Difference Between OOP And POP & Other Concepts Explained In Detail
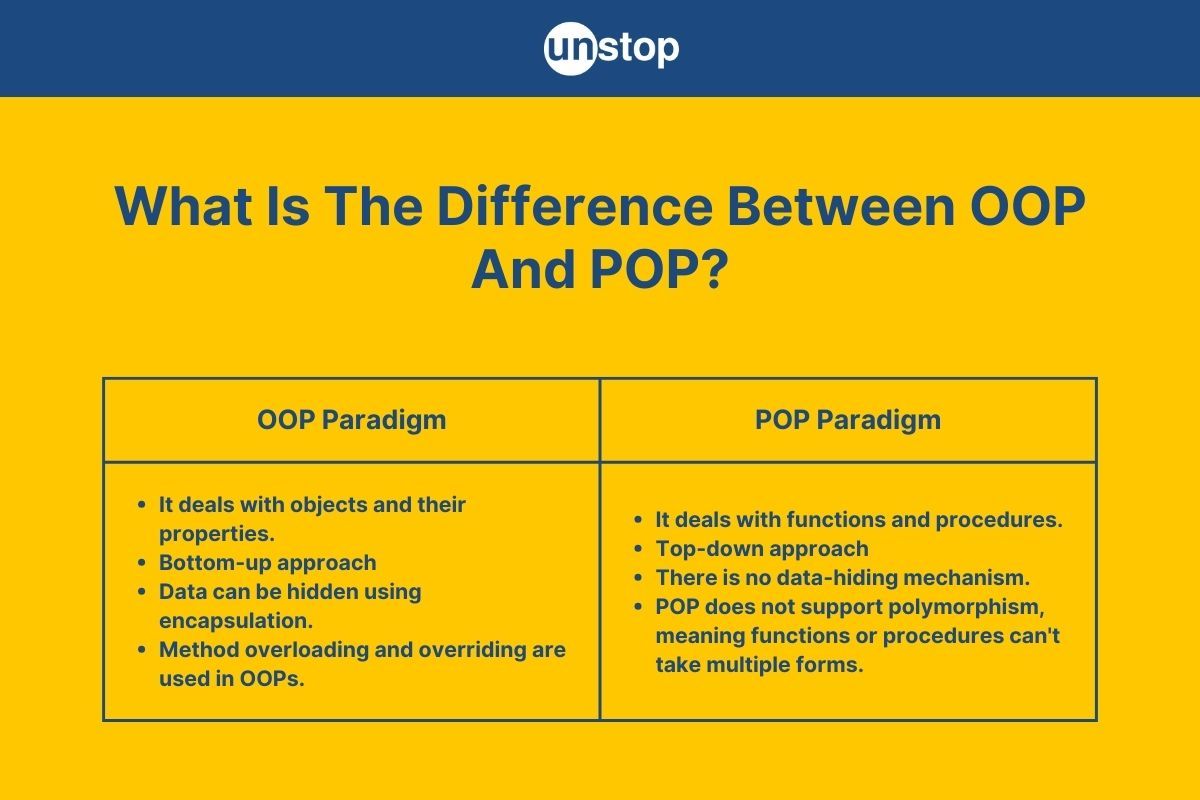
Computer programming languages are designed to operate with high-level structures that have been pre-defined. 'Programming Paradigm' is the technical term used to describe these structures, i.e., how a computer program's structure and elements are organised. Object-oriented programming (OOP) and procedure-oriented programming (POP) are two paradigms for categorising programming architectures commonly used in software development.
In this article, we will discuss the difference between OOP and POP, along with a detailed description of both.
Origin Of Structured Programming
Structured programming first became popular in the 1970s, introducing a set of principles that made it easier to write and maintain code. POP was one of the first methods of programming in structured programming. OOP came later, in the 1980s. Today, it's one of the most popular programming languages.
- Both of these programming techniques, OOP and POP, are used in a wide range of applications. They each take a distinct approach to their task.
- On one hand, the OOP programming style separates an entire program into objects.
- The POP programming style, on the other hand, divides it into functions.
- Thus, the basic difference between OOP and POP is that the former treats data as objects, while the latter treats code as a sequence of steps.
A programmer utilises programming languages when there is no straightforward solution to a problem. This article goes on to detail the nuances of both, object-oriented programming language and procedure-oriented programming language and how the two programming processes aid in software development.
Differences Between OOP and POP (Comparison Table)
Let's take a look at the key differences between object-oriented programming and procedure-oriented programming:
Parameter | Object Oriented Programming(OOP) | Procedure Oriented Programming(POP) |
Definition | OOP refers to object-oriented programming. It deals with objects and their properties. | POP refers to procedural-oriented programming and deals with programs and functions. |
Approach | An object-oriented program uses the bottom-up approach. | A procedure-oriented program uses the top-down approach. |
Access Control | Access control is supported by access modifiers. These include public, private and protected. | No access modifiers are supported. |
Data Hiding | Data can be hidden using encapsulation. | There is no data-hiding mechanism. Data is globally accessible, as there are no access specifiers. |
Entity Linkage | In OOP, objects communicate with each other via message passing, where objects invoke methods on other objects, enabling interaction and collaboration. | In POP, functions are executed sequentially, and communication happens through direct function calls, with parameters passed between functions. |
Polymorphism | Method overloading and method overriding are used in OOP to achieve polymorphism. | POP does not support polymorphism, meaning functions or procedures can't take multiple forms. |
Virtual Function and Inheritance | OOP supports inheritance and virtual functions and virtual classes via it. | There is no concept of inheritance in POP and neither does it support the use of virtual classes or virtual functions. |
Code Reuse | OOP supports code reusability. | No code reusability is provided by POP. |
Operator Overloading | It is allowed in OOP. | Operator overloading is not allowed in POP. |
Object-Oriented Programming
Object-oriented programming (OOP) is a programming paradigm that organises software design around data, rather than functions and logic. It is a high-level programming language that divides the program into chunks called objects. An object is a data field with its own set of properties and behaviour.
- Object-oriented programming (OOP) focuses on the objects that developers desire to handle rather than the logic that is required to manipulate them.
- This kind of programming is ideally suited to big, complicated, and frequently updated or maintained projects.
- This encompasses manufacturing and design software, as well as mobile applications; for example, OOP may be used to simulate manufacturing systems.
Concepts of Object-Oriented Programming
There are 6 major concepts that encompass the OOP approach to programming. The fundamental concepts include:
1. Class
A class is a blueprint or template that defines the properties (data members) and behaviors (member functions or methods) of objects. It does not represent any specific instance of data but serves as a model for creating multiple objects that share the same structure and behavior.
Think of a class as the blueprint of a house. The blueprint itself is not a house, but it can be used to build many houses (objects) that follow the same design.
2. Objects
An object is an instance of a class. While a class is the blueprint, an object is the actual entity created based on that blueprint. It holds real data and interacts with other objects through its methods.
If a class is the blueprint for a house, the object is an actual house built from that blueprint. Each house (object) has its own unique features, such as the color of the walls or the number of rooms, but all houses share the same basic design.
3. Inheritance
Inheritance is a key feature of Object-Oriented Programming that allows one class (called the derived or child class) to inherit properties and methods from another class (called the parent or base class). Class inheritance promotes code reusability, allowing developers to build upon existing classes without rewriting code.
Think of inheritance like genetics. Just as children inherit traits (eye color, hair type) from their parents, a derived class inherits attributes and behaviors from its parent class. However, the child can also have its own unique traits (new methods or attributes).
4. Polymorphism
Polymorphism refers to an object or function's ability to take on multiple forms. In OOP, this concept allows a method to behave differently depending on the object calling it.
-
Method Overloading: This allows multiple methods in the same class to have the same name but different parameters. Think of it as a Swiss Army knife—the same tool (method) can perform different functions based on the input.
-
Method Overriding: When a subclass provides its own implementation of a method inherited from a parent class, that's method overriding. It's like two people having the same name but behaving differently in different situations.
Polymorphism provides flexibility, letting you write more generalized and reusable code.
5. Abstraction
Abstraction is the practice of hiding complex implementation details and exposing only the necessary parts of an object to the user. It focuses on what an object does rather than how it does it.
Example: Consider a car. When driving, you only need to know how to accelerate, brake, and steer. You don't need to understand the internal combustion engine or how the car's electronics work—that's abstraction in action.
In OOP, abstraction is achieved through abstract classes and interfaces. These define what an object should do (method signatures) without detailing how it should do it (method implementation). This not only simplifies coding but also facilitates future modifications.
6. Encapsulation
It is the process of wrapping data (variables) and the methods (functions) that operate on the data into a single unit, typically a class. The core idea of encapsulation is to restrict direct access to some of an object's components and to bundle them together in a controlled environment.
This means that the internal representation of an object is hidden from the outside world, and access to that data is only allowed through defined methods (getters and setters). This promotes the concept of data hiding and security.
Now that we've understood the key OOP concept, let's take a look at the advantages and disadvantages of OOP (Object-Oriented Programming).
Advantages of OOP
- Rather than needing to start writing code from scratch, programs can be built from standard functioning modules that communicate with one another, which saves time and increases productivity. The OOP language allows us to divide the program down into bite-sized problems that can be solved quickly (one object at a time).
- The new technology promises higher programmer efficiency, improved software quality, and lower maintenance costs.
- Small to large OOP systems can be simply upgraded.
- It is possible for numerous instances of objects to coexist without interfering with one other, and it is very simple to divide work into a project based on objects.
- It is feasible to map problem domain objects to program objects.
- The data-hiding principle aids programmers in creating secure programs that are not vulnerable to code in other areas of the program and can't be accessed by external functions.
- Duplicate code can be minimized and increase the use of existing classes by using inheritance.
- For communication between objects, message-passing mechanisms are utilised, which simplifies the interface specifications with external systems.
- OOP simplifies the debugging process because objects can be isolated and examined independently from other parts of the code.
- Data-centred designs can be used to get an implementable model in order to capture more information.
Disadvantages of OOP
- The structure of OOP programs and the complexity may be higher than compared to procedural code, potentially leading to larger programs, especially for smaller tasks. As the program grows in size, it takes longer to execute, resulting in slower program execution.
- Since OOP is not a universal language, it cannot be used everywhere. It is only used when absolutely necessary. It is not appropriate for all types of issues.
- Using OOP is a little challenging; programmers must have excellent design and programming skills, as well as adequate planning.
- Learning OOPs takes time. For some people, the thinking process involved with object-oriented programming is not natural.
- Everything in OOP is considered as an object, we must have strong thinking skills before using it.
Procedure-Oriented Programming (POP)
Procedural Oriented Programming is a programming method that focuses on the functions or procedures needed for computation rather than the data itself.
The procedural approach uses a sequence of instructions to break down the program into functions, and each task is completed in order. The global data or variables are shared by these functions, and data is exchanged between them. Essentially POP uses a step-by-step approach.
Examples of procedural programming languages are C, Pascal, and FORTRAN.
Characteristics Of POP (Procedure-Oriented Programming)
Listed below are the key features of POP:
- It focuses on the algorithm.
- Larger programs are broken down into functions, which are smaller blocks of code. The functions are followed by a sequence of sequential computational steps.
- Global variables can be used to communicate across functions.
- Data can flow freely from one function to the next.
- Data can be changed by functions at any time and from any location.
- It employs a top-down programming approach.
Advantages of POP Language
- The programming simplicity, as well as the ease of implementation of compilers and interpreters, make procedural programming ideal for general-purpose programming.
- A wide range of books and online course materials on tested algorithms are available, making it easy to study as you go.
- Because the source code is portable, it can also be used to target a different CPU.
- It is not necessary to replicate the code because it can be reused in different portions of the program.
- The memory need is also reduced using the Procedural Programming approach.
- The program flow can be simply followed.
Disadvantages of POP Language
- When Procedural Programming is used, the computer code is more difficult to write.
- As procedural code is frequently not reusable, it may be necessary to rebuild it if it is required for usage in another application.
- A correlation with real-world objects is difficult. Since real-world objects have their own data and behaviour, POP makes it difficult to model real-world scenarios
- The procedure is prioritised over the data, which may cause problems in data-sensitive situations.
- Since the data is visible to the entire program, it is not very secure.
Conclusion
The two major programming paradigms mentioned in this article, OOP and POP, are the most widely used languages by software developers. While POP is a common programming style, OOP is a step ahead of it and solves POP's limitations.
Frequently Asked Questions
1. Which is better - OOP or POP?
Though OOP has an edge over POP, both languages have their own strength and weaknesses. While OOP is more flexible and powerful than POP, it's also more complex and difficult to learn. Thus, which is a better programming language depends on the need of the projects.
2. What are some popular examples of OOP and POP?
Some popular examples of OOP are - C++, JAVA, C#
Some popular examples of POP are - C, VB, Pascal
3. How is OOP better than POP?
OOP came after POP, resolving several issues that software developers faced while working with POP.
Since OOP supports access control (via access specifiers) and data hiding, it's more secure than POP. OOP also allows for easier maintenance of code. OOP also supports code reusability, inheritance, polymorphism and virtual functions - all features absent in POP.
4. What are some disadvantages of OOP?
One of the major disadvantages of OOP is that it's complex. Programs in OOP tend to be lengthy and difficult to test and debug. There is also no data portability in OOP.
5. What are some advantages of POP?
The main advantage that POP has as a programming paradigm is that it's simple and portable. They also tend to be more efficient and faster, since there isn't a need to create and garbage objects.
This compiles our discussion on the difference between OOP and POP. Do check out the following
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment