Table of content:
- General Algorithm For Converting String To Date In Java
- Different Methods To Convert String To Date In Java
- Convert String To Date In Java Using Instant Class
- Convert String To Date In Java Using DateTimeFormatter Class
- Convert String To Date In Java Using SimpleDateFormat Class
- When To Use Which Method To Convert String To Date In Java
- Conclusion
- Frequently Asked Questions
Convert String To Date In Java | 3 Different Ways With Examples
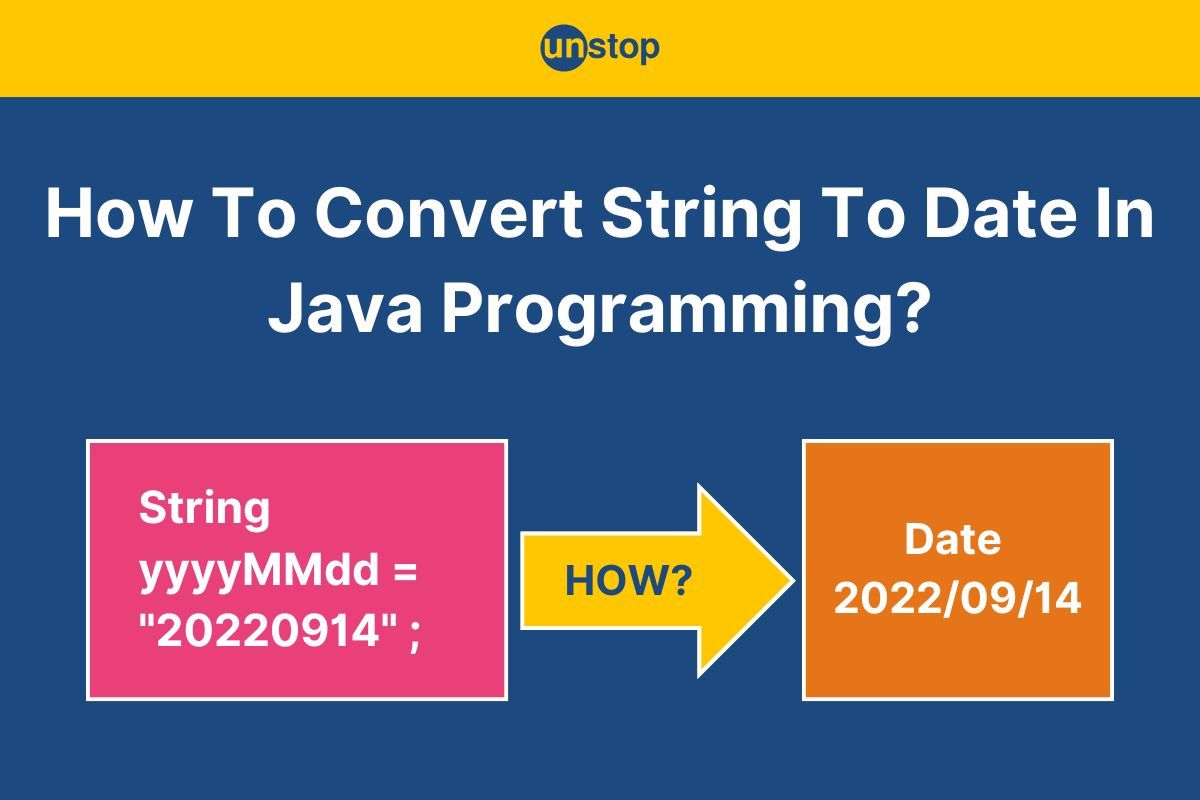
As a college student, you're likely to encounter scenarios where you need to work with dates, whether it's for deadlines, event scheduling, or managing academic records. In many cases, dates are provided as strings, and converting them into proper date objects is an essential skill in Java. Understanding how to convert a string into a Date or LocalDate object can help you efficiently manage and manipulate dates in your projects. In this article, we will guide you through the process of converting strings to dates in Java, focusing on simple methods that are easy to understand and implement. By the end, you'll be able to confidently handle date conversions in your Java applications!
General Algorithm For Converting String To Date In Java
Here’s a general algorithm for converting a String to a Date in Java programming:
- Input the Date String: Accept the date string that needs to be converted into a date object. Ensure the string is in the correct format for parsing.
- Select the Appropriate Date-Time Class: Decide which Java class to use based on the required format:
- If working with precise timestamps in UTC, use Instant.
- If working with a custom date format, use DateTimeFormatter.
- For legacy support, use SimpleDateFormat (but avoid for new code if possible).
- Define the Date-Time Format (if necessary): If the string is not in ISO-8601 format, define the appropriate date-time format using:
- DateTimeFormatter.ofPattern("your-pattern") for DateTimeFormatter.
- SimpleDateFormat("your-pattern") for SimpleDateFormat.
- Parse the String: Use the corresponding parsing method to convert the string:
- For Instant, use Instant.parse(string).
- For DateTimeFormatter, use parse() method with the string and formatter.
- For SimpleDateFormat, use parse() method with the string.
- Handle Parse Exceptions: Wrap the parsing logic in a try-catch block to handle any exceptions (e.g., ParseException for SimpleDateFormat or DateTimeParseException for DateTimeFormatter).
- Return or Use the Date Object: Once successfully parsed, the Date or Instant object can be returned or used for further operations, such as comparisons, formatting, or calculations.
Explore this amazing course and master all the key concepts of Java programming effortlessly!
Different Methods To Convert String To Date In Java
There are several methods and techniques available for converting a String value into a date format. Some of the most commonly used methods are as follows:
- Using Instant Class
- Using DateTimeFormatter Class
- Using SimpleDateFormat Class
We will now explore each of these methods in detail in the sections ahead.
Convert String To Date In Java Using Instant Class
The Instant class in Java is part of the java.time package introduced in Java 8, designed to handle timestamps and date-time values in a machine-friendly way. It represents a point on the universal timeline in UTC (Coordinated Universal Time), which makes it a useful tool when working with precise time values. While it doesn't directly handle date formats like Date or LocalDate, you can use it to convert a String into an Instant object by first parsing the string into an Instant using the DateTimeFormatter or by directly using Instant.parse().
Working Approach:
To convert a string to an Instant object, the string must be in the ISO-8601 format (yyyy-MM-ddTHH:mm:ssZ). This format includes the date, time, and timezone information. If your string matches this format, you can easily use Instant.parse() to convert it into an Instant object.
Code Example:
import java.time.Instant;
public class StringToInstant {
public static void main(String[] args) {
String dateString = "2024-11-20T15:30:00Z"; // ISO-8601 format
Instant instant = Instant.parse(dateString); // Parsing the string to Instant
System.out.println("Converted Instant: " + instant); // Output the Instant
}
}
Output (set code file name as StringToInstant.java):
Converted Instant: 2024-11-20T15:30:00Z
Explanation:
In the above code example-
- We start by importing the Instant class from the java.time package, which is part of the Java standard library and helps in dealing with time-related tasks.
- In the main() method, we define a String variable dateString, which holds a date-time in ISO-8601 format: "2024-11-20T15:30:00Z". This format is widely used for representing date and time.
- We then create an Instant object by calling Instant.parse(dateString). This method parses the given string into an Instant object. The Instant represents a specific point on the timeline in UTC (Coordinated Universal Time).
- Finally, we print the converted Instant using System.out.println(), which displays the Instant object, showing the date-time in the standard ISO-8601 format with the UTC time zone.
Convert String To Date In Java Using DateTimeFormatter Class
The DateTimeFormatter class in Java is a powerful tool for parsing and formatting date-time values. It allows you to define custom patterns for converting a String into a LocalDate, LocalDateTime, ZonedDateTime, etc. This is particularly useful when your date string is in a specific format that doesn't align with default parsing methods.
Working Approach:
To use DateTimeFormatter, you need to specify the format of the date string and then apply that format to the parse() method. You can use predefined formatters like DateTimeFormatter.ISO_DATE or create a custom format by specifying patterns (e.g., yyyy-MM-dd for year-month-day).
Code Example:
import java.time.LocalDate;
import java.time.format.DateTimeFormatter;
public class StringToDateTimeFormatter {
public static void main(String[] args) {
String dateString = "2024-11-20"; // Custom date format
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("yyyy-MM-dd"); // Custom format
LocalDate date = LocalDate.parse(dateString, formatter); // Parsing string to LocalDate
System.out.println("Converted Date: " + date); // Output the date
}
}
Output (set code file name as StringToDateTimeFormatter.java):
Converted Date: 2024-11-20
Explanation:
In the above code example-
- We begin by importing the necessary classes: LocalDate from the java.time package for working with dates, and DateTimeFormatter to define custom date formats.
- In the main() method, we define a String variable dateString containing a date in the format "2024-11-20". This format doesn't include time information, so it's a simple date representation.
- We create a DateTimeFormatter object named formatter using the method DateTimeFormatter.ofPattern("yyyy-MM-dd"). This defines the custom pattern "yyyy-MM-dd", which matches the format of the dateString.
- We then use LocalDate.parse(dateString, formatter) to convert the dateString into a LocalDate object. The formatter ensures that the string is parsed correctly according to the custom date pattern.
- Finally, we print the LocalDate object using System.out.println(), which outputs the converted date in the same format, displaying "2024-11-20".
Sharpen your coding skills with Unstop's 100-Day Coding Sprint and compete now for a top spot on the leaderboard!
Convert String To Date In Java Using SimpleDateFormat Class
The SimpleDateFormat class, available since the early versions of Java, is part of the java.text package and provides a way to format and parse dates in a customizable manner. It is a commonly used class, but it has been replaced by the DateTimeFormatter class in newer Java versions for better thread safety and flexibility. However, SimpleDateFormat remains widely used for backward compatibility.
Working Approach:
To use SimpleDateFormat, you need to create an instance of the class with a specific date pattern. The parse() method is then used to convert the string into a Date object based on that pattern. Unlike DateTimeFormatter, SimpleDateFormat works with the Date object, which is a legacy class for date representation in Java.
Code Example:
import java.text.SimpleDateFormat;
import java.util.Date;
public class StringToSimpleDateFormat {
public static void main(String[] args) {
String dateString = "2024-11-20"; // Custom date format
SimpleDateFormat formatter = new SimpleDateFormat("yyyy-MM-dd"); // Custom format
try {
Date date = formatter.parse(dateString); // Parsing string to Date
System.out.println("Converted Date: " + date); // Output the Date
} catch (Exception e) {
System.out.println("Error parsing date: " + e.getMessage());
}
}
}
Output (set code file name as StringToSimpleDateFormat.java):
Converted Date: Wed Nov 20 00:00:00 GMT 2024
Explanation:
In the above code example-
- We begin by importing SimpleDateFormat from the java.text package, which is used for parsing and formatting dates, and Date from java.util, which represents a specific point in time.
- Inside the main() method, we define a String variable dateString containing the date "2024-11-20", in a custom format.
- We create a SimpleDateFormat object named formatter using the constructor new SimpleDateFormat("yyyy-MM-dd"). This defines the custom date format "yyyy-MM-dd", which matches the structure of the dateString.
- Using a try-catch block, we attempt to parse the dateString into a Date object by calling formatter.parse(dateString). The parse() method converts the string to a Date object based on the custom format.
- If the parsing is successful, we print the Date object using System.out.println(). The output will show the date in the default format of the Date class, which is usually "Wed Nov 20 00:00:00 UTC 2024".
- If there's an error during parsing (e.g., if the string doesn't match the format), the catch block catches the exception and prints an error message explaining the issue.
When To Use Which Method To Convert String To Date In Java
Here's a detailed table that summarizes when to use each method for converting a string to a date in Java:
Method |
When to Use |
Advantages |
Limitations |
Using Instant Class |
- When working with timestamps in UTC (e.g., ISO-8601 format). - When you need to handle precise time (with timezone) and the string is in ISO-8601 format. |
- Simple and concise for UTC timestamps. - Automatically handles time zone information. |
- Limited to ISO-8601 format. - Doesn't support all date-time formats. |
Using DateTimeFormatter |
- When dealing with custom date-time formats (e.g., yyyy-MM-dd, dd/MM/yyyy). - When you need to work with modern date-time classes (LocalDate, LocalDateTime). |
- Highly flexible with custom formats. - Compatible with modern java.time API. |
- Slightly more complex for basic formats. - Requires careful formatting pattern. |
Using SimpleDateFormat |
- When working with legacy code or older versions of Java. - When the string follows a specific pattern like yyyy-MM-dd. |
- Flexible for custom date formats. - Easy to use in legacy systems. |
- Thread safety issues. - Deprecated in favor of DateTimeFormatter. |
Are you looking for someone to answer all your programming-related queries? Let's find the perfect mentor here.
Conclusion
Working with dates in Java can often be a necessity, whether it's for managing deadlines, event planning, or handling academic data. By understanding the different methods for converting strings into date objects, you can handle a wide range of scenarios in your projects. From using the Instant class for precise timestamps to leveraging DateTimeFormatter and SimpleDateFormat for custom formats, you now have the tools to easily work with date data in Java.
Remember, choosing the right method depends on the format of the string and the type of date object you need, so it’s essential to understand each approach and apply it accordingly. With these skills, you’ll be well-equipped to handle date manipulation in your Java applications, whether for class assignments or future projects!
Frequently Asked Questions
Q. What is the difference between Instant, LocalDate, and Date in Java?
- Instant: Represents a point in time (timestamp) in UTC. It's a part of the java.time package, introduced in Java 8. It's primarily used for precise time measurements and handles timestamps down to nanoseconds.
- LocalDate: Represents a date without time or timezone information (e.g., 2024-11-20). It’s part of the java.time package and is used when you don’t need time or timezone details, such as storing a birthdate.
- Date: A legacy class from the java.util package, representing a specific moment in time with millisecond precision. It's often used with older Java code but is being replaced by the more flexible and thread-safe java.time classes.
Q. What format should my date string be in for Instant.parse() to work?
The string passed to Instant.parse() must be in ISO-8601 format. It should look like this:
YYYY-MM-DDTHH:MM:SSZ (e.g., 2024-11-20T15:30:00Z).
The T separates the date from the time, and the Z denotes that the time is in UTC. This format is a requirement for Instant parsing, so you cannot use other formats directly.
Q. Can I use SimpleDateFormat with java.time classes like LocalDate?
No, SimpleDateFormat works with the legacy Date class and not the newer java.time classes like LocalDate, LocalDateTime, or Instant. If you are working with java.time classes, it is recommended to use DateTimeFormatter instead, as it is more flexible and supports the java.time API. However, if you need to convert a Date object to LocalDate or similar types, you can first convert the Date to an Instant and then to the java.time class you need.
Q. What happens if my date string does not match the expected format?
If the string does not match the expected date format, the parsing method (whether it's Instant.parse(), DateTimeFormatter.parse(), or SimpleDateFormat.parse()) will throw a DateTimeParseException or ParseException. To handle this, you should use a try-catch block to catch and handle these exceptions, providing meaningful feedback or default values.
Q. Which method is better: DateTimeFormatter or SimpleDateFormat?
- DateTimeFormatter: This is the recommended method for parsing and formatting dates in modern Java (Java 8 and above). It is part of the java.time package, is thread-safe, and works with the new date-time API (LocalDate, LocalDateTime, ZonedDateTime).
- SimpleDateFormat: While SimpleDateFormat is still widely used, it is considered outdated for newer projects. It’s part of the java.text package and works with the Date class. However, it is not thread-safe and is less flexible than DateTimeFormatter.
In most cases, DateTimeFormatter should be preferred due to its modern design and better compatibility with the java.time package.
Q. How do I handle time zones when converting a string to a date?
When working with time zones, you should use ZonedDateTime or OffsetDateTime along with DateTimeFormatter to parse strings that include time zone information. For example, a string like 2024-11-20T15:30:00+02:00 (with the offset +02:00) can be parsed into a ZonedDateTime using a custom DateTimeFormatter. The Instant class, however, only represents a time in UTC and doesn't handle time zones directly, so you'd need to convert between time zones if you need localized times.
With this, we conclude our discussion on the different techniques for converting string to date in Java. Here are a few other topics that you might be interested in reading:
- 15 Popular JAVA Frameworks Use In 2023
- What Is Scalability Testing? How Do You Test Application Scalability?
- Top 50+ Java Collections Interview Questions
- 10 Best Books On Java In 2024 For Successful Coders
- Difference Between Java And JavaScript Explained In Detail
- Top 15+ Difference Between C++ And Java Explained! (+Similarities)
I’m a Computer Science graduate with a knack for creative ventures. Through content at Unstop, I am trying to simplify complex tech concepts and make them fun. When I’m not decoding tech jargon, you’ll find me indulging in great food and then burning it out at the gym.
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
Comments
Add comment