Table of content:
- How To Sort An Array In Java?
- Sorting An Integer Array In Java In Ascending Order
- Sorting An Integer Array In Java In Descending Order
- Sorting An Array Of Strings In Java
- Sort Subarray In Java
- Difference Between Arrays.sort() And Collections.sort() In Java
- Tips And Best Practices For Sorting An Array In Java
- Conclusion
- Frequently Asked Questions
Learn How To Sort An Array In Java (With Detailed Code Examples)
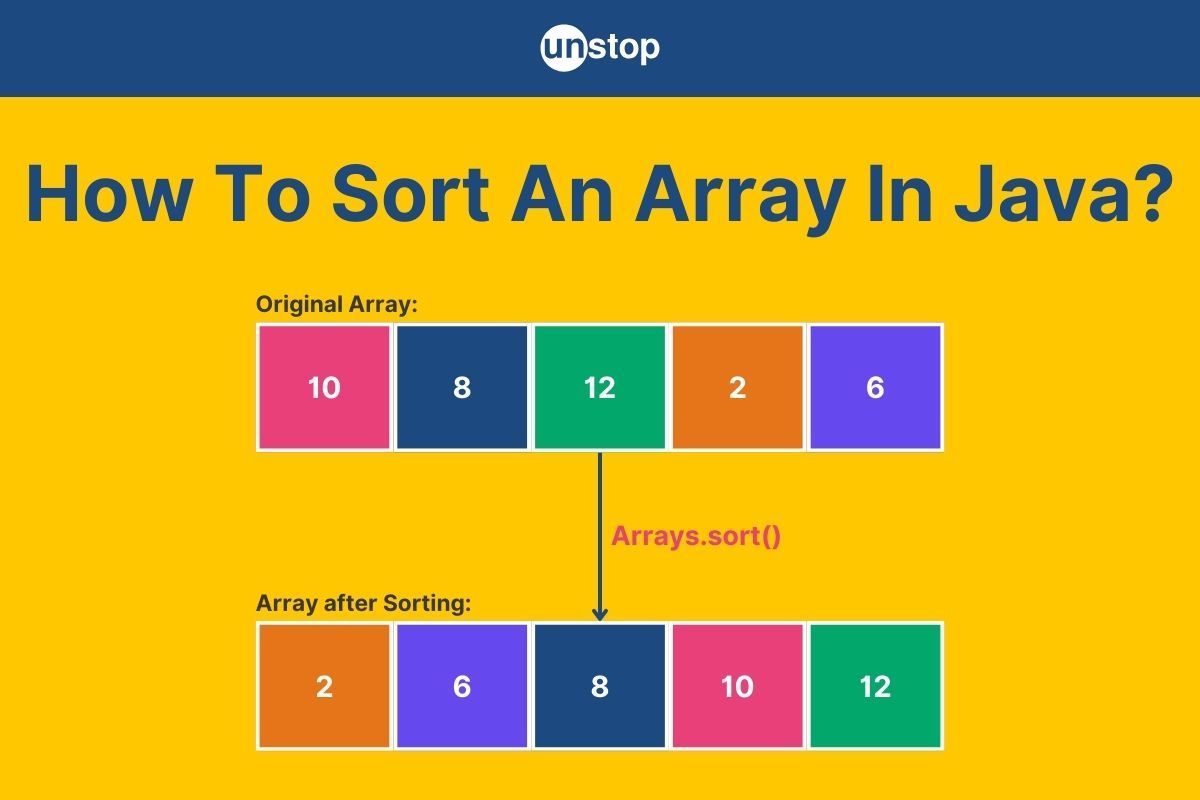
Sorting is a fundamental operation in programming that helps organize data in a specific order, making it easier to search, analyze, or manipulate. In Java, we have several ways to sort arrays, including using built-in methods like Arrays.sort() and implementing custom sorting algorithms.
In this article, we will guide you through the different approaches on how to sort arrays in Java, highlighting their usage, performance considerations, and how to choose the best method for your specific needs.
How To Sort An Array In Java?
Sorting an array in Java programming is a common operation where the elements of the array are arranged in a specific order (either ascending or descending). Java provides a built-in method Arrays.sort() in the java.util.Arrays class to sort arrays.
Syntax:
Arrays.sort(array);
Here,
- Arrays: Refers to the Arrays class in java.util package.
- sort(): A static method used to sort an array.
- array: It represents the array to be sorted. This can be an array of primitive types (e.g., int[], char[]) or objects (e.g., String[]).
Sorting An Integer Array In Java In Ascending Order
Sorting an Integer array in ascending order means arranging the elements from the smallest to the largest. Java provides an efficient and simple way to perform this operation using the Arrays.sort() method from the java.util.Arrays class. This method sorts the array in-place, meaning it directly modifies the original array without needing a new array.
Code Example:
import java.util.Arrays;
public class Main {
public static void main(String[] args) {
// Initial unsorted array
int[] arr = {5, 2, 8, 1, 9};
// Sorting the array in ascending order
Arrays.sort(arr);
// Printing the sorted array
System.out.println(Arrays.toString(arr)); // Output: [1, 2, 5, 8, 9]
}
}
Output:
[1, 2, 5, 8, 9]
Explanation:
In the above code example,
- We start by importing the Arrays class from the java.util package, which provides utility methods to work with arrays, including sorting and converting arrays to strings.
- Inside the main() method, we define an integer array arr and initialize it with some unsorted values: {5, 2, 8, 1, 9}.
- Next, we use the Arrays.sort(arr) method to sort the elements of the array in ascending order. This method modifies the array in place, arranging its elements from the smallest to the largest.
- After sorting, we use Arrays.toString(arr) to convert the array into a readable string format, Then, using System.out.println(), we print the sorted array as [1, 2, 5, 8, 9] to the console.
Explore this amazing course and master all the key concepts of Java programming effortlessly!
Sorting An Integer Array In Java In Descending Order
Sorting an array in descending order means arranging the elements from the largest to the smallest. In Java programming, while the Arrays.sort() method sorts an array by default in ascending order, we can modify the sorting behavior to arrange the elements in descending order using a custom comparator.
Code Example:
import java.util.Arrays;
import java.util.Collections;
public class Main {
public static void main(String[] args) {
// Initial unsorted array
Integer[] arr = {5, 2, 8, 1, 9};
// Sorting the array in descending order using Collections.reverseOrder()
Arrays.sort(arr, Collections.reverseOrder());
// Printing the sorted array
System.out.println(Arrays.toString(arr)); // Output: [9, 8, 5, 2, 1]
}
}
Output:
[9, 8, 5, 2, 1]
Explanation:
In the above code example-
- We begin by importing the Arrays class from java.util, which provides methods for array manipulation, and the Collections class, which offers methods to work with collections and provides functionality like reversing the order of elements.
- In the main() method, we define an array of Integer objects called arr and initialize it with unsorted values: {5, 2, 8, 1, 9}. The Integer type is used here instead of the primitive int because Collections.reverseOrder() requires an object type rather than a primitive.
- We then use Arrays.sort(arr, Collections.reverseOrder()) to sort the array in descending order. The Collections.reverseOrder() method returns a comparator that sorts the array in reverse (descending) order, and this comparator is passed to the Arrays.sort() method to sort the array accordingly.
- After sorting the array, we use Arrays.toString(arr) to convert the array into a string representation, which is printed to the console using System.out.println(). The output is the sorted array in descending order: [9, 8, 5, 2, 1].
Sorting An Array Of Strings In Java
Sorting an array of strings in Java means arranging the strings alphabetically (from A to Z). Just like with integers, Java provides the Arrays.sort() method to sort arrays. When used with an array of strings, it sorts the strings in lexicographical (dictionary) order, which means the strings are sorted based on their Unicode values.
Code Example:
import java.util.Arrays;
public class Main {
public static void main(String[] args) {
// Initial unsorted array of strings
String[] strArr = {"Banana", "Apple", "Cherry", "Mango"};
// Sorting the array of strings in alphabetical order
Arrays.sort(strArr);
// Printing the sorted array
System.out.println(Arrays.toString(strArr)); // Output: [Apple, Banana, Cherry, Mango]
}
}
Output:
[Apple, Banana, Cherry, Mango]
Explanation:
In the above code example-
- We begin by importing the Arrays class from the java.util package, which provides various utility methods for working with arrays, including sorting.
- Inside the main() method, we define an array of strings called strArr and initialize it with the unsorted values: {"Banana", "Apple", "Cherry", "Mango"}.
- We then use the Arrays.sort(strArr) method to sort the array in alphabetical order. This method sorts the elements of the array in ascending order based on their lexicographical (dictionary) order.
- After sorting, we use Arrays.toString(strArr) to convert the array into a string representation, which is printed to the console using System.out.println(). The output shows the sorted array: [Apple, Banana, Cherry, Mango].
Sharpen your coding skills with Unstop's 100-Day Coding Sprint and compete now for a top spot on the leaderboard!
Sort Subarray In Java
A subarray is a contiguous segment of an array. This means it is a sequence of elements from the original array that are adjacent to each other and maintain their order as in the original array.
- Sorting a subarray in Java means sorting only a specific portion of an array while leaving the rest of the array unchanged.
- The Arrays.sort() method provides an overloaded version that allows you to specify the range (start and end indices) of the array to sort.
- How It Works: The Arrays.sort() method with range parameters (fromIndex and toIndex) sorts the elements of the array from the specified fromIndex (inclusive) to toIndex (exclusive). The rest of the array remains unaffected.
Syntax:
Arrays.sort(array, fromIndex, toIndex);
Here:
- array: The array to be partially sorted.
- fromIndex: The starting index (inclusive) of the subarray to be sorted.
- toIndex: The ending index (exclusive) of the subarray to be sorted.
Code Example:
import java.util.Arrays;
public class Main {
public static void main(String[] args) {
// Initial unsorted array
int[] arr = {5, 3, 8, 6, 2, 7, 4, 1};
// Sorting a subarray (indices 2 to 6)
Arrays.sort(arr, 2, 6);
// Printing the array after sorting the subarray
System.out.println(Arrays.toString(arr)); // Output: [5, 3, 2, 6, 7, 8, 4, 1]
}
}
Output:
[5, 3, 2, 6, 7, 8, 4, 1]
Explanation:
In the above code example-
- We start by importing the Arrays class from the java.util package, which provides useful methods for array manipulation, including sorting a specific range within an array.
- Inside the main() method, we define an integer array arr and initialize it with unsorted values: {5, 3, 8, 6, 2, 7, 4, 1}.
- Next, we use Arrays.sort(arr, 2, 6) to sort a specific portion of the array. This method sorts the elements from index 2 (inclusive) to index 6 (exclusive) in ascending order. The rest of the array remains unaffected.
- After sorting the subarray, we use Arrays.toString(arr) to convert the entire array into a string representation, which is printed to the console using System.out.println(). The output reflects the updated array: [5, 3, 2, 6, 7, 8, 4, 1]. Only the elements from index 2 to 5 have been rearranged in ascending order.
Difference Between Arrays.sort() And Collections.sort() In Java
Both Arrays.sort() and Collections.sort() are used for sorting, but they differ in their usage, functionality, and the types of data they work with:
Feature |
Arrays.sort() |
Collections.sort() |
Usage |
Sorts arrays of primitive types or objects. |
Sorts collections like List. |
Input Type |
Works on arrays (int[], String[], etc.). |
Works on List (e.g., ArrayList, LinkedList). |
Package |
java.util.Arrays |
java.util.Collections |
Mutability |
Directly modifies the original array. |
Directly modifies the original List. |
Comparator Support |
Supports custom Comparator for object arrays. |
Requires a Comparator or elements must be Comparable. |
Sorting Order |
Default is ascending; can use a custom Comparator. |
Default is ascending; can use a custom Comparator. |
Performance |
Optimized for arrays; uses Dual-Pivot Quicksort. |
Slightly slower as it works on collections. |
Null Values |
Does not allow null for primitive arrays; may throw NullPointerException for object arrays. |
Allows sorting List containing null elements if a custom comparator handles them. |
Flexibility |
Works only on fixed-size arrays. |
More flexible, works on dynamically sized collections. |
Example Input |
int[], String[]. |
ArrayList<Integer>, LinkedList<String>. |
Let’s look at a code example where we use collections.sort() method in Java-
Code Example:
import java.util.ArrayList;
import java.util.Collections;
public class Main {
public static void main(String[] args) {
// List of integers
ArrayList<Integer> list = new ArrayList<>();
list.add(5);
list.add(3);
list.add(8);
list.add(1);
list.add(2);
// Sorting the list
Collections.sort(list);
// Printing the sorted list
System.out.println(list);
}
}
Output:
[1, 2, 3, 5, 8]
Explanation:
In the above code example-
- We begin by importing the ArrayList class from java.util, which provides a dynamic array implementation, and the Collections class, which offers utility methods for working with collections, including sorting.
- Inside the main() method, we create an ArrayList of integers named list. We add elements 5, 3, 8, 1, and 2 to the list using the add() method, resulting in an unsorted list.
- We then use the Collections.sort(list) method to sort the elements in ascending order. This method modifies the list directly, rearranging its elements from the smallest to the largest.
- Finally, we print the sorted list using System.out.println(list). The output displays the elements in sorted order: [1, 2, 3, 5, 8].
Tips And Best Practices For Sorting An Array In Java
Sorting arrays is a fundamental operation in Java programming. Here are some tips and best practices to ensure efficient and effective array sorting:
1. Choose the Appropriate Sorting Method
- Arrays.sort(): Use for general sorting of primitive or object arrays in ascending order.
- Arrays.parallelSort(): Use for large arrays to leverage multi-threading for better performance.
- Custom Sorting: Use a custom Comparator if you need sorting based on specific rules.
2. Optimize for Primitive Arrays
Use Arrays.sort() for primitive types (e.g., int[], char[]) as it is highly optimized using the Dual-Pivot Quicksort algorithm.
3. Use Custom Comparators for Object Arrays
For arrays of objects, define custom sorting logic using a Comparator if natural ordering (as defined by Comparable) is insufficient. For Example-
Arrays.sort(objectsArray, Comparator.comparing(Object::getFieldName));
4. Be Aware of Sorting Order
- Default Order: Arrays.sort() sorts in ascending order.
- Descending Order: Use a custom Comparator or Collections.reverseOrder() for object arrays. For Example-
Arrays.sort(arr, Collections.reverseOrder());
5. Avoid Sorting Large Arrays in a Single Thread
For very large arrays, consider Arrays.parallelSort(), which uses parallelization to improve performance on multi-core systems.
6. Handle Null Values in Object Arrays
Ensure that object arrays do not contain null values when using Arrays.sort(). If null values are expected, handle them explicitly in the Comparator. For Example-
Arrays.sort(arr, Comparator.nullsFirst(Comparator.naturalOrder()));
7. Ensure Contiguity When Sorting Subarrays
When sorting only part of an array, ensure the indices are within bounds and correctly defined as inclusive for fromIndex and exclusive for toIndex in Arrays.sort(array, fromIndex, toIndex).
8. Preserve Original Array if Needed
If the original array is required later, create a copy using Arrays.copyOf() before sorting. For Example-
int[] originalArray = {5, 3, 8, 1};
int[] sortedArray = Arrays.copyOf(originalArray, originalArray.length);
Arrays.sort(sortedArray);
9. Test Sorting on Large Datasets
When dealing with performance-critical applications, test the sorting with large datasets to ensure that the chosen method meets performance expectations.
10. Use Built-in Utilities Over Manual Sorting
Avoid writing custom sorting algorithms unless necessary. Built-in methods like Arrays.sort() are optimized and less error-prone.
11. Sort Multidimensional Arrays Carefully
When sorting a multidimensional array, define sorting criteria explicitly. For instance, sort based on the first column or a specific attribute of the inner array. For Example-
Arrays.sort(matrix, Comparator.comparingInt(a -> a[0]));
12. Validate the Input
Always validate the array to ensure it is not null or empty before attempting to sort.
13. Use Debugging Tools
For debugging, use Arrays.toString() (for 1D arrays) or Arrays.deepToString() (for multidimensional arrays) to print and verify sorted results.
14. Favor Efficiency Over Convenience
If performance is a concern, avoid redundant operations like converting arrays to collections for sorting unless necessary.
Are you looking for someone to answer all your programming-related queries? Let's find the perfect mentor here.
Conclusion
Sorting arrays is a fundamental skill for any Java programmer, and Java provides powerful tools like Arrays.sort() and Arrays.parallelSort() to make this process efficient and straightforward. Whether you're sorting primitive arrays, object arrays, or even subarrays, understanding the right method for your use case is essential for optimal performance and code clarity. By leveraging built-in utilities and best practices, you can efficiently sort arrays in ascending or descending order, handle custom sorting needs, and ensure robust, maintainable code. Mastering these techniques will not only simplify your code but also prepare you to tackle complex data-handling tasks with ease.
Frequently Asked Questions
Q. What are the primary methods to sort an array in Java?
Java offers two primary methods for sorting arrays:
- Arrays.sort(): This method sorts arrays in ascending order by default. It works for both primitive and object arrays. For object arrays, it uses the natural order or a custom comparator if provided.
- Arrays.parallelSort(): Introduced in Java 8, it divides the array into segments, sorts them in parallel threads, and then merges them. It is faster than Arrays.sort() for large datasets on multi-core systems.
Q. How does Arrays.sort() handle sorting for primitive and object arrays?
- For primitive arrays (int[], double[], etc.), Arrays.sort() uses Dual-Pivot Quicksort, which is an efficient, stable algorithm for general-purpose sorting.
- For object arrays (String[], Integer[], etc.), it uses the Timsort algorithm, which is optimized for partially sorted data. Objects must implement the Comparable interface, or a custom Comparator should be provided.
Q. What is the difference between ascending and descending order sorting in Java?
- Ascending Order: The default sorting order for both Arrays.sort() and Collections.sort(). It arranges elements from the smallest to the largest.
- Descending Order: Achieved by using a custom Comparator or Collections.reverseOrder(). For example-
Arrays.sort(arr, Collections.reverseOrder());
Q. How does Arrays.parallelSort() differ from Arrays.sort()?
- Concurrency: Arrays.parallelSort() uses multiple threads to sort segments of the array concurrently, which makes it faster for large arrays on multi-core systems.
- Performance: It is typically more efficient for arrays with over 10,000 elements, as the overhead of parallelism may not be justified for smaller arrays.
- Algorithm: Internally, it divides the array into sub-arrays, sorts them using ForkJoinPool, and then merges the results.
Q: Why is Arrays.parallelSort() considered better for large datasets, and when should it be avoided?
Arrays.parallelSort() is designed to leverage modern multi-core processors by dividing the array into smaller subarrays, sorting them in parallel using multiple threads, and then merging the results. This makes it significantly faster than Arrays.sort() for large datasets, especially when the size of the array exceeds 10,000 elements. The method utilizes the ForkJoinPool framework to manage parallelism efficiently, ensuring that each segment of the array is processed independently.
- However, there are scenarios where Arrays.parallelSort() might not be ideal. For smaller arrays (e.g., fewer than 10,000 elements), the overhead of thread management can outweigh the benefits of parallelism, making Arrays.sort() a better choice.
- Additionally, in environments with limited CPU cores or where the system is already under heavy load, Arrays.parallelSort() may not provide significant performance gains and could even cause contention.
- Another consideration is thread safety—while the sorting itself is internally synchronized, the array being sorted should not be modified by other threads during the operation, as this could lead to undefined behavior.
Q. What is the significance of custom comparators in sorting?
- Custom comparators allow you to define a specific sorting order beyond the natural order. This is particularly useful for:
- Sorting objects based on specific fields (e.g., sorting a list of employees by age or name).
- Handling null values in arrays.
- Reversing the default order. Example-
Arrays.sort(employees, Comparator.comparing(Employee::getSalary).reversed());
Q. Can null values be sorted in Java arrays?
For primitive arrays, null values are not applicable. For object arrays, null values can cause a NullPointerException unless explicitly handled. A custom comparator like Comparator.nullsFirst() or Comparator.nullsLast() can sort arrays with null values safely:
Arrays.sort(arr, Comparator.nullsLast(Comparator.naturalOrder()));
With this, we conclude our discussion on how to sort ana array in Java. Here are a few other topics that you might be interested in reading:
- Convert String To Date In Java | 3 Different Ways With Examples
- Final, Finally & Finalize In Java | 15+ Differences With Examples
- Super Keyword In Java | Definition, Applications & More (+Examples)
- How To Find LCM Of Two Numbers In Java? Simplified With Examples
- How To Find GCD Of Two Numbers In Java? All Methods With Examples
- Volatile Keyword In Java | Syntax, Working, Uses & More (+Examples)
- Throws Keyword In Java | Syntax, Working, Uses & More (+Examples)
I’m a Computer Science graduate with a knack for creative ventures. Through content at Unstop, I am trying to simplify complex tech concepts and make them fun. When I’m not decoding tech jargon, you’ll find me indulging in great food and then burning it out at the gym.
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
Comments
Add comment