Bookmark These Important React Interview Questions With Answers (2022)
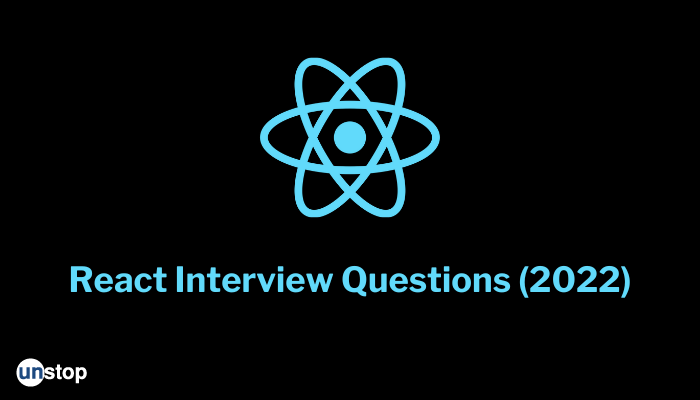
The React library is written in JavaScript and its primary purpose is to facilitate the creation of declarative user interfaces (UIs) by utilizing a component-based approach. In 2011, a software programmer named Jordane Walke who worked for Facebook made it public with the name React.js. Leading tech companies use React as a tool for different projects and that is why candidates need to have a detailed knowledge of React to secure a job in one of these leading tech companies.
Interviews for React technical jobs are detailed but if you have already practiced questions that are most likely to be asked, you will be in a better position to secure the job. To help with the same, we have compiled an extensive list of frequently asked React.js interview questions that are commonly asked during job interviews for the position of React.js developer. We have also provided supporting answers to help you prepare for and respond to these questions well.
Technical Questions for React Interview
These are some of the frequently asked technical questions in React interviews that you must be aware of:
1. Is the class functional component stateless?
Depending on the phase of the class component, the class component is instantiated and a separate lifecycle method is then maintained alive and triggered alongside. They are also referred to as stateless components because they take in data and present it in some manner. These components are primarily responsible for rendering user interfaces.
2. What is an uncontrolled component?
Uncontrolled components are ones in which the document object model (DOM) itself manages the form data for the component in question. The term 'uncontrolled' refers to the absence of any control exerted over these components by that of the current React component state. The values of the form components are usually controlled by and saved on DOM.
3. What is a native event in React.js?
For React Native, events are delivered through the bridge that connects native code with React. In a nutshell, anytime a View is generated. Events in React additionally transmit its ID number to the native. This enables natives to be eligible to receive all events that are connected to the element that was just produced.
4. What are synthetic events in React.js?
React has developed a wrapper that is the same as the native browser so that it can function as a cross-browser application. This was done to avoid the need to create multiple implementations for multiple methods across multiple browsers. This resulted in the creation of common names for all events that occurred across all browsers. Therefore, if we are attempting to trigger an event in a React.js zx component, we are not actively attempting to trigger the real DOM event. Rather, we are making use of the React.js custom event type known as the synthetic event. 'onClick()', 'onBlur()', and 'onChange()' are a few examples of what is known as synthetic events ().
5. What is a higher-order component?
Higher-order components, abbreviated as HOCs, are an advanced method in the React framework for reusing component logic. The 'React API' does not strictly speak. It includes support for HOCs. These are a pattern that results from the compositional structure of React. In its most basic form, a higher-order component can be thought of as a function that accepts an existing component as an argument and produces another component as its output.
6. What are third-party DOM libraries in React?
The native app functionalities, which are not provided by React Native features, are made available by a third-party library. If we consult the documentation for React Native, we will see that there are many functionalities that are unavailable (such as maps). Because of this, we have to incorporate libraries belonging to third parties into our project.
7. Is React.js suitable for an entire application?
Both 'Angular' and 'React' are capable of being utilized to effectively construct massive applications that are suitable for usage in an enterprise setting. It is usually a good idea to go with the alternative that your dedicated development team suggests or whichever option it determines to be the best suitable for the requirements of your project.
8. How does a React application work?
An application built with React consists of numerous components, each of which is in charge of rendering a discrete and reusable section of HTML. It is possible to nest components within one another, which enables sophisticated applications to be constructed out of relatively straightforward building pieces.
9. What is a predictable state container?
Redux is just a state container for JavaScript apps that can be reliably predicted. This ensures that each of your application's states is stored in a single location and you can determine the status of your application anywhere at any point in time.
10. What are props in React?
When passing data from one component to another (such as from a parent component to a child component or components), we make use of React's props. Properties can also be referred to by their abbreviated form, 'props.' They are helpful if you desire the flow of data in the React app to just be dynamic, which is one use case for which they are useful.
11. What is the use of context?
While working with nested components, the sole method that has been available to pass down settings and data to child components has already been through the use of props. This method has been around for a long time. As a result, to pass in data, the parent component must set a prop just on the child component, just as the child component would set a prop on its child. This can be an issue in particular whenever a piece of configuration is needed simultaneously across numerous components within an application tree or structure.
One of the more recent approaches to solving this issue is to make use of context, in which you provide a context variable with the value that you want to apply to descendant components. This is one of the more recent ways to handle this issue. After that, a context provider will surround the child component hierarchy. And post that, every component in that tree will be able to become a consumer and retrieve the data from the context.
12. What is inline templating in React.js?
You can add CSS inline styles to elements in a React application by writing them as attributes and passing them to the elements. You can blend CSS syntax with JSX code when you use the inline styles feature. It is important to keep in mind that the use of the style attribute as the primary technique for styling elements is generally not advised.
13. What do you call the React API onClick?
To retrieve data in React when a button is clicked, the 'onClick' property of a button element should be set. Perform an HTTP request whenever the button is clicked. It is necessary to use the render function to manage the data and then update the state variables.
14. What is a single source of truth in React?
Within a React application, there must be a solitary 'source of truth' for all of the data that undergoes modifications. In most cases, the state is initially added to the user component that requires it to complete the rendering process. Then, if additional components also require it, you can bring it up to their most recent shared ancestor if you like to do so.
15. What is the function of a component?
In most cases, a component will provide a particular purpose or a series of interrelated functions. When designing computer programs, a system is typically broken down into its constituent parts, which are then organized into modules. Testing a component involves verifying that all of its modules, which come together to constitute the component as a whole, are compatible with one another.
16. What is a component in React?
Code that may be used multiple times and is self-contained makes up components. They operate independently and return HTML but fulfill the same role that JavaScript functions would otherwise. There are two different kinds of components: class and functional components.
17. What are interactive components?
You can develop prototype interactions between different variants in a component set through the use of interactive components. When you add an object to your designs, the corresponding interactions are automatically configured and made available for use. When making prototypes, you won't waste as much time, thanks to this. And, you'll also avoid noodle soup.
18. How are two child elements related to each other?
It is said that an element's descendants consist of all of the elements that the element in question contains. For an element to be deemed a child, it must be positioned in the hierarchy precisely beneath the element that constitutes its parent.
19. What are event handlers in React?
When an event is 'fired,' it is the responsibility of event handlers to decide what course of action should be taken. This may involve clicking a button or making a modification to the text that is input.
Event handlers are the primary components responsible for enabling users to engage in conversation with your React application. With a few minor variations, the process of handling events with React elements is very similar to the process of handling events with DOM elements.
20. What are event listeners in React?
An event listener is just a callback function that either waits for the formation of an event object or is called when that event occurs. The term given to a function that performs exactly this — that is, accepts other functions as such an argument — is known as a callback function. Once an element in DOM has been formed, you typically do not need to call the 'addEventListener' method when working with React to add listeners to the element. Instead, you should just supply a listener whenever the element is being rendered for the first time. When working with 'JSX callbacks', you need to be very cautious about the implications of this.
21. How do you make an event listener in React?
For further information on how to utilize the addEventListener methods in function components in React: Adjust the ref prop so that it points to the element. To gain access to the element, make use of the existing property that the ref has. In the use effect hook, you should add the event listener.
22. What is meant by unidirectional data flow?
A one-way data flow is referred to as a unidirectional data flow. In this type of data flow, the data could move in just one direction while being exchanged between the various components of the program. The data flow of React, which is a 'Javascript library', only goes in one direction. The information inherited from the parent is referred to as props.
23. What are development servers?
Development server is one that was created specifically to assist software developers in the creation and testing of various types of programs, websites, software, and apps. It offers a run-time environment as well as both software and hardware facilities that are necessary for the development and debugging of programs.
24. What are the properties in React?
The storage locations for a component's data can be either its attributes or state. The values are passed into the component through its properties. They are utilized during the process of rendering the component as well as initiating the state.
25. What are the advantages of React?
It is a JavaScript framework that is open-source and scalable. And, it enables you to develop user interfaces (UIs) that are straightforward. This library is particularly useful for single-page apps. Whether you're building mobile or online mobile applications, React can manage the view layer for you in an effective and fast manner to help you build better mobile applications. In addition to that, it facilitates the separation of UI into components that can be reused.
The benefits are:
- Boosts enterprise productivity
- Faster rendering
- Code stability
- Cost-effective
- Flexibility
- Performance
- Usability
- Rich user interfaces
26. What do you mean by virtual DOM?
A virtual document object model (virtual DOM) is an object created in JavaScript that is very lightweight and which was initially just a duplicate of the real DOM. This is a node tree that lists the elements, together with their characteristics and content as objects, as well as the properties of those objects. The render function of React constructs a node tree out of the individual React components. It then updates this tree in reaction to the mutations within the data model that have occurred as a result of various activities taken either by the user or by the system. These mutations are triggered by various actions taken by either the user or the system.
27. What are refs in the context of React?
The abbreviation for References in the programming language React is refs. It is an attribute that helps to keep a reference to a specific React element or component. This reference can then be returned by the render configuration function of the component. Returning references to a specific element or component that was returned by render is the purpose of this function (). They come in handy whenever we require DOM measurements or we want to add methods to components.
28. What are the keys used in React and what is their significance?
The use of keys allows for the identification of individual virtual DOM elements, together with the data that is associated with them, which drives the user interface. They assist React in maximizing the efficiency of rendering by recycling all of the items that are already present in DOM. When you use these keys, which must be a one-of-a-kind number or string, React will not re-render the elements; instead, it will just rearrange their current order. This ultimately results in an improvement in the performance of the application.
29. What is Redux?
In the current market, among the libraries for front-end development that are seeing the greatest amount of popularity is Redux. It is a container for JavaScript applications that can maintain a predictable state and is employed for the administration of the application's state in its entirety. Applications built with Redux are simple to test and can function normally in a variety of situations while retaining their original characteristics.
30. What are reducers?
Reducers are purely functional pieces of code that, in response to an “ACTION”, define how the state of the application will be altered. The prior state and action are both taken into consideration by reducers before the function is carried out, after which the reducer returns a new state. It first evaluates what kind of update needs to be performed by looking at the type of action, and then it returns the updated values after making its determination. If no additional work is required to be completed, it reverts to the state that it was in before.
31. What is a React router?
The application can benefit from the addition of new screens and flows; thanks to the use of the strong routing library known as React router, which is developed on top of the React framework. This ensures that the URL is always up to date with the data that is currently being presented on the web page. Constructing single-page web applications, it adheres to a consistent structure and behaves in a predetermined manner. The React router offers an easy-to-use application programming interface.
32. Is there any way to use AJAX in conjunction with React?
Yes, it is possible to use any AJAX library with React in a straightforward manner. Some examples of such libraries include Axios and jQuery AJAX. Maintaining the states of the components is an essential part of the process and in this regard as well, the props are handed down from the parent components to the child components.
It is still not possible for child components to transmit back props to their parents. Taking into account the effects of this limitation, there is a significant positive impact on rendering efficiency.
33. What exactly are 'controlled components' in the React environment?
When discussing React, the term 'controlled components' refers to the components which can keep their state. The data is entirely under the control of the parent component and its most recent value may be retrieved by making use of the component's props. When callbacks are being used, this is done to alert you about any changes that may have occurred.
34. In the system of React, what exactly is considered pure components?
Pure components are independent entities that are developed using the React programming language. They can replace a component that just has the render() function and may be written quickly and easily. Additionally, they have the capability of doing so. This is done to keep the program's code as straightforward and uncomplicated as possible while still maintaining a high level of performance in the application.
35. What is meant by the term 'reconciliation'?
When the props or state of a component are modified, React examines the freshly returned element and compares it to the one that was rendered earlier to determine whether or not an actual DOM update is required. If they do not equal one another, React will update the DOM. The procedure that we are referring to here is called reconciliation.
36. What exactly are these things that are called 'React hooks'?
You can use state and other React capabilities without having to write a class if you take advantage of 'React's hooks'. Developers can employ state as well as lifecycle methods within functional components; thanks to these built-in functions. They do not carry out their duties while students are present in the room. By utilizing hooks, you can fully skip the utilization of lifecycle methods, such as 'componentDidMount', 'componentDidUpdate', and component will unmount.
37. What is an arrow function in React?
In JavaScript, the function syntax known as an arrow function is one of the available types. In contrast to the other way, which makes use of a bind call while it is still inside the function 'Object() { [native code] }', arrow functions do not redefine the value of the 'this' variable within their function bodies. 'This' variable has a scope that encompasses the entire program and is not restricted to the calling function in any way. Because of this, it is much simpler to predict their behavior when it is supplied as a callback. And so, it eliminates any errors that could have been introduced by the use of 'this' within callbacks.
38. What is meant by the term 'CSS module style' when referring to React?
The CSS module file differs significantly from the standard CSS file in a significant way. By default, the local scope of every class name and animation contained within a CSS module is set to be the component that is responsible for its import. It is only accessible for the component that imported it. And, other components won't be able to use it at all until you give explicit permission to do so. This essentially means that one could use nearly any acceptable name for the classes without having to worry about the names of other classes already present in the application causing a conflict. It is possible to create a CSS module file by adding the the.module.css extension to the file.
39. What are stateless components?
A component can be considered stateless if the behavior it exhibits is unaffected by the state it is in. When it comes to building stateless components, you have the option of employing either a function or a class. But, unless you need to include a lifecycle hook in your components, you should choose the function components instead.
40. What is the component of suspense?
If the module containing the dynamic import has not been imported by the time the parent component has finished rendering, you are required to display some fallback content along with a loading indicator while you wait for the module to finish loading. This is something that can be accomplished with the help of the suspense component.
41. In Redux, how do changes to the state take place?
Emitting an action, which is an object that describes what took place, is the sole means to bring about a change in the current state. This guarantees that neither the views nor even the network callbacks could ever write directly to the state of the application again. Instead, they make clear statements about their desire to alter the state. There is no need to be wary of any oblique forms of competition because all of the modifications are managed in a centralized location and implemented sequentially in a predetermined manner. Because actions are nothing more than standard objects, it is possible to record, serialize, and save them so that they can be retrieved and replayed at a later time for the purposes of testing or debugging.
42. Explain the differences between Relay and Redux?
Both Redux and Relay make use of a solitary store, which is one of the similarities between the two. The most significant distinction is that Relay only manages the state that was initially generated by the server and all direct access to the state is accomplished through the use of GraphQL queries (to read data) and changes (for changing data). Relay will store the data in its cache for you and will optimize the retrieval of data for you by just retrieving modified data and skipping anything else.
HR Questions for React Interview
We have compiled a list of the most probable HR questions that will help you with your job interview:
43. Why do you want to work for React?
44. Will you be able to handle stressful situations during working hours?
45. How would you be able to resolve any dispute among your team?
46. Do you have any experience in handling a team of 20 people?
47. What do you think about office culture?
48. What do you think about productivity at work?
49. What is the best way to handle a tough deadline?
50. What do you desire after getting this job role?
About React
The JavaScript library known as React.js is open-source and free to use. It is most commonly referred to as React. When constructing user interfaces, it is more effective to put together whole websites by piecing together various portions of code, known as components. Having been constructed initially by Facebook, it is now being maintained by Meta as well as the open-source community. You are free to make either as much or as little use of React as you deem fit, which is one of the many benefits of this library. For instance, you might construct the entirety of your website using React or you could just make use of a single React component on a single page.
JSX, which is a hybrid of JavaScript and XML, is the primary construction tool for React.js. The JSX markup language is used to build the elements and then JavaScript is used to render them on your website. Even though it has a lengthy learning curve for just a junior developer, React is swiftly rapidly becoming one of the most prominent and in-demand JavaScript frameworks. This is even when it comes with a steep learning curve.
You may also like to read:
- Understanding Time Complexity Of Algorithms In Detail
- List Of Important PHP Interview Questions With Answers (2022)
- Best Collection Of CSS Interview Questions With Answers (2022)
- Competitive Coding: Frequently Asked Questions | Tips For Competitive Coding Events
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Blogs you need to hog!
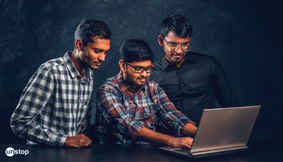
This Is My First Hackathon, How Should I Prepare? (Tips & Hackathon Questions Inside)
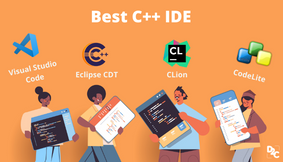
10 Best C++ IDEs That Developers Mention The Most!
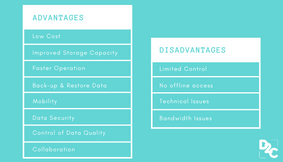
Advantages and Disadvantages of Cloud Computing that you should know!
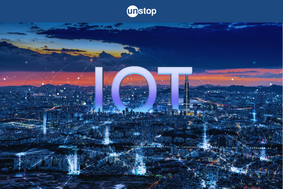
Comments
Add comment