Python Programming Table of content:
Python pow() Function | Parameters, Exceptions & More (+Examples)
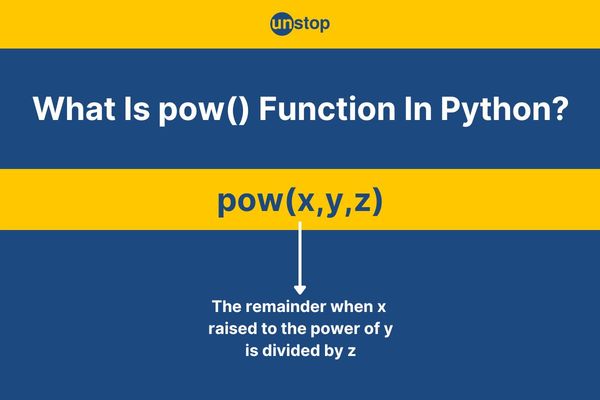
In Python programming language, the pow() function is a built-in utility that serves two primary purposes: it can raise a number to a specified power and compute the modulus of the result. This versatility makes it an essential tool for mathematical computations, whether you're dealing with simple exponentiation or more complex modular arithmetic.
In this article, we'll explore how the pow() function works, its syntax, and its practical applications in various programming scenarios. By the end, you'll have a solid understanding of how to effectively use the pow() function in your Python projects.
What Is Python pow() Function?
The pow() function in Python is a built-in function that is used to calculate the power of a number. It takes a base number and an exponent and returns the result of the base raised to the exponent.
Syntax Of Python pow() Function:
pow(base, exponent, mod)
Here:
- The base parameter represents the number that will be raised to the power.
- The exponent is the power to which the base will be raised.
- The modulus parameter mod is an optional argument. If provided, it performs the modulus operation on the result of the base ** exponent with mod.
Return Value Of Python pow() Function:
The return value of the Python pow() function depends on the number of arguments provided:
- When Two Arguments are Provided (base, exponent): The Python pow() function returns the result of raising the base to the power of exponent. For Example-
result = pow(2, 3) # Output: 8
- When Three Arguments are Provided (base, exponent, mod): In this case, the Python pow() function returns the result of (base ** exponent) % mod. This means it first computes the base raised to the exponent and then takes the modulus with mod. For Example-
result = pow(2, 3, 3) # Output: 2, since (2 ** 3) % 3 = 8 % 3 = 2
Exceptions Of Python pow() Function:
The Python pow() function can raise certain exceptions under specific conditions. Below are the potential exceptions you might encounter:
- TypeError: It is raised if the arguments are not of the correct numeric types. For Example:
result = pow('2', 3) # Raises TypeError: must be real number, not str
- ValueError: It is raised if the mod argument is 0 since division or modulus by zero is undefined. For Example:
result = pow(2, 3, 0) # Raises ValueError: pow() 3rd argument cannot be 0
- OverflowError: It is raised if the result of the base raised to the exponent is too large (greater than 1e308) to be represented within the available memory. For Example:
result = pow(10, 10000) # Might raise OverflowError depending on the system's limitations
By keeping these exceptions in mind, you can ensure safer and more reliable use of the built-in pow Python function in your programs. Let's now explore various code examples to understand how the pow() function works in Python programming.
Python pow() Function Example
The pow() function in Python is straightforward and easy to use. We can simply provide the base and exponent as parameters.
Code Example:
Output:
125
Explanation:
In this example, we use the pow() function with integer values to calculate 5 to the power of 3, which results in 125.
Python pow() Function With Modulus (Three Parameters)
The Python pow() function can also perform modular exponentiation by providing a third parameter for the modulus.
Code Example:
Output:
3
Explanation:
In this example, we use the pow() function with three parameters to calculate 7 to the power of 3 and then take the result modulo 5, giving output as 3 since 343 mod 5 equals 3.
Python pow() Function With Complex Numbers
The Python pow() function is also used with complex numbers. It enables you to perform exponentiation on complex values seamlessly. When you raise a complex number to a power, Python computes the result using the properties of complex arithmetic.
Code Example:
Output:
(-5+12j)
Explanation:
In this example, we use the pow() function to calculate the power of complex number i.e. (2 + 3j)^2. Combining the real and imaginary parts gives the final result as
.Python pow() Function With Floating-Point Arguments And Modulus
The pow() function does not support floating-point numbers when using the modulus argument. It raises a TypeError if you try to pass floating-point values along with a modulus.
Code Example:
Output:
TypeError: pow() 3rd argument not allowed unless all arguments are integers
Explanation:
In this case, using a floating-point base like 3.5 with a modulus results in a TypeError. The pow() function requires all arguments to be integers when using the modulus parameter.
Python pow() Function Implementation Cases
The pow() function in Python calculates the power of a number x raised to the exponent y. The table below summarizes the different cases that can arise based on the signs of x and y:
- Positive x, Positive y: The result is always positive, as a positive number raised to any positive power is positive.
- Negative x, Positive y: The result is negative if y is odd and positive if y is even. This is because a negative number raised to an odd power is negative, while a negative number raised to an even power is positive.
- Positive x, Negative y: The result is the reciprocal of x raised to the positive power of y. This is because a number raised to a negative power is the same as 1 divided by the number raised to the positive power.
- Negative x, Negative y: The result is the reciprocal of x raised to the positive power of y. The sign of the result depends on whether y is odd or even, as explained in case 2.
Code Example:
Output:
Positive x and positive y: 32
Negative x and positive y: 16
Positive x and negative y: 0.1111111111111111
Negative x and negative y: 0.1111111111111111
Explanation:
In the above code example, we use the Python pow() function to handle different combinations of positive and negative bases and exponents:
- For positive numbers raised to positive powers, like 2^5, the result is 32.
- A negative base raised to an even positive power, like -2^4, results in a positive value 16.
- When a positive number is raised to a negative power, like 3^-2, it returns a reciprocal.
- Similarly, raising a negative base to an even negative exponent, like -3^-2, also gives a positive reciprocal.
Difference Between Inbuilt-pow() And math.pow() Function
The pow() function is a built-in Python function that calculates power and supports two or three arguments for basic and modular exponentiation. In contrast, math.pow(), from the math module, uses only two arguments and always returns a float value. Given below are the key differences between these functions:
Feature | The pow() Function | The math.pow() Function |
---|---|---|
Definition | Built-in function for exponentiation and modular exponentiation. | Function from the math module for exponentiation. |
Arguments | Accepts 2 or 3 arguments (base, exponent, and optionally mod). | Accepts only 2 arguments (base and exponent). |
Return Type | Returns an integer or float (depends on input types). | It always returns a float. |
Modular Exponentiation | Supports modular exponentiation with three arguments. | Does not support modular exponentiation. |
Type Handling | It can handle integers and floats for all arguments. | It supports integers and floats, but returns float. |
Performance | Optimized for large integers, especially in modular arithmetic. | Generally optimized for float calculations. |
Usage | Used for general exponentiation needs, especially in programming contexts. | Typically used in mathematical computations where floating-point results are required. |
Conclusion
The pow() function is a powerful and versatile tool for handling exponentiation. It not only simplifies the process of raising numbers to power but also supports modular exponentiation, which is critical in areas like cryptography. Additionally, its ability to handle negative exponents and complex numbers makes it an essential function for various mathematical operations. Whether you’re working with large numbers or need to optimize calculations, the pow() function provides an efficient and flexible solution for Python developers.
Frequently Asked Questions
Q. What is the purpose of the pow() function in Python?
The Python pow() function is an inbuilt function for calculating the power of a number. It takes a base number and an exponent and returns the result of the base raised to the exponent.
Syntax of pow:
pow(base, exponent, mod)
Q. How does the mod parameter work in the pow() function?
The mod parameter in the pow() function calculates the remainder of the division between base**exponent and mod. This is useful for performing modular exponentiation, which is often used in cryptography and other mathematical applications.
Q. Can the pow() function handle negative exponents?
The pow() function in Python can handle negative exponents when two arguments are provided, returning the reciprocal of the base raised to the non-negative exponent (e.g., pow(2, -3) returns 0.125, which is 1 / (2 ** 3)).
However, when a third argument (mod) is included, using a negative exponent raises a ValueError, as modular exponentiation with negative exponents is not supported.
Q. What is the difference between pow() and the ** operator in Python?
Here's a small table that explains the difference between pow() and the ** operator in Python:
Feature | Power Function pow() | ** Operator |
---|---|---|
Basic Exponentiation | Supports exponentiation: pow(base, exp) | Performs exponentiation: base ** exp |
Modular Exponentiation | It supports modulus operations | It doesn't support modulus operation |
Negative Exponents | Handles negative exponents | Handles negative exponents |
Arguments | It can take 2 or 3 arguments | It only takes two arguments |
Return Type | Returns float for negative exponent | Returns float for negative exponent |
Use Case | Preferred for modular exponentiation | Used for simple exponentiation |
Q. Can Python pow() function handle very large numbers?
Yes, pow() in Python can handle very large numbers, thanks to Python’s support for arbitrary-precision integers. When using pow() without a modulus, Python can compute extremely large numbers without overflow issues. For example:
pow(2, 1000) # Output: A very large number
However, for computations involving very large numbers where performance is a concern, using the third argument (mod) can make the calculation more efficient by performing modular exponentiation:
pow(2, 1000, 1009) # Output: 136
This is particularly useful in cryptography and number theory, where working with large numbers and modulo operations is common.
Test Your Knowledge Of Python’s pow() Function!
QUIZZ SNIPPET IS HERE
QUIZZ SNIPPET IS HERE
QUIZZ SNIPPET IS HERE
You might also be interested in reading the following:
- Hello, World! Program In Python | 7 Easy Methods (With Examples)
- Calculator Program In Python - Explained With Code Examples
- Swap Two Variables In Python- Different Ways | Codes + Explanation
- Python Logical Operators, Short-Circuiting & More (With Examples)
- Random Number Generator Python Program (16 Ways + Code Examples)