Tips For Cracking Coding Interview Questions
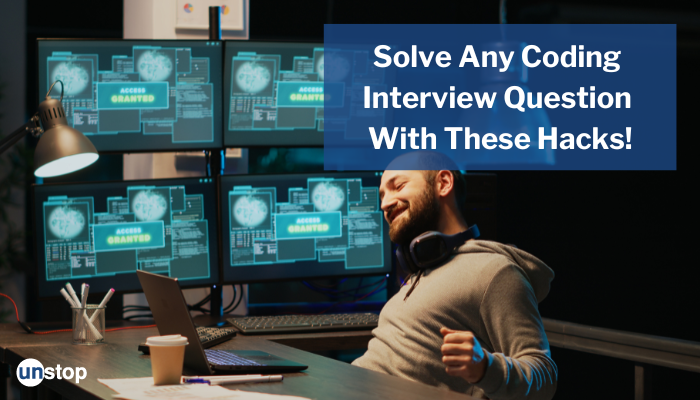
Many candidates fear getting stuck on a question during coding interviews with their dream tech company. They dread the thought of not being able to find a solution to the problems presented to them during the programming job interviews and missing their chance of bagging their dream job. Fret not, there is a structured way of approaching any coding question and solving it like a pro. In this article, we have shared how you can approach a coding problem and easily find answers to it. We have included some quick hacks/tips and examples that will give you an overall idea of topics that come up in programming job interviews. These tips will help you hit the ball out of the park!
How to easily find solutions to the coding interview questions?
These are some problem-solving approaches you can use in your programming job interviews. Irrespective of the level of programming job you are applying for, your interviewer is sure to throw some curveballs at you, but these tips and hacks will make things easier for you.
Draw out the problem
Drawing diagrams could be an easy way out! A significant part of coding is understanding how the internal state of programs changes. Diagrams can be useful tools for representing the state of internal data structure. A visual representation will help you approach the problem in a step-by-step manner. This technique is ideal if the question requires you to input trees, graphs, matrices, and linked lists.
Quick hack:
If you are asked to return all elements of a matrix in spiral order, you can draw out the matrix and the steps your iterator needs to take in each direction. This will help you in identifying the pattern and then you can easily work your way out.
Give coding a break
Do not dive directly into writing a code as soon as you see the problem. Give the problem a thought from a non-coder's perspective, and think about how they'd find a solution to it. This is called solving the problem by hand.
Sometimes solutions are just a code version of the manual approach. If you find a concrete set of rules around this approach that can work for every example or variation, you can easily write code for it. This approach can be a great starting point.
Quick hack:
Think about how you can validate if a tree is a valid Binary Search Tree without writing any code. You can approach this question in this manner--- First, check if the left subtree contains only values less than the root. Next, check that the right subtree contains only values bigger than the root, then repeat for each node. Voila! now you just have to process this manual approach into code.
Break the question into smaller parts
To deal with a coding problem that is lengthy, begin with a high-level function and break it down into smaller functions and then solve each one of them separately. This will prevent you from getting overwhelmed by the details of doing everything at once. This will also give you a systematic structure you can work with.
This will also give interviewers an idea that you have an approach, even if you fail to finish the coding of all the smaller functions.
Quick hack:
If you encounter any Group Anagrams problem, you can break it down into two parts. The two parts can be hashing a string and grouping the strings together. This will allow you to solve each part separately with independent implementation details. Here is an example code for the same:
def group_anagrams(strings):
def hash(string):
#Fill in later
pass
def group_strings(strings_hashes):
# Fill in later
pass
strings_hashes = [(string.hash(string)) for string in strings]
return group_strings(strings_hashes)
After this bifurcation, proceed to fill in the implementation of each function. Note that sometimes, even the most efficient solutions will require you to break some abstractions and do multiple operations in one pass of the input. This could be one possible path forward if your interviewer asks you to optimize based on your well-abstracted solution.
Try to apply basic algorithms problem-solving techniques for common coding
Algorithm questions are smaller and can be easily solved within the duration of the interview. Most concepts taught in college come to the rescue in solving coding questions during the interviews.
A hacky approach to solving coding interview questions is putting the common data structures and basic algorithms to use. The number of common data structures and algorithms is finite, so you can easily work with them and apply them to most coding interview questions.
Quick hack:
Keep these data structures handy:
If you are asked to make lookup efficient, you can use Hash Maps. If you are given data as associations between entities then you might be able to model the question as a graph and can employ basic graph algorithms to solve the coding interview question.
To parse a string with nested properties, like a mathematical equation, you can use stack and queue. Questions involving scheduling/ordering based on some priority can be solved by heap. If you are asked to store strings in a space-efficient manner and look for existing strings, tree/trie will be of use.
Pseudocode is the way to go!
Writing a pseudocode for your common questions presented during coding interviews will help you reach the solution effectively. A pseudocode can help you define the structures of your code and will help you write every line of the code that you need. By reading pseudocode you can get a clear idea of all the codes you are supposed to write.
Do not skip this step as this can make writing your final code a lot easier. Towards the end, all you have to do is translate the pseudocode into actual code.
Quick hack:
If you are asked to write the code that returns the array of even numbers, you can go with this pseudocode:
function getEvenNumbers
evenNumbers = [ ]
for i = 0 to i = length of evenNumbers
if (element % 2 === 0)
add to that to the array evenNumbers
return evenNumbers
Top 10 rules to follow to solve any type of question in your coding interviews!
- If you are dealing with top/maximum/minimum/closest 'K' elements among 'N' elements, you can use a Heap.
- If the given input is a sorted array or a list, you will either be using Binray Search or the Two Pointers strategy.
- If you need to try all combinations or permutations of the input, you can either use Backtracking or Breadth First Search.
- Most of the questions related to Trees or Graphs can be solved either through Breadth First Search or Depth First Search.
- Every recursive solution can be converted to an iterative solution using a Stack.
- For a problem involving arrays, if there is a solution in O(n^2) time and O(1) space, there must exist two other solutions:
1) Using a HashMap or a Set for O(n) time and O(n) space.
2) Using sorting for O(n log n) time and O(1) space. - If a problem calls for optimization (e.g., maximization or minimization), it is ideal to use Dynamic Programming.
- If you need to find some common substring among a set of strings, you will be using a HashMap or a Trie.
- If you need to search/manipulate a bunch of strings, resort to Trie as it is the best data structure.
- If the problem is related to a LinkedList and you can't use extra space, then resort to the Fast & Slow Pointer approach.
- If you have to return the preorder traversal of its nodes' values when a binary tree is given, always use the stack.
- To find the lowest common ancestor (LCA) of a binary tree, consider its two nodes--- n1 and n2.
- If you are looking for the optimal solution for integer array/string questions, use the sliding window concept.
- Remember that efficiency and optimization go a long way, as opposed to brute-force solutions.
Apart from problem-solving skills, prepare a comprehensive roadmap to keep yourself updated with the behavioral interview questions. Many candidates overlook behavioral interview questions but employers ask them to determine if the candidate is a good fit for the company culture. The way you answer these behavioral interview questions contribute greatly to your chances of getting hired. Click here if you wish to learn popular behavioral interview questions and how to answer them.
You might also be interested in:
- 51 Competitive Coding Questions (With Solutions) - Do NOT Skip These!
- Most Common Programming Interview Questions With Answers 2022
- Competitive Coding: Frequently Asked Questions | Tips For Competitive Coding Events
- Importance Of C Programming Interview Questions
- Most-asked Microsoft Interview Questions And Answers
Comments
Add commentLogin to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Blogs you need to hog!
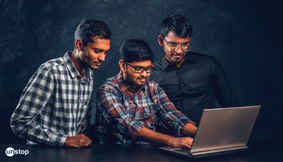
This Is My First Hackathon, How Should I Prepare? (Tips & Hackathon Questions Inside)
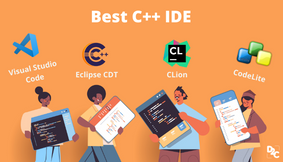
10 Best C++ IDEs That Developers Mention The Most!
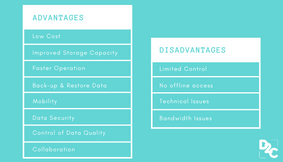
Advantages and Disadvantages of Cloud Computing that you should know!
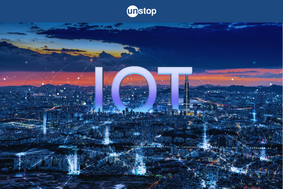
Dai Software 1 year ago