Table of content:
Python String Manipulation | Handbook With Techniques & Examples
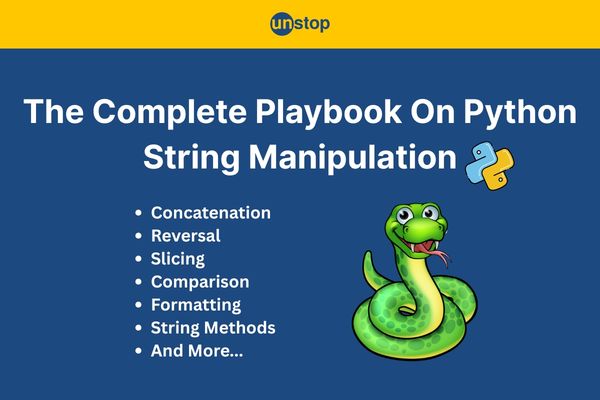
Imagine a world without words! How would we communicate? In programming, strings are our words, shaping everything from user inputs to database queries. Whether you're printing "Hello, World!" or parsing complex text, string manipulation in Python programs is a core skill for every developer.
In this article, we'll explore how Python handles strings and walk through the most common string manipulation operations. Consider this your ultimate guide to handling/manipulating strings in Python like a pro!
What Is A String & How Python Handles It?
A string in Python language is a sequence of characters enclosed in single ('), double ("), or even triple (''' or """) quotes. Strings are immutable, meaning that once created, they cannot be changed.
The snippet below illustrates these three ways of creating a string.
str1 = 'Hello' #Single quotes method
str2 = "Python" #Double quotes method
str3 = '''Triple-quoted strings can span multiple lines.'''
Key Features Of Strings In Python
- Indexed and Iterable: Access characters using indexing (s[0], s[-1]).
- Immutable: Cannot modify an existing string; new ones must be created.
- Supports Various Operations: Python allows multiple operations on strings, like concatenation, slicing, formatting, and more.
- Dynamic Length: No fixed size; it adjusts based on content.
- Unicode Support: It can handle characters from multiple languages.
- Rich Built-in Methods: The Python library comes with multiple methods to perform string manipulations like searching, modifying, and analyzing text (.lower(), .replace(), .find(), etc.).
We will discuss these in the following sections. For more information on strings, read– Python Strings | Create, Format, Reassign & More (+Examples)
With this foundation, let's explore the techniques for string manipulation in Python!
Quick Knowledge Check
QUIZZ SNIPPET IS HERE
Concatenation For String Manipulation In Python
Concatenation is the process of joining two or more strings together to create a new string. The primary way to do this is by using the plus operator (+), but we can also use the join() method. The example below illustrates this.
Code Example:
Output:
Hello Python
Here, we create two strings and then use the plus concatenation operators to join them, creating a new string result. We use a blank white space to connect the two. Alternatively, we could have used other connectors like a comma, etc.
Want to know more about concatenation techniques? Read our detailed guide on Python String Concatenation In 10 Easy Ways Explained (+Examples).
String Comparison In Python
String comparison is used to check equality or determine the lexicographical order of two strings. The operators we use for these comparisons include the relational operators (==, !=, <, >, <=, and >=) and the Python logical operators (and, or, not).
Code Example:
Output:
False
True
Here, we create two strings and then use the equality (==) and the less than (<) relational operators to compare them. The first returns False as the strings are not the same, and the other returns True as lexicographically ‘apple’ comes before ‘banana’ (i.e., a comes before b).
Want to learn all about ways to compare strings? Check out our guide– 12 Ways To Compare Strings In Python Explained (With Examples)
Slicing For String Manipulation in Python
Slicing is a common manipulation technique that allows you to extract a portion of a string using index positions. Index refers to the character's position in a string, which begins from 0 for the 1st character and so on.
When slicing, you must specify the start, end, and step values in the string. Look at the syntax and example below to understand this better.
Syntax:
string[start:end:step]
Here,
- start– The index where the slice begins (inclusive).
- end– The index where the slice stops (exclusive).
- step– The interval between characters (optional).
Code Example:
Output:
Python
Python
rogramming
PtoPormig
Here, we create a string called text. Then, we use the slice operator with different index values to extract substrings.
- In the first statement, we use [0:6], extracting the substring starting from index 0 (1st character) up to index 5 (6th character) since the stop parameter is exclusive.
- Similarly, we extract the substring after the character at the 8th position (i.e., [7:]) and a substring containing every 2nd character.
Want to explore all the slicing techniques? Read our detailed guide– String Slicing In Python | Syntax, Usage & More (+Code Examples)
Quick Knowledge Check
QUIZZ SNIPPET IS HERE
String Replacement Manipulation In Python
String replacement allows you to modify parts of a string by replacing specific characters or substrings with new values. The most common method to carry out replacement is by using the built-in Python function replace().
Code Example:
Output:
I love Unstop
Here, we create a string called text with the value “I love Python” and then use the replace() method on the text string to replace the substring “Python” with “Unstop”.
Want to learn more about the manipulation of strings by replacement? Read our detailed guide– Python String.Replace() and 8 Other Ways Explained (+Examples)
Reversion String Manipulation In Python
Reversing a string means flipping its characters so that the last character becomes the first and so on in a sequence. We often need reversal manipulation techniques for text formatting, palindrome checks, and data transformation tasks.
There are multiple ways to do this in Python, such as slicing (the most efficient), iterative methods like the for loop or the while loop, recursion, and more. The example below illustrates how we can use the slicing notation to get this done.
Code Example:
Output:
nohtyP
Here, we use the notation [::-1] to extract the whole string but from the last character and hence in reverse order.
Learn about all string reversal techniques: How To Reverse A String In Python? 10 Easy Ways With Examples.
String Formatting In Python
String formatting refers to the process of formatting string information to meet specific requirements and dynamically inserting values into a string in a structured way. The most common way to manipulate string format in Python is the use of f-strings.
Code Example:
Output:
My name is Shivani and I am 35 years old.
Here, we create two variables (one string and one integer for age) and then use f-string to present the information in a structured way.
Want to explore all how to format strings in Python? Read our detailed guide: f-string In Python | Syntax & Usage Explained (+Code Examples).
The Length Of A String In Python
One important manipulation technique for strings in Python is to determine the length/count of the number of characters in a string. The simplest way to do this is by using the len() function, which counts all characters, including spaces and special symbols.
Code Example:
Output:
14
Here, we use the “Hello, World!” example. Then, we use the len() function inside the Python print() function to display the number of characters, including white spaces and special characters.
To learn about all methods to calculate the length of a string, read– Find Length Of A String In Python | 10 Ways With Code Examples
Conversion Of String In Python
Another important type of string manipulation in Python is converting it to other data types for compatibility and other reasons. Python provides built-in functions for seamless type conversion, including:
- Convert an integer/float to a string→ Use str().
- Convert a string to an integer→ Use int().
- Convert a string to a list of characters→ Use list().
Code Example:
Output:
100
123
['P', 'y', 't', 'h', 'o', 'n']
Here, we showcase three types of conversion in reference to strings.
- First, we create an integer data type variable and convert it to string type using the str() function.
- Then, we convert string variables text (value “123”) and word (value “Python) to integer type (using int() function) and a Python list (using the list() function), respectively.
Want to explore more? Read our guides on Convert Int to String in Python and Python List to String.
String Methods For String Manipulation In Python
Python offers several built-in string methods specifically to analyze, modify, and manipulate text (i.e., string type variables) efficiently. Some key operations include (but are not limited to):
- Case Conversion→ Convert text to uppercase (.upper()), lowercase (.lower()), or capitalize words (.capitalize()).
- Finding & Searching→ Locate substrings using .find(), .index(), .startswith(), or .endswith().
- Replacing & Modifying→ Replace words with .replace(), trim spaces with .strip(), or split text using .split().
- Joining Strings→ Use .join() to merge a list of strings into a single string.
- Checking Content/Charcater Type→ Verify if a string is numeric (.isdigit()), alphabetic (.isalpha()), or whitespace (.isspace()).
Learn more about all built-in string functions (with examples) in our comprehensive guide: 40+ Python String Methods Explained | List, Syntax & Code Examples
Quick Knowledge Check
QUIZZ SNIPPET IS HERE
Conclusion
String manipulation in Python is an essential skill, enabling efficient text processing and data handling. From creating and modifying strings to formatting and searching within them, Python provides a variety of built-in methods and techniques to make these operations seamless. By mastering these techniques, you can write cleaner, more efficient, and powerful Python programs.
For a deep dive into any specific operation, explore the linked guides above and take your Python string manipulation & handling skills to the next level!
Looking for guidance? Find the perfect mentor from select experienced coding & software development experts here.
Frequently Asked Questions
Q1. Why are strings immutable in Python?
Strings in Python are immutable to ensure memory efficiency and security. Any modification creates a new string rather than altering the original, preventing unintended side effects in programs.
Q2. How do I efficiently concatenate multiple strings?
Using the join() method is more efficient than the plus operator (+) for concatenating multiple strings, especially in loops, as it minimizes memory overhead.
Q3. What is the best way to reverse a string in Python?
The most efficient way to reverse a string in Python is the slicing technique. Alternatively, the reversed() with join() also works for string reversal.
reversed_text = text[::-1]
Q4. How can I check if a string contains only digits?
We can use the built-in function/string method .isdigit() to check the type of string value, as shown in the example below:
text = "12345"
print(text.isdigit()) # Output: True
Here, we use the isdigit() method on the text string to check if it contains only digits.
Ready to upskill your Python game? Dive deeper into actual problem statements and elevate your coding game with hands-on practice!
Q5. What is the difference between find() and index()?
Both functions locate a substring within a string, but the find() method returns -1 if not found, whereas the index() raises an error.
This brings our discussion on Python string manipulation techniques. Here are a few more blogs you will love:
- Python Bitwise Operators | Positive & Negative Numbers (+Examples)
- Python Program To Add Two Numbers | 8 Techniques With Code Examples
- Python IDLE | The Ultimate Beginner's Guide With Images & Codes
- If-Else Statement In Python | All Conditional Statements + Examples
- How To Print Without Newline In Python? (Mulitple Ways + Examples)
- Python Modules | Definition, Usage, Lists & More (+Code Examples)