Java Programming Language Table of content:
Lean How To Find Leap Year Program In Java (With Code Examples)
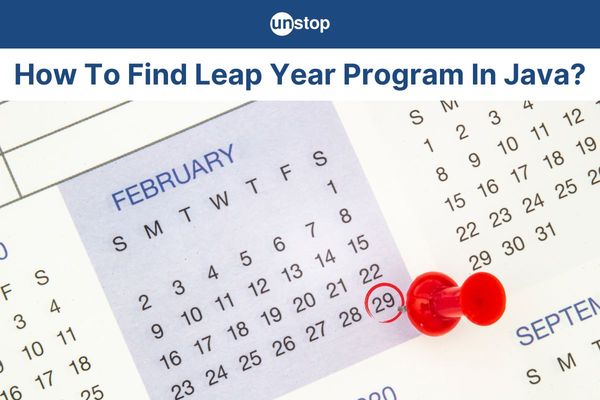
A leap year is a year that is evenly divisible by 4, except for years that are both divisible by 100 and not divisible by 400. This adjustment ensures that our calendar remains synchronized with the Earth's revolutions around the Sun.
In this article, we will explore how to write a Java program to check whether a given year is a leap year. We will break down the logic, discuss different approaches, and provide step-by-step implementations to ensure clarity.
Understanding The Leap Year Concept
A leap year exists to keep our calendar in sync with Earth's orbit around the Sun. While we typically consider a year to be 365 days, Earth's actual journey around the Sun takes approximately 365.2425 days—about 6 hours longer than our standard calendar year.
If we ignored these extra hours, our seasons would slowly drift out of alignment with the calendar. Over centuries, summer would start appearing in December! To correct this, we add an extra day (February 29) every four years—this is called a leap year.
How Do We Determine A Leap Year?
A year is a leap year if:
- It is divisible by 4 (e.g., 2024 ÷ 4 = 506, so it's a leap year).
- However, if it is also divisible by 100, it must also be divisible by 400 to be a leap year (e.g., 1900 is not a leap year, but 2000 is).
Examples Of Leap And Non-Leap Years
✅ Leap Years: 2000, 2024, 2028 (Divisible by 4 and follows the special 100/400 rule).
❌ Non-Leap Years: 1900, 2023, 2025 (Either not divisible by 4 or fails the 100/400 rule).
By following this leap year rule, we ensure that our calendar stays aligned with Earth's orbit, keeping our seasons predictable and stable.
Approach To Check A Leap Year In Java
To determine whether a given year is a leap year, we follow a structured approach based on divisibility rules.
Step 1: Understand The Leap Year Rules
A year is a leap year if:
- It is divisible by 4 → Possible leap year.
- However, if the year is divisible by 100, it must also be divisible by 400 to be a leap year.
This rule ensures that our calendar remains synchronized with Earth's orbit around the Sun.
Step 2: Implement The Logic In Java
We use a simple if-else condition to check these rules:
- If the year is divisible by 4 (year % 4 == 0), check further.
- If the year is divisible by 100 (year % 100 == 0), check if it’s also divisible by 400 (year % 400 == 0).
- If yes, it's a leap year.
- If no, it's not a leap year.
- If the year is not divisible by 100, it is a leap year (since it was already confirmed to be divisible by 4).
Code Implementation
Output (set code file name as LeapYearChecker.java):
Enter a year: 2024
2024 is a Leap Year.
Explanation:
- We start by importing the Scanner class from java.util to take user input.
- The LeapYearChecker class contains the main() method, which serves as the entry point of our program.
- We create a Scanner object to read input from the user.
- The program prompts the user to enter a year using System.out.print().
- The entered year is stored in an integer variable year using scanner.nextInt().
- To determine if the given year is a leap year, we apply the standard leap year conditions:
- A year is a leap year if it is divisible by 4 but not by 100, or if it is divisible by 400.
- If the condition evaluates to true, we print that the year is a leap year; otherwise, we print that it is not.
- Finally, we close the Scanner object to free up resources.
Step 3: Dry Run (Example Walkthrough)
Let's check with different years:
Year |
Divisible by 4? |
Divisible by 100? |
Divisible by 400? |
Leap Year? |
2024 |
Yes |
No |
No |
Yes |
1900 |
Yes |
Yes |
No |
No |
2000 |
Yes |
Yes |
Yes |
Yes |
2023 |
No |
No |
No |
No |
Step 4: Time Complexity Analysis
- Checking divisibility using the % operator runs in O(1) time complexity (constant time).
- The overall approach is highly efficient since it uses simple mathematical checks.
Alternative Approach To Check A Leap Year In Java
Instead of using a single if-else condition, we can use separate conditional checks to improve readability.
Step 1: Understanding the Alternative Approach
We break the leap year conditions into distinct checks:
- First, check if the year is divisible by 400 → If true, it is a leap year.
- If not, check if the year is divisible by 100 → If true, it is not a leap year.
- If neither of the above conditions is met, check if the year is divisible by 4 → If true, it is a leap year.
- Otherwise, it is not a leap year.
This method enhances clarity by using a step-by-step conditional approach.
Code Implementation:
Output (set code file name as LeapYearCheckerAlternative.java):
Enter a year: 2017
2017 is NOT a Leap Year.
Explanation:
In the above code example-
- We begin by importing the Scanner class from java.util to take user input.
- The LeapYearCheckerAlternative class contains the main() method, which acts as the program's entry point.
- We create a Scanner object to read the user's input.
- The program prompts the user to enter a year using System.out.print().
- The entered year is stored in the integer variable year using scanner.nextInt().
- We initialize a boolean variable isLeap as false to keep track of whether the year is a leap year.
- The leap year check is done in separate if-else conditions:
- If the year is divisible by 400, it is a leap year.
- Else, if the year is divisible by 100 but not by 400, it is not a leap year.
- Else, if the year is divisible by 4 but not by 100, it is a leap year.
- Otherwise, it is not a leap year.
- The final result is printed based on the value of isLeap.
- Finally, we close the Scanner object to free up system resources.
Step 2: Dry Run (Example Walkthrough)
Year |
Divisible by 400? |
Divisible by 100? |
Divisible by 4? |
Leap Year? |
2024 |
No |
No |
Yes |
Yes |
1900 |
No |
Yes |
Yes |
No |
2000 |
Yes |
Yes |
Yes |
Yes |
2023 |
No |
No |
No |
No |
Step 3: Time Complexity Analysis
- The program checks at most three conditions before determining whether a year is a leap year.
- Since each condition uses the modulus operator %, the time complexity remains O(1) (constant time).
Conclusion
Understanding the leap year concept is essential to keeping our calendar synchronized with Earth's orbit around the Sun. By following a simple set of divisibility rules, we can determine whether a given year qualifies as a leap year. In Java, we explored two approaches—one using a single if-else condition for efficiency and another using separate conditional checks for clarity. Both methods effectively ensure accurate results with constant time complexity O(1).
By implementing these approaches, we can confidently determine leap years and appreciate the logic behind our calendar adjustments. Whether you're coding for practical applications or learning fundamental programming concepts, checking for leap years is a great exercise in understanding conditions and decision-making in Java.
Frequently Asked Questions
Q. Why do we have leap years?
Leap years exist to keep our calendar synchronized with Earth's orbit around the Sun. Since a year is approximately 365.2425 days, adding an extra day every four years helps correct this slight misalignment.
Q. What are the exact conditions for a leap year?
A year is a leap year if:
- It is divisible by 4.
- However, if it is also divisible by 100, it must be divisible by 400 to remain a leap year.
Q. Why was the year 1900 not a leap year, but 2000 was?
- 1900 was divisible by 4 and 100, but not by 400, so it was not a leap year.
- 2000 was divisible by 4, 100, and 400, so it was a leap year.
Q. What happens if we don’t account for leap years?
Without leap years, the calendar would gradually drift out of sync with the seasons, leading to incorrect alignment of months with weather patterns over time.
Q. Can a leap year ever be skipped?
Yes! If a year is divisible by 100 but not by 400, it is not a leap year, even though it is divisible by 4. This prevents too many leap years from being added unnecessarily.
Suggested Reads:
- 15 Popular JAVA Frameworks Use In 2023
- What Is Scalability Testing? How Do You Test Application Scalability?
- Top 50+ Java Collections Interview Questions
- 10 Best Books On Java In 2024 For Successful Coders
- Difference Between Java And JavaScript Explained In Detail
- Top 15+ Difference Between C++ And Java Explained! (+Similarities)