Wipro Coding Questions 2025: Top 5 Coding Problems with Solutions
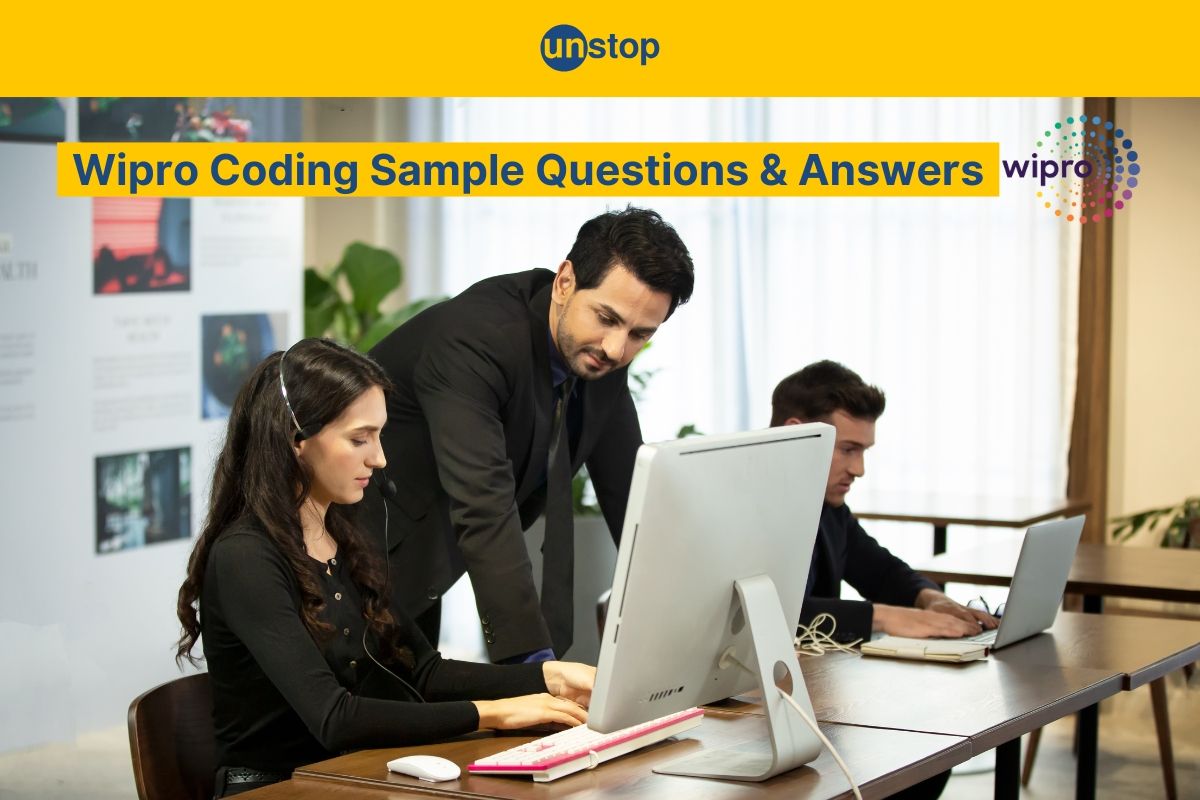
Securing a position at Wipro as a global IT and consulting leader is a highly competitive process. Among the essential stages of Wipro's recruitment journey is the coding test—an assessment that evaluates a candidate’s programming skills, logical reasoning, and problem-solving abilities.
Fresh graduates preparing for this stage can benefit greatly from understanding the types of coding questions Wipro asks, practising with previous year's questions, and getting familiar with commonly repeated coding problems.
Wipro Coding Test Overview
The coding test consists of one or two questions that assess your ability to solve problems using efficient algorithms and data structures. Here’s a quick overview of what to expect:
Test Section |
Topics Covered |
Duration |
Difficulty |
Coding |
Arrays, Strings, Sorting, Recursion, Dynamic Programming |
45-60 mins |
Moderate to Advanced |
Candidates may use languages such as C, C++, Java, or Python to solve coding challenges.
Types of Coding Questions in Wipro's Coding Test
Understanding the kinds of coding questions you may encounter can help in focusing your preparation. Here’s a breakdown of the typical coding topics in Wipro’s test:
Data Structures and Algorithms
Mastering data structures and algorithms is essential. Questions usually involve using these to solve practical coding problems.
Topic |
Sample Question |
Arrays |
Find the smallest missing positive integer in an unsorted array. |
Linked Lists |
Reverse a linked list and explain its time complexity. |
Trees |
Find the depth of a binary tree. |
Graphs |
Implement a breadth-first search (BFS) traversal of a graph. |
Stacks/Queues |
Check for balanced parentheses in an expression. |
String Manipulation
String manipulation tests your ability to work with characters and substrings efficiently.
Topic |
Sample Question |
String Reversal |
Reverse the words in a given sentence while keeping the order. |
Check if a string is a palindrome. |
|
Anagrams |
Verify if two strings are anagrams. |
Pattern Matching |
Implement a function to locate a substring in a main string. |
Sorting and Searching
Sorting and searching are critical in efficient data handling and are often tested.
Topic |
Sample Question |
Binary Search |
Find an element in a sorted array using binary search. |
Quick Sort |
Sort an array using quick sort. |
Merge Sort |
Implement merge sort for an integer array. |
Sorting with Custom Comparisons |
Sort an array of strings by length without using built-in functions. |
Recursion and Dynamic Programming
These questions test your approach to solving problems with recursive methods and optimized solutions.
Topic |
Sample Question |
Fibonacci Series |
Generate the nth Fibonacci series using recursion. |
Knapsack Problem |
Solve the 0/1 knapsack problem using dynamic programming. |
Subset Sum |
Check if a subset with a given sum exists in an array. |
Longest Common Subsequence |
Find the longest common subsequence between two strings. |
Are you preparing for the upcoming Wipro Coding test? Click here to access coding practice sessions from moderate to challenging levels.
Wipro Coding Questions from Previous Years
Studying previous years’ questions can provide insight into frequently asked topics and question formats. Here are some typical coding questions from recent Wipro assessments:
Year |
Coding Question |
Topic |
2023 |
Find the nearest greater element to the right for each element in an array. |
Arrays |
2022 |
Calculate the minimum deletions to make a string a palindrome. |
Strings |
2021 |
Check if two binary trees are identical. |
Trees |
2020 |
Determine if a subset with a target sum exists in an integer set. |
Dynamic Programming |
2019 |
Find the first non-repeating character in a string. |
Strings |
Top 5 Wipro Coding Questions with Solutions
Problem Statement 1
Alice challenged Bob to write the same word as his on a typewriter. Both are kids and are making some mistakes in typing and are making use of the ‘#’ key on a typewriter to delete the last character printed on it. An empty text remains empty even after backspaces.
Input Format
The first line contains a string typed by Bob.
The second line contains a string typed by Alice.
Output Format
The first line contains ‘YES’ if Alice is able to print the exact words as Bob , otherwise ‘NO’.
Constraints
1 <= Bob.length
Alice.length <= 10^5
Bob and Alice only contain lowercase letters and '#' characters.
Solution C++
Solution 2 Java
Solution Python
Problem Statement 2
One day, Jack finds a string of characters. He is very keen to arrange the characters in reverse order, i.e., first characters become the last characters, second characters become the second-last characters, and so on.
Now, he wants your help to find the kth character from the new string formed after reversing the original string.
Note: String contains only lowercase Latin letters.
Input Format
The first line contains two integers n, k — the length of the array and the value of k, respectively.
The second line contains a string containing n characters.
Output Format
Print a single line containing the kth character of the string.
Constraints
1 ≤ k ≤ n≤ 10^6
Solution C++
Solution Java
Solution Python
Problem Statement 3
There are n spaceships a given light years away from the Earth and traveling to reach a distant star system at k lightyears away from Earth. You are given two integer arrays, position and speed, both of length n, where
P[i] is the current distance of the ith spaceship
S[i] is the speed of the ith spaceship in lightyears per year.
As the spaceships travel toward the star system, an interesting phenomenon occurs: when a faster spaceship catches up to a slower one, it reduces its speed to match the slower spaceship's speed, forming a fleet. A fleet is a group of one or more spaceships that travel together at the same speed.
Given this information, determine the number of distinct spacecraft fleets that will arrive at the destination star system. A fleet is considered distinct if no other fleet arrives at the destination at the same time while travelling together.
Input Format
The first line contains an integer 'n', representing the total number of spaceships.
The second line contains an integer 'k', representing the distance of the star system from Earth.
The third line contains 'n' space-separated integers denoting the current distance of the i-th spaceship from Earth.
The fourth line contains 'n' space-separated integers denoting the speed of the i-th spaceship.
Output Format
Return the number of spacecraft fleets that will arrive at the destination.
Constraints
1 <= n <= 10^5
0 < k <= 10^6
0 <= P[i] < target
0 < S[i] <= 10
Solution C++
Solution Java
Solution Python
Problem Statement 4
During the night of the street race, there are N cars on the street, each with a given speed. The police will be alerted if the total number of speed variation pairs exceeds a certain threshold, X.
A pair of cars (i, j) has a speed variation if the absolute difference in their speeds is at least K. The total speed variation count is the number of such pairs where the speed difference is at least K.
You need to determine whether the total speed variation count is greater than the given threshold, X. If it is, the police will be alerted; otherwise, they will not.
Input Format
The first line contains three space-separated integers: N, the number of cars; K, the minimum speed difference for variation; and X, the alert threshold.
The second line contains N space-separated integers representing the speeds of the cars.
Output Format
Print YES if the total speed variation count exceeds X; otherwise, print NO.
Constraints
1 <= N <= 2 * 10^5
1 <= K, X <= 10^8
1 <= S[i] <= 10^8
Solution C++
Solution Java
Solution Python
Problem Statement 5
You are given a string s of length n and an integer k. You can choose one of the first k characters of the string and move it to the end of the string.
Your task is to return the lexicographically smallest string you can obtain after applying this operation any number of times.
Note: The string s consists of lowercase English letters only.
Input Format
The first line contains the string s.
The second line contains the integer k.
Output Format
Print the lexicographically smallest string that can be obtained after applying the described operation.
Constraints
1 ≤ k ≤ n≤ 10^3
Solution C++
Solution Java
Solution Python
Frequently Repeated Coding Questions
Some topics appear repeatedly in Wipro’s coding assessments as they reflect foundational programming skills. Here’s a list of high-frequency topics:
- Array Manipulations: Common questions include finding the maximum or minimum element, identifying patterns, and rotating arrays.
- String Operations: Repeated questions include checking for palindromes, reversing strings, and finding unique characters.
- Sorting and Searching Algorithms: Questions on binary search and sorting techniques like quicksort are popular.
- Recursive and Dynamic Programming Problems: Fibonacci calculations, subset sums, and longest common subsequences.
- Linked List Manipulations: Reversal of linked lists, finding cycles, and merging sorted linked lists.
Tips to Ace the Wipro Coding Test
- Master Data Structures and Algorithms: Strengthen basics like arrays, linked lists, and trees for faster problem-solving.
- Focus on Time Complexity: Efficient code can set you apart; always aim for the least time and space complexity.
- Get Comfortable with Recursion and Dynamic Programming: Many advanced questions fall into these categories, so consistent practice can make them easier.
- Attempt Mock Tests: Simulate the actual test environment with time constraints to manage performance anxiety and gain confidence.
Conclusion
The Wipro coding test is a pivotal part of the recruitment process. By familiarising yourself with Wipro coding questions, previous year questions, and commonly repeated coding challenges, you can systematically improve your problem-solving skills and coding efficiency.
Consistent practice and a thorough understanding of fundamental data structures and algorithms are key to excelling. With the right preparation, you’ll be well-positioned to tackle Wipro’s coding challenges and advance towards a promising career.
Disclaimer: While we have gathered as much information from Wipro's official website as possible, we have included sources gathered from available online sources. Readers are advised to check and stay updated with the official website.
Frequently Asked Questions (FAQs)
1. What programming languages can I use in the Wipro coding test?
Wipro allows languages like C, C++, Java, and Python during their coding assessments.
2. What types of coding questions are asked in Wipro's coding test?
Topics often include arrays, strings, dynamic programming, recursion, sorting, and searching algorithms.
3. Where can I find Wipro’s previous year coding questions?
You can find previous year questions on coding practice sites, placement prep platforms, and forums.
4. Is the Wipro coding test timed, and what’s the difficulty level?
Yes, it’s timed (45-60 minutes) with moderate to advanced questions covering data structures and algorithms.
5. Are coding test questions in Wipro repeated over the years?
While the exact questions may vary, similar topics like arrays, strings, and dynamic programming recur often.
Suggested reads:
- Tech Mahindra Placement for Freshers 2025: Exam Syllabus & Pattern
- TCS NQT Hiring Process 2025: A Comprehensive Guide for Freshers
- TCS Codevita Interview Experience, Process, and Preparation Tips
- TCS CodeVita Syllabus for Season 12: Latest Exam Pattern and Tips
- CoCubes Previous Year Paper Structure with Tips for Freshers 2025
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Blogs you need to hog!
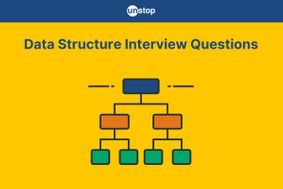
55+ Data Structure Interview Questions For 2024 (Detailed Answers)
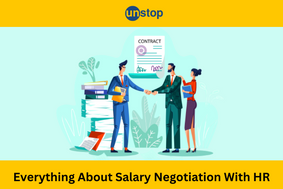
How To Negotiate Salary With HR: Tips And Insider Advice
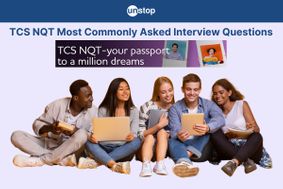
Best 80+ TCS NQT Most Commonly Asked Interview Questions for 2025
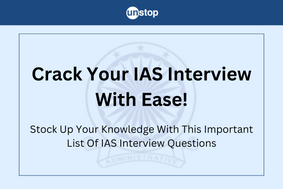
Comments
Add comment