Table of content:
- Printing Arrays In Java
- Using A For Loop To Print Arrays In Java
- Using A While Loop To Print Arrays In Java
- Using A For-each Loop To Print Arrays In Java
- Using Arrays.toString() Method To Print Arrays In Java
- Using Arrays.deepToString() Method To Print Arrays In Java
- Using Arrays.asList() Method To Print Arrays In Java
- Using Iterator Interface To Print Arrays In Java
- Using Stream API To Print Arrays In Java
- Tips And Best Practices For Printing Array In Java
- Conclusion
- Frequently Asked Questions
8 Methods To Print Array In Java Explained (With Tips & Examples)
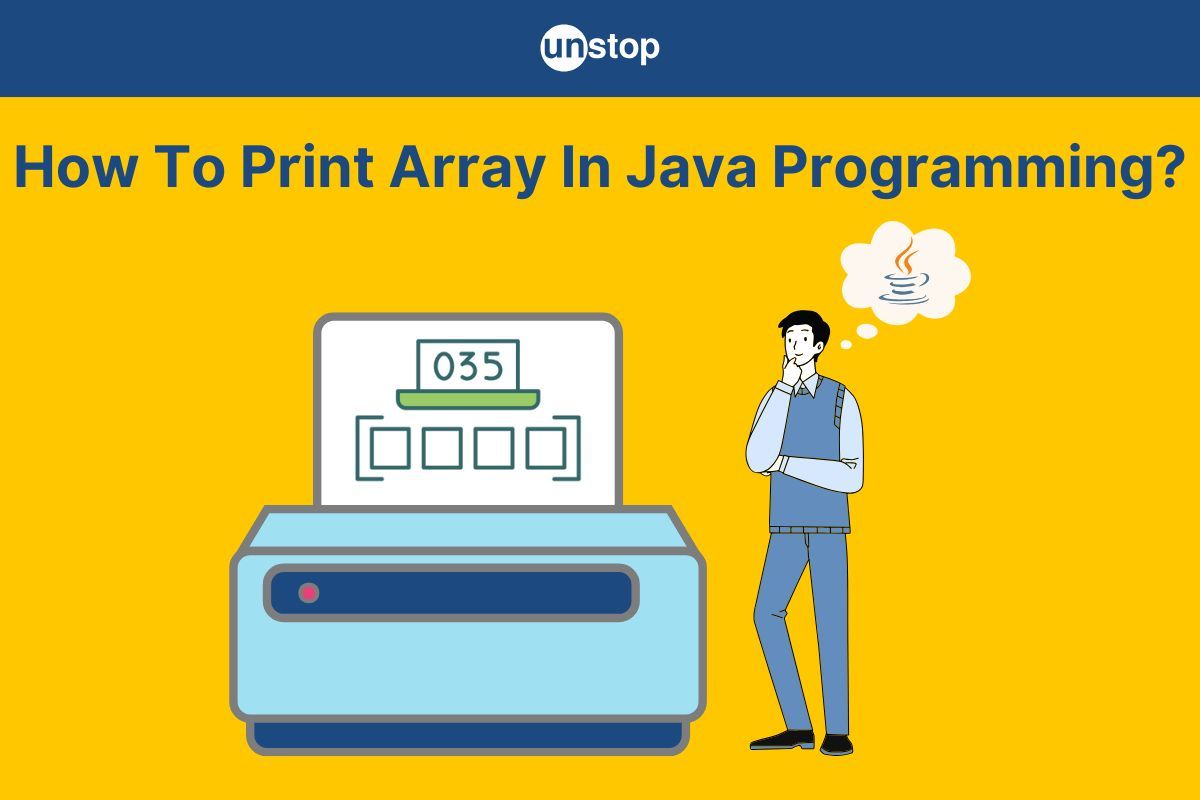
Printing an array in Java is a common task, whether for debugging or displaying data. While Java doesn’t offer a direct method to print arrays as a whole, it provides various techniques to achieve this. In this article, we'll explore different ways to print arrays in Java, from basic loops to using utility methods, making the process straightforward and efficient.
Printing Arrays In Java
When working with arrays in Java programming, we often need to display their contents for debugging, logging, or simply understanding the program's flow. However, Java does not provide a direct way to print arrays using the System.out.println() method, as doing so prints the array's memory reference rather than its actual contents.
To address this, we can leverage various techniques and utilities to print arrays effectively. Some of the commonly used methods are:
- Java for loop
- Java while loop
- Java for-each loop
- Java Arrays.toString() method
- Java Arrays.deepToString() method
- Java Arrays.asList() method
- Java Iterator Interface
- Java Stream API
We will now understand each of these methods in detail, along with code examples.
Using A For Loop To Print Arrays In Java
The for loop is a traditional way to traverse an array by accessing elements through their indices. It provides complete control over iteration but requires manual management of the loop variable.
Syntax:
for (int i = 0; i < array.length; i++) {
// Access array[i]
}
Code Example:
public class PrintArrayForLoop {
public static void main(String[] args) {
int[] numbers = {10, 20, 30, 40, 50};
for (int i = 0; i < numbers.length; i++) {
System.out.print(numbers[i] + " ");
}
}
}
Output:
10 20 30 40 50
Explanation:
In the above code example-
- We start by defining a class named PrintArrayForLoop, which holds our code.
- Inside the main() method, we declare and initialize an array called numbers containing five integers: 10, 20, 30, 40, 50.
- To iterate through the array, we use a for loop. The loop begins with i set to 0, which represents the index of the first element in the array.
- The loop condition i < numbers.length ensures we stop once i reaches the length of the array, preventing out-of-bounds errors.
- In each iteration, we use System.out.print(numbers[i] + " ") to print the current element of the array followed by a space.
- As i increments by 1 in each loop iteration, we move through the array elements sequentially, printing all of them on the same line.
- Once the loop completes, all the array elements are displayed in a single line with spaces in between.
Using A While Loop To Print Arrays In Java
The while loop iterates through an array based on a condition. It is ideal when the number of iterations is not predetermined.
Syntax:
int i = 0;
while (i < array.length) {
// Access array[i]
i++;
}
Code:
public class PrintArrayWhileLoop {
public static void main(String[] args) {
int[] numbers = {10, 20, 30, 40, 50};
int i = 0;
while (i < numbers.length) {
System.out.print(numbers[i] + " ");
i++;
}
}
}
Output:
10 20 30 40 50
Explanation:
In the above code example-
- We begin by defining a class named PrintArrayWhileLoop, where our program resides.
- Inside the main() method, we declare and initialize an array called numbers containing five integers: 10, 20, 30, 40, 50.
- Next, we declare an integer variable i and initialize it to 0, representing the index of the first array element.
- A while loop is used to iterate through the array. The loop condition i < numbers.length ensures that the loop runs as long as i is within the bounds of the array.
- Inside the loop, we use System.out.print(numbers[i] + " ") to print the current element of the array followed by a space.
- After printing, we increment i by 1 using i++, allowing us to move to the next element in the array.
- The loop continues until all elements are printed, resulting in the array elements being displayed on the same line with spaces in between.
Using A For-each Loop To Print Arrays In Java
The for-each loop simplifies iteration by directly accessing each element without requiring an index, making it cleaner and more readable.
Syntax:
for (int element : array) {
// Access element
}
Code Example:
public class PrintArrayForEachLoop {
public static void main(String[] args) {
int[] numbers = {10, 20, 30, 40, 50};
for (int num : numbers) {
System.out.print(num + " ");
}
}
}
Output:
10 20 30 40 50
Explanation:
In the above code example-
- We define a class named PrintArrayForEachLoop, where our program logic is written.
- Inside the main() method, we declare and initialize an array called numbers containing five integers: 10, 20, 30, 40, 50.
- To iterate through the array, we use a for-each loop, which simplifies the process by directly accessing each element in the array.
- In the loop, the variable num sequentially takes the value of each element in the array during each iteration.
- We use System.out.print(num + " ") to print the current value of num followed by a space.
- The loop automatically progresses through all the elements in the array, printing each one on the same line with spaces in between.
Using Arrays.toString() Method To Print Arrays In Java
This method converts the array into a string representation, displaying all elements in a single line. It is concise and works well for 1D arrays.
Syntax:
Arrays.toString(array)
Code Example:
import java.util.Arrays;
public class PrintArrayToString {
public static void main(String[] args) {
int[] numbers = {10, 20, 30, 40, 50};
System.out.println(Arrays.toString(numbers));
}
}
Output:
[10, 20, 30, 40, 50]
Explanation:
In the above code example-
- We start by importing the java.util.Arrays package, which provides utility methods for working with arrays.
- The program is contained within a class named PrintArrayToString.
- Inside the main() method, we declare and initialize an array called numbers containing five integers: 10, 20, 30, 40, 50.
- To print the array, we use the Arrays.toString(numbers) method. This method converts the array into a string representation where elements are enclosed in square brackets and separated by commas.
- We pass this string representation to System.out.println, which displays the entire array in a readable format: [10, 20, 30, 40, 50].
- This approach provides a quick and concise way to display all elements of the array in a structured manner.
Using Arrays.deepToString() Method To Print Arrays In Java
Specifically designed for multidimensional arrays, this method provides a formatted string representation, showing nested arrays clearly.
Syntax:
Arrays.deepToString(array)
Code Example:
import java.util.Arrays;
public class PrintArrayDeepToString {
public static void main(String[] args) {
int[][] numbers = {{10, 20}, {30, 40}, {50}};
System.out.println(Arrays.deepToString(numbers));
}
}
Output:
[[10, 20], [30, 40], [50]]
Explanation:
In the above code example-
- We start by importing the java.util.Arrays package, which includes utility methods for handling arrays.
- The program is defined within a class named PrintArrayDeepToString.
- Inside the main() method, we declare and initialize a two-dimensional array called numbers containing three sub-arrays: {10, 20}, {30, 40}, and {50}.
- To print the two-dimensional array, we use the Arrays.deepToString(numbers) method. This method converts multi-dimensional arrays into a readable string format, displaying each sub-array enclosed in square brackets and separating elements with commas.
- The resulting string representation is passed to System.out.println, which prints the entire two-dimensional array in a clear format: [[10, 20], [30, 40], [50]].
- This method is especially useful for displaying nested arrays in a structured and easy-to-read manner.
Explore this amazing course and master all the key concepts of Java programming effortlessly!
Using Arrays.asList() Method To Print Arrays In Java
This method converts an array into a List, which can be printed directly. It’s useful when transitioning between array and collection operations.
Syntax:
Arrays.asList(array)
Code Example:
import java.util.Arrays;
public class PrintArrayAsList {
public static void main(String[] args) {
String[] fruits = {"Apple", "Banana", "Cherry"};
System.out.println(Arrays.asList(fruits));
}
}
Output:
[Apple, Banana, Cherry]
Explanation:
In the above code example-
- We begin by importing the java.util.Arrays package, which provides helpful methods for working with arrays.
- The program is written inside a class named PrintArrayAsList.
- In the main() method, we declare and initialize an array called fruits, containing three strings: "Apple", "Banana", and "Cherry".
- To convert the array into a list, we use the Arrays.asList(fruits) method. This method converts the array into a fixed-size list, allowing us to treat the array as a list for easier manipulation and display.
- The resulting list is then printed using System.out.println, which outputs the list in a readable format: [Apple, Banana, Cherry].
- This method provides a simple way to work with arrays as lists and display their contents in a structured manner.
Using Iterator Interface To Print Arrays In Java
The Iterator interface provides a way to traverse collections, including arrays converted to lists, while offering more flexibility in traversal.
Syntax:
Iterator<String> it = collection.iterator();
while (it.hasNext()) {
// Access it.next()
}
Code Example:
import java.util.*;
public class PrintArrayIterator {
public static void main(String[] args) {
List<String> fruits = Arrays.asList("Apple", "Banana", "Cherry");
Iterator<String> it = fruits.iterator();
while (it.hasNext()) {
System.out.print(it.next() + " ");
}
}
}
Output:
Apple Banana Cherry
Explanation:
In the above code example-
- We begin by importing the java.util.* package, which includes useful classes like List, Arrays, and Iterator.
- The program is written within a class named PrintArrayIterator.
- Inside the main() method, we create a list called fruits using Arrays.asList and initialize it with three strings: "Apple", "Banana", and "Cherry".
- We then create an Iterator object it by calling fruits.iterator(), which allows us to iterate through the list.
- Using a while loop, we check if there are more elements to iterate over with it.hasNext(). If true, we use it.next() to get the next element from the list.
- In each iteration, we print the current element followed by a space using System.out.print(it.next() + " ").
- The loop continues until all elements have been accessed, and the list is printed with each element separated by a space.
Sharpen your coding skills with Unstop's 100-Day Coding Sprint and compete now for a top spot on the leaderboard!
Using Stream API To Print Arrays In Java
Introduced in Java 8, Streams provide a functional programming approach to process and print arrays with minimal boilerplate code.
Syntax:
Arrays.stream(array).forEach(element -> {
// Process element
});
Code Example:
import java.util.Arrays;
public class PrintArrayStream {
public static void main(String[] args) {
int[] numbers = {10, 20, 30, 40, 50};
Arrays.stream(numbers).forEach(num -> System.out.print(num + " "));
}
}
Output:
10 20 30 40 50
Explanation:
In the above code example-
- We begin by importing the java.util.Arrays package, which provides methods for working with arrays.
- The program is defined within a class named PrintArrayStream.
- Inside the main() method, we declare and initialize an array called numbers containing five integers: 10, 20, 30, 40, 50.
- To process the array using streams, we call Arrays.stream(numbers), which converts the array into a stream.
- We then use the forEach method on the stream, which allows us to perform an action on each element. The action is defined by a lambda expression num -> System.out.print(num + " "), which prints each element followed by a space.
- The stream processes each element in the array one by one, and the entire array is printed in a single line, with elements separated by spaces.
- This approach leverages Java's stream API to efficiently process and print array elements.
Tips And Best Practices For Printing Array In Java
When working with arrays in Java, printing their contents can sometimes be tricky, especially when dealing with large or multidimensional arrays. Here are some tips and best practices to ensure you print arrays efficiently and clearly:
- Use Arrays.toString() for 1D Arrays: Best for simple, one-dimensional arrays to quickly get a formatted string representation.
- Use Arrays.deepToString() for Multidimensional Arrays: Ideal for printing multidimensional arrays in a readable format, showing nested arrays clearly.
- Avoid Manual Loops for Small Arrays: For small arrays, prefer Arrays.toString() for simplicity instead of using for or while loops.
- Use Stream API for Functional Style: Leverage Streams for complex or functional operations when you need additional transformations before printing.
- Use forEach() for Collection-Based Arrays: For arrays backed by collections like List, Set, or any Collection, use forEach() for concise iteration.
- Avoid Printing Large Arrays Entirely: For large arrays, print only a portion (e.g., first few elements) or use a message indicating the array is too large.
- Ensure Proper Formatting: Keep the output clean, avoiding extra spaces or unclear formatting, and focus on making the array content readable.
- Use Enhanced for-each Loop for Readability: Prefer for-each loops for cleaner and more readable code when you don't need the index.
- Use StringBuilder for Custom Formatting: If you need custom formatting or a specific delimiter, use StringBuilder to efficiently build the string for printing.
- Handle Null and Empty Arrays: Always check if the array is null or empty before attempting to print, to avoid errors and provide meaningful output.
- Limit Output for Debugging: When debugging, print only a small part of the array to avoid cluttering the output, especially with large arrays.
Are you looking for someone to answer all your programming-related queries? Let's find the perfect mentor here.
Conclusion
In Java, printing arrays may seem straightforward, but choosing the right method can make a significant difference in readability and efficiency. From simple approaches like Arrays.toString() for 1D arrays to more advanced techniques like Stream API for complex operations, understanding when and how to use each method is key. By following best practices such as handling large arrays appropriately, ensuring proper formatting, and considering performance implications, you can make array printing both effective and clear. These techniques not only improve the clarity of your code but also enhance its maintainability and ease of debugging.
Frequently Asked Questions
Q. What is the simplest way to print a one-dimensional array in Java?
The simplest way to print a one-dimensional array in Java is by using the Arrays.toString() method. This method converts the array into a string format where each element is separated by commas and enclosed in square brackets. It is concise and efficient for 1D arrays.
Q. How do I print a multidimensional array in Java?
For multidimensional arrays (like 2D or 3D arrays), the Arrays.deepToString() method is recommended. Unlike Arrays.toString(), which only works for 1D arrays, Arrays.deepToString() will handle nested arrays and print them in a readable, formatted manner.
Q. Can I print large arrays without cluttering the output?
Yes, for large arrays, it’s a good practice to print only a portion of the array to avoid overwhelming the output. You can use methods like Arrays.copyOfRange() to print a subset of the array. Alternatively, you can print a message indicating that the array is too large for a full display.
Q. What should I do if the array is null or empty?
Before attempting to print an array, always check if it is null or empty. If the array is null, you can print a message like "Array is null." If the array is empty, print a message such as "Array is empty." This helps avoid NullPointerException and provides meaningful output.
Q. Is using a for-each loop better than a traditional for loop for printing arrays?
The for-each loop is often preferred over a traditional for loop for printing arrays because it is cleaner, more readable, and eliminates the need to manually manage the array index. It’s particularly useful when you don’t need the index and want a concise solution.
Q. Can I format the array output in a custom way?
Yes, you can use a StringBuilder or the Stream API to create custom formatting for printing arrays. These tools allow you to add delimiters, adjust the structure, or apply any custom logic before printing. For example, you can join array elements with a specific separator or format the output in a structured manner.
With this, we come to an end of our discussion on how to print an array in Java. Here are a few other topics that you might be interested in reading:
- Convert String To Date In Java | 3 Different Ways With Examples
- Final, Finally & Finalize In Java | 15+ Differences With Examples
- Super Keyword In Java | Definition, Applications & More (+Examples)
- How To Find LCM Of Two Numbers In Java? Simplified With Examples
- How To Find GCD Of Two Numbers In Java? All Methods With Examples
- Volatile Keyword In Java | Syntax, Working, Uses & More (+Examples)
I’m a Computer Science graduate with a knack for creative ventures. Through content at Unstop, I am trying to simplify complex tech concepts and make them fun. When I’m not decoding tech jargon, you’ll find me indulging in great food and then burning it out at the gym.
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
Comments
Add comment