handled (via try-catch), the program continues normally.
- If it is propagated and no higher-level handler is found, the program terminates abnormally, displaying the exception stack trace.
We will now look at some code examples to understand how throws keyword functions in Java programming language.
Throwing A Checked Exception Using throws In Java
In Java, checked exceptions are exceptions that the compiler mandates to either be handled with a try-catch block or declared in the method signature using the throws keyword. These exceptions typically arise due to external factors, such as file handling or network communication issues.
By declaring a checked exception with throws, a method communicates to its caller that an exceptional situation might occur, and the caller is responsible for handling it. This mechanism promotes robust error handling and ensures that potential issues are acknowledged during program execution.
Code Example:
Output (set code file name as CheckedExceptionExample.java):
Exception caught: File not found: nonexistent.txt
Explanation:
In the above code example-
- We start by defining a class CheckedExceptionExample with a method readFile() that handles file reading operations.
- In the readFile() method, we declare that it might throw an IOException, which is a checked exception, ensuring we handle potential issues during file operations.
- Next, a File object is created using the provided file name, representing the file we intend to read.
- Before proceeding, we verify if the file exists using file.exists():
- If it doesn't, we throw an IOException with a clear error message indicating the file is not found.
- If the file exists, we create a FileReader object to read the file's content. A success message is printed to confirm the file was read successfully.
- After the reading operation, we close the FileReader using reader.close() to release system resources and avoid potential resource leaks.
- In the main() method, we instantiate the CheckedExceptionExample class to invoke the readFile() method.
- We wrap the method call in a try-catch block to handle any IOException thrown during file reading.
- When we attempt to read a non-existent file, the exception is caught, and a message is printed with the error details, demonstrating how exceptions are gracefully managed.
Throwing Multiple Exceptions Using throws In Java
In Java, a method can declare multiple exceptions in its signature by separating them with commas. This is useful when the method can potentially encounter different types of exceptions. By declaring these exceptions using the throws keyword, the method alerts its callers about the variety of issues they may need to handle. This approach ensures clarity in code and allows callers to prepare for all possible exceptional scenarios.
Code Example:
Output (set code file name as MultipleExceptionsExample.java):
IOException caught: File not found: nonexistent.txt
Explanation:
In the above code example-
- We define a class MultipleExceptionsExample with a method processFileAndDate() that handles both file operations and date parsing.
- The method declares it may throw two exceptions: IOException for file-related issues and ParseException for date parsing errors.
- We start by creating a File object with the given file name and checking if it exists using file.exists():
- If it doesn’t, we throw an IOException with an appropriate error message.
- If the file exists, we create a FileReader object to simulate reading the file, print a success message, and close the reader to release resources.
- Next, we handle the date validation by creating a SimpleDateFormat object with the format yyyy-MM-dd and setting setLenient(false) to enforce strict parsing rules.
- We attempt to parse the provided date using dateFormat.parse(date), which may throw a ParseException if the date format or values are invalid. If parsing succeeds, we print a success message with the parsed date.
- In the main() method, we create an instance of the MultipleExceptionsExample class and call the processFileAndDate() method.
- The method call is wrapped in a try-catch block to handle exceptions separately. If a file issue occurs, we catch and print an IOException message. If a date issue arises, we catch and print a ParseException message.
- When processing a non-existent file and an invalid date (2024-11-31), the code demonstrates how to handle and report each exception effectively.
Throwing A Custom Exception Using throws In Java
In Java, we can create custom exceptions by extending the Exception class (for checked exceptions) or the RuntimeException class (for unchecked exceptions). This is useful when the standard exceptions do not adequately describe the specific error scenario in your application. By using the throws keyword, a method can declare that it might throw a custom exception, signaling to its caller that they need to handle this specific scenario.
Custom exceptions improve code readability by providing meaningful names and descriptions for error conditions, making it easier to debug and maintain.
Code Example:
Output (set code file name as CustomExceptionExample.java):
Custom Exception Caught: Age must be 18 or older for registration.
Explanation:
In the above code example-
- We define a custom exception class InvalidAgeException that extends the built-in Exception class, allowing us to create exceptions specific to our application's needs.
- The constructor of InvalidAgeException takes a message and passes it to the parent Exception class using super keyword, enabling us to provide meaningful error messages when the exception is thrown.
- In the CustomExceptionExample class, we define a method registerUser() that declares it may throw the custom InvalidAgeException.
- Inside registerUser, we check if the user’s age is less than 18:
- If it is, we throw an instance of InvalidAgeException with a message explaining that the user must be 18 or older to register.
- If the age is valid, the method prints a success message indicating that the user has been registered.
- In the main() method, we create an instance of CustomExceptionExample and attempt to register a user named "Alia" with an invalid age of 16.
- The method call is enclosed in a try-catch block to handle the custom exception. When the exception is thrown, it is caught, and the message provided during the exception's creation is displayed.
- Thus, this example demonstrates how to define, throw, and handle custom exceptions to enforce specific rules in our application.
Sharpen your coding skills with Unstop's 100-Day Coding Sprint and compete now for a top spot on the leaderboard!
When To Use The throws Keyword In Java
The throws keyword in Java allows the method to delegate the responsibility of handling exceptions to its caller rather than managing them internally. This is particularly useful for maintaining code modularity and ensuring that the exception is addressed at an appropriate level of the application.
- Propagating Checked Exceptions: Use throws when a method is likely to encounter checked exceptions, such as IOException, SQLException, or ParseException, and the handling logic is better suited to its caller. For Example- File operations, database interactions.
- Separating Error Handling from Business Logic: In cases where a method performs critical tasks (like processing user input or data) but should not be responsible for handling exceptions, throws allows the caller to decide how to handle the errors.
- For Frameworks and APIs: Methods in APIs often declare exceptions using throws to provide flexibility for developers to handle them according to their use case. For Example- Many methods in the java.io package use throws to propagate exceptions.
- When the Exception is Unpredictable: If the exception depends on external factors (e.g., user input, network availability), using throws ensures the caller is aware of the potential issue and can prepare accordingly.
- Custom Exceptions: When creating and using custom exceptions, throws allows you to propagate these exceptions up the call stack, ensuring they are handled appropriately.
- For Delegating Responsibility: When the current method has no meaningful way to handle the exception, it can delegate the responsibility to the caller using throws.
Difference Between throw and throws Keyword In Java
Java provides throw and throws as keywords to handle exceptions, but they serve distinct purposes. While throw is used to actually throw an exception at runtime, throws is used in method declarations to indicate that the method might throw certain exceptions.
Given below are the key differences between the two keywords in Java:
Feature |
throw |
throws |
Definition |
Used to explicitly throw an exception within a method or block of code. |
Used in method signatures to declare exceptions that the method might throw. |
Purpose |
Transfers control to the nearest catch block or the caller by throwing an exception. |
Alerts the caller of the method about the exceptions it must handle. |
Scope |
Operates within the method body. |
Declared at the method signature level. |
Usage |
Followed by an instance of an exception. |
Followed by one or more exception class names, separated by commas. |
Exception Type |
Can throw both checked and unchecked exceptions. |
Used only for checked exceptions. |
Syntax |
throw new ExceptionType("message"); |
void methodName() throws ExceptionType { } |
Runtime or Compile-Time |
Causes an exception at runtime. |
Checked during compilation for proper handling. |
Example |
throw new IllegalArgumentException("Invalid input!"); |
public void readFile() throws IOException { } |
Therefore:
- Use throw to create and throw an actual exception where an error occurs.
- Use throws to declare the potential exceptions a method might encounter, leaving the responsibility of handling them to its caller.
Code Example:
Output (set code file name as ThrowThrowsExample.java):
IOException: nonexistentfile.txt (No such file or directory)
Custom Exception: Age must be 18 or older to register.
Explanation:
In the above code example-
- We start by defining a custom exception class InvalidAgeException, which extends Exception. This class allows us to create a specific exception for invalid ages, and it takes a message that will be passed to the parent Exception class to describe the error.
- In the ThrowThrowsExample class, we define the method validateUser(), which declares that it may throw two exceptions: InvalidAgeException and IOException. This indicates that the method might encounter an issue with the user’s age or with file operations.
- Inside validateUser, we first check if the provided age is less than 18. If it is, we use throw to manually trigger an InvalidAgeException with a message that the user must be 18 or older to register.
- Next, we attempt to read a file using a Scanner object. We create a File object with the provided file name, and if the file exists, we print a message confirming the file was found. If the file is not found or another file-related issue occurs, it throws an IOException, which is handled in the method declaration.
- In the main() method, we create an instance of ThrowThrowsExample and call the validateUser() method twice.
- In the first call, we pass a valid age but an invalid file name (nonexistentfile.txt). This triggers an IOException, which is caught and handled in the catch block.
- In the second call, we pass an invalid age (16), which triggers the custom InvalidAgeException. This exception is caught and its message is printed.
The example demonstrates how to use throw to explicitly raise custom exceptions and throws to declare potential exceptions in a method, with proper handling using try-catch blocks.
Are you looking for someone to answer all your programming-related queries? Let's find the perfect mentor here.
Best Practices For Using The throws Keyword In Java
The throws keyword is a powerful tool in Java for managing exceptions, but it should be used thoughtfully to maintain clean, robust, and readable code. Below are some best practices to follow when working with the throws keyword:
- Declare Only Necessary Exceptions: Avoid declaring unnecessary or broad exceptions like Exception or Throwable in the method signature. Be specific about the exceptions that a method might throw to provide clarity to the caller.
For Example:
public void readFile(String fileName) throws IOException { ... }
Try To Avoid:
public void readFile(String fileName) throws Exception { ... }
- Keep Exception Handling Close to the Source (If Possible): If a method can meaningfully handle an exception, handle it locally rather than propagating it with throws. Use throws only when the handling responsibility lies with the caller.
- Group Multiple Exceptions Judiciously: If a method can throw multiple exceptions, list them explicitly. Avoid overloading the caller with too many exceptions if they are unrelated. For unrelated exceptions, consider dividing the method into smaller methods.
For Example:
public void processFile(String fileName) throws IOException, ParseException { ... }
- Document the Exceptions: Always document the exceptions declared using throws in your method signature with Javadoc comments. This helps other developers understand why the method might throw an exception.
For Example:
/**
* Reads a file and processes its content.
* @param fileName the name of the file to read
* @throws IOException if the file cannot be accessed
*/
public void readFile(String fileName) throws IOException { ... }
- Use Custom Exceptions for Specific Scenarios: Create and declare custom exceptions to describe specific application errors. This makes the code more readable and easier to debug.
For Example:
public void validateAge(int age) throws InvalidAgeException { ... }
- Avoid Declaring Unchecked Exceptions: Do not use the throws keyword for unchecked exceptions (subclasses of RuntimeException). These do not require declaration and are usually due to programming errors.
- Maintain Code Readability: Avoid cluttering method signatures with too many exceptions. If necessary, refactor to simplify the logic and manage exceptions more effectively.
- Understand Exception Propagation: Be aware that using throws propagates exceptions up the call stack. Ensure the caller is adequately equipped to handle these exceptions.
- Avoid Using throws for Cleanup Tasks: For resources like files or database connections, always use try-with-resources or close the resources explicitly in a finally block rather than propagating exceptions with throws.
Example With Try-With-Resources:
public void readFile(String fileName) throws IOException {
try (BufferedReader reader = new BufferedReader(new FileReader(fileName))) {
System.out.println(reader.readLine());
}
}
- Follow API Design Principles: When designing APIs, declare exceptions that make sense for the functionality provided. Avoid leaking implementation details through the exception types declared.
Conclusion
The throws keyword in Java is a crucial tool for handling exceptions in a structured and maintainable way. It allows developers to declare that a method may throw specific checked exceptions, ensuring that the caller is aware of potential errors and can handle them appropriately. By separating exception handling from business logic, throws improves code clarity and promotes better error management practices.
When used in conjunction with the throw keyword, which explicitly triggers exceptions, throws enables more flexible and robust exception propagation, allowing for modular and clean error handling. Following best practices such as declaring only necessary exceptions, documenting exception types, and using custom exceptions where applicable, ensures that your code remains both readable and reliable.
In summary, understanding and utilizing the throws keyword properly helps in building fault-tolerant Java applications by ensuring that potential issues are properly communicated and addressed at the right level in the call stack.
Frequently Asked Questions
Q. What is the difference between throw and throws in Java?
The throw and throws keywords both deal with exceptions in Java, but they serve different purposes:
- The throw is used to explicitly throw an exception in the method body. It is followed by an instance of an exception class, for example, throw new IOException("File not found");.
- The throws is used in the method signature to declare that a method might throw certain exceptions, allowing the caller of the method to handle them. It is followed by one or more exception types, for example, public void readFile() throws IOException, FileNotFoundException { }.
Q. When should I use the throws keyword in my methods?
You should use the throws keyword in the method signature when the method might throw a checked exception (i.e., an exception that is a subclass of Exception but not RuntimeException). This includes exceptions like IOException, SQLException, and FileNotFoundException. By using throws, you inform the caller that the method may throw certain exceptions, and the caller must handle them with a try-catch block or propagate them further with throws.
Q. Can I use throws for unchecked exceptions?
No, you do not need to use the throws keyword for unchecked exceptions (subclasses of RuntimeException), such as NullPointerException, ArrayIndexOutOfBoundsException, or IllegalArgumentException. These exceptions are not required to be declared or caught. They are typically used to indicate programming errors, and it is usually better to handle them locally where they are likely to occur rather than propagating them with throws.
Q. Can I declare multiple exceptions in a method signature using throws?
Yes, you can declare multiple exceptions in a method signature by separating them with commas. This is useful when your method can throw more than one type of checked exception. For example-
public void processFile(String fileName) throws IOException, FileNotFoundException, ParseException {
// Method implementation
}
This declaration informs the caller that the method might throw any of the three specified exceptions, and the caller needs to handle or propagate them.
Q. What happens if a method with throws does not handle the declared exceptions?
If a method declares exceptions using the throws keyword but does not handle them, it is the responsibility of the method's caller to either handle the exception with a try-catch block or declare the exception in its own method signature using throws. Failing to do so will result in a compile-time error. The Java compiler ensures that the exception handling mechanism is respected, enforcing proper exception management.
Q. How does throws impact exception propagation in Java?
The throws keyword allows exceptions to propagate from the called method to the caller. When a method throws an exception declared with throws, the exception is passed up the call stack, meaning that the caller is responsible for catching or further propagating the exception. This mechanism enables structured error handling in larger applications, allowing exceptions to be handled at a higher level if necessary. For example-
public void methodA() throws IOException {
methodB();
}public void methodB() throws IOException {
throw new IOException("File error");
}
In this case, IOException from methodB() is propagated to methodA(), and it must be either handled or declared in the caller’s signature.
With this, we come to an end to our discussion on the throws keyword in Java. Here are a few other topics that you might be interested in reading:
- Convert String To Date In Java | 3 Different Ways With Examples
- Final, Finally & Finalize In Java | 15+ Differences With Examples
- Super Keyword In Java | Definition, Applications & More (+Examples)
- How To Find LCM Of Two Numbers In Java? Simplified With Examples
- How To Find GCD Of Two Numbers In Java? All Methods With Examples
- Volatile Keyword In Java | Syntax, Working, Uses & More (+Examples)
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment