Top 50+ JavaScript Interview Questions And Answers
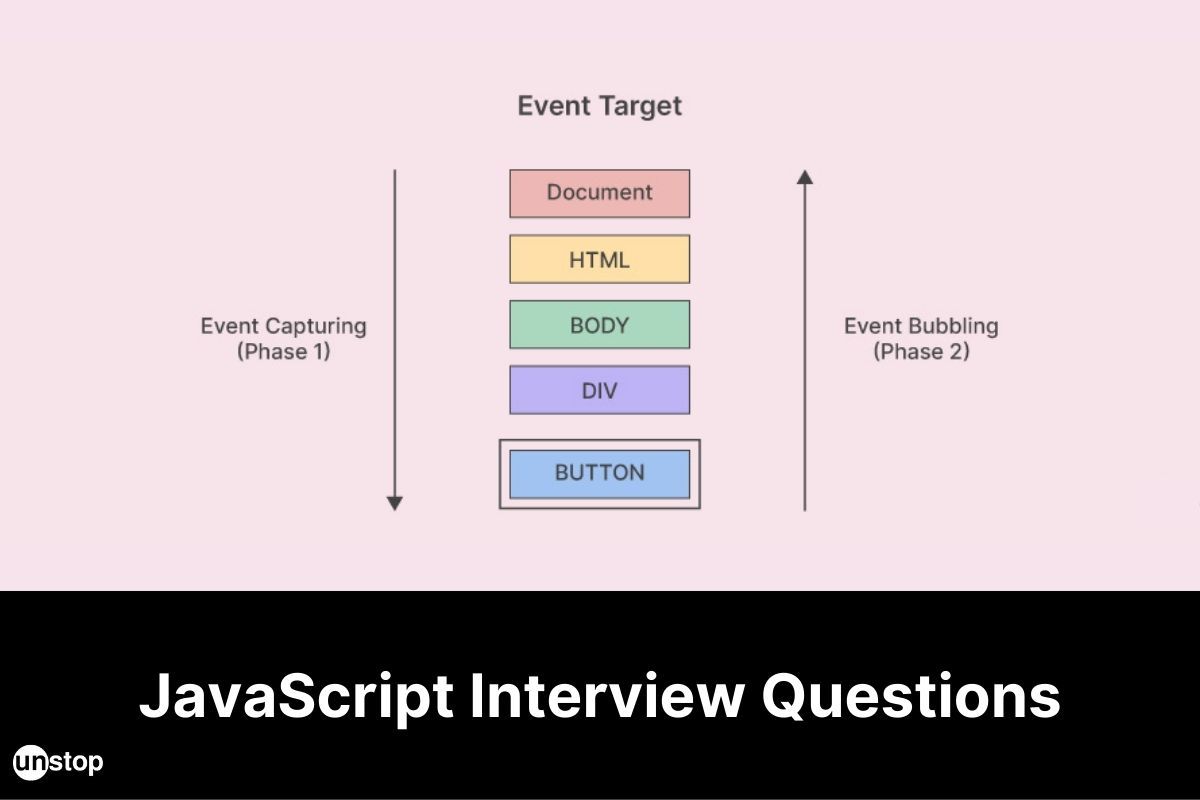
JavaScript (also abbreviated as JS) is the world's most used language. It is one of the core languages of the World Wide Web (www) along with HTML and CSS. Developed by Brendan Eich, JavaScript is used for both client-server sides to make the webpage interactive. All major web browsers have a dedicated JS engine to execute the code on users' devices.
JS engines were originally used only in web browsers, but are now core components of some servers and a variety of applications. The most popular runtime system for this usage is Node.js. Although Java and JavaScript are similar in name, syntax and respective standard libraries the two languages are distinct and differ greatly in design.
Some examples of using this scripting language are:
- Loading new web page content without reloading the page, via Ajax or a Websocket For example, users of social media can send and receive messages without leaving the current page
- Web page animations, such as fading objects in and out, resizing and moving them
- Playing browser games
- Controlling the playback of streaming media
- Generating pop-up-adds
- Validating input values of web form before the data is sent to the web browser.
- Logging data about the user's behavior and then sending it to a server. The website owner can use this data for analytics, ad tracking, and personalization
- Redirecting a user to another page
JavaScript Interview Questions
If you are applying for the role of a web developer, some of these basic JavaScript interview questions will help you prepare better.
Q1. What is JavaScript?
JavaScript is a high-level, interpreted programming language that is primarily used for creating interactive and dynamic web pages. It was originally developed by Brendan Eich at Netscape Communications in the mid-1990s and has since become one of the most popular programming languages in the world.
JavaScript is primarily known for its use in web development, where it is used to enhance the functionality and interactivity of websites. It is a core technology of the web alongside HTML and CSS. JavaScript allows developers to add dynamic behavior to web pages, handle user interactions, manipulate and modify web page content, and communicate with servers to retrieve or send data asynchronously.
Q2. What is ECMA Script?
ECMAScript (or ES) is a scripting language specification standardized by Ecma International. It is the official standard for JavaScript, providing guidelines and specifications for the language syntax, features, and behavior. The terms "JavaScript" and "ECMAScript" are often used interchangeably, but it's important to note that JavaScript is the most popular implementation of the ECMAScript standard.
ECMAScript defines the core features and functionalities of the JavaScript programming language. It specifies the syntax, data types, control structures, object-oriented programming concepts, and built-in objects and functions that are available in JavaScript.
The ECMAScript specification is periodically updated to introduce new features, improvements, and modifications to the language. The different versions of ECMAScript are often referred to by their edition number, such as ECMAScript 5 (ES5), ECMAScript 6 (ES6), ECMAScript 2015 (ES2015), ECMAScript 2016 (ES2016), and so on.
New features and enhancements introduced in later editions of ECMAScript have significantly expanded the capabilities of JavaScript, enabling developers to write more efficient, concise, and expressive code. Some notable features introduced in recent ECMAScript versions include arrow functions, classes, template literals, object destructuring assignments, spread syntax, async/await, and more.
JavaScript engines implemented in web browsers and other JavaScript runtime environments adhere to the ECMAScript specifications to ensure consistency and compatibility across different platforms.
In summary, ECMAScript is the standardized specification that defines the syntax and features of the JavaScript programming language, and JavaScript is the most widely used implementation of that standard.
Q3. What are the different data types in Javascript?
JavaScript provides different data types to hold different types of values. There are two types of data types in JavaScript.
- Primitive data type
- Non-primitive (reference) data type
Primitive Values (immutable datum represented directly at the lowest level of the language):- All types except objects define immutable values (that is, values that can't be changed). For example (and unlike in C), Strings are immutable. We refer to values of these types as "primitive values".
Boolean Type | Boolean represents a logical entity and can have two values., i.e, TRUE or FALSE |
Undefined Type | A variable that has not been assigned a value has the value undefined, i.e, let a; |
Number Type | An integer or a floating-point number. i.e, 3, 3.54 |
BigInt |
The BigInt type is a numeric primitive in JavaScript that can represent integers with arbitrary precision. Example- 900719925124740999n |
StringType |
JavaScript's String type is used to represent textual data. It is a set of "elements" of 16-bit unsigned integer values. Example- "hello world" |
Symbol Type |
Symbol is a unique and immutable primitive value and may be used as the key. In some programming languages, Symbols are called "atoms". Example: Let value = Symbol ('hello'); |
Non-Primitive data types
1. Objects: In computer science, an object is a value in memory that is possibly referenced by an identifier.
2. Properties: In JavaScript, objects can be seen as a collection of properties. With the object literal syntax, a limited set of properties are initialized; then properties can be added and removed. Property values can be values of any type, including other objects, which enables building complex data structures. Properties are identified using key values. A key value is either a string value or a symbol value.
There are mainly two properties,
- Data Property: The data property sets or returns the value of the data attribute of an <object> element.
- Accessor Property: Property accessors provide access to an object's properties by using the dot notation or the bracket notation.
Q4. What are callbacks?
In JavaScript, a callback is a function that is passed as an argument to another function and is executed later, typically after an asynchronous operation has completed or when a certain event occurs. Callbacks are a way to ensure that certain code snippet is executed only when a particular task is completed, allowing for asynchronous and non-blocking behavior in JavaScript.
Callbacks are commonly used in scenarios such as handling asynchronous operations like fetching data from a server, performing separate file I/O operations, or responding to user events. When an asynchronous operation is initiated, the callback function is provided as an argument, and it gets invoked by the system or the calling function once the operation is completed.
Here's an example to illustrate the concept of callbacks:
ZnVuY3Rpb24gcHJvY2Vzc0RhdGEoaW5wdXQpIHsKICAgIC8vRW50ZXIgeW91ciBjb2RlIGhlcmUKfSAKCnByb2Nlc3Muc3RkaW4ucmVzdW1lKCk7CnByb2Nlc3Muc3RkaW4uc2V0RW5jb2RpbmcoImFzY2lpIik7Cl9pbnB1dCA9ICIiOwpwcm9jZXNzLnN0ZGluLm9uKCJkYXRhIiwgZnVuY3Rpb24gKGlucHV0KSB7CiAgICBfaW5wdXQgKz0gaW5wdXQ7Cn0pOwoKcHJvY2Vzcy5zdGRpbi5vbigiZW5kIiwgZnVuY3Rpb24gKCkgewogICBwcm9jZXNzRGF0YShfaW5wdXQpOwp9KTs=
In the above example, the function simulates an asynchronous operation that takes some time to complete (here simulated using ). It accepts a callback function as an argument. Once the asynchronous operation is finished (after a 2-second delay), it invokes the provided callback function () and passes the fetched data as an argument.
The function is defined separately and passed as a callback to the function. It receives the fetched data as a parameter and processes it by logging it to the console.
By using callbacks, you can ensure that the function is executed only after the data has been fetched, even though the actual fetching process takes some time. This allows for non-blocking execution and helps handle asynchronous operations in JavaScript effectively.
Q5. What are the scopes of variables in JavaScript?
In JavaScript, variables have different types of scope, which determine where a variable is accessible and how long it persists. The types of scope of variables in JavaScript are as follows:
Global Scope |
|
Local scope |
|
Function Scope |
|
Block Scope (Introduced in ES6) |
|
Q6. What is the unshift() method?
This method is functional at the start of the array, unlike the push(). It adds the desired number of elements to the top of an array. For example –
dmFyIG5hbWUgPSBbICJteXNvcmUiIF07Cm5hbWUudW5zaGlmdCggImJhbmdhbG9yZSIgKTsKbmFtZS51bnNoaWZ0KCAidHJpdmFuZHJ1bSIsICJjaGVubmFpIiApOwpjb25zb2xlLmxvZyhuYW1lKTs=
The output is shown below:
Q7. Explain string in JavaScript.
A string is a sequence of characters, which is enclosed within a single (’ ’) or double (" ") quote.
Two ways to create a string in JS are:
1. String Literal: The string literal is created using single or double quotes.
Its syntax is as follows:
var stringname= "stringvalue";
Output: Unstop
2. String Object (Using Keyword)
The syntax for creating objects using new keywords is as follows:
var stringname=new String(”stringvalue”);
Output: Unstop
Some of the methods in JS string are as follows:
charAt() | Provides the value of the character present at the specified index |
concat() | A combination of two or more strings can be done using the concat() method |
indexOf() | Provides the position of the char value present in a given string. |
search() | It searches a specified regular expression in a given string and returns its position if a match occurs. |
match() | It searches a specified regular expression in a given string and returns the regular expression if the match occurs. |
replace() |
It is used to replace the given string with a specified replacement. |
substr() | It is used to fetch a part of the given string on the basis of a specified starting position and length. |
slice() | It is used to fetch the part of the given string. It allows us to assign positive as well negative indexes. |
trim() | It is used to trim the white spaces from the left and right sides of the string |
valueOf() | It provides the primitive value of the string object |
toUpper() | It converts the entire string into uppercase. |
toLower() | It converts the entire string into lowercase. |
Q8. Difference between search() and indexOf()?
The and methods in JavaScript are used to search for a substring within a string. Here are the differences between these two methods:
Method |
Method |
The method searches for a specified substring within a string and returns the index of the first occurrence of the substring. |
The method searches for a specified substring within a string and returns the index of the first occurrence of the substring. |
It does not support regular expressions; it performs a simple string search. |
It allows you to use regular expressions for searching. |
If the substring is found, the method returns the index of the first character of the match. If the substring is not found, it returns -1. |
If the substring is found, the method returns the index of the first character of the match. If the substring is not found, it returns -1. |
Example: var str = 'Hello World'; |
Example: var str = 'Hello World'; |
In summary, the main differences between and are:
- allows the use of regular expressions for searching, while performs a simple string search.
- returns the index using regular expression matching, while returns the index based on exact string matching.
- Both methods return -1 if the substring is not found in the string.
indexOf(): Provides the position of the char value present in a given string.
Q9. Difference between an array and an object?
Here's a comparison between an array and an object :
Array | Object | |
---|---|---|
Definition | An ordered collection of values | An unordered collection of key-value pairs |
Access | Access elements using numeric indices | Access values using keys |
Order | Elements are ordered | Key-value pairs are unordered |
Length | Length can change dynamically | Number of key-value pairs can vary |
Iteration | Iterated using loops or array methods | Iterated using loops or object methods |
Elements | Elements have implicit indices | Properties have explicit keys |
Duplication | Allows duplicate values | Keys must be unique, values may or may not be unique |
Data Storage | Typically used for homogeneous data | Typically used for heterogeneous and structured data |
Use Cases | Storing and accessing multiple values | Representing entities, records, or structured data |
Arrays are primarily used when you need to store and access multiple values of the same type or when maintaining the order of elements is important. You can perform various operations on arrays, such as adding, removing, or modifying elements.
Objects, on the other hand, are used to represent entities, records, or structured data. They allow you to associate values (properties) with keys, making it easier to access and manipulate data based on meaningful names or identifiers.
In JavaScript, both arrays and objects have their distinct purposes and usage scenarios. They can be used in combination to create complex data structures and represent various aspects of a program or application.
Q10. Difference between "==" and "===" operators?
In JavaScript, the "==" (equality) and "===" (strict equality) operators are used for comparison between two values. Here's the difference between the two:
== (Equality Operator) | === (Strict Equality Operator) |
The "==" operator checks for equality between two values, allowing type coercion if necessary. |
The "===" operator checks for strict equality between two values without performing type coercion. |
If the types of the two values being compared are different, JavaScript attempts to convert one or both values to a common type before making the comparison. If the converted values are equal, the "==" operator returns ; otherwise, it returns . |
It compares both the values and their types. If both the values and types are exactly the same, the "===" operator returns ; otherwise, it returns . |
In general, it is recommended to use the "===" (strict equality) operator for most comparisons, as it ensures both value and type equality, without any unexpected type coercion. It helps prevent subtle bugs that can occur due to unintended type conversions. The "==" (equality) operator should be used with caution when type coercion is desired or explicitly handled.
Q11. Is JS static and dynamically typed?
JavaScript is a dynamically typed language, meaning that variable types are determined at runtime rather than explicitly declared. In JavaScript, you can assign values of different types to the same variable without explicitly specifying the variable's type.
Dynamic typing allows for greater flexibility and ease of use in JavaScript, as variables can be used to hold values of different types as needed. However, it also introduces the potential for type-related errors if not handled carefully. Developers need to pay attention to variable types and ensure that operations and functions are used correctly with the appropriate type of data.
Q12. What is isNaN() in Javascript?
In JavaScript, the function is used to determine whether a value is "Not-a-Number" (NaN) or not. It checks if the provided value is not a valid number and returns a boolean value indicating the result.
The function has the following behavior:
- If the provided value is not a number or cannot be converted to a number, it returns .
- If the provided value is a valid number or can be converted to a number, it returns .
Here are some examples:
isNaN(42); // false, 42 is a valid number
isNaN('42'); // false, '42' can be converted to the number 42
isNaN('Hello'); // true, 'Hello' cannot be converted to a number
isNaN(undefined); // true, undefined cannot be converted to a number
isNaN(NaN); // true, NaN is considered as Not-a-Number
It's worth noting that the isNaN() function coerces the provided value to a number if it is not already a number. This coercion is done using the Number() function. If the value cannot be converted to a number, isNaN() returns true.
Q13. Explain pass-by-reference and pass-by-value.
In programming, "pass-by-reference" and "pass-by-value" are two different ways of passing arguments to functions or assigning values to variables. They determine how the data is handled and accessed within the function or variable assignment.
Pass-by-Value | Pass-by-Reference |
Pass-by-value is a mechanism where a copy of the value is passed to a function or assigned to a variable. |
Pass-by-reference is a mechanism where the memory address or reference of the value is passed to a function or assigned to a variable. |
In pass-by-value, any modifications made to the function parameter or variable inside the function do not affect the original value outside the function. |
In pass-by-reference, any modifications made to the function parameter or variable inside the function affect the original value outside the function. |
Primitive data types such as numbers, booleans, and strings are typically passed by value. |
Non-primitive data types such as objects and arrays are typically passed by reference. |
Example: function increment(number) { var x = 5; In this example, the value of is passed by value to the function. The function increments the copy of to 6, but the original value of remains unchanged outside the function. |
Example: function changeName(person) { var user = { changeName(user); In this example, the reference of the object is passed to the function. The function modifies the property of the object, which affects the original object outside the function. |
Q14. Which company developed JavaScript?
JavaScript was developed by Brendan Eich, who was working at Netscape Communications Corporation (now known as Netscape Communications), during the mid-1990s. It was initially created for the Netscape Navigator web browser, which was one of the dominant browsers of that era. The development of JavaScript was driven by the need for a scripting language that could add interactivity and dynamic functionality to web pages. Brendan Eich designed and implemented JavaScript in just 10 days in May 1995, and it was first introduced in Netscape Navigator 2.0 in September 1995.
Q 15. What are the advantages of JS?
JavaScript (JS) offers several advantages that contribute to its popularity and widespread usage. Some of the key advantages of JavaScript include:
Client-Side Interactivity |
JavaScript is primarily used as a client-side scripting language, allowing developers to enhance the interactivity and user experience of web pages. It enables dynamic content updates, form validation, DOM manipulation, and interactive features without the need for server-side communication. |
Wide Adoption and Support |
JavaScript is supported by all major web browsers, making it a universal language for web development. It has a large and active community of developers, which leads to extensive resources, libraries, and frameworks that simplify development tasks. |
Versatility | JavaScript is a versatile language that can be used for various purposes, including web development, mobile app development (using frameworks like React Native and Ionic), server-side development (with Node.js), desktop application development (using frameworks like Electron), and even IoT (Internet of Things) development. |
Asynchronous Programming |
JavaScript supports asynchronous programming using features such as callbacks, Promises, and async/await. This allows non-blocking execution and efficient handling of tasks like fetching data from servers, performing animations, or handling user input without blocking the user interface. |
Easy Integration |
JavaScript can be easily integrated into existing web projects as it can be embedded directly within HTML or included from external files. It seamlessly interacts with HTML and CSS, making it straightforward to enhance the functionality and behavior of web pages. |
Rapid Development |
JavaScript promotes rapid development with its lightweight syntax and dynamic nature. It offers immediate feedback during development, making it easy to iterate and test code quickly. Additionally, the extensive ecosystem of libraries and frameworks (such as React, Vue.js, Angular) provides ready-made solutions for common tasks, reducing development time. |
Cross-Platform Compatibility |
JavaScript can run on various platforms, including different operating systems and devices. It powers web applications across desktops, laptops, tablets, smartphones, and even embedded systems, enabling developers to reach a wide audience with their applications. |
Scalability |
JavaScript allows developers to build scalable applications by leveraging modern frameworks and tools. With the introduction of server-side JavaScript frameworks like Node.js, developers can create highly scalable and efficient server-side applications using the same language as the client side. |
Q16. What are the disadvantages of JS?
JavaScript, like any programming language, has its disadvantages along with its strengths. Some of the disadvantages of JavaScript include:
Browser Compatibility |
JavaScript implementations may vary across different web browsers, leading to inconsistencies and compatibility issues. Developers often have to write code that accounts for these differences to ensure cross-browser compatibility. |
Security Vulnerabilities |
JavaScript executed on the client side is visible to the user, which makes it susceptible to manipulation and abuse. Malicious users can exploit vulnerabilities in JavaScript code to perform actions that compromise security, such as cross-site scripting (XSS) attacks.
|
Lack of Strong Typing |
JavaScript is a dynamically-typed language, which means variable types are determined at runtime. This flexibility can lead to issues and bugs due to unexpected type conversions or mismatches. It requires careful attention to variable types and may lead to runtime errors that could have been caught with static typing. |
Performance Limitations |
JavaScript is an interpreted language, and compared to compiled languages, it can have slower execution speeds. Although modern JavaScript engines have become highly optimized, JavaScript may not perform as well as lower-level languages in computationally intensive tasks. |
Limited File Access |
Due to security restrictions, JavaScript has limited access to the user's file system. It cannot directly read or write files on the user's machine for security reasons, which can be a limitation for certain types of applications. |
Lack of Multithreading | JavaScript is single-threaded, meaning it can only execute one operation at a time. This can be a disadvantage for highly parallel or CPU-intensive tasks, as it may lead to blocking and slower execution. |
Debugging Challenges |
Debugging JavaScript code can be challenging, especially when dealing with complex asynchronous operations or code that runs in different environments (browsers, server-side, etc.). Proper debugging tools and techniques are required to effectively troubleshoot issues. |
Q17. Define a named function in JS.
In JavaScript, a named function is a function that is assigned a name and can be referred to by that name for calling or referencing it later in the code. It is defined using the keyword followed by the function name, parentheses for optional parameters, and curly braces to enclose the function body.
Named functions provide several advantages, including:
Readability and self-documentation | The name of the function describes its purpose, making the code easier to understand and maintain. |
Reusability | Named functions can be called multiple times from different parts of the code, avoiding code duplication. |
Recursion | Named functions can call themselves recursively by using their own name, allowing repetitive tasks to be solved elegantly. |
Q18. What are the looping structures in JavaScript?
JavaScript provides several looping structures to repeat a block of code multiple times. The commonly used looping structures in JavaScript are:
loop |
The loop is used to iterate over a block of code for a specific number of times. |
It consists of three parts: initialization, condition, and increment/decrement. |
|
Example: for (var i = 0; i < 5; i++) { |
|
loop |
The loop repeatedly executes a block of code as long as a specified condition is true. |
It evaluates the condition before each iteration. |
|
Example: var i = 0; while (i < 5) { console.log(i); i++; } |
|
loop |
The loop is similar to the loop, but it evaluates the condition after executing the block of code. |
This ensures that the block of code is executed at least once, even if the condition is initially false. | |
Example: var i = 0; do { console.log(i); i++; } while (i < 5); |
|
loop |
The loop is used to iterate over the properties of an object. |
It loops through each enumerable property of an object. | |
Example: var person = { for (var key in person) { |
|
loop |
The loop is introduced in ES6 and is used to iterate over iterable argument objects, such as arrays or strings. |
It provides a simpler shorter syntax compared to other looping structures. |
|
Example: var colors = ['red', 'green', 'blue']; for (var color of colors) { |
Q19. Define closure.
In JavaScript, closure is a combination of a function and the lexical environment within which that function was declared. It has access ti variables in its parent scope and allows a function to access variables from its outer (enclosing) scope even after the outer function has finished executing.
A closure is created when an inner function is returned from an outer function inside the parent scope, and the inner function retains a reference to the variables of the outer function, even if the outer function has already been completed. This allows the inner function within the parent scope to access and manipulate the variables of its parent function, preserving their values.
Closures are powerful because they provide a way to create and access private variables and encapsulate functionality. They are commonly used to create data privacy and to implement modules and factories in JavaScript.
Q20. How to write "hello world" in JavaScript?
“Hello World” is a simple program and is used as an introduction program for beginners. We can print it using three ways:
To write "hello world" in JavaScript, you can use the function to output the text to the browser console. Here's an example:
console.log("Hello, world!");
When you run this code in a JavaScript environment that supports the function (such as a browser console or a Node.js environment), it will print "Hello, world!" as the output.
If you want to display "hello world" directly on a web page, you can use JavaScript to manipulate the HTML content. Here's an example of how you can achieve that:
HTML:
<!DOCTYPE html>
<html>
<head>
<title>Hello World</title>
</head>
<body>
<div></div><script src="script.js"></script>
</body>
</html>
JavaScript (script.js):
var outputDiv = document.getElementById("output");
outputDiv.textContent = "Hello, world!";
In this example, the JavaScript code selects the element with the id "output" using the method and sets its property to "Hello, world!". This will display "Hello, world!" on the web page inside the element.
Q21. What are the key differences between Java and JavaScript?
JAVA | JAVASCRIPT |
---|---|
Java is a strongly typed language and variables must be declared first to use in the program. In Java, the type of a variable is checked at compile-time. | JavaScript is a weakly typed language and has a more relaxed syntax and rules. |
Java is an object-oriented programming language. | JavaScript is an object-based scripting language. |
Java applications can run in any virtual machine(JVM) or browser. | JavaScript code used to run only in the browser, but now it can run on the server via Node.js. |
Objects of Java are class-based even we can’t make any program in java without creating a class. | JavaScript Objects are prototype-based. |
Java program has the file extension “.Java” and translates source code into bytecodes which are executed by JVM(Java Virtual Machine). | JavaScript file has the file extension “.js” and it is interpreted but not compiled, every browser has a Javascript interpreter to execute JS code. |
Java is a Standalone language. | contained within a web page and integrates with its HTML content. |
Java has a thread-based approach to concurrency. | Javascript has an event-based approach to concurrency. |
Java supports multithreading. | Javascript doesn’t support multi-threading. |
Q22. What is BOM?
BOM stands for Browser Object Model. It is a JavaScript API provided by web browsers that allows interaction with the browser window, frames, and other browser-specific features. The BOM provides access to various object types and methods that can be used to manipulate and control the browser itself.
The BOM includes owner objects such as:
Window Object |
The object represents the browser window or tab and serves as the global object in client-side JavaScript. |
It provides methods and properties for controlling the browser window, navigating to URLs, opening and closing windows, and handling events related to the window. |
|
Location Object |
The object represents the current URL of the web page loaded in the browser window. |
It provides properties and methods to manipulate the URL, such as redirecting to a new page, accessing query parameters, or reloading the current page. |
|
History Object |
The object represents the browser's session history, including the URLs visited by the user. |
It provides methods for navigating through the user's history, such as going back or forward to previous pages. |
|
Navigator Object |
The object provides information about the browser and the user's system. |
It includes properties that reveal details about the browser name, version, platform, and user agent. |
|
Screen Object |
The object provides information about the user's screen or monitor. |
It includes properties for screen dimensions, color depth, and other display-related information. |
The BOM allows JavaScript to interact with the browser, control the window behavior, navigate through history, detect user settings, and perform various browser-specific tasks. However, it's important to note that the BOM is not standardized, and different browsers may have variations in their implementation and available features. Therefore, it's recommended to use BOM-related features judiciously and be aware of cross-browser compatibility issues.
Q23. What is DOM?
DOM stands for Document Object Model. It is a programming interface for web documents, which represents the structure and content of an HTML or XML document as a tree-like model. The DOM provides a way to interact with and manipulate the elements, attributes, and text within a document.
In simple terms, the DOM can be visualized as a representation of the HTML document in memory, organized in a hierarchical structure. Each element, attribute, and text node in the document is represented as a node in the DOM tree.
Key features of the DOM include:
Tree-like Structure |
The DOM represents an HTML document as a hierarchical tree structure, where each element, attribute, and text node is a node in the tree. |
Nodes are connected to each other based on their parent-child relationships, allowing traversal and manipulation of the document structure. |
|
Document Access and Manipulation |
The DOM provides methods and properties to access, create, modify, and delete elements, attributes, and text within the document. |
Developers can use these APIs to dynamically update the content and structure of a web page based on user interactions or other events. |
|
Cross-Language Compatibility |
The DOM is language-neutral, meaning it can be accessed and manipulated using programming languages like JavaScript, Python, Java, etc. |
Different programming languages provide their own DOM APIs to interact with the document structure. |
By using the DOM, web developers can dynamically update the content of web pages, change styles, add or remove elements, handle events, and perform other operations to create interactive and dynamic web applications.
Q24. How to create an array in JavaScript?
There are 3 ways to create an array in JavaScript.
By array literal
PHNjcmlwdD4KdmFyIGVtcD1bIlNvbm9vIiwiVmltYWwiLCJSYXRhbiJdOwpmb3IgKGk9MDtpPGVtcC5sZW5ndGg7aSsrKXsKZG9jdW1lbnQud3JpdGUoZW1wW2ldICsgIjxici8+Iik7Cn0KPC9zY3JpcHQ+
By using an Array keyword
PHNjcmlwdD4KdmFyIGk7CnZhciBlbXAgPSBuZXcgQXJyYXkoKTsKZW1wWzBdID0gIkFydW4iOwplbXBbMV0gPSAiVmFydW4iOwplbXBbMl0gPSAiSm9obiI7CmZvciAoaT0wO2k8ZW1wLmxlbmd0aDtpKyspewpkb2N1bWVudC53cml0ZShlbXBbaV0gKyAiPGJyPiIpOwp9Cjwvc2NyaXB0Pg==
By Using Array Constructor
PHNjcmlwdD4KdmFyIGVtcD1uZXcgQXJyYXkoIkphaSIsIlZpamF5IiwiU21pdGgiKTsKZm9yIChpPTA7aTxlbXAubGVuZ3RoO2krKyl7CmRvY3VtZW50LndyaXRlKGVtcFtpXSArICI8YnI+Iik7Cn0KPC9zY3JpcHQ+
Q25. Explain hoisting in JS.
Hoisting is a JavaScript behavior where variable and function declarations are moved to the top of their containing scope during the compilation phase. This means that regardless of where variables and functions are declared in the code, they are conceptually moved to the top of their scope and can be used before they are actually declared.
Hoisting applies to both variable declarations and function declarations, but there are some differences in how they are hoisted:
Variable Hoisting |
Variable declarations using the keyword are hoisted to the top of their scope. |
Only the declaration is hoisted, not the initialization. The value of the variable is until it is explicitly assigned a value. |
|
Function Hoisting |
Function declarations are fully hoisted, meaning both the function declaration and its implementation are moved to the top of the scope. |
Function declarations can be called before they are declared in the code. |
It's important to note that hoisting only applies to declarations, not initializations or assignments. Variables declared with and are also hoisted, but they are not accessible until the line of code where they are declared.
In JavaScript, Hoisting is the default behavior of moving all the declarations at the top of the scope before code execution. Basically, it gives us an advantage that no matter where functions and variables are declared, they are moved to the top of their scope regardless of whether their scope is global or local.
Q26. Explain "this" keyword.
In JavaScript, the keyword refers to the context in which a function is executed. It is a special variable that holds a reference to the object on which a method is being called or the object that is currently being constructed by a constructor function.
The value of is determined dynamically at runtime and depends on how a function is invoked. The keyword can have different values in different situations:
Global Context |
When is used outside of any function or object, it refers to the global object, which is in browsers or in Node.js. |
Function Context |
When a function is called as a standalone function, refers to the global object ( in browsers, in Node.js) in non-strict mode or in strict mode. |
Method Context |
When a function is called as a method of an object, refers to the object itself. |
Constructor Context |
When a function is used as a constructor function using the keyword, refers to the newly created object. |
Event Handler Context: |
When a function is used as an event handler, typically refers to the element that triggered the event. |
Q27. Given a string, reverse each word in a sentence.
To reverse each word in a sentence, you can follow these steps:
- Split the sentence into an array of words using the method.
- Iterate over each word in the array.
- Reverse each word using various string manipulation techniques such as converting the word into an array, reversing the array, and joining the reversed array back into a string.
- Store the reversed words in a new array.
- Join the reversed words array back into a sentence using the method.
- Return the reversed sentence.
Here's an example JavaScript function that implements this logic:
UTI3LiBHaXZlbiBhIHN0cmluZywgcmV2ZXJzZSBlYWNoIHdvcmQgaW4gYSBzZW50ZW5jZS4KCm8gcmV2ZXJzZSBlYWNoIHdvcmQgaW4gYSBzZW50ZW5jZSwgeW91IGNhbiBmb2xsb3cgdGhlc2Ugc3RlcHM6CgpTcGxpdCB0aGUgc2VudGVuY2UgaW50byBhbiBhcnJheSBvZiB3b3JkcyB1c2luZyB0aGUgc3BsaXQoKSBtZXRob2QuCkl0ZXJhdGUgb3ZlciBlYWNoIHdvcmQgaW4gdGhlIGFycmF5LgpSZXZlcnNlIGVhY2ggd29yZCB1c2luZyB2YXJpb3VzIHN0cmluZyBtYW5pcHVsYXRpb24gdGVjaG5pcXVlcyBzdWNoIGFzIGNvbnZlcnRpbmcgdGhlIHdvcmQgaW50byBhbiBhcnJheSwgcmV2ZXJzaW5nIHRoZSBhcnJheSwgYW5kIGpvaW5pbmcgdGhlIHJldmVyc2VkIGFycmF5IGJhY2sgaW50byBhIHN0cmluZy4KU3RvcmUgdGhlIHJldmVyc2VkIHdvcmRzIGluIGEgbmV3IGFycmF5LgpKb2luIHRoZSByZXZlcnNlZCB3b3JkcyBhcnJheSBiYWNrIGludG8gYSBzZW50ZW5jZSB1c2luZyB0aGUgam9pbigpIG1ldGhvZC4KUmV0dXJuIHRoZSByZXZlcnNlZCBzZW50ZW5jZS4KCkhlcmUncyBhbiBleGFtcGxlIEphdmFTY3JpcHQgZnVuY3Rpb24gdGhhdCBpbXBsZW1lbnRzIHRoaXMgbG9naWM6CgpmdW5jdGlvbiByZXZlcnNlV29yZHMoc2VudGVuY2UpIHsKLy8gU3BsaXQgdGhlIHNlbnRlbmNlIGludG8gYW4gYXJyYXkgb2Ygd29yZHMKdmFyIHdvcmRzID0gc2VudGVuY2Uuc3BsaXQoJyAnKTsKCi8vIEl0ZXJhdGUgb3ZlciBlYWNoIHdvcmQgYW5kIHJldmVyc2UgaXQKdmFyIHJldmVyc2VkV29yZHMgPSB3b3Jkcy5tYXAoZnVuY3Rpb24od29yZCkgewovLyBDb252ZXJ0IHRoZSB3b3JkIGludG8gYW4gYXJyYXksIHJldmVyc2UgaXQsIGFuZCBqb2luIGl0IGJhY2sgaW50byBhIHN0cmluZwpyZXR1cm4gd29yZC5zcGxpdCgnJykucmV2ZXJzZSgpLmpvaW4oJycpOwp9KTsKCi8vIEpvaW4gdGhlIHJldmVyc2VkIHdvcmRzIGFycmF5IGJhY2sgaW50byBhIHNlbnRlbmNlCnZhciByZXZlcnNlZFNlbnRlbmNlID0gcmV2ZXJzZWRXb3Jkcy5qb2luKCcgJyk7CgpyZXR1cm4gcmV2ZXJzZWRTZW50ZW5jZTsKfQoKLy8gRXhhbXBsZSB1c2FnZToKdmFyIHNlbnRlbmNlID0gJ0hlbGxvLCB3b3JsZCEgSG93IGFyZSB5b3U/JzsKdmFyIHJldmVyc2VkU2VudGVuY2UgPSByZXZlcnNlV29yZHMoc2VudGVuY2UpOwpjb25zb2xlLmxvZyhyZXZlcnNlZFNlbnRlbmNlKTsKCgpPdXRwdXQ6CgpvbGxlSCwgZGxyb3chIHdvSCBlcmEgP3VveQoKCgoKClEyOC4gSG93IHRvIGVtcHR5IGFuIGFycmF5IGluIEpTPwoKVGhlcmUgYXJlIDQgd2F5cyBpbiB3aGljaCB0aGUgYXJyYXkgY2FuIGJlIGVtcHRpZWTinpYKCkxldCB1cyB0YWtlIGFuIGFycmF5IGZvciBleGFtcGxlIGE9WzEsMiwzXQoKVGhlIG91dHB1dCBmb3IgYWxsIHRoZSBmb2xsb3dpbmcgdGVjaG5pcXVlcyBtZW50aW9uZWQgYmVsb3cgaXMgW10KCkFzc2lnbmluZyBpdCB0byBhIG5ldyBlbXB0eSBhcnJheeKelgoKbGV0IGE9WzEsMiwzXQphID0gW107CmNvbnNvbGUubG9nKGEpOwoKCkFzc2lnbmluZyB0aGUgbGVuZ3RoIG9mIHRoZSBhcnJheeKelgoKbGV0IGEgPSBbMSwyLDNdOwphLmxlbmd0aD0wOwpjb25zb2xlLmxvZyhhKTsKCgpVc2luZyB0aGUgc3BsaWNlKCkgbWV0aG9k4p6WCgpsZXQgYSA9IFsxLDIsM107CmEuc3BsaWNlKDAsYS5sZW5ndGgpOwpjb25zb2xlLmxvZyhhKTsKCgpVc2luZyBwb3AoKeKelgoKbGV0IGEgPSBbMSwyLDNdOwp3aGlsZShhLmxlbmd0aCA+IDApIHsKICAgIGEucG9wKCk7Cn0KY29uc29sZS5sb2coYTsKClEyOS4gV3JpdGUgYSBmdW5jdGlvbiB0aGF0IHBlcmZvcm1zIGEgYmluYXJ5IHNlYXJjaCBvbiBhIHNvcnRlZCBhcnJheS4KZnVuY3Rpb24gYmluYXJ5X1NlYXJjaChpdGVtcywgdmFsdWUpewogICAgdmFyIGZpcnN0SW5kZXggID0gMCwKICAgICAgICBsYXN0SW5kZXggICA9IGl0ZW1zLmxlbmd0aCAtIDEsCiAgICAgICAgbWlkZGxlSW5kZXggPSBNYXRoLmZsb29yKChsYXN0SW5kZXggKyBmaXJzdEluZGV4KS8yKTsKCiAgICB3aGlsZShpdGVtc1ttaWRkbGVJbmRleF0gIT0gdmFsdWUgJiYgZmlyc3RJbmRleCA8IGxhc3RJbmRleCkKICAgIHsKICAgICAgIGlmICh2YWx1ZSA8IGl0ZW1zW21pZGRsZUluZGV4XSkKICAgICAgICB7CiAgICAgICAgICAgIGxhc3RJbmRleCA9IG1pZGRsZUluZGV4IC0gMTsKICAgICAgICB9IAogICAgICBlbHNlIGlmICh2YWx1ZSA+IGl0ZW1zW21pZGRsZUluZGV4XSkKICAgICAgICB7CiAgICAgICAgICAgIGZpcnN0SW5kZXggPSBtaWRkbGVJbmRleCArIDE7CiAgICAgICAgfQogICAgICAgIG1pZGRsZUluZGV4ID0gTWF0aC5mbG9vcigobGFzdEluZGV4ICsgZmlyc3RJbmRleCkvMik7CiAgICB9CgogcmV0dXJuIChpdGVtc1ttaWRkbGVJbmRleF0gIT0gdmFsdWUpID8gLTEgOiBtaWRkbGVJbmRleDsKfQp2YXIgaXRlbXMgPSBbMSwgMiwgMywgNCwgNSwgNywgOCwgOV07CmNvbnNvbGUubG9nKGJpbmFyeV9TZWFyY2goaXRlbXMsIDEpKTsgICAKY29uc29sZS5sb2coYmluYXJ5X1NlYXJjaChpdGVtcywgNSkpOwoKUTMwLiBXaGF0IGFyZSB0aGUgdHlwZXMgb2YgUG9wIHVwIGJveGVzIGF2YWlsYWJsZSBpbiBKYXZhU2NyaXB0PwoKSmF2YVNjcmlwdCBwcm92aWRlcyB0aHJlZSB0eXBlcyBvZiBwb3AtdXAgYm94ZXMgb3IgZGlhbG9nIGJveGVzOgoKQWxlcnQ6CgpUaGUgYWxlcnQoKSBtZXRob2QgZGlzcGxheXMgYSBzaW1wbGUgZGlhbG9nIGJveCB3aXRoIGEgbWVzc2FnZSBhbmQgYW4gT0sgYnV0dG9uLgpJdCBpcyBjb21tb25seSB1c2VkIHRvIHNob3cgaW5mb3JtYXRpb25hbCBtZXNzYWdlcyBvciB0byBhbGVydCB1c2VycyBvZiBjZXJ0YWluIGNvbmRpdGlvbnMuClRoZSBkaWFsb2cgYm94IGJsb2NrcyBmdXJ0aGVyIGV4ZWN1dGlvbiB1bnRpbCB0aGUgdXNlciBjbGlja3MgdGhlIE9LIGJ1dHRvbi4KRXhhbXBsZTrCoCBhbGVydCgiVGhpcyBpcyBhbiBhbGVydCBtZXNzYWdlLiIpOwoKCkNvbmZpcm06CgpUaGUgY29uZmlybSgpIG1ldGhvZCBkaXNwbGF5cyBhIGRpYWxvZyBib3ggd2l0aCBhIG1lc3NhZ2UsIGFsb25nIHdpdGggT0sgYW5kIENhbmNlbCBidXR0b25zLgpJdCBpcyB1c2VkIHRvIGFzayB0aGUgdXNlciBmb3IgY29uZmlybWF0aW9uIG9yIHRvIGdldCBhIHllcy9ubyByZXNwb25zZS4KVGhlIGZ1bmN0aW9uIHJldHVybnMgYSBCb29sZWFuIHZhbHVlICh0cnVlIGlmIHRoZSB1c2VyIGNsaWNrcyBPSyBhbmQgZmFsc2UgaWYgdGhlIHVzZXIgY2xpY2tzIENhbmNlbCkuCkV4YW1wbGU6IHZhciByZXN1bHQgPSBjb25maXJtKCJBcmUgeW91IHN1cmUgeW91IHdhbnQgdG8gcHJvY2VlZD8iKTsKaWYgKHJlc3VsdCkgewovLyBVc2VyIGNsaWNrZWQgT0sKfSBlbHNlIHsKLy8gVXNlciBjbGlja2VkIENhbmNlbAp9CgoKUHJvbXB0OgoKVGhlIHByb21wdCgpIG1ldGhvZCBkaXNwbGF5cyBhIGRpYWxvZyBib3ggd2l0aCBhIG1lc3NhZ2UsIGFuIGlucHV0IGZpZWxkIGZvciB0aGUgdXNlciB0byBlbnRlciBkYXRhLCBhbmQgT0svQ2FuY2VsIGJ1dHRvbnMuCkl0IGlzIHVzZWQgdG8gZ2V0IHVzZXIgaW5wdXQgb3IgdG8gcHJvbXB0IHRoZSB1c2VyIGZvciBpbmZvcm1hdGlvbi4KVGhlIG1ldGhvZCB0YWtlcyBhbiBvcHRpb25hbCBkZWZhdWx0IHZhbHVlIGFzIGEgcGFyYW1ldGVyIGFuZCByZXR1cm5zIHRoZSB2YWx1ZSBlbnRlcmVkIGJ5IHRoZSB1c2VyIGFzIGEgc3RyaW5nLCBvciBudWxsIGlmIHRoZSB1c2VyIGNsaWNrcyBDYW5jZWwuCkV4YW1wbGU6IHZhciBuYW1lID0gcHJvbXB0KCJQbGVhc2UgZW50ZXIgeW91ciBuYW1lOiIsICJKb2huIERvZSIpOwppZiAobmFtZSAhPT0gbnVsbCkgewovLyBVc2VyIGVudGVyZWQgYSB2YWx1ZQp9IGVsc2UgewovLyBVc2VyIGNsaWNrZWQgQ2FuY2VsCn0KCkNPRElORyBJTlRFUlZJRVcKUTMxLiBHdWVzcyB0aGUgb3V0cHV0IG9mIHRoZSBmb2xsb3dpbmcgY29kZT8KCmZ1bmN0aW9uIGZ1bmMxKCl7CiAgc2V0VGltZW91dCgoKT0+ewogICAgY29uc29sZS5sb2coeCk7CiAgICBjb25zb2xlLmxvZyh5KTsKICB9LDMwMDApOwoKICB2YXIgeCA9IDI7CiAgbGV0IHkgPSAxMjsKfQoKZnVuYzEoKTsKCgpPdXRwdXTinpYgMgpSZWZlcmVuY2VFcnJvcjogeSBpcyBub3QgZGVmaW5lZAoKCkV4cGxhbmF0aW9uOgoKVGhlIGZ1bmMxKCkgZnVuY3Rpb24gaXMgZGVmaW5lZCBhbmQgaW52b2tlZC4KSW5zaWRlIGZ1bmMxKCksIGEgc2V0VGltZW91dCgpIGZ1bmN0aW9uIGlzIGNhbGxlZCB3aXRoIGEgY2FsbGJhY2sgYXJyb3cgZnVuY3Rpb24uIFRoZSBjYWxsYmFjayBmdW5jdGlvbiBpcyBzY2hlZHVsZWQgdG8gZXhlY3V0ZSBhZnRlciBhIGRlbGF5IG9mIDMwMDAgbWlsbGlzZWNvbmRzICgzIHNlY29uZHMpLgpUaGUgdmFyaWFibGUgeCBpcyBkZWNsYXJlZCB1c2luZyB0aGUgdmFyIGtleXdvcmQgYW5kIGFzc2lnbmVkIGEgdmFsdWUgb2YgMi4gdmFyIGRlY2xhcmF0aW9ucyBhcmUgaG9pc3RlZCwgc28gdGhlIGRlY2xhcmF0aW9uIGlzIG1vdmVkIHRvIHRoZSB0b3Agb2YgdGhlIGZ1bmN0aW9uIHNjb3BlLiBIb3dldmVyLCB0aGUgYXNzaWdubWVudCBvZiAyIHRvIHggaGFwcGVucyBhdCB0aGUgbGluZSB3aGVyZSBpdCBpcyB3cml0dGVuLgpUaGUgdmFyaWFibGUgeSBpcyBkZWNsYXJlZCB1c2luZyB0aGUgbGV0IGtleXdvcmQgYW5kIGFzc2lnbmVkIGEgdmFsdWUgb2YgMTIuIGxldCBkZWNsYXJhdGlvbnMgYXJlIGJsb2NrLXNjb3BlZCBhbmQgbm90IGhvaXN0ZWQsIHNvIHRoZSBkZWNsYXJhdGlvbiBhbmQgYXNzaWdubWVudCBvZiB5IGhhcHBlbiBhdCB0aGUgc2FtZSBsaW5lLgpXaGVuIHRoZSBjYWxsYmFjayBhcnJvdyBmdW5jdGlvbiBpbnNpZGUgc2V0VGltZW91dCgpIGlzIGV4ZWN1dGVkIGFmdGVyIHRoZSBkZWxheSwgaXQgdHJpZXMgdG8gbG9nIHRoZSB2YWx1ZXMgb2YgeCBhbmQgeS4KVGhlIHZhbHVlIG9mIHggaXMgMiBiZWNhdXNlIGl0IHdhcyBkZWNsYXJlZCB1c2luZyB2YXIgYW5kIGlzIGFjY2Vzc2libGUgd2l0aGluIHRoZSBmdW5jdGlvbiBzY29wZS4KSG93ZXZlciwgYSBSZWZlcmVuY2UgRXJyb3Igb2NjdXJzIHdoZW4gdHJ5aW5nIHRvIGxvZyB0aGUgdmFsdWUgb2YgeS4gVGhpcyBpcyBiZWNhdXNlIHkgaXMgZGVjbGFyZWQgdXNpbmcgbGV0IGFuZCBpcyBibG9jay1zY29wZWQgdG8gdGhlIGZ1bmMxKCkgZnVuY3Rpb24gYmxvY2suIEl0IGlzIG5vdCBhY2Nlc3NpYmxlIGluc2lkZSB0aGUgY2FsbGJhY2sgZnVuY3Rpb24gc2luY2UgaXQgaGFzIGl0cyBvd24gYmxvY2sgc2NvcGUuCgpUaGVyZWZvcmUsIHRoZSBjb2RlIGxvZ3MgdGhlIHZhbHVlIG9mIHggYXMgMiwgYnV0IGFuIGVycm9yIG9jY3VycyB3aGVuIHRyeWluZyB0byBsb2cgdGhlIHZhbHVlIG9mIHkuCgoKCgpRMzIuIEd1ZXNzIHRoZSBvdXRwdXQgb2YgdGhlIGZvbGxvd2luZyBjb2RlLgpmdW5jdGlvbiBmdW5jMigpewogIGZvcih2YXIgaSA9IDA7IGkgPCAzOyBpKyspewogICAgc2V0VGltZW91dCgoKT0+IGNvbnNvbGUubG9nKGkpLDIwMDApOwp9Cgp9CgpmdW5jMigpOwoKCk91dHB1dHPinpYKCjMKMwozCgoKRXhwbGFuYXRpb246CgpUaGUgZnVuYzIoKSBmdW5jdGlvbiBpcyBkZWZpbmVkIGFuZCBpbnZva2VkLgpJbnNpZGUgZnVuYzIoKSwgYSBmb3IgbG9vcCBpcyB1c2VkIHRvIGl0ZXJhdGUgZnJvbSAwIHRvIDIgKDMgaXRlcmF0aW9ucykuCkZvciBlYWNoIGl0ZXJhdGlvbiBvZiB0aGUgbG9vcCwgYSBzZXRUaW1lb3V0KCkgZnVuY3Rpb24gaXMgY2FsbGVkIHdpdGggYSBjYWxsYmFjayBhcnJvdyBmdW5jdGlvbi4gVGhlIGNhbGxiYWNrIGZ1bmN0aW9uIGlzIHNjaGVkdWxlZCB0byBleGVjdXRlIGFmdGVyIGEgZGVsYXkgb2YgMjAwMCBtaWxsaXNlY29uZHMgKDIgc2Vjb25kcykuClRoZSBjYWxsYmFjayBmdW5jdGlvbiBsb2dzIHRoZSB2YWx1ZSBvZiBpLgpTaW5jZSB0aGUgc2V0VGltZW91dCgpIGZ1bmN0aW9uIGlzIGFzeW5jaHJvbm91cywgdGhlIGxvb3AgY29udGludWVzIGV4ZWN1dGluZyB3aXRob3V0IHdhaXRpbmcgZm9yIHRoZSBjYWxsYmFjayBmdW5jdGlvbnMgdG8gZXhlY3V0ZS4KQWZ0ZXIgdGhlIGxvb3AgZmluaXNoZXMsIHRoZSB2YWx1ZSBvZiBpIGlzIDMgYmVjYXVzZSB0aGUgbG9vcCBjb25kaXRpb24gKGkgPCAzKSBiZWNvbWVzIGZhbHNlLCBhbmQgdGhlIGxvb3AgdGVybWluYXRlcy4KQXMgYSByZXN1bHQsIHdoZW4gdGhlIGNhbGxiYWNrIGZ1bmN0aW9ucyBleGVjdXRlIGFmdGVyIHRoZSBkZWxheSwgdGhleSBhY2Nlc3MgYW5kIGxvZyB0aGUgZmluYWwgdmFsdWUgb2YgaSwgd2hpY2ggaXMgMy4KVGhlcmVmb3JlLCB0aGUgY29kZSBsb2dzIDMgdGhyZWUgdGltZXMsIGluZGljYXRpbmcgdGhlIHZhbHVlIG9mIGkgYXQgdGhlIHRpbWUgdGhlIGNhbGxiYWNrIGZ1bmN0aW9ucyBleGVjdXRlLgoKCgoKUTMzLiBHdWVzcyB0aGUgb3V0cHV0IG9mIHRoZSBmb2xsb3dpbmcgY29kZS4KKGZ1bmN0aW9uKCl7CiAgc2V0VGltZW91dCgoKT0+IGNvbnNvbGUubG9nKDEpLDIwMDApOwogIGNvbnNvbGUubG9nKDIpOwogIHNldFRpbWVvdXQoKCk9PiBjb25zb2xlLmxvZygzKSwwKTsKICBjb25zb2xlLmxvZyg0KTsKfSkoKTsKCgpPdXRwdXTinpYKCjIKNAozCjEKCgpFeHBsYW5hdGlvbjoKClRoZSBjb2RlIGRlZmluZXMgYW4gaW1tZWRpYXRlbHkgaW52b2tlZCBmdW5jdGlvbiBleHByZXNzaW9uIChJSUZFKSB1c2luZyAoZnVuY3Rpb24oKXsgLi4uIH0pKCk7LiBUaGlzIGFsbG93cyB0aGUgY29kZSBpbnNpZGUgdGhlIGZ1bmN0aW9uIHRvIGJlIGV4ZWN1dGVkIGltbWVkaWF0ZWx5LgpJbnNpZGUgdGhlIElJRkUsIHRoZSBmb2xsb3dpbmcgYWN0aW9ucyBvY2N1cjoKVGhlIGZpcnN0IHNldFRpbWVvdXQoKSBmdW5jdGlvbiBpcyBjYWxsZWQgd2l0aCBhIGNhbGxiYWNrIGFycm93IGZ1bmN0aW9uLCB3aGljaCBsb2dzIDEsIGFuZCBhIGRlbGF5IG9mIDIwMDAgbWlsbGlzZWNvbmRzICgyIHNlY29uZHMpLiBUaGlzIG1lYW5zIGl0IHdpbGwgZXhlY3V0ZSBhZnRlciBhIDItc2Vjb25kIGRlbGF5LgpUaGUgdmFsdWUgMiBpcyBsb2dnZWQgdG8gdGhlIGNvbnNvbGUgaW1tZWRpYXRlbHkuClRoZSBzZWNvbmQgc2V0VGltZW91dCgpIGZ1bmN0aW9uIGlzIGNhbGxlZCB3aXRoIGEgY2FsbGJhY2sgYXJyb3cgZnVuY3Rpb24sIHdoaWNoIGxvZ3MgMywgYW5kIGEgZGVsYXkgb2YgMCBtaWxsaXNlY29uZHMuIERlc3BpdGUgdGhlIGRlbGF5IGJlaW5nIHNldCB0byAwLCBpdCBpcyBlZmZlY3RpdmVseSBhIG1pbmltdW0gZGVsYXksIGFsbG93aW5nIG90aGVyIHRhc2tzIGluIHRoZSBldmVudCBsb29wIHRvIGJlIHByb2Nlc3NlZCBiZWZvcmUgdGhlIGNhbGxiYWNrIGlzIGV4ZWN1dGVkLgpUaGUgdmFsdWUgNCBpcyBsb2dnZWQgdG8gdGhlIGNvbnNvbGUgaW1tZWRpYXRlbHkuClNpbmNlIHRoZSBzZWNvbmQgc2V0VGltZW91dCgpIGZ1bmN0aW9uIGhhcyBhIGRlbGF5IG9mIDAsIGl0IGlzIHBsYWNlZCBpbiB0aGUgZXZlbnQgcXVldWUgYW5kIHdhaXRzIGZvciB0aGUgY2FsbCBzdGFjayB0byBjbGVhciBiZWZvcmUgZXhlY3V0aW5nIGl0cyBjYWxsYmFjay4KQWZ0ZXIgdGhlIElJRkUgZmluaXNoZXMgZXhlY3V0aW5nLCB0aGUgZXZlbnQgbG9vcCBwcm9jZXNzZXMgdGhlIGNhbGxiYWNrIGZ1bmN0aW9ucyB3YWl0aW5nIGluIHRoZSBldmVudCBxdWV1ZS4KVGhlIGNhbGxiYWNrIGZ1bmN0aW9uIGZyb20gdGhlIHNlY29uZCBzZXRUaW1lb3V0KCkgKHdpdGggYSBkZWxheSBvZiAwKSBpcyBwcm9jZXNzZWQgZmlyc3QgYW5kIGxvZ3MgMy4KTGFzdGx5LCBhZnRlciBhIDItc2Vjb25kIGRlbGF5LCB0aGUgY2FsbGJhY2sgZnVuY3Rpb24gZnJvbSB0aGUgZmlyc3Qgc2V0VGltZW91dCgpIGV4ZWN1dGVzIGFuZCBsb2dzIDEuCgoKUTM0LiBHdWVzcyB0aGUgb3V0cHV0IG9mIHRoZSBmb2xsb3dpbmcgY29kZS4KZnVuY3Rpb24gcnVuRnVuYygpewogIGNvbnNvbGUubG9nKCIxIiArIDEpOwogIGNvbnNvbGUubG9nKCJBIiAtIDEpOwogIGNvbnNvbGUubG9nKDIgKyAiLTIiICsgIjIiKTsKICBjb25zb2xlLmxvZygiSGVsbG8iIC0gIldvcmxkIiArIDc4KTsKICBjb25zb2xlLmxvZygiSGVsbG8iKyAiNzgiKTsKfQoKcnVuRnVuYygpOwoKCk91dHB1dOKelgoKMTEKTmFOCjItMjIKTmFONzgKSGVsbG83OAoKCkV4cGxhbmF0aW9uOgoKVGhlIHJ1bkZ1bmMoKSBmdW5jdGlvbiBpcyBkZWZpbmVkIGFuZCBpbnZva2VkLgpJbnNpZGUgcnVuRnVuYygpLCBzZXZlcmFsIGNvbnNvbGUubG9nKCkgc3RhdGVtZW50cyBhcmUgdXNlZCB0byBwcmludCBkaWZmZXJlbnQgZXhwcmVzc2lvbnMuCkxldCdzIGJyZWFrIGRvd24gdGhlIG91dHB1dCBsaW5lIGJ5IGxpbmU6CgoiMSIgKyAxIC0gVGhlICsgb3BlcmF0b3IgcGVyZm9ybXMgY29uY2F0ZW5hdGlvbiB3aGVuIG9uZSBvciBib3RoIG9wZXJhbmRzIGFyZSBzdHJpbmdzLiBJbiB0aGlzIGNhc2UsICIxIiBpcyBhIHN0cmluZywgYW5kIDEgaXMgYSBudW1iZXIuIFRoZSBudW1iZXIgMSBpcyBjb2VyY2VkIGludG8gYSBzdHJpbmcgYW5kIGNvbmNhdGVuYXRlZCB3aXRoICIxIiwgcmVzdWx0aW5nIGluIHRoZSBzdHJpbmcgIjExIi4KCiJBIiAtIDEgLSBUaGUgLSBvcGVyYXRvciBpcyBub3QgZGVmaW5lZCBmb3Igc3RyaW5nIHN1YnRyYWN0aW9uLiBKYXZhU2NyaXB0IHRyaWVzIHRvIHBlcmZvcm0gYXJpdGhtZXRpYyBvcGVyYXRpb25zIG9uIHRoZSBvcGVyYW5kcy4gU2luY2UgIkEiIGNhbm5vdCBiZSBjb252ZXJ0ZWQgaW50byBhIG51bWJlciwgdGhlIG9wZXJhdGlvbiByZXN1bHRzIGluIE5hTiAoTm90LWEtTnVtYmVyKS4KCjIgKyAiLTIiICsgIjIiIC0gVGhlICsgb3BlcmF0b3IsIHdoZW4gdXNlZCB3aXRoIHN0cmluZ3MsIHBlcmZvcm1zIGNvbmNhdGVuYXRpb24uIFRoZSBmaXJzdCBvcGVyYXRpb24gaXMgMiArICItMiIsIHdoaWNoIGNvbmNhdGVuYXRlcyB0aGUgbnVtYmVyIDIgd2l0aCB0aGUgc3RyaW5nICItMiIsIHJlc3VsdGluZyBpbiAiMi0yIi4gVGhlIHN1YnNlcXVlbnQgY29uY2F0ZW5hdGlvbiB3aXRoIHRoZSBzdHJpbmcgIjIiIHJlc3VsdHMgaW4gIjItMjIiLgoKIkhlbGxvIiAtICJXb3JsZCIgKyA3OCAtIFRoZSAtIG9wZXJhdG9yIGlzIG5vdCBkZWZpbmVkIGZvciBzdHJpbmcgc3VidHJhY3Rpb24uIEJvdGggIkhlbGxvIiBhbmQgIldvcmxkIiBjYW5ub3QgYmUgY29udmVydGVkIGludG8gbnVtYmVycywgcmVzdWx0aW5nIGluIE5hTi4gV2hlbiBOYU4gaXMgaW52b2x2ZWQgaW4gYW55IGFyaXRobWV0aWMgb3BlcmF0aW9uLCB0aGUgcmVzdWx0IGlzIGFsd2F5cyBOYU4uIFRoZSBzdWJzZXF1ZW50IGFkZGl0aW9uIG9mIDc4IHdpdGggTmFOIHN0aWxsIHJlc3VsdHMgaW4gTmFOLgoKIkhlbGxvIiArICI3OCIgLSBCb3RoIG9wZXJhbmRzIGFyZSBzdHJpbmdzLCBzbyB0aGUgKyBvcGVyYXRvciBwZXJmb3JtcyBjb25jYXRlbmF0aW9uLiBJdCBjb25jYXRlbmF0ZXMgdGhlIHN0cmluZyAiSGVsbG8iIHdpdGggdGhlIHN0cmluZyAiNzgiLCByZXN1bHRpbmcgaW4gdGhlIHN0cmluZyAiSGVsbG83OCIuCgpRMzUuIEluIHdoaWNoIGxvY2F0aW9uIGNvb2tpZXMgYXJlIHN0b3JlZCBvbiB0aGUgaGFyZCBkaXNrPwoKQ29va2llcyBhcmUgbm90IHN0b3JlZCBkaXJlY3RseSBvbiB0aGUgaGFyZCBkaXNrIG9mIGEgdXNlcidzIGRldmljZS4gSW5zdGVhZCwgdGhleSBhcmUgc3RvcmVkIGJ5IHdlYiBicm93c2VycyBpbiBhIGRlc2lnbmF0ZWQgc3RvcmFnZSBsb2NhdGlvbiBzcGVjaWZpYyB0byBlYWNoIGJyb3dzZXIuIFRoZSBhY3R1YWwgc3RvcmFnZSBsb2NhdGlvbiBtYXkgdmFyeSBkZXBlbmRpbmcgb24gdGhlIG9wZXJhdGluZyBzeXN0ZW0gYW5kIGJyb3dzZXIgYmVpbmcgdXNlZC4gSGVyZSBhcmUgc29tZSBjb21tb24gc3RvcmFnZSBsb2NhdGlvbnMgZm9yIGNvb2tpZXM6CgpXaW5kb3dzOgoKR29vZ2xlIENocm9tZTogQzpcVXNlcnNce3VzZXJuYW1lfVxBcHBEYXRhXExvY2FsXEdvb2dsZVxDaHJvbWVcVXNlciBEYXRhXERlZmF1bHRcQ29va2llcwpNb3ppbGxhIEZpcmVmb3g6IEM6XFVzZXJzXHt1c2VybmFtZX1cQXBwRGF0YVxSb2FtaW5nXE1vemlsbGFcRmlyZWZveFxQcm9maWxlc1x7cHJvZmlsZV9mb2xkZXJ9XGNvb2tpZXMuc3FsaXRlCk1pY3Jvc29mdCBFZGdlOiBDOlxVc2Vyc1x7dXNlcm5hbWV9XEFwcERhdGFcTG9jYWxcTWljcm9zb2Z0XEVkZ2VcVXNlciBEYXRhXERlZmF1bHRcQ29va2llcwoKbWFjT1M6CgpHb29nbGUgQ2hyb21lOiB+L0xpYnJhcnkvQXBwbGljYXRpb24gU3VwcG9ydC9Hb29nbGUvQ2hyb21lL0RlZmF1bHQvQ29va2llcwpNb3ppbGxhIEZpcmVmb3g6IH4vTGlicmFyeS9BcHBsaWNhdGlvbiBTdXBwb3J0L0ZpcmVmb3gvUHJvZmlsZXMve3Byb2ZpbGVfZm9sZGVyfS9jb29raWVzLnNxbGl0ZQpTYWZhcmk6IH4vTGlicmFyeS9Db29raWVzL0Nvb2tpZXMuYmluYXJ5Y29va2llcwoKTGludXg6CgpHb29nbGUgQ2hyb21lOiB+Ly5jb25maWcvZ29vZ2xlLWNocm9tZS9EZWZhdWx0L0Nvb2tpZXMKTW96aWxsYSBGaXJlZm94OiB+Ly5tb3ppbGxhL2ZpcmVmb3gve3Byb2ZpbGVfZm9sZGVyfS9jb29raWVzLnNxbGl0ZQoKUGxlYXNlIG5vdGUgdGhhdCB0aGUgYWN0dWFsIHBhdGhzIG1heSBkaWZmZXIgYmFzZWQgb24gdGhlIHZlcnNpb24gb2YgdGhlIGJyb3dzZXIsIHVzZXIgcHJvZmlsZSwgYW5kIGJyb3dzZXIgc2V0dGluZ3MuIFRoZSBwYXRocyBwcm92aWRlZCBoZXJlIGFyZSBnZW5lcmFsIGV4YW1wbGVzLCBhbmQgaXQncyBhbHdheXMgcmVjb21tZW5kZWQgdG8gY29uc3VsdCB0aGUgYnJvd3NlcidzIGRvY3VtZW50YXRpb24gb3Igc2V0dGluZ3MgZm9yIHRoZSBwcmVjaXNlIGxvY2F0aW9uIG9mIGNvb2tpZSBzdG9yYWdlLgoKUTM2LiBXaGF0IGlzIGEgcHJvbXB0IGJveD8KClByb21wdCBCb3ggaXMgYSBwb3B1cCBib3ggYXZhaWxhYmxlIGluIEphdmEgU2NyaXB0LgoKQSBwcm9tcHQgYm94IGlzIG9mdGVuIHVzZWQgaWYgeW91IHdhbnQgdGhlIHVzZXIgdG8gaW5wdXQgYSB2YWx1ZSBiZWZvcmUgZW50ZXJpbmcgYSBwYWdlLgoKV2hlbiBhIHByb21wdCBib3ggcG9wcyB1cCwgdGhlIHVzZXIgd2lsbCBoYXZlIHRvIGNsaWNrIGVpdGhlciAiT0siIG9yICJDYW5jZWwiIHRvIHByb2NlZWQgYWZ0ZXIgZW50ZXJpbmcgYW4gaW5wdXQgdmFsdWUuCgpJZiB0aGUgdXNlciBjbGlja3MgIk9LIiB0aGUgYm94IHJldHVybnMgdGhlIGlucHV0IHZhbHVlLiBJZiB0aGUgdXNlciBjbGlja3MgIkNhbmNlbCIgdGhlIGJveCByZXR1cm5zIG51bGwuCgpTeW50YXjinpYgd2luZG93cy5wcm9tcHQo4oCdc29tZXR4dOKAnSzigJ1kZWZhdWx0dHh04oCdKTsKCmxldCBwZXJzb24gPSBwcm9tcHQoIlBsZWFzZSBlbnRlciB5b3VyIG5hbWUiLCAiSGFycnkgUG90dGVyIik7CmxldCB0ZXh0OwppZiAocGVyc29uID09IG51bGwgfHwgcGVyc29uID09ICIiKSB7CiAgdGV4dCA9ICJVc2VyIGNhbmNlbGxlZCB0aGUgcHJvbXB0LiI7Cn0gCmVsc2UgCnsKICB0ZXh0ID0gIkhlbGxvICIgKyBwZXJzb24gKyAiISBIb3cgYXJlIHlvdSB0b2RheT8iOwp9CgoKClEzOC4gV2hpY2ggc3ltYm9sIGlzIHVzZWQgZm9yIGNvbW1lbnRzIGluIEphdmFTY3JpcHQ/CgpJbiBKYXZhU2NyaXB0LCB0aGVyZSBhcmUgdHdvIHN5bWJvbHMgY29tbW9ubHkgdXNlZCBmb3IgY29tbWVudHM6CgpEb3VibGUgU2xhc2ggKC8vKToKClRoZSBkb3VibGUgc2xhc2ggLy8gaXMgdXNlZCBmb3Igc2luZ2xlLWxpbmUgY29tbWVudHMuCkFueXRoaW5nIGFmdGVyIC8vIG9uIHRoZSBzYW1lIGxpbmUgaXMgY29uc2lkZXJlZCBhIGNvbW1lbnQgYW5kIGlzIGlnbm9yZWQgYnkgdGhlIEphdmFTY3JpcHQgaW50ZXJwcmV0ZXIuCgpTbGFzaCBBc3RlcmlzayAoLyogKi8pOgoKVGhlIHNsYXNoIGFzdGVyaXNrIC8qICovIGlzIHVzZWQgZm9yIG11bHRpLWxpbmUgY29tbWVudHMgb3IgYmxvY2sgY29tbWVudHMuCkFueXRoaW5nIGJldHdlZW4gLyogYW5kICovIGlzIGNvbnNpZGVyZWQgYSBjb21tZW50IGFuZCBpcyBpZ25vcmVkIGJ5IHRoZSBKYXZhU2NyaXB0IGludGVycHJldGVyLCBldmVuIGlmIGl0IHNwYW5zIG11bHRpcGxlIGxpbmVzLgoKCgoKUTM5LiBXaGF0IGlzIHRoZSBkaWZmZXJlbmNlIGJldHdlZW4gdmlldyBzdGF0ZSBhbmQgc2Vzc2lvbiBzdGF0ZT8KClZpZXcgc3RhdGUgYW5kIHNlc3Npb24gc3RhdGUgYXJlIGJvdGggY29uY2VwdHMgcmVsYXRlZCB0byBtYW5hZ2luZyBzdGF0ZSBpbiB3ZWIgYXBwbGljYXRpb25zLCBidXQgdGhleSBzZXJ2ZSBkaWZmZXJlbnQgcHVycG9zZXMgYW5kIGFyZSB1c2VkIGluIGRpZmZlcmVudCBjb250ZXh0czoKClZpZXcgU3RhdGU6CgpWaWV3IHN0YXRlIGlzIHNwZWNpZmljIHRvIEFTUC5ORVQgd2ViIGZvcm1zIGFuZCBpcyB1c2VkIHRvIG1haW50YWluIHRoZSBzdGF0ZSBvZiBhIHdlYiBmb3JtIGFjcm9zcyBwb3N0YmFja3MuCkl0IGlzIGFuIGVuY29kZWQgcmVwcmVzZW50YXRpb24gb2YgdGhlIHN0YXRlIG9mIHRoZSBjb250cm9scyBvbiBhIHdlYiBmb3JtLCBpbmNsdWRpbmcgdGhlaXIgdmFsdWVzIGFuZCBwcm9wZXJ0aWVzLgpWaWV3IHN0YXRlIGlzIHN0b3JlZCBhcyBhIGhpZGRlbiBmaWVsZCBpbiB0aGUgSFRNTCBmb3JtIGFuZCBpcyBzZW50IGJhY2sgYW5kIGZvcnRoIGJldHdlZW4gdGhlIHNlcnZlciBhbmQgdGhlIGNsaWVudCB3aXRoIGVhY2ggcmVxdWVzdCBhbmQgcmVzcG9uc2UuCkl0IGFsbG93cyB0aGUgd2ViIGZvcm0gdG8gcmVtZW1iZXIgdXNlciBpbnB1dCBhbmQgbWFpbnRhaW4gaXRzIHN0YXRlIGR1cmluZyBwb3N0YmFja3MsIGVuYWJsaW5nIHRoZSBmb3JtIHRvIHJlc3RvcmUgaXRzIGRhdGEgYW5kIGFwcGVhcmFuY2UuClZpZXcgc3RhdGUgaXMgcHJpbWFyaWx5IHVzZWQgZm9yIG1haW50YWluaW5nIHN0YXRlIHdpdGhpbiBhIHNpbmdsZSB1c2VyIHNlc3Npb24gYW5kIGlzIHRpZWQgdG8gdGhlIGluZGl2aWR1YWwgd2ViIGZvcm0uCgpTZXNzaW9uIFN0YXRlOgoKU2Vzc2lvbiBzdGF0ZSBpcyBhIHNlcnZlci1zaWRlIG1lY2hhbmlzbSBpbiB3ZWIgZGV2ZWxvcG1lbnQgdGhhdCBhbGxvd3Mgc3RvcmluZyBhbmQgcmV0cmlldmluZyB1c2VyLXNwZWNpZmljIGRhdGEgYWNyb3NzIG11bHRpcGxlIHJlcXVlc3RzIGFuZCBzZXNzaW9ucy4KSXQgaXMgdXNlZCB0byBzdG9yZSBkYXRhIHRoYXQgbmVlZHMgdG8gYmUgcGVyc2lzdGVkIGFuZCBhY2Nlc3NlZCBhY3Jvc3MgZGlmZmVyZW50IHBhZ2VzIG9yIGludGVyYWN0aW9ucyBkdXJpbmcgYSB1c2VyIHNlc3Npb24uClNlc3Npb24gc3RhdGUgZGF0YSBpcyBzdG9yZWQgb24gdGhlIHNlcnZlciwgdXN1YWxseSBpbiBtZW1vcnkgb3IgYSBkYXRhYmFzZSwgYW5kIGlzIGFzc29jaWF0ZWQgd2l0aCBhIHVuaXF1ZSBzZXNzaW9uIGlkZW50aWZpZXIgKHVzdWFsbHkgc3RvcmVkIGluIGEgY29va2llIG9yIFVSTCBwYXJhbWV0ZXIpLgpTZXNzaW9uIHN0YXRlIGNhbiBzdG9yZSBhbnkgc2VyaWFsaXphYmxlIG9iamVjdCBvciBkYXRhLCBzdWNoIGFzIHVzZXIgcHJlZmVyZW5jZXMsIHNob3BwaW5nIGNhcnQgY29udGVudHMsIG9yIHVzZXIgYXV0aGVudGljYXRpb24gaW5mb3JtYXRpb24uClRoZSBzZXNzaW9uIHN0YXRlIGlzIGluZGVwZW5kZW50IG9mIGEgc3BlY2lmaWMgd2ViIGZvcm0gYW5kIGNhbiBiZSBhY2Nlc3NlZCBhbmQgbW9kaWZpZWQgZnJvbSBtdWx0aXBsZSBwYWdlcyB3aXRoaW4gdGhlIHNhbWUgc2Vzc2lvbi4KCkluIHN1bW1hcnksIHZpZXcgc3RhdGUgaXMgc3BlY2lmaWMgdG8gQVNQLk5FVCB3ZWIgZm9ybXMgYW5kIGlzIHVzZWQgdG8gbWFpbnRhaW4gdGhlIHN0YXRlIG9mIGEgc2luZ2xlIHdlYiBmb3JtIGFjcm9zcyBwb3N0YmFja3MsIHdoaWxlIHNlc3Npb24gc3RhdGUgaXMgYSBtb3JlIGdlbmVyYWwgbWVjaGFuaXNtIGZvciBzdG9yaW5nIGFuZCBhY2Nlc3NpbmcgdXNlci1zcGVjaWZpYyBkYXRhIGFjcm9zcyBtdWx0aXBsZSByZXF1ZXN0cyBhbmQgc2Vzc2lvbnMuCgpRNDAuIEhvdyBjYW4geW91IHN1Ym1pdCBhIGZvcm0gdXNpbmcgSmF2YVNjcmlwdD8KClRvIHN1Ym1pdCBhIGZvcm0gdXNpbmcgSmF2YVNjcmlwdCwgeW91IGNhbiB1c2UgdGhlIHN1Ym1pdCgpIG1ldGhvZCwgd2hpY2ggaXMgYXZhaWxhYmxlIG9uIHRoZSBmb3JtIGVsZW1lbnQuIFRoaXMgbWV0aG9kIGFsbG93cyB5b3UgdG8gcHJvZ3JhbW1hdGljYWxseSB0cmlnZ2VyIHRoZSBzdWJtaXNzaW9uIG9mIGEgZm9ybS4KCkhlcmUncyBhbiBleGFtcGxlIG9mIGhvdyB5b3UgY2FuIHN1Ym1pdCBhIGZvcm0gdXNpbmcgSmF2YVNjcmlwdDoKCjxmb3JtIGFjdGlvbj0iL3N1Ym1pdCIgbWV0aG9kPSJQT1NUIj4KPCEtLSBmb3JtIGZpZWxkcyAtLT4KPGlucHV0IHR5cGU9InRleHQiIG5hbWU9InVzZXJuYW1lIj4KPGlucHV0IHR5cGU9InBhc3N3b3JkIiBuYW1lPSJwYXNzd29yZCI+CjxpbnB1dCB0eXBlPSJzdWJtaXQiIHZhbHVlPSJTdWJtaXQiPgo8L2Zvcm0+Cgo8c2NyaXB0PgovLyBHZXQgYSByZWZlcmVuY2UgdG8gdGhlIGZvcm0gZWxlbWVudApjb25zdCBmb3JtID0gZG9jdW1lbnQuZ2V0RWxlbWVudEJ5SWQoJ215Rm9ybScpOwoKLy8gU3VibWl0IHRoZSBmb3JtIHByb2dyYW1tYXRpY2FsbHkKZm9ybS5zdWJtaXQoKTsKPC9zY3JpcHQ+CgpJbiB0aGlzIGV4YW1wbGUsIHdlIGhhdmUgYW4gSFRNTCBmb3JtIHdpdGggdGhlIGlkIGF0dHJpYnV0ZSBzZXQgdG8gIm15Rm9ybSIuIFdlIHRoZW4gdXNlIEphdmFTY3JpcHQgdG8gZ2V0IGEgcmVmZXJlbmNlIHRvIHRoZSBmb3JtIGVsZW1lbnQgdXNpbmcgZG9jdW1lbnQuZ2V0RWxlbWVudEJ5SWQoJ215Rm9ybScpLiBPbmNlIHdlIGhhdmUgdGhlIHJlZmVyZW5jZSwgd2UgY2FuIGNhbGwgdGhlIHN1Ym1pdCgpIG1ldGhvZCBvbiB0aGUgZm9ybSBlbGVtZW50IHRvIHRyaWdnZXIgdGhlIHN1Ym1pc3Npb24uCgpCeSBjYWxsaW5nIGZvcm0uc3VibWl0KCksIHRoZSBicm93c2VyIHdpbGwgaW5pdGlhdGUgdGhlIGZvcm0gc3VibWlzc2lvbiBwcm9jZXNzLCBzZW5kaW5nIHRoZSBmb3JtIGRhdGEgdG8gdGhlIHNwZWNpZmllZCBhY3Rpb24gVVJMIHVzaW5nIHRoZSBzcGVjaWZpZWQgSFRUUCBtZXRob2QgKG1ldGhvZCBhdHRyaWJ1dGUpLiBUaGUgYmVoYXZpb3IgYWZ0ZXIgZm9ybSBzdWJtaXNzaW9uIHdpbGwgZGVwZW5kIG9uIHRoZSBzZXJ2ZXItc2lkZSBpbXBsZW1lbnRhdGlvbiBhbmQgdGhlIGNvbnRlbnQgb2YgdGhlIGFjdGlvbiBVUkwuCgpZb3UgY2FuIHRyaWdnZXIgdGhlIGZvcm0gc3VibWlzc2lvbiB1c2luZyB2YXJpb3VzIGV2ZW50cyBvciBjb25kaXRpb25zIGluIHlvdXIgSmF2YVNjcmlwdCBjb2RlLCBzdWNoIGFzIGNsaWNraW5nIGEgYnV0dG9uLCB2YWxpZGF0aW5nIGZvcm0gZGF0YSwgb3IgcHJvZ3JhbW1hdGljYWxseSBiYXNlZCBvbiBjZXJ0YWluIGNvbmRpdGlvbnMuCgpRNDEuIFdoYXQgZG8geW91IG1lYW4gYnkgTlVMTCBpbiBKYXZhU2NyaXB0PwoKCgoKSW4gSmF2YVNjcmlwdCwgbnVsbCBpcyBhIHNwZWNpYWwgdmFsdWUgdGhhdCByZXByZXNlbnRzIHRoZSBpbnRlbnRpb25hbCBhYnNlbmNlIG9mIGFueSBvYmplY3QgdmFsdWUuIEl0IGlzIGEgcHJpbWl0aXZlIHZhbHVlIHRoYXQgaW5kaWNhdGVzIHRoZSBhYnNlbmNlIG9mIGEgdmFsdWUgb3IgdGhlIGFic2VuY2Ugb2YgYW4gb2JqZWN0IHJlZmVyZW5jZS4KCkhlcmUgYXJlIHNvbWUga2V5IHBvaW50cyBhYm91dCBudWxsIGluIEphdmFTY3JpcHQ6CgpudWxsIGlzIGEgZGF0YSB0eXBlOiBudWxsIGlzIGEgcHJpbWl0aXZlIGRhdGEgdHlwZSBpbiBKYXZhU2NyaXB0LCBzcGVjaWZpY2FsbHkgcmVwcmVzZW50aW5nIHRoZSBhYnNlbmNlIG9mIGFuIG9iamVjdCB2YWx1ZS4KCkl0IGlzIGZhbHN5OiBudWxsIGlzIGNvbnNpZGVyZWQgYSBmYWxzeSB2YWx1ZSBpbiBKYXZhU2NyaXB0LiBXaGVuIGV2YWx1YXRlZCBpbiBhIEJvb2xlYW4gY29udGV4dCAoZS5nLiwgaW4gYW4gaWYgc3RhdGVtZW50IG9yIGFzIGEgY29uZGl0aW9uKSwgaXQgaXMgdHJlYXRlZCBhcyBmYWxzZS4KCkl0IGlzIG5vdCBhbiBvYmplY3Q6IERlc3BpdGUgYmVpbmcgYSBmYWxzeSB2YWx1ZSwgbnVsbCBpcyBub3QgYW4gb2JqZWN0LiBJdCBpcyBhIHByaW1pdGl2ZSB2YWx1ZS4gSXQgaXMgZGlzdGluY3QgZnJvbSB0aGUgdW5kZWZpbmVkIHZhbHVlLCB3aGljaCByZXByZXNlbnRzIGFuIHVuaW5pdGlhbGl6ZWQgb3IgbWlzc2luZyB2YWx1ZS4KClR5cGUgb2YgbnVsbCBpcyAib2JqZWN0IjogSW50ZXJlc3RpbmdseSwgdGhlIHR5cGUgb2Ygb3BlcmF0b3IgaW4gSmF2YVNjcmlwdCByZXR1cm5zICJvYmplY3QiIHdoZW4gYXBwbGllZCB0byBudWxsLiBUaGlzIGlzIGEgaGlzdG9yaWNhbCBxdWlyayBpbiBKYXZhU2NyaXB0IGFuZCBub3QgYSB0cnVlIHJlZmxlY3Rpb24gb2YgbnVsbCBiZWluZyBhbiBvYmplY3QuIEl0IGlzIGNvbnNpZGVyZWQgYSBtaXN0YWtlIGluIHRoZSBsYW5ndWFnZSdzIGRlc2lnbi4KCkhlcmUncyBhbiBleGFtcGxlIGlsbHVzdHJhdGluZyB0aGUgdXNlIG9mIG51bGw6CgpmdW5jdGlvbiBnZXRWb3dlbHMoc3RyKSB7CiAgY29uc3QgbSA9IHN0ci5tYXRjaCgvW2FlaW91XS9naSk7CiAgaWYgKG0gPT09IG51bGwpIHsKICAgIHJldHVybiAwOwogIH0KICByZXR1cm4gbS5sZW5ndGg7Cn0KCmNvbnNvbGUubG9nKGdldFZvd2Vscygnc2t5JykpOwovLyBleHBlY3RlZCBvdXRwdXQ6IDAKCgoKUTQyLiBXaGF0IGlzIHRoZSBzdHJpY3QgbW9kZSBpbiBKYXZhU2NyaXB0IGFuZCBob3cgY2FuIGl0IGJlIGVuYWJsZWQ/CgpTdHJpY3QgbW9kZSBpcyBhIGZlYXR1cmUgaW50cm9kdWNlZCBpbiBFQ01BU2NyaXB0IDUgKEVTNSkgdGhhdCBlbmFibGVzIGEgc3RyaWN0ZXIgc2V0IG9mIHJ1bGVzIGZvciBKYXZhU2NyaXB0IGNvZGUuIEl0IGhlbHBzIGluIGF2b2lkaW5nIGNvbW1vbiBtaXN0YWtlcywgcHJvbW90ZXMgZ29vZCBjb2RpbmcgcHJhY3RpY2VzLCBhbmQgbWFrZXMgSmF2YVNjcmlwdCBjb2RlIG1vcmUgcm9idXN0LgoKVG8gZW5hYmxlIHN0cmljdCBtb2RlIGluIEphdmFTY3JpcHQsIHlvdSBuZWVkIHRvIGFkZCB0aGUgInVzZSBzdHJpY3QiOyBkaXJlY3RpdmUgYXQgdGhlIGJlZ2lubmluZyBvZiBhIHNjcmlwdCBvciBhIGZ1bmN0aW9uLiBUaGVyZSBhcmUgdHdvIHdheXMgdG8gZW5hYmxlIHN0cmljdCBtb2RlOgoKU2NyaXB0LXdpZGUgc3RyaWN0IG1vZGU6IEFkZCB0aGUgInVzZSBzdHJpY3QiOyBkaXJlY3RpdmUgYXQgdGhlIGJlZ2lubmluZyBvZiBhIHNjcmlwdCBmaWxlLiBXaGVuIGFkZGVkIGF0IHRoZSB0b3Agb2YgYSBKYXZhU2NyaXB0IGZpbGUsIHN0cmljdCBtb2RlIGFwcGxpZXMgdG8gdGhlIGVudGlyZSBmaWxlLgoKInVzZSBzdHJpY3QiOwoKLy8gUmVzdCBvZiB5b3VyIEphdmFTY3JpcHQgY29kZQoKRnVuY3Rpb24tbGV2ZWwgc3RyaWN0IG1vZGU6IEFkZCB0aGUgInVzZSBzdHJpY3QiOyBkaXJlY3RpdmUgYXQgdGhlIGJlZ2lubmluZyBvZiBhIGZ1bmN0aW9uLiBXaGVuIGFkZGVkIGluc2lkZSBhIGZ1bmN0aW9uLCBzdHJpY3QgbW9kZSBhcHBsaWVzIG9ubHkgdG8gdGhhdCBzcGVjaWZpYyBmdW5jdGlvbi4KCmZ1bmN0aW9uIG15RnVuY3Rpb24oKSB7CiJ1c2Ugc3RyaWN0IjsKCi8vIEZ1bmN0aW9uLWxldmVsIHN0cmljdCBtb2RlIGFwcGxpZXMgb25seSB0byB0aGlzIGZ1bmN0aW9uCi8vIFJlc3Qgb2YgeW91ciBKYXZhU2NyaXB0IGNvZGUKfQoKCkVuYWJsaW5nIHN0cmljdCBtb2RlIGludHJvZHVjZXMgdGhlIGZvbGxvd2luZyBjaGFuZ2VzIGFuZCByZXN0cmljdGlvbnMgdG8gdGhlIEphdmFTY3JpcHQgY29kZToKClZhcmlhYmxlcyBtdXN0IGJlIGRlY2xhcmVkIGJlZm9yZSB1c2UuIEFzc2lnbmluZyBhIHZhbHVlIHRvIGFuIHVuZGVjbGFyZWQgdmFyaWFibGUgd2lsbCB0aHJvdyBhbiBlcnJvci4KQXNzaWduaW5nIGEgdmFsdWUgdG8gYSByZWFkLW9ubHkgZ2xvYmFsIHZhcmlhYmxlIG9yIGEgbm9uLXdyaXRhYmxlIHByb3BlcnR5IHRocm93cyBhbiBlcnJvci4KRGVsZXRpbmcgdmFyaWFibGVzLCBmdW5jdGlvbnMsIG9yIGZ1bmN0aW9uIGFyZ3VtZW50cyBpcyBub3QgYWxsb3dlZC4KVGhlIHRoaXMgdmFsdWUgaW4gZnVuY3Rpb25zIGlzIHVuZGVmaW5lZCBpbiBzdHJpY3QgbW9kZSB3aGVuIHRoZSBmdW5jdGlvbiBpcyBjYWxsZWQgd2l0aG91dCBhbiBleHBsaWNpdCB0aGlzIHZhbHVlLgpPY3RhbCBsaXRlcmFscyAoZS5nLiwgMDEyMykgYXJlIG5vdCBhbGxvd2VkLgpEdXBsaWNhdGUgcGFyYW1ldGVyIG5hbWVzIGluIGZ1bmN0aW9uIGRlY2xhcmF0aW9ucyB0aHJvdyBhbiBlcnJvci4KVGhlIGV2YWwoKSBmdW5jdGlvbiBjcmVhdGVzIGEgbmV3IG91dGVyIHNjb3BlIGFuZCBkb2VzIG5vdCBoYXZlIGFjY2VzcyB0byB0aGUgY29udGFpbmluZyBzY29wZSdzIHZhcmlhYmxlcy4KU2V2ZXJhbCBwb3RlbnRpYWwgc291cmNlcyBvZiBlcnJvcnMsIGxpa2UgdXNpbmcgcmVzZXJ2ZWQga2V5d29yZHMgYXMgdmFyaWFibGUgbmFtZXMsIGFyZSByZXN0cmljdGVkLgoKQnkgZW5hYmxpbmcgc3RyaWN0IG1vZGUsIHlvdSBjYW4gY2F0Y2ggYW5kIHByZXZlbnQgY2VydGFpbiB0eXBlcyBvZiBlcnJvcnMoIHR5cGUgY29ycmVjdGlvbikgYW5kIGVuZm9yY2UgYmV0dGVyIGNvZGluZyBwcmFjdGljZXMuIEl0IGlzIHJlY29tbWVuZGVkIHRvIHVzZSBzdHJpY3QgbW9kZSBpbiBhbGwgSmF2YVNjcmlwdCBjb2RlIHRvIGltcHJvdmUgY29kZSBxdWFsaXR5IGFuZCBtYWludGFpbmFiaWxpdHkuCgoKCgpRNDMuIFdoYXQgaXMgdGhlIGRpZmZlcmVuY2UgYmV0d2VlbiAuY2FsbCgpIGFuZCAuYXBwbHkoKT8KCkJvdGggdGhlIC5jYWxsKCkgYW5kIC5hcHBseSgpIG1ldGhvZHMgaW4gSmF2YVNjcmlwdCBhcmUgdXNlZCB0byBpbnZva2UgYSBmdW5jdGlvbiB3aXRoIGEgc3BlY2lmaWVkIHRoaXMgdmFsdWUgYW5kIGFyZ3VtZW50cy4gVGhleSBhbGxvdyB5b3UgdG8gZXhlY3V0ZSBhIGZ1bmN0aW9uIGluIHRoZSBjb250ZXh0IG9mIGEgcGFydGljdWxhciBvYmplY3QgYW5kIHBhc3MgYXJndW1lbnRzIHRvIHRoZSBmdW5jdGlvbi4gVGhlIG1haW4gZGlmZmVyZW5jZSBiZXR3ZWVuIC5jYWxsKCkgYW5kIC5hcHBseSgpIGxpZXMgaW4gaG93IHRoZSBhcmd1bWVudHMgYXJlIHBhc3NlZCB0byB0aGUgZnVuY3Rpb24uCgpUaGUgLmNhbGwoKSBtZXRob2Q6CgpBY2NlcHRzIHRoZSB0aGlzIHZhbHVlIGFzIHRoZSBmaXJzdCBhcmd1bWVudCwgZm9sbG93ZWQgYnkgdGhlIGZ1bmN0aW9uIGFyZ3VtZW50cyBhcyBpbmRpdmlkdWFsIGFyZ3VtZW50cy4KVGhlIGFyZ3VtZW50cyBhcmUgcGFzc2VkIGV4cGxpY2l0bHkgYW5kIHNlcGFyYXRlZCBieSBjb21tYXMuCgpFeGFtcGxlIHVzaW5nIC5jYWxsKCk6CgpmdW5jdGlvbiBncmVldChuYW1lKSB7CmNvbnNvbGUubG9nKGBIZWxsbywgJHtuYW1lfSFgKTsKfQoKZ3JlZXQuY2FsbChudWxsLCAnSm9obicpOyAvLyBPdXRwdXQ6IEhlbGxvLCBKb2huIQoKClRoZSAuYXBwbHkoKSBtZXRob2Q6CgpBY2NlcHRzIHRoZSB0aGlzIHZhbHVlIGFzIHRoZSBmaXJzdCBhcmd1bWVudCwgZm9sbG93ZWQgYnkgYW4gYXJyYXktbGlrZSBvciBpdGVyYWJsZSBvYmplY3QgY29udGFpbmluZyB0aGUgZnVuY3Rpb24gYXJndW1lbnRzLgpUaGUgYXJndW1lbnRzIGFyZSBwYXNzZWQgYXMgYW4gYXJyYXkgb3IgYW4gYXJyYXktbGlrZSBvYmplY3QuCgpFeGFtcGxlIHVzaW5nIC5hcHBseSgpOgoKZnVuY3Rpb24gZ3JlZXQobmFtZSkgewpjb25zb2xlLmxvZyhgSGVsbG8sICR7bmFtZX0hYCk7Cn0KCmdyZWV0LmFwcGx5KG51bGwsIFsnSm9obiddKTsgLy8gT3V0cHV0OiBIZWxsbywgSm9obiEKCgpFc3NlbnRpYWxseSwgdGhlIGtleSBkaWZmZXJlbmNlIGJldHdlZW4gLmNhbGwoKSBhbmQgLmFwcGx5KCkgaXMgdGhlIHdheSBpbiB3aGljaCB0aGUgYXJndW1lbnRzIGFyZSBwYXNzZWQuIElmIHlvdSBoYXZlIHRoZSBmdW5jdGlvbiBhcmd1bWVudHMgYXMgaW5kaXZpZHVhbCB2YWx1ZXMsIHlvdSBjYW4gdXNlIC5jYWxsKCkgYW5kIHBhc3MgdGhlbSBleHBsaWNpdGx5LiBJZiB5b3UgaGF2ZSB0aGUgZnVuY3Rpb24gYXJndW1lbnRzIGluIGFuIGFycmF5IG9yIGFycmF5LWxpa2Ugb2JqZWN0LCB5b3UgY2FuIHVzZSAuYXBwbHkoKSBhbmQgcGFzcyB0aGUgYXJyYXktbGlrZSBvYmplY3QgYXMgYSBzaW5nbGUgYXJndW1lbnQuIEJvdGggbWV0aG9kcyBhbGxvdyB5b3UgdG8gY29udHJvbCB0aGlzIHZhbHVlIHdpdGhpbiB0aGUgZnVuY3Rpb24gZXhlY3V0aW9uIGNvbnRleHQsIHdoaWNoIGNhbiBiZSBoZWxwZnVsIHdoZW4gd29ya2luZyB3aXRoIG9iamVjdC1vcmllbnRlZCBwcm9ncmFtbWluZyBvciBib3Jyb3dpbmcgbWV0aG9kcyBmcm9tIG9uZSBvYmplY3QgdG8gdXNlIHdpdGggYW5vdGhlci4KClE0NC4gV2hhdCBpcyB0aGUgbWV0aG9kIG9mIHJlYWRpbmcgYW5kIHdyaXRpbmcgaW4gSmF2YVNjcmlwdAoKRmlsZXMgY2FuIGJlIHJlYWQgYW5kIHdyaXR0ZW4gYnkgdXNpbmcgamF2YSBzY3JpcHQgZnVuY3Rpb25zIOKAkyBmb3BlbigpLGZyZWFkKCkgYW5kIGZ3cml0ZSgpLiBUaGUgZnVuY3Rpb24gZm9wZW4oKSB0YWtlcyB0d28gcGFyYW1ldGVycyDigJMgMS4gUGF0aCBhbmQgMi4gTW9kZSAoMCBmb3IgcmVhZGluZyBhbmQgMyBmb3Igd3JpdGluZykuIFRoZSBmb3BlbigpIGZ1bmN0aW9uIHJldHVybnMgLTEsIGlmIHRoZSBmaWxlIGlzIHN1Y2Nlc3NmdWxseSBvcGVuZWQuCgpFeGFtcGxlOgoKZmlsZT1mb3BlbihnZXRTY3JpcHRQYXRoKCksMCk7CgpUaGUgZnVuY3Rpb24gZnJlYWQoKSBpcyB1c2VkIGZvciByZWFkaW5nIHRoZSBzb3VyY2UgZmlsZSBjb250ZW50LgoKRXhhbXBsZToKCnN0ciA9IGZyZWFkKGZpbGUsZmxlbmd0aChmaWxlKSA7CgpUaGUgZnVuY3Rpb24gZndyaXRlKCkgaXMgdXNlZCB0byB3cml0ZSB0aGUgY29udGVudHMgdG8gdGhlIHNvdXJjZSBmaWxlLgoKRXhhbXBsZToKCmZpbGUgPSBmb3BlbigiYzpcXE15RmlsZS50eHQiLCAzKTsvLyBvcGVucyB0aGUgc291cmNlIGZpbGUgZm9yIHdyaXRpbmcKCmZ3cml0ZShmaWxlLCBzdHIpOy8vIHN0ciBpcyB0aGUgY29udGVudCB0aGF0IGlzIHRvIGJlIHdyaXR0ZW4gaW50byBhIHNlcGFyYXRlIGZpbGUuCgpRNDUuIEhvdyB0byBwcmludCBhIHdlYnBhZ2UgdXNpbmcgamF2YXNjcmlwdD8KClRvIHByaW50IGEgd2VicGFnZSwgd2UgdXNlIHRoZSBwcmludCgpIG1ldGhvZC4gVGhlIHByaW50IG1ldGhvZCBvcGVucyBhIGRpYWxvZyBib3ggdGhhdCB5b3UgY2FuIGNsaWNrIGFuZCBwcmludCB0aGUgcGFnZS4KClRoZSBmb2xsb3dpbmcgY29kZSBjYW4gYmUgdXNlZOKelgoKPCFET0NUWVBFIGh0bWw+CjxodG1sPgogICA8Ym9keT4KICAgICAgPGJ1dHRvbiBvbmNsaWNrPSJkaXNwbGF5KCkiPkNsaWNrIHRvIFByaW50PC9idXR0b24+CiAgICAgIDxzY3JpcHQ+CiAgICAgICAgIGZ1bmN0aW9uIGRpc3BsYXkoKSB7CiAgICAgICAgICAgIHdpbmRvdy5wcmludCgpOwogICAgICAgICB9CiAgICAgIDwvc2NyaXB0PgogICA8L2JvZHk+CjwvaHRtbD4KCgpRNDYuIFdoYXQgaXMgdGhlIGRhdGUgb2JqZWN0IGluIEphdmFTY3JpcHQ/CgpUaGXCoERhdGUgb2JqZWN0wqBpcyBhbiBpbmJ1aWx0IGRhdGF0eXBlIG9mIEphdmFTY3JpcHQgbGFuZ3VhZ2UuIEl0IGlzIHVzZWQgdG8gd29yayB3aXRowqBkYXRlc8KgYW5kwqB0aW1lcy4gVGhlIERhdGUgb2JqZWN0IGlzIGNyZWF0ZWQgYnkgdXNpbmfCoG5ld8Kga2V5d29yZCwgaS5lLsKgbmV3IERhdGUoKS4gVGhlIERhdGUgb2JqZWN0IGNhbiBiZSB1c2VkIGRhdGUgYW5kIHRpbWUgaW4gdGVybXMgb2bCoG1pbGxpc2Vjb25kwqBwcmVjaXNpb24gd2l0aGluIDEwMCBtaWxsaW9uIGRheXMgYmVmb3JlIG9yIGFmdGVyIDEvMS8xOTcwLgoKVGhlcmUgYXJlIGZvdXIgZGlmZmVyZW50IHdheXMgdG8gZGVjbGFyZSBhIGRhdGUsIHRoZSBiYXNpYyB0aGluZyBpcyB0aGF0IHRoZSBkYXRlLW9iamVjdHMgYXJlIGNyZWF0ZWQgYnkgdGhlwqBuZXcgRGF0ZSgpwqBvcGVyYXRvcgoKbmV3IERhdGUoKSDinpZUaGUgZGF0ZSBjb25zdHJ1Y3RvciBjcmVhdGVzIGEgRGF0ZSBvYmplY3Qgd2hpY2ggc2V0cyB0aGUgY3VycmVudCBkYXRlIGFuZCB0aW1lIGRlcGVuZGluZyBvbiB0aGUgYnJvd3NlcuKAmXMgdGltZSB6b25lLiBJdCBkb2VzIG5vdCBhY2NlcHQgYW55IHZhbHVlLgoKbmV3IERhdGUobWlsbGlzZWNvbmRzKeKeliBUaGlzIG1ldGhvZCBhY2NlcHRzIHNpbmdsZSBwYXJhbWV0ZXIgbWlsbGlzZWNvbmRzIHdoaWNoIGluZGljYXRlcyBhbnkgbnVtZXJpYyB2YWx1ZS4gVGhpcyBhcmd1bWVudCBpcyB0YWtlbiBhcyB0aGUgaW50ZXJuYWwgbnVtZXJpYyByZXByZXNlbnRhdGlvbiBvZiB0aGUgZGF0ZSBpbiBtaWxsaXNlY29uZHMuCgpuZXcgRGF0ZShkYXRhU3RyaW5nKSDinpZUaGlzIG1ldGhvZCBhY2NlcHRzIGEgc2luZ2xlIHBhcmFtZXRlciBkYXRhU3RyaW5nIHdoaWNoIGluZGljYXRlcyBhbnkgc3RyaW5nIHZhbHVlLiBJdCBpcyBhIHN0cmluZyByZXByZXNlbnRhdGlvbiBvZiBhIGRhdGUgYW5kIHRoZSByZXR1cm4gc3RhdGVtZW50IHJldHVybnMgdGhlIGRhdGFzdHJpbmcgd2l0aCB0aGUgZGF5CgpuZXcgRGF0ZSh5ZWFyLCBtb250aCwgZGF0ZSwgaG91ciwgbWludXRlLCBzZWNvbmQsIG1pbGxpc2Vjb25kKeKelgoKUGFyYW1ldGVyczoKClRoaXMgbWV0aG9kIGFjY2VwdHMgc2V2ZW4gcGFyYW1ldGVycyBhcyBtZW50aW9uZWQgYWJvdmUgYW5kIGRlc2NyaWJlZCBiZWxvdzoKCnllYXI6wqBJbnRlZ2VyIHZhbHVlIHdoaWNoIHJlcHJlc2VudHMgdGhlIHllYXIuIFRoaXMgc2hvdWxkIGFsd2F5cyBzcGVjaWZ5IHRoZSB5ZWFyIGluIGZ1bGwsIGkuZS4gdXNlIDIwMTgsIGluc3RlYWQgb2YgdXNlIDE4LgoKbW9udGg6wqBJbnRlZ2VywqB2YWx1ZSB3aGljaCByZXByZXNlbnRzIHRoZSBtb250aC4gVGhlIGludGVnZXIgdmFsdWVzIHN0YXJ0aW5nIGZyb20gMCBmb3IgSmFudWFyeSB0byAxMSBmb3IgRGVjZW1iZXIuCgpkYXRlOsKgSW50ZWdlcsKgdmFsdWUgd2hpY2ggcmVwcmVzZW50cyB0aGUgZGF0ZS4KCmhvdXI6wqBJbnRlZ2VywqB2YWx1ZSB3aGljaCByZXByZXNlbnRzIHRoZSBob3VyIG9uIHRoZSAyNC1ob3VycyBzY2FsZS4KCm1pbnV0ZTrCoEludGVnZXLCoHZhbHVlIHdoaWNoIHJlcHJlc2VudHMgdGhlIG1pbnV0ZS4KCnNlY29uZDrCoEludGVnZXLCoHZhbHVlIHdoaWNoIHJlcHJlc2VudHMgdGhlIHNlY29uZC4KCm1pbGxpc2Vjb25kOsKgSW50ZWdlcsKgdmFsdWUgd2hpY2ggcmVwcmVzZW50cyB0aGUgbWlsbGlzZWNvbmQuCgpRNDcuIEhvdyB0byBoYW5kbGUgRXhjZXB0aW9uIGluIEphdmEgU2NyaXB0PwoKSW4gSmF2YVNjcmlwdCwgeW91IGNhbiBoYW5kbGUgZXhjZXB0aW9ucywgYWxzbyBrbm93biBhcyBlcnJvcnMsIHVzaW5nIHRyeS1jYXRjaCBibG9ja3MuIFRoZSB0cnktY2F0Y2ggYmxvY2sgYWxsb3dzIHlvdSB0byBlbmNsb3NlIGEgc2VjdGlvbiBvZiBjb2RlIHRoYXQgbWF5IHRocm93IGFuIGV4Y2VwdGlvbiBhbmQgc3BlY2lmeSBob3cgdG8gaGFuZGxlIHRoYXQgZXhjZXB0aW9uIGlmIGl0IG9jY3Vycy4KCkhlcmUncyB0aGUgYmFzaWMgc3ludGF4IG9mIGEgdHJ5LWNhdGNoIGJsb2NrOgoKdHJ5IHsKLy8gQ29kZSB0aGF0IG1heSB0aHJvdyBhbiBleGNlcHRpb24KfSBjYXRjaCAoZXJyb3IpIHsKLy8gQ29kZSB0byBoYW5kbGUgdGhlIGV4Y2VwdGlvbgp9CgoKV2l0aGluIHRoZSB0cnkgYmxvY2ssIHlvdSBwbGFjZSB0aGUgY29kZSB0aGF0IHlvdSB3YW50IHRvIG1vbml0b3IgZm9yIGV4Y2VwdGlvbnMuIElmIGFuIGV4Y2VwdGlvbiBvY2N1cnMgd2l0aGluIHRoZSB0cnkgYmxvY2ssIHRoZSBjYXRjaCBibG9jayBpcyBleGVjdXRlZC4gVGhlIGNhdGNoIGJsb2NrIHJlY2VpdmVzIHRoZSB0aHJvd24gZXhjZXB0aW9uIGFzIGFuIGFyZ3VtZW50LCB3aGljaCB5b3UgY2FuIHRoZW4gdXNlIHRvIGhhbmRsZSB0aGUgZXhjZXB0aW9uIGFwcHJvcHJpYXRlbHkuCgpIZXJlJ3MgYW4gZXhhbXBsZSB0aGF0IGRlbW9uc3RyYXRlcyB0aGUgdXNhZ2Ugb2YgdHJ5LWNhdGNoIGJsb2NrOgoKdHJ5IHsKLy8gQ29kZSB0aGF0IG1heSB0aHJvdyBhbiBleGNlcHRpb24KY29uc3QgcmVzdWx0ID0gMTAgLyAwOyAvLyBEaXZpc2lvbiBieSB6ZXJvCmNvbnNvbGUubG9nKHJlc3VsdCk7Cn0gY2F0Y2ggKGVycm9yKSB7Ci8vIENvZGUgdG8gaGFuZGxlIHRoZSBleGNlcHRpb24KY29uc29sZS5sb2coIkFuIGVycm9yIG9jY3VycmVkOiIsIGVycm9yKTsKfQoKCkluIHRoaXMgZXhhbXBsZSwgdGhlIGNvZGUgd2l0aGluIHRoZSB0cnkgYmxvY2sgYXR0ZW1wdHMgdG8gcGVyZm9ybSBhIGRpdmlzaW9uIGJ5IHplcm8sIHdoaWNoIHdpbGwgdGhyb3cgYW4gZXhjZXB0aW9uLiBTaW5jZSBkaXZpZGluZyBieSB6ZXJvIGlzIGFuIGludmFsaWQgb3BlcmF0aW9uLCB0aGUgY2F0Y2ggYmxvY2sgaXMgZXhlY3V0ZWQuIFRoZSBjYXRjaCBibG9jayByZWNlaXZlcyB0aGUgdGhyb3duIGV4Y2VwdGlvbiBhcyB0aGUgZXJyb3IgcGFyYW1ldGVyLCBhbmQgdGhlIGVycm9yIG1lc3NhZ2UgYWxvbmcgd2l0aCB0aGUgZXhjZXB0aW9uIGlzIGxvZ2dlZCB0byB0aGUgY29uc29sZS4KCkJ5IHVzaW5nIHRyeS1jYXRjaCBibG9ja3MsIHlvdSBjYW4gZ3JhY2VmdWxseSBoYW5kbGUgZXhjZXB0aW9ucywgcHJldmVudCB0aGVtIGZyb20gY3Jhc2hpbmcgeW91ciBwcm9ncmFtLCBhbmQgcHJvdmlkZSBtZWFuaW5nZnVsIGVycm9yIG1lc3NhZ2VzIG9yIGFsdGVybmF0aXZlIHBhdGhzIG9mIGV4ZWN1dGlvbi4gQWRkaXRpb25hbGx5LCB5b3UgY2FuIG5lc3QgbXVsdGlwbGUgdHJ5LWNhdGNoIGJsb2NrcyB0byBoYW5kbGUgZGlmZmVyZW50IHR5cGVzIG9mIGV4Y2VwdGlvbnMgb3IgcGVyZm9ybSBtb3JlIGdyYW51bGFyIGVycm9yIGhhbmRsaW5nLgoKUTQ4LiBXaGF0IGlzIHRoZSBwdXJwb3NlIG9mIHRoZSBvbkVycm9yIGV2ZW50IGhhbmRsZXIgaW4gSmF2YVNjcmlwdD8KClRoZSBvbkVycm9yIGV2ZW50IGhhbmRsZXIgaW4gSmF2YVNjcmlwdCBpcyB1c2VkIHRvIGhhbmRsZSBlcnJvcnMgdGhhdCBvY2N1ciBkdXJpbmcgdGhlIGV4ZWN1dGlvbiBvZiBhIHNjcmlwdCBvciB0aGUgbG9hZGluZyBvZiBleHRlcm5hbCByZXNvdXJjZXMsIHN1Y2ggYXMgaW1hZ2VzLCBzY3JpcHRzLCBvciBzdHlsZXNoZWV0cy4gSXQgYWxsb3dzIHlvdSB0byBkZWZpbmUgYSBmdW5jdGlvbiB0aGF0IHdpbGwgYmUgZXhlY3V0ZWQgd2hlbiBhbiBlcnJvciBvY2N1cnMuCgpUaGUgb25FcnJvciBldmVudCBoYW5kbGVyIGlzIGNvbW1vbmx5IHVzZWQgaW4gc2NlbmFyaW9zIGxpa2U6CgoxLiBFcnJvciBoYW5kbGluZyBmb3Igc2NyaXB0czoKCjxzY3JpcHQ+CmZ1bmN0aW9uIGhhbmRsZVNjcmlwdEVycm9yKCkgewpjb25zb2xlLmxvZygiQW4gZXJyb3Igb2NjdXJyZWQgd2hpbGUgbG9hZGluZyB0aGUgc2NyaXB0LiIpOwp9Cjwvc2NyaXB0Pgo8c2NyaXB0IHNyYz0ic2NyaXB0LmpzIiBvbkVycm9yPSJoYW5kbGVTY3JpcHRFcnJvcigpIj48L3NjcmlwdD4KCgoyLiBFcnJvciBoYW5kbGluZyBmb3IgaW1hZ2VzOgoKPGltZyBzcmM9ImltYWdlLnBuZyIgb25FcnJvcj0iaGFuZGxlSW1hZ2VFcnJvcigpIj4KCgpUaGUgb25FcnJvciBldmVudCBoYW5kbGVyIHByb3ZpZGVzIGEgd2F5IHRvIGNhdGNoIGFuZCBoYW5kbGUgZXJyb3JzIGluIGEgY29udHJvbGxlZCBtYW5uZXIuIEJ5IGRlZmluaW5nIGFuIGVycm9yIGhhbmRsaW5nIGZ1bmN0aW9uIGFuZCBhc3NpZ25pbmcgaXQgdG8gdGhlIG9uRXJyb3IgZXZlbnQsIHlvdSBjYW4gcGVyZm9ybSBhY3Rpb25zIHN1Y2ggYXMgbG9nZ2luZyB0aGUgZXJyb3IsIGRpc3BsYXlpbmcgYSB1c2VyLWZyaWVuZGx5IG1lc3NhZ2UsIG9yIHRha2luZyBjb3JyZWN0aXZlIG1lYXN1cmVzLgoKSXQncyBpbXBvcnRhbnQgdG8gbm90ZSB0aGF0IHRoZSBvbkVycm9yIGV2ZW50IGhhbmRsZXIgaXMgbm90IGxpbWl0ZWQgdG8gc2NyaXB0IGFuZCBpbWFnZSBlbGVtZW50cy4gSXQgY2FuIGFsc28gYmUgdXNlZCB3aXRoIG90aGVyIEhUTUwgZWxlbWVudHMgbGlrZSA8YXVkaW8+LCA8dmlkZW8+LCA8bGluaz4sIGFuZCA8c3R5bGU+LCBhcyB3ZWxsIGFzIHZhcmlvdXMgSmF2YVNjcmlwdCBBUElzIHRoYXQgc3VwcG9ydCBlcnJvciBldmVudHMsIHN1Y2ggYXMgWE1MSHR0cFJlcXVlc3Qgb3IgV2ViU29ja2V0LgoKQWRkaXRpb25hbGx5LCBtb2Rlcm4gSmF2YVNjcmlwdCBwcm92aWRlcyBtb3JlIHJvYnVzdCBlcnJvci1oYW5kbGluZyBtZWNoYW5pc21zLCBzdWNoIGFzIHRyeS1jYXRjaCBibG9ja3MgYW5kIHRoZSB3aW5kb3cub25lcnJvciBldmVudCBoYW5kbGVyLCB3aGljaCBvZmZlciBncmVhdGVyIGNvbnRyb2wgYW5kIGZsZXhpYmlsaXR5IGluIGhhbmRsaW5nIGVycm9ycyB3aXRoaW4gSmF2YVNjcmlwdCBjb2RlLgoKUTQ5LiBXaGF0IHdvdWxkIGJlIHRoZSByZXN1bHQgb2YgMysyK+KAnTfigLM/CgpJbiBKYXZhU2NyaXB0LCB0aGUgKyBvcGVyYXRvciBpcyB1c2VkIGZvciBib3RoIGFkZGl0aW9uIGFuZCBzdHJpbmcgY29uY2F0ZW5hdGlvbi4gV2hlbiB0aGUgKyBvcGVyYXRvciBpcyB1c2VkIHdpdGggbnVtYmVycywgaXQgcGVyZm9ybXMgYWRkaXRpb24uIEhvd2V2ZXIsIHdoZW4gb25lIG9yIGJvdGggb2YgdGhlIG9wZXJhbmRzIGFyZSBzdHJpbmdzLCB0aGUgKyBvcGVyYXRvciBjb25jYXRlbmF0ZXMgdGhlIHN0cmluZ3MgdG9nZXRoZXIuCgpJbiB0aGUgZXhwcmVzc2lvbiAzICsgMiArICI3IiwgdGhlIGZpcnN0IHR3byBvcGVyYW5kcyBhcmUgbnVtYmVycyAoMyBhbmQgMiksIGFuZCB0aGUgbGFzdCBvcGVyYW5kIGlzIGEgc3RyaW5nICgiNyIpLgoKSGVyZSdzIHRoZSBzdGVwLWJ5LXN0ZXAgZXZhbHVhdGlvbiBvZiB0aGUgZXhwcmVzc2lvbjoKCjMgKyAyIGV2YWx1YXRlcyB0byA1IChhZGRpdGlvbiBvZiBudW1iZXJzKS4KNSArICI3IiBldmFsdWF0ZXMgdG8gIjU3IiAoY29uY2F0ZW5hdGlvbiBvZiB0aGUgbnVtYmVyIDUgd2l0aCB0aGUgc3RyaW5nICI3IikuCgpUaGVyZWZvcmUsIHRoZSByZXN1bHQgb2YgMyArIDIgKyAiNyIgaXMgIjU3Ii4gVGhlIG51bWVyaWMgYWRkaXRpb24gb2YgMyArIDIgcmVzdWx0cyBpbiA1LCBhbmQgdGhlbiB0aGF0IHJlc3VsdCBpcyBjb25jYXRlbmF0ZWQgd2l0aCB0aGUgc3RyaW5nICI3IiwgcHJvZHVjaW5nIHRoZSBzdHJpbmcgIjU3Ii4KClE1MC4gSXMgamF2YXNjcmlwdCBjYXNlLXNlbnNpdGl2ZT8KClllcywgSmF2YVNjcmlwdCBpcyBjYXNlLXNlbnNpdGl2ZS4gVGhpcyBtZWFucyB0aGF0IEphdmFTY3JpcHQgdHJlYXRzIHVwcGVyY2FzZSBhbmQgbG93ZXJjYXNlIGxldHRlcnMgYXMgZGlzdGluY3QgYW5kIHNlcGFyYXRlIGVudGl0aWVzLiBUaGUgY2FzZSBvZiBsZXR0ZXJzIGluIHZhcmlhYmxlIG5hbWVzLCBmdW5jdGlvbiBuYW1lcywgb2JqZWN0IHByb3BlcnRpZXMsIGFuZCBrZXl3b3JkcyBtYXR0ZXJzIGFuZCBtdXN0IGJlIHVzZWQgY29uc2lzdGVudGx5LgoKRm9yIGV4YW1wbGUsIGluIEphdmFTY3JpcHQsIHRoZSB2YXJpYWJsZXMgbXlWYXJpYWJsZSwgbXl2YXJpYWJsZSwgYW5kIE1ZVkFSSUFCTEUgYXJlIGNvbnNpZGVyZWQgdG8gYmUgdGhyZWUgZGlmZmVyZW50IHZhcmlhYmxlcy4gU2ltaWxhcmx5LCBmdW5jdGlvbiBuYW1lcywgc3VjaCBhcyBteUZ1bmN0aW9uIGFuZCBteWZ1bmN0aW9uLCBhcmUgdHJlYXRlZCBhcyBzZXBhcmF0ZSBmdW5jdGlvbnMuCgpRNTEuIFdoYXQgaXMgYW4gYW5vbnltb3VzIGZ1bmN0aW9uPwoKQW4gYW5vbnltb3VzIGZ1bmN0aW9uLCBhbHNvIGtub3duIGFzIGEgZnVuY3Rpb24gZXhwcmVzc2lvbiwgaXMgYSBmdW5jdGlvbiBpbiBKYXZhU2NyaXB0IHRoYXQgaXMgZGVmaW5lZCB3aXRob3V0IGEgbmFtZS4gVW5saWtlIGEgbmFtZWQgZnVuY3Rpb24sIHdoaWNoIGlzIGRlY2xhcmVkIHdpdGggYSBzcGVjaWZpYyBpZGVudGlmaWVyLCBhbiBhbm9ueW1vdXMgZnVuY3Rpb24gZG9lcyBub3QgaGF2ZSBhbiBleHBsaWNpdCBuYW1lIGFzc29jaWF0ZWQgd2l0aCBpdC4KCkFub255bW91cyBmdW5jdGlvbnMgYXJlIHR5cGljYWxseSB1c2VkIHdoZW4geW91IG5lZWQgYSBmdW5jdGlvbiBmb3IgYSBzcGVjaWZpYyB0YXNrIGFuZCBkbyBub3QgcmVxdWlyZSBpdCB0byBiZSByZWZlcmVuY2VkIG9yIGNhbGxlZCBlbHNld2hlcmUgaW4geW91ciBjb2RlLiBUaGV5IGFyZSBjb21tb25seSB1c2VkIGFzIGNhbGxiYWNrIGZ1bmN0aW9ucywgZnVuY3Rpb24gYXJndW1lbnRzLCBvciBpbW1lZGlhdGVseSBpbnZva2VkIGZ1bmN0aW9uIGV4cHJlc3Npb25zIChJSUZFKS4KCkFub255bW91cyBmdW5jdGlvbnMgYXJlIHVzZWZ1bCBpbiBzY2VuYXJpb3Mgd2hlcmUgeW91IG5lZWQgYSBzaG9ydC1saXZlZCBvciBvbmUtdGltZSBmdW5jdGlvbiB3aXRob3V0IHRoZSBuZWVkIGZvciBhIHNwZWNpZmljIG5hbWUuIFRoZXkgaGVscCBpbiB3cml0aW5nIG1vcmUgY29uY2lzZSBjb2RlIGFuZCBjYW4gYmUgdXNlZCBpbmxpbmUgd2l0aG91dCBjbHV0dGVyaW5nIHRoZSBnbG9iYWwgbmFtZXNwYWNlIHdpdGggdW5uZWNlc3NhcnkgZnVuY3Rpb24gbmFtZXMuCgpJdCdzIGltcG9ydGFudCB0byBub3RlIHRoYXQgYW5vbnltb3VzIGZ1bmN0aW9ucyBjYW4gYWxzbyBiZSBhc3NpZ25lZCB0byB2YXJpYWJsZXMgb3IgdXNlZCBhcyBhcnJvdyBmdW5jdGlvbnMsIHByb3ZpZGluZyBmbGV4aWJpbGl0eSBpbiB2YXJpb3VzIGNvZGluZyBzY2VuYXJpb3MuCgpRNTIuIFdoYXQgaXMgUnVudGltZSBFcnJvcj8KCkEgcnVudGltZSBlcnJvciwgYWxzbyBrbm93biBhcyBhbiBleGNlcHRpb24sIGlzIGFuIGVycm9yIHRoYXQgb2NjdXJzIGR1cmluZyB0aGUgZXhlY3V0aW9uIG9mIGEgcHJvZ3JhbSBvciBzY3JpcHQuIEl0IGhhcHBlbnMgd2hlbiB0aGUgcHJvZ3JhbSBlbmNvdW50ZXJzIGFuIHVuZXhwZWN0ZWQgY29uZGl0aW9uIG9yIGJlaGF2aW9yIHRoYXQgcHJldmVudHMgaXQgZnJvbSBjb250aW51aW5nIGl0cyBub3JtYWwgZXhlY3V0aW9uLgoKUnVudGltZSBlcnJvcnMgYXJlIGRpc3RpbmN0IGZyb20gY29tcGlsZS10aW1lIGVycm9ycywgd2hpY2ggYXJlIGRldGVjdGVkIGJ5IHRoZSBjb21waWxlciBiZWZvcmUgdGhlIHByb2dyYW0gaXMgZXhlY3V0ZWQuIFJ1bnRpbWUgZXJyb3JzIG9jY3VyIHdoaWxlIHRoZSBwcm9ncmFtIGlzIHJ1bm5pbmcgYW5kIG9mdGVuIHJlc3VsdCBmcm9tIGxvZ2ljYWwgZXJyb3JzLCBpbnZhbGlkIGRhdGEsIG9yIHVuZm9yZXNlZW4gY2lyY3Vtc3RhbmNlcy4KCkhlcmUgYXJlIGEgZmV3IGNvbW1vbiBleGFtcGxlcyBvZiBydW50aW1lIGVycm9yczoKCkRpdmlzaW9uIGJ5IHplcm86IGNvbnN0IHJlc3VsdCA9IDEwIC8gMDsgLy8gVGhyb3dzIGEgcnVudGltZSBlcnJvciAoZGl2aXNpb24gYnkgemVybykKCgpVbmRlZmluZWQgdmFyaWFibGU6IGNvbnNvbGUubG9nKHgpOyAvLyBUaHJvd3MgYSBydW50aW1lIGVycm9yICh2YXJpYWJsZSB4IGlzIG5vdCBkZWZpbmVkKQoKCk51bGwgcmVmZXJlbmNlOiBjb25zdCBvYmogPSBudWxsOwpjb25zb2xlLmxvZyhvYmoucHJvcGVydHkpOyAvLyBUaHJvd3MgYSBydW50aW1lIGVycm9yIChhY2Nlc3NpbmcgcHJvcGVydHkgb2YgbnVsbCkKClR5cGUgbWlzbWF0Y2g6IGNvbnN0IHJlc3VsdCA9ICI1IiArIDI7IC8vIFRocm93cyBhIHJ1bnRpbWUgZXJyb3IgKGNvbmNhdGVuYXRpbmcgc3RyaW5nIHdpdGggYSBudW1iZXIpCgoKV2hlbiBhIHJ1bnRpbWUgZXJyb3Igb2NjdXJzLCBpdCBpbnRlcnJ1cHRzIHRoZSBub3JtYWwgZmxvdyBvZiB0aGUgcHJvZ3JhbSBhbmQgbWF5IGNhdXNlIGl0IHRvIHRlcm1pbmF0ZSBwcmVtYXR1cmVseS4gSW4gbWFueSBwcm9ncmFtbWluZyBsYW5ndWFnZXMsIGluY2x1ZGluZyBKYXZhU2NyaXB0LCBydW50aW1lIGVycm9ycyBjYW4gYmUgaGFuZGxlZCBhbmQgY29udHJvbGxlZCB0aHJvdWdoIHRoZSB1c2Ugb2YgZXhjZXB0aW9uIGhhbmRsaW5nIG1lY2hhbmlzbXMsIHN1Y2ggYXMgdHJ5LWNhdGNoIGJsb2Nrcy4KCkJ5IHVzaW5nIHRyeS1jYXRjaCBibG9ja3MsIHlvdSBjYW4gY2F0Y2ggYW5kIGhhbmRsZSBydW50aW1lIGVycm9ycyBncmFjZWZ1bGx5LCBwcmV2ZW50aW5nIHRoZW0gZnJvbSBjYXVzaW5nIHRoZSBwcm9ncmFtIHRvIGNyYXNoLiBUaGlzIGFsbG93cyB5b3UgdG8gcHJvdmlkZSBhbHRlcm5hdGl2ZSBwYXRocyBvZiBleGVjdXRpb24gb3IgZGlzcGxheSBlcnJvciBtZXNzYWdlcyB0byB0aGUgdXNlci4KClE1My4gV2hhdCBpcyBBcnJvdyBGdW5jdGlvbj8KCkFuIGFycm93IGZ1bmN0aW9uLCBhbHNvIGtub3duIGFzIGEgZmF0IGFycm93IGZ1bmN0aW9uLCBpcyBhIGNvbmNpc2Ugc3ludGF4IGludHJvZHVjZWQgaW4gRUNNQVNjcmlwdCAyMDE1IChFUzYpIGZvciBjcmVhdGluZyBmdW5jdGlvbnMgaW4gSmF2YVNjcmlwdC4gQXJyb3cgZnVuY3Rpb25zIHByb3ZpZGUgYSBtb3JlIGNvbXBhY3QgYW5kIHN0cmVhbWxpbmVkIHdheSB0byB3cml0ZSBmdW5jdGlvbiBleHByZXNzaW9ucy4KClRoZSBzeW50YXggZm9yIGFuIGFycm93IGZ1bmN0aW9uIGluY2x1ZGVzIGEgcGFyYW1ldGVyIGxpc3QgKGlmIGFueSksIGZvbGxvd2VkIGJ5IHRoZSBhcnJvdyAoPT4pIGFuZCB0aGVuIHRoZSBmdW5jdGlvbiBib2R5LiBUaGUgZnVuY3Rpb24gYm9keSBjYW4gYmUgZWl0aGVyIGFuIGV4cHJlc3Npb24gdGhhdCBpcyBpbXBsaWNpdGx5IHJldHVybmVkIG9yIGEgYmxvY2sgb2Ygc3RhdGVtZW50cyBlbmNsb3NlZCBpbiBjdXJseSBicmFjZXMgKHt9KS4gVGhlIGFycm93IGZ1bmN0aW9ucyBoYXZlIGEgbGV4aWNhbCB0aGlzIGJpbmRpbmcsIG1lYW5pbmcgdGhhdCB0aGV5IGNhcHR1cmUgdGhlIHZhbHVlIG9mIHRoaXMgZnJvbSB0aGUgc3Vycm91bmRpbmcgY29udGV4dCBhdCB0aGUgdGltZSBvZiB0aGVpciBjcmVhdGlvbi4KCkFycm93IGZ1bmN0aW9ucyBhcmUgZXNwZWNpYWxseSB1c2VmdWwgd2hlbiB5b3Ugd2FudCB0byB3cml0ZSBjb25jaXNlIGFuZCBpbmxpbmUgZnVuY3Rpb25zIG9yIHdoZW4geW91IG5lZWQgdG8gcHJlc2VydmUgdGhlIGxleGljYWwgdGhpcyBjb250ZXh0LiBIb3dldmVyLCBpdCdzIGltcG9ydGFudCB0byBub3RlIHRoYXQgYXJyb3cgZnVuY3Rpb25zIGhhdmUgc29tZSBkaWZmZXJlbmNlcyBjb21wYXJlZCB0byB0cmFkaXRpb25hbCBmdW5jdGlvbiBleHByZXNzaW9ucy4gRm9yIGV4YW1wbGUsIGFycm93IGZ1bmN0aW9ucyBkbyBub3QgaGF2ZSB0aGVpciBvd24gdGhpcywgYXJndW1lbnRzLCBvciBzdXBlciBiaW5kaW5ncywgYW5kIHRoZXkgY2Fubm90IGJlIHVzZWQgYXMgY29uc3RydWN0b3JzIHdpdGggdGhlIG5ldyBrZXl3b3JkLiBUaGVzZSBmYWN0b3JzIHNob3VsZCBiZSBjb25zaWRlcmVkIHdoZW4gZGVjaWRpbmcgd2hldGhlciB0byB1c2UgYXJyb3cgZnVuY3Rpb25zIGluIHNwZWNpZmljIHNjZW5hcmlvcy4KCgoKUTU0LiBXaGF0IGlzIGEgY2FsbGJhY2sgZnVuY3Rpb24gaW4gSmF2YVNjcmlwdD8KCkluIEphdmFTY3JpcHQsIGEgY2FsbGJhY2sgZnVuY3Rpb24gaXMgYSBmdW5jdGlvbiB0aGF0IGlzIHBhc3NlZCBhcyBhbiBhcmd1bWVudCB0byBhbm90aGVyIGZ1bmN0aW9uIGFuZCBpcyBleGVjdXRlZCBhdCBhIGxhdGVyIHBvaW50IGluIHRpbWUuIFRoZSBwdXJwb3NlIG9mIGEgY2FsbGJhY2sgZnVuY3Rpb24gaXMgdG8gcHJvdmlkZSBhIHdheSB0byBoYW5kbGUgYXN5bmNocm9ub3VzIG9yIGV2ZW50LWRyaXZlbiBvcGVyYXRpb25zLCBhbGxvd2luZyBjb2RlIHRvIGJlIGV4ZWN1dGVkIHdoZW4gYSBwYXJ0aWN1bGFyIHRhc2sgb3IgZXZlbnQgaGFzIGNvbXBsZXRlZC4KCkNhbGxiYWNrcyBhcmUgY29tbW9ubHkgdXNlZCBpbiBzY2VuYXJpb3Mgc3VjaCBhcyBtYWtpbmcgYXN5bmNocm9ub3VzIHJlcXVlc3RzLCBoYW5kbGluZyB1c2VyIGludGVyYWN0aW9ucywgYW5kIGltcGxlbWVudGluZyBldmVudC1kcml2ZW4gcHJvZ3JhbW1pbmcuIFRoZXkgYWxsb3cgeW91IHRvIHNwZWNpZnkgd2hhdCBzaG91bGQgaGFwcGVuIGFmdGVyIGEgcGFydGljdWxhciB0YXNrIG9yIGV2ZW50IGZpbmlzaGVzIGV4ZWN1dGluZy4KCkhlcmUncyBhbiBleGFtcGxlIG9mIGEgY2FsbGJhY2sgZnVuY3Rpb24gdXNlZCB3aXRoIHNldFRpbWVvdXQoKToKCmZ1bmN0aW9uIGdyZWV0KG5hbWUsIGNhbGxiYWNrKSB7CmNvbnNvbGUubG9nKCJIZWxsbywgIiArIG5hbWUgKyAiISIpOwpjYWxsYmFjaygpOwp9CgpmdW5jdGlvbiBzYXlHb29kYnllKCkgewpjb25zb2xlLmxvZygiR29vZGJ5ZSEiKTsKfQoKZ3JlZXQoIkFsaWNlIiwgc2F5R29vZGJ5ZSk7CgoKSW4gdGhpcyBleGFtcGxlLCB0aGUgZ3JlZXQoKSBmdW5jdGlvbiB0YWtlcyB0d28gYXJndW1lbnRzOiBuYW1lIGFuZCBjYWxsYmFjay4gSXQgbG9ncyBhIGdyZWV0aW5nIG1lc3NhZ2UgdG8gdGhlIGNvbnNvbGUgYW5kIHRoZW4gZXhlY3V0ZXMgdGhlIGNhbGxiYWNrIGZ1bmN0aW9uLiBUaGUgc2F5R29vZGJ5ZSgpIGZ1bmN0aW9uIGlzIGRlZmluZWQgc2VwYXJhdGVseSBhbmQgcGFzc2VkIGFzIGEgY2FsbGJhY2sgdG8gZ3JlZXQoKS4gV2hlbiBncmVldCgiQWxpY2UiLCBzYXlHb29kYnllKSBpcyBjYWxsZWQsIGl0IGZpcnN0IHByaW50cyAiSGVsbG8sIEFsaWNlISIgYW5kIHRoZW4gZXhlY3V0ZXMgdGhlIHNheUdvb2RieWUoKSBmdW5jdGlvbiwgd2hpY2ggcHJpbnRzICJHb29kYnllISIuCgpDYWxsYmFja3MgcHJvdmlkZSBhIHdheSB0byBjb250cm9sIHRoZSBmbG93IG9mIGV4ZWN1dGlvbiBpbiBhc3luY2hyb25vdXMgb3IgZXZlbnQtZHJpdmVuIGVudmlyb25tZW50cywgYXMgdGhleSBhbGxvdyB5b3UgdG8gc3BlY2lmeSB0aGUgY29kZSB0byBiZSBleGVjdXRlZCBvbmNlIGEgY2VydGFpbiB0YXNrIG9yIGV2ZW50IGlzIGNvbXBsZXRlZC4gVGhpcyBlbmFibGVzIGhhbmRsaW5nIG9mIHJlc3VsdHMsIGVycm9yIGhhbmRsaW5nLCBhbmQgY29udGludWF0aW9uIG9mIGV4ZWN1dGlvbiBhZnRlciB0aGUgYXN5bmNocm9ub3VzIG9wZXJhdGlvbiBmaW5pc2hlcy4KClE1NS4gV2hhdCBpcyBhIGJsdXIgZnVuY3Rpb24/CgpJbiBKYXZhU2NyaXB0LCB0aGUgYmx1ciBmdW5jdGlvbiBpcyBhbiBldmVudCBoYW5kbGVyIHRoYXQgaXMgdHJpZ2dlcmVkIHdoZW4gYW4gZWxlbWVudCBsb3NlcyBmb2N1cy4gV2hlbiBhbiBlbGVtZW50LCBzdWNoIGFzIGFuIGlucHV0IGZpZWxkIG9yIGEgYnV0dG9uLCBpcyBpbiBmb2N1cyBhbmQgdGhlIHVzZXIgaW50ZXJhY3RzIHdpdGggYW5vdGhlciBlbGVtZW50LCBzdWNoIGFzIGNsaWNraW5nIG91dHNpZGUgdGhlIGN1cnJlbnQgZWxlbWVudCwgdGhlIGJsdXIgZXZlbnQgaXMgZmlyZWQuCgpUaGUgYmx1ciBldmVudCBpcyBjb21tb25seSB1c2VkIHRvIHBlcmZvcm0gYWN0aW9ucyBvciB2YWxpZGF0aW9uIHdoZW4gYW4gZWxlbWVudCBsb3NlcyBmb2N1cy4gRm9yIGV4YW1wbGUsIHlvdSBtaWdodCB3YW50IHRvIHZhbGlkYXRlIHRoZSBpbnB1dCBpbiBhIGZvcm0gZmllbGQgd2hlbiB0aGUgdXNlciBtb3ZlcyB0byB0aGUgbmV4dCBmaWVsZCBvciBjbGlja3Mgb3V0c2lkZSB0aGUgZmllbGQuCgpUaGUgYmx1ciBldmVudCBjYW4gYmUgdXNlZnVsIGZvciBlbmhhbmNpbmcgdXNlciBleHBlcmllbmNlIGFuZCBwcm92aWRpbmcgZmVlZGJhY2sgb3IgcGVyZm9ybWluZyBzcGVjaWZpYyBhY3Rpb25zIHdoZW4gZWxlbWVudHMgbG9zZSBmb2N1cy4gSXQgaXMgY29tbW9ubHkgdXNlZCBpbiBmb3JtIHZhbGlkYXRpb24gc2NlbmFyaW9zLCB3aGVyZSB5b3Ugd2FudCB0byB2YWxpZGF0ZSB1c2VyIGlucHV0IGFzIHRoZXkgbW92ZSBhd2F5IGZyb20gYW4gaW5wdXQgZmllbGQuCgoKCgpRNTYuIFdoYXQgZG8geW91IHVuZGVyc3RhbmQgYnkgY29va2llcyBpbiBKYXZhU2NyaXB0PwoKSW4gSmF2YVNjcmlwdCwgY29va2llcyBhcmUgc21hbGwgcGllY2VzIG9mIGRhdGEgdGhhdCBjYW4gYmUgc3RvcmVkIG9uIGEgdXNlcidzIGNvbXB1dGVyIGJ5IGEgd2Vic2l0ZS4gVGhleSBhcmUgdXNlZCB0byBzdG9yZSBpbmZvcm1hdGlvbiBhYm91dCB0aGUgdXNlciBvciB0aGVpciBpbnRlcmFjdGlvbnMgd2l0aCB0aGUgd2Vic2l0ZS4gQ29va2llcyBhcmUgcHJpbWFyaWx5IHVzZWQgdG8gbWFpbnRhaW4gc3RhdGVmdWwgaW5mb3JtYXRpb24gYWNyb3NzIG11bHRpcGxlIHJlcXVlc3RzIG9yIHNlc3Npb25zLgoKV2hlbiBhIHdlYnNpdGUgc2VuZHMgYSByZXNwb25zZSB0byB0aGUgdXNlcidzIGJyb3dzZXIsIGl0IGNhbiBpbmNsdWRlIGEgIlNldC1Db29raWUiIGhlYWRlciwgd2hpY2ggY29udGFpbnMgdGhlIGRhdGEgdG8gYmUgc3RvcmVkIGluIGEgY29va2llLiBUaGUgYnJvd3NlciB0aGVuIHN0b3JlcyB0aGlzIGRhdGEgbG9jYWxseS4gT24gc3Vic2VxdWVudCByZXF1ZXN0cyB0byB0aGUgc2FtZSB3ZWJzaXRlLCB0aGUgYnJvd3NlciBhdXRvbWF0aWNhbGx5IGluY2x1ZGVzIHRoZSBzdG9yZWQgY29va2llIGRhdGEgaW4gdGhlICJDb29raWUiIGhlYWRlciBvZiB0aGUgcmVxdWVzdCwgYWxsb3dpbmcgdGhlIHdlYnNpdGUgdG8gYWNjZXNzIGFuZCB1c2UgdGhhdCBpbmZvcm1hdGlvbi4KCkNvb2tpZXMgaGF2ZSB2YXJpb3VzIHByb3BlcnRpZXMgdGhhdCBjYW4gYmUgc2V0LCBzdWNoIGFzIHRoZSBleHBpcmF0aW9uIGRhdGUsIHBhdGgsIGFuZCBkb21haW4uIFRoZXNlIHByb3BlcnRpZXMgZGV0ZXJtaW5lIHdoZW4gYW5kIHdoZXJlIHRoZSBjb29raWUgaXMgc2VudCBiYWNrIHRvIHRoZSBzZXJ2ZXIuIEZvciBleGFtcGxlLCBhIGNvb2tpZSBjYW4gYmUgc2V0IHRvIGV4cGlyZSBhZnRlciBhIGNlcnRhaW4gcGVyaW9kIG9mIHRpbWUgb3IgYmUgcmVzdHJpY3RlZCB0byBhIHNwZWNpZmljIHN1YmRvbWFpbi4KCkluIEphdmFTY3JpcHQsIHlvdSBjYW4gaW50ZXJhY3Qgd2l0aCBjb29raWVzIHVzaW5nIHRoZSBkb2N1bWVudC5jb29raWUgcHJvcGVydHkuIEl0IHByb3ZpZGVzIGFjY2VzcyB0byB0aGUgY29va2llcyBhc3NvY2lhdGVkIHdpdGggdGhlIGN1cnJlbnQgZG9jdW1lbnQuIFRoZSBkb2N1bWVudC5jb29raWUgcHJvcGVydHkgYWxsb3dzIHlvdSB0byByZWFkLCB3cml0ZSwgYW5kIGRlbGV0ZSBjb29raWVzLgoKSGVyZSdzIGFuIGV4YW1wbGUgb2YgaG93IHRvIHNldCBhIGNvb2tpZSB1c2luZyBKYXZhU2NyaXB0OgoKZG9jdW1lbnQuY29va2llID0gInVzZXJuYW1lPUpvaG4gRG9lOyBleHBpcmVzPVRodSwgMTkgSnVuIDIwMjUgMTI6MDA6MDAgVVRDOyBwYXRoPS8iOwoKCkl0J3MgaW1wb3J0YW50IHRvIG5vdGUgdGhhdCBjb29raWVzIGhhdmUgY2VydGFpbiBsaW1pdGF0aW9ucywgc3VjaCBhcyB0aGVpciBsaW1pdGVkIHN0b3JhZ2Ugc2l6ZSBhbmQgcG90ZW50aWFsIHNlY3VyaXR5IHJpc2tzIChlLmcuLCBjcm9zcy1zaXRlIHNjcmlwdGluZyB2dWxuZXJhYmlsaXRpZXMpLiBBcyBhIHJlc3VsdCwgYWx0ZXJuYXRpdmUgbWV0aG9kcyBsaWtlIGJyb3dzZXIgc3RvcmFnZSBBUElzIChsb2NhbFN0b3JhZ2UsIHNlc3Npb25TdG9yYWdlKSBhbmQgc2VydmVyLXNpZGUgc2Vzc2lvbiBtYW5hZ2VtZW50IGFyZSBvZnRlbiB1c2VkIGluIG1vZGVybiB3ZWIgZGV2ZWxvcG1lbnQuCgpRNTcuIFdoYXQgaXMgQXN5bmNocm9ub3VzIHRlc3RpbmcgaW4gSmF2YVNjcmlwdD8KCkl0J3MgY29tbW9uIGluIEphdmFTY3JpcHQgZm9yIGNvZGUgdG8gcnVuIGFzeW5jaHJvbm91c2x5LiBXaGVuIHlvdSBoYXZlIGNvZGUgdGhhdCBydW5zIGFzeW5jaHJvbm91c2x5LgoKPHNjcmlwdD4KCmRvY3VtZW50LndyaXRlKCJIaSIpOwoKZG9jdW1lbnQud3JpdGUoIjxicj4iKTsKCnNldFRpbWVvdXQoKCkgPT4gewoKCmRvY3VtZW50LndyaXRlKCJMZXQgdXMgc2VlIHdoYXQgaGFwcGVucyIpOwoKfSwgMjAwMCk7Cgpkb2N1bWVudC53cml0ZSgiPGJyPiIpOwoKCmRvY3VtZW50LndyaXRlKCJFbmQiKTsKCmRvY3VtZW50LndyaXRlKCI8YnI+Iik7Cgo8L3NjcmlwdD4KClNvLCB3aGF0IHRoZSBjb2RlIGRvZXMgaXMgZmlyc3QgaXQgbG9ncyBpbsKgSGnCoHRoZW4gcmF0aGVyIHRoYW4gZXhlY3V0aW5nIHRoZcKgc2V0VGltZW91dMKgZnVuY3Rpb24gaXQgbG9ncyBpbsKgRW5kwqBhbmQgdGhlbiBpdCBydW5zIHRoZcKgc2V0VGltZW91dMKgZnVuY3Rpb24uCgpBdCBmaXJzdCwgYXMgdXN1YWwsIHRoZcKgSGnCoCB0aGUgc3RhdGVtZW50IGdvdCBsb2dnZWQgaW4uIEFzIHdlIHVzZSBicm93c2VycyB0byBydW4gSmF2YVNjcmlwdCwgdGhlcmUgYXJlIHdlYiBBUElzIHRoYXQgaGFuZGxlIHRoZXNlIHRoaW5ncyBmb3IgdXNlcnMuIFNvLCB3aGF0IEphdmFTY3JpcHQgZG9lcyBpcywgaXQgcGFzc2VzIHRoZSBzZXRUaW1lb3V0IGZ1bmN0aW9uIGluIHN1Y2ggd2ViIEFQSSBhbmQgdGhlbiB3ZSBrZWVwIG9uIHJ1bm5pbmcgb3VyIGNvZGUgYXMgdXN1YWwuIFNvIGl0IGRvZXMgbm90IGJsb2NrIHRoZSByZXN0IG9mIHRoZSBjb2RlIGZyb20gZXhlY3V0aW5nIGFuZCBhZnRlciBhbGwgdGhlIGNvZGUgaXMgZXhlY3V0ZWQsIGl0IGdldHMgcHVzaGVkIHRvIHRoZSBjYWxsIHN0YWNrIGFuZCB0aGVuIGZpbmFsbHkgZ2V0cyBleGVjdXRlZC4gVGhpcyBpcyB3aGF0IGhhcHBlbnMgaW4gYXN5bmNocm9ub3VzIEphdmFTY3JpcHQKCsKgUTU4LiBXaGF0IGlzIGFuIEFzeW5jIEZ1bmN0aW9uPwoKQW4gYXN5bmMgZnVuY3Rpb24gaXMgYSB0eXBlIG9mIGZ1bmN0aW9uIGluIEphdmFTY3JpcHQgdGhhdCBlbmFibGVzIGFzeW5jaHJvbm91cyBiZWhhdmlvciBhbmQgYWxsb3dzIHlvdSB0byB3cml0ZSBhc3luY2hyb25vdXMgY29kZSBpbiBhIG1vcmUgc3luY2hyb25vdXMtbGlrZSBtYW5uZXIuIEl0IHdhcyBpbnRyb2R1Y2VkIGluIEVDTUFTY3JpcHQgMjAxNyAoRVM4KSBhbmQgYnVpbHQgdXBvbiBQcm9taXNlcywgcHJvdmlkaW5nIGEgc2ltcGxlciBhbmQgY2xlYW5lciBzeW50YXggZm9yIGhhbmRsaW5nIGFzeW5jaHJvbm91cyBvcGVyYXRpb25zLgoKVG8gZGVmaW5lIGFuIGFzeW5jIGZ1bmN0aW9uLCB5b3UgdXNlIHRoZSBhc3luYyBrZXl3b3JkIGJlZm9yZSB0aGUgZnVuY3Rpb24gZGVjbGFyYXRpb24uIEFuIGFzeW5jIGZ1bmN0aW9uIGFsd2F5cyByZXR1cm5zIGEgUHJvbWlzZSwgd2hpY2ggcmVwcmVzZW50cyB0aGUgZXZlbnR1YWwgY29tcGxldGlvbiBvciBmYWlsdXJlIG9mIGFuIGFzeW5jaHJvbm91cyBvcGVyYXRpb24uIFdpdGhpbiBhbiBhc3luYyBmdW5jdGlvbiwgeW91IGNhbiB1c2UgdGhlIGF3YWl0IGtleXdvcmQgdG8gcGF1c2UgdGhlIGV4ZWN1dGlvbiBvZiB0aGUgZnVuY3Rpb24gdW50aWwgYSBQcm9taXNlIGlzIHJlc29sdmVkIG9yIHJlamVjdGVkLgoKVXNpbmcgYXN5bmMvYXdhaXQgc2ltcGxpZmllcyB0aGUgaGFuZGxpbmcgb2YgYXN5bmNocm9ub3VzIG9wZXJhdGlvbnMgYnkgZWxpbWluYXRpbmcgdGhlIG5lZWQgZm9yIG5lc3RlZCBjYWxsYmFja3Mgb3IgY2hhaW5pbmcgbXVsdGlwbGUgdGhlbigpIG1ldGhvZHMuIEl0IGFsbG93cyB5b3UgdG8gd3JpdGUgYXN5bmNocm9ub3VzIGNvZGUgaW4gYSBtb3JlIGxpbmVhciBhbmQgcmVhZGFibGUgd2F5LCByZXNlbWJsaW5nIHN5bmNocm9ub3VzIGNvZGUgZmxvdy4gQWRkaXRpb25hbGx5LCBhc3luYyBmdW5jdGlvbnMgcHJvdmlkZSBhIGNvbnZlbmllbnQgd2F5IHRvIGhhbmRsZSBlcnJvcnMgYnkgdXRpbGl6aW5nIHRoZSB0cnkvY2F0Y2ggYmxvY2suCgpJdCdzIGltcG9ydGFudCB0byBub3RlIHRoYXQgYXN5bmMgZnVuY3Rpb25zIGFyZSBub24tYmxvY2tpbmcsIG1lYW5pbmcgdGhhdCB3aGlsZSBhd2FpdGluZyBhIFByb21pc2UsIHRoZSBleGVjdXRpb24gb2YgdGhlIGZ1bmN0aW9uIGlzIHBhdXNlZCwgYWxsb3dpbmcgb3RoZXIgY29kZSB0byBydW4gaW4gdGhlIG1lYW50aW1lLiBUaGlzIG1ha2VzIHRoZW0gcGFydGljdWxhcmx5IHVzZWZ1bCB3aGVuIGRlYWxpbmcgd2l0aCB0aW1lLWNvbnN1bWluZyB0YXNrcywgc3VjaCBhcyBuZXR3b3JrIHJlcXVlc3RzIG9yIHNvdXJjZSBmaWxlIG9wZXJhdGlvbnMsIHdpdGhvdXQgYmxvY2tpbmcgdGhlIGV4ZWN1dGlvbiBvZiBvdGhlciBwYXJ0cyBvZiB5b3VyIGNvZGUuCgpRNTkuIFdoYXQgaXMgZXZlbnQgYnViYmxpbmcgaW4gSmF2YVNjcmlwdD8KCkV2ZW50IGJ1YmJsaW5nLCBhbHNvIGtub3duIGFzIGV2ZW50IHByb3BhZ2F0aW9uLCBpcyBhIG1lY2hhbmlzbSBpbiBKYXZhU2NyaXB0IHdoZXJlIGFuIGV2ZW50IHRyaWdnZXJlZCBvbiBhIG5lc3RlZCBlbGVtZW50IGlzIHByb3BhZ2F0ZWQgb3IgImJ1YmJsZWQgdXAiIHRocm91Z2ggaXRzIHBhcmVudCBlbGVtZW50cyBpbiB0aGUgRE9NIGhpZXJhcmNoeS4gVGhpcyBtZWFucyB0aGF0IHdoZW4gYW4gZXZlbnQgb2NjdXJzIG9uIGFuIGVsZW1lbnQsIHN1Y2ggYXMgYSBjbGljayBldmVudCwgdGhlIGV2ZW50IGlzIGZpcnN0IGhhbmRsZWQgYnkgdGhlIGlubmVybW9zdCBlbGVtZW50IGFuZCB0aGVuIHNlcXVlbnRpYWxseSBwcm9wYWdhdGVkIHRvIGl0cyBwYXJlbnQgZWxlbWVudHMsIGFsbCB0aGUgd2F5IHVwIHRvIHRoZSByb290IG9mIHRoZSBkb2N1bWVudC4KCkhlcmUncyBhbiBleGFtcGxlIHRvIGlsbHVzdHJhdGUgZXZlbnQgYnViYmxpbmc6Cgo8ZGl2Pgo8ZGl2Pgo8YnV0dG9uPkNsaWNrIG1lPC9idXR0b24+CjwvZGl2Pgo8L2Rpdj4KCgpkb2N1bWVudC5nZXRFbGVtZW50QnlJZCgnYnV0dG9uJykuYWRkRXZlbnRMaXN0ZW5lcignY2xpY2snLCBmdW5jdGlvbihldmVudCkgewpjb25zb2xlLmxvZygnQnV0dG9uIGNsaWNrZWQnKTsKY29uc29sZS5sb2coJ1RhcmdldDonLCBldmVudC50YXJnZXQpOwpjb25zb2xlLmxvZygnQ3VycmVudCBUYXJnZXQ6JywgZXZlbnQuY3VycmVudFRhcmdldCk7Cn0pOwoKCkluIHRoaXMgZXhhbXBsZSwgd2hlbiB0aGUgYnV0dG9uIGlzIGNsaWNrZWQsIHRoZSBldmVudCBmaXJzdCB0cmlnZ2VycyB0aGUgZXZlbnQgaGFuZGxlciBhdHRhY2hlZCB0byB0aGUgYnV0dG9uLiBIb3dldmVyLCBhZnRlciB0aGUgYnV0dG9uJ3MgZXZlbnQgaGFuZGxlciBmaW5pc2hlcyBleGVjdXRpb24sIHRoZSBldmVudCBjb250aW51ZXMgdG8gcHJvcGFnYXRlIHVwIHRoZSBET00gdHJlZS4gSW4gdGhpcyBjYXNlLCB0aGUgZXZlbnQgaGFuZGxlciBpcyBub3QgZGVmaW5lZCBmb3IgdGhlIHBhcmVudCBlbGVtZW50cyBleHBsaWNpdGx5LiBCdXQgaWYgdGhlcmUgd2VyZSBldmVudCBoYW5kbGVycyBkZWZpbmVkIGZvciB0aGUgcGFyZW50IGVsZW1lbnRzIChzdWNoIGFzIHRoZSBkaXZzIHdpdGggSURzICJpbm5lciIgYW5kICJvdXRlciIpLCB0aGUgZXZlbnQgd291bGQgY29udGludWUgdG8gcHJvcGFnYXRlIGFuZCB0cmlnZ2VyIHRob3NlIGV2ZW50IGhhbmRsZXJzIGFzIHdlbGwuCgpFdmVudCBidWJibGluZyBhbGxvd3MgeW91IHRvIGhhbmRsZSBldmVudHMgYXQgZGlmZmVyZW50IGxldmVscyBvZiB0aGUgRE9NIGhpZXJhcmNoeS4gSXQgc2ltcGxpZmllcyBldmVudCBoYW5kbGluZyBieSBhbGxvd2luZyB5b3UgdG8gYXR0YWNoIGEgc2luZ2xlIGV2ZW50IGxpc3RlbmVyIHRvIGEgcGFyZW50IGVsZW1lbnQvIHBhcmVudCBvYmplY3QgYW5kIGhhbmRsZSBldmVudHMgZm9yIGl0cyBjaGlsZCBlbGVtZW50cyBhcyB3ZWxsLiBUaGlzIGNhbiBiZSBiZW5lZmljaWFsIGZvciBtYW5hZ2luZyBldmVudCBkZWxlZ2F0aW9uIGFuZCByZWR1Y2luZyB0aGUgbnVtYmVyIG9mIGV2ZW50IGxpc3RlbmVycyBhdHRhY2hlZCB0byBpbmRpdmlkdWFsIGVsZW1lbnRzLiBIb3dldmVyLCBpdCdzIGltcG9ydGFudCB0byBiZSBhd2FyZSBvZiBldmVudCBidWJibGluZyBhbmQgcHJvcGVybHkgaGFuZGxlIGV2ZW50IHByb3BhZ2F0aW9uIGlmIG5lY2Vzc2FyeSB0byBwcmV2ZW50IHVuaW50ZW5kZWQgYmVoYXZpb3Igb3IgY29uZmxpY3RzLgoKUTYwLiBXaGF0IGlzIEFzeW5jaHJvbm91cyBjb2RlIGluIEphdmFTY3JpcHQ/CgpBc3luY2hyb25vdXMgSmF2YVNjcmlwdDogQXN5bmNocm9ub3VzIGNvZGUgYWxsb3dzIHRoZSBwcm9ncmFtIHRvIGJlIGV4ZWN1dGVkIGltbWVkaWF0ZWx5IHdoZXJlYXMgdGhlIHN5bmNocm9ub3VzIGNvZGUgd2lsbCBibG9jayBmdXJ0aGVyIGV4ZWN1dGlvbiBvZiB0aGUgcmVtYWluaW5nIGNvZGUgdW50aWwgaXQgZmluaXNoZXMgdGhlIGN1cnJlbnQgb25lLiBUaGlzIG1heSBub3QgbG9vayBsaWtlIGEgYmlnIHByb2JsZW0gYnV0IHdoZW4geW91IHNlZSBpdCBpbiBhIGJpZ2dlciBwaWN0dXJlIHlvdSByZWFsaXplIHRoYXQgaXQgbWF5IGxlYWQgdG8gZGVsYXlpbmcgdGhlIFVzZXIgSW50ZXJmYWNlLgoKTGV0IHVzIHNlZSB0aGUgZXhhbXBsZSBvZiBob3cgQXN5bmNocm9ub3VzIEphdmFTY3JpcHQgcnVucy4KCjxzY3JpcHQ+CgrCoMKgwqDCoGRvY3VtZW50LndyaXRlKCJIaSIpOwoKwqDCoMKgwqBkb2N1bWVudC53cml0ZSgiPGJyPiIpOwoKwqDCoAoKwqDCoMKgwqBzZXRUaW1lb3V0KCgpID0+IHsKCsKgwqDCoMKgwqDCoMKgwqBkb2N1bWVudC53cml0ZSgiTGV0IHVzIHNlZSB3aGF0IGhhcHBlbnMiKTsKCsKgwqDCoMKgfSwgMjAwMCk7CgrCoMKgCgrCoMKgwqDCoGRvY3VtZW50LndyaXRlKCI8YnI+Iik7CgrCoMKgwqDCoGRvY3VtZW50LndyaXRlKCJFbmQiKTsKCsKgwqDCoMKgZG9jdW1lbnQud3JpdGUoIjxicj4iKTsKCjwvc2NyaXB0PgoKT3V0cHV0OgoKClNvLCB3aGF0IHRoZSBjb2RlIGRvZXMgaXMgZmlyc3QgaXQgbG9ncyBpbsKgSGnCoHRoZW4gcmF0aGVyIHRoYW4gZXhlY3V0aW5nIHRoZcKgc2V0VGltZW91dMKgZnVuY3Rpb24gaXQgbG9ncyBpbsKgRW5kLMKgYW5kIHRoZW4gaXQgcnVucyB0aGXCoHNldFRpbWVvdXTCoGZ1bmN0aW9uLgoKQXQgZmlyc3QsIGFzIHVzdWFsLCB0aGXCoEhpIHN0YXRlbWVudCBnb3QgbG9nZ2VkIGluLiBBcyB3ZSB1c2UgYnJvd3NlcnMgdG8gcnVuIEphdmFTY3JpcHQsIHRoZXJlIGFyZSB3ZWIgQVBJcyB0aGF0IGhhbmRsZSB0aGVzZSB0aGluZ3MgZm9yIHVzZXJzLiBTbywgd2hhdCBKYXZhU2NyaXB0IGRvZXMgaXMsIGl0IHBhc3NlcyB0aGXCoHNldFRpbWVvdXQgZnVuY3Rpb24gaW4gc3VjaCB3ZWIgQVBJIGFuZCB0aGVuIHdlIGtlZXAgb24gcnVubmluZyBvdXIgY29kZSBhcyB1c3VhbC4gU28gaXQgZG9lcyBub3QgYmxvY2sgdGhlIHJlc3Qgb2YgdGhlIGNvZGUgZnJvbSBleGVjdXRpbmcgYW5kIGFmdGVyIGFsbCB0aGUgY29kZSBpcyBleGVjdXRlZCwgaXQgZ2V0cyBwdXNoZWQgdG8gdGhlIGNhbGwgc3RhY2sgYW5kIHRoZW4gZmluYWxseSBnZXRzIGV4ZWN1dGVkLiBUaGlzIGlzIHdoYXQgaGFwcGVucyBpbiBhc3luY2hyb25vdXMgSmF2YVNjcmlwdC4KClE2MS4gV2hhdCBpcyByZXN0IFN5bnRheCBvciBSZXN0IE9wZXJhdG9yLCBhbmQgaG93IGlzIGl0IG9wcG9zaXRlIHRvIHNwcmVhZCBvcGVyYXRvciBvciBzcHJlYWQgc3ludGF4PwoKUmVzdCBzeW50YXggb3IgcmVzdCBwYXJhbWV0ZXIgaXMgYSBmZWF0dXJlIGludHJvZHVjZWQgaW4gRUNNQVNjcmlwdCAyMDE1IChFUzYpIHRoYXQgYWxsb3dzIHlvdSB0byByZXByZXNlbnQgYW4gaW5kZWZpbml0ZSBudW1iZXIgb2YgYXJndW1lbnRzIGFzIGFuIGFycmF5IHdpdGhpbiBhIGZ1bmN0aW9uIGRlY2xhcmF0aW9uLiBJdCBpcyBkZW5vdGVkIGJ5IHVzaW5nIHRocmVlIGRvdHMgKC4uLikgZm9sbG93ZWQgYnkgYSBwYXJhbWV0ZXIgbmFtZSBpbiB0aGUgZnVuY3Rpb24gZGVjbGFyYXRpb24uIFRoZSByZXN0IHBhcmFtZXRlciBnYXRoZXJzIGFsbCB0aGUgcmVtYWluaW5nIGFyZ3VtZW50cyBpbnRvIGFuIGFycmF5LgoKSGVyZSdzIGFuIGV4YW1wbGUgdGhhdCBkZW1vbnN0cmF0ZXMgdGhlIHVzZSBvZiByZXN0IHN5bnRheDoKCmZ1bmN0aW9uIHN1bSguLi5udW1iZXJzKSB7CmxldCB0b3RhbCA9IDA7CmZvciAobGV0IG51bWJlciBvZiBudW1iZXJzKSB7CnRvdGFsICs9IG51bWJlcjsKfQpyZXR1cm4gdG90YWw7Cn0KCmNvbnNvbGUubG9nKHN1bSgxLCAyLCAzLCA0LCA1KSk7IC8vIE91dHB1dDogMTUKCgpJbiB0aGlzIGV4YW1wbGUsIHRoZSBzdW0gZnVuY3Rpb24gdXNlcyB0aGUgcmVzdCBzeW50YXggd2l0aCB0aGUgcGFyYW1ldGVyIG51bWJlcnMuIEl0IGFsbG93cyB5b3UgdG8gcGFzcyBhbnkgbnVtYmVyIG9mIGFyZ3VtZW50cywgYW5kIHRoZXkgYXJlIGF1dG9tYXRpY2FsbHkgY29sbGVjdGVkIGludG8gYW4gYXJyYXkgY2FsbGVkIG51bWJlcnMuIFdpdGhpbiB0aGUgZnVuY3Rpb24gYm9keSwgeW91IGNhbiB0aGVuIHdvcmsgd2l0aCB0aGlzIGFycmF5IGFzIG5lZWRlZC4KCk9uIHRoZSBvdGhlciBoYW5kLCBzcHJlYWQgc3ludGF4IG9yIHNwcmVhZCBvcGVyYXRvciAoYWxzbyBkZW5vdGVkIGJ5IHRocmVlIGRvdHMgLi4uKSBpcyB1c2VkIHRvIGV4cGFuZCBhbiBhcnJheSBvciBpdGVyYWJsZSBvYmplY3QgaW50byBpbmRpdmlkdWFsIGVsZW1lbnRzLiBJdCBjYW4gYmUgdXNlZCBpbiBmdW5jdGlvbiBjYWxscywgYXJyYXkgbGl0ZXJhbHMsIG9yIG9iamVjdCBsaXRlcmFscyB0byBzcHJlYWQgdGhlIGVsZW1lbnRzIG9yIHByb3BlcnRpZXMuCgpIZXJlJ3MgYW4gZXhhbXBsZSBvZiB0aGUgc3ByZWFkIHN5bnRheDoKCmNvbnN0IG51bWJlcnMgPSBbMSwgMiwgMywgNCwgNV07CmNvbnNvbGUubG9nKC4uLm51bWJlcnMpOyAvLyBPdXRwdXQ6IDEgMiAzIDQgNQoKY29uc3QgYXJyYXkxID0gWzEsIDIsIDNdOwpjb25zdCBhcnJheTIgPSBbNCwgNSwgNl07CmNvbnN0IGNvbWJpbmVkQXJyYXkgPSBbLi4uYXJyYXkxLCAuLi5hcnJheTJdOwpjb25zb2xlLmxvZyhjb21iaW5lZEFycmF5KTsgLy8gT3V0cHV0OiBbMSwgMiwgMywgNCwgNSwgNl0KCgpJbiB0aGUgZmlyc3QgZXhhbXBsZSwgdGhlIHNwcmVhZCBzeW50YXggaXMgdXNlZCB3aXRoaW4gdGhlIGNvbnNvbGUubG9nKCkgc3RhdGVtZW50IHRvIGV4cGFuZCB0aGUgbnVtYmVycyBhcnJheSBpbnRvIGluZGl2aWR1YWwgZWxlbWVudHMuIFRoaXMgcmVzdWx0cyBpbiBlYWNoIGVsZW1lbnQgYmVpbmcgcHJpbnRlZCBzZXBhcmF0ZWx5LgoKSW4gdGhlIHNlY29uZCBleGFtcGxlLCB0aGUgc3ByZWFkIHN5bnRheCBpcyB1c2VkIHRvIGNvbWJpbmUgdGhlIGVsZW1lbnRzIG9mIGFycmF5MSBhbmQgYXJyYXkyIGludG8gYSBuZXcgYXJyYXkgY2FsbGVkIGNvbWJpbmVkQXJyYXkuIFRoZSByZXN1bHRpbmcgYXJyYXkgY29udGFpbnMgYWxsIHRoZSBlbGVtZW50cyBmcm9tIGFycmF5MSBmb2xsb3dlZCBieSBhbGwgdGhlIGVsZW1lbnRzIGZyb20gYXJyYXkyLgoKVG8gc3VtbWFyaXplLCB0aGUgcmVzdCBzeW50YXggaXMgdXNlZCB3aXRoaW4gYSBmdW5jdGlvbiBkZWNsYXJhdGlvbiB0byBjb2xsZWN0IG11bHRpcGxlIGFyZ3VtZW50cyBpbnRvIGFuIGFycmF5LCB3aGlsZSB0aGUgc3ByZWFkIHN5bnRheCBpcyB1c2VkIHRvIHNwcmVhZCBhbiBhcnJheSBvciBpdGVyYWJsZSBvYmplY3QgaW50byBpbmRpdmlkdWFsIHBpdm90IGVsZW1lbnRzIG9yIGNvbWJpbmUgbXVsdGlwbGUgZm9ybSBvZiBhcnJheXMvb2JqZWN0cyBpbnRvIGEgbmV3IGFycmF5L29iamVjdC4KCjYyLiBXaGF0IGlzIGEgTmVzdGVkIEZ1bmN0aW9uPwoKQSBuZXN0ZWQgZnVuY3Rpb24sIGFsc28ga25vd24gYXMgYW4gaW5uZXIgZnVuY3Rpb24sIGlzIGEgZnVuY3Rpb24gZGVmaW5lZCB3aXRoaW4gYW5vdGhlciBmdW5jdGlvbi4gSW4gcHJvZ3JhbW1pbmcgbGFuZ3VhZ2VzIHRoYXQgc3VwcG9ydCBuZXN0ZWQgZnVuY3Rpb25zLCB0aGUgaW5uZXIgZnVuY3Rpb24gaXMgZW5jbG9zZWQgd2l0aGluIHRoZSBib2R5IG9mIHRoZSBvdXRlciBmdW5jdGlvbi4gVGhpcyBtZWFucyB0aGF0IHRoZSBpbm5lciBmdW5jdGlvbiBjYW4gb25seSBiZSBhY2Nlc3NlZCBhbmQgY2FsbGVkIGZyb20gd2l0aGluIHRoZSBzY29wZSBvZiB0aGUgb3V0ZXIgZnVuY3Rpb24uCgpIZXJlJ3MgYW4gZXhhbXBsZSBpbiBQeXRob24gdG8gaWxsdXN0cmF0ZSB0aGUgY29uY2VwdDoKCmZ1bmN0aW9uIG91dGVyRnVuY3Rpb24oKSB7CmNvbnNvbGUubG9nKCJUaGlzIGlzIHRoZSBvdXRlciBmdW5jdGlvbi4iKTsKCmZ1bmN0aW9uIGlubmVyRnVuY3Rpb24oKSB7CmNvbnNvbGUubG9nKCJUaGlzIGlzIHRoZSBpbm5lciBmdW5jdGlvbi4iKTsKfQoKLy8gQ2FsbGluZyB0aGUgaW5uZXIgZnVuY3Rpb24gZnJvbSB3aXRoaW4gdGhlIG91dGVyIGZ1bmN0aW9uCmlubmVyRnVuY3Rpb24oKTsKfQoKLy8gQ2FsbGluZyB0aGUgb3V0ZXIgZnVuY3Rpb24Kb3V0ZXJGdW5jdGlvbigpOwoKCkluIHRoaXMgSmF2YVNjcmlwdCBleGFtcGxlLCBpbm5lckZ1bmN0aW9uKCkgaXMgZGVmaW5lZCB3aXRoaW4gb3V0ZXJGdW5jdGlvbigpLiBUaGUgaW5uZXIgZnVuY3Rpb24gY2FuIGFjY2VzcyB2YXJpYWJsZXMgYW5kIHBhcmFtZXRlcnMgb2YgdGhlIG91dGVyIGZ1bmN0aW9uLCBhbmQgaXQgY2FuIGFsc28gaGF2ZSBpdHMgb3duIGxvY2FsIHZhcmlhYmxlcy4gSG93ZXZlciwgaXQgY2Fubm90IGJlIGRpcmVjdGx5IGNhbGxlZCBmcm9tIG91dHNpZGUgdGhlIHNjb3BlIG9mIHRoZSBvdXRlciBmdW5jdGlvbi4KCk5lc3RlZCBmdW5jdGlvbnMgaW4gSmF2YVNjcmlwdCBvZmZlciBzaW1pbGFyIGJlbmVmaXRzIGFzIGluIG90aGVyIHByb2dyYW1taW5nIGxhbmd1YWdlcywgYWxsb3dpbmcgZm9yIGNvZGUgb3JnYW5pemF0aW9uLCBpbXByb3ZlZCByZWFkYWJpbGl0eSwgYW5kIGNvbnRyb2xsZWQgYWNjZXNzIHRvIGZ1bmN0aW9ucy4KCgoKNjMuIFdoYXQgYXJlIGVzY2FwZSBjaGFyYWN0ZXJzIGluIGphdmFzY3JpcHQ/wqAKCkVzY2FwZSBjaGFyYWN0ZXJzIGluIEphdmFTY3JpcHQgYXJlIHNwZWNpYWwgY2hhcmFjdGVycyB0aGF0IGFyZSB1c2VkIHRvIHJlcHJlc2VudCBjZXJ0YWluIG5vbi1wcmludGFibGUgb3IgcmVzZXJ2ZWQgY2hhcmFjdGVycyB3aXRoaW4gYSBzdHJpbmcuIFRoZXkgYXJlIGRlbm90ZWQgYnkgYSBiYWNrc2xhc2ggKFwpIGZvbGxvd2VkIGJ5IGEgc3BlY2lmaWMgY2hhcmFjdGVyIG9yIHNlcXVlbmNlIG9mIGNoYXJhY3RlcnMuIFdoZW4gZW5jb3VudGVyZWQgaW4gYSBzdHJpbmcsIGVzY2FwZSBjaGFyYWN0ZXJzIG1vZGlmeSB0aGUgaW50ZXJwcmV0YXRpb24gb3IgYmVoYXZpb3Igb2YgdGhlIGZvbGxvd2luZyBjaGFyYWN0ZXIuCgpIZXJlIGFyZSBzb21lIGNvbW1vbmx5IHVzZWQgZXNjYXBlIGNoYXJhY3RlcnMgaW4gSmF2YVNjcmlwdDoKClxuOiBOZXdsaW5lIGNoYXJhY3Rlci4gSW5zZXJ0cyBhIGxpbmUgYnJlYWsuClx0OiBUYWIgY2hhcmFjdGVyLiBJbnNlcnRzIGEgaG9yaXpvbnRhbCB0YWIuClxyOiBDYXJyaWFnZSByZXR1cm4gY2hhcmFjdGVyLiBNb3ZlcyB0aGUgY3Vyc29yIHRvIHRoZSBiZWdpbm5pbmcgb2YgdGhlIGxpbmUuCioqJzogU2luZ2xlIHF1b3RlIGNoYXJhY3Rlci4gVXNlZCB0byBlc2NhcGUgc2luZ2xlIHF1b3RlcyB3aXRoaW4gYSBzdHJpbmcuCioqIjogRG91YmxlIHF1b3RlIGNoYXJhY3Rlci4gVXNlZCB0byBlc2NhcGUgZG91YmxlIHF1b3RlcyB3aXRoaW4gYSBzdHJpbmcuClw6IEJhY2tzbGFzaCBjaGFyYWN0ZXIuIFVzZWQgdG8gZXNjYXBlIGEgbGl0ZXJhbCBiYWNrc2xhc2guClx1WFhYWDogVW5pY29kZSBlc2NhcGUgc2VxdWVuY2UuIFJlcHJlc2VudHMgYSBVbmljb2RlIGNoYXJhY3RlciBiYXNlZCBvbiBpdHMgaGV4YWRlY2ltYWwgY29kZSAoWFhYWCkuCgoKNjQuIEV4cGxhaW4gSGlnaGVyIE9yZGVyIEZ1bmN0aW9ucz8KCkluIEphdmFTY3JpcHQsIGhpZ2hlci1vcmRlciBmdW5jdGlvbnMgYXJlIGZ1bmN0aW9ucyB0aGF0IGNhbiBhY2NlcHQgb3RoZXIgZnVuY3Rpb25zIGFzIGFyZ3VtZW50cyBhbmQvb3IgcmV0dXJuIGZ1bmN0aW9ucyBhcyB0aGVpciByZXN1bHRzLiBFc3NlbnRpYWxseSwgYSBoaWdoZXItb3JkZXIgZnVuY3Rpb24gdHJlYXRzIGZ1bmN0aW9ucyBhcyBmaXJzdC1jbGFzcyBjaXRpemVucywgYWxsb3dpbmcgdGhlbSB0byBiZSBtYW5pcHVsYXRlZCBhbmQgb3BlcmF0ZWQgdXBvbiBqdXN0IGxpa2UgYW55IG90aGVyIHZhbHVlLgoKVGhlcmUgYXJlIHR3byBtYWluIHdheXMgaW4gd2hpY2ggaGlnaGVyLW9yZGVyIGZ1bmN0aW9ucyBhcmUgdXNlZDoKCkFjY2VwdGluZyBGdW5jdGlvbnMgYXMgQXJndW1lbnRzOiBBIGhpZ2hlci1vcmRlciBmdW5jdGlvbiBjYW4gdGFrZSBvbmUgb3IgbW9yZSBmdW5jdGlvbnMgYXMgYXJndW1lbnRzLiBUaGlzIGFsbG93cyBmb3IgZmxleGliaWxpdHkgYW5kIGVuYWJsZXMgdGhlIGhpZ2hlci1vcmRlciBmdW5jdGlvbiB0byBwZXJmb3JtIG9wZXJhdGlvbnMgYmFzZWQgb24gdGhlIHByb3ZpZGVkIGZ1bmN0aW9ucy4gVGhlIGZ1bmN0aW9uIGRlZmluaXRpb24gYXJndW1lbnRzIGNhbiBiZSBpbnZva2VkIHdpdGhpbiB0aGUgaGlnaGVyLW9yZGVyIGZ1bmN0aW9uIG9yIHVzZWQgdG8gbW9kaWZ5IGl0cyBiZWhhdmlvci4KUmV0dXJuaW5nIEZ1bmN0aW9uczogQSBoaWdoZXItb3JkZXIgZnVuY3Rpb24gY2FuIGFsc28gZ2VuZXJhdGUgYW5kIHJldHVybiBhIG5ldyBmdW5jdGlvbi4gVGhpcyBlbmFibGVzIHRoZSBjcmVhdGlvbiBvZiBzcGVjaWFsaXplZCBvciBwYXJ0aWFsbHkgYXBwbGllZCBmdW5jdGlvbnMgYmFzZWQgb24gdGhlIHByb3ZpZGVkIGFyZ3VtZW50cyBvciBjb25kaXRpb25zLgo2NS4gV2hhdCBpcyBhIHByb3RvdHlwZSBwcm9wZXJ0eT8KCkluIEphdmFTY3JpcHQsIHRoZSBwcm90b3R5cGUgcHJvcGVydHkgaXMgYSBwcm9wZXJ0eSBvZiBjb25zdHJ1Y3RvciBmdW5jdGlvbnMgdGhhdCBhbGxvd3MgcHJlZGVmaW5lZCBvYmplY3RzIGNyZWF0ZWQgZnJvbSB0aG9zZSBjb25zdHJ1Y3RvcnMgdG8gaW5oZXJpdCBwcm9wZXJ0aWVzIGFuZCBtZXRob2RzLiBFdmVyeSBKYXZhU2NyaXB0IG9iamVjdCBoYXMgYSBwcm90b3R5cGUgcHJvcGVydHksIHdoaWNoIHJlZmVyZW5jZXMgdGhlIHByb3RvdHlwZSBvYmplY3QgYXNzb2NpYXRlZCB3aXRoIGl0cyBjb25zdHJ1Y3RvciBmdW5jdGlvbi4KClRoZSBwcm90b3R5cGUgb2JqZWN0IGlzIGVzc2VudGlhbGx5IGEgYmx1ZXByaW50IG9yIHRlbXBsYXRlIGZvciBjcmVhdGluZyBvdGhlciBwcmVkZWZpbmVkIG9iamVjdHMuIEl0IGNvbnRhaW5zIHByb3BlcnRpZXMgYW5kIG1ldGhvZHMgdGhhdCBhcmUgc2hhcmVkIGFtb25nIGFsbCBpbnN0YW5jZXMgY3JlYXRlZCBmcm9tIHRoZSBjb25zdHJ1Y3RvciBmdW5jdGlvbi4gV2hlbiBhIHByb3BlcnR5IG9yIG1ldGhvZCBpcyBhY2Nlc3NlZCBvbiBhbiBvYmplY3QsIEphdmFTY3JpcHQgZmlyc3QgY2hlY2tzIGlmIHRoZSBvYmplY3QgaXRzZWxmIGhhcyB0aGF0IHByb3BlcnR5IG9yIG1ldGhvZC4gSWYgbm90IGZvdW5kLCBpdCBsb29rcyBmb3IgaXQgaW4gdGhlIHByb3RvdHlwZSBvYmplY3QuCgrCoDY2LiBXaGF0IGRvZXMgc3ludGF4IGVycm9yIG1lYW4gaW4gSmF2YVNjcmlwdD8KCkluIEphdmFTY3JpcHQsIGEgc3ludGF4IGVycm9yIG9jY3VycyB3aGVuIHRoZSBKYXZhU2NyaXB0IGVuZ2luZSBlbmNvdW50ZXJzIGNvZGUgdGhhdCBkb2VzIG5vdCBjb25mb3JtIHRvIHRoZSBzeW50YXggcnVsZXMgb2YgdGhlIGxhbmd1YWdlLiBJdCBtZWFucyB0aGF0IHRoZSBjb2RlIGlzIG5vdCB3cml0dGVuIGNvcnJlY3RseSBhY2NvcmRpbmcgdG8gdGhlIGdyYW1tYXIgYW5kIHN0cnVjdHVyZSBkZWZpbmVkIGJ5IEphdmFTY3JpcHQuCgpXaGVuIGEgc3ludGF4IGVycm9yIGNvbmRpdGlvbiBpcyBlbmNvdW50ZXJlZCwgdGhlIEphdmFTY3JpcHQgZW5naW5lIGlzIHVuYWJsZSB0byBpbnRlcnByZXQgYW5kIGV4ZWN1dGUgdGhlIGNvZGUgYmVjYXVzZSBpdCBjYW5ub3QgdW5kZXJzdGFuZCB0aGUgaW50ZW5kZWQgbWVhbmluZyBkdWUgdG8gdGhlIHZpb2xhdGlvbiBvZiBzeW50YXggcnVsZXMuIEFzIGEgcmVzdWx0LCB0aGUgSmF2YVNjcmlwdCBlbmdpbmUgdGhyb3dzIGEgc3ludGF4IGVycm9yIGFuZCB0eXBpY2FsbHkgcHJvdmlkZXMgYW4gZXJyb3IgbWVzc2FnZSB0aGF0IGluZGljYXRlcyB0aGUgc3BlY2lmaWMgaXNzdWUgaXQgZW5jb3VudGVyZWQuCgoKCjY3LiBFeHBsYWluIGdlbmVyYXRvciBmdW5jdGlvbnMuCgpHZW5lcmF0b3IgZnVuY3Rpb25zIGFyZSBhIHNwZWNpYWwgdHlwZSBvZiBmdW5jdGlvbiBpbiBKYXZhU2NyaXB0IHRoYXQgY2FuIGJlIHBhdXNlZCBhbmQgcmVzdW1lZCBkdXJpbmcgZXhlY3V0aW9uLiBUaGV5IGFsbG93IGZvciB0aGUgZ2VuZXJhdGlvbiBvZiBhIHNlcXVlbmNlIG9mIHZhbHVlcyBvdmVyIGV4cGlyYXRpb24gdGltZSwgcHJvdmlkaW5nIGEgbW9yZSBmbGV4aWJsZSBhbmQgZWZmaWNpZW50IHdheSB0byBpdGVyYXRlIHRocm91Z2ggZGF0YSBjb21wYXJlZCB0byB0cmFkaXRpb25hbCBmdW5jdGlvbnMuCgpUbyBkZWZpbmUgYSBnZW5lcmF0b3IgZnVuY3Rpb24sIHlvdSB1c2UgdGhlIGZ1bmN0aW9uKiBzeW50YXg6CgpmdW5jdGlvbiogbXlHZW5lcmF0b3IoKSB7Ci8vIEdlbmVyYXRvciBmdW5jdGlvbiBib2R5CnlpZWxkIHZhbHVlMTsKeWllbGQgdmFsdWUyOwovLyAuLi4KfQoKClRoZSBmdW5jdGlvbioga2V5d29yZCBkZW5vdGVzIHRoYXQgdGhlIGZ1bmN0aW9uIGlzIGEgZ2VuZXJhdG9yIGZ1bmN0aW9uLiBJbnNpZGUgdGhlIGdlbmVyYXRvciBmdW5jdGlvbiwgdGhlIHlpZWxkIGtleXdvcmQgaXMgdXNlZCB0byBwYXVzZSB0aGUgZXhlY3V0aW9uIGFuZCBwcm9kdWNlIGEgdmFsdWUuIFRoZSBnZW5lcmF0b3IgZnVuY3Rpb24gY2FuIHRoZW4gYmUgaXRlcmF0ZWQgb3ZlciB1c2luZyBhIGZvci4uLm9mIGxvb3Agb3IgYnkgbWFudWFsbHkgY2FsbGluZyB0aGUgbmV4dCgpIG1ldGhvZCBvbiB0aGUgZ2VuZXJhdG9yIG9iamVjdC4KCldoZW4gYSBnZW5lcmF0b3IgZnVuY3Rpb24gaXMgaW52b2tlZCwgaXQgcmV0dXJucyBhIGdlbmVyYXRvciBvYmplY3QsIHdoaWNoIHNlcnZlcyBhcyBhbiBpdGVyYXRvci4gVGhlIGdlbmVyYXRvciBvYmplY3QgaGFzIGEgbmV4dCgpIG1ldGhvZCB0aGF0LCB3aGVuIGNhbGxlZCwgcmVzdW1lcyB0aGUgZXhlY3V0aW9uIG9mIHRoZSBzaW1wbGUgZnVuY3Rpb24gdW50aWwgdGhlIG5leHQgeWllbGQgc3RhdGVtZW50IGlzIGVuY291bnRlcmVkLiBUaGUgbmV4dCgpIG1ldGhvZCByZXR1cm5zIGFuIG9iamVjdCB3aXRoIHR3byBwcm9wZXJ0aWVzOiB2YWx1ZSAodGhlIHlpZWxkZWQgdmFsdWUpIGFuZCBkb25lIChhIGJvb2xlYW4gaW5kaWNhdGluZyB3aGV0aGVyIHRoZSBnZW5lcmF0b3IgZnVuY3Rpb24gaGFzIGZpbmlzaGVkKS4KCgoKNjguIFdoYXQgaXMgYSBzZWxmLWludm9raW5nIGZ1bmN0aW9uPwoKQSBzZWxmLWludm9raW5nIGZ1bmN0aW9uLCBhbHNvIGtub3duIGFzIGFuIEltbWVkaWF0ZWx5IEludm9rZWQgRnVuY3Rpb24gRXhwcmVzc2lvbiAoSUlGRSksIGlzIGEgSmF2YVNjcmlwdCBmdW5jdGlvbiB0aGF0IGlzIGV4ZWN1dGVkIGltbWVkaWF0ZWx5IGFmdGVyIGl0IGlzIGRlZmluZWQuIEl0IGlzIGEgd2F5IHRvIGVuY2Fwc3VsYXRlIHN0YXRlbWVudCBjb2RlIGFuZCBjcmVhdGUgYSBuZXcgc2NvcGUgd2l0aG91dCBwb2xsdXRpbmcgdGhlIGdsb2JhbCBzY29wZS4KClRoZSBzeW50YXgoIGNvZGUgc25pcHBldCkgZm9yIGEgc2VsZi1pbnZva2luZyBmdW5jdGlvbiBpcyBhcyBmb2xsb3dzOgoKKGZ1bmN0aW9uKCkgewovLyBOb3JtYWwgRnVuY3Rpb24gYm9keSBvciBib2R5IGVsZW1lbnQKfSkoKTsKCgoKCjY5LiBXaGF0IGFyZSB0aGUgdHlwZXMgb2Ygb3BlcmF0b3JzIGluIEphdmFTY3JpcHQ/CgpJbiBKYXZhU2NyaXB0LCBvcGVyYXRvcnMgYXJlIHN5bWJvbHMgb3Iga2V5d29yZHMgdGhhdCBwZXJmb3JtIG9wZXJhdGlvbnMgb24gb3BlcmFuZHMgKHZhbHVlcyBvciB2YXJpYWJsZXMpLiBUaGV5IGFsbG93IHlvdSB0byBwZXJmb3JtIG1hdGhlbWF0aWNhbCBjYWxjdWxhdGlvbnMsIGNvbXBhcmUgdmFsdWVzLCBhc3NpZ24gdmFsdWVzLCBhbmQgbW9yZS4gSmF2YVNjcmlwdCBoYXMgc2V2ZXJhbCB0eXBlcyBvZiBvcGVyYXRvcnMsIGluY2x1ZGluZzoKCkFyaXRobWV0aWMgT3BlcmF0b3JzOiBUaGVzZSBvcGVyYXRvcnMgcGVyZm9ybSBtYXRoZW1hdGljYWwgY2FsY3VsYXRpb25zIG9uIG51bWVyaWMgb3BlcmFuZHMuCgpBZGRpdGlvbiAoKykKU3VidHJhY3Rpb24gKC0pCk11bHRpcGxpY2F0aW9uICgqKQpEaXZpc2lvbiAoLykKTW9kdWx1cyAoJSkKRXhwb25lbnRpYXRpb24gKCoqKQpJbmNyZW1lbnQgKCsrKQpEZWNyZW1lbnQgKC0tKQpDb21tYSBvcGVyYXRvcgoKQXNzaWdubWVudCBPcGVyYXRvcnM6IFRoZXNlIG9wZXJhdG9ycyBhc3NpZ24gdmFsdWVzIHRvIHZhcmlhYmxlcy4KCkFzc2lnbm1lbnQgKD0pCkFkZGl0aW9uIGFzc2lnbm1lbnQgKCs9KQpTdWJ0cmFjdGlvbiBhc3NpZ25tZW50ICgtPSkKTXVsdGlwbGljYXRpb24gYXNzaWdubWVudCAoKj0pCkRpdmlzaW9uIGFzc2lnbm1lbnQgKC89KQpNb2R1bHVzIGFzc2lnbm1lbnQgKCU9KQoKCkNvbXBhcmlzb24gT3BlcmF0b3JzOiBUaGVzZSBvcGVyYXRvcnMgY29tcGFyZSB2YWx1ZXMgYW5kIHJldHVybiBhIEJvb2xlYW4gcmVzdWx0ICh0cnVlIG9yIGZhbHNlKS4KCkVxdWFsIHRvICg9PSkKTm90IGVxdWFsIHRvICghPSkKU3RyaWN0IGVxdWFsIHRvICg9PT0pClN0cmljdCBub3QgZXF1YWwgdG8gKCE9PSkKR3JlYXRlciB0aGFuICg+KQpMZXNzIHRoYW4gKDwpCkdyZWF0ZXIgdGhhbiBvciBlcXVhbCB0byAoPj0pCkxlc3MgdGhhbiBvciBlcXVhbCB0byAoPD0pCgpMb2dpY2FsIE9wZXJhdG9yczogVGhlc2Ugb3BlcmF0b3JzIHBlcmZvcm0gbG9naWNhbCBvcGVyYXRpb25zIG9uIEJvb2xlYW4gb3BlcmFuZHMuCgpMb2dpY2FsIEFORCAoJiYpCkxvZ2ljYWwgT1IgKHx8KQpMb2dpY2FsIE5PVCAoISkKCkNvbmRpdGlvbmFsIChUZXJuYXJ5KSBPcGVyYXRvcjogSXQgaXMgYSBzaG9ydGhhbmQgd2F5IHRvIHdyaXRlIGNvbmRpdGlvbmFsIHN0YXRlbWVudHMuCgpDb25kaXRpb25hbCAoY29uZGl0aW9uID8gdmFsdWUxIDogdmFsdWUyKQoKVW5hcnkgT3BlcmF0b3JzOiBUaGVzZSBvcGVyYXRvcnMgb3BlcmF0ZSBvbiBhIHNpbmdsZSBvcGVyYW5kLgoKVW5hcnkgcGx1cyAoKykKVW5hcnkgbWludXMgKC0pCkxvZ2ljYWwgTk9UICghKQpJbmNyZW1lbnQgKCsrKQpEZWNyZW1lbnQgKC0tKQpUeXBlb2YgKHR5cGVvZikKRGVsZXRlIG9wZXJhdG9yIChkZWxldGUpClZvaWQgKHZvaWQpCgpCaXR3aXNlIE9wZXJhdG9yczogVGhlc2Ugb3BlcmF0b3JzIHBlcmZvcm0gYml0d2lzZSBvcGVyYXRpb25zIG9uIGludGVnZXIgb3BlcmFuZHMuCgpCaXR3aXNlIEFORCAoJikKQml0d2lzZSBPUiAofCkKQml0d2lzZSBYT1IgKF4pCkJpdHdpc2UgTk9UICh+KQpMZWZ0IHNoaWZ0ICg8PCkKUmlnaHQgc2hpZnQgKD4+KQpVbnNpZ25lZCByaWdodCBzaGlmdCAoPj4+KQoKU3RyaW5nIE9wZXJhdG9yczogVGhlc2Ugb3BlcmF0b3JzIGFyZSB1c2VkIHRvIGNvbmNhdGVuYXRlIHN0cmluZ3MuCgpTdHJpbmcgY29uY2F0ZW5hdGlvbiAoKykKClRoZXNlIGFyZSB0aGUgbWFpbiB0eXBlcyBvZiBvcGVyYXRvcnMgaW4gSmF2YVNjcmlwdC4gVW5kZXJzdGFuZGluZyBhbmQgdXRpbGl6aW5nIHRoZXNlIG9wZXJhdG9ycyBpcyBlc3NlbnRpYWwgZm9yIHBlcmZvcm1pbmcgdmFyaW91cyBvcGVyYXRpb25zIGluIEphdmFTY3JpcHQsIHN1Y2ggYXMgbWF0aGVtYXRpY2FsIGNhbGN1bGF0aW9ucywgbG9naWNhbCBldmFsdWF0aW9ucywgdmFyaWFibGUgYXNzaWdubWVudHMsIGFuZCBtb3JlLgoKU3VtbWluZyB1cC4uLgoKSmF2YVNjcmlwdCBpcyBvbmUgb2YgdGhlIG1vc3QgdmVyc2F0aWxlIGxhbmd1YWdlcy7CoCBPbmUgY2FuIGFjZSB0aGUgaW50ZXJ2aWV3IHdpdGggZGV0ZXJtaW5hdGlvbiBhbmQgaGFyZCB3b3JrLsKgIEphdmFTY3JpcHQgaXMgb25lIG9mIHRoZSBoaWdoZXN0LXBheWluZyBqb2JzIGluIHRoZSBpbmR1c3RyeS7CoAoKWW91IG5lZWQgdG8gYnJ1c2ggdXAgb24geW91ciBiYXNpY3MgYW5kIGFkdmFuY2VkIGtub3dsZWRnZSBvZiBqYXZhc2NyaXB0LCB1c2luZyB0aGVzZSBpbnRlcnZpZXcgcXVlc3Rpb25zIGlzIGEgZ3JlYXQgd2F5IHRvIGxhbmQgeW91ciBkcmVhbSBqb2IsIGluIGFueSB0b3AgdGVjaG5pY2FsIGZpcm0uwqAKCkFsbCB0aGUgYmVzdCEKCgoKCllvdSBtaWdodCBhbHNvIGJlIGludGVyZXN0ZWQgaW4gcmVhZGluZzoKCgoKCldoYXQgSXMgT2JqZWN0LU9yaWVudGVkIFByb2dyYW1taW5nPwpEaWZmZXJlbmNlIEJldHdlZW4gU3RydWN0dXJlIEFuZCBDbGFzcyBJbiBDKysKV2hhdCBJcyBQYWdpbmcgSW4gT3BlcmF0aW5nIFN5c3RlbT8KRGlmZmVyZW5jZSBCZXR3ZWVuIEphdmFTY3JpcHQgQW5kIGpRdWVyeQpEaWZmZXJlbmNlIEJldHdlZW4gSGFyZHdhcmUgYW5kIFNvZnR3YXJl
Output:
olleH, dlrow! woH era ?uoy
Q28. How to empty an array in JS?
There are 4 ways in which the array can be emptied➖
Let us take an array for example
The output for all the following techniques mentioned below is
Assigning it to a new empty array➖
bGV0IGE9WzEsMiwzXQphID0gW107CmNvbnNvbGUubG9nKGEpOw==
Assigning the length of the array➖
bGV0IGEgPSBbMSwyLDNdOwphLmxlbmd0aD0wOwpjb25zb2xlLmxvZyhhKTs=
Using the splice() method➖
bGV0IGEgPSBbMSwyLDNdOwphLnNwbGljZSgwLGEubGVuZ3RoKTsKY29uc29sZS5sb2coYSk7
Using pop()➖
bGV0IGEgPSBbMSwyLDNdOwp3aGlsZShhLmxlbmd0aCA+IDApIHsKICAgIGEucG9wKCk7Cn0KY29uc29sZS5sb2coYTs=
Q29. Write a function that performs a binary search on a sorted array.
Here's an example of a function that performs a binary search on a sorted array:
IGZ1bmN0aW9uIGJpbmFyeV9TZWFyY2goaXRlbXMsIHZhbHVlKXsKICAgIHZhciBmaXJzdEluZGV4ICA9IDAsCiAgICAgICAgbGFzdEluZGV4ICAgPSBpdGVtcy5sZW5ndGggLSAxLAogICAgICAgIG1pZGRsZUluZGV4ID0gTWF0aC5mbG9vcigobGFzdEluZGV4ICsgZmlyc3RJbmRleCkvMik7CgogICAgd2hpbGUoaXRlbXNbbWlkZGxlSW5kZXhdICE9IHZhbHVlICYmIGZpcnN0SW5kZXggPCBsYXN0SW5kZXgpCiAgICB7CiAgICAgICBpZiAodmFsdWUgPCBpdGVtc1ttaWRkbGVJbmRleF0pCiAgICAgICAgewogICAgICAgICAgICBsYXN0SW5kZXggPSBtaWRkbGVJbmRleCAtIDE7CiAgICAgICAgfSAKICAgICAgZWxzZSBpZiAodmFsdWUgPiBpdGVtc1ttaWRkbGVJbmRleF0pCiAgICAgICAgewogICAgICAgICAgICBmaXJzdEluZGV4ID0gbWlkZGxlSW5kZXggKyAxOwogICAgICAgIH0KICAgICAgICBtaWRkbGVJbmRleCA9IE1hdGguZmxvb3IoKGxhc3RJbmRleCArIGZpcnN0SW5kZXgpLzIpOwogICAgfQoKIHJldHVybiAoaXRlbXNbbWlkZGxlSW5kZXhdICE9IHZhbHVlKSA/IC0xIDogbWlkZGxlSW5kZXg7Cn0KdmFyIGl0ZW1zID0gWzEsIDIsIDMsIDQsIDUsIDcsIDgsIDldOwpjb25zb2xlLmxvZyhiaW5hcnlfU2VhcmNoKGl0ZW1zLCAxKSk7ICAgCmNvbnNvbGUubG9nKGJpbmFyeV9TZWFyY2goaXRlbXMsIDUpKTsKIA==
Q30. What are the types of Pop up boxes available in JavaScript?
JavaScript provides three types of pop-up boxes or dialog boxes:
-
Alert:
- The method displays a simple dialog box with a message and an OK button.
- It is commonly used to show informational messages or to alert users of certain conditions.
- The dialog box blocks further execution until the user clicks the OK button.
- Example:
-
Confirm:
- The method displays a dialog box with a message, along with OK and Cancel buttons.
- It is used to ask the user for confirmation or to get a yes/no response.
- The function returns a Boolean value ( if the user clicks OK and if the user clicks Cancel).
- Example:
dmFyIHJlc3VsdCA9IGNvbmZpcm0oIkFyZSB5b3Ugc3VyZSB5b3Ugd2FudCB0byBwcm9jZWVkPyIpOwppZiAocmVzdWx0KSB7Ci8vIFVzZXIgY2xpY2tlZCBPSwp9IGVsc2UgewovLyBVc2VyIGNsaWNrZWQgQ2FuY2VsCn0=
-
Prompt:
- The method displays a dialog box with a message, an input field for the user to enter data, and OK/Cancel buttons.
- It is used to get user input or to prompt the user for information.
- The method takes an optional default value as a parameter and returns the value entered by the user as a string, or if the user clicks Cancel.
- Example:
dmFyIG5hbWUgPSBwcm9tcHQoIlBsZWFzZSBlbnRlciB5b3VyIG5hbWU6IiwgIkpvaG4gRG9lIik7CmlmIChuYW1lICE9PSBudWxsKSB7Ci8vIFVzZXIgZW50ZXJlZCBhIHZhbHVlCn0gZWxzZSB7Ci8vIFVzZXIgY2xpY2tlZCBDYW5jZWwKfQ==
Q31. Guess the output of the following code.
ZnVuY3Rpb24gZnVuYzEoKXsgc2V0VGltZW91dCgoKT0+eyBjb25zb2xlLmxvZyh4KTsgY29uc29sZS5sb2coeSk7IH0sMzAwMCk7IHZhciB4ID0gMjsgbGV0IHkgPSAxMjsgfSBmdW5jMSgpOw==
ReferenceError: y is not defined
Explanation:
- The function is defined and invoked.
- Inside , a function is called with a callback arrow function. The callback function is scheduled to execute after a delay of 3000 milliseconds (3 seconds).
- The variable is declared using the keyword and assigned a value of . declarations are hoisted, so the declaration is moved to the top of the function scope. However, the assignment of to happens at the line where it is written.
- The variable is declared using the keyword and assigned a value of . declarations are block-scoped and not hoisted, so the declaration and assignment of happen at the same line.
- When the callback arrow function inside is executed after the delay, it tries to log the values of and .
- The value of is because it was declared using and is accessible within the function scope.
- However, a occurs when trying to log the value of . This is because is declared using and is block-scoped to the function block. It is not accessible inside the callback function since it has its own block scope.
Therefore, the code logs the value of as , but an error occurs when trying to log the value of .
Q32. Guess the output of the following code.
ZnVuY3Rpb24gZnVuYzIoKXsgZm9yKHZhciBpID0gMDsgaSA8IDM7IGkrKyl7IHNldFRpbWVvdXQoKCk9PiBjb25zb2xlLmxvZyhpKSwyMDAwKTsgfSB9IGZ1bmMyKCk7
3
3
3
Explanation:
- The function is defined and invoked.
- Inside , a loop is used to iterate from to (3 iterations).
- For each iteration of the loop, a function is called with a callback arrow function. The callback function is scheduled to execute after a delay of 2000 milliseconds (2 seconds).
- The callback function logs the value of .
- Since the function is asynchronous, the loop continues executing without waiting for the callback functions to execute.
- After the loop finishes, the value of is because the loop condition () becomes false, and the loop terminates.
- As a result, when the callback functions execute after the delay, they access and log the final value of , which is .
- Therefore, the code logs three times, indicating the value of at the time the callback functions execute.
Q33. Guess the output of the following code.
KGZ1bmN0aW9uKCl7IHNldFRpbWVvdXQoKCk9PiBjb25zb2xlLmxvZygxKSwyMDAwKTsgY29uc29sZS5sb2coMik7IHNldFRpbWVvdXQoKCk9PiBjb25zb2xlLmxvZygzKSwwKTsgY29uc29sZS5sb2coNCk7IH0pKCk7
2
4
3
1
Explanation:
- The code defines an immediately invoked function expression (IIFE) using . This allows the code inside the function to be executed immediately.
- Inside the IIFE, the following actions occur:
- The first function is called with a callback arrow function, which logs , and a delay of 2000 milliseconds (2 seconds). This means it will execute after a 2-second delay.
- The value is logged to the console immediately.
- The second function is called with a callback arrow function, which logs , and a delay of 0 milliseconds. Despite the delay being set to 0, it is effectively a minimum delay, allowing other tasks in the event loop to be processed before the callback is executed.
- The value is logged to the console immediately.
- Since the second function has a delay of 0, it is placed in the event queue and waits for the call stack to clear before executing its callback.
- After the IIFE finishes executing, the event loop processes the callback functions waiting in the event queue.
- The callback function from the second (with a delay of 0) is processed first and logs .
- Lastly, after a 2-second delay, the callback function from the first executes and logs .
Q34. Guess the output of the following code.
ZnVuY3Rpb24gcnVuRnVuYygpeyBjb25zb2xlLmxvZygiMSIgKyAxKTsgY29uc29sZS5sb2coIkEiIC0gMSk7IGNvbnNvbGUubG9nKDIgKyAiLTIiICsgIjIiKTsgY29uc29sZS5sb2coIkhlbGxvIiAtICJXb3JsZCIgKyA3OCk7IGNvbnNvbGUubG9nKCJIZWxsbyIrICI3OCIpOyB9IHJ1bkZ1bmMoKTs=
11
NaN
2-22
NaN78
Hello78
Explanation:
- The function is defined and invoked.
- Inside , several statements are used to print different expressions.
- Let's break down the output line by line:
-
- The operator performs concatenation when one or both operands are strings. In this case, is a string, and is a number. The number is coerced into a string and concatenated with , resulting in the string .
-
- The operator is not defined for string subtraction. JavaScript tries to perform arithmetic operations on the operands. Since cannot be converted into a number, the operation results in (Not-a-Number).
-
- The operator, when used with strings, performs concatenation. The first operation is , which concatenates the number with the string , resulting in . The subsequent concatenation with the string results in .
-
- The operator is not defined for string subtraction. Both and cannot be converted into numbers, resulting in . When is involved in any arithmetic operation, the result is always . The subsequent addition of with still results in .
-
- Both operands are strings, so the operator performs concatenation. It concatenates the string with the string , resulting in the string .
Q35. In which location are cookies stored on the hard disk?
Cookies are not stored directly on the hard disk of a user's device. Instead, they are stored by web browsers in a designated storage location specific to each browser. The actual storage location may vary depending on the operating system and browser being used. Here are some common storage locations for cookies:
-
Windows:
- Google Chrome:
- Mozilla Firefox:
- Microsoft Edge:
-
macOS:
- Google Chrome:
- Mozilla Firefox:
- Safari:
-
Linux:
- Google Chrome:
- Mozilla Firefox:
Please note that the actual paths may differ based on the version of the browser, user profile, and browser settings. The paths provided here are general examples, and it's always recommended to consult the browser's documentation or settings for the precise location of cookie storage.
Q36. What is a prompt box?
Prompt Box is a popup box available in JavaScript.
A prompt box is often used if you want the user to input a value before entering a page.
When a prompt box pops up, the user will have to click either "OK" or "Cancel" to proceed after entering an input value.
If the user clicks "OK" the box returns the input value. If the user clicks "Cancel" the box returns null.
Syntax➖ windows.prompt(”sometxt”,”defaulttxt”);
Example:
ZnVuY3Rpb24gcnVuRnVuYygpeyBjb25zb2xlLmxvZygiMSIgKyAxKTsgY29uc29sZS5sb2coIkEiIC0gMSk7IGNvbnNvbGUubG9nKDIgKyAiLTIiICsgIjIiKTsgY29uc29sZS5sb2coIkhlbGxvIiAtICJXb3JsZCIgKyA3OCk7IGNvbnNvbGUubG9nKCJIZWxsbyIrICI3OCIpOyB9IHJ1bkZ1bmMoKTs=
Q38. Which symbol is used for comments in JavaScript?
In JavaScript, there are two symbols commonly used for comments:
-
Double Slash (//):
- The double slash is used for single-line comments.
- Anything after on the same line is considered a comment and is ignored by the JavaScript interpreter.
-
Slash Asterisk (/* */):
- The slash asterisk is used for multi-line comments or block comments.
- Anything between and is considered a comment and is ignored by the JavaScript interpreter, even if it spans multiple lines.
Q39. What is the difference between view state and session state?
View state and session state are both concepts related to managing state in web applications, but they serve different purposes and are used in different contexts:
View State | Session State |
View state is specific to ASP.NET web forms and is used to maintain the state of a web form across postbacks. |
Session state is a server-side mechanism in web development that allows storing and retrieving user-specific data across multiple requests and sessions. |
It is an encoded representation of the state of the controls on a web form, including their values and properties. |
It is used to store data that needs to be persisted and accessed across different pages or interactions during a user session. |
View state is stored as a hidden field in the HTML form and is sent back and forth between the server and the client with each request and response. |
Session state data is stored on the server, usually in memory or a database, and is associated with a unique session identifier (usually stored in a cookie or URL parameter). |
It allows the web form to remember user input and maintain its state during postbacks, enabling the form to restore its data and appearance. |
Session state can store any serializable object or data, such as user preferences, shopping cart contents, or user authentication information. |
View state is primarily used for maintaining state within a single user session and is tied to the individual web form. |
The session state is independent of a specific web form and can be accessed and modified from multiple pages within the same session. |
In summary, view state is specific to ASP.NET web forms and is used to maintain the state of a single web form across postbacks, while session state is a more general mechanism for storing and accessing user-specific data across multiple requests and sessions.
Q40. How can you submit a form using JavaScript?
To submit a form using JavaScript, you can use the method, which is available on the form element. This method allows you to programmatically trigger the submission of a form.
Here's an example of how you can submit a form using JavaScript:
PGZvcm0gYWN0aW9uPSIvc3VibWl0IiBtZXRob2Q9IlBPU1QiPgo8IS0tIGZvcm0gZmllbGRzIC0tPgo8aW5wdXQgdHlwZT0idGV4dCIgbmFtZT0idXNlcm5hbWUiPgo8aW5wdXQgdHlwZT0icGFzc3dvcmQiIG5hbWU9InBhc3N3b3JkIj4KPGlucHV0IHR5cGU9InN1Ym1pdCIgdmFsdWU9IlN1Ym1pdCI+CjwvZm9ybT4KCjxzY3JpcHQ+Ci8vIEdldCBhIHJlZmVyZW5jZSB0byB0aGUgZm9ybSBlbGVtZW50CmNvbnN0IGZvcm0gPSBkb2N1bWVudC5nZXRFbGVtZW50QnlJZCgnbXlGb3JtJyk7CgovLyBTdWJtaXQgdGhlIGZvcm0gcHJvZ3JhbW1hdGljYWxseQpmb3JtLnN1Ym1pdCgpOwo8L3NjcmlwdD4=
In this example, we have an HTML form with the attribute set to . We then use JavaScript to get a reference to the form element using . Once we have the reference, we can call the method on the form element to trigger the submission.
By calling , the browser will initiate the form submission process, sending the form data to the specified URL using the specified HTTP method ( attribute). The behavior after form submission will depend on the server-side implementation and the content of the URL.
You can trigger the form submission using various events or conditions in your JavaScript code, such as clicking a button, validating form data, or programmatically based on certain conditions.
Q41. What do you mean by NULL in JavaScript?
In JavaScript, is a special value that represents the intentional absence of any object value. It is a primitive value that indicates the absence of a value or the absence of an object reference.
Here are some key points about in JavaScript:
-
is a data type: is a primitive data type in JavaScript, specifically representing the absence of an object value.
-
It is falsy: is considered a falsy value in JavaScript. When evaluated in a Boolean context (e.g., in an statement or as a condition), it is treated as .
-
It is not an object: Despite being a falsy value, is not an object. It is a primitive value. It is distinct from the value, which represents an uninitialized or missing value.
-
Type of is "object": Interestingly, the type of operator in JavaScript returns "object" when applied to . This is a historical quirk in JavaScript and not a true reflection of being an object. It is considered a mistake in the language's design.
Here's an example illustrating the use of :
ZnVuY3Rpb24gZ2V0Vm93ZWxzKHN0cikgewogIGNvbnN0IG0gPSBzdHIubWF0Y2goL1thZWlvdV0vZ2kpOwogIGlmIChtID09PSBudWxsKSB7CiAgICByZXR1cm4gMDsKICB9CiAgcmV0dXJuIG0ubGVuZ3RoOwp9Cgpjb25zb2xlLmxvZyhnZXRWb3dlbHMoJ3NreScpKTsKLy8gZXhwZWN0ZWQgb3V0cHV0OiAw
Q42. What is the strict mode in JavaScript and how can it be enabled?
Strict mode is a feature introduced in ECMAScript 5 (ES5) that enables a stricter set of rules for JavaScript code. It helps in avoiding common mistakes, promotes good coding practices, and makes JavaScript code more robust.
To enable strict mode in JavaScript, you need to add the directive at the beginning of a script or a function. There are two ways to enable strict mode:
- Script-wide strict mode: Add the directive at the beginning of a script file. When added at the top of a JavaScript file, strict mode applies to the entire file.
"use strict";
// Rest of your JavaScript code
- Function-level strict mode: Add the directive at the beginning of a function. When added inside a function, strict mode applies only to that specific function.
function myFunction() {
"use strict";// Function-level strict mode applies only to this function
// Rest of your JavaScript code
}
Enabling strict mode introduces the following changes and restrictions to the JavaScript code:
- Variables must be declared before use. Assigning a value to an undeclared variable will throw an error.
- Assigning a value to a read-only global variable or a non-writable property throws an error.
- Deleting variables, functions, or function arguments is not allowed.
- The value in functions is undefined in strict mode when the function is called without an explicit value.
- Octal literals (e.g., ) are not allowed.
- Duplicate parameter names in function declarations throw an error.
- The function creates a new outer scope and does not have access to the containing scope's variables.
- Several potential sources of errors, like using reserved keywords as variable names, are restricted.
By enabling strict mode, you can catch and prevent certain types of errors( type correction) and enforce better coding practices. It is recommended to use strict mode in all JavaScript code to improve code quality and maintainability.
Q43. What is the difference between .call() and .apply()?
Both the and methods in JavaScript are used to invoke a function with a specified value and arguments. They allow you to execute a function in the context of a particular object and pass arguments to the function. The main difference between and lies in how the arguments are passed to the function.
The method:
- Accepts the value as the first argument, followed by the function arguments as individual arguments.
- The arguments are passed explicitly and separated by commas.
Example using :
ZnVuY3Rpb24gZ3JlZXQobmFtZSkgewpjb25zb2xlLmxvZyhgSGVsbG8sICR7bmFtZX0hYCk7Cn0KCmdyZWV0LmNhbGwobnVsbCwgJ0pvaG4nKTsgLy8gT3V0cHV0OiBIZWxsbywgSm9obiE=
The method:
- Accepts the value as the first argument, followed by an array-like or iterable object containing the function arguments.
- The arguments are passed as an array or an array-like object.
Example using :
ZnVuY3Rpb24gZ3JlZXQobmFtZSkgewpjb25zb2xlLmxvZyhgSGVsbG8sICR7bmFtZX0hYCk7Cn0KCmdyZWV0LmFwcGx5KG51bGwsIFsnSm9obiddKTsgLy8gT3V0cHV0OiBIZWxsbywgSm9obiE=
Essentially, the key difference between .call() and .apply() is the way in which the arguments are passed. If you have the function arguments as individual values, you can use .call() and pass them explicitly. If you have the function arguments in an array or array-like object, you can use .apply() and pass the array-like object as a single argument. Both methods allow you to control this value within the function execution context, which can be helpful when working with object-oriented programming or borrowing methods from one object to use with another.
Q44. What is the method of reading and writing in JavaScript
Files can be read and written by using java script functions – fopen(),fread() and fwrite(). The function fopen() takes two parameters – 1. Path and 2. Mode (0 for reading and 3 for writing). The fopen() function returns -1, if the file is successfully opened.
Example:
The function fread() is used for reading the source file content.
Example:
The function fwrite() is used to write the contents to the source file.
Example:
// opens the source file for writing
// str is the content that is to be written into a separate file.
Q45. How to print a webpage using javascript?
To print a webpage, we use the print() method. The print method opens a dialog box that you can click and print the page.
The following code can be used➖
PCFET0NUWVBFIGh0bWw+IDxodG1sPiA8Ym9keT4gPGJ1dHRvbiBvbmNsaWNrPSJkaXNwbGF5KCkiPkNsaWNrIHRvIFByaW50PC9idXR0b24+IDxzY3JpcHQ+IGZ1bmN0aW9uIGRpc3BsYXkoKSB7IHdpbmRvdy5wcmludCgpOyB9IDwvc2NyaXB0PiA8L2JvZHk+IDwvaHRtbD4=
Q46. What is the date object in JavaScript?
The Date object is an inbuilt datatype of JavaScript language. It is used to work with dates and times. The Date object is created by using new keyword, i.e. new Date(). The Date object can be used date and time in terms of millisecond precision within 100 million days before or after 1/1/1970.
There are four different ways to declare a date, the basic thing is that the date-objects are created by the new Date() operator
new Date() ➖The date constructor creates a Date object which sets the current date and time depending on the browser’s time zone. It does not accept any value.
new Date(milliseconds)➖ This method accepts single parameter milliseconds which indicates any numeric value. This argument is taken as the internal numeric representation of the date in milliseconds.
new Date(dataString) ➖This method accepts a single parameter dataString which indicates any string value. It is a string representation of a date and the return statement returns the data string with the day.
new Date(year, month, date, hour, minute, second, millisecond)➖
Parameters:
This method accepts seven parameters as mentioned above and described below:
year: Integer value which represents the year. This should always specify the year in full, i.e. use 2018, instead of use 18.
month: Integer value which represents the month. The integer values starting from 0 for January to 11 for December.
date: Integer value which represents the date.
hour: Integer value which represents the hour on the 24-hours scale.
minute: Integer value which represents the minute.
second: Integer value which represents the second.
millisecond: Integer value which represents the millisecond.
Q47. How to handle Exception in Java Script?
In JavaScript, you can handle exceptions, also known as errors, using try-catch blocks. The try-catch block allows you to enclose a section of code that may throw an exception and specify how to handle that exception if it occurs.
Here's the basic syntax of a try-catch block:
try {
// Code that may throw an exception
} catch (error) {
// Code to handle the exception
}
Within the try block, you place the code that you want to monitor for exceptions. If an exception occurs within the try block, the catch block is executed. The catch block receives the thrown exception as an argument, which you can then use to handle the exception appropriately.
Here's an example that demonstrates the usage of try-catch block:
dHJ5IHsKLy8gQ29kZSB0aGF0IG1heSB0aHJvdyBhbiBleGNlcHRpb24KY29uc3QgcmVzdWx0ID0gMTAgLyAwOyAvLyBEaXZpc2lvbiBieSB6ZXJvCmNvbnNvbGUubG9nKHJlc3VsdCk7Cn0gY2F0Y2ggKGVycm9yKSB7Ci8vIENvZGUgdG8gaGFuZGxlIHRoZSBleGNlcHRpb24KY29uc29sZS5sb2coIkFuIGVycm9yIG9jY3VycmVkOiIsIGVycm9yKTsKfQ==
In this example, the code within the try block attempts to perform a division by zero, which will throw an exception. Since dividing by zero is an invalid operation, the catch block is executed. The catch block receives the thrown exception as the parameter, and the error message along with the exception is logged to the console.
By using try-catch blocks, you can gracefully handle exceptions, prevent them from crashing your program, and provide meaningful error messages or alternative paths of execution. Additionally, you can nest multiple try-catch blocks to handle different types of exceptions or perform more granular error handling.
Q48. What is the purpose of the onError event handler in JavaScript?
The event handler in JavaScript is used to handle errors that occur during the execution of a script or the loading of external resources, such as images, scripts, or stylesheets. It allows you to define a function that will be executed when an error occurs.
The event handler is commonly used in scenarios like:
1. Error handling for scripts:
<script>
function handleScriptError() {
console.log("An error occurred while loading the script.");
}
</script>
<script src="script.js" onError="handleScriptError()"></script>
2. Error handling for images:
<img src="image.png" onError="handleImageError()">
The event handler provides a way to catch and handle errors in a controlled manner. By defining an error handling function and assigning it to the event, you can perform actions such as logging the error, displaying a user-friendly message, or taking corrective measures.
It's important to note that the event handler is not limited to script and image elements. It can also be used with other HTML elements like , , , and , as well as various JavaScript APIs that support error events, such as XMLHttpRequest or WebSocket.
Additionally, modern JavaScript provides more robust error-handling mechanisms, such as try-catch blocks and the event handler, which offer greater control and flexibility in handling errors within JavaScript code.
Q49. What would be the result of 3+2+”7″?
In JavaScript, the operator is used for both addition and string concatenation. When the operator is used with numbers, it performs addition. However, when one or both of the operands are strings, the operator concatenates the strings together.
In the expression , the first two operands are numbers ( and ), and the last operand is a string ().
Here's the step-by-step evaluation of the expression:
- evaluates to (addition of numbers).
- evaluates to (concatenation of the number with the string ).
Therefore, the result of is . The numeric addition of results in , and then that result is concatenated with the string , producing the string .
Q50. Is JavaScript case-sensitive?
Yes, JavaScript is case-sensitive. This means that JavaScript treats uppercase and lowercase letters as distinct and separate entities. The case of letters in variable names, function names, object properties, and keywords matters and must be used consistently.
For example, in JavaScript, the variables , , and are considered to be three different variables. Similarly, function names, such as and , are treated as separate functions.
Q51. What is an anonymous function?
An anonymous function, also known as a function expression, is a function in JavaScript that is defined without a name. Unlike a named function, which is declared with a specific identifier, an anonymous function does not have an explicit name associated with it.
Anonymous functions are typically used when you need a function for a specific task and do not require it to be referenced or called elsewhere in your code. They are commonly used as callback functions, function arguments, or immediately invoked function expressions (IIFE).
Anonymous functions are useful in scenarios where you need a short-lived or one-time function without the need for a specific name. They help in writing more concise code and can be used inline without cluttering the global namespace with unnecessary function names.
It's important to note that anonymous functions can also be assigned to variables or used as arrow functions, providing flexibility in various coding scenarios.
Q52. What is runtime error?
A runtime error, also known as an exception, is an error that occurs during the execution of a program or script. It happens when the program encounters an unexpected condition or behavior that prevents it from continuing its normal execution.
Runtime errors are distinct from compile-time errors, which are detected by the compiler before the program is executed. Runtime errors occur while the program is running and often result from logical errors, invalid data, or unforeseen circumstances.
Here are a few common examples of runtime errors:
-
Division by zero: const result = 10 / 0; // Throws a runtime error (division by zero)
-
Undefined variable: console.log(x); // Throws a runtime error (variable x is not defined)
- Null reference: const obj = null;
console.log(obj.property); // Throws a runtime error (accessing property of null) - Type mismatch: const result = "5" + 2; // Throws a runtime error (concatenating string with a number)
When a runtime error occurs, it interrupts the normal flow of the program and may cause it to terminate prematurely. In many programming languages, including JavaScript, runtime errors can be handled and controlled through the use of exception handling mechanisms, such as blocks.
By using blocks, you can catch and handle runtime errors gracefully, preventing them from causing the program to crash. This allows you to provide alternative paths of execution or display error messages to the user.
Q53. What is arrow function?
An arrow function, also known as a fat arrow function, is a concise syntax introduced in ECMAScript 2015 (ES6) for creating functions in JavaScript. Arrow functions provide a more compact and streamlined way to write function expressions.
The syntax for an arrow function includes a parameter list (if any), followed by the arrow () and then the function body. The function body can be either an expression that is implicitly returned or a block of statements enclosed in curly braces (). The arrow functions have a lexical binding, meaning that they capture the value of from the surrounding context at the time of their creation.
Arrow functions are especially useful when you want to write concise and inline functions or when you need to preserve the lexical context. However, it's important to note that arrow functions have some differences compared to traditional function expressions. For example, arrow functions do not have their own , , or bindings, and they cannot be used as constructors with the keyword. These factors should be considered when deciding whether to use arrow functions in specific scenarios.
Q54. What is a callback function in JavaScript?
In JavaScript, a callback function is a function that is passed as an argument to another function and is executed at a later point in time. The purpose of a callback function is to provide a way to handle asynchronous or event-driven operations, allowing code to be executed when a particular task or event has completed.
Callbacks are commonly used in scenarios such as making asynchronous requests, handling user interactions, and implementing event-driven programming. They allow you to specify what should happen after a particular task or event finishes executing.
Here's an example of a callback function used with :
ZnVuY3Rpb24gZ3JlZXQobmFtZSwgY2FsbGJhY2spIHsKY29uc29sZS5sb2coIkhlbGxvLCAiICsgbmFtZSArICIhIik7CmNhbGxiYWNrKCk7Cn0KCmZ1bmN0aW9uIHNheUdvb2RieWUoKSB7CmNvbnNvbGUubG9nKCJHb29kYnllISIpOwp9CgpncmVldCgiQWxpY2UiLCBzYXlHb29kYnllKTs=
In this example, the function takes two arguments: and . It logs a greeting message to the console and then executes the function. The function is defined separately and passed as a callback to . When is called, it first prints "Hello, Alice!" and then executes the function, which prints "Goodbye!".
Callbacks provide a way to control the flow of execution in asynchronous or event-driven environments, as they allow you to specify the code to be executed once a certain task or event is completed. This enables handling of results, error handling, and continuation of execution after the asynchronous operation finishes.
Q55. What is a blur function?
In JavaScript, the function is an event handler that is triggered when an element loses focus. When an element, such as an input field or a button, is in focus and the user interacts with another element, such as clicking outside the current element, the event is fired.
The event is commonly used to perform actions or validation when an element loses focus. For example, you might want to validate the input in a form field when the user moves to the next field or clicks outside the field.
The event can be useful for enhancing user experience and providing feedback or performing specific actions when elements lose focus. It is commonly used in form validation scenarios, where you want to validate user input as they move away from an input field.
Q56. What do you understand by cookies in JavaScript?
In JavaScript, cookies are small pieces of data that can be stored on a user's computer by a website. They are used to store information about the user or their interactions with the website. Cookies are primarily used to maintain stateful information across multiple requests or sessions.
When a website sends a response to the user's browser, it can include a "Set-Cookie" header, which contains the data to be stored in a cookie. The browser then stores this data locally. On subsequent requests to the same website, the browser automatically includes the stored cookie data in the "Cookie" header of the request, allowing the website to access and use that information.
Cookies have various properties that can be set, such as the expiration date, path, and domain. These properties determine when and where the cookie is sent back to the server. For example, a cookie can be set to expire after a certain period of time or be restricted to a specific subdomain.
In JavaScript, you can interact with cookies using the property. It provides access to the cookies associated with the current document. The property allows you to read, write, and delete cookies.
Here's an example of how to set a cookie using JavaScript:
ZG9jdW1lbnQuY29va2llID0gInVzZXJuYW1lPUpvaG4gRG9lOyBleHBpcmVzPVRodSwgMTkgSnVuIDIwMjUgMTI6MDA6MDAgVVRDOyBwYXRoPS8iOw==
It's important to note that cookies have certain limitations, such as their limited storage size and potential security risks (e.g., cross-site scripting vulnerabilities). As a result, alternative methods like browser storage APIs (localStorage, sessionStorage) and server-side session management are often used in modern web development.
Q57. What is Asynchronous testing in JavaScript?
It's common in JavaScript for code to run asynchronously. When you have code that runs asynchronously:
PHNjcmlwdD4KCmRvY3VtZW50LndyaXRlKCJIaSIpOwoKZG9jdW1lbnQud3JpdGUoIjxicj4iKTsKCnNldFRpbWVvdXQoKCkgPT4gewoKZG9jdW1lbnQud3JpdGUoIkxldCB1cyBzZWUgd2hhdCBoYXBwZW5zIik7Cgp9LCAyMDAwKTsKCmRvY3VtZW50LndyaXRlKCI8YnI+Iik7Cgpkb2N1bWVudC53cml0ZSgiRW5kIik7Cgpkb2N1bWVudC53cml0ZSgiPGJyPiIpOwoKPC9zY3JpcHQ+
So, what the code does is first it logs in then rather than executing the function it logs in and then it runs the function.
At first, as usual, the the statement got logged in. As we use browsers to run JavaScript, there are web APIs that handle these things for users. So, what JavaScript does is, it passes the function in such web API and then we keep on running our code as usual. So it does not block the rest of the code from executing and after all the code is executed, it gets pushed to the call stack and then finally gets executed. This is what happens in asynchronous JavaScript
Q58. What is an Async Function?
An async function is a type of function in JavaScript that enables asynchronous behavior and allows you to write asynchronous code in a more synchronous-like manner. It was introduced in ECMAScript 2017 (ES8) and built upon Promises, providing a simpler and cleaner syntax for handling asynchronous operations.
To define an async function, you use the keyword before the function declaration. An async function always returns a Promise, which represents the eventual completion or failure of an asynchronous operation. Within an async function, you can use the keyword to pause the execution of the function until a Promise is resolved or rejected.
Using async/await simplifies the handling of asynchronous operations by eliminating the need for nested callbacks or chaining multiple methods. It allows you to write asynchronous code in a more linear and readable way, resembling synchronous code flow. Additionally, async functions provide a convenient way to handle errors by utilizing the block.
It's important to note that async functions are non-blocking, meaning that while awaiting a Promise, the execution of the function is paused, allowing other code to run in the meantime. This makes them particularly useful when dealing with time-consuming tasks, such as network requests or source file operations, without blocking the execution of other parts of your code.
Q59. What is event bubbling in JavaScript?
Event bubbling, also known as event propagation, is a mechanism in JavaScript where an event triggered on a nested element is propagated or "bubbled up" through its parent elements in the DOM hierarchy. This means that when an event occurs on an element, such as a click event, the event is first handled by the innermost element and then sequentially propagated to its parent elements, all the way up to the root of the document.
Here's an example to illustrate event bubbling:
PGRpdj4KPGRpdj4KPGJ1dHRvbj5DbGljayBtZTwvYnV0dG9uPgo8L2Rpdj4KPC9kaXY+Cgpkb2N1bWVudC5nZXRFbGVtZW50QnlJZCgnYnV0dG9uJykuYWRkRXZlbnRMaXN0ZW5lcignY2xpY2snLCBmdW5jdGlvbihldmVudCkgewpjb25zb2xlLmxvZygnQnV0dG9uIGNsaWNrZWQnKTsKY29uc29sZS5sb2coJ1RhcmdldDonLCBldmVudC50YXJnZXQpOwpjb25zb2xlLmxvZygnQ3VycmVudCBUYXJnZXQ6JywgZXZlbnQuY3VycmVudFRhcmdldCk7Cn0pOw==
In this example, when the button is clicked, the event first triggers the event handler attached to the button. However, after the button's event handler finishes execution, the event continues to propagate up the DOM tree. In this case, the event handler is not defined for the parent elements explicitly. But if there were event handlers defined for the parent elements (such as the divs with IDs "inner" and "outer"), the event would continue to propagate and trigger those event handlers as well.
Event bubbling allows you to handle events at different levels of the DOM hierarchy. It simplifies event handling by allowing you to attach a single event listener to a parent element/ parent object and handle events for its child elements as well. This can be beneficial for managing event delegation and reducing the number of event listeners attached to individual elements. However, it's important to be aware of event bubbling and properly handle event propagation if necessary to prevent unintended behavior or conflicts.
Q60. What is Asynchronous code in JavaScript?
Asynchronous JavaScript: Asynchronous code allows the program to be executed immediately whereas the synchronous code will block further execution of the remaining code until it finishes the current one. This may not look like a big problem but when you see it in a bigger picture you realize that it may lead to delaying the User Interface.
Let us see the example of how Asynchronous JavaScript runs:
PHNjcmlwdD4KCsKgwqDCoMKgZG9jdW1lbnQud3JpdGUoIkhpIik7CgrCoMKgwqDCoGRvY3VtZW50LndyaXRlKCI8YnI+Iik7CgrCoMKgCgrCoMKgwqDCoHNldFRpbWVvdXQoKCkgPT4gewoKwqDCoMKgwqDCoMKgwqDCoGRvY3VtZW50LndyaXRlKCJMZXQgdXMgc2VlIHdoYXQgaGFwcGVucyIpOwoKwqDCoMKgwqB9LCAyMDAwKTsKCsKgwqAKCsKgwqDCoMKgZG9jdW1lbnQud3JpdGUoIjxicj4iKTsKCsKgwqDCoMKgZG9jdW1lbnQud3JpdGUoIkVuZCIpOwoKwqDCoMKgwqBkb2N1bWVudC53cml0ZSgiPGJyPiIpOwoKPC9zY3JpcHQ+
Output:
So, what the code does is first it logs in Hi then rather than executing the setTimeout function it logs in End, and then it runs the setTimeout function.
At first, as usual, the Hi statement got logged in. As we use browsers to run JavaScript, there are web APIs that handle these things for users. So, what JavaScript does is, it passes the setTimeout function in such web API and then we keep on running our code as usual. So it does not block the rest of the code from executing and after all the code is executed, it gets pushed to the call stack and then finally gets executed. This is what happens in asynchronous JavaScript.
Q61. What is rest Syntax or Rest Operator, and how is it opposite to spread operator or spread syntax?
Rest syntax or rest parameter is a feature introduced in ECMAScript 2015 (ES6) that allows you to represent an indefinite number of arguments as an array within a function declaration. It is denoted by using three dots () followed by a parameter name in the function declaration. The rest parameter gathers all the remaining arguments into an array.
Here's an example that demonstrates the use of rest syntax:
ZnVuY3Rpb24gc3VtKC4uLm51bWJlcnMpIHsKbGV0IHRvdGFsID0gMDsKZm9yIChsZXQgbnVtYmVyIG9mIG51bWJlcnMpIHsKdG90YWwgKz0gbnVtYmVyOwp9CnJldHVybiB0b3RhbDsKfQoKY29uc29sZS5sb2coc3VtKDEsIDIsIDMsIDQsIDUpKTsgLy8gT3V0cHV0OiAxNQ==
In this example, the function uses the rest syntax with the parameter . It allows you to pass any number of arguments, and they are automatically collected into an array called . Within the function body, you can then work with this array as needed.
On the other hand, spread syntax or spread operator (also denoted by three dots ) is used to expand an array or iterable object into individual elements. It can be used in function calls, array literals, or object literals to spread the elements or properties.
Here's an example of the spread syntax:
const numbers = [1, 2, 3, 4, 5];
console.log(...numbers); // Output: 1 2 3 4 5const array1 = [1, 2, 3];
const array2 = [4, 5, 6];
const combinedArray = [...array1, ...array2];
console.log(combinedArray); // Output: [1, 2, 3, 4, 5, 6]
In the first example, the spread syntax is used within the statement to expand the array into individual elements. This results in each element being printed separately.
In the second example, the spread syntax is used to combine the elements of and into a new array called . The resulting array contains all the elements from followed by all the elements from .
To summarize, the rest syntax is used within a function declaration to collect multiple arguments into an array, while the spread syntax is used to spread an array or iterable object into individual pivot elements or combine multiple forms of arrays/objects into a new array/object.
62. What is a nested function?
A nested function, also known as an inner function, is a function defined within another function. In programming languages that support nested functions, the inner function is enclosed within the body of the outer function. This means that the inner function can only be accessed and called from within the scope of the outer function.
Here's an example in JavaScript to illustrate the concept:
ZnVuY3Rpb24gb3V0ZXJGdW5jdGlvbigpIHsKY29uc29sZS5sb2coIlRoaXMgaXMgdGhlIG91dGVyIGZ1bmN0aW9uLiIpOwoKZnVuY3Rpb24gaW5uZXJGdW5jdGlvbigpIHsKY29uc29sZS5sb2coIlRoaXMgaXMgdGhlIGlubmVyIGZ1bmN0aW9uLiIpOwp9CgovLyBDYWxsaW5nIHRoZSBpbm5lciBmdW5jdGlvbiBmcm9tIHdpdGhpbiB0aGUgb3V0ZXIgZnVuY3Rpb24KaW5uZXJGdW5jdGlvbigpOwp9CgovLyBDYWxsaW5nIHRoZSBvdXRlciBmdW5jdGlvbgpvdXRlckZ1bmN0aW9uKCk7
In this JavaScript example, is defined within . The inner function can access variables and parameters of the outer function, and it can also have its own local variables. However, it cannot be directly called from outside the scope of the outer function.
Nested functions in JavaScript offer similar benefits as in other programming languages, allowing for code organization, improved readability, and controlled access to functions.
63. What are escape characters in JavaScript?
Escape characters in JavaScript are special characters that are used to represent certain non-printable or reserved characters within a string. They are denoted by a backslash () followed by a specific character or sequence of characters. When encountered in a string, escape characters modify the interpretation or behavior of the following character.
Here are some commonly used escape characters in JavaScript:
- \n: Newline character. Inserts a line break.
- \t: Tab character. Inserts a horizontal tab.
- \r: Carriage return character. Moves the cursor to the beginning of the line.
- **': Single quote character. Used to escape single quotes within a string.
- **": Double quote character. Used to escape double quotes within a string.
- \: Backslash character. Used to escape a literal backslash.
- \uXXXX: Unicode escape sequence. Represents a Unicode character based on its hexadecimal code (XXXX).
64. Explain higher-order functions.
In JavaScript, higher-order functions are functions that can accept other functions as arguments and/or return functions as their results. Essentially, a higher-order function treats functions as first-class citizens, allowing them to be manipulated and operated upon just like any other value.
There are two main ways in which higher-order functions are used:
- Accepting Functions as Arguments: A higher-order function can take one or more functions as arguments. This allows for flexibility and enables the higher-order function to perform operations based on the provided functions. The function definition arguments can be invoked within the higher-order function or used to modify its behavior.
- Returning Functions: A higher-order function can also generate and return a new function. This enables the creation of specialized or partially applied functions based on the provided arguments or conditions.
65. What is a prototype property?
In JavaScript, the prototype property is a property of constructor functions that allows predefined objects created from those constructors to inherit properties and methods. Every JavaScript object has a prototype property, which references the prototype object associated with its constructor function.
The prototype object is essentially a blueprint or template for creating other predefined objects. It contains properties and methods that are shared among all instances created from the constructor function. When a property or method is accessed on an object, JavaScript first checks if the object itself has that property or method. If not found, it looks for it in the prototype object.
66. What does syntax error mean in JavaScript?
In JavaScript, a syntax error occurs when the JavaScript engine encounters code that does not conform to the syntax rules of the language. It means that the code is not written correctly according to the grammar and structure defined by JavaScript.
When a syntax error condition is encountered, the JavaScript engine is unable to interpret and execute the code because it cannot understand the intended meaning due to the violation of syntax rules. As a result, the JavaScript engine throws a syntax error and typically provides an error message that indicates the specific issue it encountered.
67. Explain generator functions.
Generator functions are a special type of function in JavaScript that can be paused and resumed during execution. They allow for the generation of a sequence of values over expiration time, providing a more flexible and efficient way to iterate through data compared to traditional functions.
To define a generator function, you use the syntax:
function* myGenerator() {
// Generator function body
yield value1;
yield value2;
// ...
}
The keyword denotes that the function is a generator function. Inside the generator function, the keyword is used to pause the execution and produce a value. The generator function can then be iterated over using a loop or by manually calling the method on the generator object.
When a generator function is invoked, it returns a generator object, which serves as an iterator. The generator object has a method that, when called, resumes the execution of the simple function until the next statement is encountered. The method returns an object with two properties: (the yielded value) and (a boolean indicating whether the generator function has finished).
68. What is a self-invoking function?
A self-invoking function, also known as an Immediately Invoked Function Expression (IIFE), is a JavaScript function that is executed immediately after it is defined. It is a way to encapsulate statement code and create a new scope without polluting the global scope.
The syntax( code snippet) for a self-invoking function is as follows:
(function() {
// Normal Function body or body element
})();
69. What are the types of operators in JavaScript?
In JavaScript, operators are symbols or keywords that perform operations on operands (values or variables). They allow you to perform mathematical calculations, compare values, assign values, and more. JavaScript has several types of operators, including:
-
Arithmetic Operators: These operators perform mathematical calculations on numeric operands.
- Addition ()
- Subtraction ()
- Multiplication ()
- Division ()
- Modulus ()
- Exponentiation ()
- Increment ()
- Decrement ()
- Comma operator
-
Assignment Operators: These operators assign values to variables.
- Assignment ()
- Addition assignment ()
- Subtraction assignment ()
- Multiplication assignment ()
- Division assignment ()
- Modulus assignment ()
-
Comparison Operators: These operators compare values and return a Boolean result ( or ).
- Equal to ()
- Not equal to ()
- Strict equal to ()
- Strict not equal to ()
- Greater than ()
- Less than ()
- Greater than or equal to ()
- Less than or equal to ()
-
Logical Operators: These operators perform logical operations on Boolean operands.
- Logical AND ()
- Logical OR ()
- Logical NOT ()
-
Conditional (Ternary) Operator: It is a shorthand way to write conditional statements.
- Conditional ()
-
Unary Operators: These operators operate on a single operand.
- Unary plus ()
- Unary minus ()
- Logical NOT ()
- Increment ()
- Decrement ()
- Typeof ()
- Delete operator ()
- Void ()
-
Bitwise Operators: These operators perform bitwise operations on integer operands.
- Bitwise AND ()
- Bitwise OR ()
- Bitwise XOR ()
- Bitwise NOT ()
- Left shift ()
- Right shift ()
- Unsigned right shift ()
-
String Operators: These operators are used to concatenate strings.
- String concatenation ()
These are the main types of operators in JavaScript. Understanding and utilizing these operators is essential for performing various operations in JavaScript, such as mathematical calculations, logical evaluations, variable assignments, and more.
Summing up...
JavaScript is one of the most versatile languages. One can ace the interview with determination and hard work. JavaScript is one of the highest-paying jobs in the industry.
You need to brush up on your basics and advanced knowledge of javascript, using these interview questions is a great way to land your dream job, in any top technical firm.
All the best!
You might also be interested in reading:
Comments
Add commentLogin to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Saloni Kelodiya 1 hour ago